In MATLAB, you can easily import CSV files using the `readtable` function, which creates a table from the CSV data for easy manipulation and analysis.
data = readtable('filename.csv');
Getting Started with MATLAB
Setting Up Your Environment
Before diving into how to matlab import csv files, it’s crucial to ensure you have a functioning MATLAB environment. Installing MATLAB is straightforward; simply follow the prompts on [MathWorks’ website](https://www.mathworks.com/downloads/) based on your operating system.
Once installed, open MATLAB. You’ll be greeted by the Command Window, which is where you will input commands. Familiarize yourself with the interface, including the Workspace and Command History, as these tools will be helpful for tracking your datasets and commands.
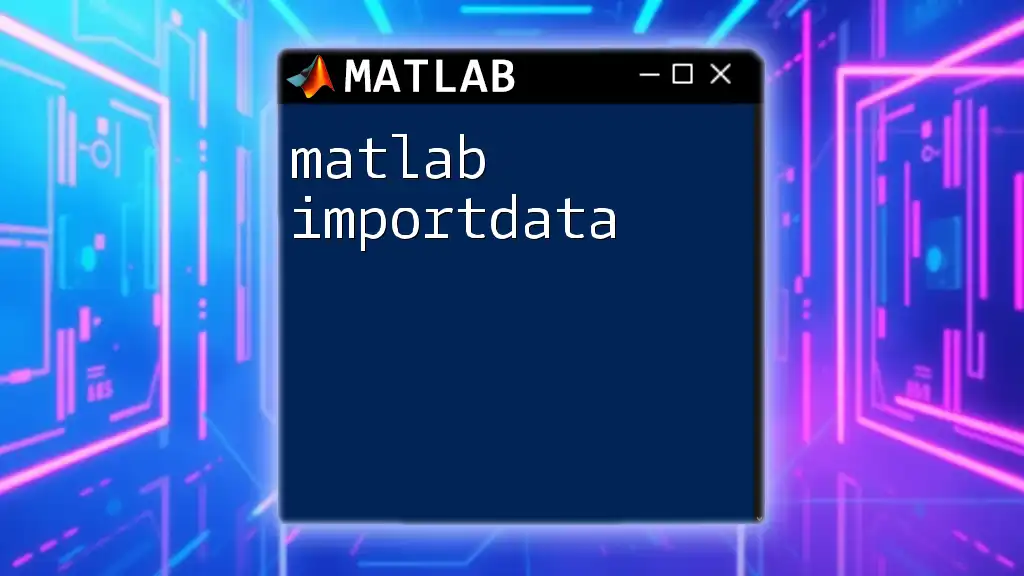
Importing CSV Files: Basic Techniques
Using the `readtable` Function
One of the most versatile functions for importing CSV files in MATLAB is `readtable`. This function reads a comma-separated values (CSV) file and stores the data in a table format, which is advantageous for analysis.
Syntax and Parameters: The basic syntax is:
data = readtable('filename.csv');
Here, `'filename.csv'` is the path to your CSV file.
Example: Basic usage to import a CSV file:
data = readtable('datafile.csv');
In this example, MATLAB will create a table variable `data` containing the contents of `datafile.csv`. The columns of the CSV file become variables in the table, while each row corresponds to an observation.
Using the `csvread` Function
For simpler cases, you might consider using the `csvread` function. While it's less flexible than `readtable`, it is efficient for straightforward data.
Overview and Limitations: `csvread` can only import numeric data, so if your CSV file contains text or mixed data types, it’s better to use `readtable`.
Syntax:
data = csvread('filename.csv', row, column);
You can specify the starting row and column to read from.
Example: Simple CSV import using `csvread`:
data = csvread('datafile.csv', 1, 0); % Skips first row
In this example, the function starts reading from the second row (index 1), effectively ignoring the header row.
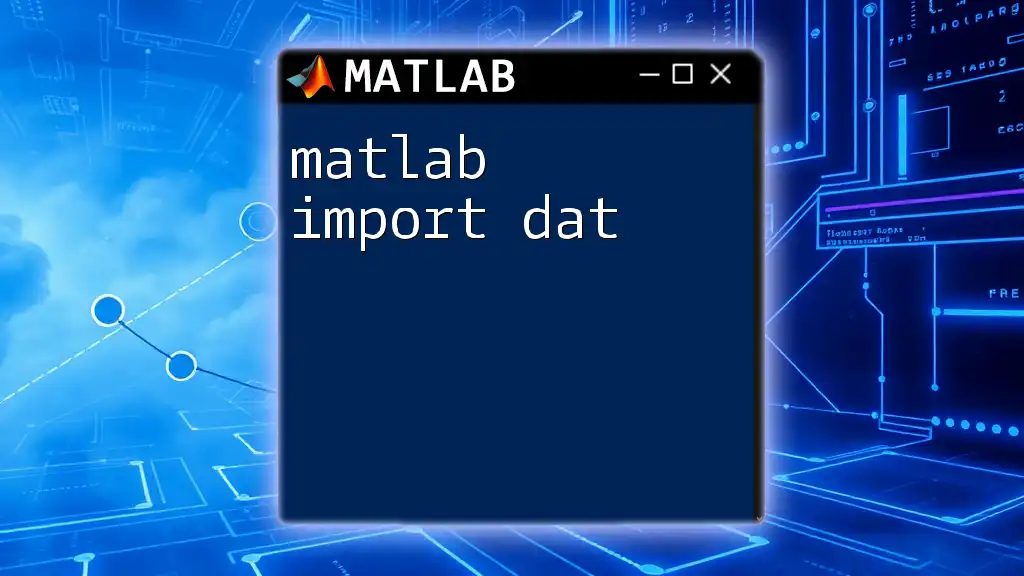
Advanced Techniques for Importing CSV Files
Handling Different Data Formats
MATLAB provides options to tailor the import process according to your data's needs. When using `readtable`, you can specify how to handle different variable types by creating import options.
Example: Importing with custom criteria:
opts = detectImportOptions('datafile.csv');
opts.VariableNames = {'Column1', 'Column2'};
data = readtable('datafile.csv', opts);
The `detectImportOptions` function automatically detects variable types and improves the import process by applying your specified variable names.
Skipping Rows and Selecting Specific Data
Sometimes you may want to skip initial rows, such as headers or comments, and focus on specific data.
Example: Skip specific rows and select columns with `readtable`:
data = readtable('datafile.csv', 'HeaderLines', 1);
selectedData = data(:, {'Column2', 'Column4'});
This command skips the first row of the CSV during import and retrieves only `Column2` and `Column4` from the imported data.
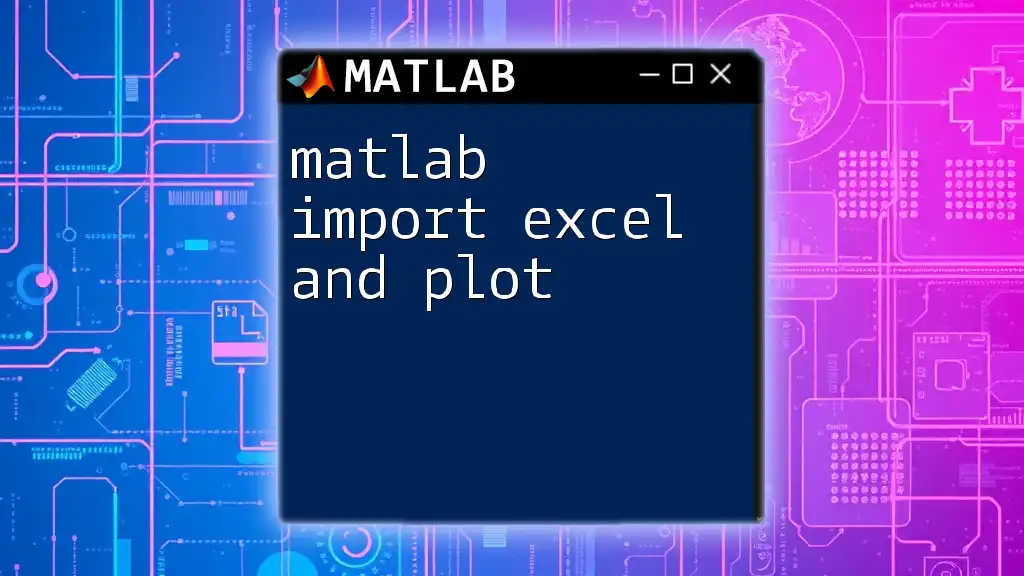
Troubleshooting Common Issues
Error Messages and Their Solutions
When working with `matlab import csv`, users may encounter various error messages. Common issues include:
- File not found: Double-check the file path for accuracy.
- Format issues: Ensure the CSV file is correctly formatted; a few misplaced commas can disrupt data import.
Data Consistency
After importing, it’s essential to check that the data types are consistent for further analysis. Use the `class` function to examine variable types. If you find inconsistencies, consider using casting functions, like `double()` or `string()`, to convert them appropriately.
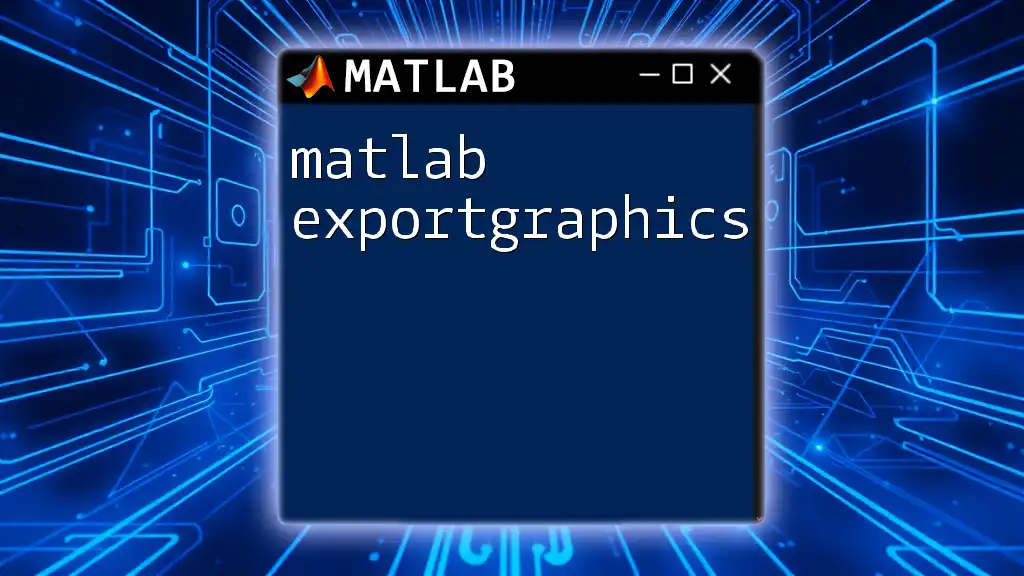
Post-Import Processing
Exploring the Imported Data
Once you have successfully imported the CSV data, it’s time to explore it. Use built-in functions to view summaries and insights.
Example: Display summary statistics:
summary(data);
This command provides an overview of your data, showing the number of entries, variable types, and summary statistics.
Data Visualization
Visualizing your imported data is key for analysis. MATLAB’s plotting functions can quickly generate insightful graphics.
Example: Plotting a simple graph:
plot(data.Column1, data.Column2);
xlabel('Column 1');
ylabel('Column 2');
title('Column 1 vs Column 2');
This code snippet plots `Column1` against `Column2`, offering a visual interpretation of their relationship.
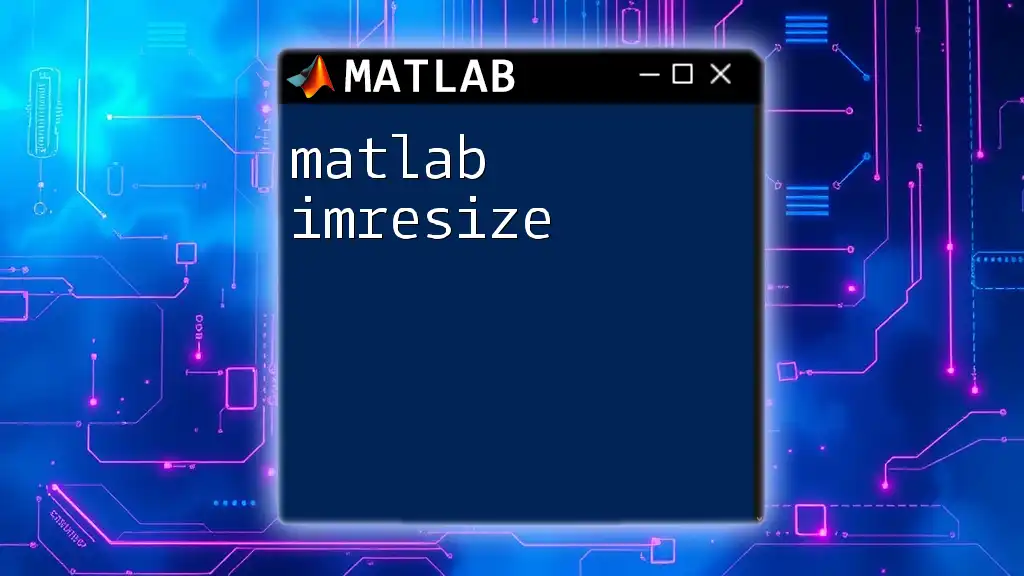
Tips and Best Practices
Choosing the Right Import Function
Opt for `readtable` when flexibility is needed, especially with mixed data formats. Use `csvread` for numeric-only datasets with simple structures.
Maintaining File System Organization
Keep your CSV files organized in a structured directory to support easy access. Naming convention matters; consider including relevant dates and descriptors for ease of identification.
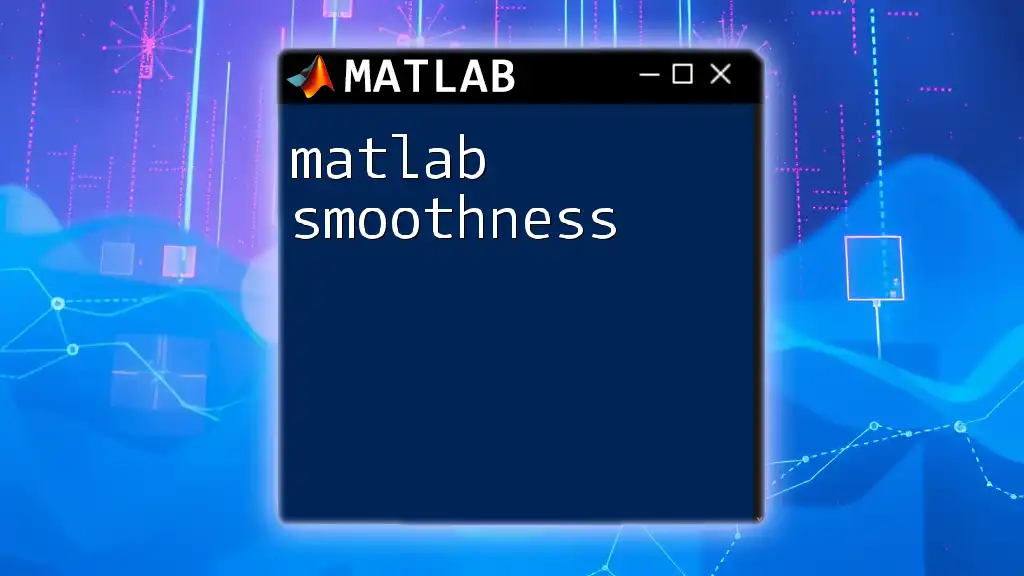
Conclusion
Understanding how to matlab import csv files is critical for data analysis and manipulation. The methods outlined above—from basic functions like `readtable` and `csvread` to advanced techniques for handling data formats—allow you to import, preprocess, and visualize your data efficiently.
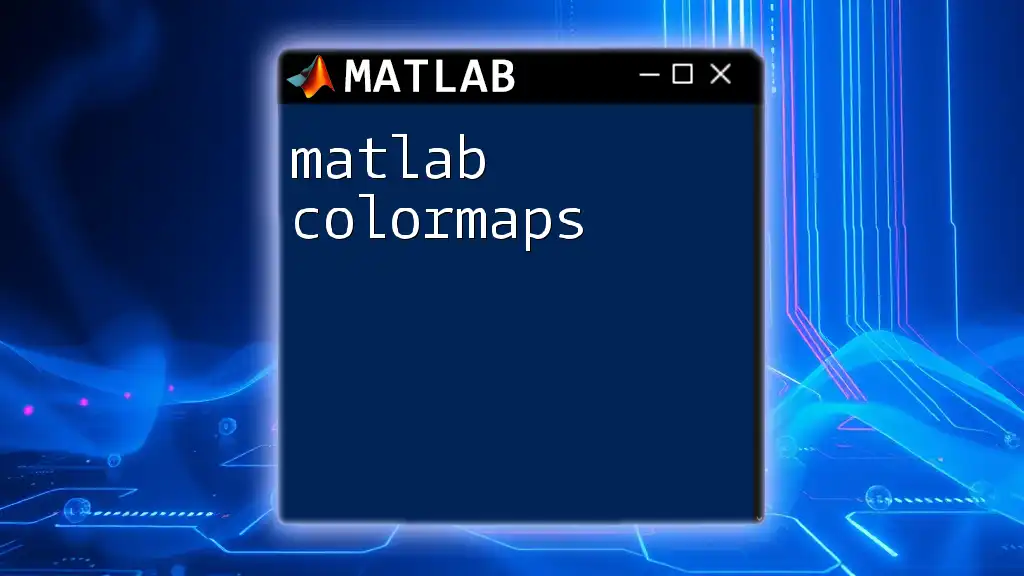
Call to Action
Now that you have a solid foundation, it’s time to practice your skills! Engage with MATLAB and the community, explore additional resources, and continue to enhance your data manipulation abilities. For more quick tips and tutorials, stay tuned for our upcoming posts!