In MATLAB, indexing is the process of accessing specific elements, rows, or columns of arrays and matrices using integer indices.
Here’s a code snippet demonstrating how to index a matrix in MATLAB:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Create a 3x3 matrix
element = A(2, 3); % Access the element at the 2nd row and 3rd column, which is 6
Overview of MATLAB Indexing
What is Indexing?
Indexing in MATLAB is a crucial concept that allows users to access and modify the data stored in arrays. In essence, indexing is the way we refer to the positions of elements within an array or matrix. It is fundamental for manipulating data effectively, whether it involves selecting specific elements, subarrays, or even reshaping data.
Why Indexing Matters in MATLAB
MATLAB is designed to handle large datasets efficiently, and proper indexing is key to optimizing performance. Using efficient indexing methods can lead to significant improvements in runtime, especially when dealing with large matrices. Moreover, understanding how to index properly can enhance code readability, making it easier to debug or modify code in the future.
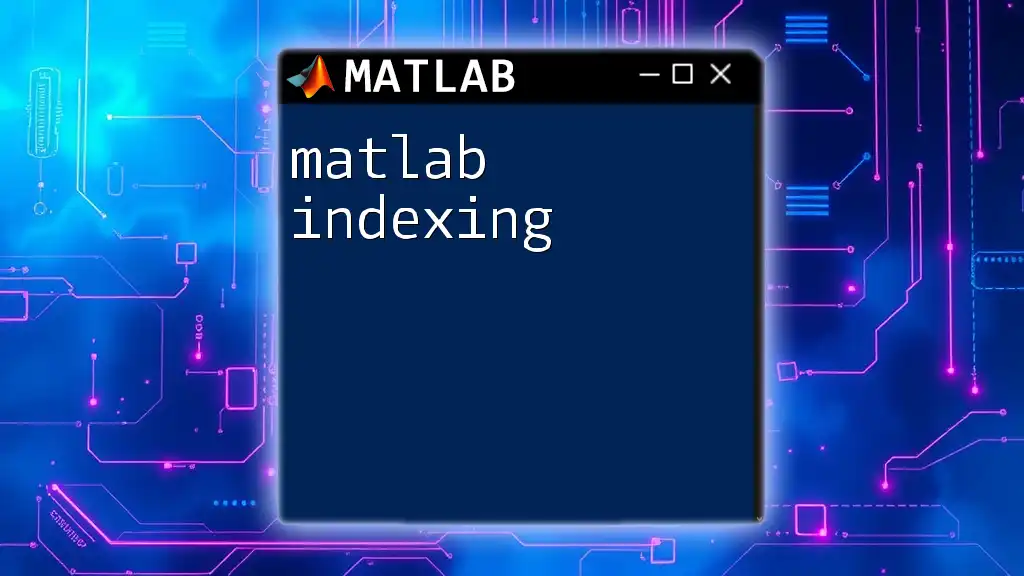
Types of Indexing in MATLAB
Linear Indexing
Linear indexing refers to accessing elements in a MATLAB array as if it were a single column vector, regardless of its original shape. MATLAB stores array data in a column-major order, meaning elements are indexed sequentially column by column.
For example, consider the following code:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
% Accessing element at row 2, column 3
element = A(2, 3); % Output will be 6
% Using linear index to access the same element
element_lin = A(5); % Output will also be 6
In this example, the 5th element in linear indexing corresponds to the element at row 2, column 3 in the original matrix.
Categorical Indexing
Categorical indexing allows users to access elements based on specific criteria defined by categories or groups, enabling more flexible data manipulation.
For instance, let's say we have the following data:
categories = [1, 1, 2, 2, 3];
data = [10, 20, 30, 40, 50];
% Accessing elements corresponding to category 2
indexed_data = data(categories == 2); % Output will be [30, 40]
This allows users to easily filter and extract elements corresponding to a specific category.
Logical Indexing
Logical indexing is another powerful technique that involves creating a logical array that specifies which elements to access based on a condition. This method is highly efficient as it allows for concise data selection.
Consider the following example:
A = [1, 2, 3, 4, 5];
% Creating a logical index for values greater than 3
logical_index = A > 3; % Output will be [false, false, false, true, true]
selected_values = A(logical_index); % Output will be [4, 5]
In this case, we generated a logical array based on a condition and used it to extract only the values greater than 3.
Cell Array Indexing
When working with cell arrays, indexing operates differently. Cell arrays can contain data of various types and sizes, which introduces unique indexing capabilities.
Here's how to access elements in a cell array:
C = {'Hello', 42, [1,2,3]};
% Accessing the second element (42)
value = C{2}; % Output will be 42
In this example, the curly braces `{}` indicate that we are accessing the content at that cell rather than the cell array itself.
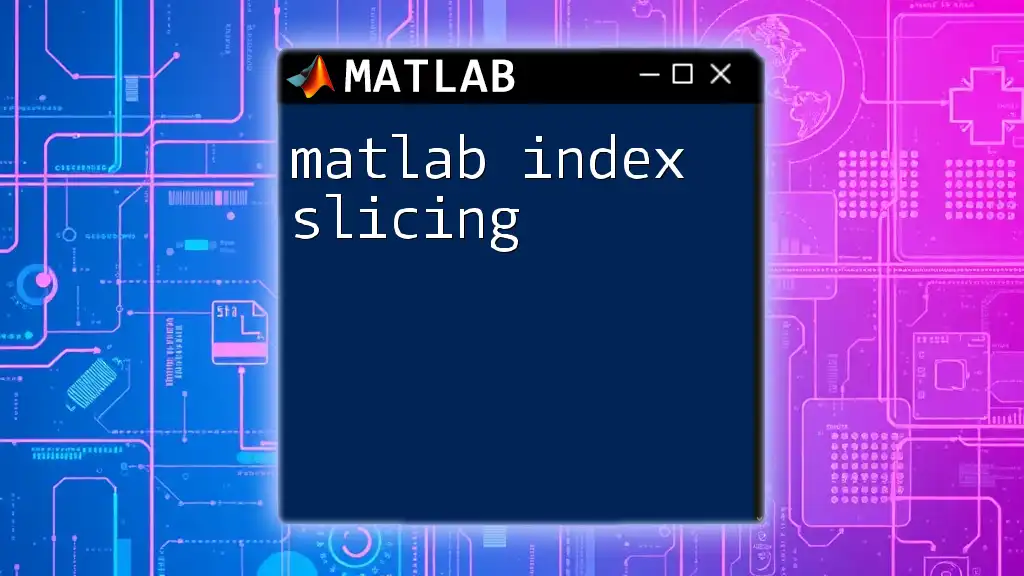
Ways to Index Data in MATLAB
Single Indexing
Single indexing is straightforward—each element in an array can be accessed using its row and column indices.
A = [10, 20; 30, 40];
single_index = A(1); % Output will be 10
Here, the first element in `A` is accessed directly using its index.
Range Indexing
Range indexing allows users to extract multiple elements at once, simplifying the process when you need a subarray.
range = A(1, :); % Output will be [10, 20]
In this case, using the colon operator `:` allows for the selection of all elements in the first row.
Multi-Dimensional Indexing
MATLAB supports indexing in multi-dimensional arrays, which is essential for advanced data analysis.
For example:
B = rand(3, 3, 3); % Creating a 3D array
specific_slice = B(:, :, 2); % Accessing the second slice
Here, we accessed the second slice of the three-dimensional array using `:` to include all rows and columns.
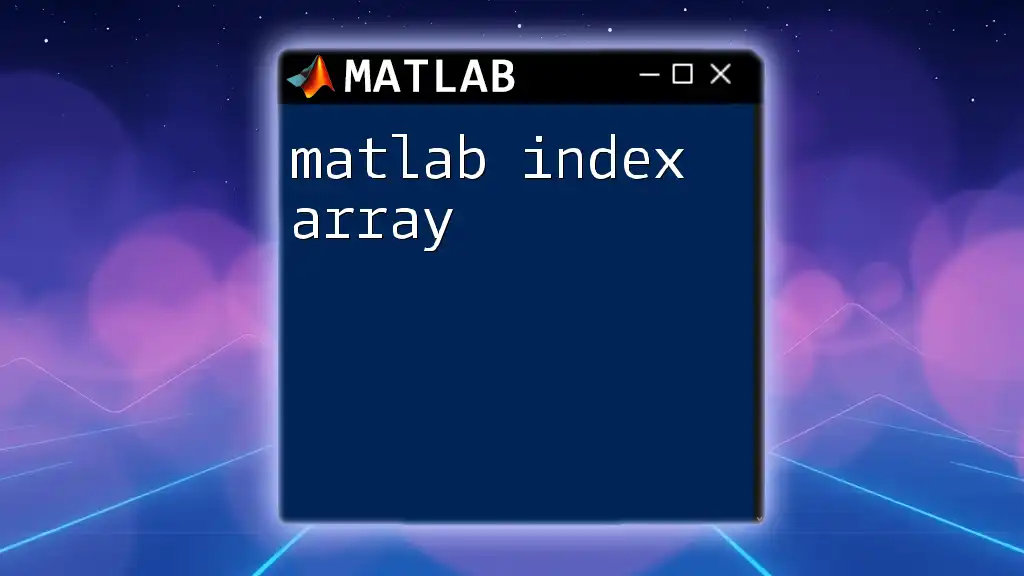
Advanced Indexing Techniques
Using the `end` Keyword
MATLAB provides the `end` keyword to allow for dynamic indexing. This is particularly useful when the size of your array may vary.
For example:
A = [1, 2, 3; 4, 5, 6];
last_element = A(end); % Output will be 6
In this case, `end` automatically refers to the last index of the array, regardless of its size.
Indexing with Functions
MATLAB also allows for functional indexing, enabling more complex data access patterns using built-in functions like `find` and `reshape`.
Here’s an illustration:
A = [1, 2, 3, 4, 5];
indices = find(A > 3); % Output will be [4, 5]
Using `find`, we efficiently located all indices of the elements in `A` that are greater than 3.
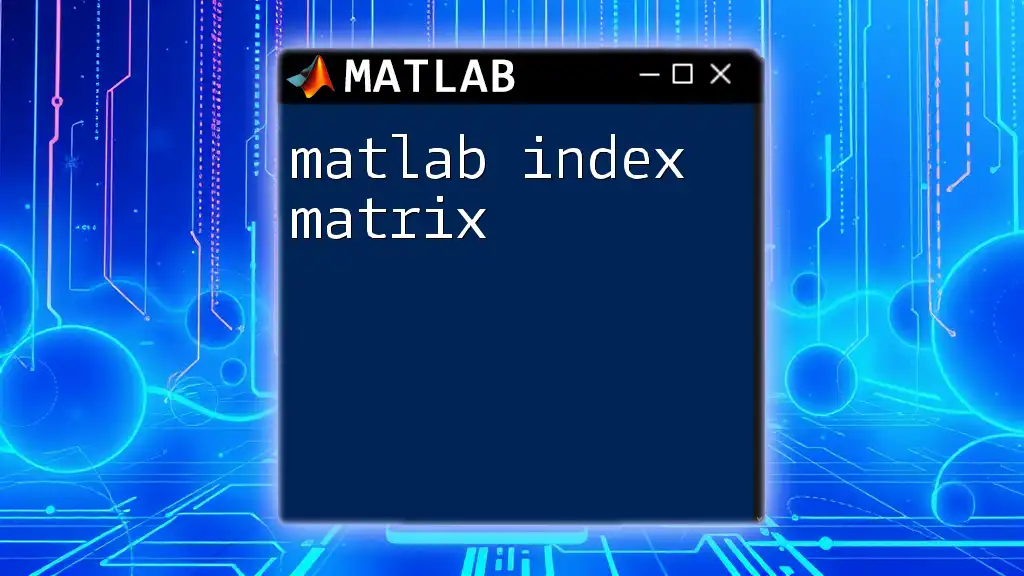
Common Mistakes in Indexing
Off-By-One Errors
One prevalent mistake in indexing is the off-by-one error, which often occurs when users forget that MATLAB arrays are 1-indexed. Unlike other programming languages with 0-based indexing, starting from 1 can lead to unintended results if not considered.
Misunderstanding Dimensions
Misunderstanding the dimensions of arrays can also cause issues. Always verify the size of an array before attempting to index, as it ensures that you are accessing valid elements. The `size` function can be invaluable in this regard.
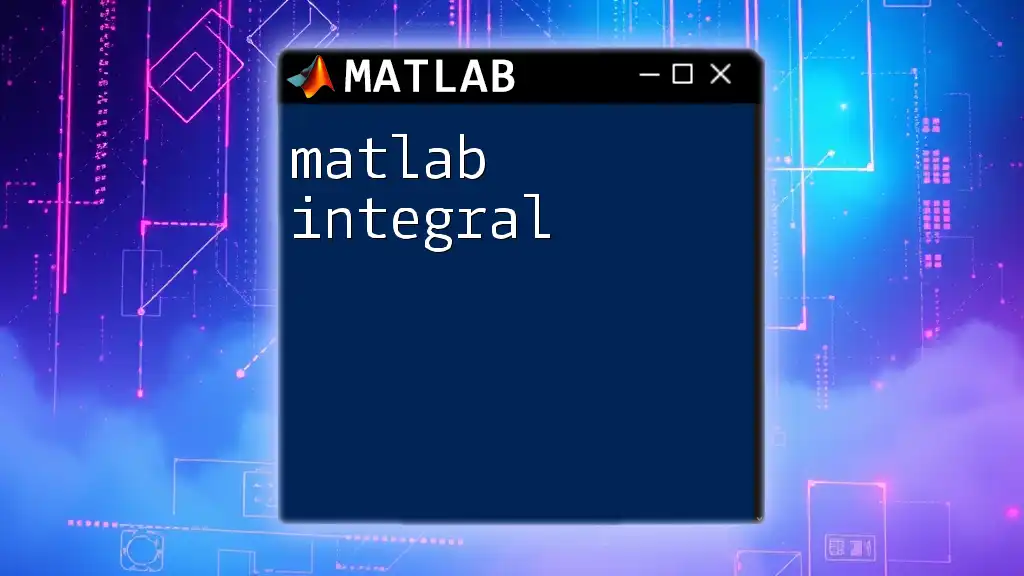
Best Practices for Indexing in MATLAB
Writing Clear and Concise Code
To maintain clarity, it’s important to use descriptive variable names that reflect the contents of the arrays, especially when they are being manipulated with various indexing techniques. Additionally, commenting on complex indexing operations is a good practice that can enhance code readability for yourself and others in the future.
Performance Considerations
Finally, considering indexing performance is vital when dealing with large datasets. Effective indexing not only speeds up computations but also lowers the memory footprint. Avoid unnecessary copying of large matrices, and take advantage of logical indexing and other efficient methods that MATLAB provides.
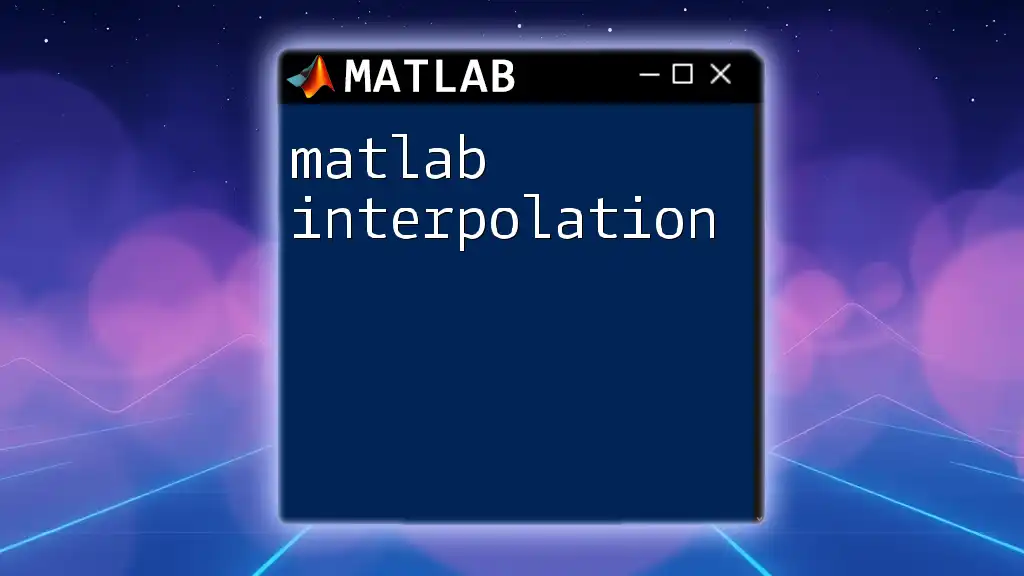
Conclusion
In conclusion, mastering MATLAB indexing is an essential skill for anyone looking to become proficient in data manipulation and analysis within MATLAB. Whether you are using linear, categorical, logical, or cell array indexing, understanding these concepts will substantially improve your coding efficiency and capability. For further learning, consult the official MATLAB documentation and participate in community forums to deepen your knowledge of these powerful indexing techniques.