An identity matrix in MATLAB is a square matrix with ones on the diagonal and zeros elsewhere, which can be created using the `eye` function.
I = eye(3); % Creates a 3x3 identity matrix
Understanding the Identity Matrix
Definition of Identity Matrix
An identity matrix, often denoted as \( I_n \) for an \( n \times n \) square matrix, serves as the multiplicative identity in the realm of matrices. This means that when any matrix \( A \) (of compatible dimensions) is multiplied by an identity matrix, the result remains \( A \).
Properties of Identity Matrix
The identity matrix possesses several critical properties that are essential for linear algebra:
-
Multiplicative Identity: For any matrix \( A \), multiplying it by the identity matrix yields the original matrix: \[ I \cdot A = A \cdot I = A \]
-
Square Matrix: Identity matrices are always square in structure. They have an equal number of rows and columns (m x m).
-
Eigenvalues and Eigenvectors: The eigenvalues of an identity matrix are always equal to one, with corresponding eigenvectors being the standard basis vectors. This implies that every vector remains untouched upon multiplication by the identity matrix.
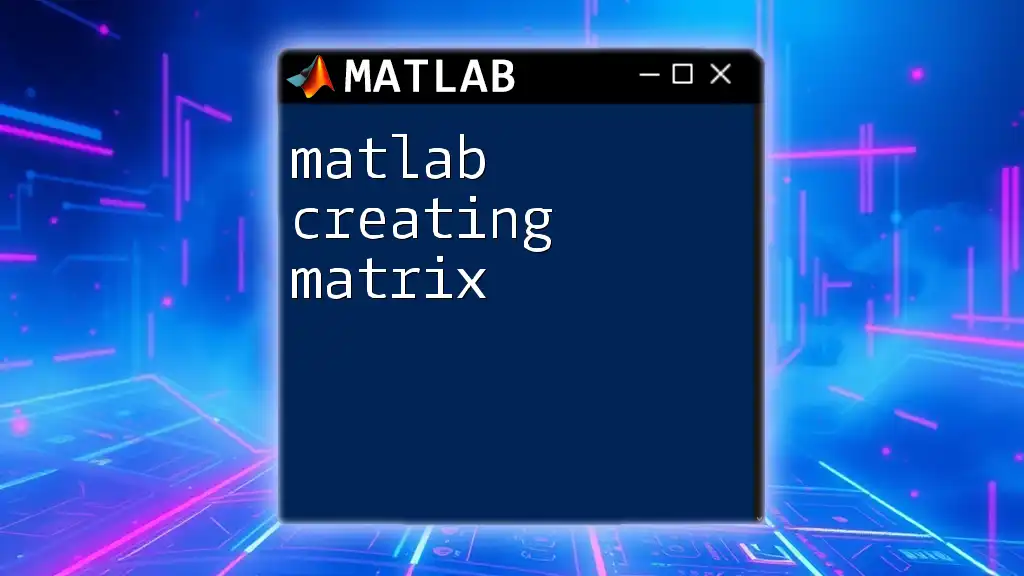
Creating an Identity Matrix in MATLAB
Syntax: `eye()`
In MATLAB, the function to create an identity matrix is `eye()`. Using this function is straightforward but highly effective:
- Basic syntax: To create an \( n \times n \) identity matrix, you simply use:
I = eye(n);
Example Code Snippet:
To generate a 3x3 identity matrix, execute the following:
I = eye(3); % Creates a 3x3 identity matrix
disp(I);
The output will be:
1 0 0
0 1 0
0 0 1
Generating Identity Matrices of Different Sizes
The `eye()` function allows for the creation of identity matrices of various sizes. You can create a 2x2 or a 5x5 identity matrix with ease.
Example Code Snippets:
To create different identity matrices, you might use:
I2 = eye(2); % 2x2 Identity Matrix
I5 = eye(5); % 5x5 Identity Matrix
This flexibility makes the identity matrix a powerful tool in many matrix operations.
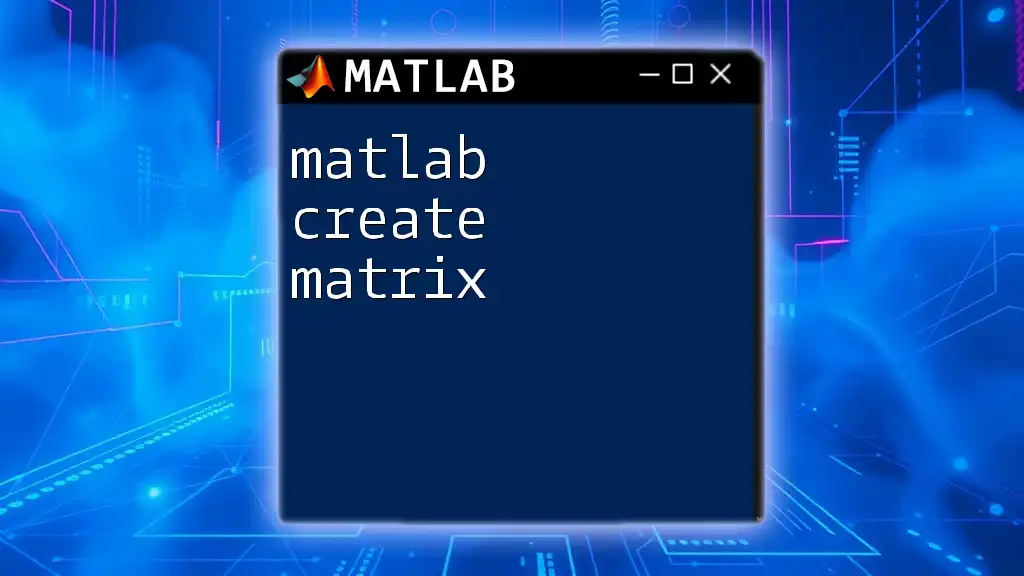
Using Identity Matrices in MATLAB
Matrix Operations Involving Identity Matrices
Identity matrices facilitate a variety of matrix operations, particularly addition and subtraction with other matrices.
Addition and Subtraction
When you add an identity matrix to another matrix of the same dimensions, you are effectively incrementing the diagonal elements by 1.
Example Code for Addition:
To illustrate this, consider:
A = [1, 2; 3, 4];
result = A + eye(2); % Adds 2x2 identity matrix to A
disp(result);
The output will be:
2 2
3 5
Here, the diagonal elements of matrix \( A \) are incremented, showcasing the interaction with the identity matrix.
Solving Linear Equations
Identity matrices play a crucial role in solving linear equations. They help to simplify and manipulated equations of the form \( Ax = b \).
Example Code:
Suppose you need to solve the linear system represented by the matrix:
A = [1, 2; 3, 4];
b = [5; 11];
x = inv(A) * b; % Alternatively, this can also be done using A \ b
disp(x);
This operation allows you to find the solution vector \( x \) effectively, showcasing the practical applications of identity matrices in solving complex problems.
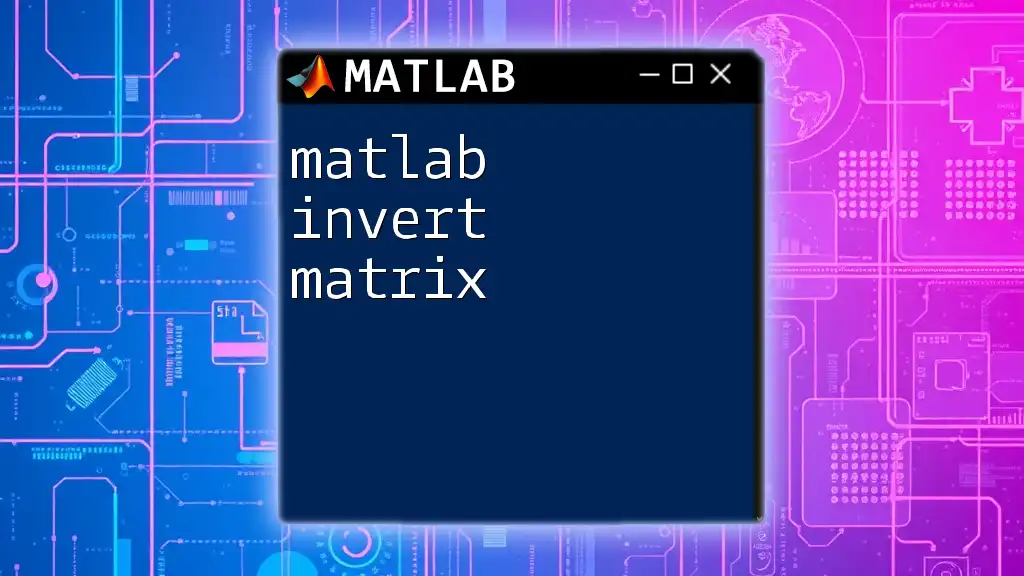
Advanced Applications of Identity Matrices
Identity Matrix in Eigenvalue Problems
In linear algebra, eigenvalue problems are highly pertinent. The identity matrix plays a role in these problems specifically when decomposing matrices into their eigenvalues and eigenvectors.
Example Code Snippet:
To compute the eigenvalues and eigenvectors of a matrix:
A = [2, 1; 1, 2];
[V, D] = eig(A); % Where D is a diagonal matrix with eigenvalues
Here, \( D \) will contain the eigenvalues along its diagonal. The corresponding eigenvectors are stored in \( V \).
Identity Matrix in Control Theory
In control theory, the identity matrix is integral when representing states in dynamical systems. It aids in defining controllable states and plays a part in system stability analysis.
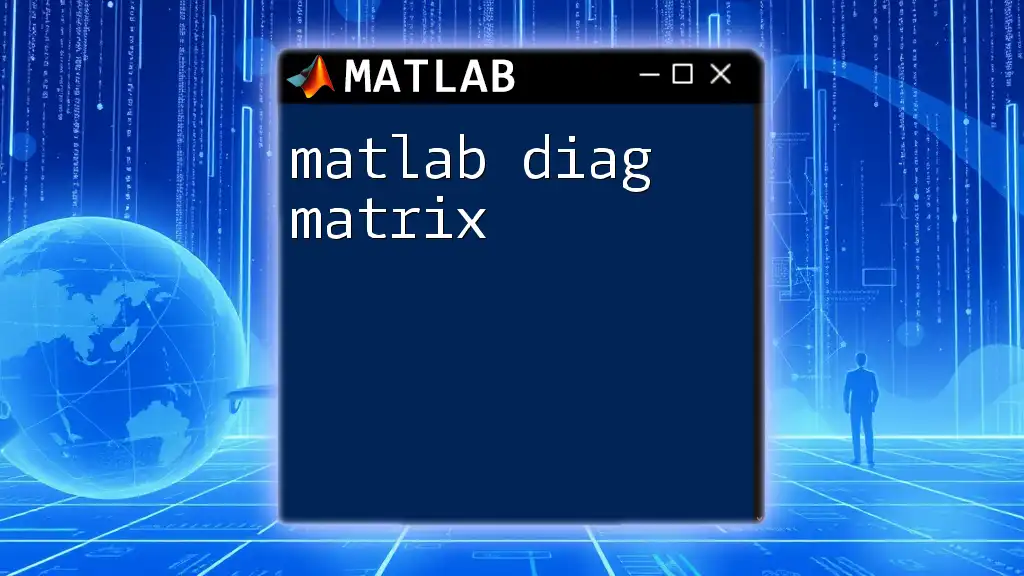
Common Errors and Troubleshooting
Common Pitfalls When Working with Identity Matrices
When working with identity matrices, several common pitfalls may arise, particularly in how they interact with other matrices.
Errors in Dimensions and Size Mismatches
It is essential to ensure that any matrix operation involving identity matrices adheres to the correct dimensional rules.
Example of a Common Coding Error:
Consider the following erroneous operation:
% Mistakenly trying to add a 2x2 to a 3x3 matrix
B = eye(3);
wrong_result = A + B; % This will throw an error
Properly understanding the constraints of dimensionality is crucial to avoid errors.
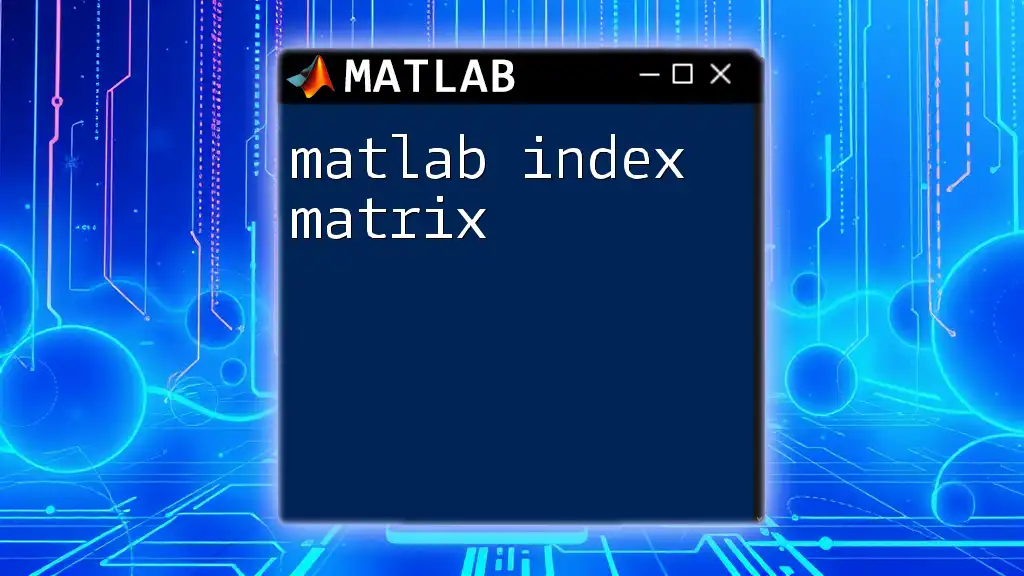
Conclusion
In summation, the MATLAB identity matrix serves as a vital mathematical tool, providing simplicity and efficiency in various mathematical operations. Understanding its creation, properties, and applications enhances your MATLAB skills and empowers you to tackle more complex problems effectively. Feel free to experiment and explore the numerous functionalities associated with identity matrices in MATLAB. For further learning, check out additional tutorials available on our platform for an in-depth dive into all things MATLAB.
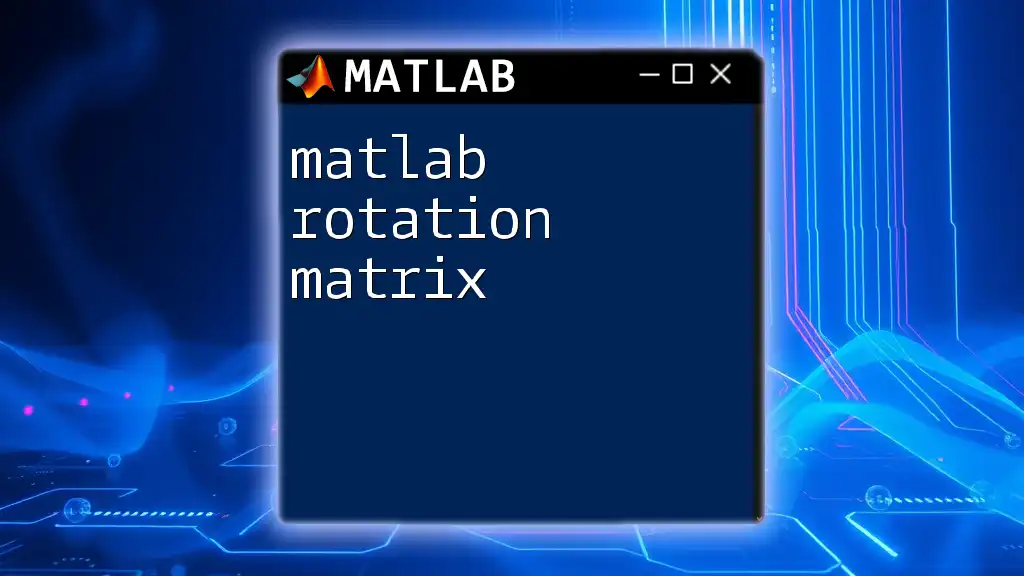
Additional Resources
For those looking to deepen their understanding, recommendations include textbooks and online courses focusing on both MATLAB and linear algebra. Additionally, consult MATLAB’s comprehensive documentation and engage with community forums to solve queries and improve your coding practices.