The `ind2sub` function in MATLAB converts linear indices into subscript indices for a given array size, allowing you to retrieve the row and column positions of elements seamlessly.
Here’s a code snippet demonstrating how to use `ind2sub`:
% Example: Convert linear indices to subscripts for a 3x3 matrix
linearIndices = [1, 5, 7]; % Linear indices
matrixSize = [3, 3]; % Size of the matrix
[subscriptRow, subscriptCol] = ind2sub(matrixSize, linearIndices);
% Display the results
disp([subscriptRow', subscriptCol']);
What is `ind2sub`?
The `matlab ind2sub` function is a powerful tool within the MATLAB programming environment used for converting linear indices into subscripts. It allows you to easily map a one-dimensional index to its corresponding row and column locations in a multi-dimensional array. This capability is essential when you need to reference specific elements of arrays or matrices without ambiguity.
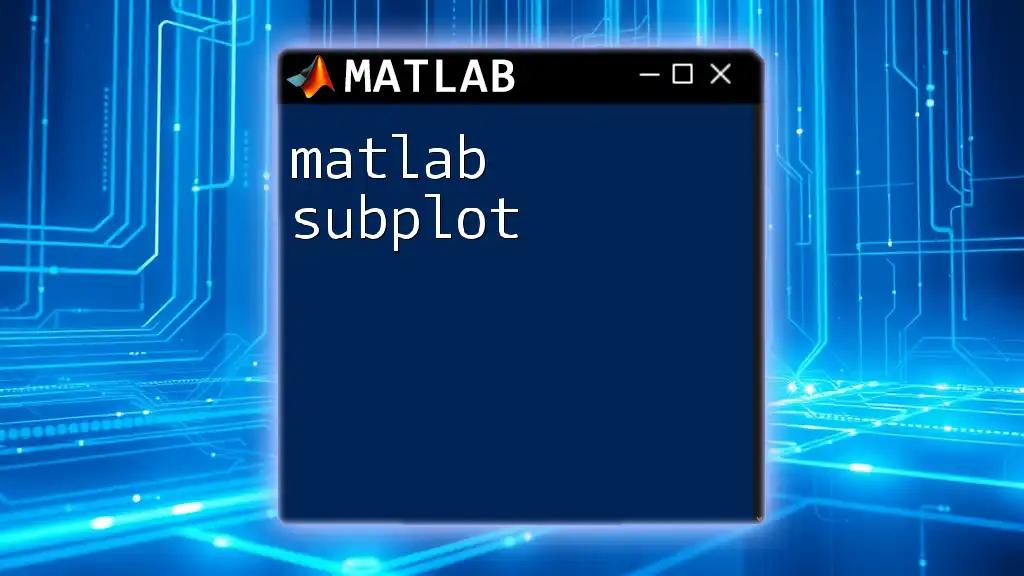
When to Use `ind2sub`?
There are several scenarios where `ind2sub` proves to be invaluable:
- Data analysis: When you're working with matrices and need to extract or manipulate specific elements based on their linear indices.
- Image processing: It's particularly beneficial when handling image data represented as matrices, enabling pixel access via linear indices.
- Matrix computations: When interacting with results from algorithms that return linear indices and require your next step to reference those locations.
Understanding when to use `ind2sub` can enhance your efficiency in MATLAB programming and data manipulation.
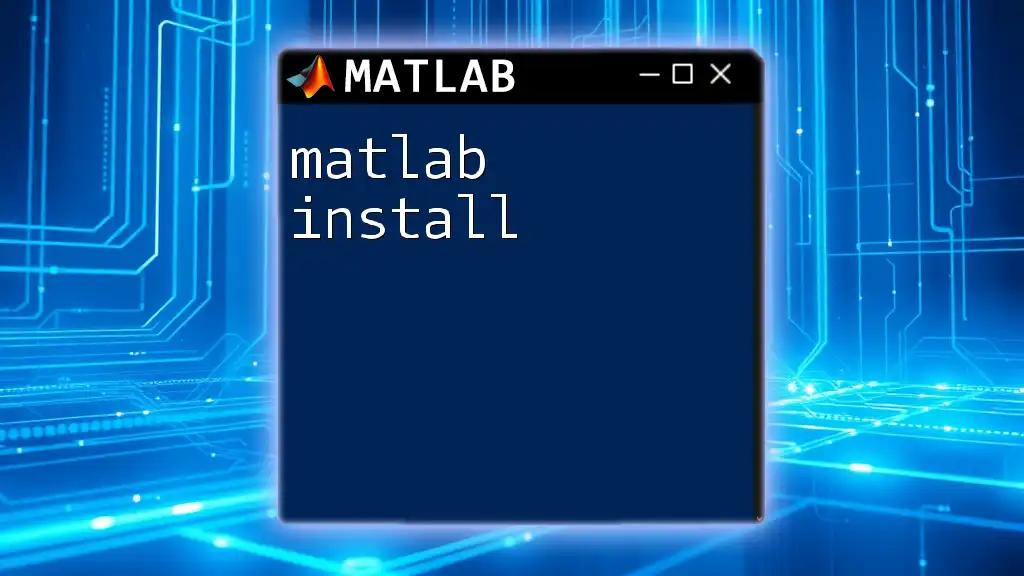
Understanding Indexing in MATLAB
Linear vs. Subscript Indexing
Linear Indexing involves representing a multi-dimensional array as a single-dimensional vector. For example, in a 2D matrix of size `m x n`, each element can be accessed using a single index ranging from 1 to `m*n`.
Subscript Indexing, on the other hand, uses multiple indices to specify the location of an element within its respective dimensions, such as `(row, col)` for a 2D array or `(x, y, z)` for a 3D array.
How `ind2sub` Bridges the Two
`ind2sub` provides a seamless transition between these two forms of indexing. It allows programmers to convert linear indices back to more interpretable subscript indices that clearly indicate the corresponding row and column positions in an array or matrix. This is particularly useful when the output of calculations is provided in linear index form.
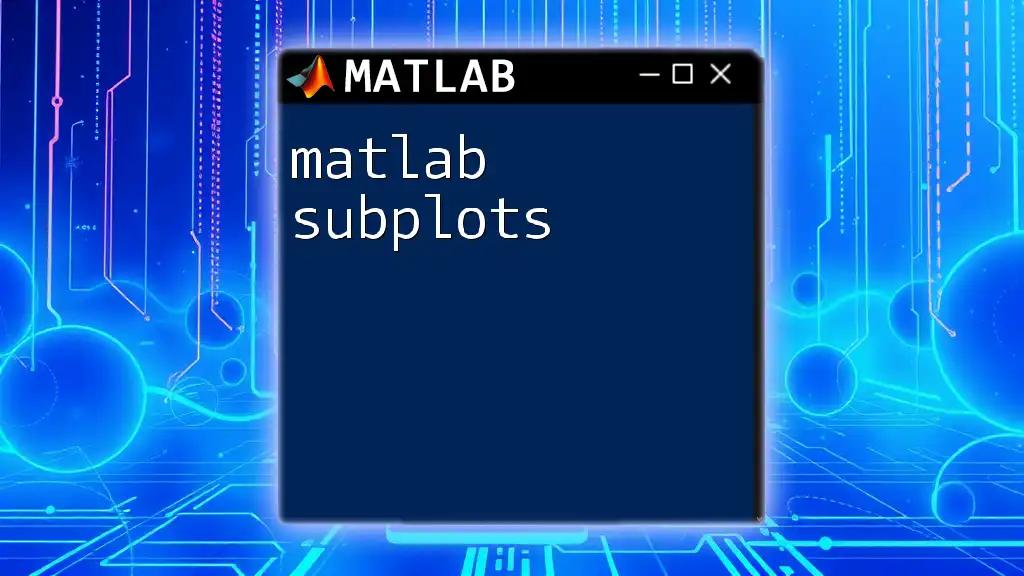
Syntax and Parameters of `ind2sub`
Basic Syntax
The syntax for the `matlab ind2sub` function is straightforward:
[row, col] = ind2sub(array_size, linear_index);
Parameters Explained
- array_size: This argument specifies the dimensions of the array you are working with. It can be a vector defining the size of each dimension—for example, `[3, 4]` for a 3x4 matrix.
- linear_index: This is the linear index you wish to convert, represented as an integer or an array of integers. It indicates the position of the element in one-dimensional terms.
The function also gracefully handles multi-dimensional arrays, converting a single linear index into the respective subscripts for as many dimensions as are present in the input array.
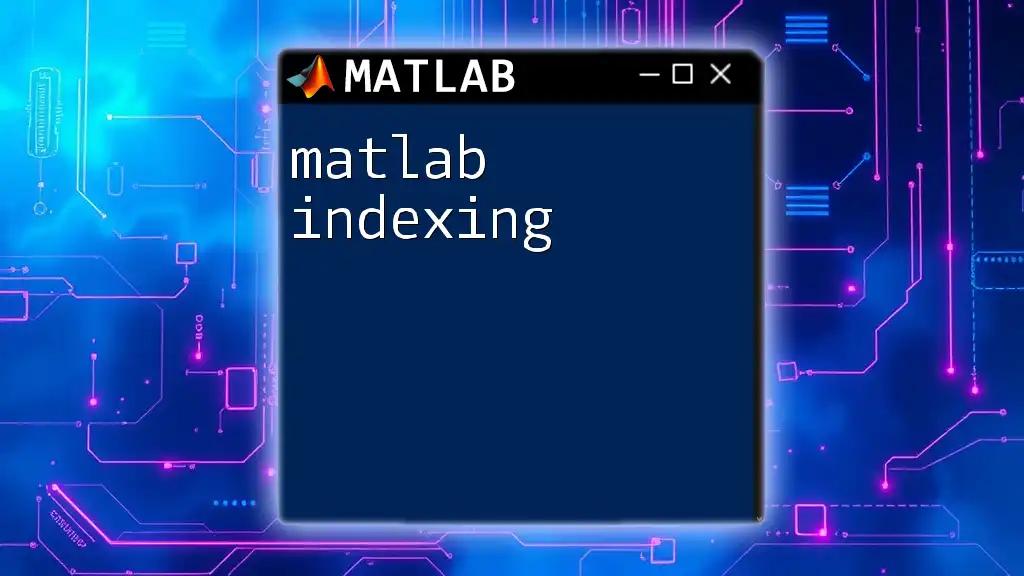
Step-by-Step Usage of `ind2sub`
Example 1: Basic Usage
To illustrate the basic use of `ind2sub`, consider the following scenario: you want to convert a linear index to its corresponding row and column position in a matrix.
A = magic(4); % Create a 4x4 magic square
index = 5; % Select a linear index
[row, col] = ind2sub(size(A), index);
disp(['Row: ' num2str(row) ', Column: ' num2str(col)]);
In this example, the output will tell you which row and column correspond to the fifth position in the 4x4 magic square matrix.
Example 2: Working with Multidimensional Arrays
The `matlab ind2sub` function also excels when dealing with multi-dimensional arrays. For instance:
B = rand(2, 3, 4); % Create a 2x3x4 random array
index = 7; % Linear index for 3D array
[x, y, z] = ind2sub(size(B), index);
disp(['X: ' num2str(x) ', Y: ' num2str(y) ', Z: ' num2str(z)]);
Here, this code converts the linear index to 3D subscripts, indicating the position in the three-dimensional array.
Example 3: Batch Conversion of Indices
`ind2sub` is capable of processing multiple linear indices simultaneously, making it efficient for batch operations.
indices = [2, 5, 7]; % Linear indices for conversion
[rows, cols] = ind2sub(size(A), indices);
disp([rows', cols']); % Display the respective rows and columns
By using the output format, you will receive a matrix where each row corresponds to the subscript position of the respective linear index.

Practical Applications of `ind2sub`
Image Processing and Analysis
In the realm of image processing, `ind2sub` is crucial for pixel manipulation. For example, if you want to analyze a specific pixel in an image matrix, knowing its linear index from a filtered operation is beneficial. You can use `ind2sub` to fetch the row and column corresponding to that index and adjust pixel values or access neighboring pixels.
Data Extraction in Machine Learning
In machine learning, arrays hold essential data, such as features extracted from datasets. When obtaining results from classification algorithms, you might receive indices that identify specific features or samples. Using `ind2sub` will allow you to retrieve and manipulate the data efficiently, ensuring that you are working on the correct elements within your dataset.
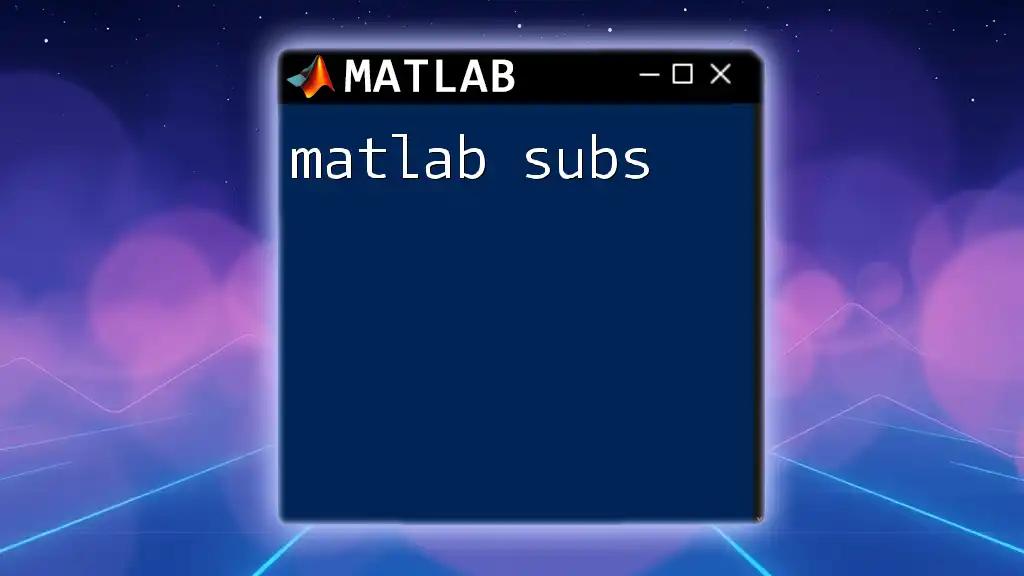
Common Issues and Troubleshooting
Errors Related to `ind2sub`
When using `ind2sub`, it’s common to encounter various errors:
- Dimension Mismatch: This occurs when the size of the linear index provided does not match the expected dimensions of the array. Always double-check the dimensions of your input to avoid this issue.
- Index Out of Bounds: Ensure that the linear indices are within the valid range from 1 to `numel(array)`, i.e., the total number of elements in the array.
Best Practices
To leverage `matlab ind2sub` effectively, consider these best practices:
- Always verify the dimensions of the array when passing `array_size`.
- Keep your indices within bounds, especially when working with dynamic array sizes.
- Document your code for readability, especially when performing multiple conversions.
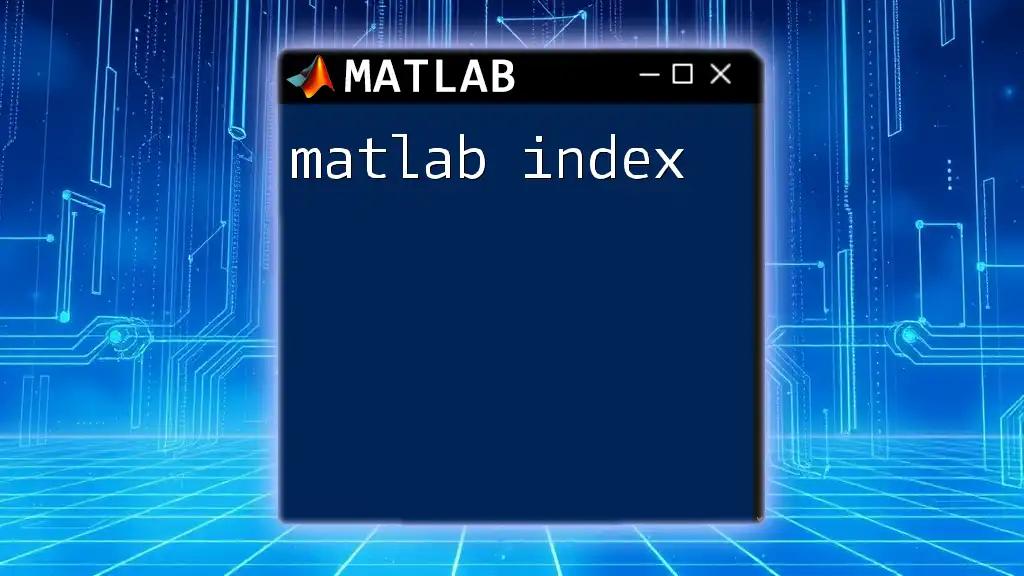
Conclusion
The `matlab ind2sub` function is an essential part of any MATLAB programmer's toolkit, simplifying the task of working with multi-dimensional arrays. By understanding its usage, applications, and best practices, you can enhance your coding efficiency and accuracy in MATLAB.
Call to Action
Practice the `ind2sub` function using various matrices and datasets to solidify your understanding. Explore related topics to expand your MATLAB skills even further.

Additional Resources
MATLAB Documentation
For more detailed information, refer to the official MATLAB documentation for `ind2sub`, guiding you through its numerous capabilities and examples.
Community Forums
Leverage forums and communities like MATLAB Central for discussions, troubleshooting help, and sharing experiences with the `ind2sub` function and other MATLAB features.