In MATLAB, an index matrix is a matrix of indices that can be used to access or modify elements in another matrix based on their positions.
Here's a simple code snippet demonstrating the use of an index matrix:
% Example of using an index matrix to access elements
A = [10, 20, 30; 40, 50, 60; 70, 80, 90];
indexMatrix = [1, 3; 2, 1]; % Indices to access elements
result = A(indexMatrix) % Access elements at these positions
Understanding Indexing in MATLAB
What is Indexing?
Indexing is a fundamental concept in MATLAB that allows you to access and manipulate elements within arrays, matrices, and tables. Understanding how indexing works is crucial for effective programming and data handling. By leveraging indexing, you can retrieve specific data points based on their position or condition, making data analysis and manipulation much more efficient.
Types of Indexing
-
Logical Indexing: This method uses a logical array (true/false values) to specify which elements to access. For example, if you have a matrix and want to select elements that meet certain conditions, logical indexing simplifies this task significantly.
-
Linear Indexing: MATLAB provides a unique way to access elements using single indices to represent linear positions across matrices. This method is especially useful when dealing with multi-dimensional arrays.
-
Subscript Indexing: This is one of the most common forms of indexing, where you use row and column numbers to specify the elements you want to access. Subscript indexing is straightforward and intuitive, making it a preferred choice for many users.
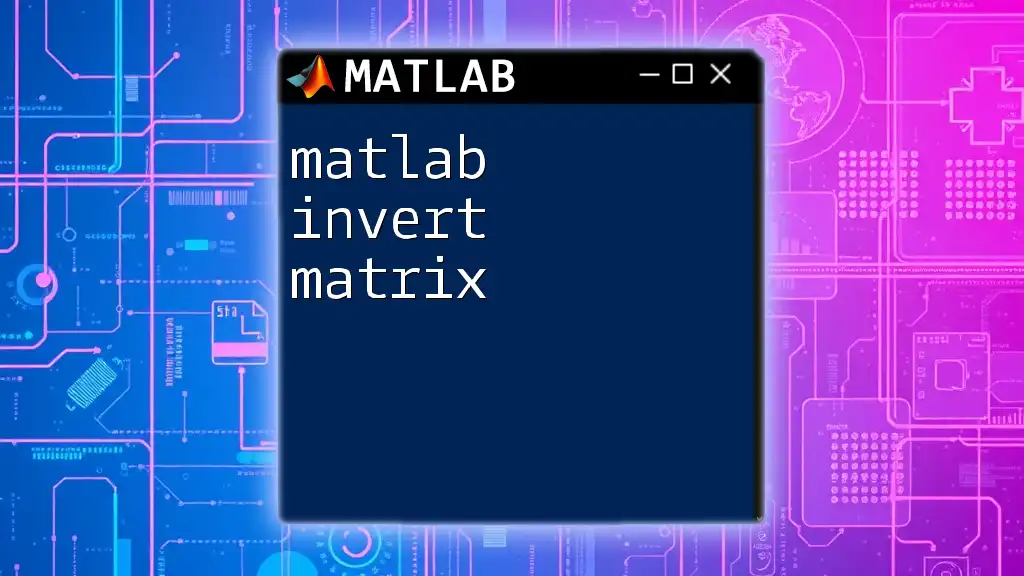
Creating an Index Matrix
Definition of an Index Matrix
An index matrix is a specialized matrix used to control and access data within another matrix. Instead of storing numerical values, an index matrix contains indices pointing to the elements of the original matrix. This technique allows for efficient data manipulation and retrieval, particularly when handling large datasets.
Constructing an Index Matrix
Creating an index matrix in MATLAB involves specifying rows and columns to subset the original matrix. Below is a step-by-step example:
Suppose you have a 3x3 matrix as follows:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
To create an index matrix that contains a subset, you can do:
indexMatrix = A(1:2, 2:3);
In this case, `indexMatrix` will be:
2 3
5 6
This example illustrates how selecting specific rows and columns from `A` forms a new matrix.
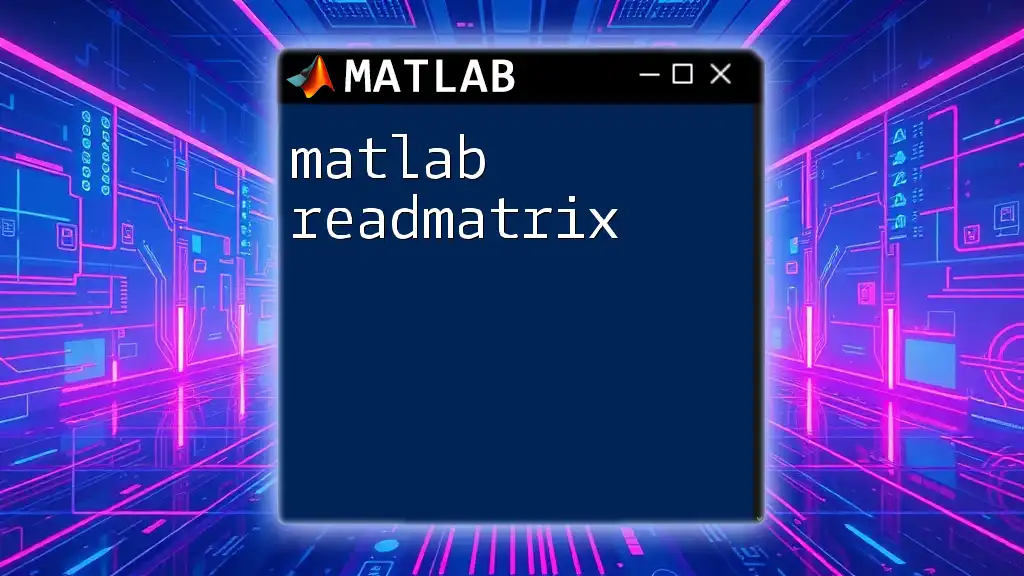
Using Index Matrices for Data Selection
Accessing Data with Index Matrices
Index matrices can be employed to retrieve specific data points based on given conditions. For instance, if you wanted to access the elements of `A` that form the first two rows and the last column, your command would look something like this:
selectedData = A(1:2, end);
Here, `selectedData` would yield:
2
5
This demonstrates the practical utility of index matrices in data selection.
Modifying Data with Index Matrices
Index matrices not only allow you to access data but also to modify it. For example, if you want to change the values in the first and third rows of matrix `A`, you can use:
A([1,3], :) = [10, 11, 12];
After executing this command, matrix `A` will now look like:
10 11 12
4 5 6
10 11 12
This feature is particularly useful for customizing datasets based on specific criteria or conditions.
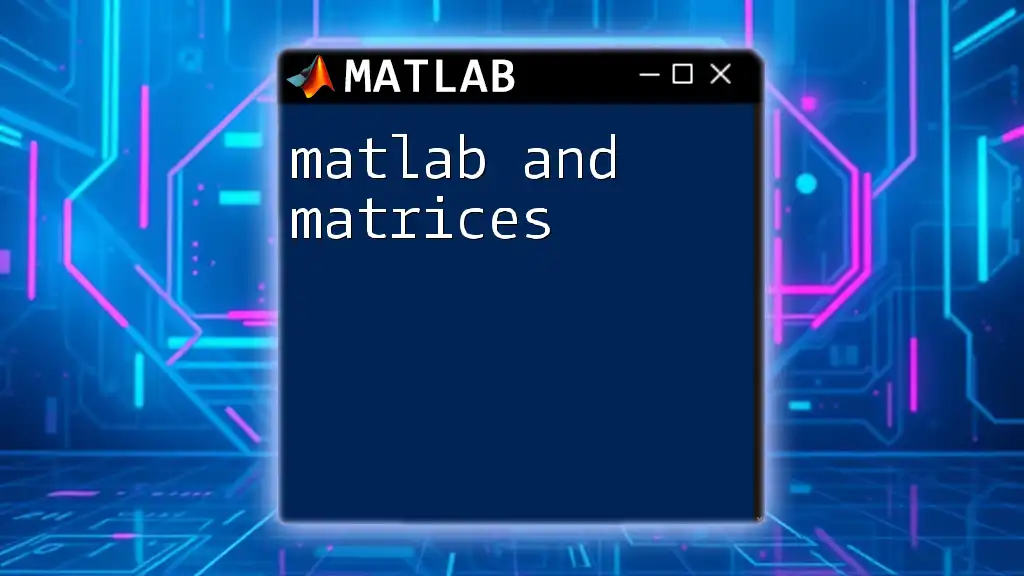
Advanced Indexing Techniques
Multi-dimensional Indexing
When you're working with multi-dimensional arrays, indexing can become more complex but highly beneficial. For example, consider a 3D index matrix. Suppose you have a 3D matrix representing a stack of images:
B = rand(5, 5, 3); % Creates a random 5x5x3 matrix
To access a specific "slice" of this 3D matrix, you can use:
firstSlice = B(:, :, 1);
This would extract the first 2D layer, allowing you to work with it independently.
Using the FIND Function
The `find` function is an essential tool for advanced indexing. This function locates the indices of non-zero elements or elements that meet a specified condition. For instance:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
indices = find(A > 4);
Here, `indices` would return:
5
6
7
8
9
This allows you to conveniently pinpoint and operate on only the elements meeting a condition.
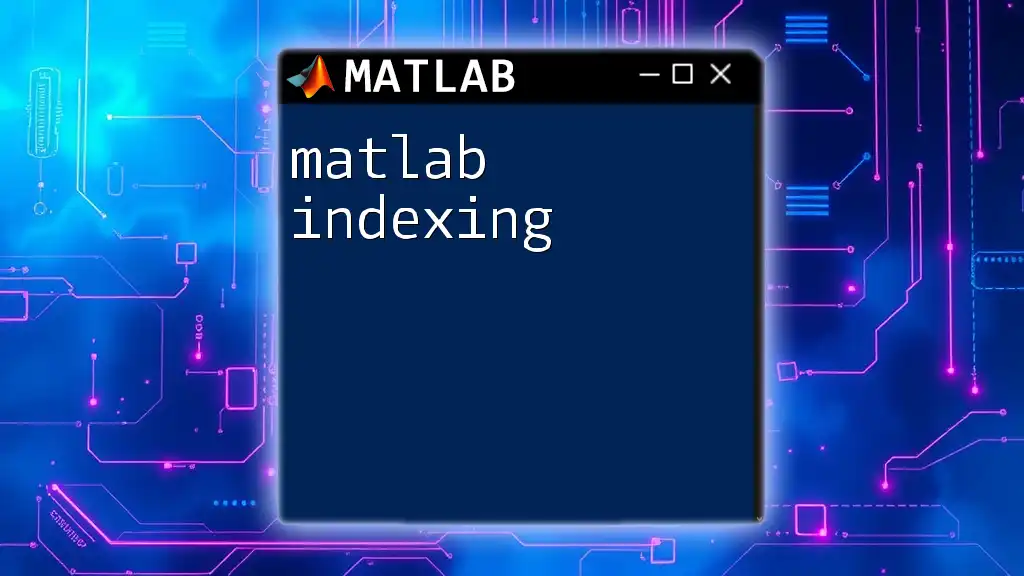
Practical Applications of Indexing
Data Analysis Scenarios
Index matrices are essential when performing data analysis. For example, if you're tasked with filtering a dataset to include only those entries that meet specific requirements, you can use indexing effectively:
data = rand(10, 3); % Creates a random 10x3 matrix
filteredData = data(data(:, 1) > 0.5, :);
In this command, filteredData captures only those rows where the first column's values exceed 0.5, facilitating targeted analysis.
Image Processing
In image processing, indexing is a powerful method for manipulating pixel values. For instance, to access a block of pixels in an image matrix:
img = imread('image.jpg');
pixelBlock = img(1:10, 1:10, :);
In this example, you extract a 10x10 pixel block from the top left corner of the image, which can then be modified or analyzed as needed.
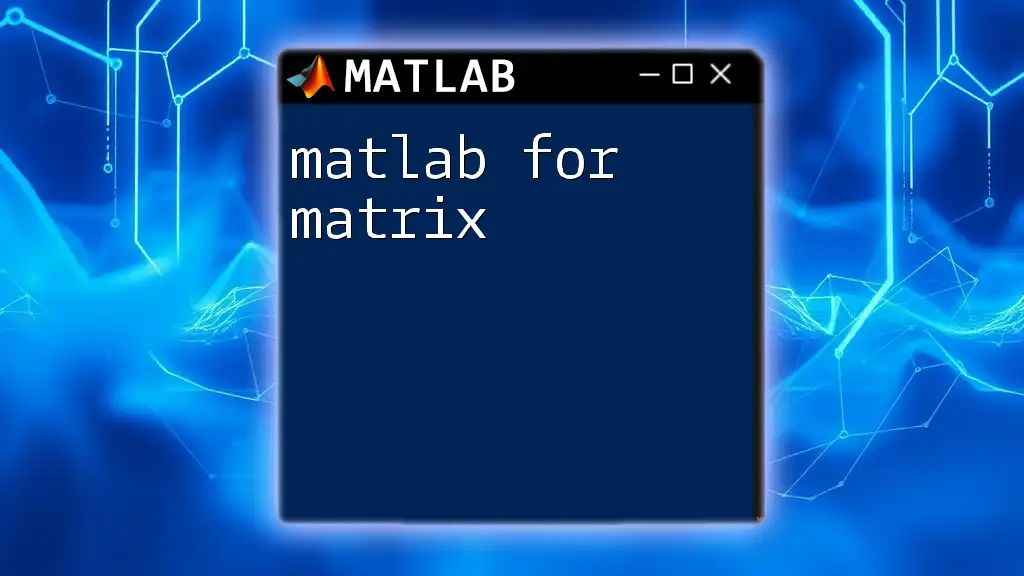
Common Mistakes and Troubleshooting
Common Errors with Indexing
Indexing errors are common, particularly when dealing with matrices of varying sizes. One frequent mistake is attempting to access an element outside the matrix bounds, which results in an "index exceeds matrix dimensions" error. For instance:
A(4, 2); % This will generate an error if A is 3x3
To avoid such mistakes, always ensure you check the dimensions of your matrices before indexing.
Debugging Techniques
If you encounter issues when using index matrices, consider using debugging tools available in MATLAB. The `disp` function can help print matrices to the console, allowing you to verify their contents and index values effectively.
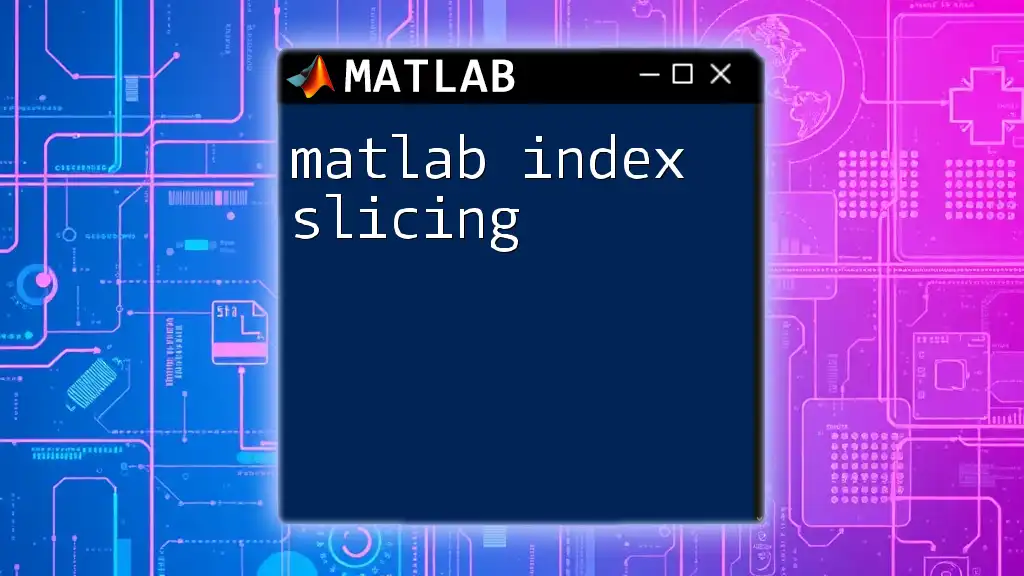
Conclusion
Understanding the concept of the MATLAB index matrix and its applications is vital for enhancing your MATLAB skills. By mastering various indexing techniques, you can dramatically improve your efficiency in data manipulation and analysis.
To further your knowledge, practice the examples provided and explore additional MATLAB tutorials focused on indexing. You will discover how this powerful tool can elevate your data handling capabilities.