MATLAB index slicing allows you to extract specific elements or subsets from arrays by specifying their indices in a concise manner.
Here’s a code snippet demonstrating basic index slicing:
% Create a sample array
A = [10, 20, 30, 40, 50];
% Extract elements from index 2 to 4
B = A(2:4); % B will be [20, 30, 40]
Understanding Indexing in MATLAB
What is Indexing?
Indexing is a fundamental concept in programming that allows us to access and manipulate data stored in arrays, matrices, and other types of data structures. In MATLAB, indexing plays a crucial role in the ease of data manipulation, enabling users to extract specific elements or subsets from larger data sets quickly.
Types of Indexing in MATLAB
MATLAB supports various types of indexing, each serving different purposes:
-
Numeric Indexing: This is the most straightforward approach, where you use integers to pinpoint specific elements in an array.
-
Logical Indexing: Here, you leverage logical arrays (arrays composed of `true` and `false` values) to access elements conditionally.
-
Cell Indexing: Particularly useful when dealing with cell arrays, cell indexing lets you access elements that may vary in size or type.
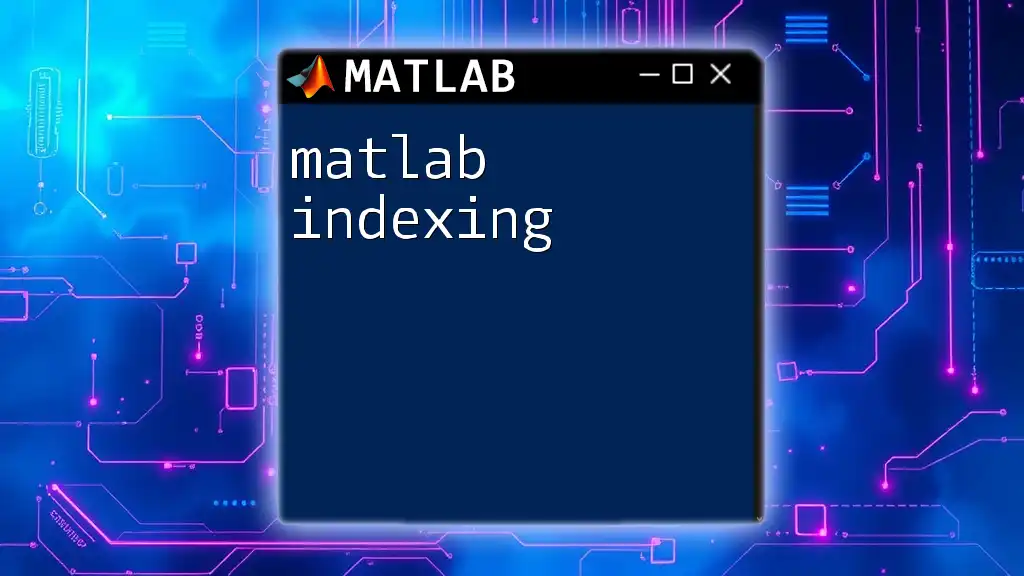
MATLAB Index Slicing Basics
What is Index Slicing?
Index slicing refers to accessing a range of data rather than individual elements. It provides a flexible way to extract subsets of data without manually specifying each element. This capability transforms indexing into a powerful tool for data manipulation.
Basic Syntax for Index Slicing
The basic syntax for index slicing in MATLAB is:
array(startIndex:endIndex)
In this syntax, `startIndex` denotes the position from which you want to begin your slice, and `endIndex` indicates where the slice should end. This functionality is foundational for efficiently handling data.
Examples of Basic Index Slicing
Here’s a simple example:
A = [1, 2, 3, 4, 5];
slice_A = A(2:4); % Result will be [2, 3, 4]
This code snippet takes elements from positions 2 to 4 in the array `A`, yielding the result `[2, 3, 4]`. Such clarity in slicing simplifies operations on arrays.
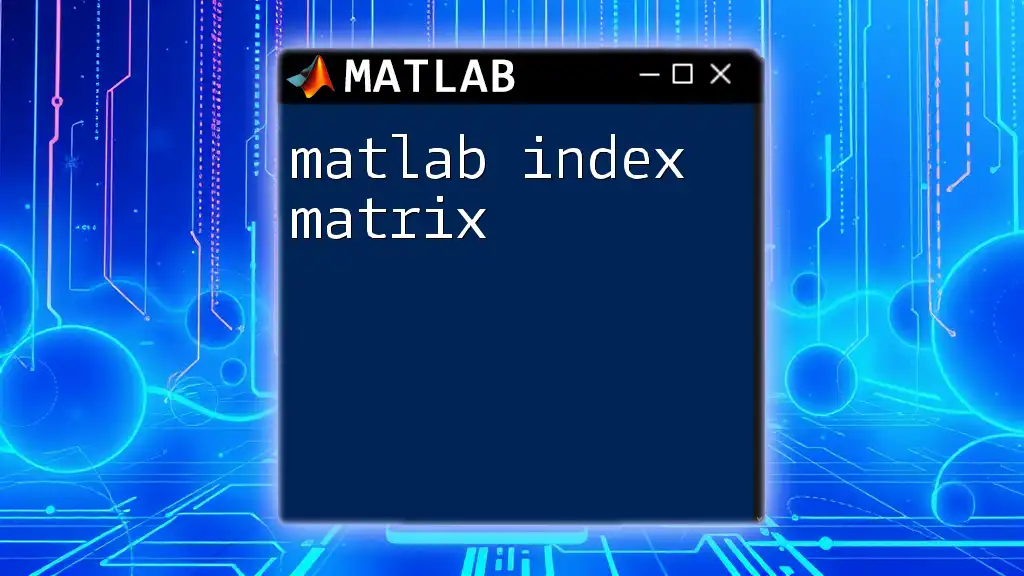
Advanced Index Slicing Techniques
Slicing Multidimensional Arrays
Accessing Rows and Columns
MATLAB's powerful slicing capabilities extend to multidimensional arrays. For example:
B = [1, 2; 3, 4; 5, 6];
row_slice = B(1, :); % Accessing the first row
col_slice = B(:, 2); % Accessing the second column
In this case, `row_slice` will yield `[1, 2]`, while `col_slice` will provide `[2; 4; 6]`. Using `:` is effective for indicating all elements in a respective dimension.
Slicing Higher Dimensional Arrays
When dealing with higher-dimensional arrays, slicing is performed similarly but requires specifying the dimensional indices. For instance:
C = rand(3, 3, 3); % Creating a 3D array
slice_C = C(:,:,2); % Accessing the second matrix in the 3D matrix
In this code, `slice_C` will extract the second 3D slice from the array `C`. This versatility is instrumental in advanced data processing tasks.
Conditional Index Slicing
Conditional slicing is a powerful feature that allows you to extract elements based on specified conditions. For example:
D = [1, 4, 6, 9, 2];
new_slice = D(D > 4); % Accessing elements greater than 4
In this instance, `new_slice` will yield `[6, 9]` as those elements meet the defined condition. This capability is particularly useful in data analysis and filtering operations.
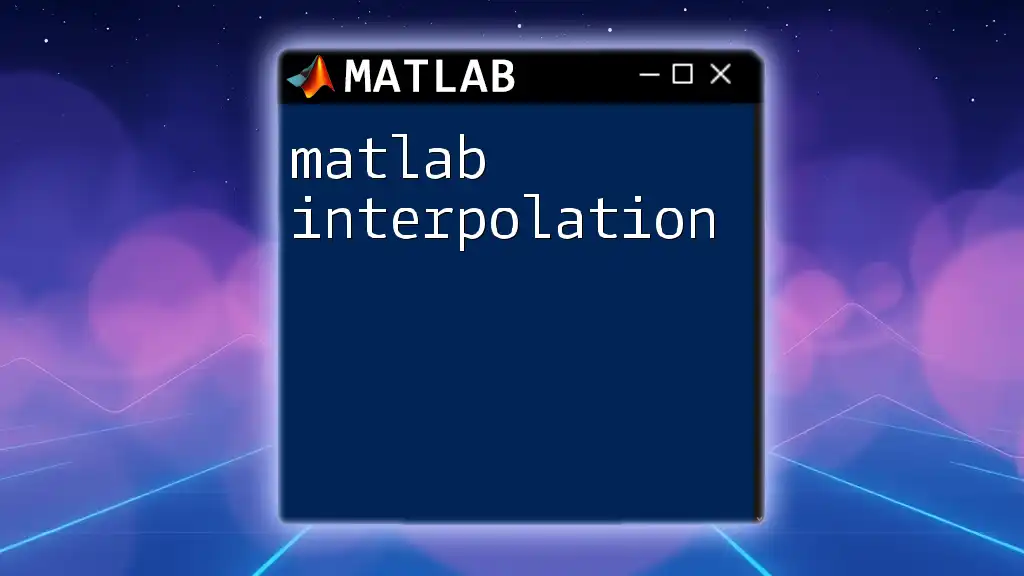
Tips and Best Practices for Index Slicing
Avoiding Common Mistakes
One of the most prevalent mistakes beginners make in MATLAB is off-by-one errors. Remember, MATLAB indices start at 1, not 0. Always verify the dimensions of your array before attempting to slice it to avoid runtime errors.
Performance Considerations
When working with large datasets, slicing can significantly affect performance. To ensure efficient indexing, always use the most specific slices possible and avoid unnecessary copying of large arrays. Instead of creating multiple copies of slices, consider operations that modify data in place when feasible.
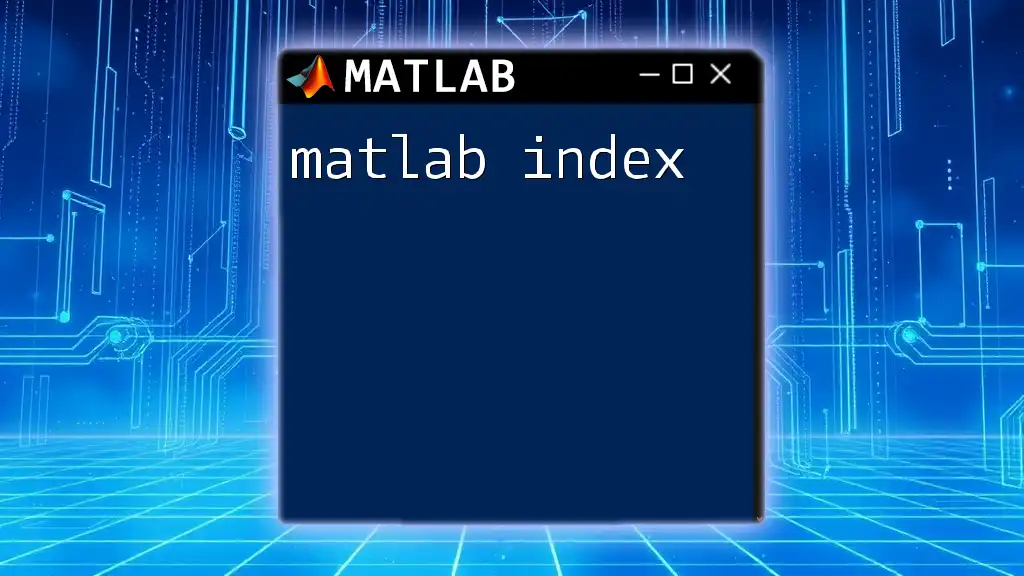
Real-world Applications of Index Slicing
Data Analysis
In data analysis, slicing is essential for preprocessing datasets. For instance, you can use index slicing to extract relevant columns from a larger dataset or to isolate specific rows based on conditions. This process allows for the quick manipulation and analysis of data, providing insights without the need for extensive coding.
Image Processing
In image processing, slices can be used to manipulate specific regions of images. For instance, when applying filters or transformations, you can directly work on a sub-region of an image by slicing it into manageable pieces before reassembling it.
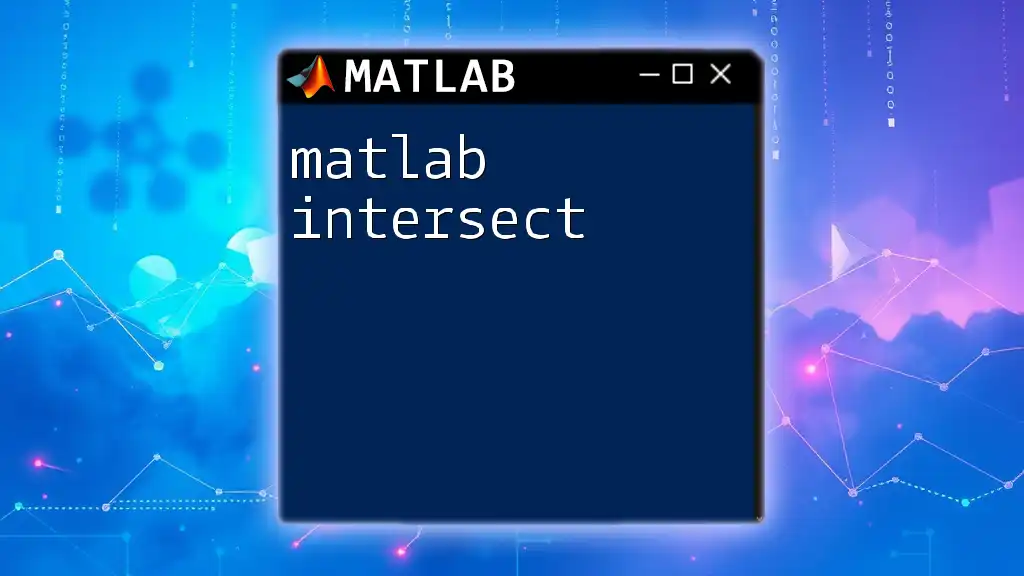
Conclusion
In conclusion, mastering MATLAB index slicing empowers users to handle data with greater efficiency and flexibility. From basic slicing techniques to advanced applications, understanding how to effectively utilize this feature is crucial in a variety of contexts. Dive into practicing these concepts, and explore the incredible capabilities MATLAB offers for data manipulation.
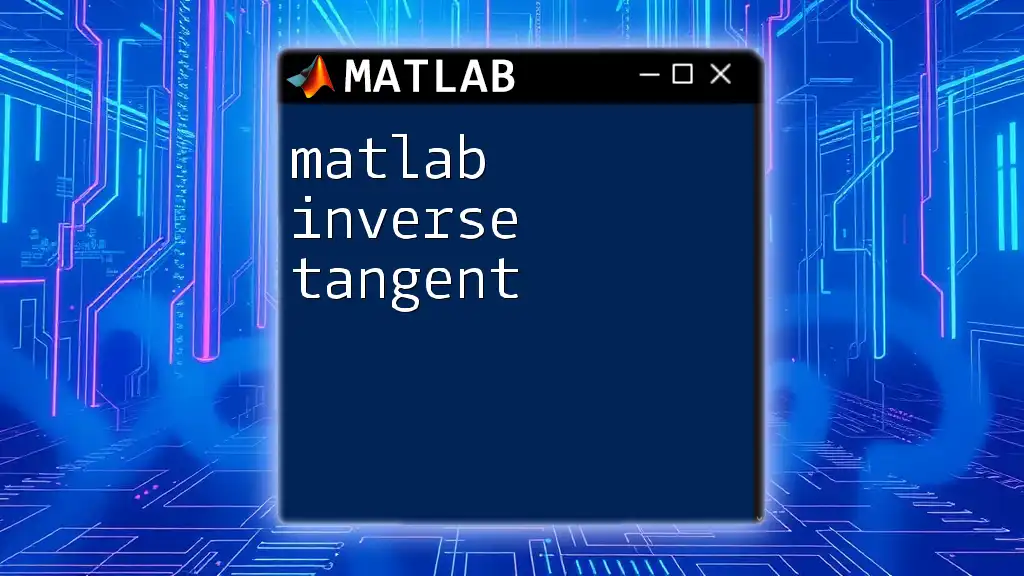
Additional Resources
For further reading and advanced techniques, refer to the official MATLAB documentation and other educational materials on MATLAB programming and index slicing. These resources provide valuable insights into maximizing your efficiency while working with MATLAB.
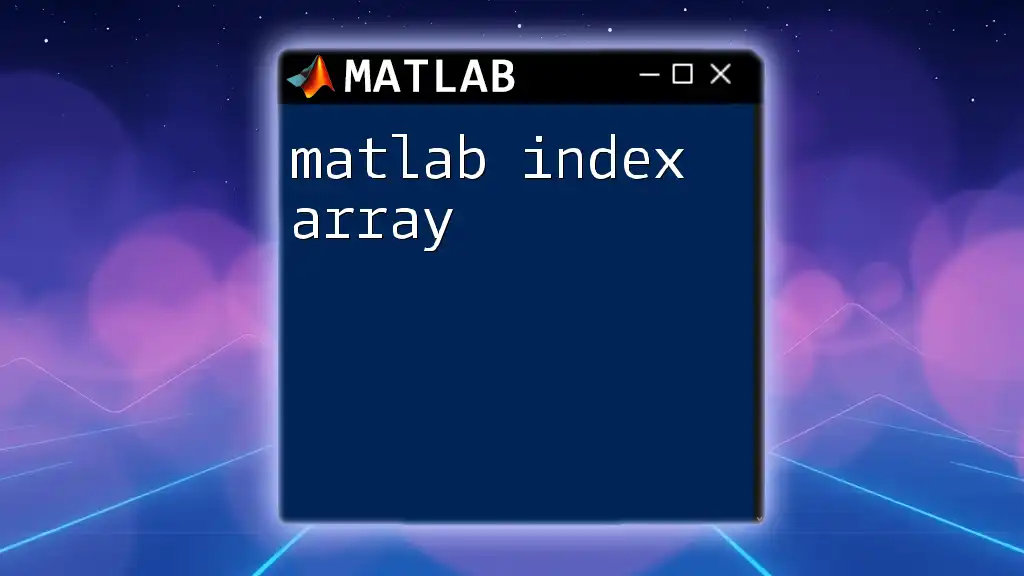
Call to Action
Don’t just read—practice! Explore your datasets using MATLAB index slicing, and consider joining our upcoming workshop for deeper learning and hands-on experience with MATLAB commands. Expand your skills and unlock your potential in data analysis!