A MATLAB structure is a data type that allows you to group related data of different types together using fields, making it easier to manage and organize complex datasets.
Here’s a simple example of how to create and access a MATLAB structure:
% Creating a structure with fields 'name', 'age', and 'grades'
student.name = 'John Doe';
student.age = 21;
student.grades = [90, 85, 88];
% Accessing a field from the structure
disp(student.name); % Output: John Doe
What is a Structure in MATLAB?
A structure in MATLAB is a data type that allows you to group related data of different types under a single variable name. Structures are especially useful for organizing complex data sets. Each piece of data within the structure is stored in a field, and these fields can contain various types of data, including numbers, characters, arrays, or even other structures.
The main benefit of structures is their ability to provide a flexible framework to store heterogeneous data. For example, rather than using multiple variables to store someone's name, age, and job title, you can create a single structure that encapsulates all of this information.
Importance of Structures in Data Organization
Using a MATLAB structure improves data management significantly. Structures allow you to:
- Maintain clarity by grouping related data.
- Enhance readability in complex data management tasks.
- Facilitate easier data manipulation and access.
Structures find utility in many real-world applications, such as in databases, scientific computations, and simulations, where you oftentimes need to handle various types of related information.
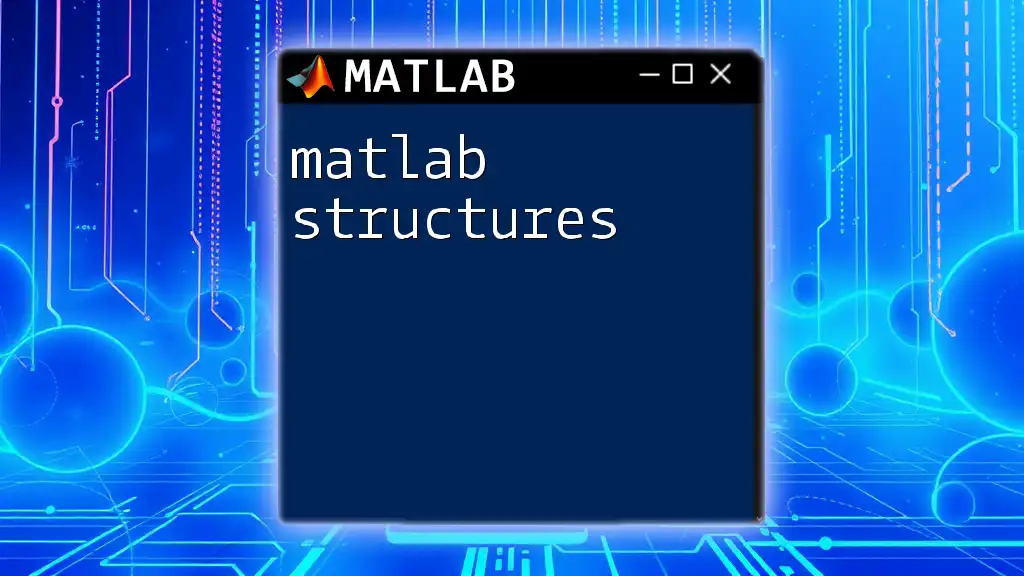
Creating MATLAB Structures
Defining a Structure
To create a structure, you use the following syntax:
structureName.fieldName = value;
For example, to define a structure for a person, you might do the following:
person.name = 'John Doe';
person.age = 30;
person.job = 'Engineer';
In this example, `person` is a structure with three fields: `name`, `age`, and `job`. Each field can hold different types of data, which showcases the primary advantage of using structures.
Initializing Structures with Multiple Fields
You can also create an array of structures, which is particularly useful for representing multiple records. Here’s how to initialize it:
people(1).name = 'Alice';
people(1).age = 28;
people(2).name = 'Bob';
people(2).age = 35;
In this example, `people` is an array of structures, where each element represents a different person. This setup allows easy management of similar data types, making it simpler to perform operations on them collectively.
Accessing Structure Fields
Accessing Individual Fields
To access the value of a specific field, you can use the dot notation:
person.name
This command retrieves the name field from the `person` structure.
Accessing Structure Arrays
For accessing specific fields in structure arrays, you can do the following:
people(1).age
This command accesses the age of the first person in the `people` array. You can also loop through the structure array to access fields for each record efficiently:
for i = 1:length(people)
disp(people(i).name);
end
This loop will display the names of all individuals stored in the `people` structure array.
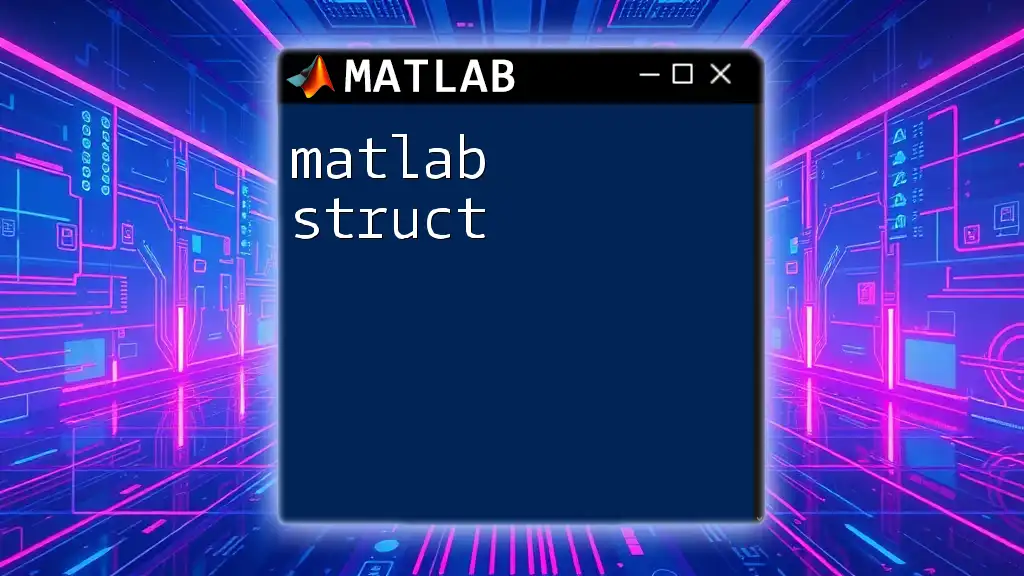
Modifying MATLAB Structures
Adding Fields to a Structure
You can easily add new fields to an existing structure. For example, if you want to add a salary field:
person.salary = 70000;
This command dynamically adds the `salary` field to the `person` structure without requiring an initial definition of this field.
Updating Existing Fields
To update the value of an existing field, use the same dot notation:
person.age = 31;
In this instance, you are changing the age of `person` to 31, showcasing how straightforward it is to modify structure data.
Removing Fields
If you need to remove a field from a structure, you can use the `rmfield` function:
person = rmfield(person, 'job');
After executing this command, the `job` field will be removed from the `person` structure. This function allows you to manage the structure dynamically as your requirements change.
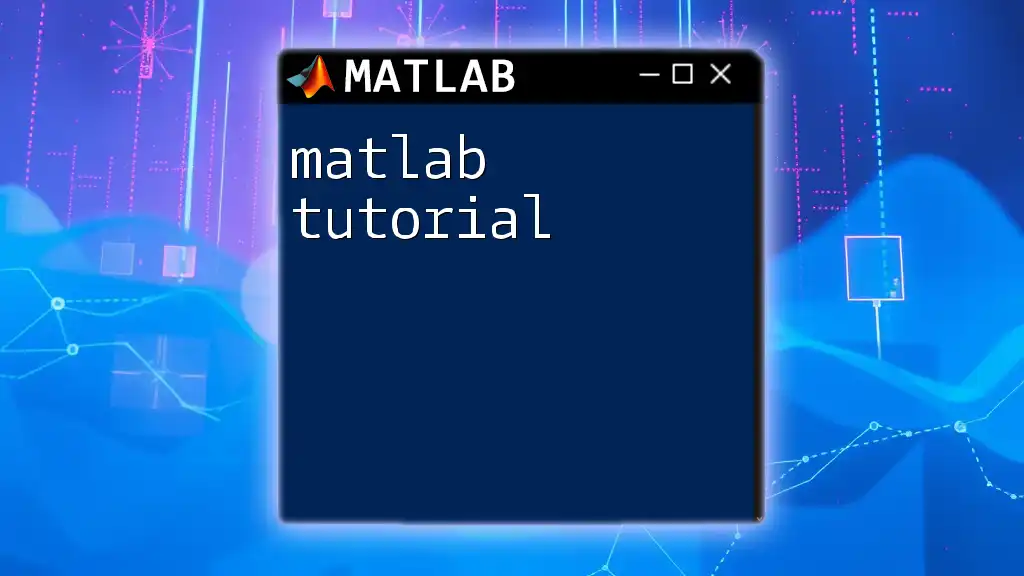
Advanced Use Cases of Structures
Nested Structures
A nested structure is a structure that contains other structures as its fields. For instance, you can create an employee structure that includes an address structure:
employee.name = 'John Doe';
employee.address.city = 'New York';
In this case, `address` is a sub-structure containing additional relevant data about the employee.
Structures and Functions
Structures can be passed to and returned from functions, enhancing modularity in your programming:
function employee = createEmployee(name, salary)
employee.name = name;
employee.salary = salary;
end
This function allows you to neatly create and return employee structures. By encapsulating functionality, you'll create reusable code.
Using Structures with Cell Arrays and Tables
Structures can also be integrated with cell arrays and tables in MATLAB, which can be especially useful for handling mixed data types. Understanding the distinctions between these data formats can significantly enhance your ability to manipulate data in MATLAB.
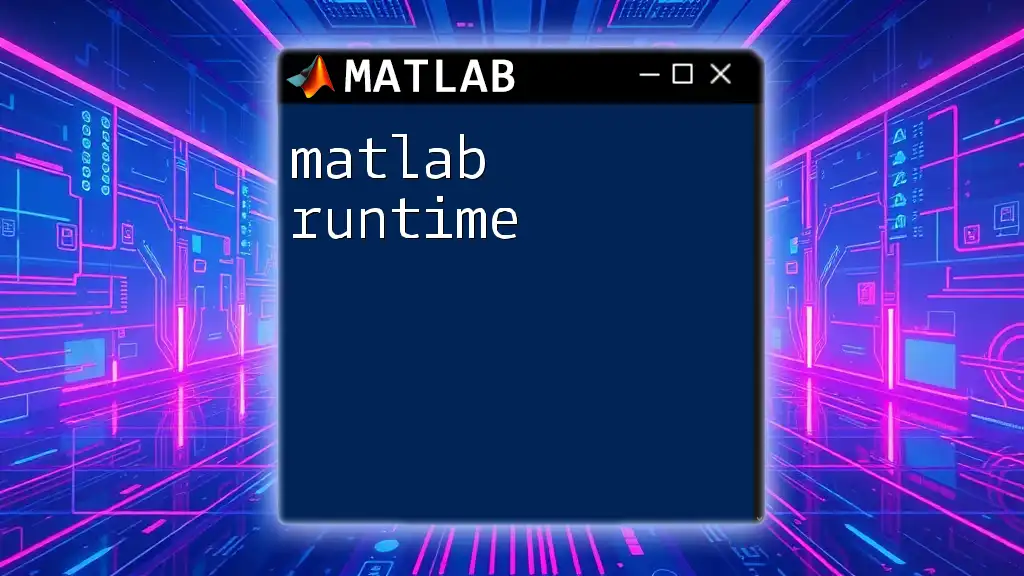
Common Operations on Structures
Looping Through Structures
You can loop through each structure within an array of structures as shown previously. This allows you to perform operations on each record efficiently. The syntax is easy to implement and makes it clear how data is structured within MATLAB.
Applying Functions to Structure Fields
You can use functions like `arrayfun` to apply operations over fields in a structure array. For instance, to collect ages from an array of individuals:
ages = arrayfun(@(x) x.age, people);
This command will return an array containing the ages of all people listed in the structure array, demonstrating the power of structures in data manipulation.
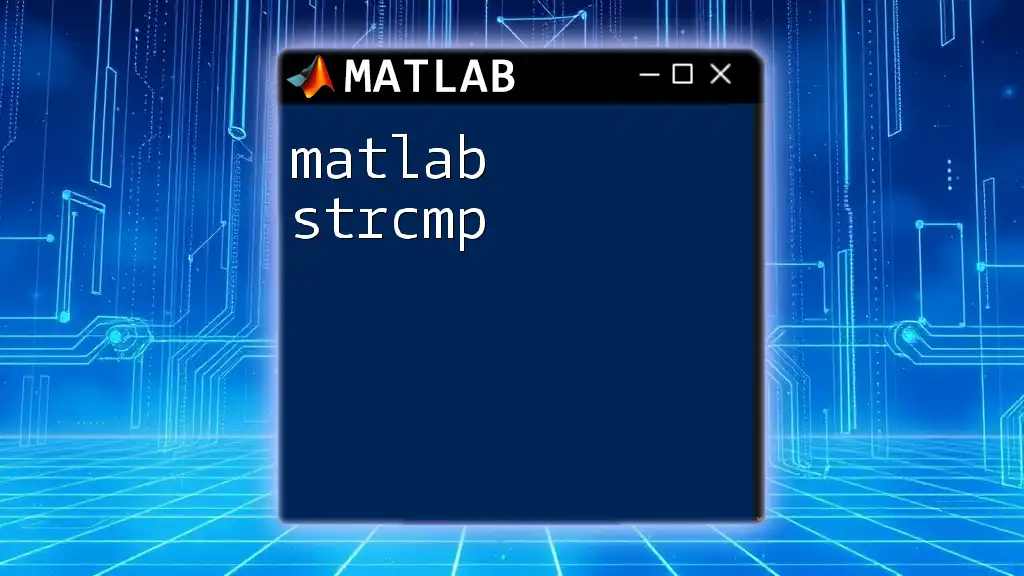
Best Practices for Using Structures in MATLAB
When to Use Structures
Structures are best utilized when you need to maintain clarity and organization in your data management. They are particularly effective when you need to manage a collection of records with varying data types.
Tips for Efficient Structure Management
- Organize your structures logically. Use meaningful field names to make them self-descriptive.
- Keep documentation clear and updated, making it easy to understand the structure of your data over time.
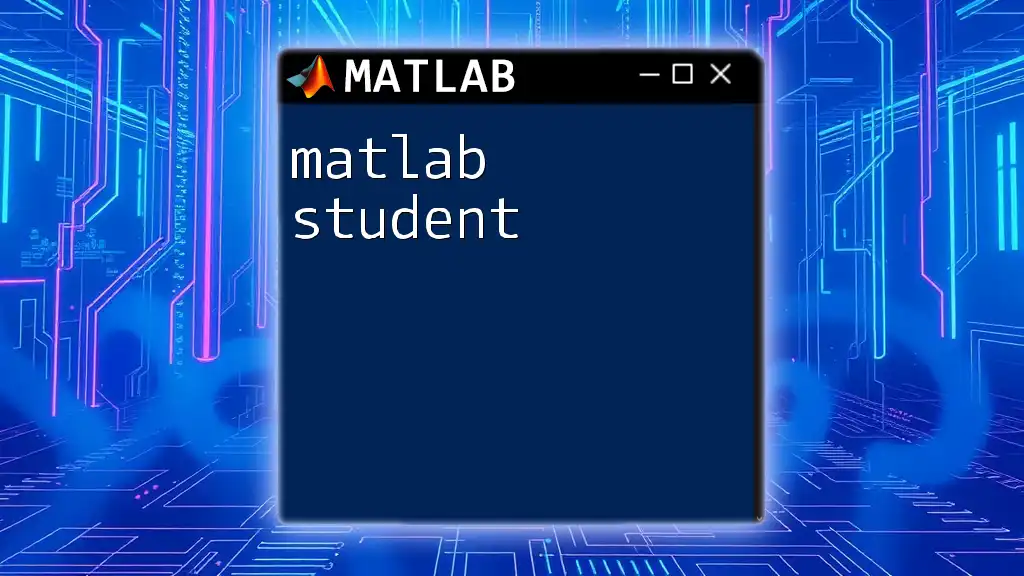
Conclusion
In summary, MATLAB structures offer a versatile approach to organizing data in a concise and manageable way. By leveraging structures, you stand to gain clarity in your programming, making it easier to understand and manipulate your data. As you practice, consider the multitude of ways structures can enhance your coding efficiency. Don't hesitate to explore the vast capabilities that structures provide, and remember, the more you practice, the more adept you'll become at data management in MATLAB!
Additional Resources
For further reading and exploration, check out MATLAB's official documentation on structures, and consider reinforcing your knowledge through online video tutorials and courses focused on MATLAB structures to solidify your understanding.