MATLAB structures are data types that allow you to group related data of varying types under a single variable name, making it easier to organize and access complex data sets.
% Create a structure with fields for name, age, and height
person.name = 'John Doe';
person.age = 30;
person.height = 5.9;
Introduction to MATLAB Structures
MATLAB structures are data types that allow you to group related data using data containers called fields. Each field can store different types of data, making structures ideal for organizing complex data sets. The power of structures in MATLAB lies in their ability to encapsulate heterogeneous data, allowing for better data management and easier access.
The benefits of using structures are numerous. They provide a simple and organized approach to handle multiple data items as a single unit, which greatly enhances code readability and maintainability.
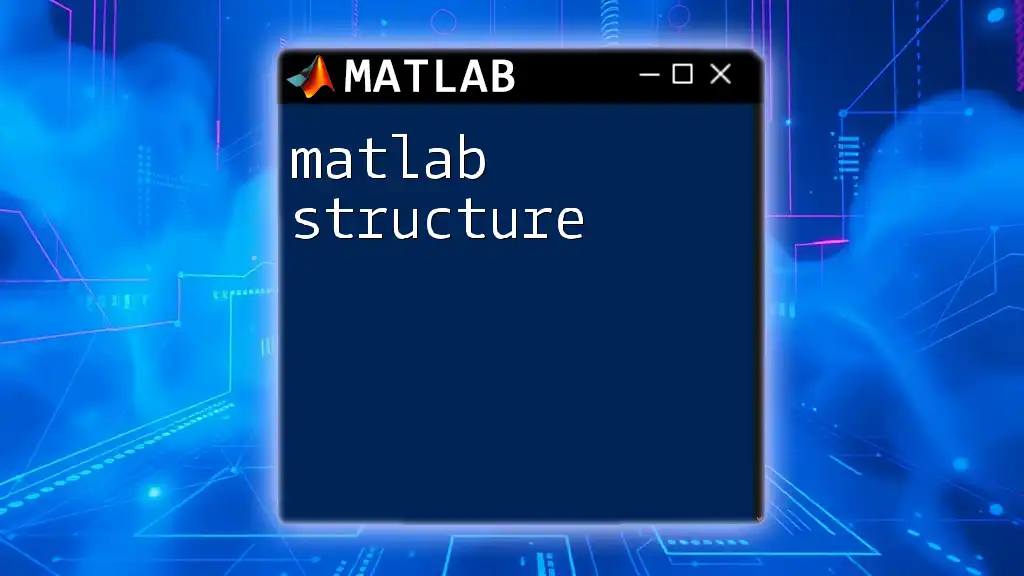
Creating MATLAB Structures
Defining Structures
You can create structures using the `struct` function. The basic syntax follows this format:
myStructure = struct('Field1', value1, 'Field2', value2);
For instance, if you want to create a structure for a student with fields for name and age, it would look like this:
student = struct('Name', 'John Doe', 'Age', 20);
Each field can hold different types of data, such as strings, numbers, arrays, or even other structures.
Initializing Fields
Once you have created a structure, you can initialize its fields post-creation. For example, creating an empty structure can be done as follows:
emptyStruct = struct();
You can then add fields individually:
emptyStruct.Field1 = 'First Value';
emptyStruct.Field2 = 42;
This step allows for flexibility in your structure design, letting you define the contents dynamically.
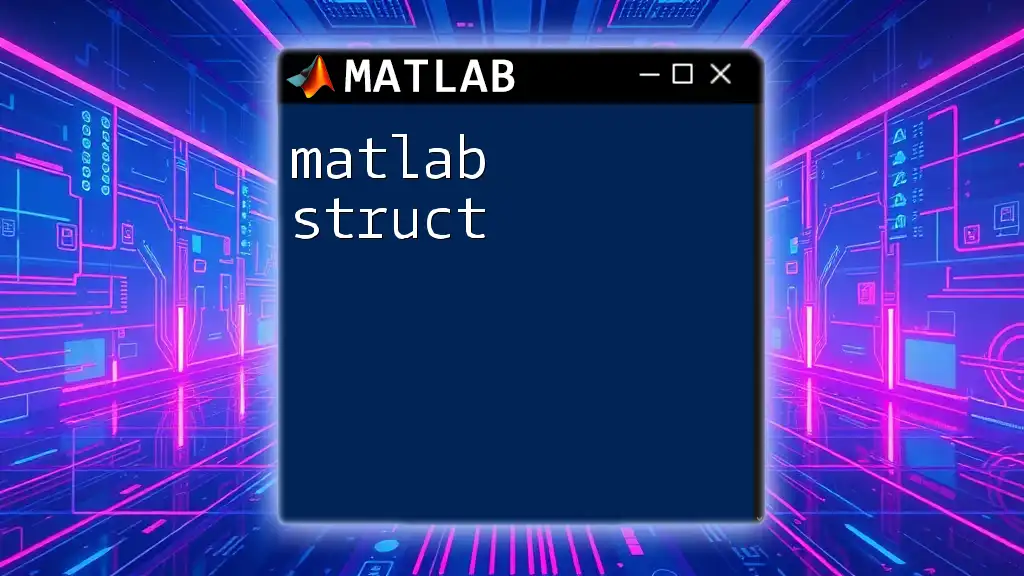
Accessing Structure Data
Accessing Fields
To retrieve data from a structure, you utilize dot notation. For example:
name = student.Name;
Here, the variable `name` will hold the value `'John Doe'`. This method of access is intuitive and provides clarity when working with multiple fields in a structure.
Modifying Structure Fields
Updating values in a structure is straightforward. Simply refer to the field you wish to change:
student.Age = 21;
After executing this code, the `Age` field of `student` is updated to `21`. This ease of modification contributes to the adaptability of structures in your workspace.
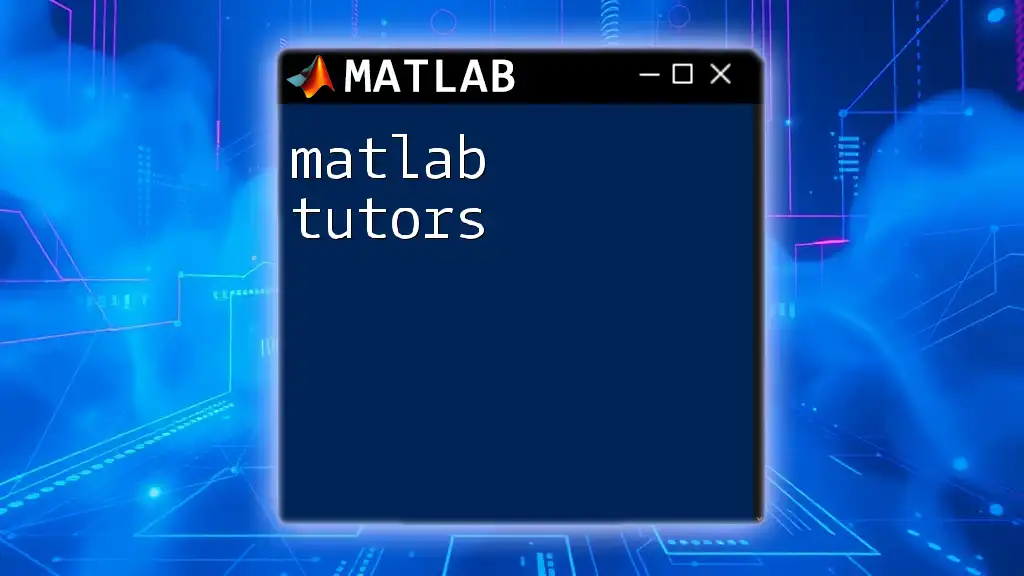
Nested Structures
What are Nested Structures?
Nested structures are structures that contain other structures as their fields. This feature allows you to create complex data representations, mimicking real-world relationships. For instance, if you want to include contact information with nested addresses, you may set it up like this:
contact = struct('Name', 'John Doe', 'Address', struct('Street', '123 Main St', 'City', 'Hometown'));
Creating Nested Structures
When creating nested structures, make sure to clearly define each layer. Here's an example that outlines a nested structure with students and their courses:
course1 = struct('CourseName', 'Mathematics', 'Credits', 3);
course2 = struct('CourseName', 'Science', 'Credits', 4);
student = struct('Name', 'John Doe', 'Courses', [course1, course2]);
This organization allows seamless management of more intricate data types while maintaining data integrity.
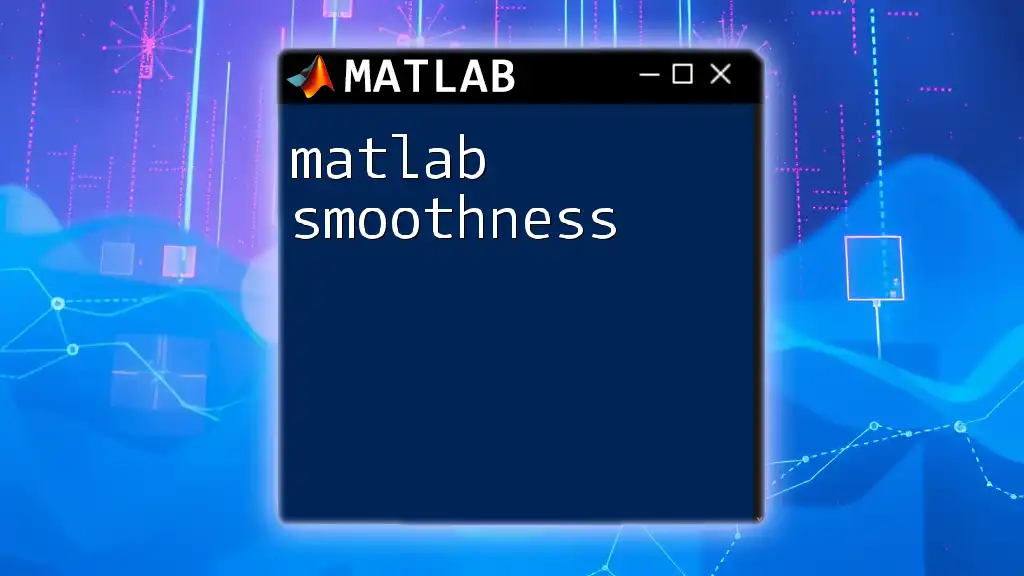
Array of Structures
Creating an Array of Structures
You can leverage the power of arrays by creating an array of structures, which is useful for grouping multiple entries of similar data types. Here’s how you can do this:
studentArray = struct('Name', {'Alice', 'Bob', 'Charlie'}, 'Age', {22, 23, 21});
In this example, `studentArray` holds three different student records, making it easier to loop through and manage similar data easily.
Accessing Array Elements
To access specific elements within a structure array, you can use indexing:
firstStudentName = studentArray(1).Name;
This will store `'Alice'` in the variable `firstStudentName`. Using MATLAB's indexing capabilities in combination with structures allows for efficient retrieval and manipulation of data.
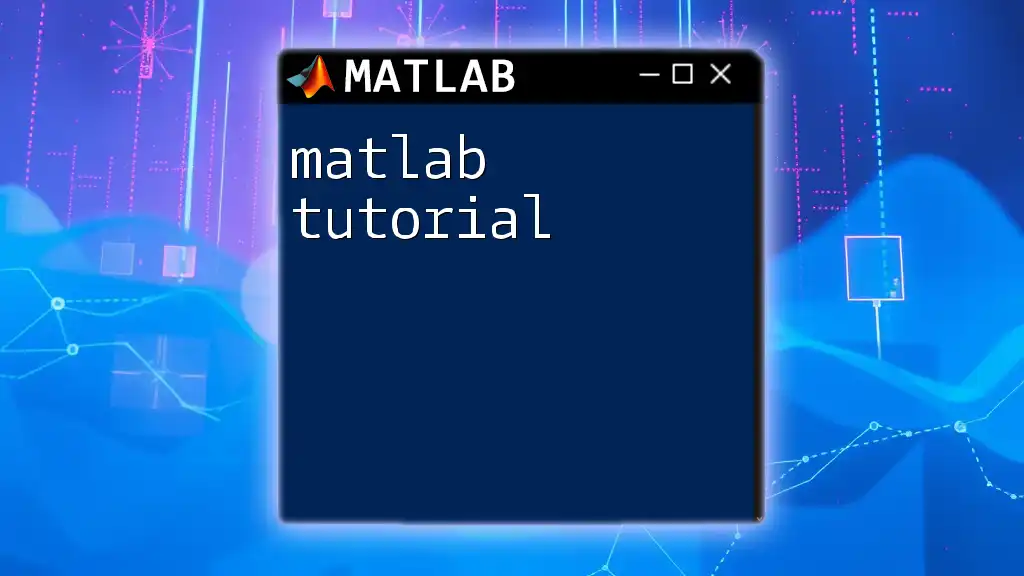
Operations on Structures
Concatenation
MATLAB allows you to concatenate structures using the `cat` function. This can be particularly useful when you want to combine multiple structure datasets:
combinedStructures = cat(1, studentArray, newStudentsArray);
This method helps merge related data into a single, more manageable structure.
Field Names and Struct Function
To further understand the fields in a structure, you can retrieve the field names using the `fieldnames` function:
fieldNames = fieldnames(student);
This command will return a list of field names contained within the `student` structure, assisting in better structure data management.
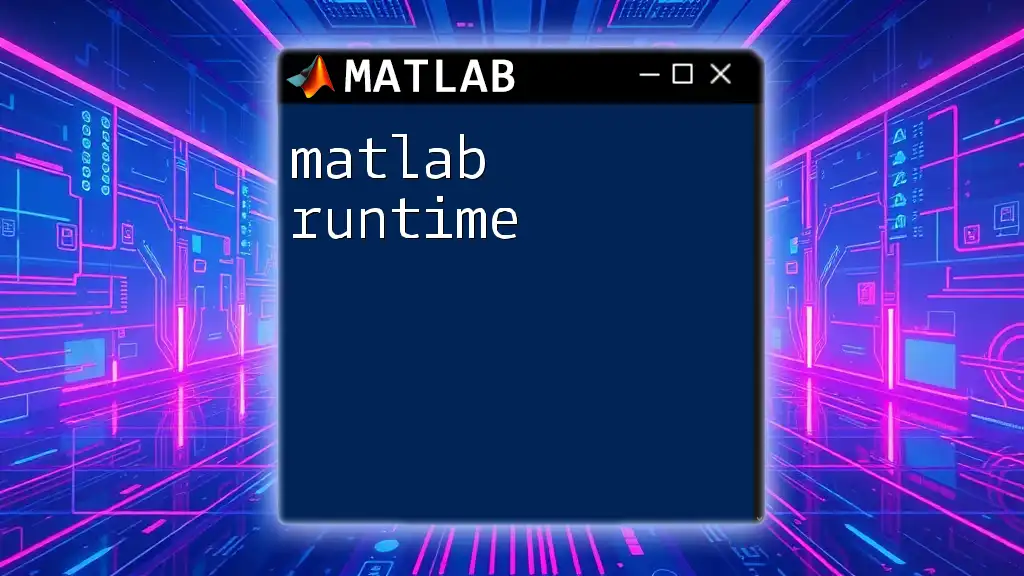
Functionality with Structures
Passing Structures to Functions
You can define functions that utilize structures as inputs. The following code demonstrates this concept:
function displayStudentInfo(s)
fprintf('Name: %s, Age: %d\n', s.Name, s.Age);
end
Calling this function with `displayStudentInfo(student)` will display the student's name and age, enhancing modularity in your code.
Returning Structures from Functions
You may also design functions to return structures. For instance, consider a function that creates and returns a structure for a new student:
function newStudent = createStudent(name, age)
newStudent = struct('Name', name, 'Age', age);
end
When you call `newStudent = createStudent('David', 24);`, the function creates a structured record for David, illustrating the effective reuse of structure templates.
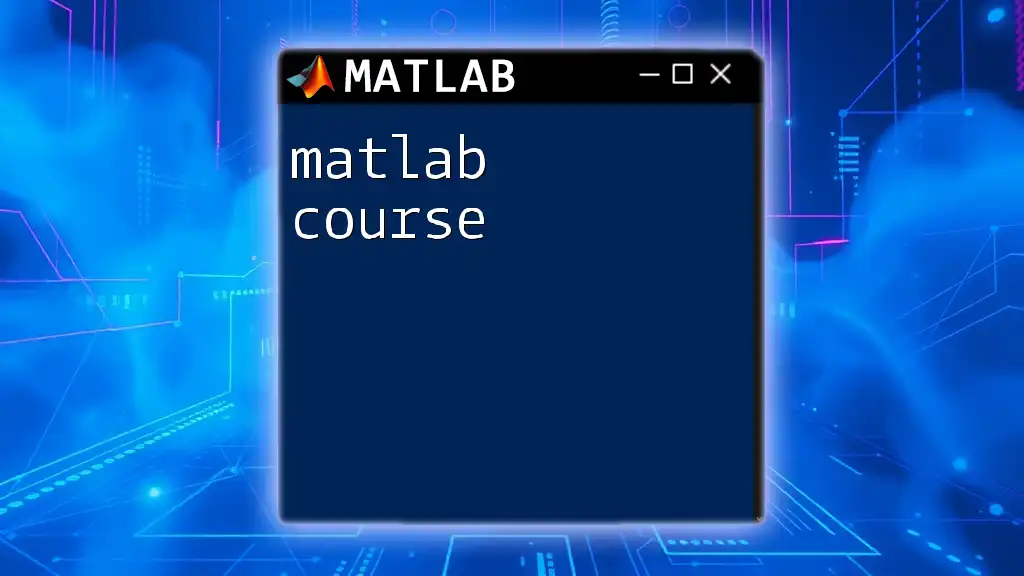
Best Practices for Using Structures
When to Use Structures
Using structures is particularly beneficial when you have related information that needs to be grouped for accessibility. Ideal scenarios include:
- Grouping database entries.
- Managing datasets where items have differing attributes but share some common information.
Common Pitfalls
Despite their flexibility, one must be cautious of common pitfalls such as:
- Field type mismatches: Ensure you maintain consistency in the data types stored within fields.
- Forgetting to preallocate: Avoid dynamically growing structures in loops, as this could lead to performance degradation. Preallocating space when possible is advisable.
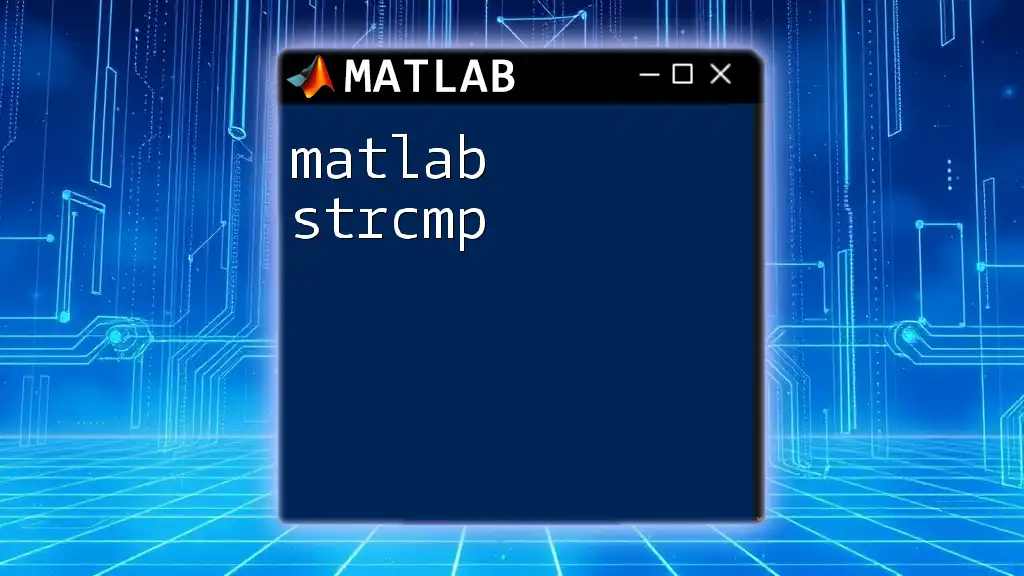
Conclusion
In this comprehensive exploration of MATLAB structures, you have learned how to create, access, and manipulate these powerful data types. Their versatility allows for organized data management and efficient coding practices crucial for any MATLAB user. Remember to implement best practices to avoid common pitfalls as you grow in your understanding and application of structures in MATLAB.
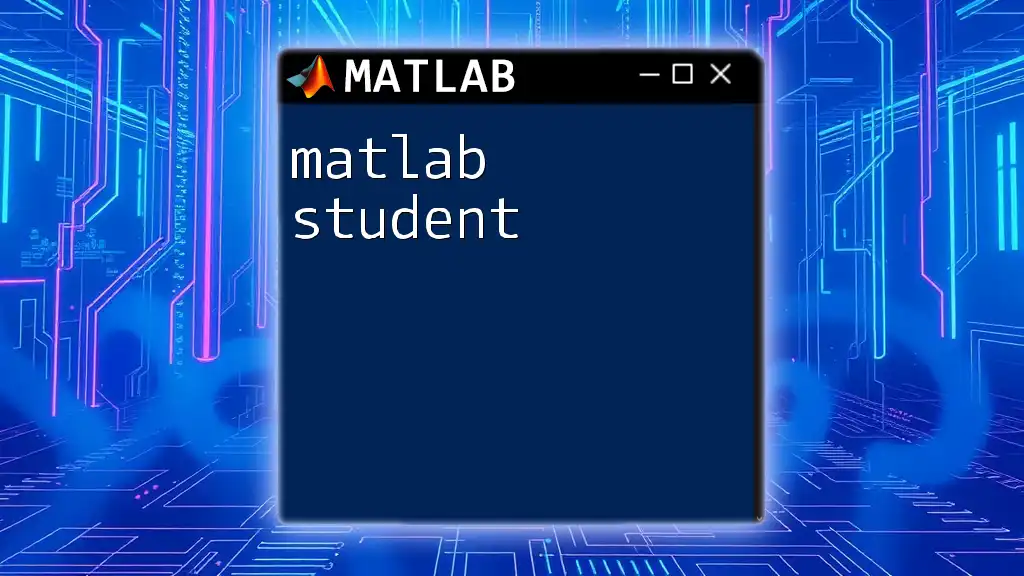
Additional Resources
For further exploration, consult the official MATLAB documentation regarding structures or seek out tutorials that take a deep dive into advanced usage scenarios. Hands-on practice is crucial to mastering structures, so don’t hesitate to engage with example datasets and exercises to strengthen your skills.
Code Snippet Gallery
Here, you can find a collection of essential code snippets you can reference as you work with MATLAB structures:
% Create a basic structure
basicStruct = struct('Field1', 10, 'Field2', 'Hello');
% Accessing a field
value = basicStruct.Field1;
% Modifying a field
basicStruct.Field2 = 'World';
% Nested structure example
nestedStruct = struct('OuterField', 'OuterValue', 'InnerStruct', struct('InnerField1', 100));
Feel free to use these snippets as a quick reference to streamline your work with MATLAB structures.