In MATLAB, normalization is the process of adjusting the values in a dataset to a common scale, often to improve the convergence of algorithms or to prepare the data for analysis.
Here's a code snippet to normalize an array in MATLAB:
data = [4, 2, 8, 6]; % Original data
normalized_data = (data - min(data)) / (max(data) - min(data)); % Normalization
What is Normalization?
Definition of Normalization
Normalization is the process of adjusting values in a dataset to a common scale. This operation transforms the data so that it fits within a specific range or distribution. The primary goal is to make different features comparable, especially when they are measured in different units or have different scales. Unlike raw numbers, normalized data helps to eliminate biases that can skew results in data analysis and modeling.
Why is Normalization Important?
Normalization plays a crucial role in data preprocessing, particularly when working with algorithms sensitive to the scale of input data. Here are some important reasons why normalization should be considered:
-
Creating a Level Playing Field: Normalization ensures all features contribute equally to the distance computations and gradient descent-based optimizations.
-
Impact on Algorithms: Many machine learning algorithms (e.g., k-nearest neighbors, gradient descent) require normalized input to perform well. If the data is not normalized, features with larger ranges may disproportionately influence the model’s predictions.
-
Use Cases Across Domains: Normalization is essential in various fields, including machine learning, computer vision, and statistical modeling. For instance, in image processing, pixel values need normalization to enhance image quality.
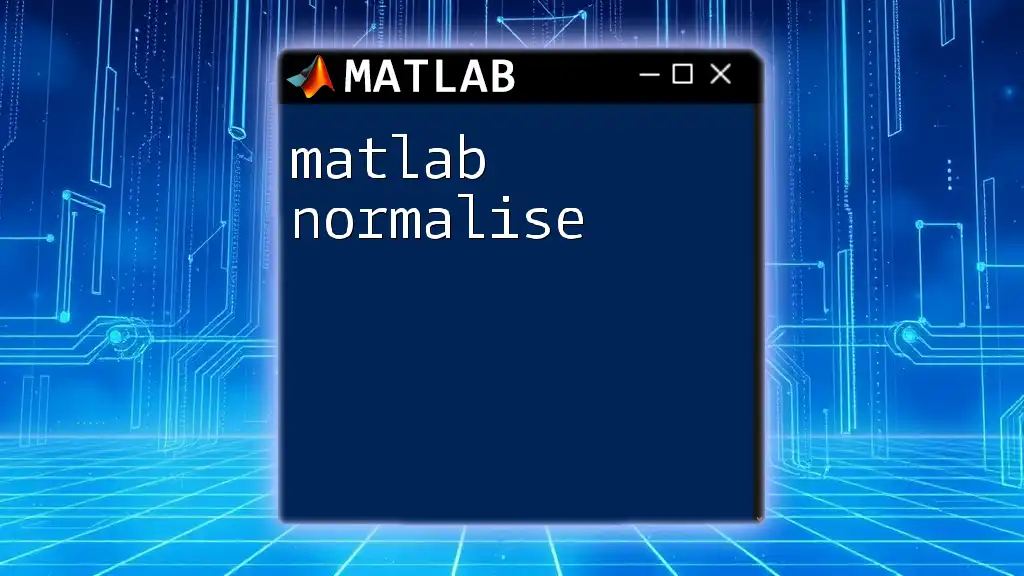
Types of Normalization Techniques
Min-Max Normalization
Min-Max Normalization rescales the features to a specific range, typically [0, 1]. This method is useful when you want to maintain the relational characteristics of the original data.
Mathematical Formula: \[ x_{\text{norm}} = \frac{x - \text{min}(X)}{\text{max}(X) - \text{min}(X)} \]
MATLAB Implementation:
function normalizedData = minMaxNormalization(data)
normalizedData = (data - min(data))./(max(data) - min(data));
end
Example: Consider a dataset with values ranging from 10 to 100. After applying min-max normalization, a value of 50 would be transformed to 0.5 when scaled to the range [0, 1].
Z-Score Normalization (Standardization)
Z-Score Normalization, also known as standardization, transforms data to have a mean of 0 and a standard deviation of 1. This technique is beneficial when data follows a Gaussian distribution.
Mathematical Formula: \[ z = \frac{x - \mu}{\sigma} \]
MATLAB Implementation:
function standardizedData = zScoreNormalization(data)
standardizedData = (data - mean(data)) / std(data);
end
Example: Applying z-score normalization to a dataset with a mean of 50 and a standard deviation of 10 would transform a value of 60 into 1, indicating it’s one standard deviation above the mean.
Robust Scaler Normalization
Robust Scaler Normalization utilizes robust statistics, making it less sensitive to outliers by using the median and the interquartile range (IQR).
MATLAB Implementation:
function robustNormalizedData = robustScaler(data)
med = median(data);
iqr_value = iqr(data);
robustNormalizedData = (data - med) / iqr_value;
end
Example: If a dataset contains extreme outliers, traditional normalization methods may yield biased results. Using robust scaling, the impact of these outliers on the normalized values is minimized.
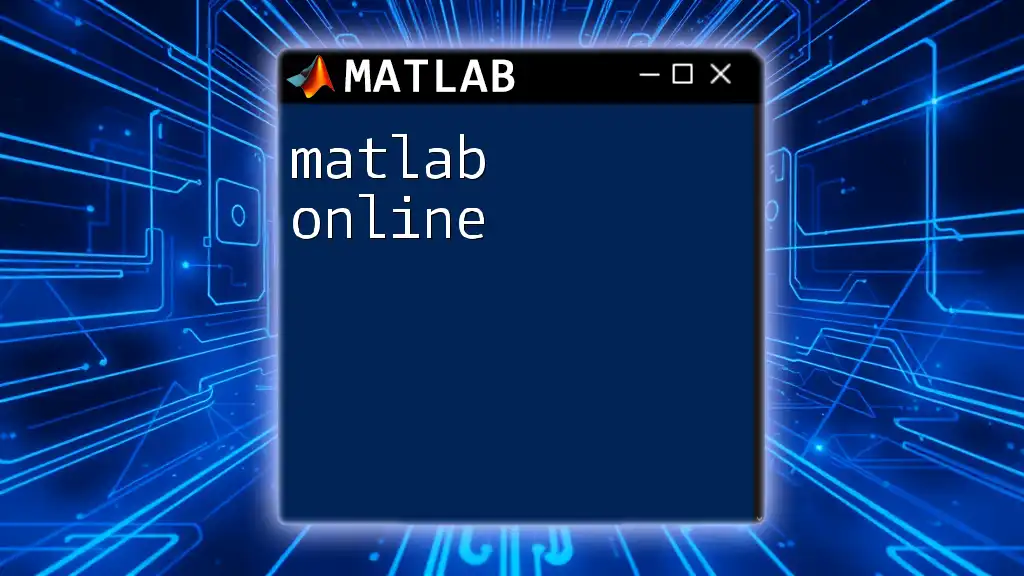
How to Normalize Data in MATLAB
Built-in Functions for Normalization
MATLAB provides several built-in functions for data normalization, one of the most useful being the `normalize` function. This function accommodates various normalization methods based on the user’s needs.
Example of using the `normalize` function:
data = rand(10, 3); % Sample data
normalizedData = normalize(data);
Step-by-Step Guide for Normalizing Data
-
Load Your Data
Utilizing MATLAB's data import functions is essential:
data = readmatrix('data.csv');
-
Choose the Normalization Method
Decide which normalization technique suits your analysis goals. Consider the nature of your data and any potential outliers.
-
Implement the Normalization
Based on your chosen method, implement the respective MATLAB function. For example, if opting for z-score normalization:
normalizedData = zScoreNormalization(data);
-
Visualizing Normalized Data
Visual aids can further enhance understanding. Use MATLAB's plotting functions, such as boxplots, to visualize the effects of normalization:
boxplot(data);
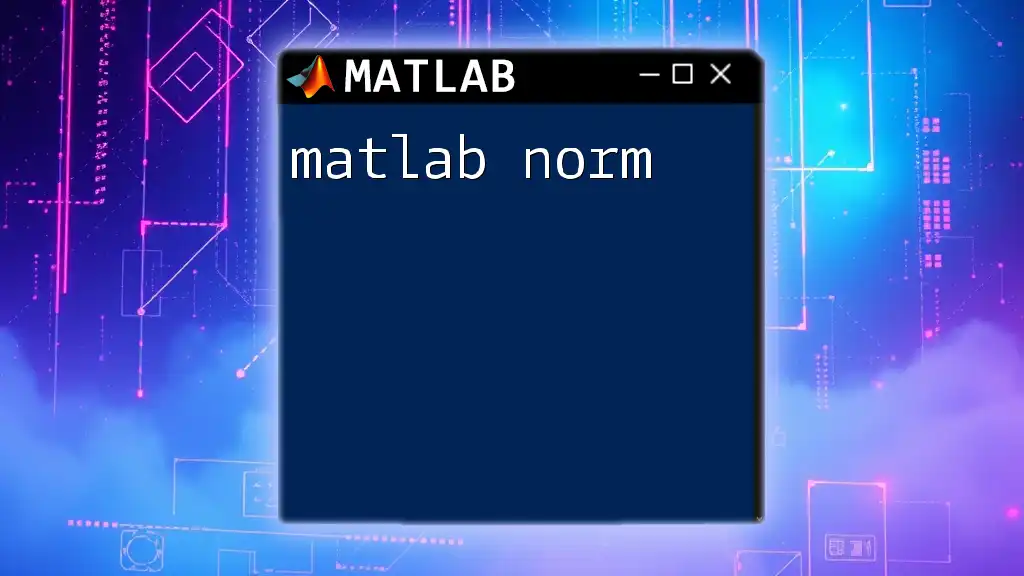
Best Practices for Normalization
When to Normalize
Normalization should be applied when preparing data for machine learning models, particularly in scenarios where features are on different scales. Always consider applying normalization before training the model to ensure optimal performance.
Potential Pitfalls
While normalization is advantageous, there are some pitfalls to navigate:
-
Over-Normalization: Be cautious of situations where normalization might overcomplicate the interpretability of your data.
-
Loss of Interpretability: In certain analyses, original data scales might hold significant meaning; normalizing could obscure this information.
-
Dealing with Sparse Data: Sparse datasets might present challenges in effective normalization. Continuous evaluation is necessary.
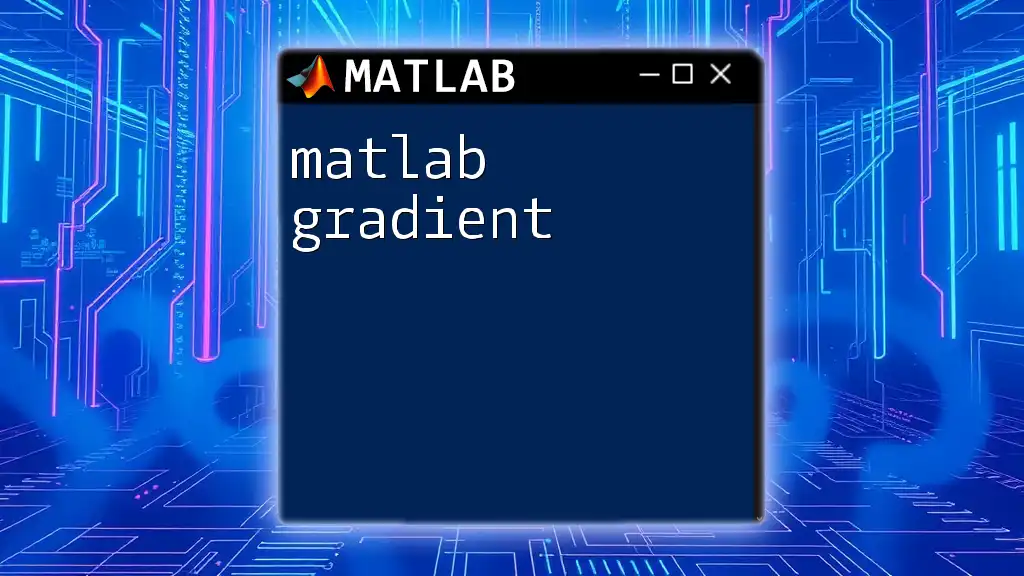
Conclusion
The process of normalization in MATLAB is a fundamental skill that empowers data analysts and scientists to create effective, robust datasets for their projects. Understanding different normalization techniques, their implementations, and their importance can significantly influence data analysis and modeling outcomes. Regular practice with these methods will enhance your proficiency and confidence in working with MATLAB. Remember, a well-normalized dataset not only improves algorithm performance but also leads to clearer insights and more informed decision-making.