The `imresize` function in MATLAB is used to change the size of an image by specifying the new dimensions or a scaling factor.
Here’s a simple example of using `imresize` in MATLAB:
% Read an image
img = imread('example.jpg');
% Resize the image to half its original size
resizedImg = imresize(img, 0.5);
% Display the resized image
imshow(resizedImg);
Understanding the Syntax of `imresize`
Basic Syntax
The basic syntax of the `imresize` function in MATLAB is straightforward. You use it to change the size of an image A by a specific scale factor:
B = imresize(A, scale)
In this command:
- A: This is your input image, which can be in various formats such as grayscale, RGB, or binary.
- scale: A numeric value that determines how much you want to resize the image. If you set `scale` to 0.5, the image will become half of its original size. Conversely, if set to 2, it will double in size.
Additional Syntax Options
You can also specify target dimensions directly by using a two-element vector representing the desired number of rows and columns. The syntax looks like this:
B = imresize(A, [numRows numCols])
This allows for precise control over the output dimensions rather than relying solely on scale factors.
Moreover, `imresize` supports additional parameters that can significantly enhance the output quality:
B = imresize(A, scale, 'method', interpolationMethod)
Here, `method` refers to the interpolation technique you want to use. This flexibility is crucial for achieving the best visual quality depending on your project's needs.
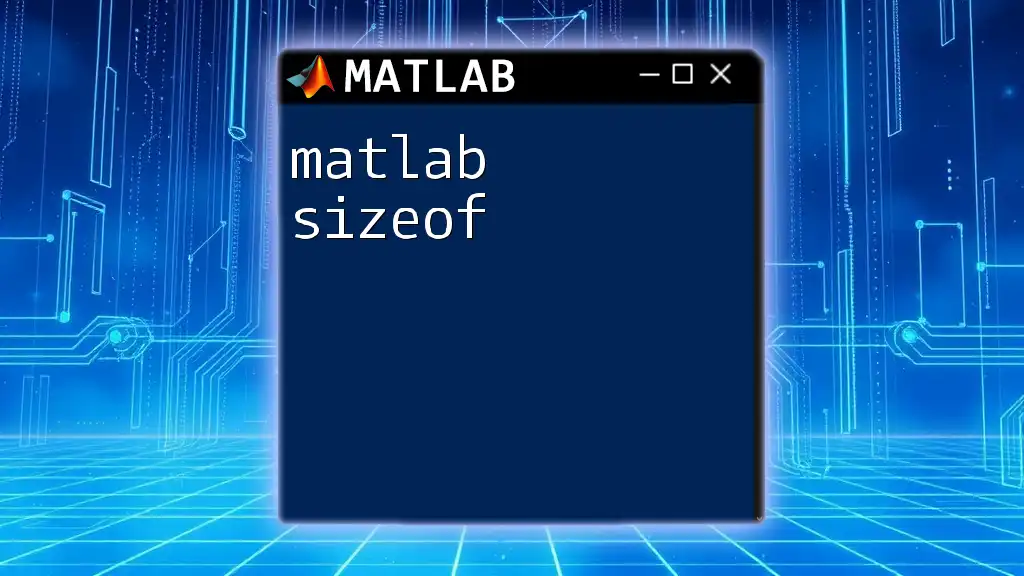
Resizing Techniques
Interpolation Methods
Interpolation is a mathematical technique that estimates new pixel values for the resized image. The selection of interpolation method can affect the quality of the output image significantly.
-
'nearest': The simplest method, which assigns the value of the nearest pixel. This technique is fast but can produce blocky results.
-
'bilinear': This method takes the average of the four closest pixel values, creating smoother images, especially when enlarging.
-
'bicubic': A more advanced technique that considers the sixteen nearest pixels. This method usually results in the smoothest and highest quality images.
When to use each method depends on the specific requirements of your project; for faster processing where quality isn't a primary concern, use 'nearest'. For most general applications, 'bicubic' is often the best choice due to its high-quality output.
Antialiasing in `imresize`
Antialiasing is a technique used to reduce artifacts and jagged edges in images when resizing, especially during downscale operations. Activating antialiasing will help in smoothing out the edges, providing a more visually appealing result.
You can enable it in your `imresize` command as follows:
B = imresize(A, scale, 'Antialiasing', true)
Using antialiasing can be particularly important when reducing image size, as it helps maintain the integrity of the visual components.
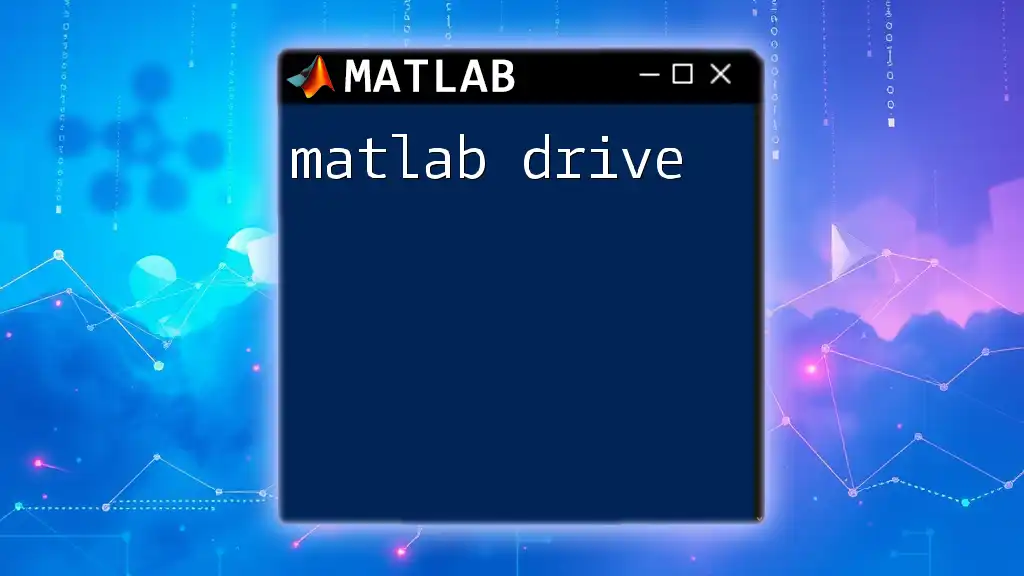
Practical Examples of `imresize`
Example 1: Basic Image Resizing
Let's start with the simplest example of resizing an image by a scale factor:
A = imread('image.jpg'); % Read the image
B = imresize(A, 0.5); % Resize to half its original size
imshow(B); % Display the resized image
In this snippet, we read an image file called 'image.jpg', resize it to half its original dimensions, and then display the resized output. This is a common operation in preparing images for web use or applications requiring smaller file sizes.
Example 2: Resizing to Specific Dimensions
When you need to resize an image to specific dimensions, this can be easily done:
targetSize = [256 256]; % Desired size
B = imresize(A, targetSize); % Resize the image to 256 by 256 pixels
imshow(B); % Display the resized image
This command directly sets the resized image to exactly 256 rows and 256 columns. It’s vital to note that resizing without maintaining the aspect ratio can lead to distorted images. So, always consider the aspect ratio based on your needs.
Example 3: Advanced Interpolation Methods
When working with high-quality images, you may want to experiment with different interpolation methods. Here's how you can compare two methods:
B_bilinear = imresize(A, 2, 'bilinear'); % Resize with bilinear interpolation
B_bicubic = imresize(A, 2, 'bicubic'); % Resize with bicubic interpolation
imshow(B_bilinear); % Display the bilinear resized image
imshow(B_bicubic); % Display the bicubic resized image
This example compares the bilinear and bicubic methods when scaling the image by a factor of 2. You will notice that while both can produce satisfactory results, 'bicubic' generally yields a smoother output.
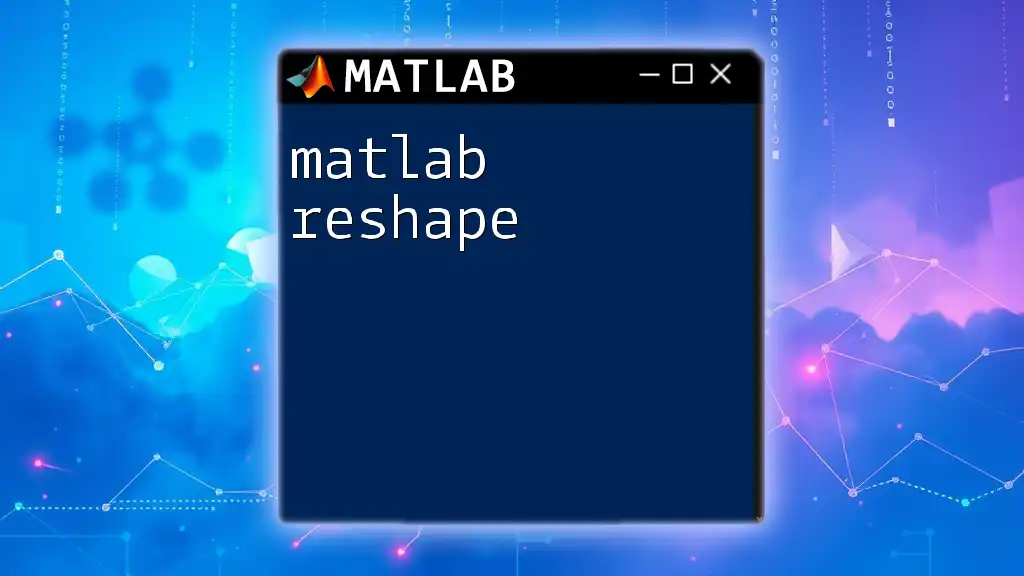
Common Pitfalls and How to Avoid Them
Misunderstanding Scale Factors
One common issue when using `imresize` is misjudging the scale factor. Developers often unintentionally set a scale that disrupts the intended presentation of their image. Always ensure that you understand whether you are applying a reduction or enlargement!
Choosing the Right Interpolation Method
Selecting the correct interpolation method can often lead to unexpected results, especially if the wrong method is chosen. For example, using 'nearest' for scaling up images can make them appear pixelated. A careful evaluation of the image content and project requirements can guide you in choosing the most appropriate method.
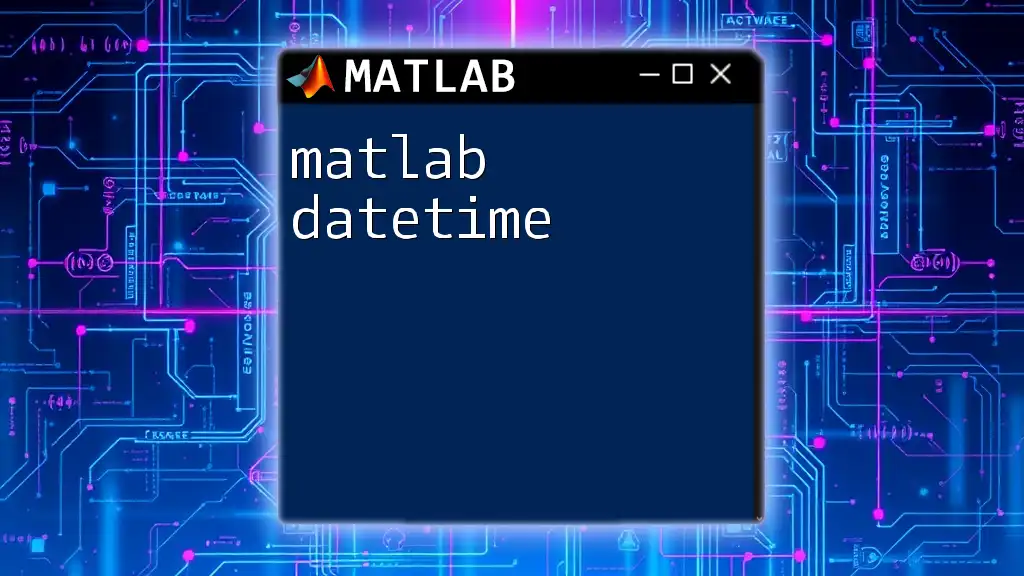
Optimizing Image Quality after Resizing
Assessing Image Quality
After resizing your image, it is crucial to evaluate its quality. While visual inspection is an intuitive approach, it may be beneficial to use quantitative metrics such as Peak Signal-to-Noise Ratio (PSNR) or Structural Similarity Index (SSIM) to objectively assess changes in quality.
Recommended Practices
To optimize your resizing workflow, consider the following practices:
- Preserve original images to allow for revisions if necessary.
- Experiment with different interpolation methods for varied results.
- Use antialiasing techniques to reduce visual artifacts in downscaled images.
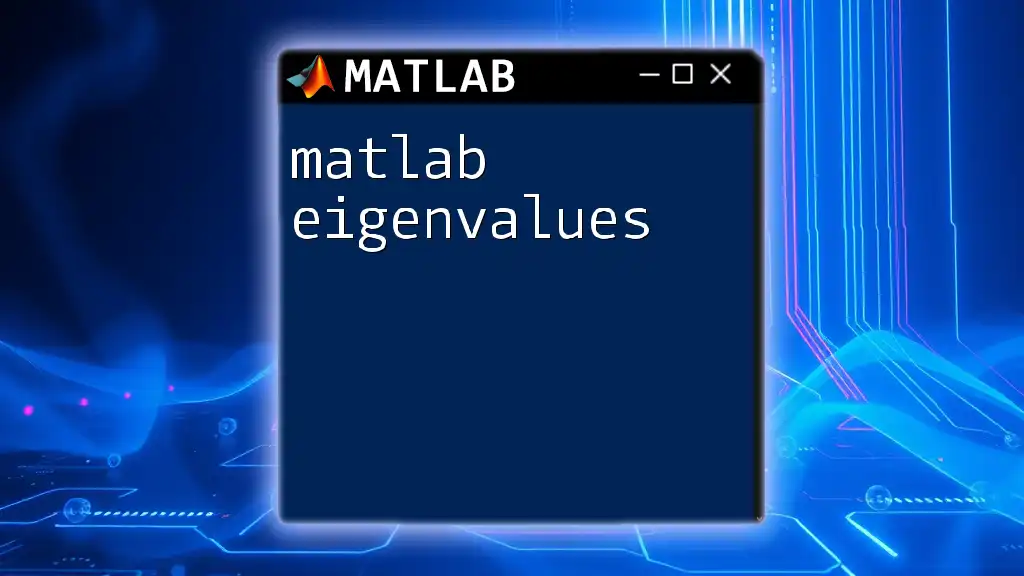
Conclusion
The `matlab imresize` function is an essential tool in image processing within MATLAB, allowing users to effectively resize images while maintaining image quality. Understanding its syntax, application methods, and potential pitfalls can significantly enhance your image manipulation capabilities.
Call to Action
Don’t hesitate to experiment with `imresize` in your projects. Apply the techniques discussed here, and explore the vibrant capabilities of image processing in MATLAB.
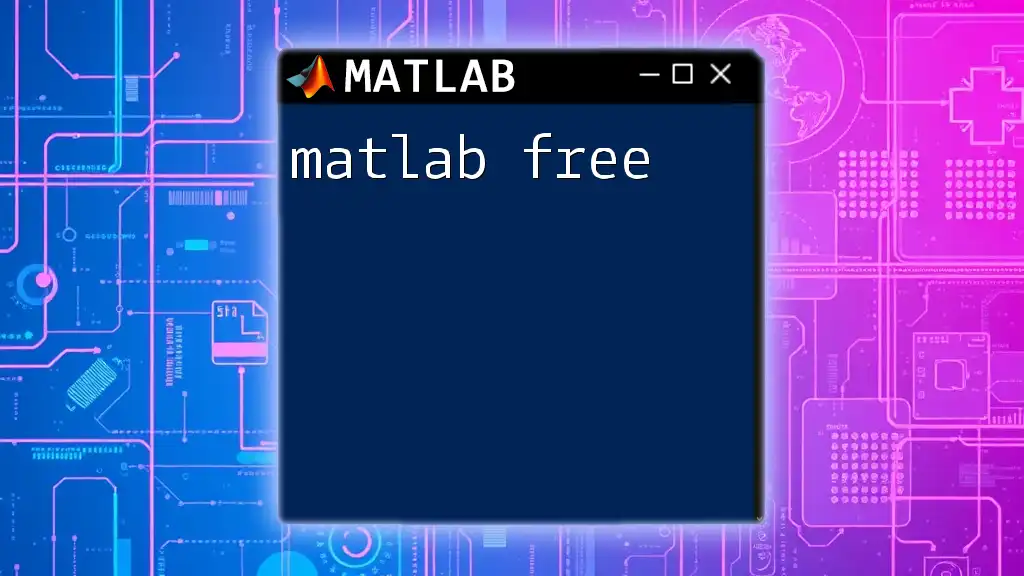
Additional Resources
For further studies, consult MATLAB’s official documentation on `imresize` and related image processing functions to broaden your understanding and refine your skills.