The `solve` function in MATLAB is used to find the solutions of algebraic equations or systems of equations.
% Example of using solve to find the roots of the equation x^2 - 4 = 0
syms x;
solution = solve(x^2 - 4 == 0, x);
disp(solution);
Understanding the Basics of MATLAB's `solve`
What is `solve`?
`solve` is a powerful function within the MATLAB environment that allows users to find solutions to algebraic equations, whether linear or nonlinear. The general syntax for the `solve` function is:
sol = solve(equation, variable)
In this syntax, `equation` represents the mathematical equation you want to solve, while `variable` is the symbolic variable for which you are seeking a solution. The `solve` function is an essential tool in both academic and professional domains, especially in fields such as engineering, physics, and applied mathematics.
Key Features of `solve`
One of the standout features of `solve` is its ability to provide both symbolic and numeric solutions.
- Symbolic Solutions: When you request a symbolic solution, MATLAB expresses the answer in terms of variables rather than in a decimal form. This is particularly beneficial when dealing with complex equations where exact values are more useful.
- Numeric Solutions: Conversely, when you need to approximate a solution, you might use numeric methods. Numeric solutions provide a decimal representation, which can be easier for computation but may lose some exactness.
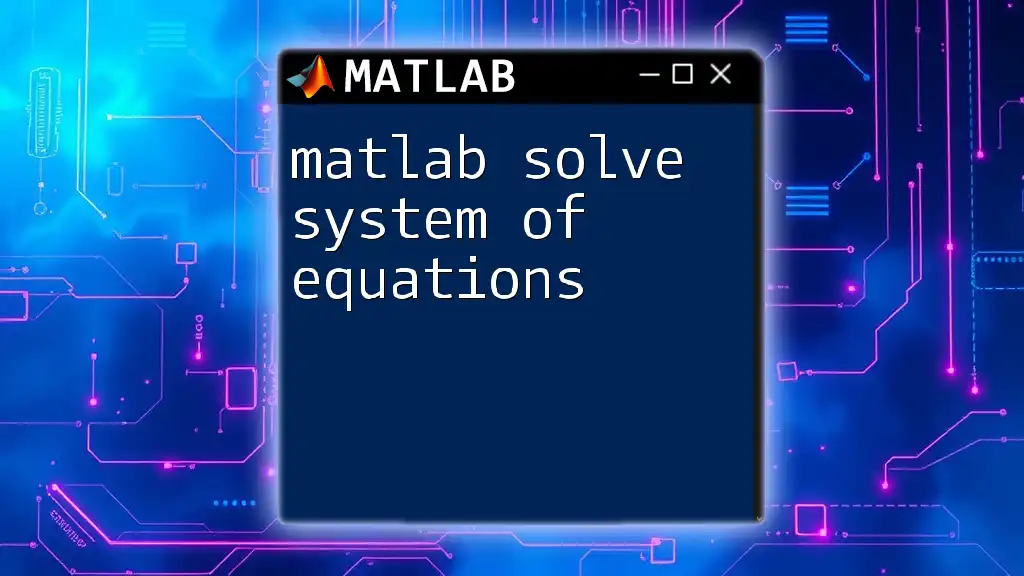
Setting Up Your Environment
Installing Symbolic Math Toolbox
To utilize the `solve` function, it is crucial to have the Symbolic Math Toolbox installed. This toolbox provides capabilities for symbolic computation, which is fundamental when dealing with equations. Ensure that you have this toolbox set up in your MATLAB environment, as it is not bundled with the base MATLAB installation.
Initializing Symbolic Variables
Before using `solve`, you must define your symbolic variables. This is accomplished using the `syms` function. By initializing these variables, MATLAB recognizes them as symbolic, allowing you to incorporate them into your equations seamlessly.
syms x y
This line declares `x` and `y` as symbolic variables, which you can now use in equations.
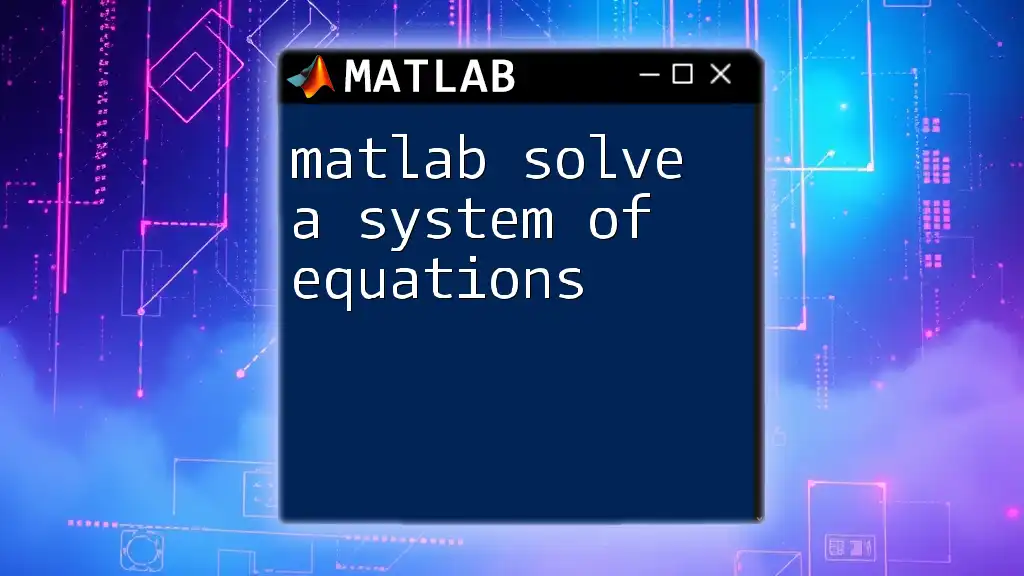
Using `solve` for Different Types of Equations
Solving Linear Equations
When dealing with linear equations, `solve` makes it straightforward to find solutions. For example, consider the linear equation:
eq = 2*x + 3 == 7;
sol = solve(eq, x)
In this case, `solve` will manipulate the equation and yield an output that represents the value of `x` satisfying that equation. You will find that such straightforward equations typically have one unique solution.
Solving Polynomial Equations
`solve` can address polynomial equations of varying degrees. For instance, let’s look at a quadratic equation:
eq = x^2 - 5*x + 6 == 0;
sol = solve(eq, x);
Using `solve`, you receive two solutions for `x`: the roots of the polynomial, which represent the points where the polynomial intersects the x-axis. Understanding the roots is fundamental in many applications, such as optimization and curve fitting.
Solving Systems of Equations
In real-world scenarios, you often need to solve systems involving multiple equations. `solve` is equipped to solve such systems efficiently. For example, consider the following set of simultaneous equations:
eq1 = x + y == 10;
eq2 = x - y == 2;
sol = solve([eq1, eq2], [x, y]);
Here, `solve` returns values for both `x` and `y` as a vector. The solutions give you the point of intersection for the two lines represented by these equations, demonstrating their relationship.
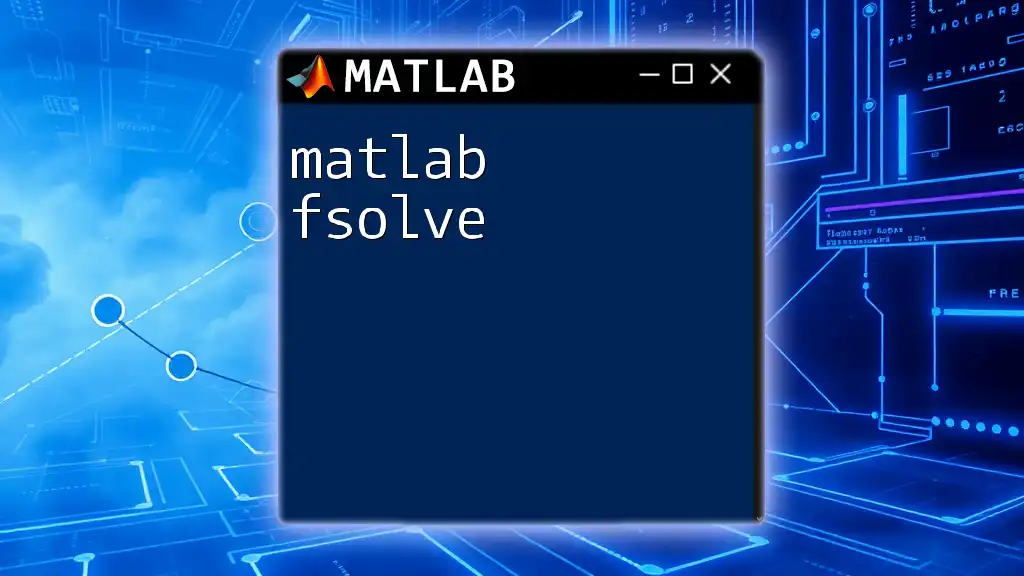
Advanced Applications of `solve`
Using `solve` with Inequalities
Apart from equations, `solve` can also handle inequalities. To demonstrate:
sol = solve(x^2 < 4, x)
The output will provide solutions that define the range of `x` satisfying the inequality condition. Solutions in this context indicate the values of `x` that keep the quadratic expression less than 4, which can be critical for understanding the behavior of functions.
Leveraging `solve` in Calculus
The `solve` function can be integrated into calculus for finding critical points of functions. This is useful in optimization problems where you need to identify maxima and minima.
f = x^3 - 3*x^2 + 2; % Define the function
df = diff(f); % Take the derivative
sol = solve(df == 0, x); % Solve for critical points
The value(s) returned by `solve` will indicate where the slope of the function is zero—the potential points for maximum or minimum values. Understanding where these points lie can substantially enhance your analytical capabilities in various applications.
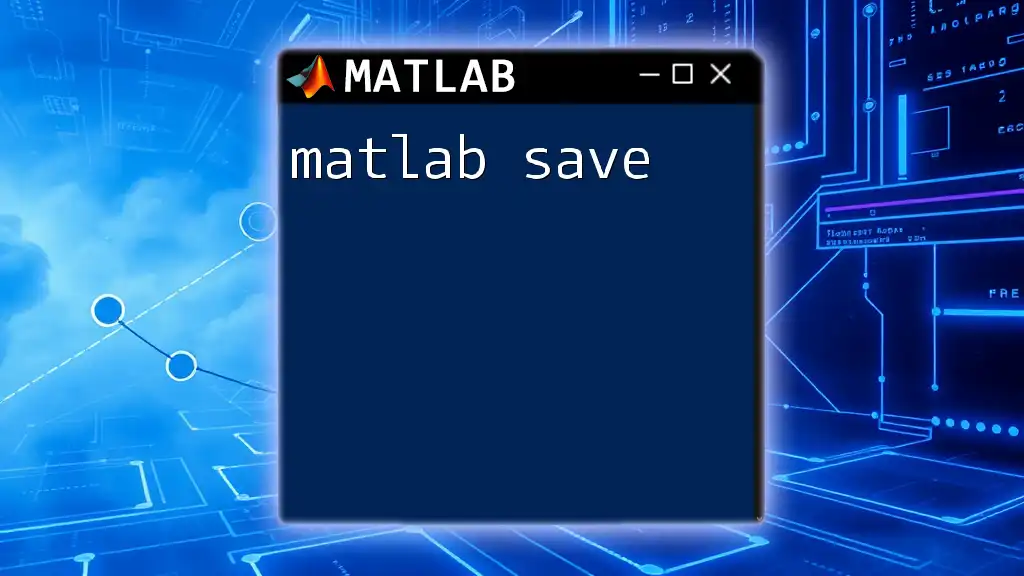
Common Issues and Troubleshooting
Error Messages and Warnings
While using `solve`, you may occasionally encounter error messages or warnings. Common issues include not having the symbolic toolbox installed, forgetting to declare symbolic variables, or attempting to solve equations that don't have analytical solutions. Addressing these errors often involves revisiting the assumptions about the equations you are working with.
Validating Solutions
It is always good practice to check your solutions, especially when dealing with critical calculations. You can use the `subs` function to substitute back into the original equation and verify:
subs(eq, x, sol)
This method allows you to confirm that your computed solutions are correct, which is vital for ensuring accuracy in critical work.
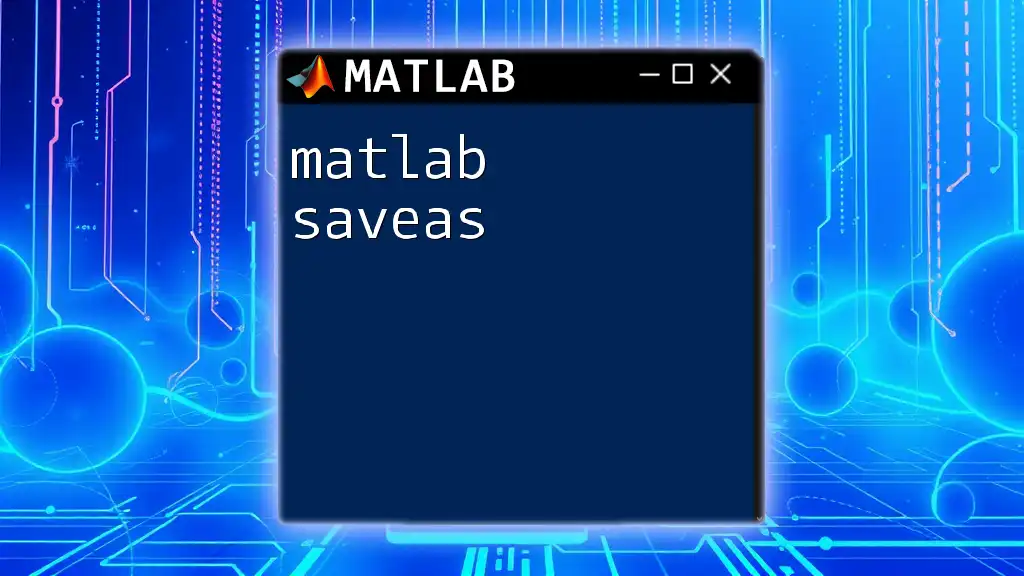
Conclusion
This comprehensive guide provides an in-depth look at the `solve` function in MATLAB. From basic understanding to advanced applications, mastering `solve` will empower you to tackle various mathematical problems efficiently. Experimenting with this function will not only solidify your understanding of MATLAB but also enhance your problem-solving repertoire.
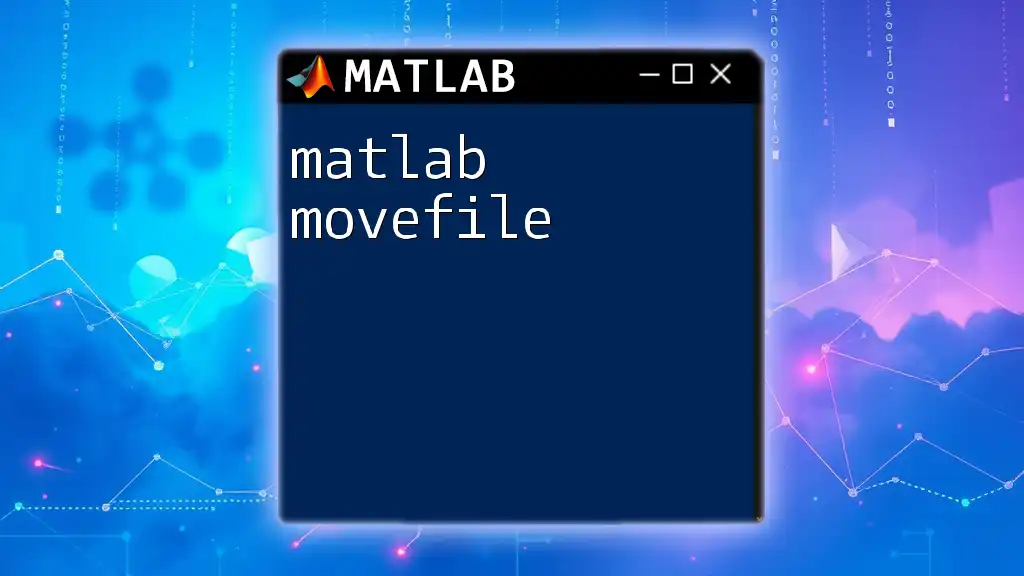
Additional Resources
To further deepen your knowledge, consider exploring MATLAB's extensive documentation on the `solve` function and related tutorials. Delve into specialized books and online courses that focus on MATLAB for advanced topics, ensuring you have the tools to succeed in your mathematical endeavors.