In MATLAB, normalization is the process of adjusting the values in a dataset to a common scale, often achieved by scaling the data to have a mean of zero and a standard deviation of one.
Here’s a simple code snippet to normalize a vector:
data = [1, 2, 3, 4, 5]; % Example data
normalizedData = (data - mean(data)) / std(data); % Normalized data
What is Normalization?
Normalization is a crucial process in data analysis, which involves scaling the values of a dataset to a specific range. The primary purpose of normalization is to ensure that the features of the data have equal weight during analysis and modeling. This is particularly essential in mathematical computations where some features might be disproportionately large or small compared to others, leading to biased results.
Normalization is widely applied across various fields such as machine learning, image processing, and statistics. By transforming features to a common scale, normalization enhances the performance and accuracy of algorithms.
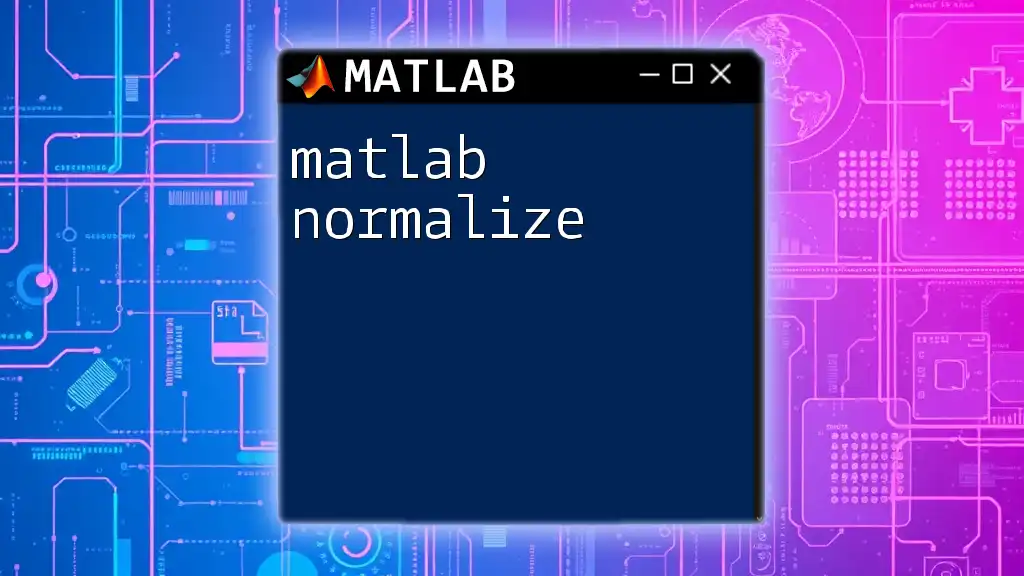
Why Use MATLAB for Normalization?
MATLAB is an excellent choice for performing normalization due to its powerful computational capabilities and intuitive programming environment. It offers several built-in functions specifically designed for data manipulation, making it easy to apply normalization techniques efficiently.
Using MATLAB to normalize datasets provides benefits like:
- Speed: Built-in functions are optimized for performance.
- Ease of Use: MATLAB’s syntax is straightforward, which allows for quick implementations.
- Visualizations: MATLAB’s plotting features enable users to visualize the effects of normalization on data.
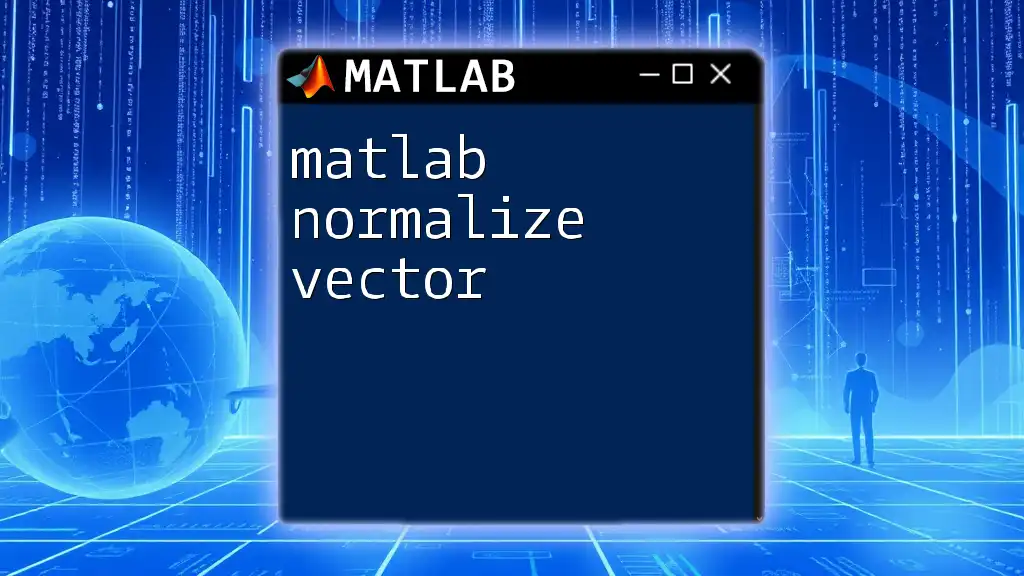
Understanding Data Normalization
Types of Normalization
Min-Max Normalization
Min-Max normalization rescales the values of the dataset to a specified range, typically [0, 1]. This approach is particularly useful when working with algorithms that utilize distance metrics, like k-means clustering.
Formula: \[ \text{X}{\text{scaled}} = \frac{(\text{X} - \text{X}{\text{min}})}{(\text{X}{\text{max}} - \text{X}{\text{min}})} \]
Z-Score Normalization
Z-Score normalization, also known as standardization, transforms the dataset to have a mean of 0 and a standard deviation of 1, which can be useful for identifying outliers.
Formula: \[ Z = \frac{(X - \mu)}{\sigma} \]
Other Methods
Other normalization methods include:
- Decimal Scaling: Moves the decimal point of values to bring them into a specified range.
- Robust Normalization: Focuses on using medians and percentiles to reduce the influence of outliers.
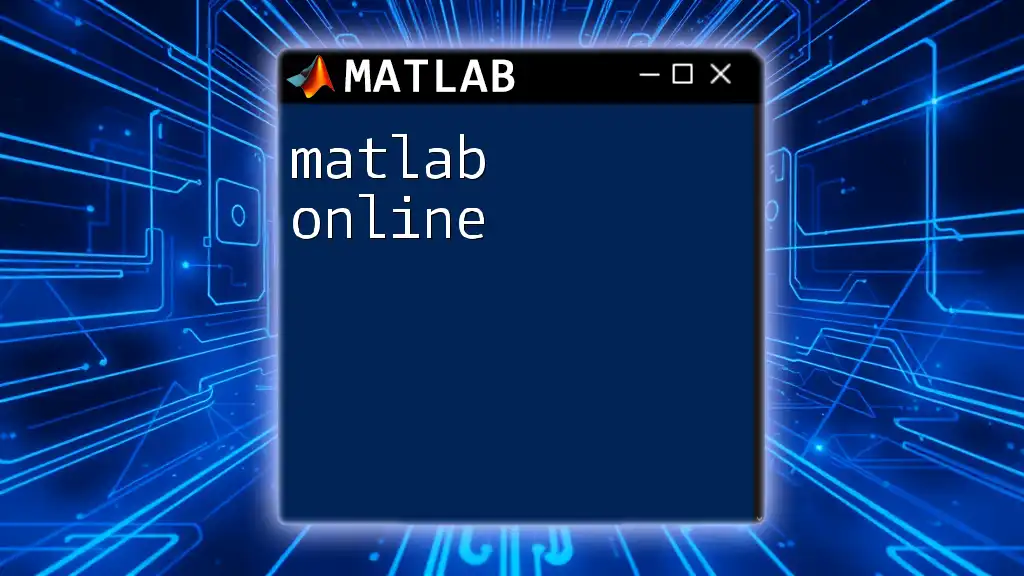
MATLAB Functions for Normalization
Using the `normalize` Function
The `normalize` function in MATLAB provides a quick and effective way to normalize a dataset.
Description: This function enables users to apply various normalization methods, including Min-Max and Z-Score normalization.
Syntax and Parameters:
Y = normalize(X, 'norm');
In this command, `X` is the input data and `'norm'` is the type of normalization specified. Depending on the parameter passed—'zscore', 'range', or 'norm'—MATLAB adjusts the data accordingly.
Example of Min-Max Normalization
To apply Min-Max normalization, the following code snippet can be used:
data = [1, 2, 3, 4, 5];
normalizedData = normalize(data, 'range');
This operation rescales the values of the dataset, ensuring they fall within the range of 0 to 1.
Custom Normalization Functions
Creating custom normalization functions in MATLAB can be valuable for specific applications.
Creating Your Own Function for Min-Max Normalization
You can define a function like so:
function normalizedData = minMaxNormalize(data)
normalizedData = (data - min(data)) / (max(data) - min(data));
end
Example Usage
To apply this custom function:
sampleData = [10, 20, 30, 40, 50];
normalizedSample = minMaxNormalize(sampleData);
This code takes a sample dataset and normalizes it to the range [0, 1].
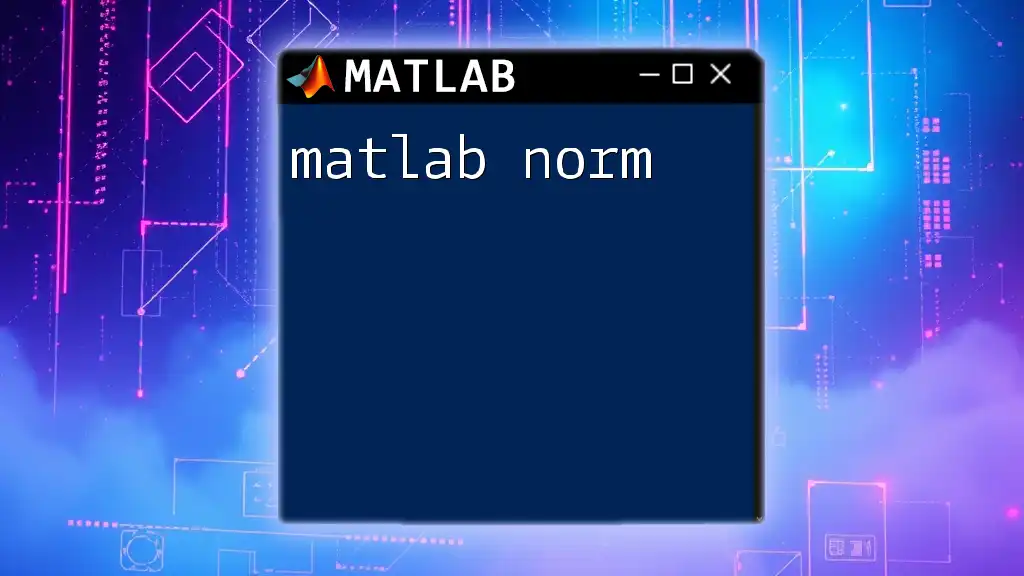
Practical Applications of Normalization
Normalization in Machine Learning
In machine learning, normalization plays a pivotal role in improving model performance. Most algorithms rely on distances or gradients, and unnormalized data can lead to inaccurate results.
For example, when comparing models trained on raw data versus normalized data, it is often seen that normalization leads to faster convergence and improved accuracy. Standardized data allows algorithms to treat all features equally, enhancing the quality of predictions.
Image Processing Normalization
Normalization in image processing can lead to improved image quality and analysis. Techniques like histogram equalization enhance contrast, making images appear clearer and more distinct.
Code Example
A common practice for image normalization involves the following code snippet:
img = imread('example.jpg');
imgNorm = im2double(img);
imgNorm = (imgNorm - min(imgNorm(:))) / (max(imgNorm(:)) - min(imgNorm(:)));
imshow(imgNorm);
This code reads an image, normalizes its pixel values, and displays the enhanced image.
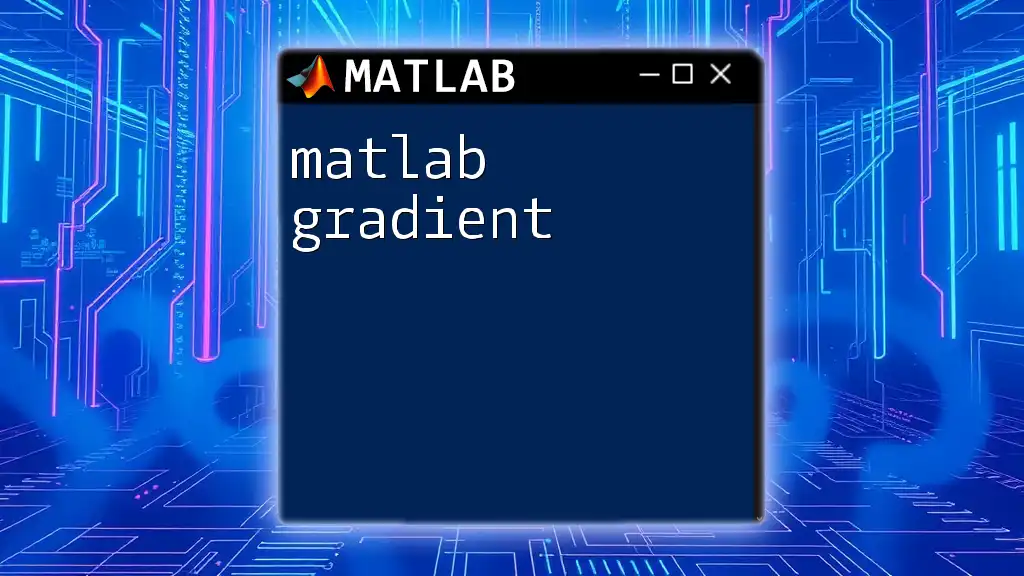
Advanced Normalization Techniques
Robust Normalization
Robust normalization uses statistics resistant to outliers, such as the median and interquartile range (IQR). This technique is especially beneficial when dealing with non-normally distributed data.
Example Code
You can implement robust normalization using the following code:
function robustData = robustNormalize(data)
medianVal = median(data);
IQR = prctile(data, 75) - prctile(data, 25);
robustData = (data - medianVal) / IQR;
end
Normalization with Multiple Features
Multi-dimensional datasets often require special attention during normalization. When normalizing multiple features, it is essential to apply the same normalization strategy across all features to maintain the relationships within the data.
For instance, normalizing a feature matrix might look something like this:
dataMatrix = [1 2 3; 4 5 6; 7 8 9];
normalizedMatrix = normalize(dataMatrix);
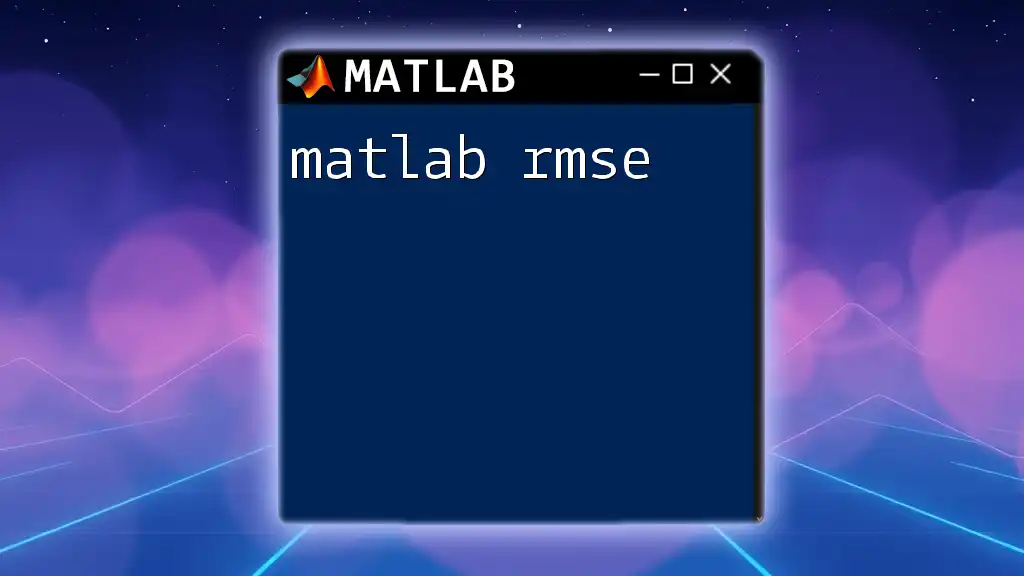
Common Pitfalls in Normalization
Understanding Data Distribution
One must assess the distribution of the data before normalization, as certain techniques may not be appropriate for highly skewed datasets. For example, Min-Max normalization might compress a wide range of values into a small scale if outliers are present.
Feature Scaling versus Normalization
It's vital to distinguish between normalization and feature scaling. While normalization often refers specifically to adjusting the range of data values, feature scaling can encompass additional techniques, including transformations based on the standard deviation and mean.
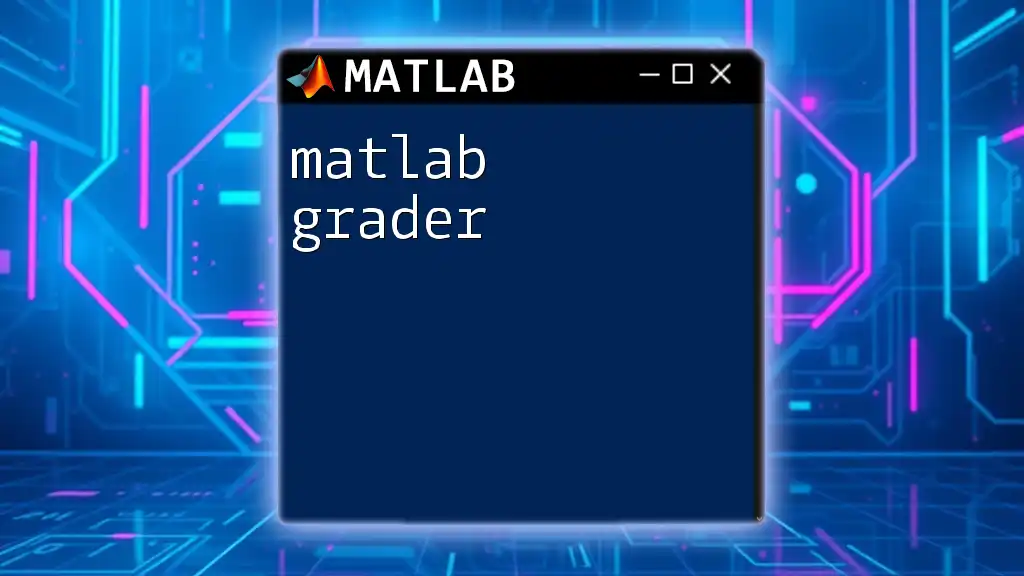
Conclusion
In summary, normalization is a fundamental process in data preparation that plays a crucial role in ensuring accurate analytical outcomes. Understanding different normalization techniques and their implementation in MATLAB can significantly enhance your data analysis capabilities.
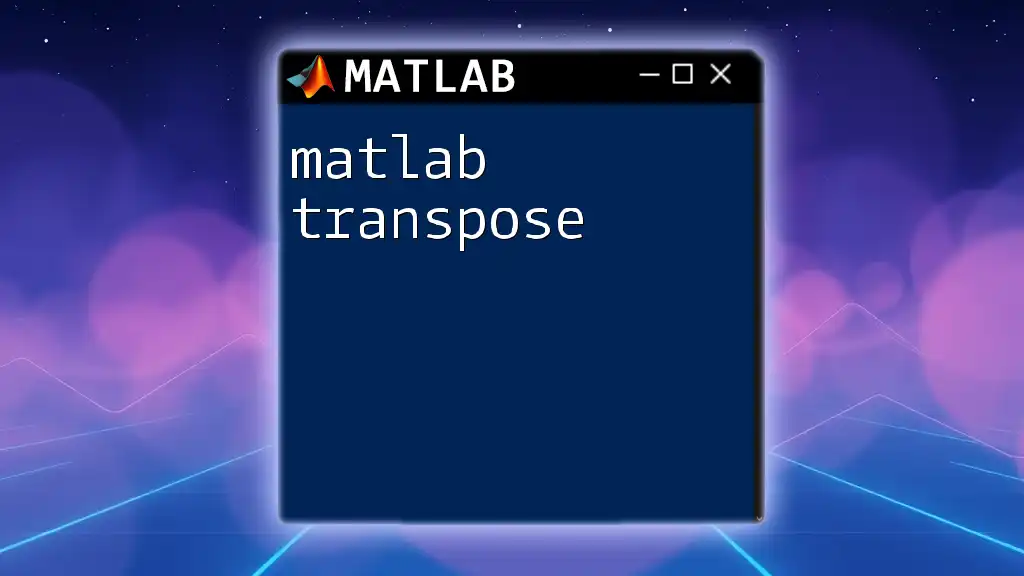
Additional Resources
To deepen your knowledge of MATLAB normalization techniques, refer to the official MATLAB documentation and explore online tutorials. Engaging with community forums can also be a great way to learn from fellow MATLAB users and enhance your understanding further.
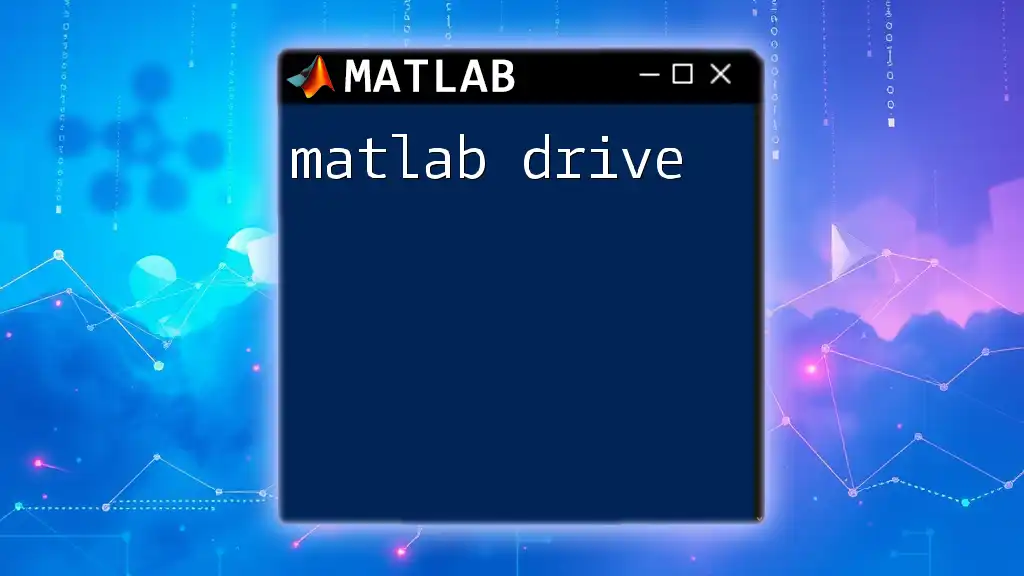
Call to Action
Join our MATLAB community to access hands-on classes and personalized training sessions tailored to mastering normalization techniques in MATLAB! Whether you're a beginner or looking to enhance your skills, we have resources to support your learning journey.