The `disp` function in MATLAB is used to print text or variables to the command window in a straightforward manner.
Here's a code snippet demonstrating its usage:
disp('Hello, MATLAB!')
Understanding MATLAB's Display Functions
Common Functions for Printing Text
`disp` Function
The `disp` function is one of the simplest ways to display text in MATLAB. It takes a string input and outputs it directly to the Command Window. This function is especially handy for quickly printing messages or values without any formatting.
Syntax and Usage:
disp(value);
Example: Basic Text Display:
disp('Hello, MATLAB!');
When using `disp`, the text is displayed as is, which makes it easy to use in scripts and during debugging. Unlike some other functions, `disp` does not return output, making it less useful when you need to capture or manipulate the text later.
`fprintf` Function
The `fprintf` function is more versatile than `disp` because it allows for formatted output. This means you can control how the text is displayed, including adjusting the width, alignment, and even the precision of numerical values.
Syntax Breakdown:
fprintf(formatSpec, A, ...)
Where `formatSpec` is a string that specifies the format of the output and `A` represents the variables to display.
Formatted Output Capabilities: You can include placeholders (like `%s` for strings or `%.2f` for floating-point numbers) in your format specification to format the output accordingly.
Example: Printing Formatted Strings:
fprintf('The value of pi is approximately %.2f\n', pi);
In this example, `%.2f` specifies that the value of pi will be displayed with two decimal places. The `\n` at the end moves the cursor to a new line after printing.
Understanding Escape Sequences: `fprintf` supports various escape sequences for formatting, such as:
- `\\` for a backslash
- `\n` for a new line
- `\t` for a tab
These sequences can enhance the readability of your output.
Use Cases for Formatted Output: Utilizing `fprintf` is advantageous in scenarios where precise control over the output presentation is required, such as generating reports or displaying tables of data.
`sprintf` Function
While `fprintf` prints directly to the console, `sprintf` generates a formatted string that can then be stored in a variable for later use.
Similarities and Differences with `fprintf`: The syntax is almost identical, with the key difference being that `sprintf` returns a string instead of directly displaying it.
Example: Generating a Text String:
str = sprintf('The value of e is %.4f', exp(1));
Here, `str` will contain the formatted string, which is useful for further manipulation or display later in your script.
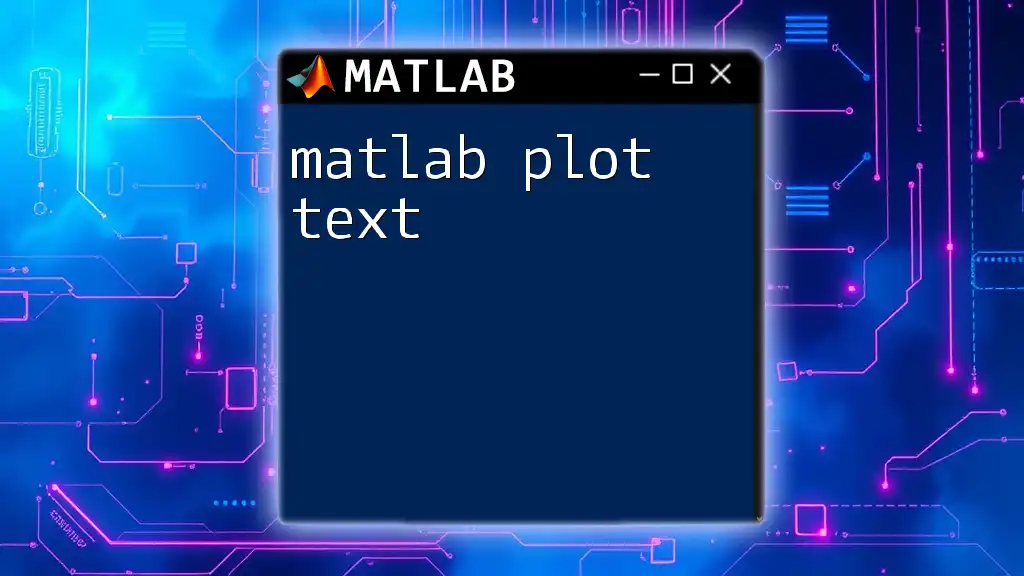
Advanced Text Display Techniques
Combining Text and Variables
MATLAB allows for easy combination of strings and variables. This is particularly useful when generating dynamic messages based on variable values.
Concatenation of Strings: Using square brackets, you can concatenate strings and numeric values (converted to strings) effortlessly.
Example: Concatenating Strings and Numbers:
userAge = 25;
disp(['User age is: ', num2str(userAge)]);
In this example, `num2str` converts the numeric variable `userAge` into a string, allowing it to be concatenated with the other string.
Controlling Text Formatting
With `fprintf`, you have the ability to align your text output neatly for better readability. By using width specifiers, you can format your output in tabular form.
Aligning Text Output: You can specify widths for your output, which is particularly useful when printing lists or tables.
Example: Left and Right Alignment:
fprintf('%-10s %5s\n', 'Item', 'Price');
fprintf('%-10s %5.2f\n', 'Apple', 1.20);
fprintf('%-10s %5.2f\n', 'Banana', 0.50);
In this example, the first string `%-10s` is left-aligned and takes up 10 characters, while the price `%.2f` occupies 5 characters and is right-aligned.
Using Cell Arrays for Complex Outputs
For more complex data that requires multi-dimensional output, MATLAB supports cell arrays. These allow for storing strings and outputting them easily.
Creating Cell Arrays of Text: You can create a cell array to store items with their corresponding prices.
Example:
items = {'Apple', 1.2; 'Banana', 0.5};
disp(items);
This displays a matrix of text and numbers, but we can format it more cleanly with loops.
Using Loops for Dynamic Outputs: If you want to format each entry of the array neatly, you can use a loop.
Example: Looping through a Cell Array:
for i = 1:size(items, 1)
fprintf('%s: $%.2f\n', items{i, 1}, items{i, 2});
end
This loops through each item in the `items` cell array and prints them in a clear format.
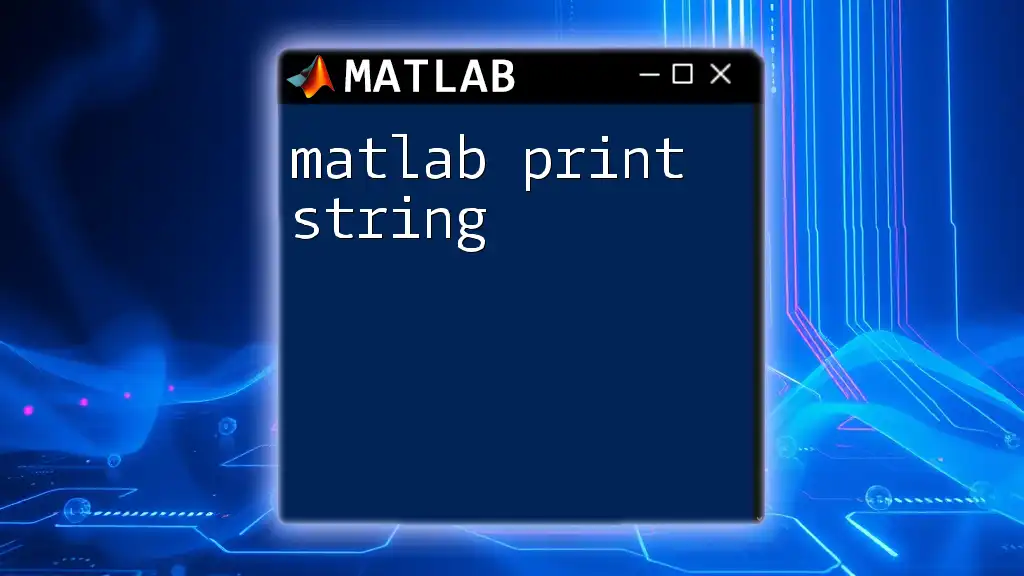
Practical Applications of Printing Text
Debugging with Display Functions
Printing text can be invaluable for debugging. By giving you insight into variable states and program flow, you can easily identify issues.
Using `disp` and `fprintf` for Debugging: You can leverage these functions to provide status updates in your code.
Example: Debugging with Timed Outputs:
startTime = tic;
disp('Starting process...');
pause(2); % Simulating a process
fprintf('Process completed in %.2f seconds.\n', toc(startTime));
This example not only informs you when a process starts but also tracks how long it takes to complete, which is essential for optimizing performance.
User Interaction
MATLAB also allows for interactive user sessions where the program can ask for input and respond accordingly.
Getting User Input and Displaying It: The `input` function can be used to retrieve information from the user effectively.
Example: Printing User Responses:
name = input('Enter your name: ', 's');
fprintf('Hello, %s!\n', name);
Here, the user is prompted to enter their name, and the program responds with a personalized greeting.
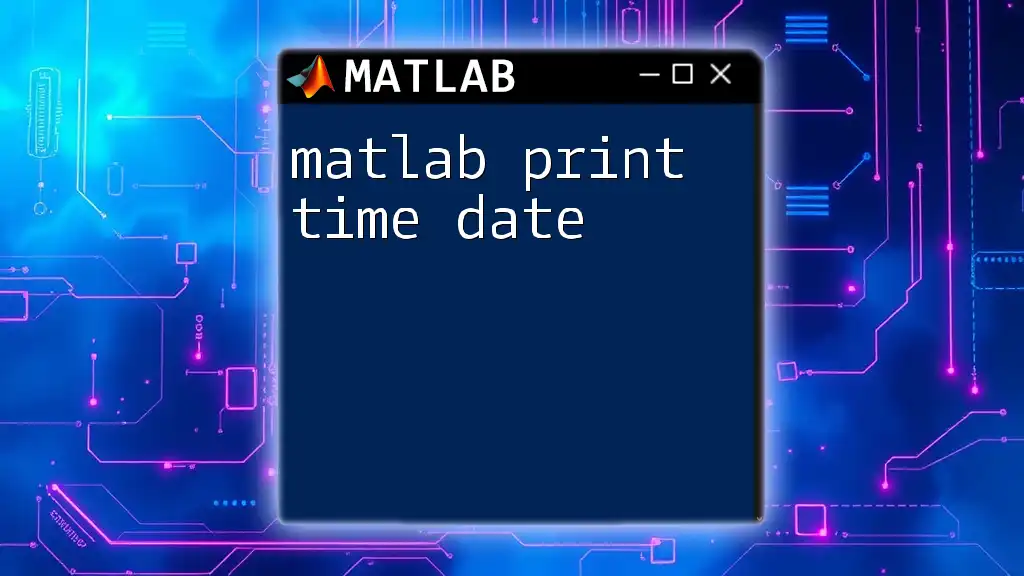
Best Practices for Displaying Text in MATLAB
Readability and Clarity
When dealing with output, ensuring that your text is organized, clear, and easy to read is paramount. Following consistent formatting practices keeps your output professional.
Maintaining Output Consistency:
- Use consistent terminology in your printed messages to avoid confusion.
- Format the output in a way that adheres to logical structures, making it intuitive to read.
Performance Considerations
When printing text in MATLAB, the choice of function matters. While `disp` is quick and easy, for formatted outputs, consider using `fprintf` or `sprintf`. This not only improves the readability of your output but also enhances the efficiency of your code.
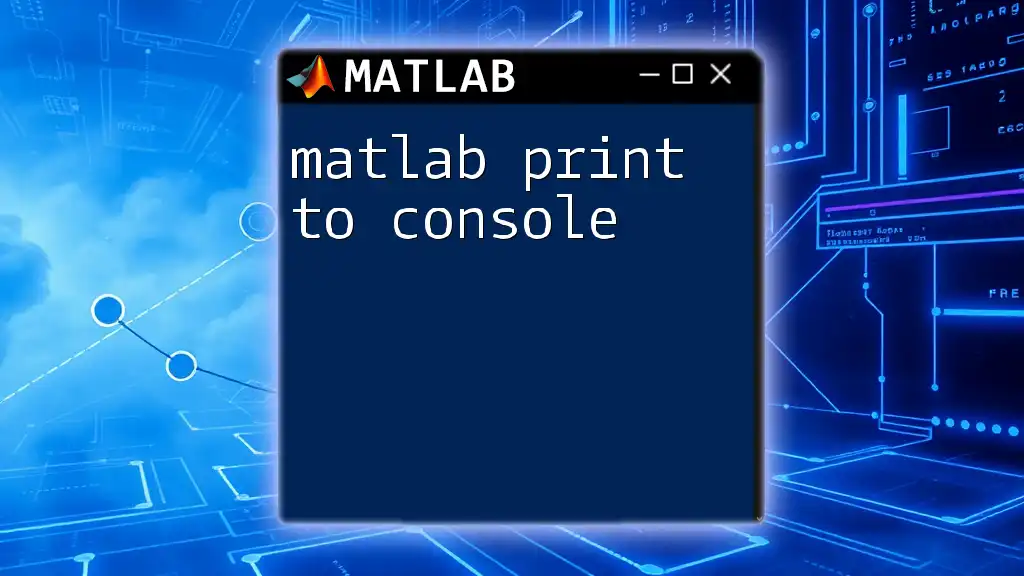
Conclusion
Summary of Key Points
In this article, we explored various ways to matlab print text effectively, from using basic functions like `disp` to more advanced techniques like formatted output with `fprintf` and dynamic string generation with `sprintf`. Alongside combining variables, aligning text, and utilizing cell arrays, these tools empower you to display informative messages clearly.
Encouragement for Practice
Experiment with these functions in your own MATLAB environment. Try to create scripts that utilize dynamic printing to enhance your coding projects and debugging sessions.
Call to Action
Engage with the MATLAB community by sharing your experiences with output techniques and how they've improved your coding practices!