The `fprintf` function in MATLAB is used to print formatted text to the screen or a file, allowing for precise control over the output format.
fprintf('The value of pi is approximately %.2f\n', pi);
Introduction to `fprintf`
What is `fprintf`?
`fprintf` is a powerful function in MATLAB that allows users to write formatted data to the Command Window or a file. The versatility of `fprintf` makes it a preferred choice for developers and data analysts who wish to present their output in a structured manner.
Why use `fprintf`?
Using `fprintf` offers several advantages over other output functions in MATLAB, such as `disp` or `sprintf`. Primarily, `fprintf` provides greater control over the formatting and presentation of output data. This is particularly useful when displaying numerical results where precision is crucial or when outputting complex data structures. Moreover, `fprintf` supports various format specifiers, which let you control the appearance of your output, ensuring clarity and readability.
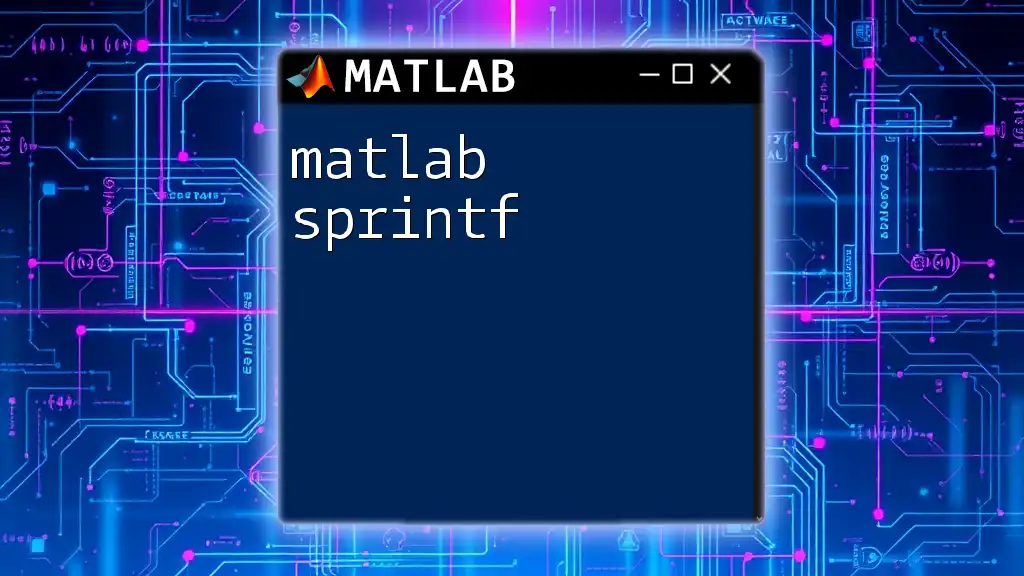
Basic Syntax of `fprintf`
Understanding the Syntax
The basic syntax for `fprintf` is:
fprintf(formatSpec, A1, A2, ..., An)
- `formatSpec`: A string that specifies how to format the output. This string can include text and format specifiers.
- `A1, A2, ... An`: The values you want to display, corresponding to the format specifiers in `formatSpec`.
Example of Basic Usage
For simple outputs, `fprintf` can be utilized as follows:
fprintf('Hello, World!\n');
In this example, the text "Hello, World!" is printed to the Command Window, followed by a newline character due to `\n`.
Common Placeholders and Format Specifiers
Here is a list of common format specifiers that can be used with `fprintf`:
- `%d`: Used for integers.
- `%f`: Used for floating-point numbers.
- `%s`: Used for strings.
Details on Each Specifier:
- `%d`: This specifier will format the output as an integer. For example, `fprintf('The value is: %d\n', 42);` prints "The value is: 42".
- `%f`: This specifier formats floating-point numbers. You can control the number of decimal places by including additional formatting, like `%.2f` for two decimal places.
- `%s`: This is utilized for string outputs. For instance, `fprintf('My name is: %s\n', 'Alice');` will output "My name is: Alice".
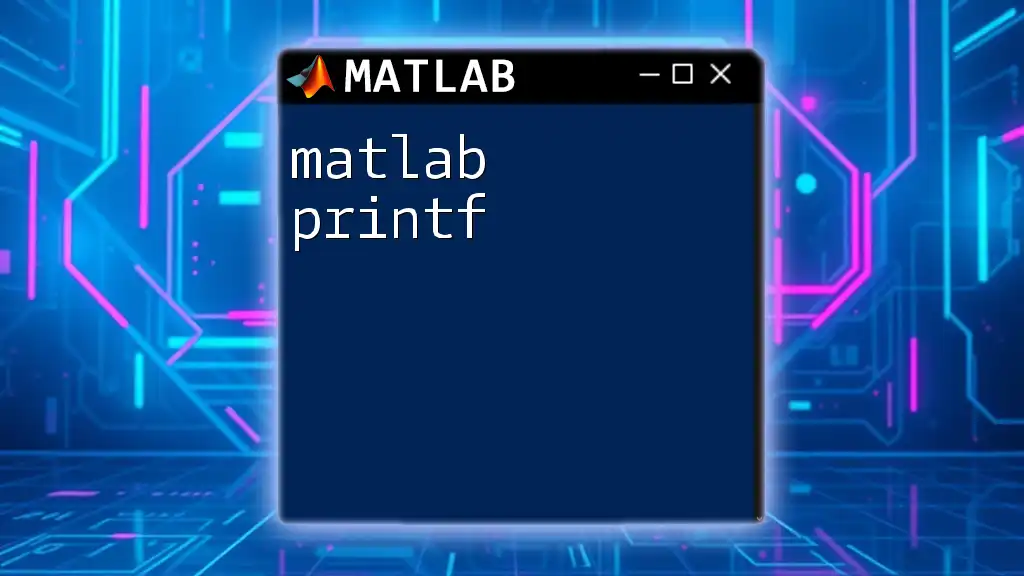
Advanced Formatting Options
Controlling Decimal Places
When working with floating-point numbers, controlling the number of decimal places can be essential.
fprintf('Value: %.2f\n', 123.456);
In this case, the output will display "Value: 123.46", rounding the number to two decimal places.
Padding and Aligning Text
To enhance the readability of outputs, `fprintf` allows you to define the width for your output strings.
fprintf('%10s\n', 'Right');
fprintf('%-10s\n', 'Left');
In the first example, "Right" will be right-aligned in a field of 10 characters, while "Left" will be left-aligned.
Scientific Notation
For large or small numbers, displaying values in scientific notation can be useful.
fprintf('Scientific: %.2e\n', 12345.6789);
The output will be formatted as "Scientific: 1.23e+04", providing a concise representation of the number.
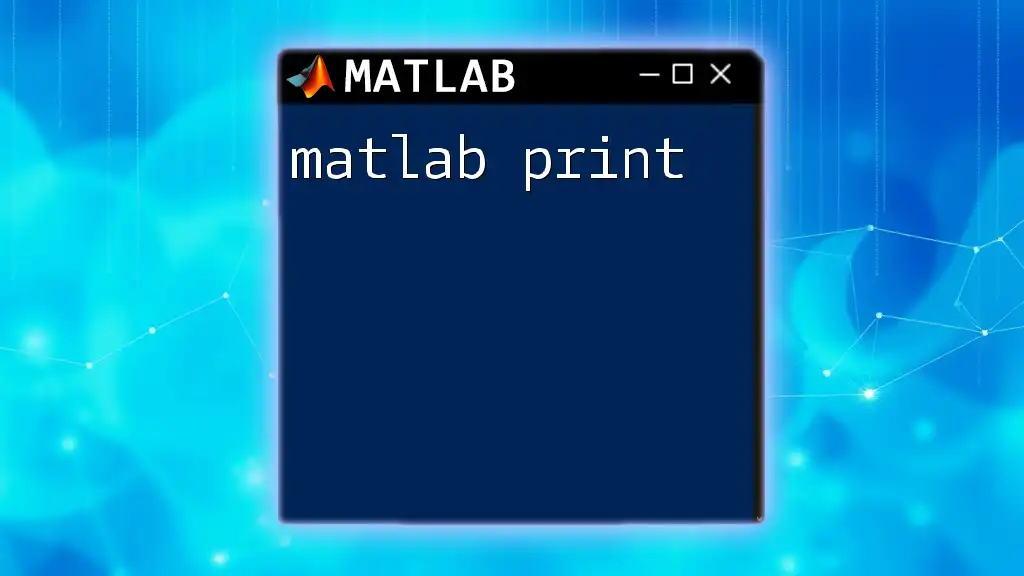
Writing Output to a File
How to Open a File for Writing
To write output to a file instead of the Command Window, you'll first need to open a file using `fopen`.
fid = fopen('output.txt', 'w');
In this command, `fid` is the file identifier that will be used to reference the opened file. The 'w' mode signifies that the file is opened for writing.
Using `fprintf` with Files
After opening the file, you can use `fprintf` to write data directly to it using the file identifier.
fprintf(fid, 'Writing to a file: %.2f\n', 123.456);
Here, the formatted string is written to "output.txt". It's important to ensure that the format of the data being passed matches the specifiers.
Closing the File
After completing your file-writing tasks, it’s crucial to close the file to free resources.
fclose(fid);
This step prevents any potential data loss and ensures that all data is saved correctly.
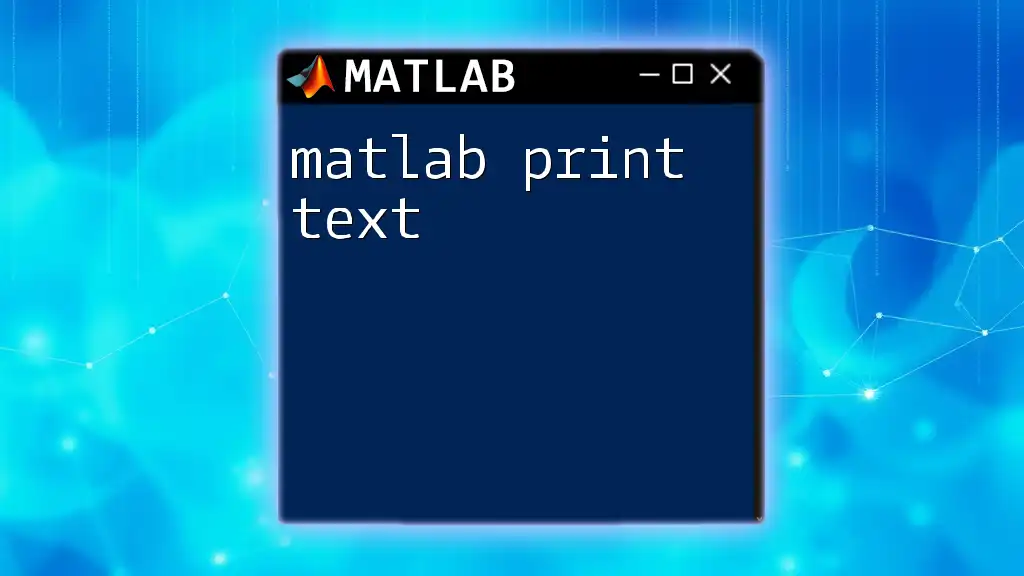
Multiline Output with `fprintf`
Creating reports or displaying outputs across multiple lines can be easily accomplished using `\n` to signify new lines.
fprintf('Line 1\nLine 2\n');
The output will present two lines, enhancing readability, especially in reports or data summaries.
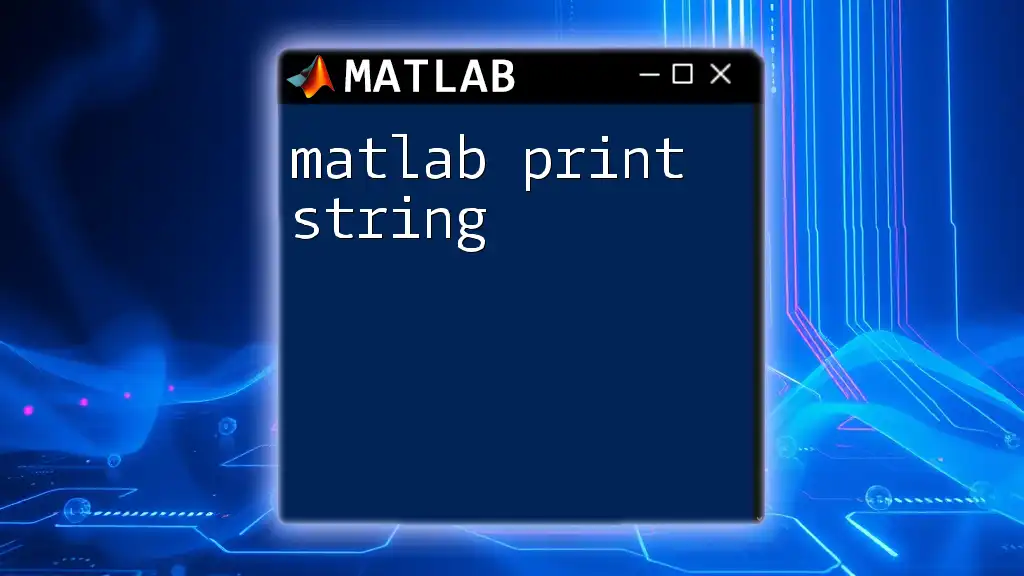
Using `fprintf` in Loops
When you need to display multiple outputs, especially in iterative processes, `fprintf` can be efficiently employed within loops.
for i = 1:5
fprintf('Iteration %d: Value = %.2f\n', i, rand() * 100);
end
This loop prints the iteration index alongside a random value, generating five lines of output.
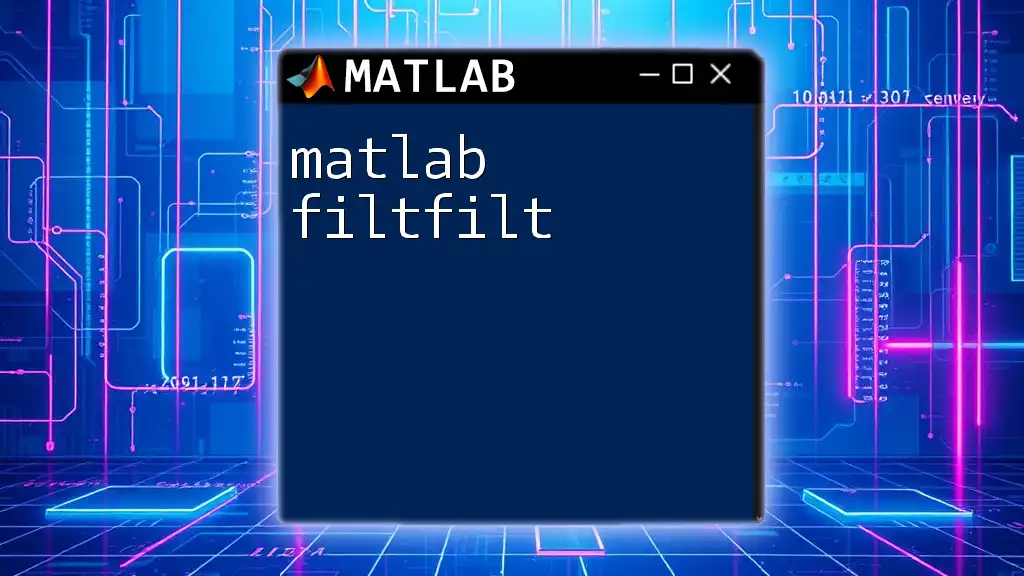
Common Errors and Troubleshooting
Common Mistakes with `fprintf`
One of the most frequent errors encountered while using `fprintf` is the absence of format specifiers or mismatched data types. For example, if you were to write:
value = 10;
fprintf('The value is: %f\n', value);
...while `value` is an integer, and `%d` should be used instead with integer values.
Tips for Debugging
To avoid errors, ensure you are using the correct format types corresponding to the data you are outputting. Always check file permissions when attempting to write to a file, as insufficient permissions can lead to runtime errors.
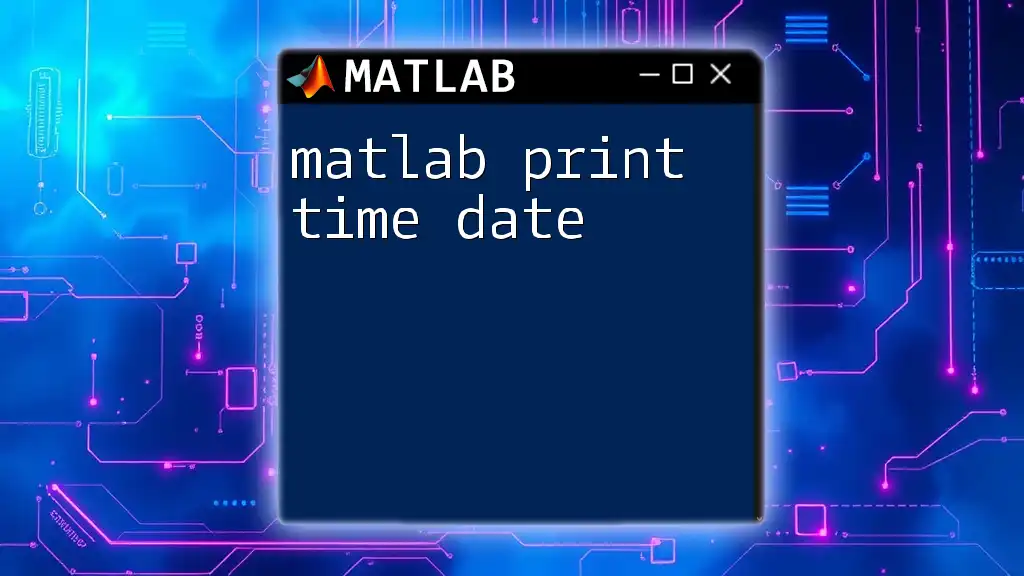
Summary and Best Practices
Quick Recap of `fprintf` Features
The `fprintf` function in MATLAB is an essential tool for formatted output across various applications. Its flexibility with formatting specifiers, ability to write to files, and use within loops makes it indispensable for effective data presentation.
Best Practices
When to use `fprintf` over other output methods should be informed by the need for formatted output, especially in cases where clarity and precision matter. Performance considerations, especially with large datasets, should also steer your choice of output methods.
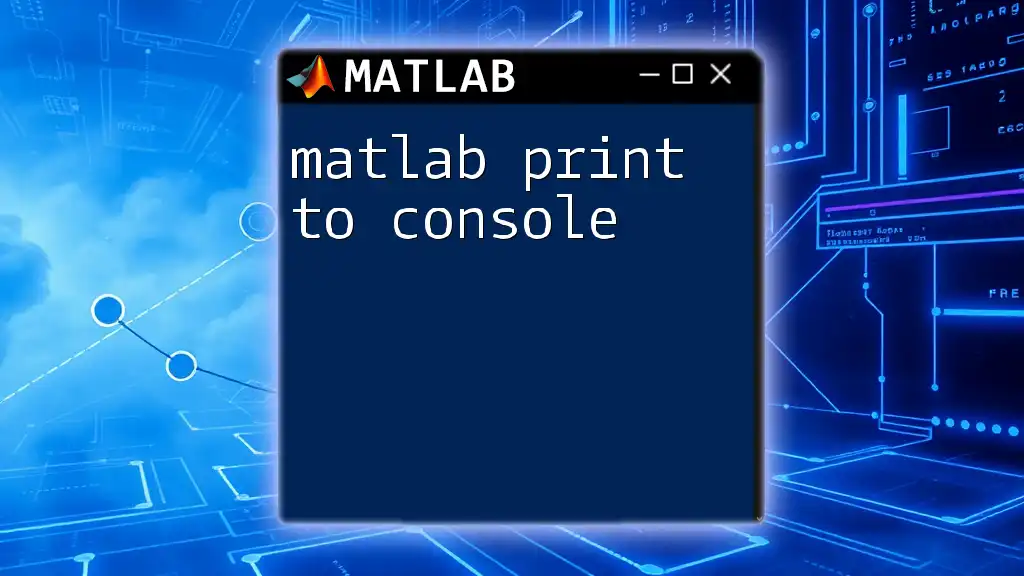
Conclusion
Practice is key to mastering the `fprintf` function in MATLAB. The outlined features and examples serve as a foundation for applying `fprintf` effectively in your coding endeavors. For further learning, dive into available resources and community forums that offer additional tips and troubleshooting advice.