In MATLAB, the `printf` function is used to format and print data to the command window, allowing for customized output with specified format specifiers.
fprintf('The value of pi is approximately: %.2f\n', pi);
Understanding the Basics of `printf`
What is `printf`?
The `printf` function in MATLAB is a powerful tool for displaying formatted text and data in the command window or a file. It allows users to output data in a way that is structured and readable, making it easier to present results. This is essential not just for debugging but also for sharing results in a clear manner.
Syntax of `printf`
The general syntax for `printf` in MATLAB is as follows:
fprintf('format_specifier', variables)
- The first argument is a format string that defines how the subsequent variables are displayed.
- The second argument (or more) specifies the values that will replace the format specifiers in the format string.
This simple structure allows for a wide variety of outputs that can be customized according to your needs.
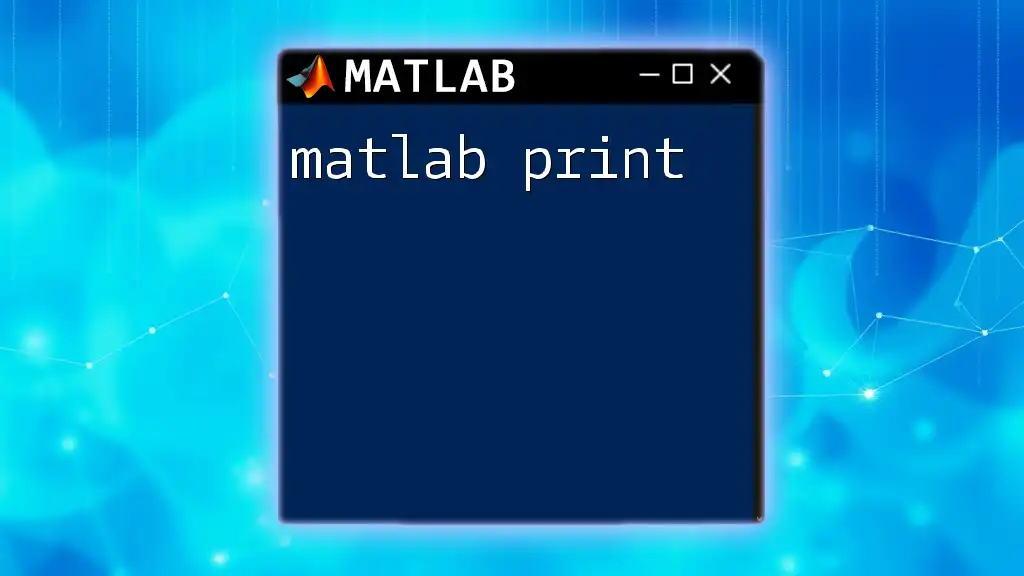
Format Specifiers in `printf`
Overview of Format Specifiers
Format specifiers are special placeholders within the format string that dictate how the data will be presented. Using the correct format specifier is critical in ensuring the output appears as expected. Common format specifiers include:
- `%d` for integers,
- `%f` for floating-point numbers,
- `%s` for strings,
- `%c` for characters.
Numeric Format Specifiers
Integer Specifiers
When you want to output integers, you can use `%d` for signed integers and `%u` for unsigned integers. Here's an example showcasing the use of the `%d` specifier:
fprintf('The integer is: %d\n', 42);
In this example, `42` replaces `%d`, resulting in the output "The integer is: 42".
Floating Point Specifiers
MATLAB provides different ways to output floating-point numbers. The most common specifier is `%f`, which represents fixed-point notation. However, `%e` is used for scientific notation, and `%g` is versatile, automatically choosing between `%f` and `%e` depending on the value.
For example:
fprintf('The value of pi is: %.2f\n', pi);
In this case, `%.2f` specifies that the output should display the value of pi with two decimal places, resulting in "The value of pi is: 3.14".
String and Character Format Specifiers
When working with strings, the `%s` format specifier comes into play. It is used to print text, and the `%c` specifier is employed to output single characters.
Here are some examples:
name = 'Alice';
fprintf('Hello, %s!\n', name);
The command above results in "Hello, Alice!".
For characters, you might write:
initial = 'A';
fprintf('Your initial is: %c\n', initial);
This outputs "Your initial is: A".
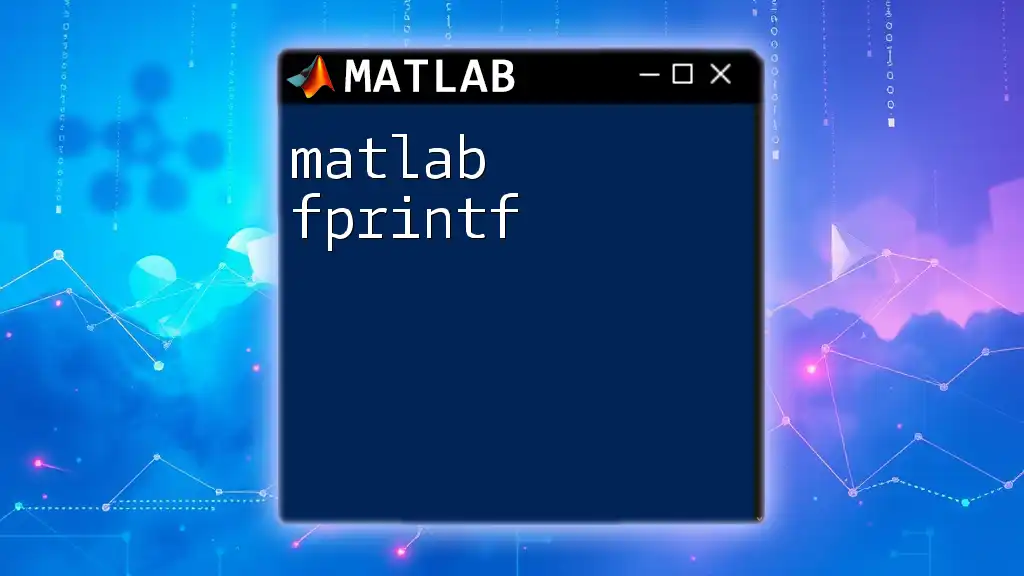
Formatting with Precision and Width
Specifying Field Width
You can control the width of the output by specifying a minimum field width. For instance:
fprintf('Number: %5d\n', 10);
In this case, `10` will occupy a field width of 5, which results in the output "Number: 10". The extra spaces are filled with blanks to meet the specified width.
Controlling Decimal Places
In addition to controlling field width, you can specify the number of decimal places for floating-point numbers. For example:
fprintf('Value: %.4f\n', 123.456789);
Here, `%.4f` restricts the output to four decimal places, resulting in "Value: 123.4568".
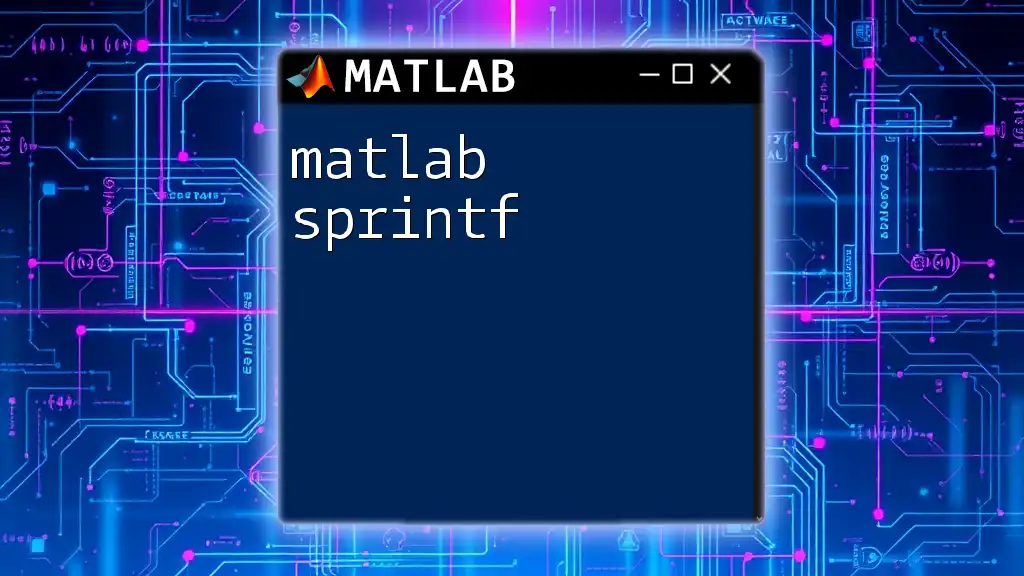
Special Characters and Escape Sequences
Common Escape Sequences
Sometimes, you might want to format your text further using escape sequences, which are special characters that have a unique meaning. The most common escape sequences are:
- `\n` for a new line,
- `\t` for a tab.
Using these can greatly improve the readability of your outputs. For instance:
fprintf('Hello,\nWorld!\n');
This code will be displayed as:
Hello,
World!
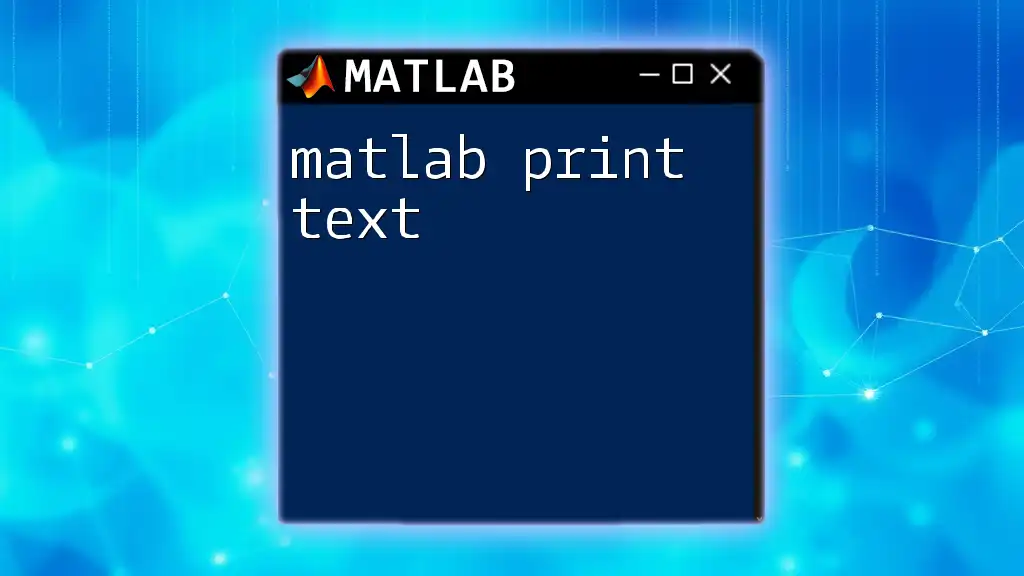
Advanced Features of `printf`
Combining Multiple Specifiers
One of the strengths of `printf` is the ability to combine multiple format specifiers in a single statement, allowing you to present multiple pieces of information succinctly. For example:
a = 5; b = 7.12345; str = 'test';
fprintf('Integer: %d, Float: %.2f, String: %s\n', a, b, str);
This outputs "Integer: 5, Float: 7.12, String: test", showcasing how different data types can be formatted and presented together in an informative manner.
Using `printf` in Loops
The `printf` function is also extremely useful when utilized within loops. This allows for dynamic output based on iterative processes. Consider the following example:
for i = 1:5
fprintf('Iteration: %d\n', i);
end
This code will print:
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Iteration: 5
By using loops, you can efficiently display progress or other dynamic information.
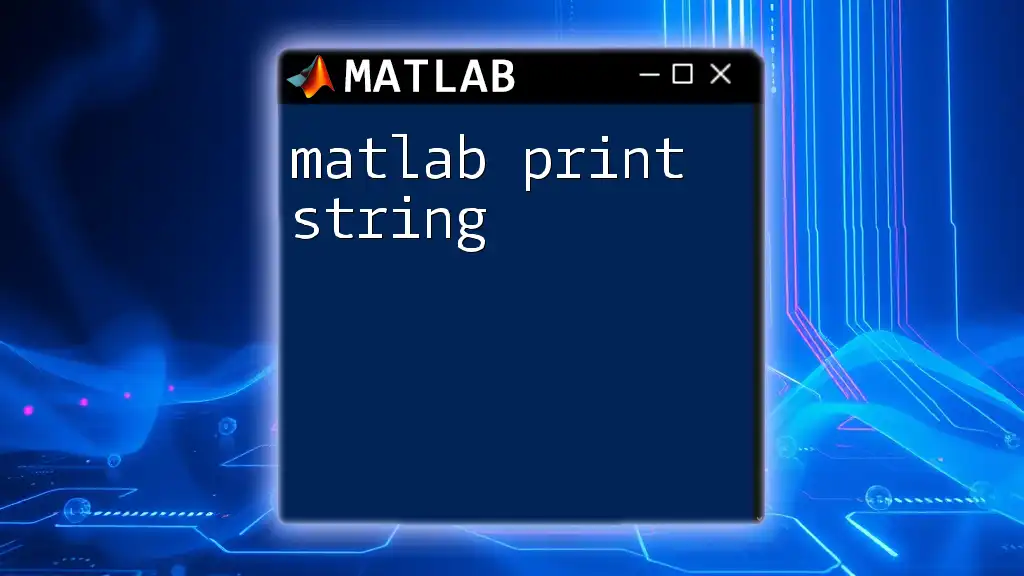
Common Mistakes and Troubleshooting
Common Errors
When using `printf`, some typical errors you might encounter include mismatched format specifiers and variables. For example, attempting to use a string specifier with an integer variable, like so:
number = 100;
fprintf('The number is: %s\n', number); % Incorrect
This will throw an error because `%s` expects a string, not an integer.
Debugging Tips
When your output does not appear as expected, make sure to check the following:
- Verify that the format specifiers match the data types of the variables being passed.
- Ensure that you are not missing any necessary newline or tab escape sequences.
Debugging these elements can save you a significant amount of time and frustration.
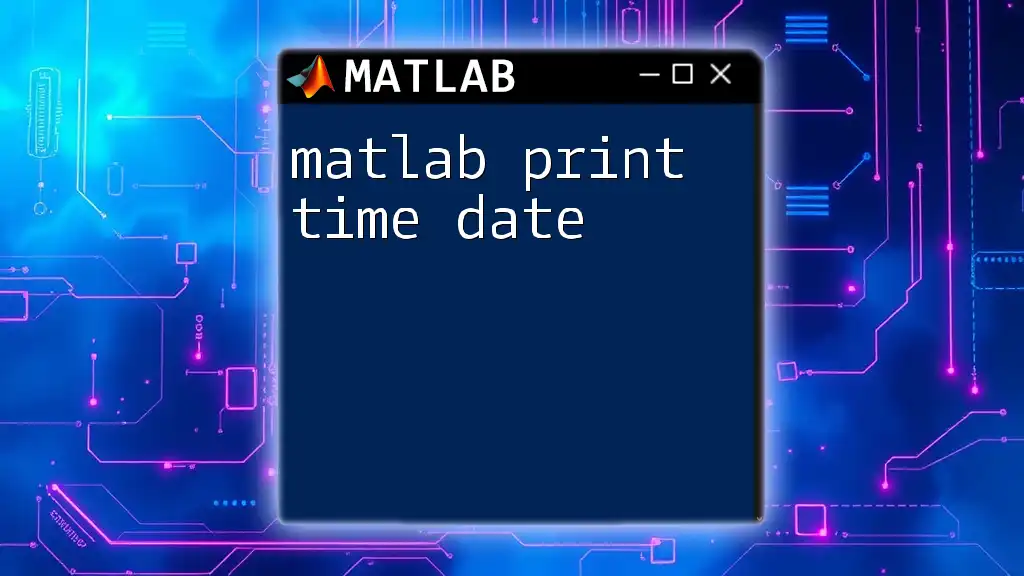
Conclusion
The `matlab printf` function is a versatile and essential tool for displaying formatted output in MATLAB. Understanding how to effectively use format specifiers, control output width and precision, and apply escape sequences can significantly enhance your ability to communicate your results clearly. Practicing with these concepts will improve your coding skills and ensure your outputs are both informative and aesthetically pleasing.
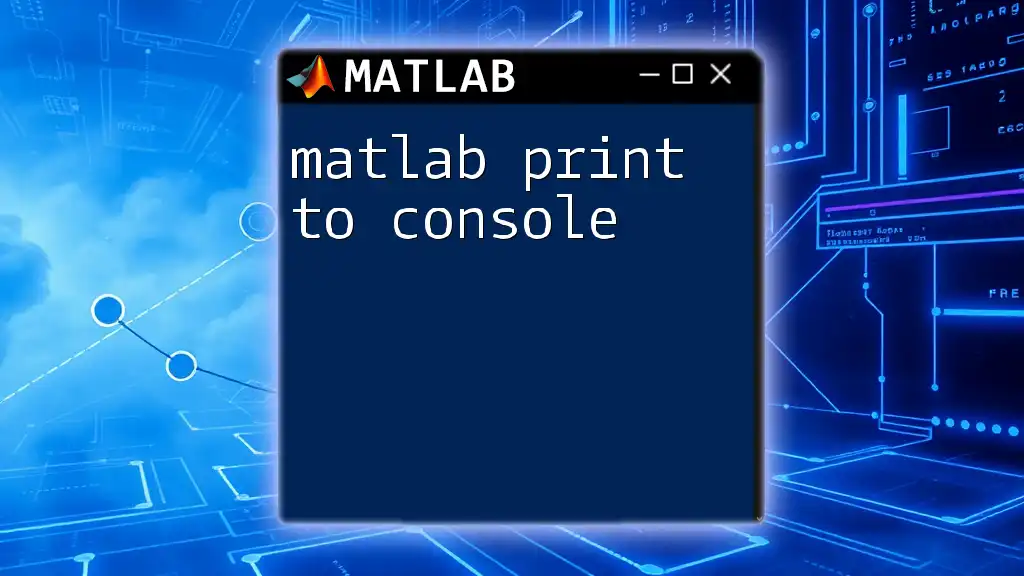
Additional Resources
For those looking to deepen their understanding of `matlab printf` and other commands, consider referring to the official MATLAB documentation or joining online forums dedicated to MATLAB programming. Engaging with a community can provide valuable insights and additional learning opportunities.
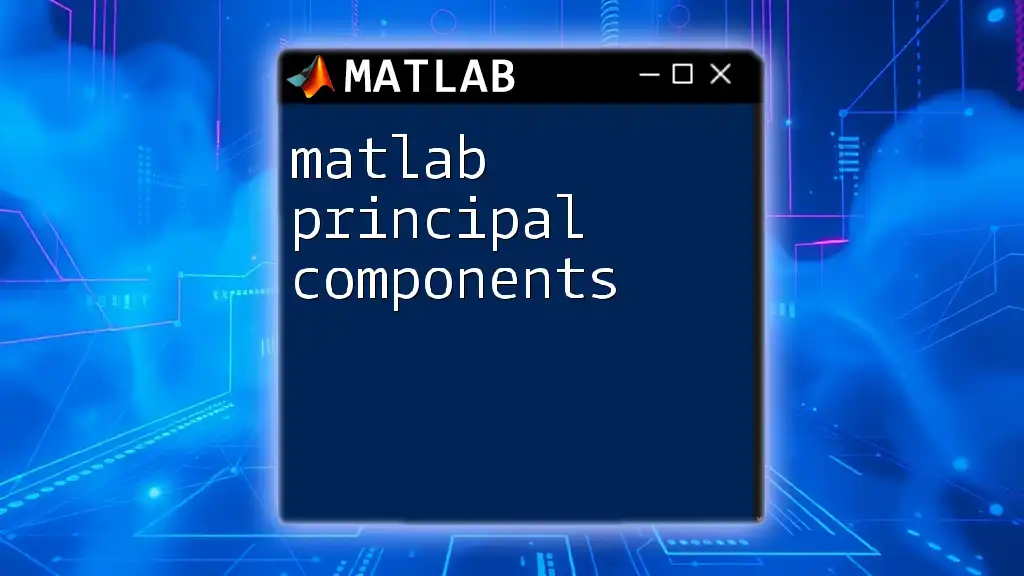
Call to Action
If you found this guide helpful and wish to explore more about MATLAB commands, consider enrolling in our courses. We offer a wide range of tutorials aimed at enhancing your MATLAB skills efficiently and effectively. Join us today to elevate your programming prowess!