Matlab graphs are essential for visualizing data and results, allowing users to create various types of plots for effective analysis and presentation.
Here’s a simple example to create a basic 2D line graph in Matlab:
x = 0:0.1:10; % Define the x-axis data
y = sin(x); % Compute the y-axis data using the sine function
plot(x, y); % Create the plot
title('Sine Wave'); % Add title to the graph
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
grid on; % Enable grid for better readability
Understanding the Basics of MATLAB Graphs
What is a MATLAB Graph?
A MATLAB graph is a visual representation of data that allows users to interpret complex information efficiently. By plotting data points in various formats, users can draw insights, identify trends, and communicate results clearly. Understanding how to create and manipulate graphs in MATLAB is vital for anyone working with data analysis or visualization.
Why Use MATLAB for Graphing?
Using MATLAB for graphing comes with multiple benefits that set it apart from other software. MATLAB is particularly powerful due to its built-in functions, ease of use, and the ability to handle large datasets. Common uses include academic research, engineering simulations, and data exploration in various scientific domains.
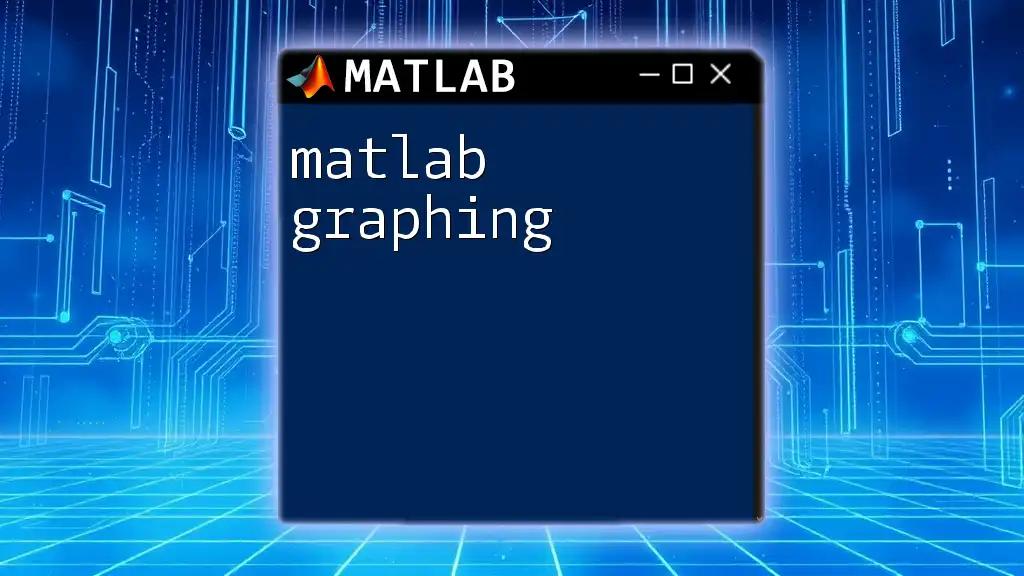
Getting Started with MATLAB Graphs
Setting Up Your MATLAB Environment
Before creating graphs, ensure MATLAB is installed on your device. Launch the application to navigate through its user-friendly interface, which consists of the Command Window, workspace, figure window, and editor. Familiarizing yourself with these components sets the groundwork for effective graphing.
Basic Graphing Commands
The `plot` Function
At the core of MATLAB graphing is the `plot` function. This command is used to create 2D line plots. The general syntax is `plot(X, Y)`, where `X` and `Y` are vectors of equal length representing the x-coordinates and corresponding y-coordinates of the points.
For example, to plot a sine wave, you can use:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
This script creates a simple line graph depicting a sine wave across the interval of 0 to 10.
Other Basic Commands
- title: To add a title to your graph, use the `title` function.
- xlabel & ylabel: Label the axes with `xlabel` and `ylabel` for better comprehension.
- grid: Activate grid lines for enhancing readability by using the `grid` command.
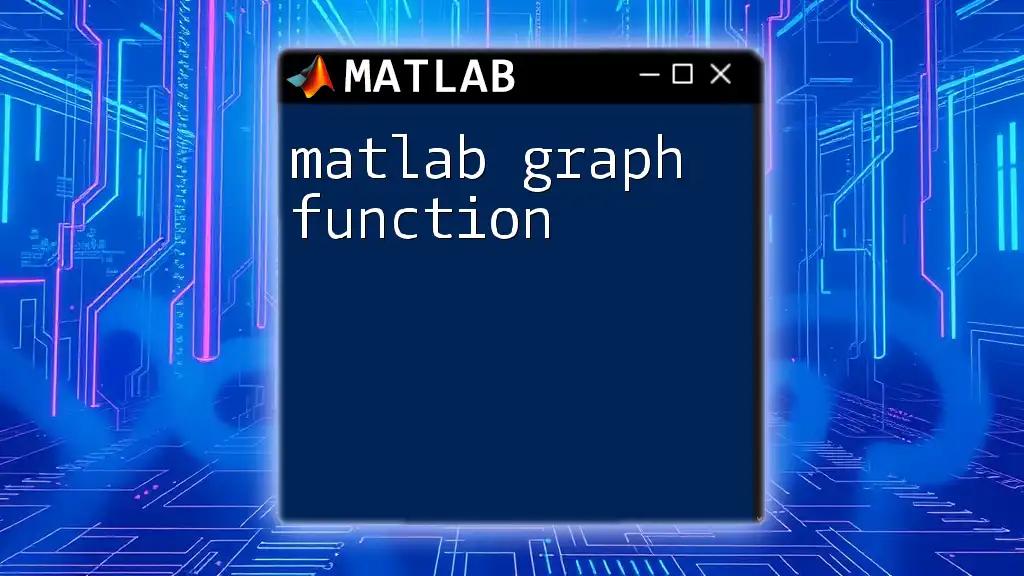
Types of MATLAB Graphs
2D Graphs
Line Graphs
Line graphs are the most common type of plot used in MATLAB. They effectively depict trends over time or another continuous variable. To create a graph with multiple lines, you can use the following example:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, 'r', x, y2, 'b--');
title('Sine and Cosine Waves');
legend('sin(x)', 'cos(x)');
In this code, two functions are plotted on the same graph, using different colors and line styles to distinguish between them.
Scatter Plots
Scatter plots represent individual data points on a Cartesian plane, making them valuable for visualizing relationships between variables. Here’s how you can create a random scatter plot:
x = rand(1, 100);
y = rand(1, 100);
scatter(x, y);
title('Random Scatter Plot');
This example generates a scatter plot with 100 random points, providing insights into data distribution.
3D Graphs
Surface Plots
Surface plots represent three-dimensional data, allowing users to visualize the relationship between three variables. Here's an example:
[X, Y] = meshgrid(-5:0.1:5, -5:0.1:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
title('3D Surface Plot');
This script generates a surface plot based on a mathematical function, revealing intricacies in the data's three-dimensional structure.
Mesh Plots
Similar to surface plots, mesh plots display three-dimensional data; however, they emphasize the edges of the surface grid rather than coloring the surface. To create a mesh plot, use:
mesh(X, Y, Z);
title('3D Mesh Plot');
This representation provides a clearer view of the structure and contour of the data.
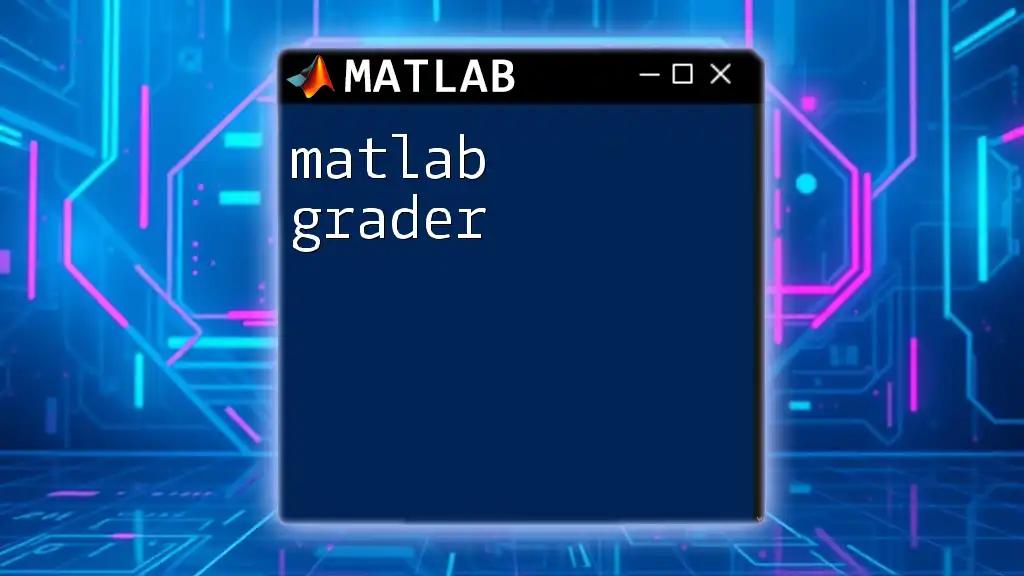
Customizing Your Graphs
Changing Colors and Line Styles
Customizing your graphs enhances clarity and aesthetics. You can change colors and line styles using additional parameters in the `plot` command. For instance:
plot(x, y, 'g:', 'LineWidth', 2);
In this example, the line is set to a green dotted style with increased line width, making it stand out.
Adding Annotations
Annotations guide viewers through the graph, pointing out significant features or data points. You can add text to your plot using:
text(5, 0, 'Sine wave peak', 'Color', 'red');
This command places a textual annotation in red at the specified coordinates, enhancing the graph's informative value.
Setting Axis Limits
Setting axis limits is crucial for focusing on a specific data range. Achieve this using the `xlim` and `ylim` functions:
xlim([0 10]);
ylim([-1 1]);
Defined limits ensure that your graph accurately reflects the data's significance without unnecessary clutter.
Adding Legends
Legends help viewers interpret different elements represented in your graph. Create a legend using the `legend` command:
legend({'Sine', 'Cosine'}, 'Location', 'best');
This code adds a legend indicating which line corresponds to which function, ensuring clarity.
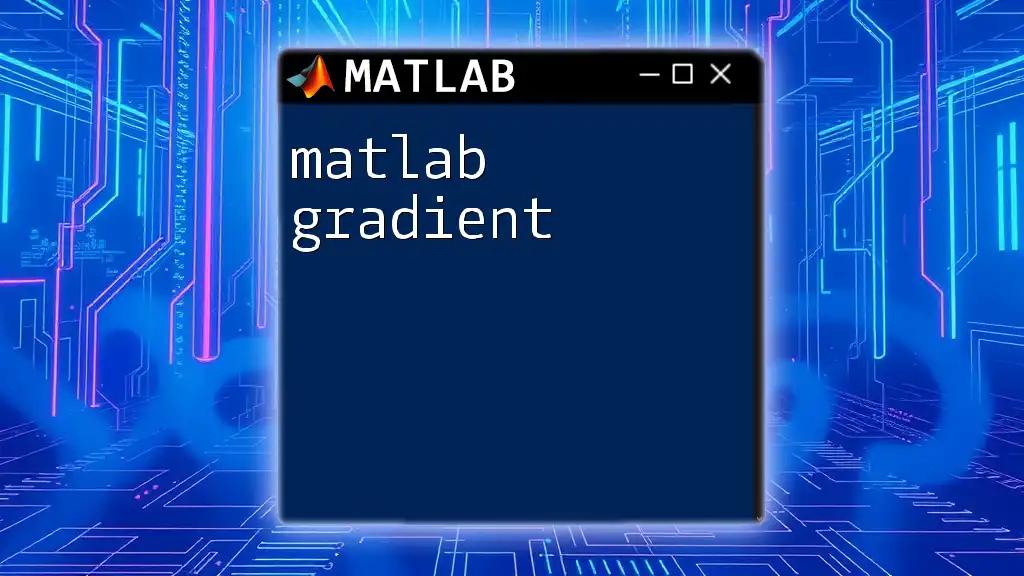
Practical Tips for Effective Graphing
Choosing the Right Type of Graph
Selecting the appropriate graph type is essential in effectively conveying data insights. For example, use line graphs to showcase trends, scatter plots to identify correlations, and surface plots for multidimensional relationships. Adapting your graph type based on the data’s nature can significantly improve the understanding of the information presented.
Using Predefined Styles
MATLAB also provides predefined styles to conserve time and maintain consistency in visualizations. You can apply default settings globally, like this:
set(groot, 'DefaultLineLineWidth', 2);
set(groot, 'DefaultAxesFontSize', 14);
This ensures that all subsequent plots maintain the set line width and font size, streamlining your graphing process.
Debugging Common Graphing Issues
Many users face challenges while graphing, whether it’s mismatched vector lengths or command syntax errors. To troubleshoot, ensure that your data vectors are of equal length and check for typos in commands, as these are common issues that can disrupt graph creation.
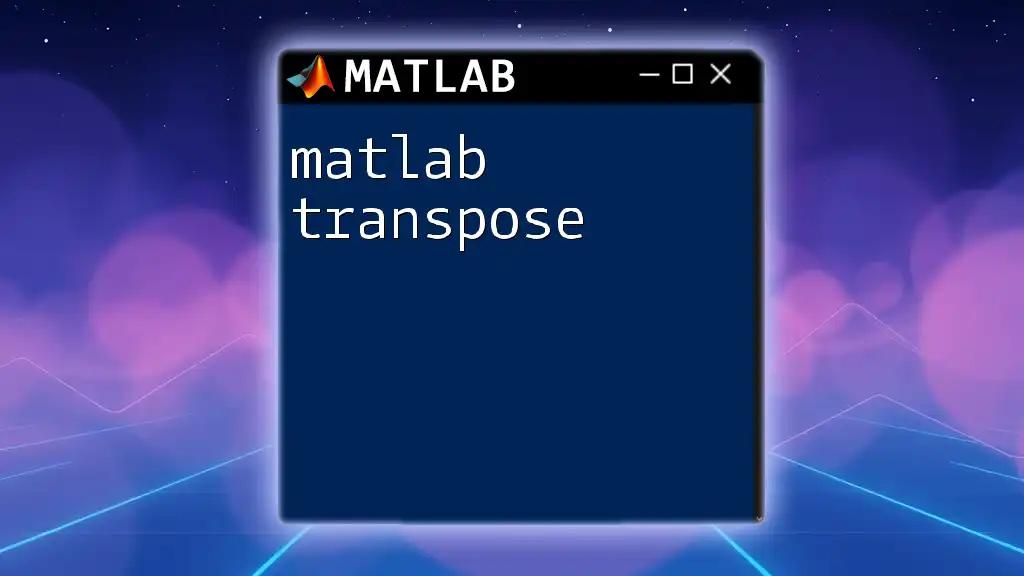
Advanced Topics in MATLAB Graphs
Creating Animated Graphs
Animated graphs add a dynamic element to visualizations, capturing the audience's attention. You can create simple animations through loops in MATLAB:
for k = 1:100
plot(x, sin(x + k/10));
pause(0.1);
end
This snippet cycles through 100 updates of the sine function, creating a visually engaging effect.
Exporting Graphs
After creating your graphs, you often need to save them for reports or presentations. MATLAB allows exporting graphs in various formats. You can save your active graph using:
saveas(gcf, 'myGraph.png');
This command saves the current figure window as a PNG file, perfect for sharing and incorporating into documentation.
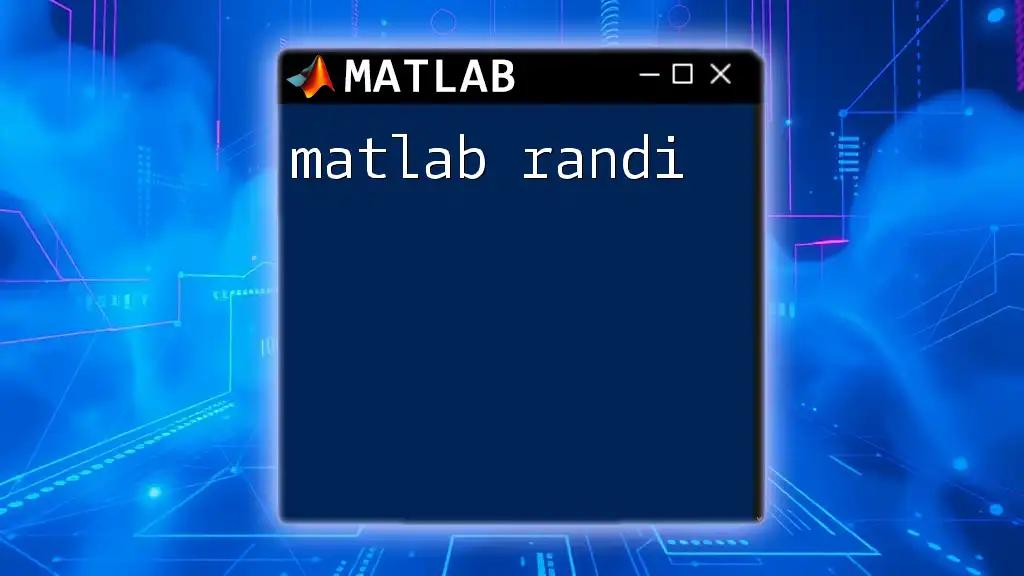
Conclusion
Creating effective MATLAB graphs is an essential skill in data visualization that can dramatically enhance your analytical capabilities. From basic line plots to advanced 3D visualizations, understanding how to graph in MATLAB empowers you to better explore and communicate data. As you become adept at these techniques, continue to experiment with different customizations and styles for even more impactful results. Explore beyond the basics, leverage MATLAB’s extensive documentation, and consider engaging with additional resources to further hone your skills in graphing and data analysis.