The MATLAB square root function calculates the principal square root of a number or array of numbers using the `sqrt` command.
% Calculate the square root of a number
result = sqrt(16); % result will be 4
Understanding Square Roots
Definition of Square Root
A square root of a number is a value that, when multiplied by itself, gives the original number. For example, the square root of 25 is 5 since \(5 \times 5 = 25\). In mathematical terms, if \(y\) is the square root of \(x\), then \(y^2 = x\). Understanding square roots is crucial in various fields, such as physics, engineering, and finance, as it helps in solving equations and analyzing data.
Properties of Square Roots
Understanding the properties of square roots aids in their application.
-
Non-negativity: The square root of a non-negative number is always non-negative. This means that \(\sqrt{x} \geq 0\) for any \(x \geq 0\).
-
Multiplicative Property: The square root function has a multiplicative property: \(\sqrt{a \cdot b} = \sqrt{a} \cdot \sqrt{b}\). This is useful when dealing with products in calculations.
-
Square Root of Negative Numbers: When attempting to extract a square root from negative numbers, we enter the realm of complex numbers. The standard definition of square roots does not suffice for negative values.

MATLAB Square Root Function
Introduction to the `sqrt` Function
In MATLAB, the primary function for computing the square root is `sqrt`. This built-in function is straightforward and highly effective for both scalar and array inputs.
Syntax of `sqrt` Function
The syntax for the `sqrt` function in MATLAB is simple:
result = sqrt(X);
-
Parameters:
- X: This can be a scalar value, vector, or matrix for which you want to calculate the square root.
-
Returns: The function returns the square root of each element in X. If X is negative, MATLAB will return complex results.
Example of `sqrt` Function
Calculating the square root of a single value is straightforward:
% Calculate the square root of a single number
result = sqrt(25); % result will be 5
Working with Arrays
Vector Input in `sqrt`
MATLAB efficiently handles array operations, enabling users to compute square roots for vectors seamlessly. Consider the following example:
% Calculate square roots of an array
array = [1, 4, 9, 16, 25];
resultArray = sqrt(array); % resultArray will be [1, 2, 3, 4, 5]
In this case, `resultArray` will contain the square roots of each element in the array.
Matrix Input in `sqrt`
Moreover, when working with matrices, MATLAB applies the `sqrt` function element-wise. Here's an example of using a 2D matrix:
% Calculate square roots of a 2D matrix
matrix = [1, 4; 9, 16];
resultMatrix = sqrt(matrix); % resultMatrix will be [1, 2; 3, 4]
In this instance, `resultMatrix` will store the square roots of each element in the matrix.
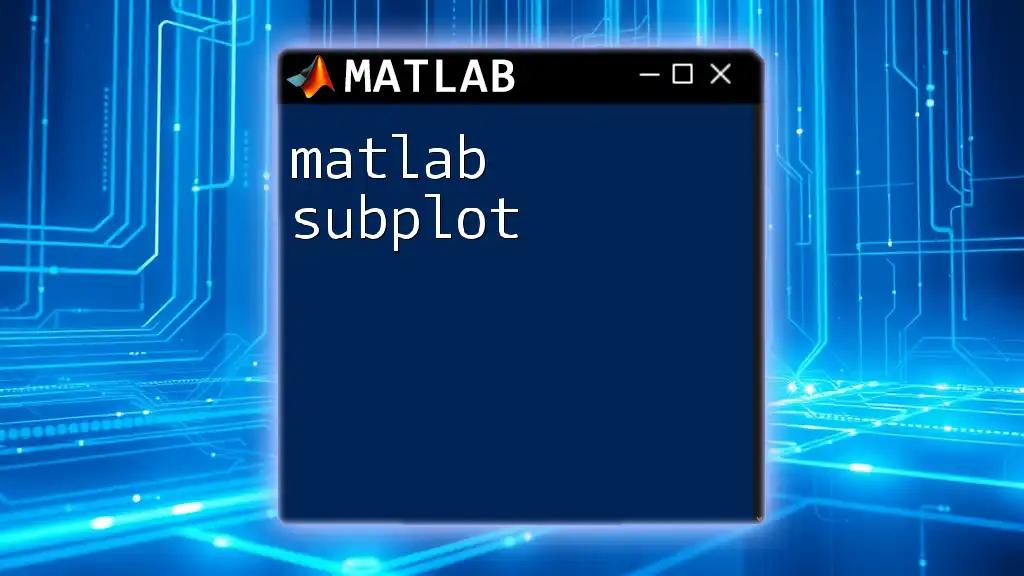
Handling Complex Numbers
Square Roots of Negative Numbers
When dealing with negative numbers, MATLAB allows for complex results. The imaginary unit i represents the square root of -1. For example, using `sqrt` on a negative number:
% Calculate square root of a negative number
resultComplex = sqrt(-16); % resultComplex will be 4*i
Thus, if you attempt to compute the square root of -16, MATLAB will return \(4i\), indicating the presence of an imaginary component.
Visualization of Complex Results
Visualizing complex results can enhance understanding. You can plot the imaginary parts of square roots of negative inputs as follows:
% Plotting the square root of complex numbers
x = -10:1:10;
y = sqrt(x);
plot(x, imag(y), 'r'); % only plotting the imaginary part
title('Square Root of Complex Numbers');
xlabel('Input');
ylabel('Imaginary Part');
This code helps to visualize how the square root behaves in the complex domain.
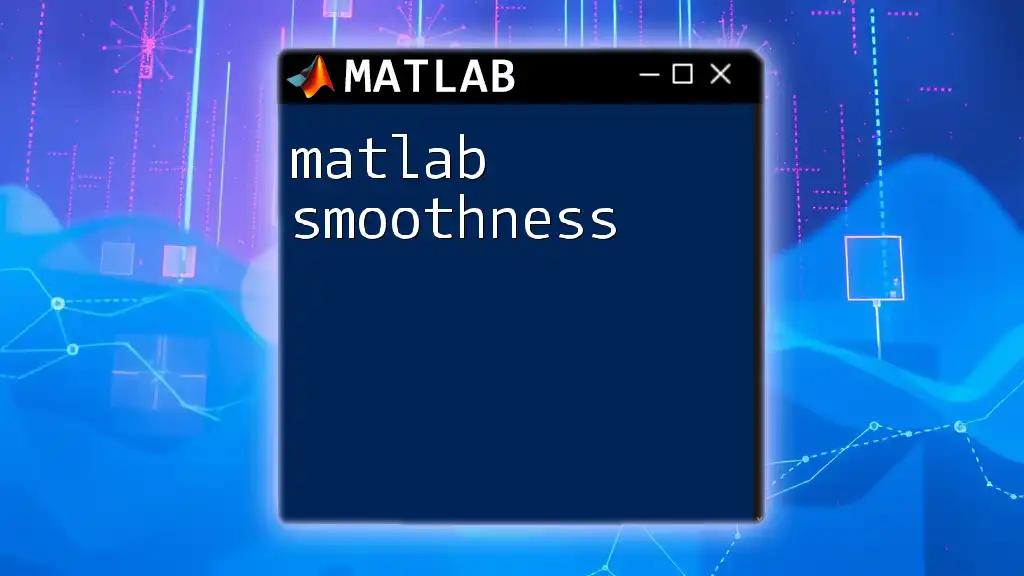
Error Handling in MATLAB
Common Errors
When using the `sqrt` function, users may encounter common errors. It’s essential to grasp the nature of these mistakes and how MATLAB responds. For instance, attempting to compute the square root of a non-numeric value results in an error:
% Attempt to calculate square root of a non-numeric value
invalidResult = sqrt('text'); % This will return an error
If you execute the above line, MATLAB will provide an error message indicating that input must be numeric.
Best Practices for Using `sqrt`
To prevent issues, consider these best practices:
- Validate Inputs: Always check input values before applying the `sqrt` function. Ensure they are non-negative if you only want to use real numbers.
% Check if input is negative before calculation
x = -4;
if x < 0
disp('Input is negative. Use complex numbers.');
else
result = sqrt(x);
end
This code snippet highlights preventative measures to ensure you're prepared to process negative inputs correctly.
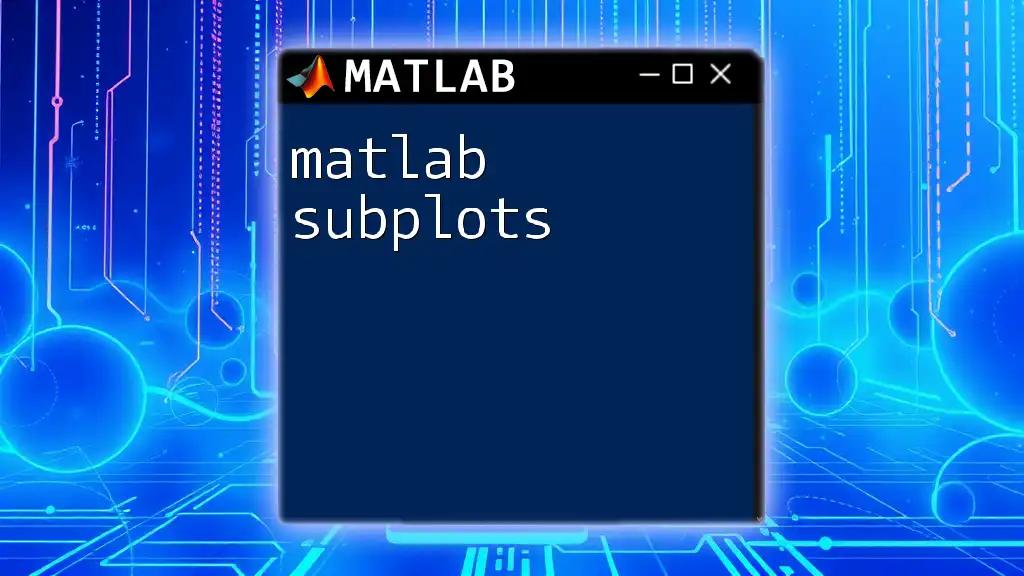
Conclusion
Mastering the MATLAB square root function is essential for anyone looking to deepen their programming and mathematical skills. With the ability to handle scalar values, arrays, and even complex numbers, `sqrt` is a versatile tool in the MATLAB toolbox. Understanding its properties, error handling, and visualization capabilities can greatly enhance your efficiency as you tackle more complex computational challenges.