In MATLAB, the `str` function is commonly used to convert numeric values to their string representation.
Here's a code snippet that demonstrates this:
num = 123;
strValue = str(num);
disp(strValue); % This will display '123' as a string
Understanding Strings in MATLAB
Definition of Strings
In MATLAB, strings are essential for handling text data. Strings can be classified into two primary types: character arrays and string arrays.
-
Character Arrays: Represent strings as arrays of characters, useful for many traditional programming tasks. For instance:
charArray = 'Hello, World!';
-
String Arrays: Introduced in MATLAB R2016b, these provide a more modern way to handle text data. This format allows for more natural integration with MATLAB functions and operations. Here’s how to create a string array:
stringArray = "Hello, World!";
Understanding the differences between these two types of strings is critical, especially since string arrays support a more extensive range of operations.
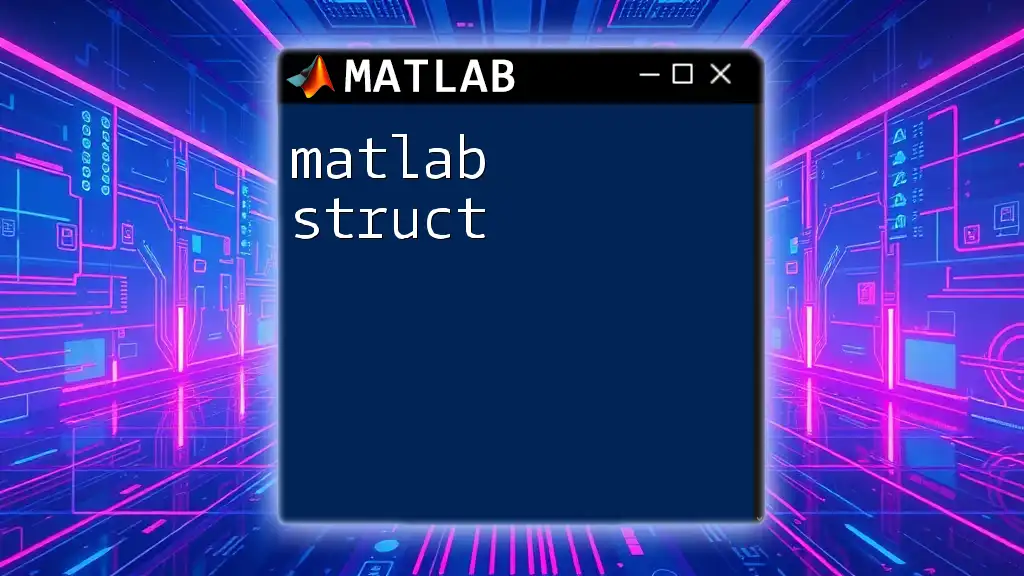
Key String Functions
`strcat`
The `strcat` function is utilized to concatenate strings or arrays of strings. It’s a simple yet powerful tool for merging textual data.
Syntax:
result = strcat(str1, str2);
Example Code Snippet:
str1 = 'Hello';
str2 = 'World';
result = strcat(str1, ', ', str2);
In this example, the output will be `'Hello, World'`. This function is particularly handy when assembling user input or generating formatted output messages.
`strfind`
The `strfind` function searches for occurrences of a substring within a string. This function returns the starting index of each occurrence, making it invaluable for text analysis and manipulation.
Syntax:
index = strfind(str, substring);
Example Code Snippet:
str = 'MATLAB is great for engineering.';
index = strfind(str, 'great');
In this case, the output will indicate the starting position of the substring `'great'` within the larger string. This function can be essential for searching through text files or validating user inputs.
`strcmp` and `strcmpi`
`strcmp` and `strcmpi` are functions used for comparing two strings — the former is case-sensitive, while the latter is case-insensitive.
Syntax:
result = strcmp(str1, str2); % Case-sensitive
result = strcmpi(str1, str2); % Case-insensitive
Example Code Snippet:
result1 = strcmp('Hello', 'hello'); % returns false
result2 = strcmpi('Hello', 'hello'); % returns true
Using these functions is crucial for validating user input or processing commands, especially in interactive MATLAB applications.
`strrep`
The `strrep` function replaces occurrences of a specified substring within a string. This function is extremely useful when you need to modify existing strings.
Syntax:
newStr = strrep(originalStr, oldSubstring, newSubstring);
Example Code Snippet:
str = 'I like MATLAB';
newStr = strrep(str, 'like', 'love');
In this case, `"I love MATLAB"` will be the output. You can use this function to correct misspellings, replace terms, or format strings to fit specific requirements in reporting or display.
`sprintf`
The `sprintf` function formats data into a string neatly. This function allows for creating structured outputs, such as reports or logs, which can include numerical and string data formatted in a user-friendly way.
Syntax:
formattedStr = sprintf(formatSpec, A, ...)
Example Code Snippet:
name = 'Alice';
age = 30;
result = sprintf('%s is %d years old.', name, age);
In this scenario, the string output will be `'Alice is 30 years old.'`. This capability facilitates the integration of variable data into text, making it incredibly valuable for generating dynamic messages in your applications.
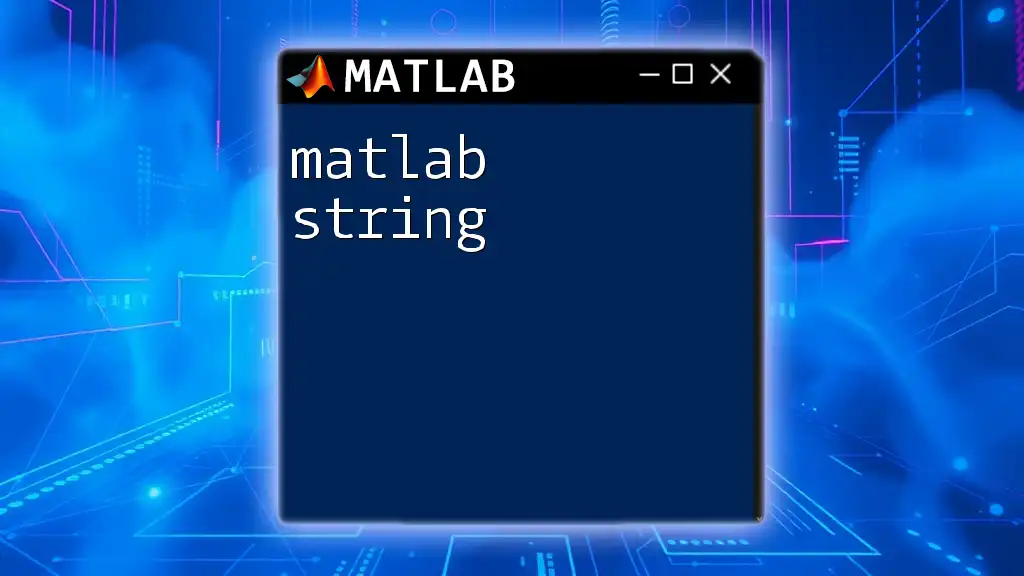
Advanced String Manipulation
String Comparison Methods
In addition to `strcmp` and `strcmpi`, MATLAB also offers `strncmp` and `strncmpi`, which compare only the first N characters of two strings, providing more flexibility when dealing with partial matches.
Example Code Snippet:
result = strncmp('abcdef', 'abc', 3); % returns true
These functions can be beneficial when checking prefixes or portions of longer strings, enhancing your text processing capabilities.
Regular Expressions with `regexp`
For more complex string search and manipulation tasks, `regexp` utilizes regular expressions, allowing for powerful and flexible patterns matching. It can extract portions of strings that conform to specific formats.
Syntax:
matches = regexp(inputStr, pattern, returnType);
Example Code Snippet:
str = 'Find 123, 456 and 789.';
numbers = regexp(str, '\d+', 'match');
In this case, the output will be an array of numbers found in the original string as strings: `{'123', '456', '789'}`. This capability is immensely beneficial for data extraction, such as parsing emails or phone numbers.
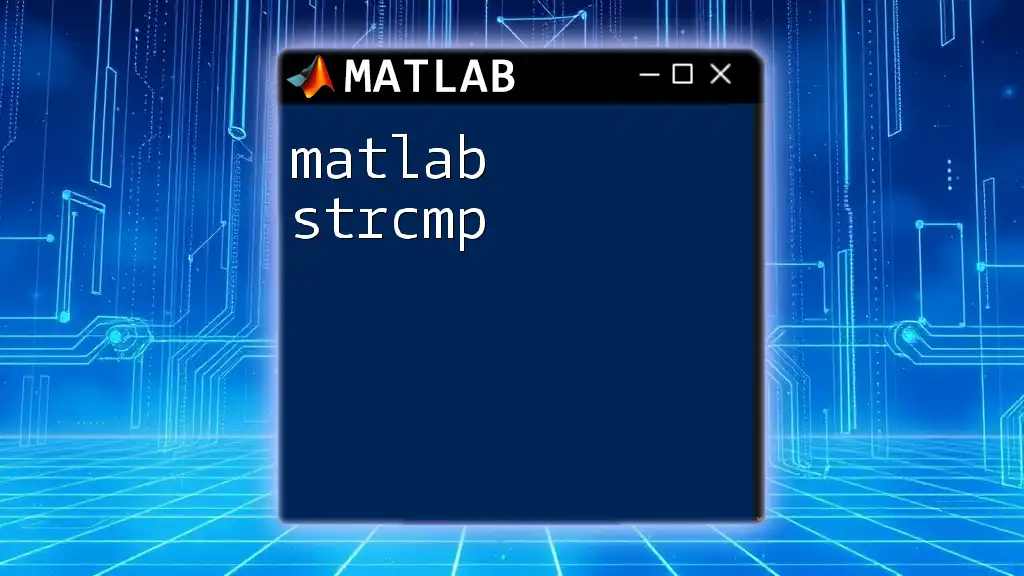
Practical Applications
GUI Applications
In MATLAB GUI programming, string functions are vital for handling textual input and output. User interface elements often rely on string manipulation for effective display and data processing.
Data Cleaning and Processing
String functions are integral to data cleaning tasks, especially when preparing data sets for analysis. For example, removing unwanted characters or adjusting text formats can be quite effectively accomplished using `strrep`, `regexprep`, or other `str` functions.
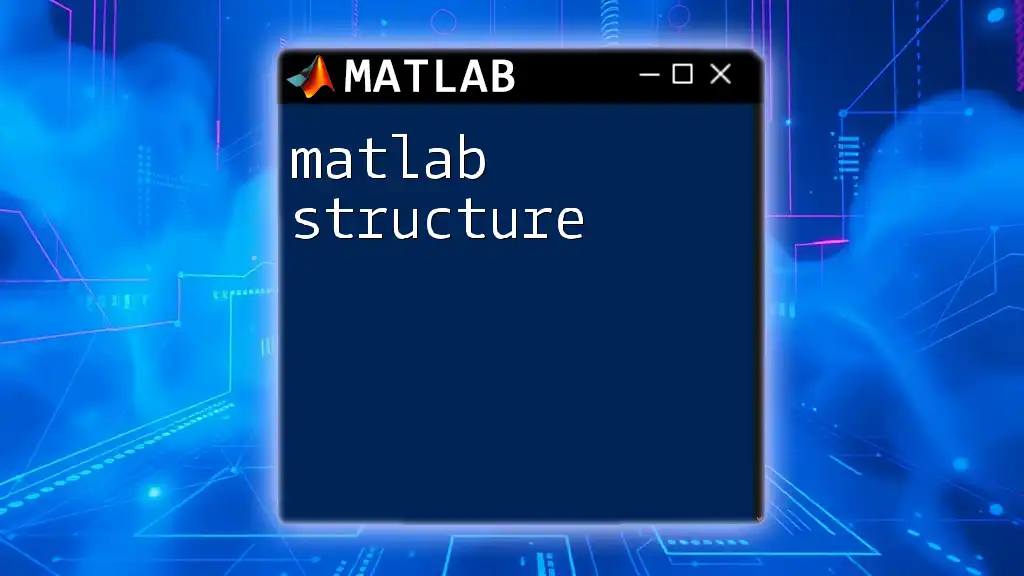
Conclusion
Mastering the `matlab str` functions is crucial for any MATLAB user who works with textual data. These functions allow for efficient string manipulation, thereby improving the overall efficiency and robustness of your code.
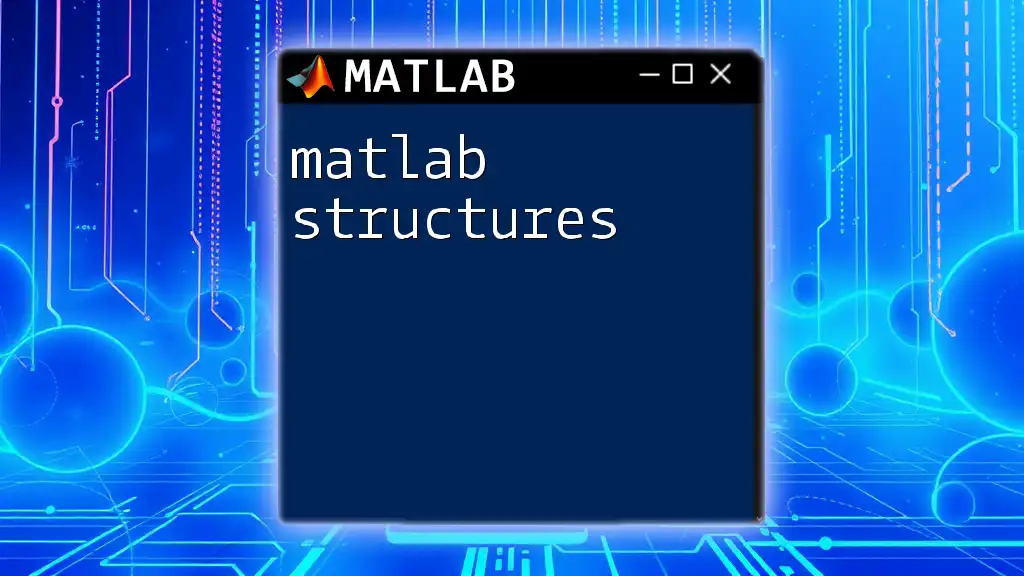
Additional Resources
For further learning, consider checking the official MATLAB documentation for each function mentioned. Engaging with practical exercises can also strengthen your understanding and proficiency with these essential tools in MATLAB.