The `strcmp` function in MATLAB is used to compare two strings for equality, returning true if they are identical and false otherwise.
Here’s a simple code snippet demonstrating its use:
str1 = 'Hello';
str2 = 'Hello';
isEqual = strcmp(str1, str2); % Returns true (1) since both strings are identical
Understanding String Comparison in MATLAB
What is String Comparison?
String comparison is an essential operation in programming that allows you to evaluate whether two sequences of characters are identical. This capability becomes paramount in scenarios such as validating user input, filtering data, or managing configuration settings. In MATLAB, the ability to compare strings is crucial for robust data analysis and manipulation.
Basic Concepts
In MATLAB, there are two primary types of strings:
- Character Arrays: Older data type that includes characters in a row (e.g., `'Hello'`).
- Strings: Introduced in newer versions of MATLAB, enclosed in double quotes (e.g., `"Hello"`).
Understanding the difference between these two types can be significant when performing comparisons, as specific functions and operations might behave differently.
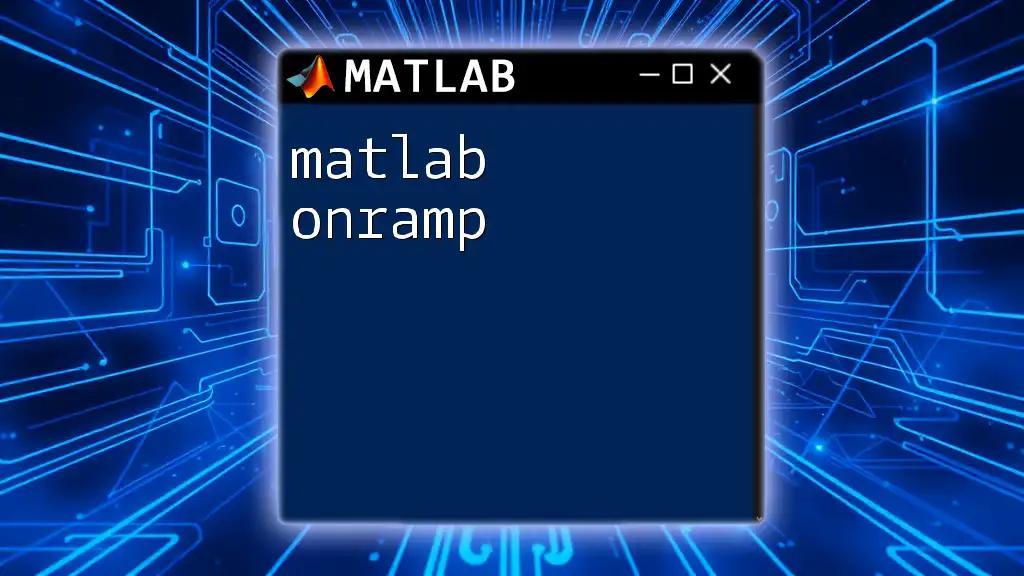
Introducing the strcmp Function
Syntax of strcmp
The `strcmp` function is designed to compare two strings. Its basic syntax is:
output = strcmp(str1, str2);
- str1: The first string or character array to compare.
- str2: The second string or character array to compare.
Return Value
The function returns:
- 1 (true): if the strings are identical.
- 0 (false): if the strings are not identical.
For example:
result = strcmp('Hello', 'Hello'); % Returns 1
This states that both strings are equal. In contrast:
result = strcmp('Hello', 'World'); % Returns 0
This indicates that the strings differ.
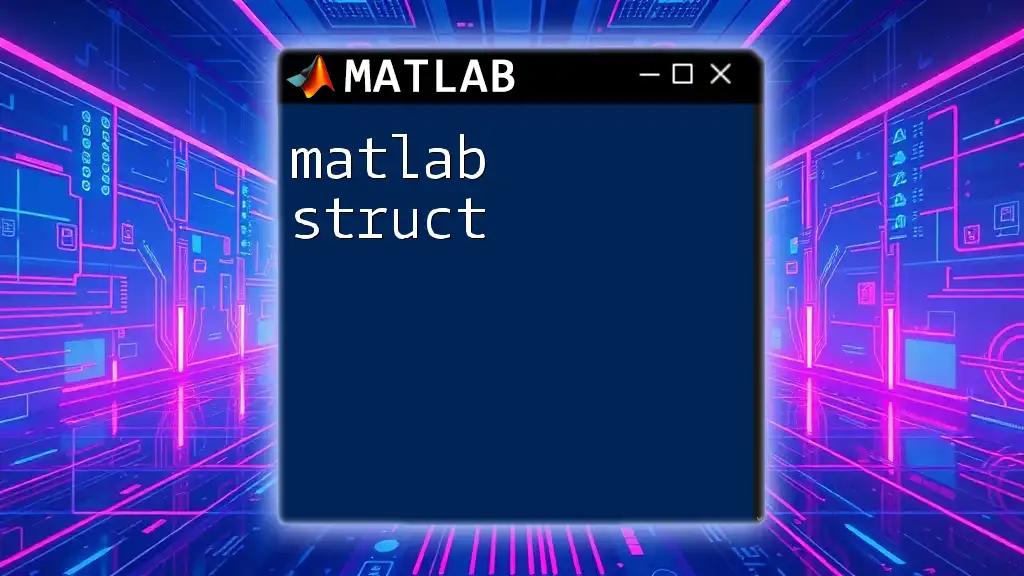
Basic Usage Examples
Comparing Two Identical Strings
To compare two identical strings using `strcmp`, take the following example:
result = strcmp('Hello', 'Hello'); % Returns 1
In this case, the function confirms that both strings are the same.
Comparing Two Different Strings
Use this example to demonstrate how `strcmp` responds to different strings:
result = strcmp('Hello', 'World'); % Returns 0
This shows that `strcmp` identifies the discrepancy between the two strings.
Case Sensitivity in Comparison
Case sensitivity is a critical factor in string comparisons. For instance:
result = strcmp('hello', 'Hello'); % Returns 0
Here, `strcmp` returns false because it treats uppercase and lowercase letters as different characters. It’s crucial to remember that `strcmp` is case-sensitive; this can lead to unexpected results if you don't account for it.
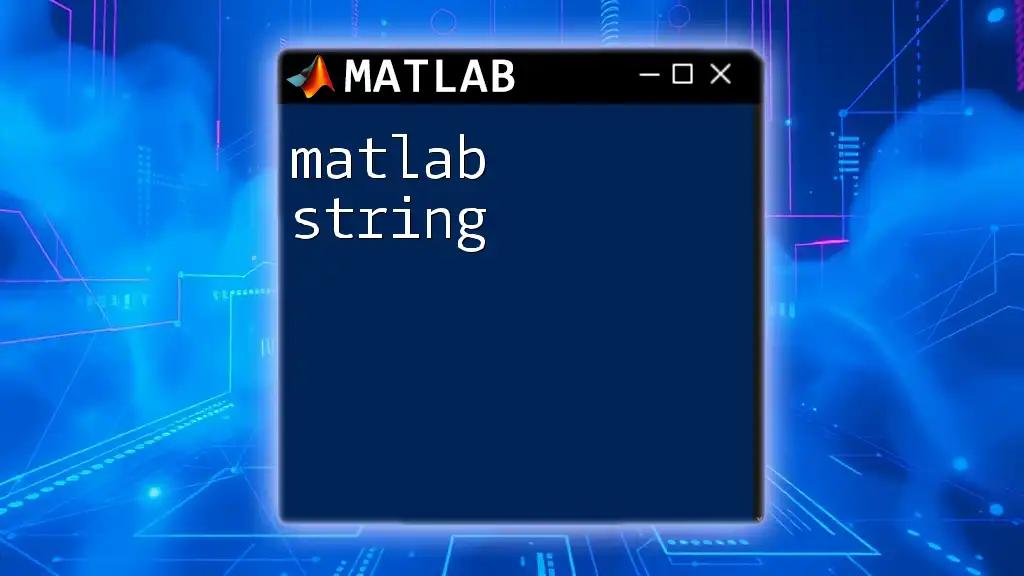
Advanced Features of strcmp
Comparing Multiple Strings
When dealing with multiple strings, you often need to check if one string matches any in a collection. Using cell arrays can help accomplish this task:
strings = {'apple', 'banana', 'cherry'};
result = strcmp('banana', strings); % Returns [0 1 0]
In this case, `strcmp` evaluates each element in the cell array, returning a logical array that indicates which strings match.
Performance Considerations
When working with large datasets, performance becomes a significant factor. `strcmp` is efficient for comparing strings due to its direct implementation. However, when tasked with comparing extensive lists of strings, ensure you’re applying best practices, such as avoiding unnecessary computations or iterations that can degrade performance.

Alternatives to strcmp
Using strcmpi for Case-Insensitive Comparison
If you require a case-insensitive comparison, `strcmpi` serves as an effective alternative. Its syntax is the same as `strcmp`:
result = strcmpi('Hello', 'hello'); % Returns 1
Utilizing `strcmpi` is particularly beneficial in user input scenarios, where capitalization can vary, but semantic meaning remains the same.
Brief Overview of Other String Functions
Besides `strcmp`, MATLAB offers various other functions for string comparison:
- strfind: Locates the indices of occurrences of a substring within a string.
- strncmp: Compares the first N characters of two strings; useful when evaluating only part of a string.
- strncmpi: Similar to `strncmp`, but case-insensitive.
Each of these functions is valuable in specific situations, and understanding when to use them can enhance your string manipulation skills.
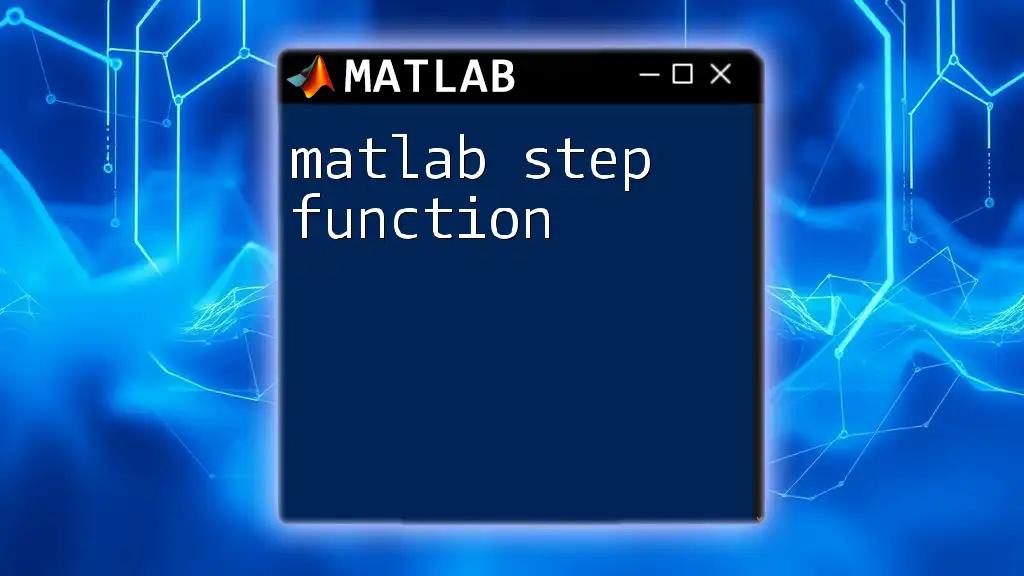
Debugging Common Issues
Common Errors When Using strcmp
It’s easy to encounter errors while using `strcmp`, especially when comparing differing data types. Always ensure that both inputs are strings or character arrays. Comparing a string with a numeric value will produce an error.
Troubleshooting Tips
If you receive unexpected results from `strcmp`, consider checking the following:
- Confirm that both strings are indeed of the same type (either both character arrays or both string objects).
- Be mindful of invisible characters or leading/trailing spaces that may cause strings to appear identical visually, but differ upon comparison.
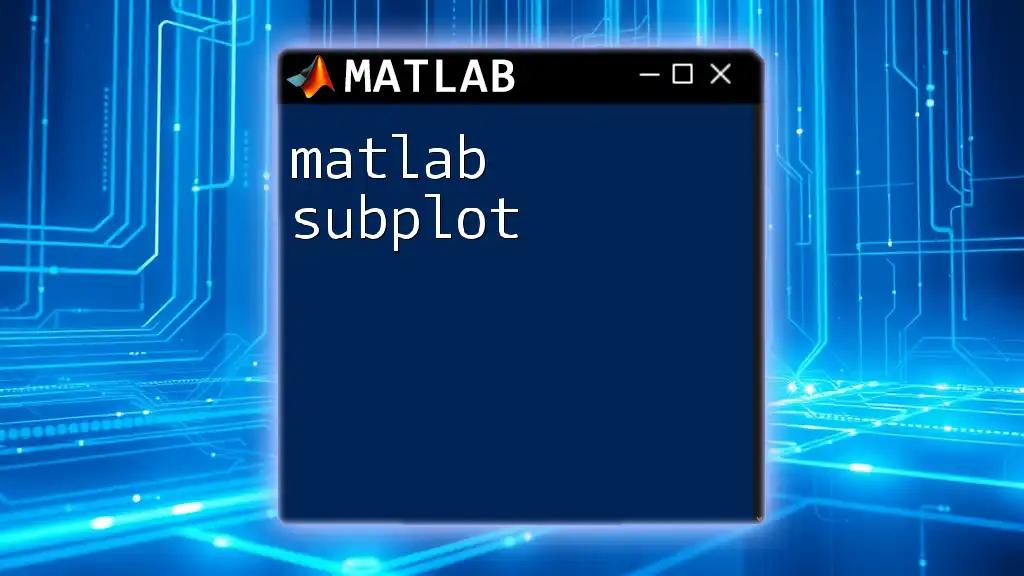
Conclusion
Understanding matlab strcmp is vital for effectively handling string comparisons within MATLAB. Whether you are validating user input, managing data, or developing sophisticated algorithms, mastering this function will empower you to enhance the functionality of your MATLAB applications.
As you continue to explore string operations, consider engaging with the wider MATLAB community or diving into advanced string handling to further refine your programming skills.
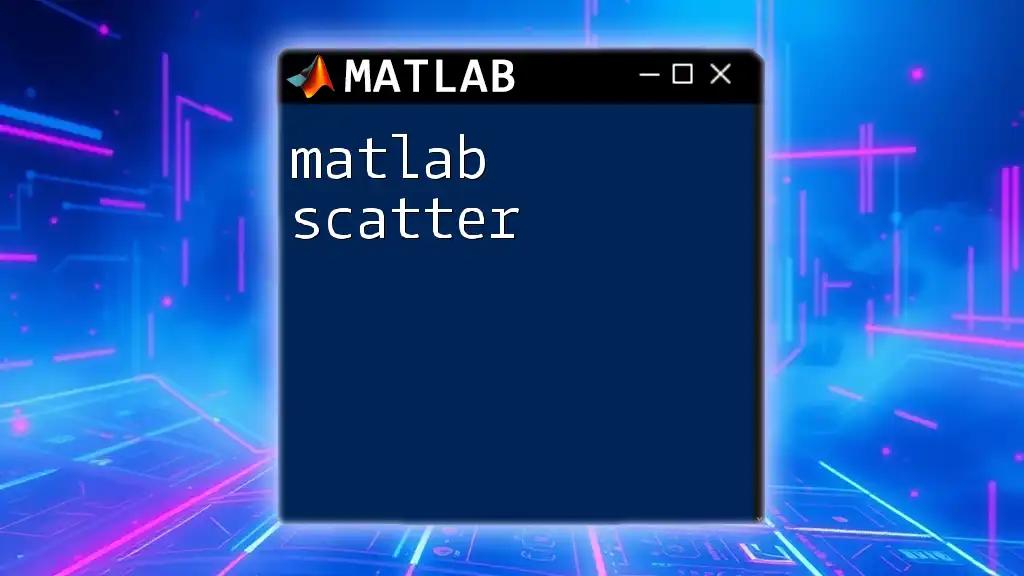
Additional Resources
For further learning, check out the official MATLAB documentation, recommended books, and tutorials available online. Engaging with resources can broaden your understanding and foster a deeper appreciation for string manipulation in MATLAB.
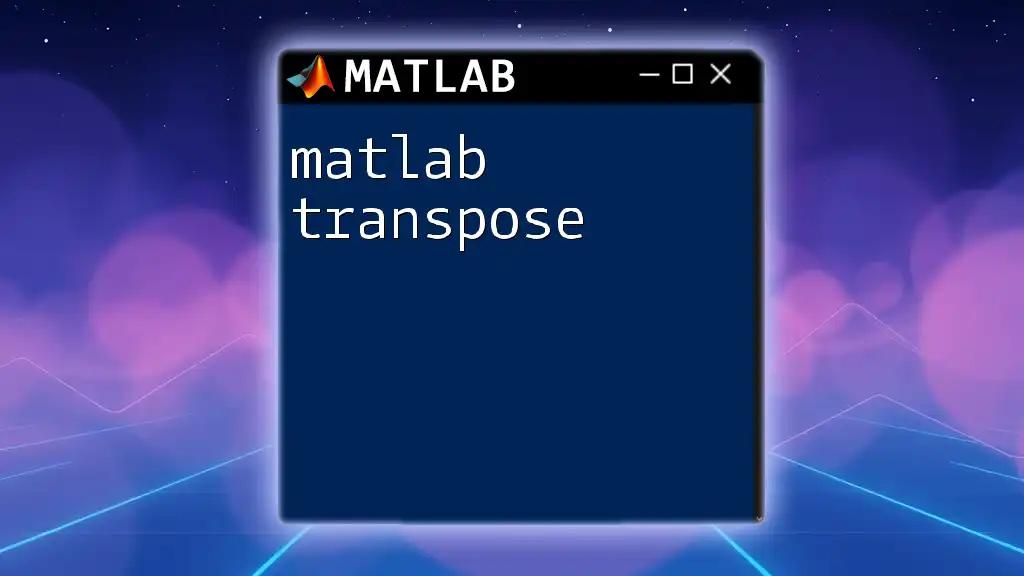
Call to Action
To solidify your knowledge of MATLAB commands like `strcmp`, consider signing up for our training sessions, and don’t forget to follow our blog for more insightful coding tips and tricks!