Certainly! The `readtable` function in MATLAB is used to import data from a file and store it as a table, making it easier to manage and analyze.
Here's a code snippet demonstrating how to use the `readtable` command:
data = readtable('filename.csv');
Getting Started with MATLAB Tables
What is a MATLAB Table?
A MATLAB table is a data type that provides a convenient way to store column-oriented or tabular data. Tables are particularly useful for handling heterogeneous data sets, allowing you to manage data with different types (numeric, text, categorical) in each column. This capability makes tables ideal for statistical analysis and data modeling, where data often comes from various sources and may differ in format.
Creating a MATLAB Table
You can create tables using existing data in MATLAB. To illustrate, consider using cell arrays or data arrays. The following code snippet demonstrates how to define a table from a cell array:
data = {1, 'John', 85; 2, 'Mary', 90; 3, 'Paul', 78};
T = cell2table(data, 'VariableNames', {'ID', 'Name', 'Score'});
In this example, we create a table T, where the first column indicates the unique ID, the second lists names, and the third shows scores. The 'VariableNames' option allows you to specify custom names for each column.
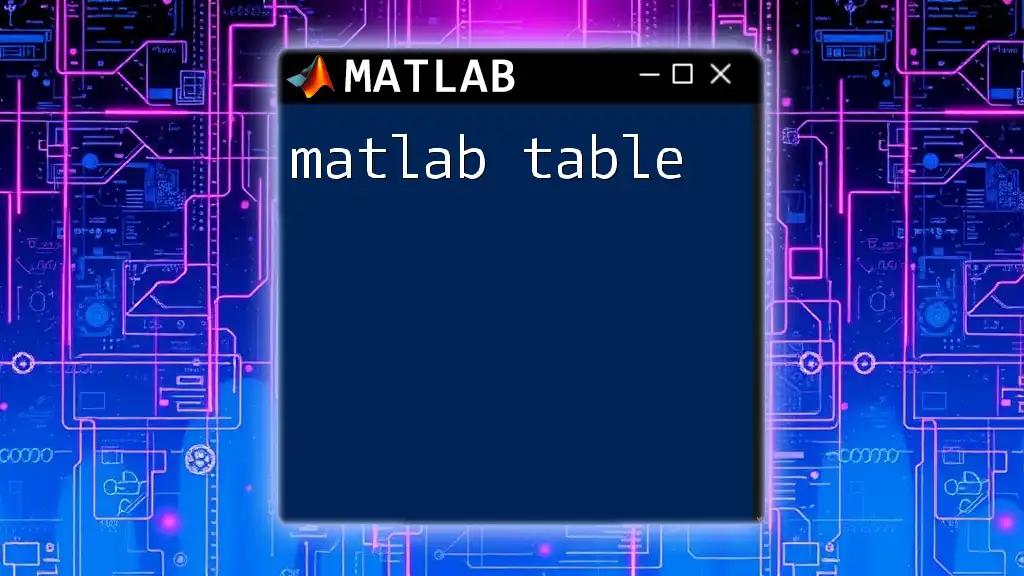
Reading Tables from Files
Overview of File Formats
MATLAB supports various file formats to read tables, including:
- CSV (Comma Separated Values): Simple text files that store tabular data.
- Excel Files (.xls, .xlsx): Widely used for data storage and manipulation.
- Text Files (.txt): General-purpose files that can contain data in various delimiters.
Each of these formats can be read into MATLAB as tables using the `readtable` function, which automatically detects the data type in each column.
Reading CSV Files
To read a CSV file into a table, you can use the `readtable` command as follows:
T = readtable('data.csv');
This command reads the entire content of data.csv into the MATLAB table T. It automatically treats the first row as the header, setting the column names accordingly. You can also specify the delimiter or the number of header rows using optional parameters:
T = readtable('data.csv', 'Delimiter', ';', 'HeaderLines', 1);
In this example, we change the delimiter to a semicolon instead of the default comma.
Reading Excel Files
MATLAB can read Excel files using a similar command structure. Here’s how you can load data from an Excel file:
T = readtable('data.xlsx', 'Sheet', 'Sheet1');
In this case, you specify the sheet name to read from. You can also define a particular cell range if needed:
T = readtable('data.xlsx', 'Range', 'A1:C10');
This command reads only the specified range of data, helping you manage large datasets more efficiently.
Reading Text Files
To read a delimited text file, the `readtable` function works just like it does for CSV files. Here’s an example for a tab-delimited text file:
T = readtable('data.txt', 'Delimiter', '\t');
You can specify different delimiters such as commas, tabs, or custom strings as needed, making it quite versatile for various text formats.
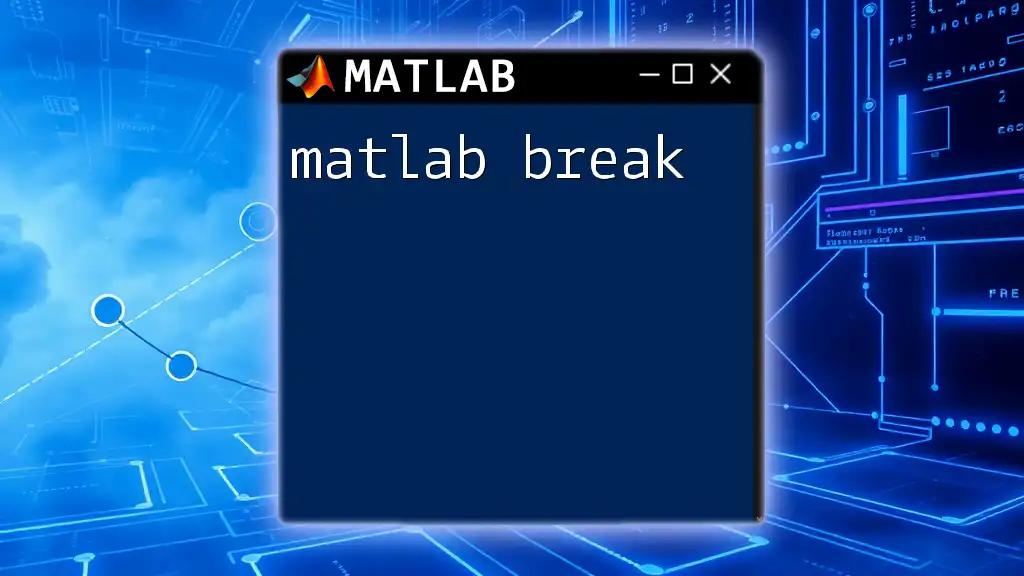
Customizing Table Import
Specifying Variable Names
When reading tables, you may want to define specific column names. You can do this by using the 'VariableNames' option. Here’s an example:
T = readtable('data.csv', 'VariableNames', {'ID', 'Name', 'Score'});
This command explicitly sets the variable names for the columns, regardless of the original header in the CSV file.
Handling Missing Data
Data sets often include missing values that need to be managed properly. When reading tables, you can specify how to treat such values. Consider the following example:
T = readtable('data.csv', 'TreatAsEmpty', {'NA', '', ' '});
In this code, we inform MATLAB to treat any of the specified entries as if they were missing, ensuring that they are stored as NaN for numeric data or empty strings for textual columns.
Subsetting Data Upon Read
You might not need the entire dataset from a file. The `Range` option allows you to read specific rows or columns:
T = readtable('data.xlsx', 'Range', 'A1:C10');
This command reads only the rows specified in the provided range, saving memory and processing time.
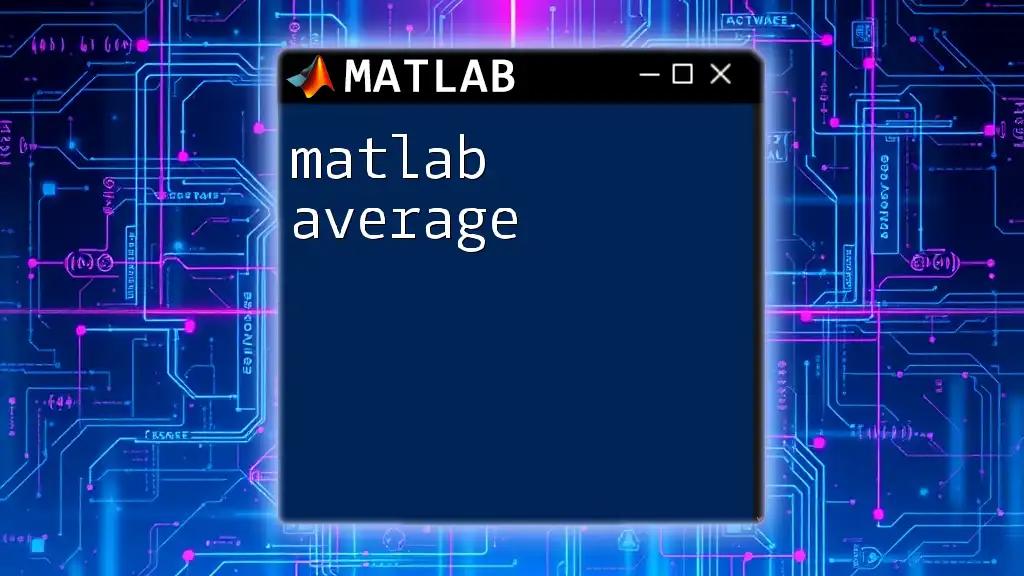
Post-Import Analysis
Exploring the Data
After loading a table, you can explore its contents with simple functions to get a sense of the data you’re dealing with. The following commands are particularly useful:
head(T);
summary(T);
height(T);
- `head(T)` displays the first few rows of the table.
- `summary(T)` provides a summary of each variable, including data types and a count of missing values.
- `height(T)` returns the number of rows, giving you a quick understanding of the data size.
Manipulating Table Data
One of the powerful features of MATLAB tables is the ability to manipulate the data efficiently. For instance, you can add new columns based on existing data. This example demonstrates how to add a pass/fail column based on scores:
T.PassFail = T.Score >= 75; % Adds a new pass/fail column
Here, T.PassFail is a new column that evaluates whether scores are 75 or above, marking them as true for "pass" or false for "fail."
Exporting Tables
Once you have processed your data, you may want to export it back to a file. The `writetable` function serves this purpose well:
writetable(T, 'updated_data.csv');
In this example, you export the modified table T back to a CSV file, effectively saving your changes.
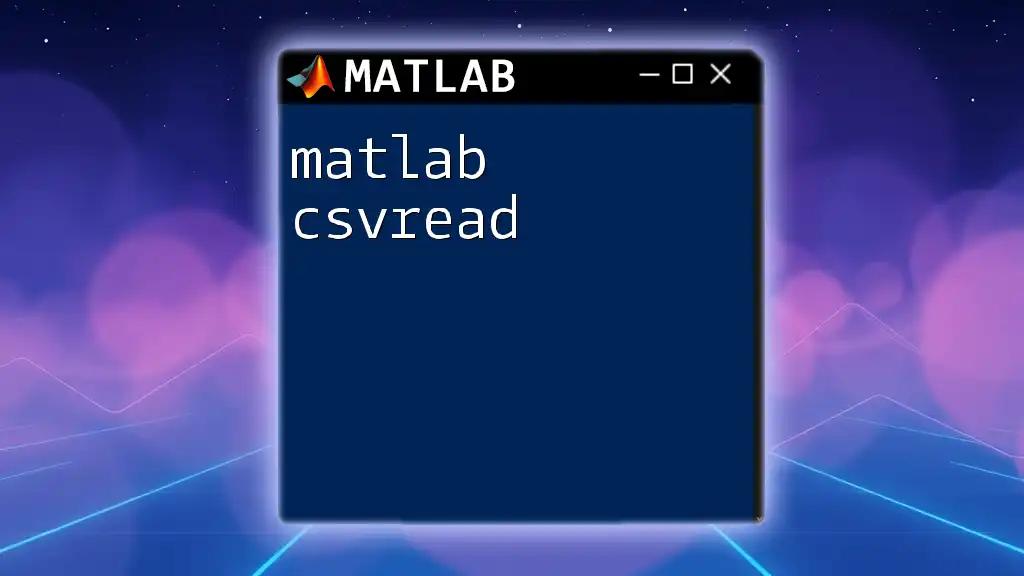
Common Pitfalls and Troubleshooting
While working with tables, you might encounter several common issues. Here are a few thoughts to keep in mind:
-
Incorrect File Paths: Always double-check your file paths. Use `fullfile` to compose paths correctly across different operating systems.
-
Inconsistent Data Types: If a column includes mixed data types, MATLAB may throw a warning or error. Clean your data set to ensure that each column is consistent.
To assist in avoiding these pitfalls, consider the following tips for efficient table handling:
- Use consistent file formats. Stick to well-defined structures, especially if working collaboratively.
- Keep data clean and organized in the source files to minimize import issues.
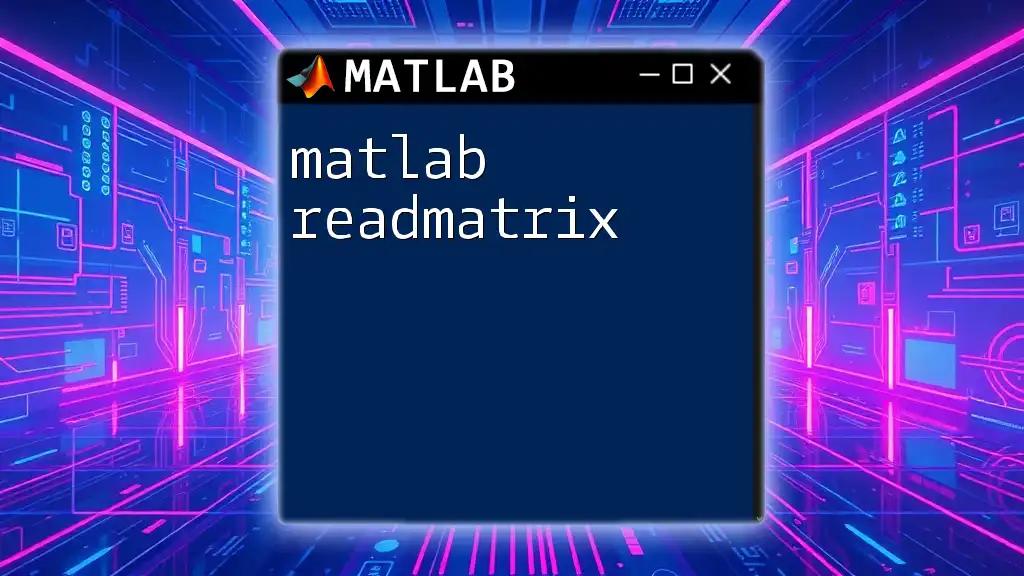
Conclusion
Understanding how to effectively perform a MATLAB table read is essential for data analysis and manipulation. Knowing how to create, read, customize, and analyze tables arms you with powerful tools to efficiently manage your data. Practice these techniques to become proficient in handling tables within MATLAB.
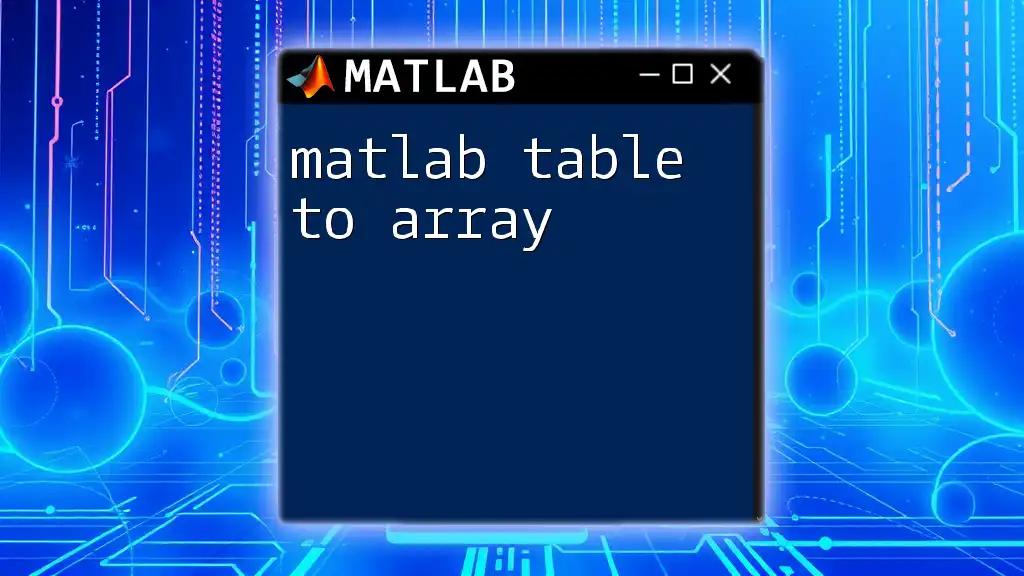
Additional Resources
For more in-depth learning, refer to the official MATLAB documentation on tables: [MATLAB Table Documentation](https://www.mathworks.com/help/matlab/ref/table.html).

Call to Action
Interested in mastering MATLAB commands like these? Sign up for our MATLAB command courses for in-depth training and hands-on practice!