`detrend` is a MATLAB function used to remove a linear trend from a dataset, allowing for a clearer analysis of the underlying signal.
Here's a code snippet demonstrating its use:
% Generate example data with a linear trend
t = 0:0.1:10;
y = 2*t + sin(t);
% Detrend the data
y_detrended = detrend(y);
% Plot original and detrended data
figure;
subplot(2,1,1);
plot(t, y);
title('Original Data with Trend');
xlabel('Time'); ylabel('Amplitude');
subplot(2,1,2);
plot(t, y_detrended);
title('Detrended Data');
xlabel('Time'); ylabel('Amplitude');
Introduction to Detrending in MATLAB
What is Detrending?
Detrending is a crucial preprocessing step in data analysis that involves removing underlying trends from a dataset, enabling analysts to focus on the fluctuations around the trend. By doing so, one can better understand the characteristics of the data that may otherwise be obscured by these long-term movements. Common scenarios for employing detrending include analyzing time series data, signal processing, and when dealing with datasets that exhibit non-stationarity.
Why Use Detrend in MATLAB?
MATLAB is a powerful tool that offers extensive features for data analysis, making it an ideal choice for executing detrending tasks. Python or R might also offer similar functionalities, but MATLAB's built-in functions are optimized for performance in handling matrices and extensive datasets. The `detrend` function simplifies the process, enabling users to focus on the main aspects of their data without getting bogged down by the complexities of implementation.
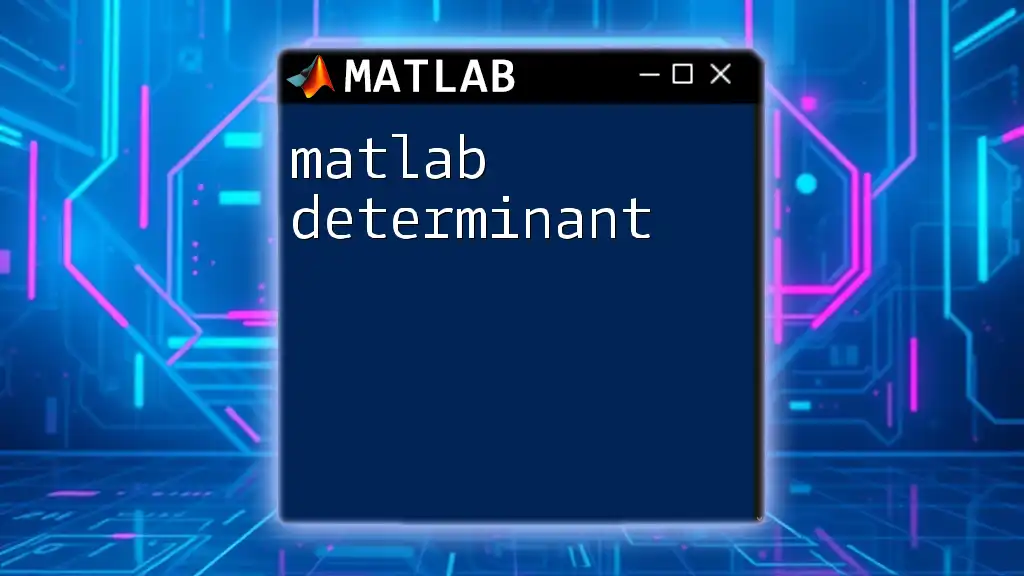
Understanding the Detrend Function
Overview of the `detrend` Function
The core of MATLAB detrending is the `detrend` function. This function subtracts the trend from your data, providing a clearer picture of the underlying patterns. The basic syntax is straightforward:
y_detrended = detrend(y)
This command will compute the trend of the input vector or matrix `y` and remove it.
Parameters and Options
The `detrend` function can accept several parameters that configure its operation:
-
Input Signals: The primary input is a vector or matrix of values. If you have multiple time series, they can be arranged in a matrix format.
-
Types of Detrending: You can specify the type of detrending method—options include:
- 'linear': For removing linear trends.
- 'constant': For removing the mean of the data.
- polynomial: Detrending via higher-order polynomial fits, indicated by an additional parameter.
Output of the Function
The function typically returns the detrended data, allowing for further analysis. By processing the output, you can identify patterns that would have otherwise gone unnoticed in the presence of a trend.
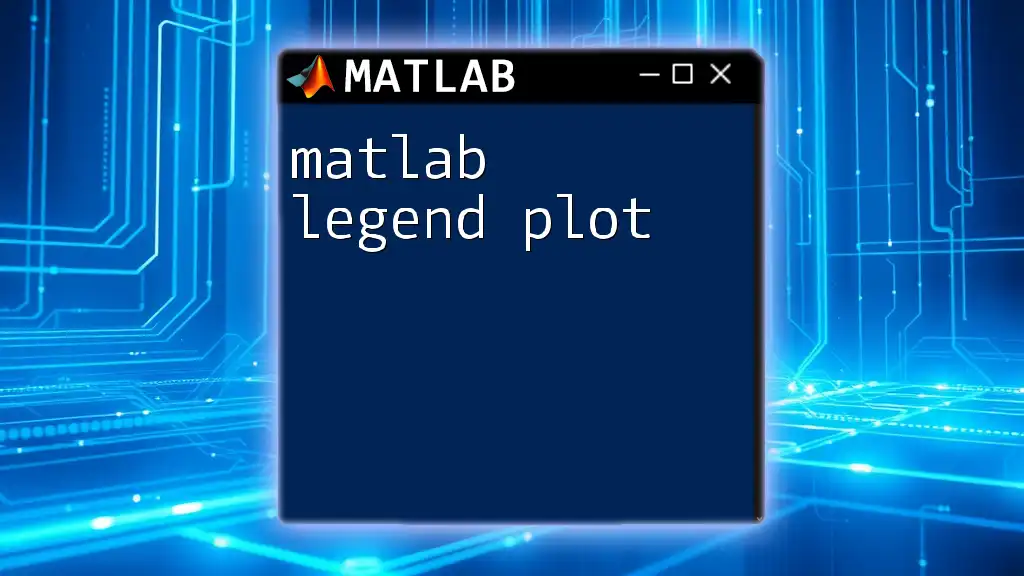
Practical Applications of Detrending
Removing Trends from Time Series Data
Removing trends is essential for creating reliable models and forecasting future values. In financial data such as stock prices, the overall trend can obscure short-term volatility. Applying the `detrend` function to such data can enhance analytical accuracy and insight.
Enhancing Frequency Analysis
Detrending also plays an important role in frequency domain analysis. When you're performing Fourier Transforms (FFT), it’s vital to address trends beforehand, as they can introduce significant low-frequency components that affect the results. After applying the `detrend` function, you can analyze data more effectively and draw actionable insights about frequency components.
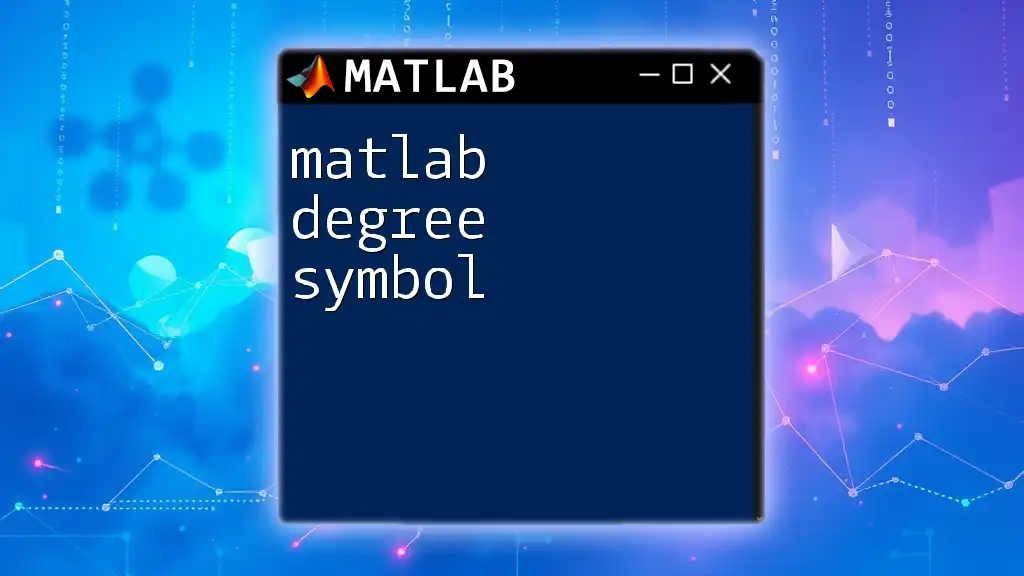
Step-by-Step Example: Detrending a Sample Data Set
Creating Sample Data
Let's create some synthetic data that embodies a trend. In this example, we will generate data with a linear trend and added noise:
x = 1:100;
y = 0.5*x + randn(1,100)*10; % Linear trend with noise
This code generates a linearly increasing trend combined with random Gaussian noise.
Visualizing the Original Data
Before becoming engrossed in the detrending process, it's crucial to visualize the original data to spot trends:
figure;
plot(x, y);
title('Original Data with Trend');
xlabel('Time');
ylabel('Value');
Applying the Detrend Function
Now, let’s apply the `detrend` function, which will subtract the underlying trend from the data:
y_detrended = detrend(y);
Visualizing the Detrended Data
Finally, we have to visualize the detrended data to see how much the trend has affected the values:
figure;
plot(x, y_detrended);
title('Detrended Data');
xlabel('Time');
ylabel('Value');
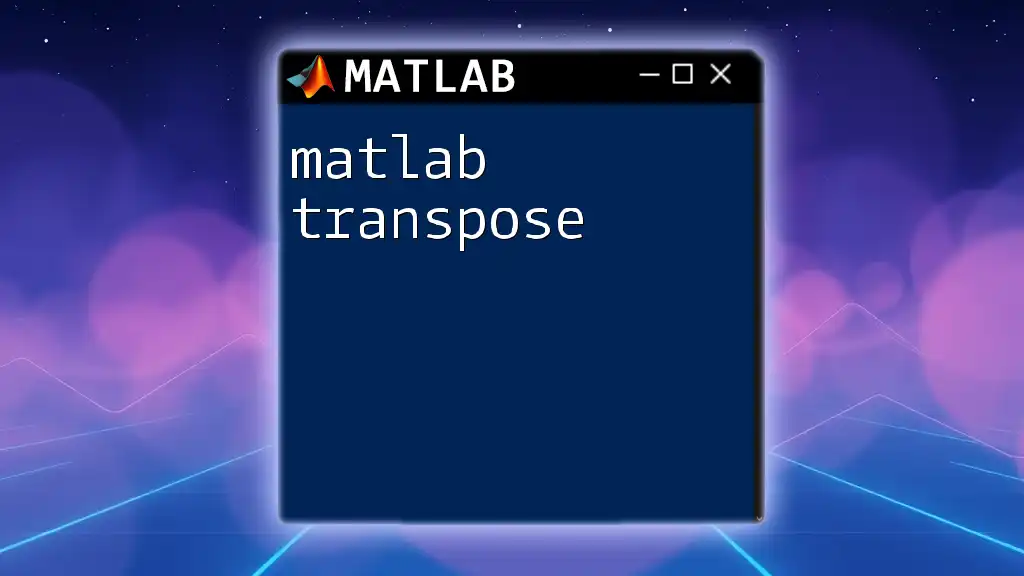
Common Mistakes and Pitfalls
Choosing the Wrong Detrending Method
Selecting the incorrect method for detrending can lead to misleading results. Always assess the nature of your data before applying a specific type of detrending. A common mistake is using a linear detrend on data that has a polynomial curve; this may not adequately remove the underlying trend.
Over-Detrending
Taking detrending too far can strip the data of meaningful information. Over-detrending leads to a loss of important patterns. When analyzing the results, watch for signs such as unrealistic fluctuations which suggest that valuable trend components were removed.
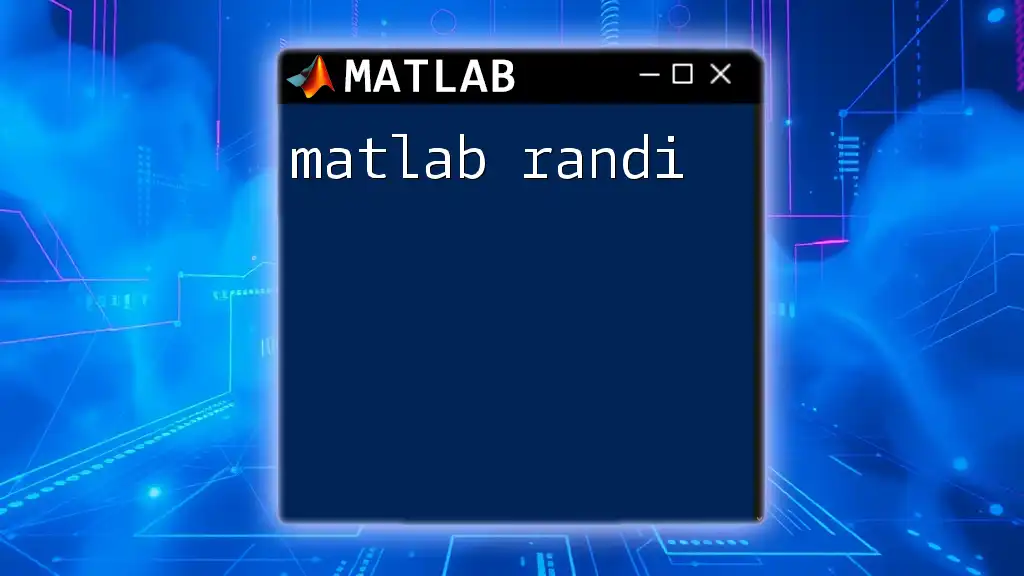
Advanced Detrending Techniques
Polynomial Detrending
Sometimes, a linear trend isn't enough to capture the underlying movements in the data. Polynomial detrending applies a more complex, fitted curve to remove trends. This is useful in cases where trends are not straight lines:
p = polyfit(x, y, 2); % Fit a quadratic polynomial
y_poly_detrended = y - polyval(p, x);
This example fits a quadratic polynomial, capturing a more nuanced trend.
Cubic Spline Detrending
For another degree of sophistication, cubic spline methods can be employed. This method allows for a more flexible fit compared to polynomial adjustments, catering to datasets that exhibit irregular trends.
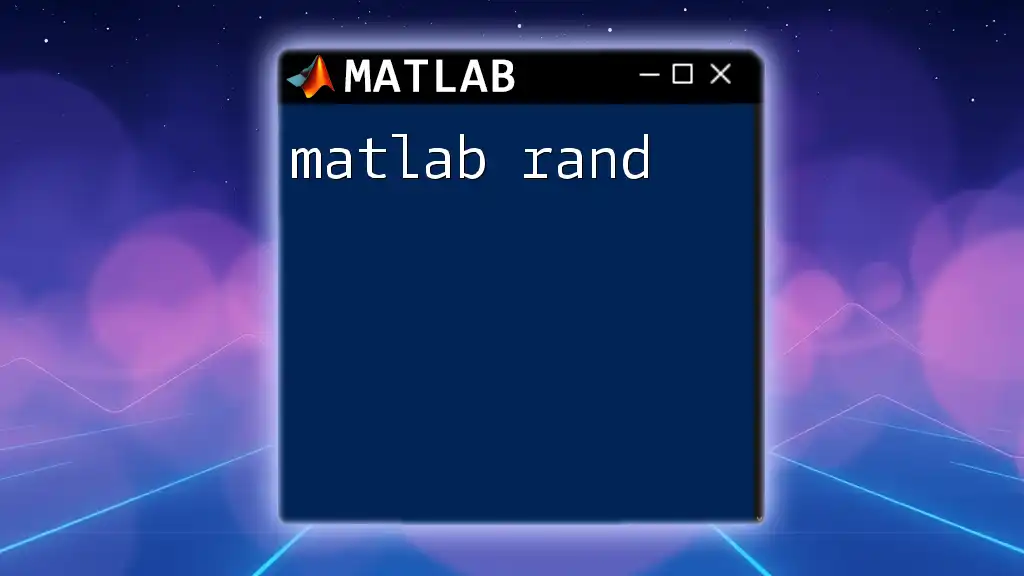
Conclusion
Summary of Key Points
Effective use of the `detrend` function in MATLAB allows analysts to focus on the salient features of their data by effectively removing unwanted trends.
Encouragement to Explore Further
Detrending opens up new avenues for analysis and insights. Consider integrating detrending with other MATLAB functionalities to enhance your data analysis capabilities.
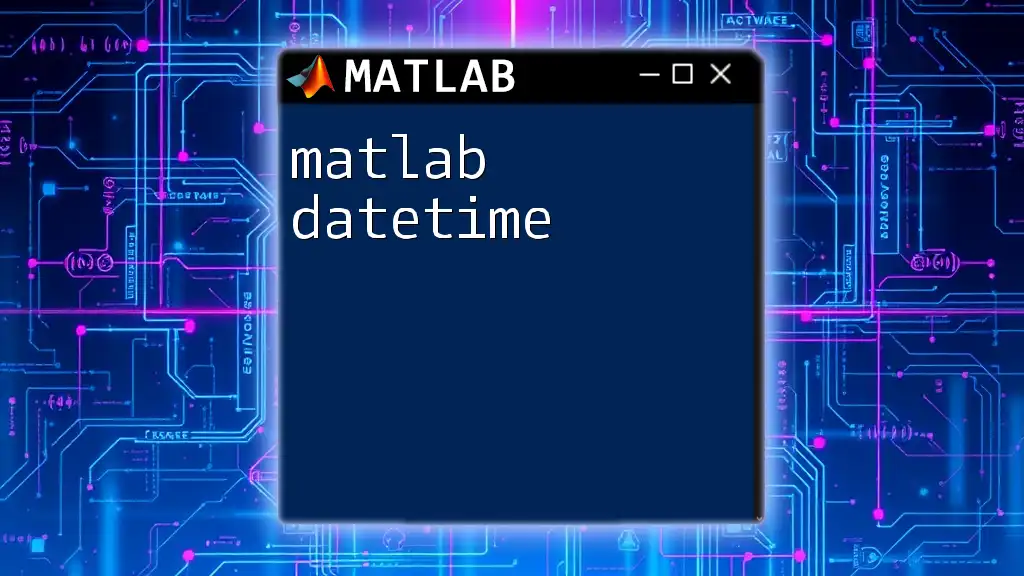
Additional Resources
For more detailed insights into detrending methods, MATLAB's official documentation provides valuable resources. Regularly explore additional tutorials and courses to sharpen your skills in MATLAB programming. Consider reading authoritative literature on time series analysis to enhance your understanding of the totality of trends in data and the intricacies of data modeling.
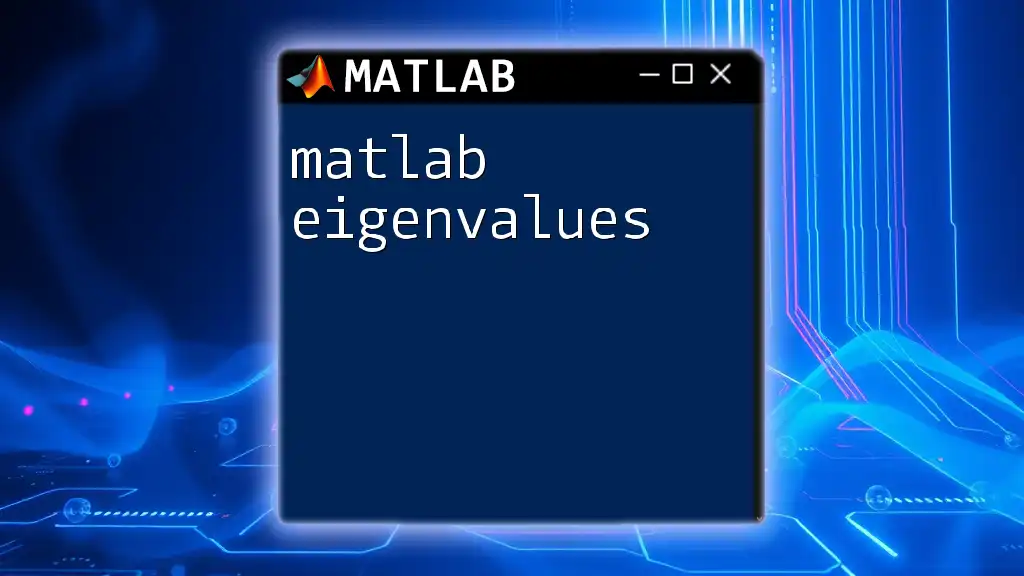
Closing Thoughts
Continuous learning is paramount to mastering MATLAB commands, including the implementation of `detrend`. As you expand your knowledge, remember that effective data analysis is not merely about using commands but understanding them deeply. Enroll in our courses to accelerate your proficiency in MATLAB detrending and beyond!