The `try-catch` construct in MATLAB allows you to manage errors by executing a block of code (the `try` section) and handling any exceptions that occur in the `catch` section, ensuring that your script runs smoothly even when unexpected issues arise.
Here's a simple example:
try
% Attempt to divide by zero
result = 10 / 0;
catch ME
% Handle the error
disp('An error occurred:');
disp(ME.message);
end
Understanding Try-Catch Syntax
Basic Structure of Try-Catch
In MATLAB, the try-catch construct allows developers to handle errors gracefully. The try block contains code that might cause an error, while the catch block defines how to respond if an error occurs. This structure improves program robustness by preventing abrupt terminations due to unhandled exceptions.
For example:
try
% Code that may throw an error
result = 10 / 0; % Division by zero
catch
% Code to handle the error
disp('An error occurred.');
end
In this snippet, dividing by zero would normally cause MATLAB to throw an error and halt execution. However, with the try-catch structure, the error is caught, and a user-friendly message is displayed instead.
Using Multiple Catches
In more complex scenarios, it is often important to address different types of errors appropriately. MATLAB allows catching specific exceptions by utilizing error identifiers.
For instance:
try
% Potentially problematic code
data = load('non_existent_file.txt');
catch ME
switch ME.identifier
case 'MATLAB:load:couldNotFindFile'
disp('File not found, please check the filename.');
otherwise
disp('An unknown error occurred.');
end
end
In this example, if the `load` command fails because the file doesn't exist, the error is caught and displayed as a specific message. This approach allows you to provide more informative feedback depending on the type of error encountered.
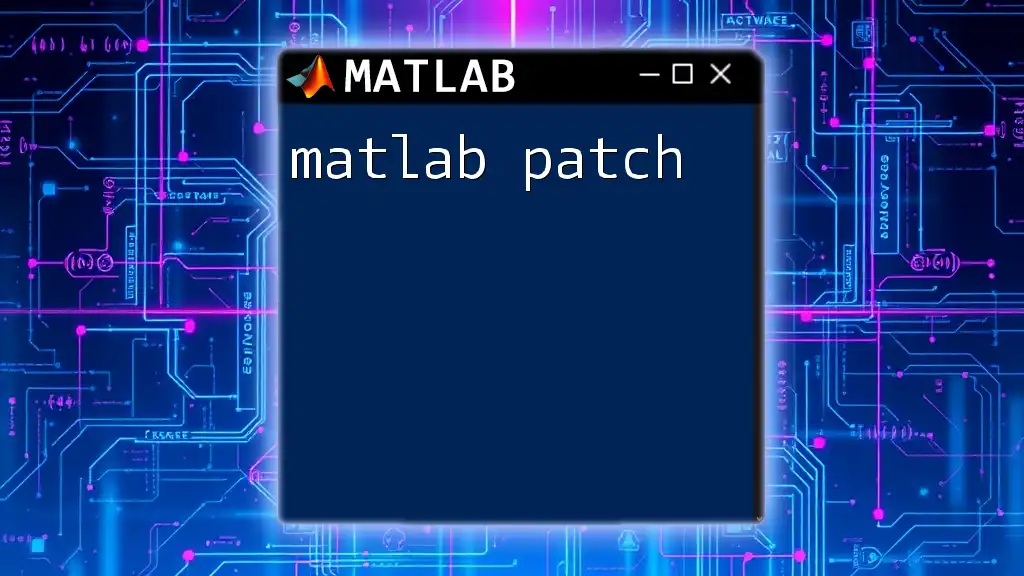
Common Use Cases for Try-Catch
Handling File Operations
File operations, such as reading data files, are often a source of potential runtime errors. Using try-catch around file reading operations can prevent unexpected crashes and enhance user experience.
For example:
try
data = readmatrix('data.csv');
catch
disp('Error reading file. Please make sure the file exists and is in the correct format.');
end
This snippet provides a mechanism to handle errors related to file input/output gracefully, informing the user if something goes wrong.
Validating User Input
When gathering input from users, it’s essential to ensure that the input is valid before proceeding with calculations or operations. A try-catch block can significantly enhance input validation.
Consider the following example:
try
user_input = input('Enter a number: ');
if ~isnumeric(user_input)
error('UserInput:InvalidType', 'Input must be numeric.');
end
catch ME
disp(ME.message);
end
In this example, if the user inputs something that is not numeric, MATLAB raises a custom error, which is then caught and displayed, helping guide users to provide correct inputs.
Time-consuming Computations
Sometimes, code execution may take longer than expected, and users may need a way to handle this gracefully. Try-catch can be used here as well, especially in long-running computations.
Here’s an example:
try
pause(10); % Simulating a long computation
disp('Completion of the computation.');
catch
disp('The computation was interrupted.');
end
In this case, if the computation is manually interrupted, the catch block captures that and provides feedback rather than letting the program crash or end abruptly.
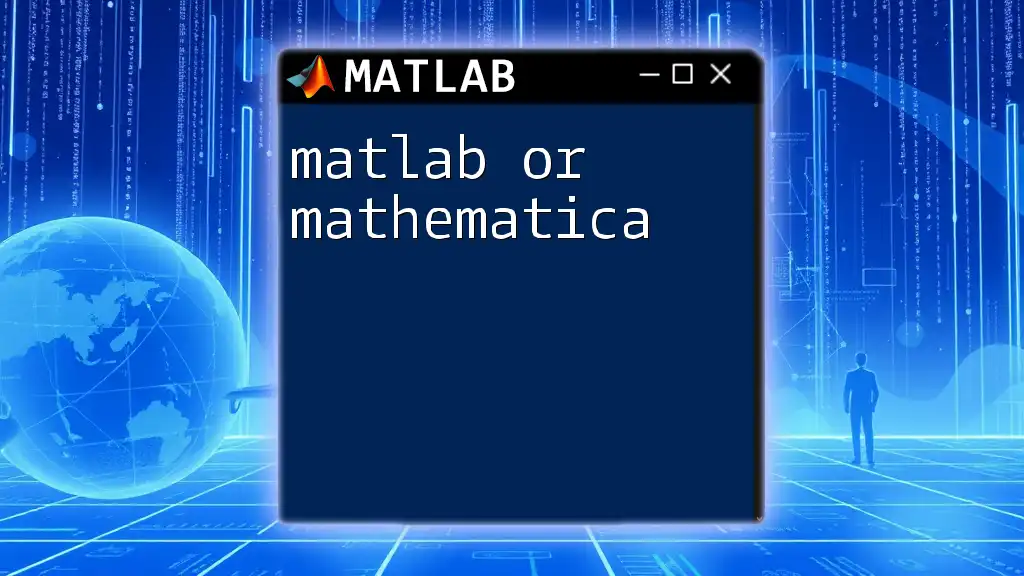
Best Practices When Using Try-Catch
Keep Try Blocks Short
One key principle when using try-catch is to keep try blocks short. The longer the code within the try block, the harder it may be to identify the source of an error. By limiting the amount of code within the try block, you make debugging easier and reduce the chances of unnecessary complications.
Specific Error Handling
Using specific exceptions in catch blocks is vital for effective error management. When you catch general exceptions, you risk masking underlying issues. Handle specific error identifiers to address different scenarios appropriately, as shown in previous examples.
Log Errors for Debugging
Logging errors provides valuable insights into how often issues arise and under what circumstances. This information can be essential for future debugging and improvement efforts.
Consider the following example of a logging mechanism:
try
% Some MATLAB code that might fail
catch ME
logError(ME); % Your custom function to log the error
end
In this case, instead of simply displaying the error, it's recorded for later analysis. This practice enhances your ability to manage and respond to recurring issues effectively.
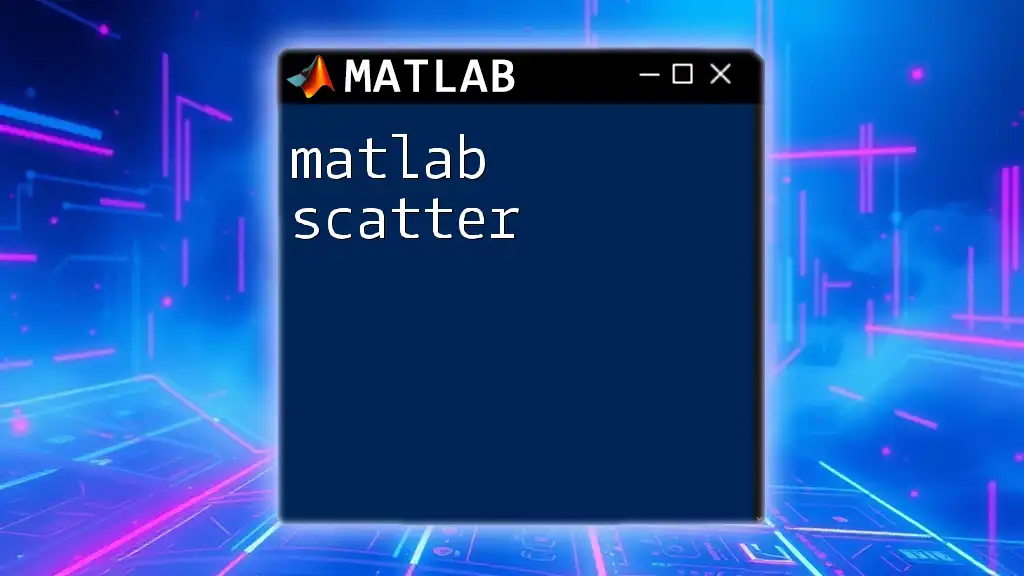
Limitations of Try-Catch
Over-Reliance on Error Handling
While try-catch is a powerful tool for error management, over-relying on it can be detrimental. It may encourage poor coding practices, where developers avoid writing clean, error-free code, presuming that error handling will cover their mistakes. Always strive to write robust and well-structured code alongside using try-catch as a safety net.
Performance Considerations
Another limitation of using try-catch is its potential impact on performance. Extensive use of try-catch blocks can lead to slower execution, especially if errors occur frequently. Use this construct judiciously to maintain the performance of your MATLAB applications.
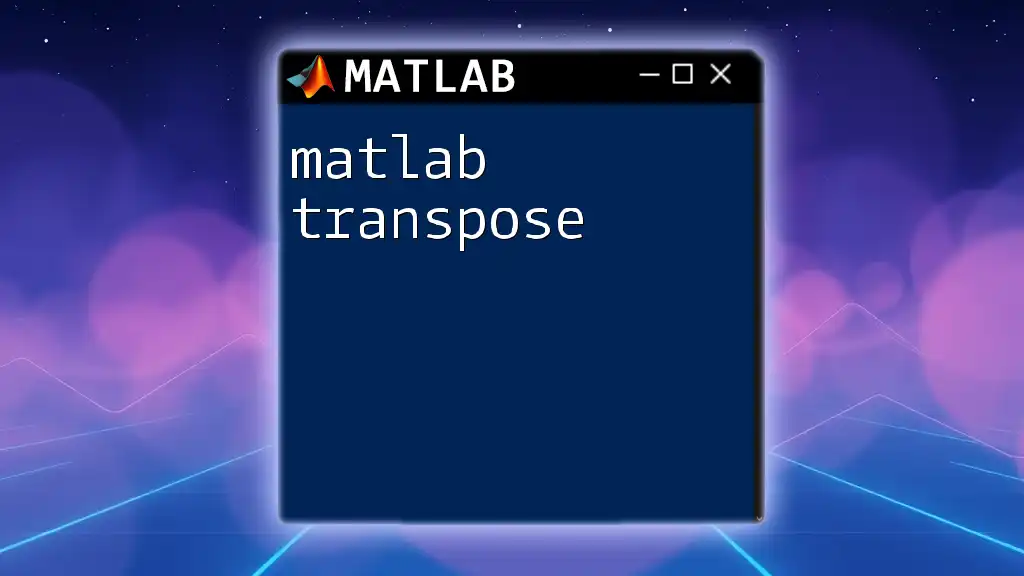
Conclusion
In summary, the MATLAB try-catch mechanism is an essential aspect of robust programming. By enabling developers to handle errors gracefully, it enhances the overall user experience and provides clearer paths for debugging. By implementing best practices, such as keeping try blocks short, using specific error handling, and logging errors, you can optimize the effectiveness of this powerful tool in your MATLAB programs.
Encourage your readers to practice incorporating try-catch into their coding routines to improve their proficiency and resiliency in MATLAB programming!