The `switch` statement in MATLAB allows you to execute different blocks of code based on the value of a variable or expression, making it a powerful tool for decision-making in your scripts.
result = 'B'; % Example variable
switch result
case 'A'
disp('You selected option A');
case 'B'
disp('You selected option B');
case 'C'
disp('You selected option C');
otherwise
disp('Invalid selection');
end
Understanding the Syntax of Switch
The MATLAB switch statement allows programmers to execute specific blocks of code based on the value of an expression. This is particularly useful when you have multiple conditions that apply to the same variable, making your code clearer and easier to maintain.
Basic Structure of a Switch Statement
The general format of a switch statement in MATLAB looks like this:
switch expression
case value1
% Code to execute for value1
case value2
% Code to execute for value2
otherwise
% Code to execute if none match
end
Each case is checked in order, and the first matching case will have its corresponding code executed. If no case matches, the code under the `otherwise` statement will run—acting as a default or catch-all option.
Components of a Switch Statement
- Expression: This is the value you want to evaluate. It can be a variable or a direct value.
- Case Statements: Each `case` checks if the expression matches a specific value. If it matches, the code following that case is executed.
- Otherwise Clause: This optional part allows you to define a block of code that runs if no previous cases are met, providing a way to handle unexpected results.
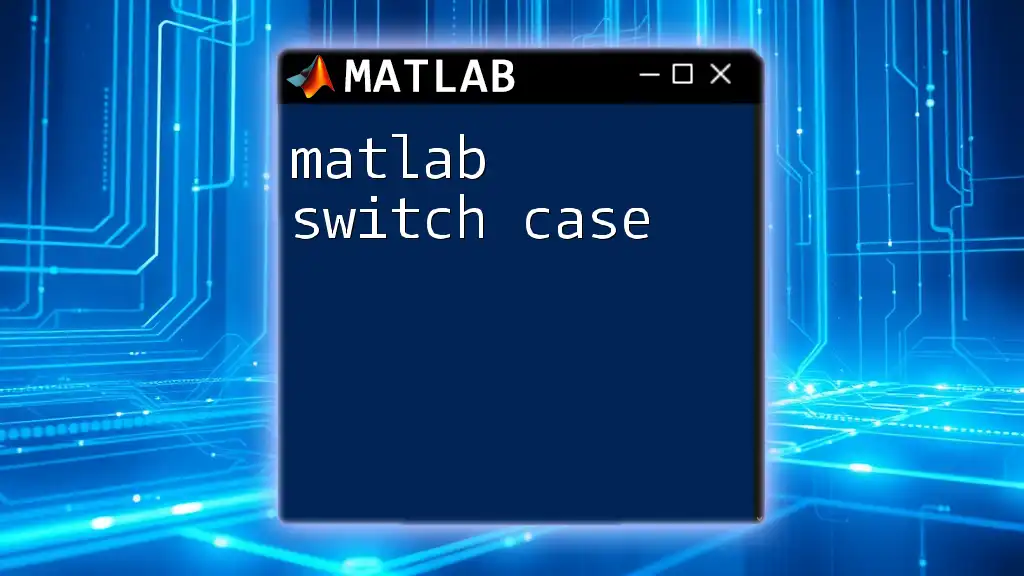
When to Use Switch Statements
Best Use Cases for Switch
The MATLAB switch statement is particularly suitable when you want to execute different blocks of code based on discrete values of a variable. Its design allows for cleaner and more readable code compared to a series of if-else statements, especially when the same condition is evaluated multiple times.
Comparison to if-else Statements
While both switch statements and if-else statements serve the same fundamental task of controlling the flow of a program based on conditions, switch statements can be more efficient and cleaner. They typically offer better performance when dealing with multiple discrete conditions, as they compile down to a jump table in the background, making your code run faster.
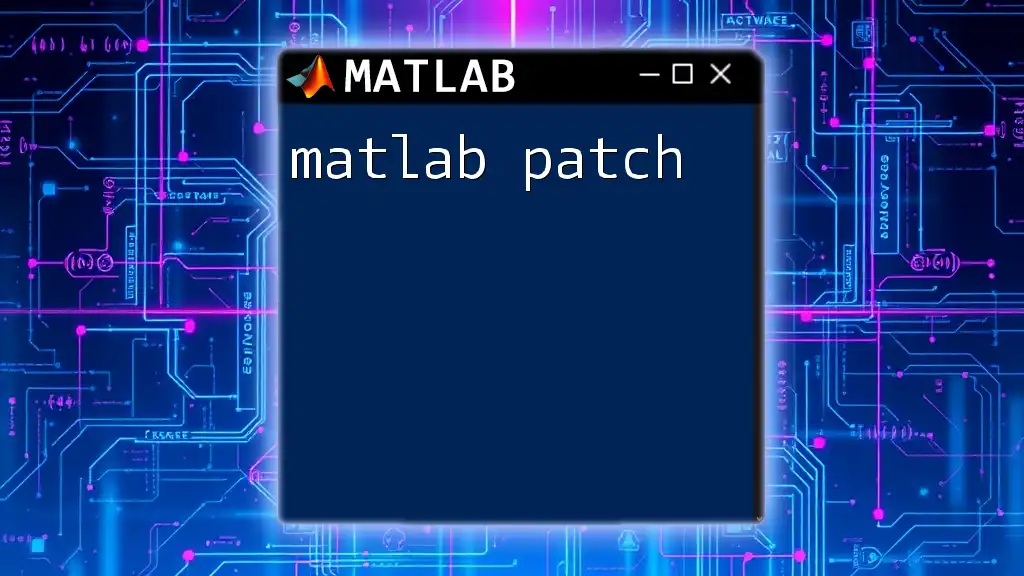
Detailed Breakdown of the Switch Structure
Example of a Simple Switch Statement
To illustrate how a switch statement functions, consider the following example where we evaluate what day of the week it is:
day = 'Tuesday';
switch day
case 'Monday'
disp('Start of the work week!');
case 'Tuesday'
disp('Second day of the week.');
case 'Wednesday'
disp('Midweek day.');
otherwise
disp('Another day.');
end
In this example, we check the variable `day`. Since it holds the value `'Tuesday'`, the second case is executed, displaying `Second day of the week.`.
Using Numeric Values in Switch
Switch statements aren’t limited to strings; they can also handle numeric comparisons efficiently.
Consider this example where we assess a student’s score to determine their grade:
score = 85;
switch score
case 90
disp('Grade: A');
case 80
disp('Grade: B');
case 70
disp('Grade: C');
otherwise
disp('Grade: F');
end
In this example, the switch checks the variable `score`. Since `score` does not match any case exactly, it triggers the `otherwise` clause, yielding `Grade: F`.
Multiple Cases in One Statement
Multiple values can respond to a single case by grouping them, which can further simplify your switch statements.
Here’s how you can use grouping cases for colors:
color = 'green';
switch color
case {'red', 'green'}
disp('The color is red or green.');
case {'blue', 'yellow'}
disp('The color is blue or yellow.');
otherwise
disp('Some other color.');
end
In this case, the output will display `The color is red or green.` since the variable `color` matches one of the cases listed.
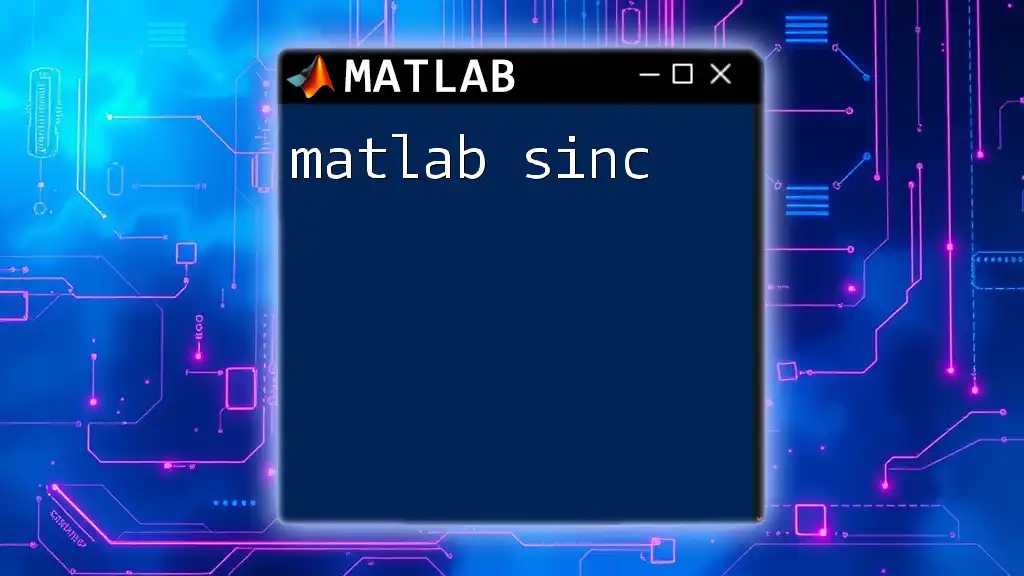
Common Pitfalls and Mistakes
Forgetting the Otherwise Clause
If you do not include an `otherwise` clause and none of the cases match, MATLAB will simply not execute any code from the switch statement. This can lead to confusion, especially if you expect a default action to take place.
For example:
fruit = 'apple';
switch fruit
case 'banana'
disp('This is a banana.');
end
Here, nothing would be displayed even though `fruit` does not match any case. Adding an `otherwise` clause would provide feedback, making debugging easier.
Using Unsupported Data Types
Switch statements in MATLAB only work with scalar values (numeric, character, and logical types). Using matrices or strings with unsupported types will result in an error. For instance:
data = [1, 2; 3, 4]; % This will cause an error in switch.
switch data
case 1
disp('Data is 1');
end
The above code will not work properly. Always ensure that your switch expression evaluates a single value.
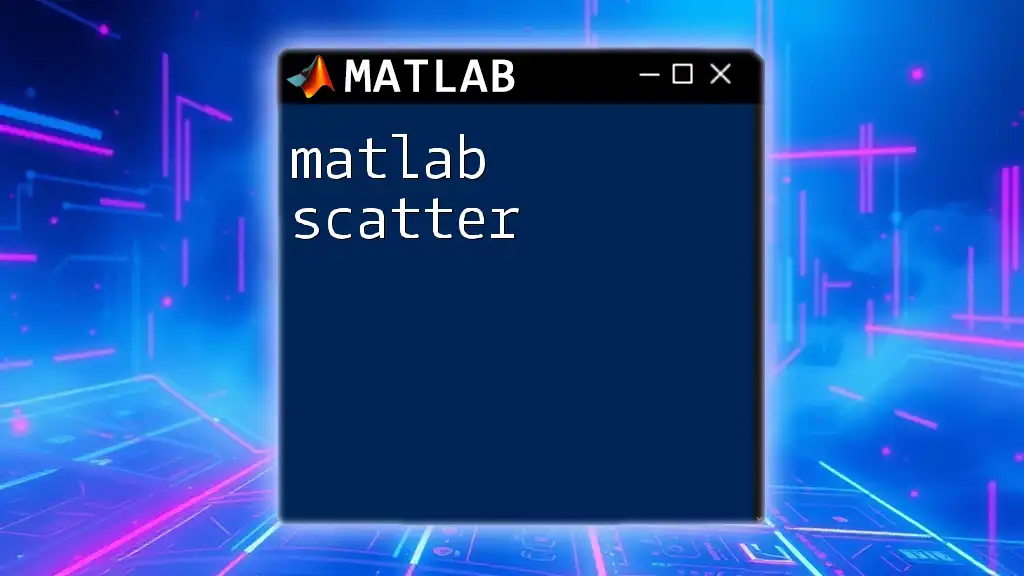
Best Practices for Writing Efficient Switch Statements
- Use Descriptive Case Labels: Ensure that the labels clearly represent what you want to achieve. This improves readability and makes your code easier to understand for others or when revisiting it later.
- Keep Case Blocks Concise: Each case should contain only the necessary code. Avoid complex operations within case blocks to maintain code clarity and prevent bugs.
- Testing for Logical Completeness: Check that all potential values are covered by case statements. This helps eliminate unforeseen issues that may arise from unhandled cases and ensures your code works correctly in all scenarios.
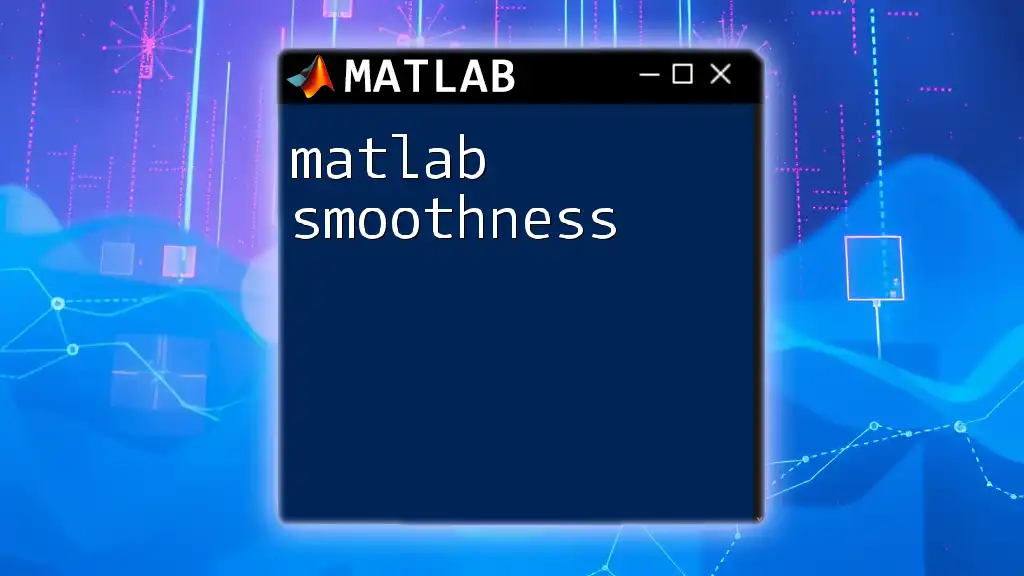
Conclusion
The MATLAB switch statement is an invaluable control structure for simplifying decision-making processes in your code. It enhances readability and efficiency, making it preferable in many scenarios involving multiple discrete conditions. Practicing the implementation of switch statements will improve your programming skills and enable you to write cleaner, more effective MATLAB scripts.
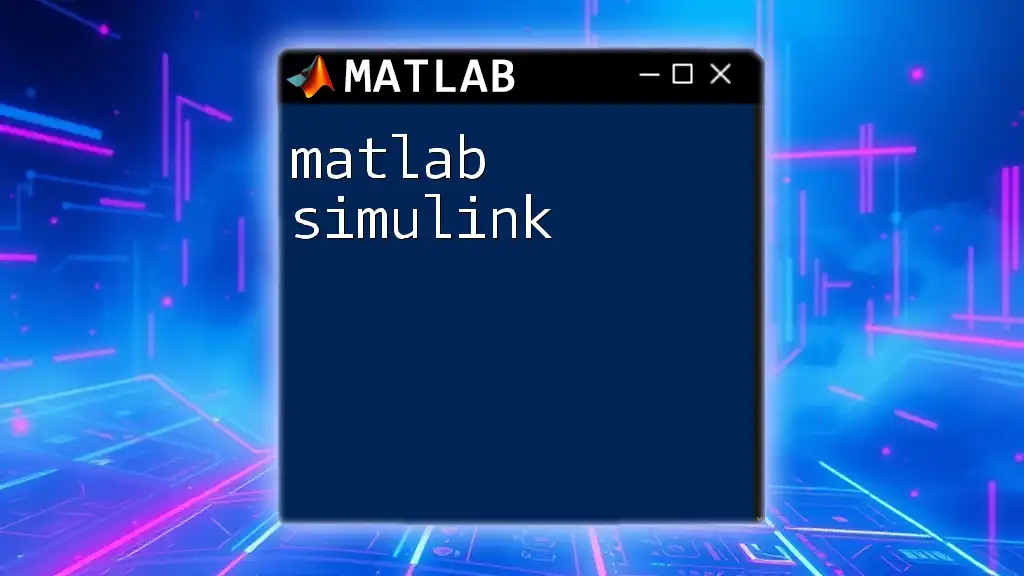
Further Resources
For those looking to dive deeper into switch statements, you can refer to [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/switch.html) for more advanced techniques, explore various MATLAB tutorials, and participate in community forums to address any questions you may have. Engaging with these resources can enhance your understanding and application of switch statements in MATLAB.