The `cat` function in MATLAB concatenates arrays along a specified dimension, allowing you to combine multiple arrays into one.
Here's a code snippet demonstrating how to use the `cat` function to concatenate two matrices along the first dimension:
A = [1 2; 3 4];
B = [5 6; 7 8];
C = cat(1, A, B); % Concatenates A and B vertically
In this example, `C` will result in a 4x2 matrix:
C =
1 2
3 4
5 6
7 8
Understanding `cat`
What is the `cat` Function?
The `cat` function in MATLAB is a powerful tool designed for array concatenation. It allows you to join multiple arrays along a specified dimension, making it integral for data manipulation and organization within your MATLAB projects. By understanding this function, you'll be able to combine matrices flexibly and effectively.
Syntax of `cat`
The basic syntax of the `cat` function is as follows:
C = cat(dim, A1, A2, ...)
Here, `dim` specifies the dimension along which the arrays will be concatenated, while `A1, A2, ...` are the arrays to be combined.
For instance, when you want to concatenate two matrices vertically, you would set `dim` to 1. On the other hand, for horizontal concatenation, `dim` would be set to 2. Understanding this syntax is essential for efficiently using the `cat` function in your own projects.
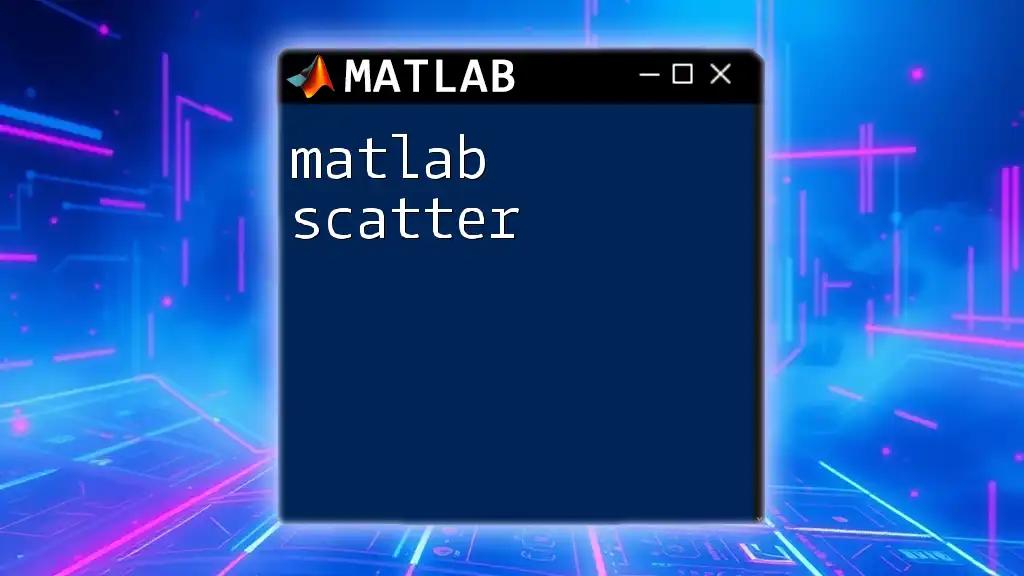
Working with Dimensions
Specifying Dimensions
When using the `cat` function, clearly specifying the dimension is crucial:
- Dimension 1 enables vertical concatenation (adding rows).
- Dimension 2 allows for horizontal concatenation (adding columns).
- Higher Dimensions: You can even concatenate multi-dimensional arrays along specified dimensions beyond the second.
Examples of Dimension-Based Concatenation
To illustrate how the dimension specification works, consider the following examples:
Example: Vertical Concatenation
A = [1; 2; 3];
B = [4; 5; 6];
C = cat(1, A, B); % Result: [1; 2; 3; 4; 5; 6]
In this example, arrays A and B are concatenated vertically, combining their rows.
Example: Horizontal Concatenation
D = [1, 2, 3];
E = [4, 5, 6];
F = cat(2, D, E); % Result: [1 2 3 4 5 6]
Here, arrays D and E are concatenated horizontally, extending their columns.
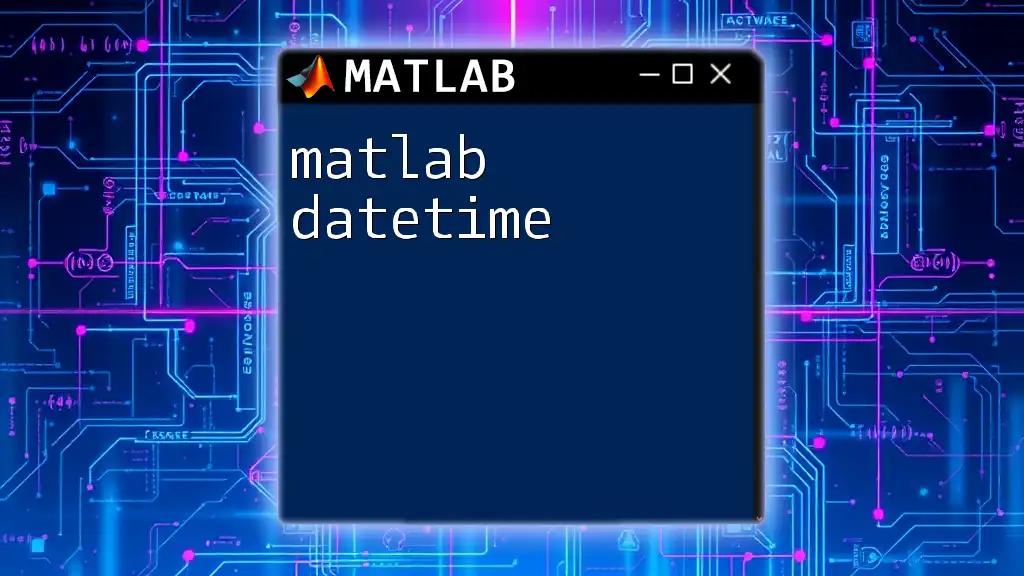
Additional Features of `cat`
Concatenating Multiple Arrays
One of the standout features of the `cat` function is its ability to concatenate more than two arrays at once, thereby enhancing your data handling capabilities.
Code Example:
G = [7; 8; 9];
H = [10; 11; 12];
I = cat(1, A, B, G, H); % Result: [1; 2; 3; 4; 5; 6; 7; 8; 9; 10; 11; 12]
In this code, arrays A, B, G, and H are concatenated vertically, demonstrating how easily multiple datasets can be combined.
Dealing with Different Array Sizes
It's essential to remember that concatenation rules dictate that dimensions must match, except for the dimension at which the concatenation occurs. For example, if you attempt to concatenate arrays of differing sizes, MATLAB will throw an error.
Example of Mismatched Sizes:
% This will throw an error
% A = [1 2; 3 4];
% B = [5; 6];
% cat(2, A, B);
In this scenario, attempting to concatenate A and B horizontally is invalid due to differing row counts.
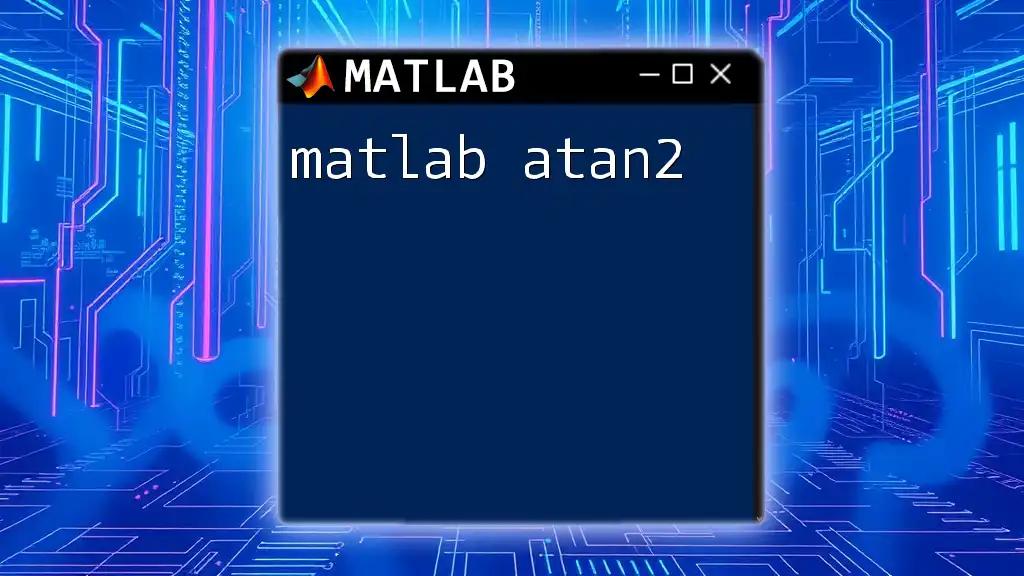
Practical Applications of `cat`
Combining Datasets
In practical applications, the `cat` function plays a vital role in combining datasets from various experiments or sources. For instance, if you have multiple test results in separate arrays, you can easily combine them into a single array for further analysis and processing.
Matrix Manipulation
When it comes to matrix manipulation, the `cat` function can be a lifesaver. You can create larger matrices from smaller ones, which is particularly useful when developing algorithms that require input of specific dimensions.
Example of Creating a 3D Array:
X = [1 2; 3 4];
Y = [5 6; 7 8];
Z = cat(3, X, Y); % Result: a 3D array with dimensions 2x2x2
This code demonstrates how two 2D matrices can be concatenated to form a 3D matrix, showcasing the versatility of the `cat` function.

Common Errors and Troubleshooting
Common Errors when Using `cat`
Despite its utility, users may encounter common errors, particularly related to dimension mismatches. It is essential to read MATLAB's error messages to understand what went wrong. These messages often provide guidance on which dimension(s) are causing the problem.
Tips for Successful Concatenation
- Always ensure the arrays you wish to concatenate are dimensionally consistent, barring the specified concatenation dimension.
- When debugging issues, consider printing the size of your arrays using the `size` function prior to concatenation, which can help identify mismatched dimensions.
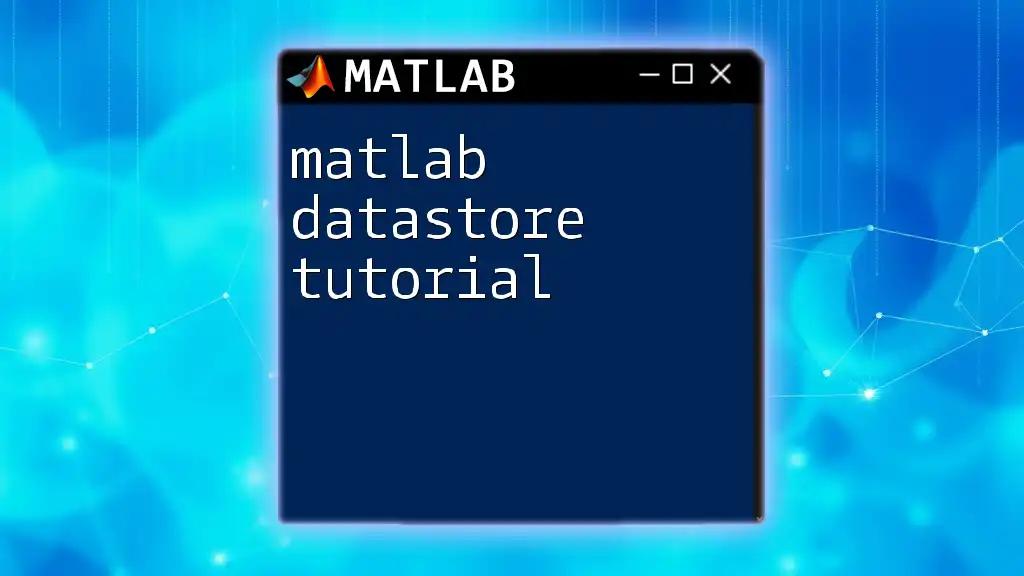
Conclusion
In conclusion, the `matlab cat` function is an indispensable tool for anyone working with MATLAB, providing the ability to concatenate arrays efficiently and effectively. Whether you’re combining datasets, manipulating matrices, or creating multi-dimensional arrays, understanding `cat` will significantly enhance your MATLAB skills.
Additional Resources
For further exploration, refer to the official MATLAB documentation on array manipulation, and consider delving into specialized books and online courses focused on mastering MATLAB's capabilities. By practicing with the `cat` function and understanding its features thoroughly, you'll be well on your way to becoming proficient in MATLAB data manipulation.