MATLAB and Python can be leveraged together, allowing users to harness the powerful computational capabilities of MATLAB while taking advantage of Python's versatility in data handling and application development.
Here’s a simple code snippet that demonstrates how to call a MATLAB function from Python using the `matlab.engine` package:
import matlab.engine
# Start MATLAB engine
eng = matlab.engine.start_matlab()
# Define a simple MATLAB function and call it
result = eng.sqrt(16.0)
print(f"The square root of 16 is: {result}")
# Stop MATLAB engine
eng.quit()
Understanding MATLAB and Python
Overview of MATLAB
What is MATLAB?
MATLAB (Matrix Laboratory) is a high-level programming language and interactive environment that specializes in numerical computing and algorithm development. Originally designed for engineers and scientists, it is highly utilized for simulations, data analysis, and many forms of mathematical computations.
Key Features of MATLAB
One of the standout features of MATLAB is its powerful built-in libraries and toolboxes, such as the Statistics and Machine Learning Toolbox or the Image Processing Toolbox. These resources simplify complex mathematical computations, allowing users to focus on problem-solving rather than coding intricacies. Its user-friendly interface enables quick prototyping and visual representation of data, making it accessible to users at all levels.
What is Python?
Introduction to Python
Python is an interpreted, high-level programming language known for its simplicity and versatility. It has grown to become one of the most popular programming languages used today, particularly in web development, data analysis, artificial intelligence, and scientific computing.
Key Features of Python
Python’s strengths lie in its wide range of libraries such as NumPy for numerical computations, SciPy for scientific computing, and Matplotlib for data visualization. This extensive ecosystem allows users to perform complex analyses and visualize data effectively, aided by its clear syntax that promotes readability and ease of use.
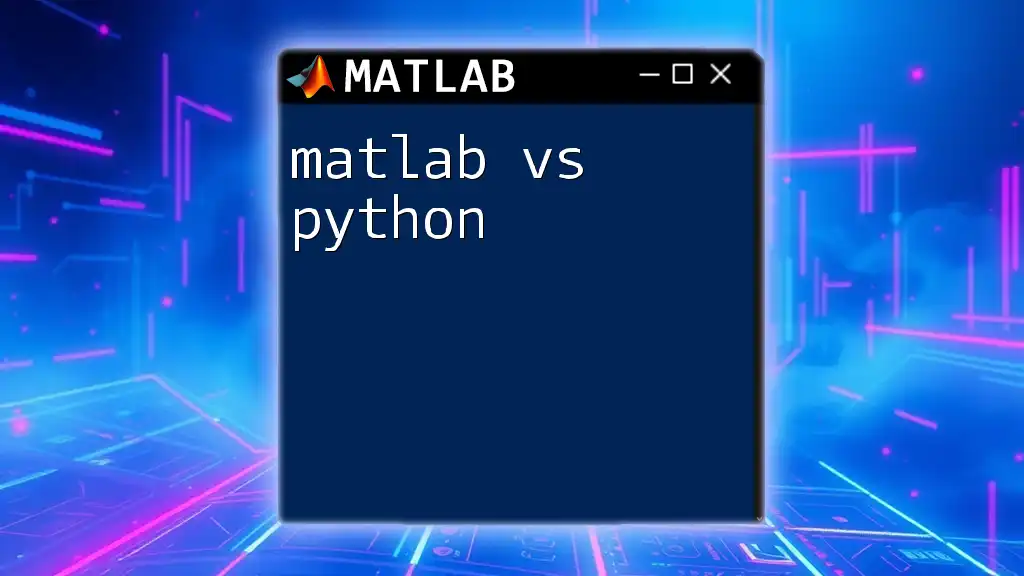
Why Use MATLAB and Python Together?
Complementary Strengths
MATLAB and Python bring unique strengths to the table. MATLAB excels in specialized mathematical computations and provides a seamless environment for handling matrices and arrays, which are pivotal for linear algebra and numerical analysis. On the other hand, Python shines in areas like data manipulation, quick scripting, and integrating with web applications and databases. Thus, using both languages can lead to enhanced productivity and foster innovation in various computing tasks.
Enhanced Productivity
Integrating MATLAB and Python can significantly accelerate project timelines. For instance, a user can code and debug in Python’s simplified syntax, process data using Python’s extensive libraries, and switch to MATLAB for sophisticated numerical computations. Such flexibility allows for more efficient workflows, optimizing both development time and resources.
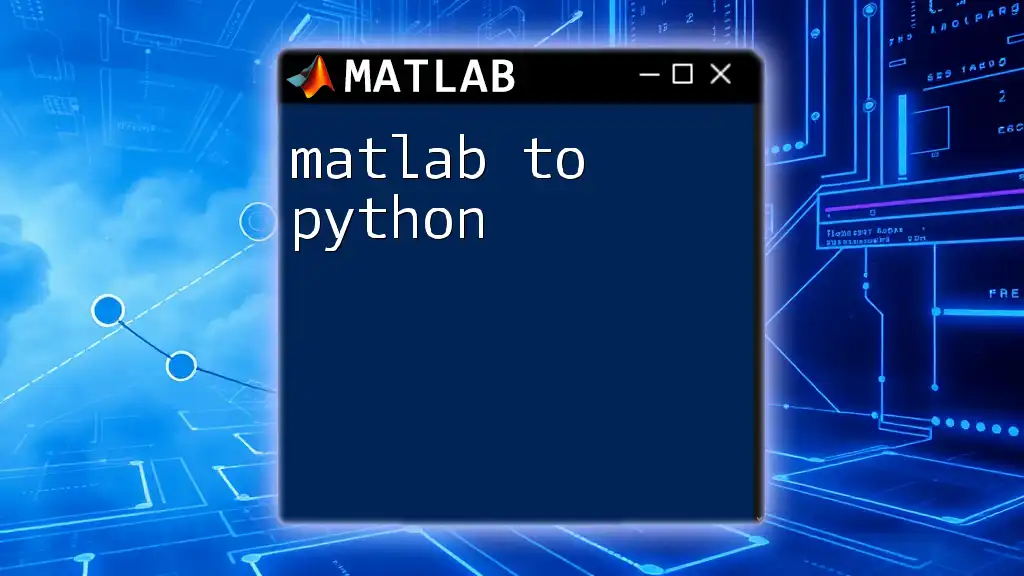
Setting Up the Environment
Installing MATLAB and Python
Requirements for Installation
To effectively work with MATLAB and Python, you will need to ensure that your system meets the necessary requirements. For MATLAB, check your operating system's compatibility and required system specifications. For Python, downloading the latest version from its official website or using a package manager like Anaconda can simplify the installation process.
Installation Steps
- Installing MATLAB: Obtain a copy of MATLAB from MathWorks (check for student or trial versions if applicable) and follow the provided installation instructions.
- Installing Python: Download the official Python installer from python.org, or utilize a distribution such as Anaconda, which comes with many useful libraries pre-installed.
Configuring the Integration
Using MATLAB Engine API for Python
The MATLAB Engine API allows for a direct interface between Python and MATLAB, thus enabling you to call MATLAB functions from Python scripts. This opens a plethora of possibilities for leveraging MATLAB’s computational capabilities while utilizing Python’s versatile programming features.
Example Code Snippet
import matlab.engine
eng = matlab.engine.start_matlab()
result = eng.sqrt(16.0)
print(result) # Output: 4.0
This straightforward example illustrates how Python can harness the power of MATLAB to quickly calculate the square root of a number.
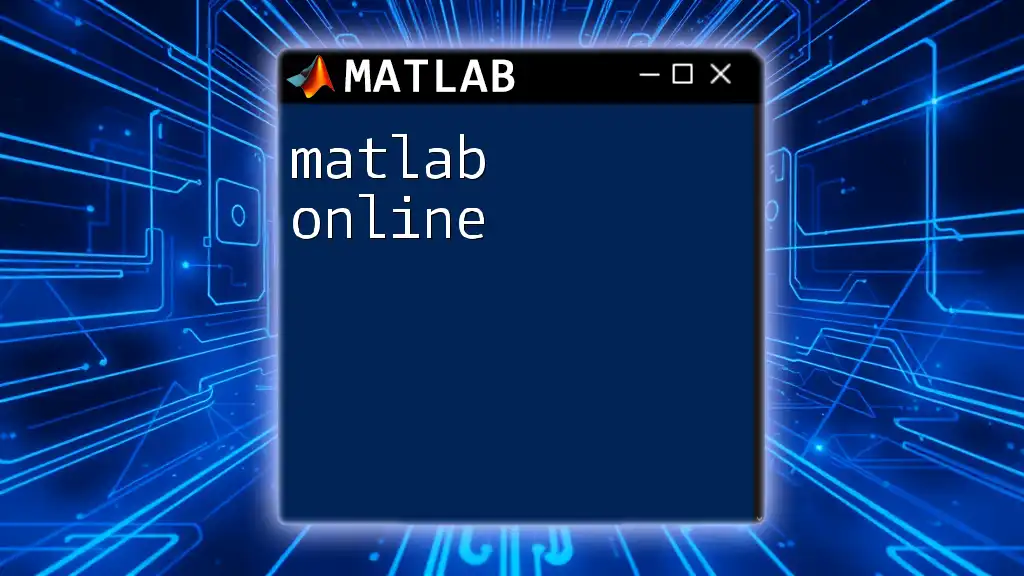
Methods for Integrating MATLAB and Python
Calling MATLAB from Python
Using MATLAB Engine API
To invoke MATLAB functions from Python, you can initiate a MATLAB session through the MATLAB Engine API. This allows you to send variables and execute commands directly from your Python environment.
Example Code Snippet
import matlab.engine
eng = matlab.engine.start_matlab()
a = matlab.double([1, 2, 3])
b = eng.mean(a)
print(b) # Output: 2.0
In this example, we're computing the mean of a list through MATLAB’s superior numerical capabilities, gathered easily within a Python script.
Using Python in MATLAB
Accessing Python Libraries from MATLAB
Conversely, MATLAB provides functionalities to access Python libraries, enabling a seamless blend of Python’s diverse functionality and MATLAB’s powerful numeric computation features.
Example Code Snippet
py.importlib.import_module('numpy');
array = py.numpy.array([1, 2, 3]);
disp(array);
Here, we are demonstrating how to load a Python module and create a NumPy array within MATLAB, showing the reverse capability of dual integration.
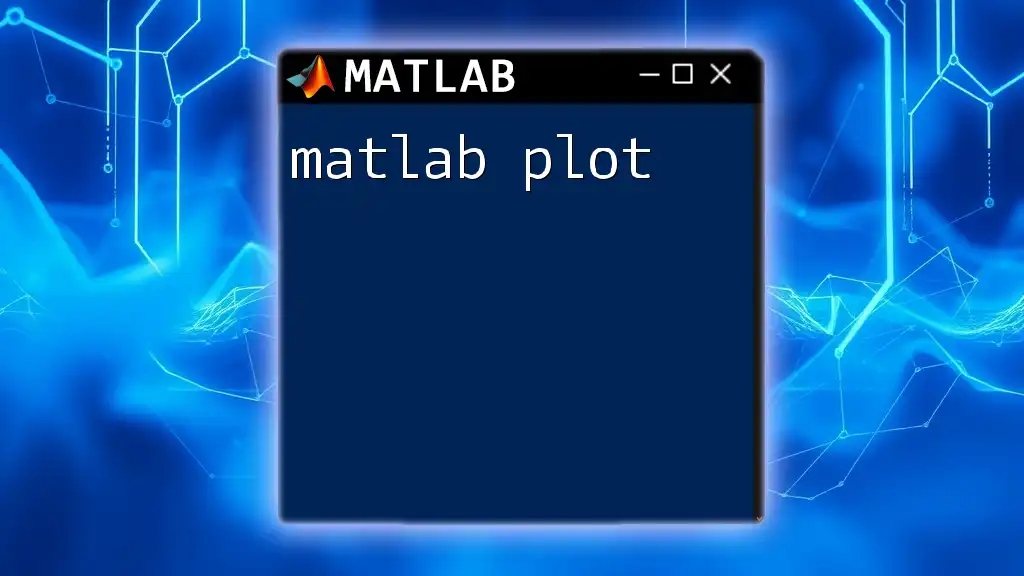
Best Practices for MATLAB and Python Integration
Performance Considerations
Optimizing Code for Efficiency
While integrating MATLAB and Python can boost productivity, performance can suffer if not managed properly. Frequent data transfers between the two can slow down execution speed. It is essential to minimize the back-and-forth communication and preprocess data in one language before handing it over to the other for intensive computations.
Clear Data Communication
Managing Data Types and Structures
Compatibility of data types is key to successful integration. Be cautious when transferring data between MATLAB and Python, ensuring that data structures such as arrays, lists, and matrices align correctly. Utilizing conversion functions can prevent errors and data loss.
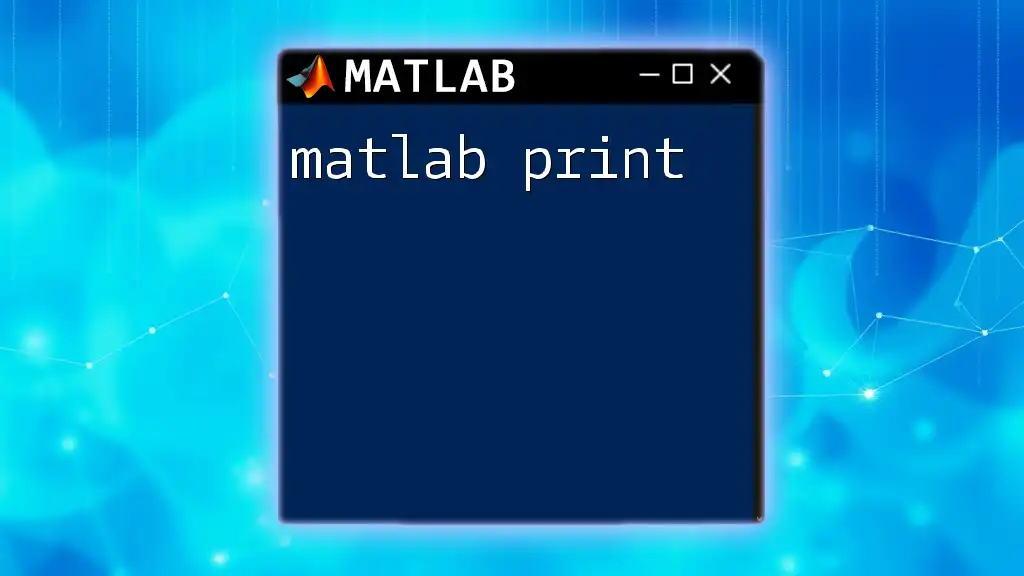
Common Use Cases for MATLAB and Python Integration
Data Analysis and Visualization
Using Python Libraries with MATLAB Visualizations
Integrating MATLAB and Python is particularly beneficial for data analysis tasks. For example, data processed in MATLAB can be visualized using Python’s libraries, allowing users to create interactive plots that engage a broader audience.
Machine Learning and Deep Learning
Combining Machine Learning Algorithms from Both Languages
Projects requiring machine learning often benefit from harnessing the strengths of both languages. Use MATLAB for rapid protyping and algorithm validation while employing Python to leverage advanced libraries like TensorFlow or scikit-learn.
Example Code Snippet
from sklearn.linear_model import LinearRegression
# Python code for training a Linear Regression model can be executed seamlessly with MATLAB's mathematical superiority for pre-processing.
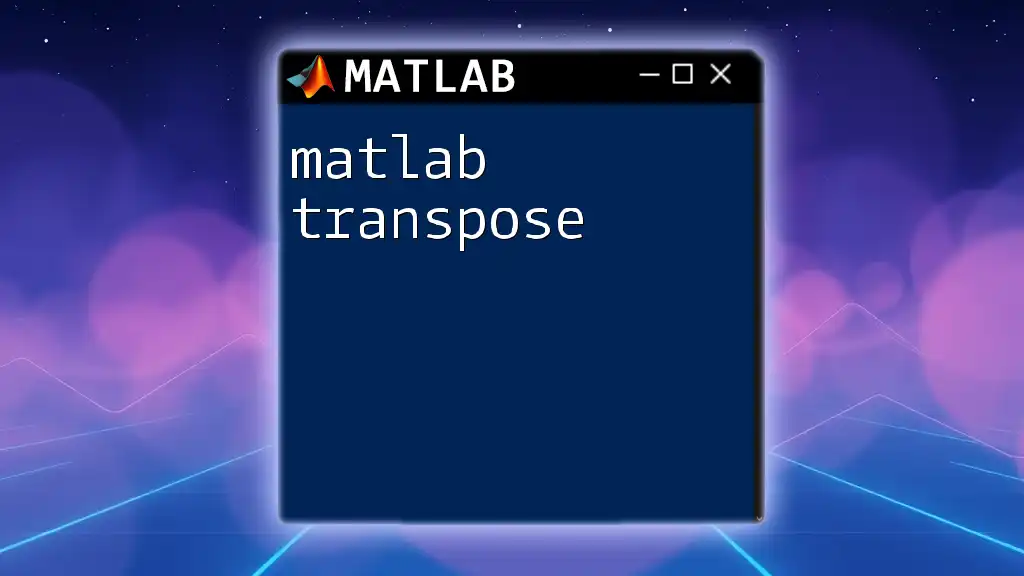
Troubleshooting Common Issues
Error Handling
Typical Errors and Solutions
While integrating MATLAB and Python, you may encounter common errors, such as data type mismatch or communication failures. Ensure that you check compatibility and perform error handling wherever necessary to provide user-friendly feedback and maintain functionality. It’s advisable to keep an eye on variable formats being passed between environments.
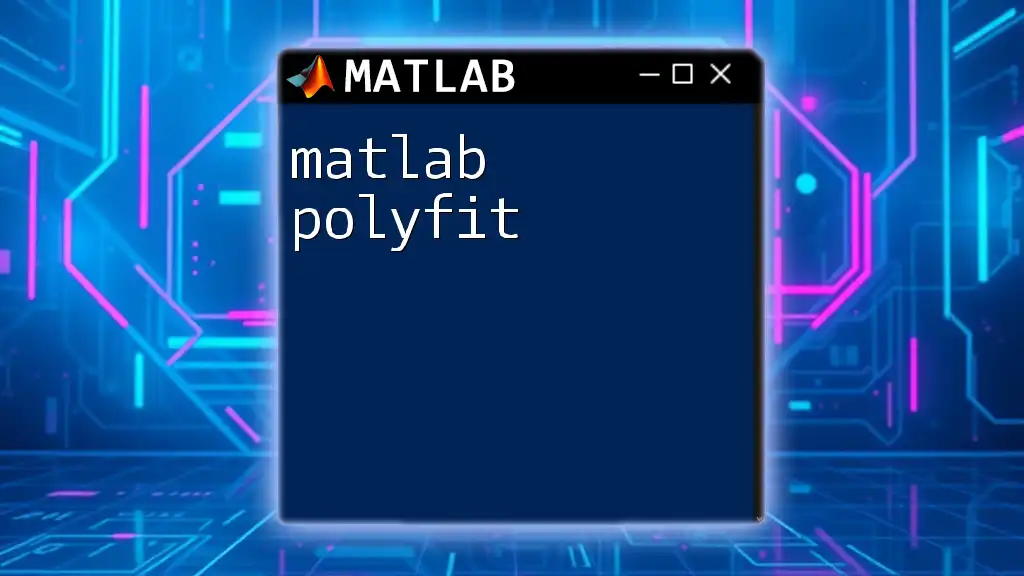
Conclusion
The Future of MATLAB and Python Integration
As technology evolves, we can expect more robust integration between platforms like MATLAB and Python. The demand for efficient, hybrid solutions will continue to grow, making this a key area for future innovations in computational software.
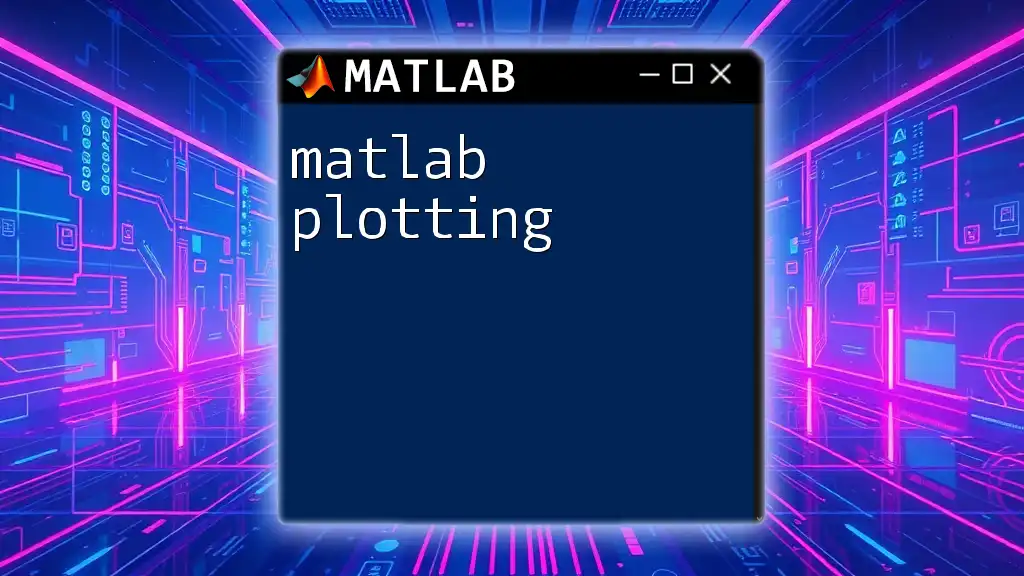
Additional Resources
Further Reading and Tutorials
For those eager to dive deeper, plenty of resources are available, from official documentation and online courses to community forums rich with expert advice. Invest some time to explore these options to enhance your proficiency in both MATLAB and Python.
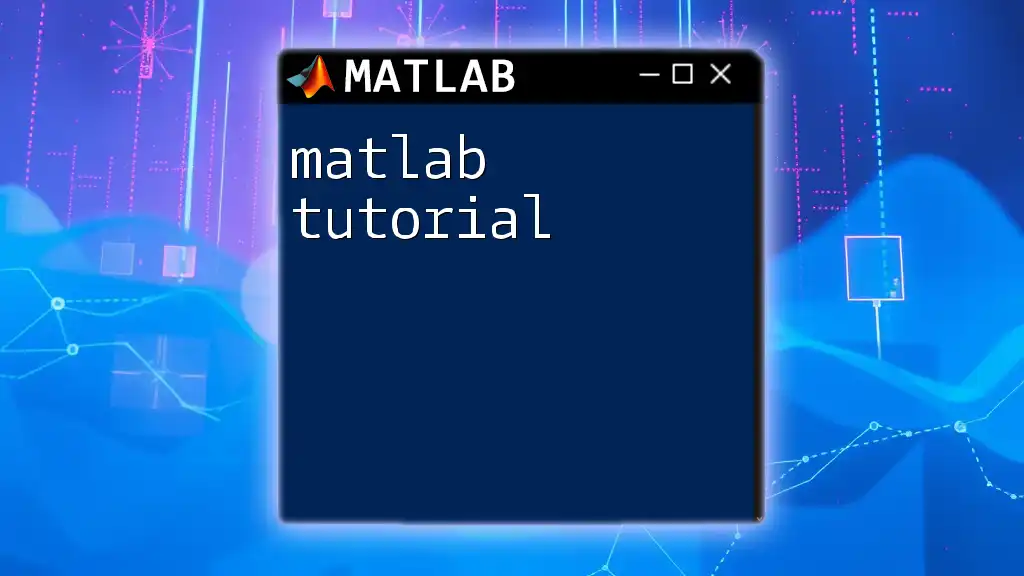
Call to Action
Join Our Community or Enroll in Our Courses
If you’re keen to deepen your knowledge in MATLAB and Python, we invite you to engage with our expert-led courses and community discussions. Embrace this opportunity to refine your skills and stay ahead in the fast-paced world of programming!