The `uigetfile` function in MATLAB opens a dialog box for users to select one or more files from the file system, returning the selected file name(s) and path.
[filename, filepath] = uigetfile({'*.m;*.mat', 'MATLAB Files (*.m, *.mat)'; '*.*', 'All Files (*.*)'}, 'Select a MATLAB file');
Understanding `uigetfile`
What is `uigetfile`?
`uigetfile` is a built-in MATLAB function that opens a graphical user interface (GUI) dialog for file selection. It allows users to navigate their file system to select files without needing to specify the entire path programmatically, making it a powerful tool for enhancing interactivity in your MATLAB scripts and applications.
Why Use `uigetfile`?
The primary advantage of `uigetfile` is its ability to simplify user interactions. This function is especially useful in scenarios where users are selecting files for analysis, visualization, or importation into their MATLAB workspace. By using a GUI for file selection, you reduce the chances of user error in entering file paths and make your MATLAB scripts more user-friendly.
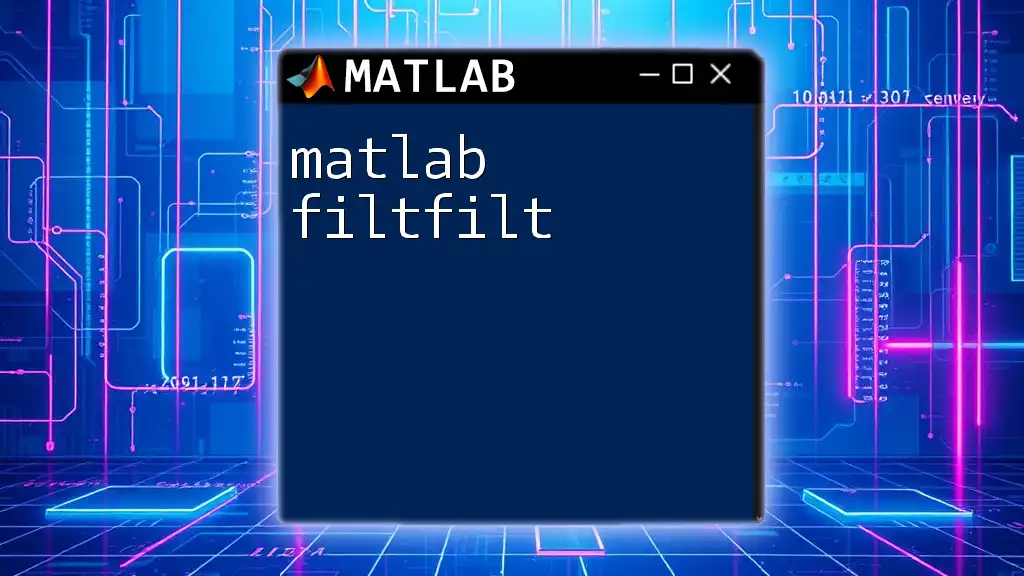
Syntax of `uigetfile`
Basic Syntax
The basic syntax of `uigetfile` is straightforward:
[filename, pathname] = uigetfile('*.txt', 'Select a Text File');
In this example, the filter (`'*.txt'`) limits the file selection to text files, while the title (`'Select a Text File'`) is displayed in the dialog window. The function returns the selected `filename` and the `pathname` where the file is located.
Optional Arguments
`uigetfile` also supports several optional arguments, allowing you to further customize the user experience. The primary optional arguments include:
-
File Filters: Specify which types of files the user can see. For example:
[filename, pathname] = uigetfile({'*.txt;*.csv', 'Text and CSV Files (*.txt, *.csv)'; '*.*', 'All Files (*.*)'}, 'Select a File');
-
Dialog Title: Change the title of the dialog to something more indicative of the task.
-
Multi-File Selection: Allow users to select multiple files at once with the argument `'MultiSelect', 'on'`.
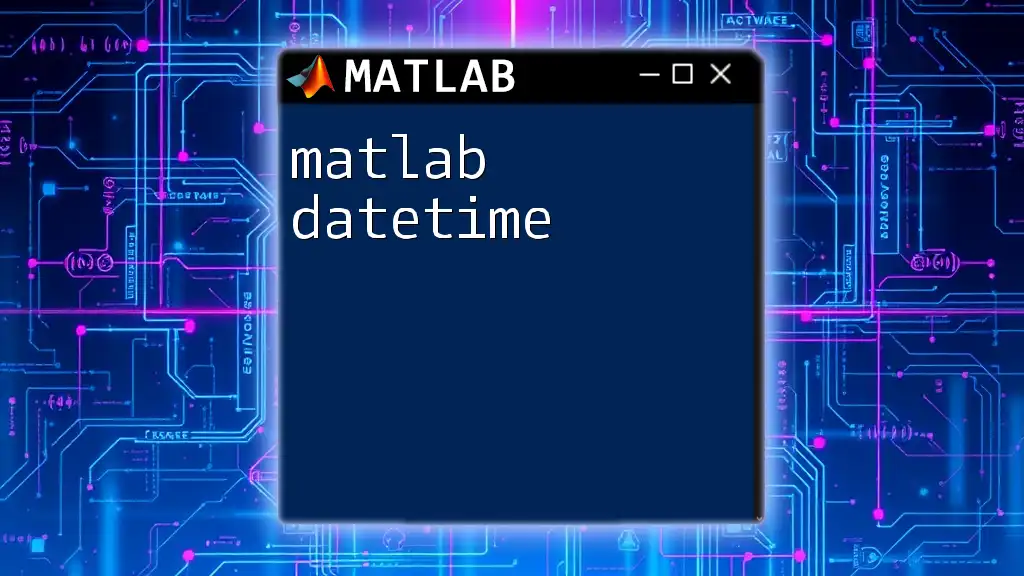
Practical Examples of Using `uigetfile`
Example 1: Selecting a Single File
In this example, we'll create a simple script that allows a user to select a `.mat` file to load into MATLAB:
[file, path] = uigetfile('*.mat', 'Select a MAT-file');
if isequal(file, 0)
disp('User canceled the operation');
else
disp(['Selected file: ', fullfile(path, file)]);
end
This script checks if the user canceled the operation. If not, it displays the full path of the selected file. This kind of interaction is essential when dealing with data that users might not remember the path for.
Example 2: Filtering File Types
`uigetfile` allows for filtering file types effectively. Below is an example where a user only sees image files in the dialog:
[file, path] = uigetfile({'*.png;*.jpg', 'Image Files (*.png, *.jpg)'}, 'Select an Image File');
In this case, users only see images in the specified formats, reducing confusion and speeding up the selection process.
Example 3: Using `uigetfile` in a Function
Creating a MATLAB function that uses `uigetfile` can enhance reusability. Here's an example function that allows users to load CSV files into MATLAB:
function data = loadData()
[file, path] = uigetfile('*.csv', 'Select a CSV File');
if isequal(file, 0)
error('File selection canceled');
end
data = readtable(fullfile(path, file));
end
In this function, if the user cancels the operation, an error is raised, ensuring that the script doesn’t proceed without a valid file.
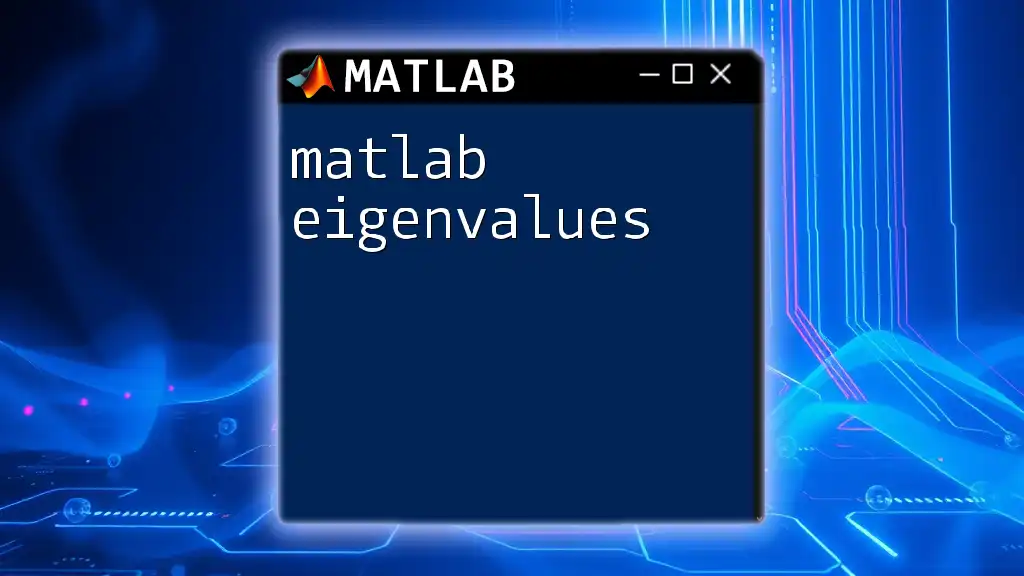
Tips for Effective Usage of `uigetfile`
Customizing Dialog Appearance
You can customize the dialog to enhance user experience. For instance, enabling multi-file selection can be done with the following code:
[files, path] = uigetfile('*.m', 'Select MATLAB Files', 'MultiSelect', 'on');
In this case, `files` will be an array of selected file names, allowing for batch processing of files.
Error Handling
Error handling is crucial when working with `uigetfile`. It's important to ensure that the program can gracefully handle situations where no file is selected:
if isequal(files, 0)
disp('No file selected');
else
disp(['Files selected: ', strjoin(files, ', ')]);
end
This provides clear feedback to users if they cancel the operation or if something goes wrong.
Integrating with Other Commands
The integration of `uigetfile` with other MATLAB commands allows users to create a seamless flow in their scripts. Here’s an example that reads an Excel file after selection:
[file, path] = uigetfile({'*.xlsx', 'Excel Files (*.xlsx)'});
if ~isequal(file, 0)
data = readtable(fullfile(path, file));
end
This script combines file selection with data importation, allowing users to efficiently load their data into MATLAB for analysis.
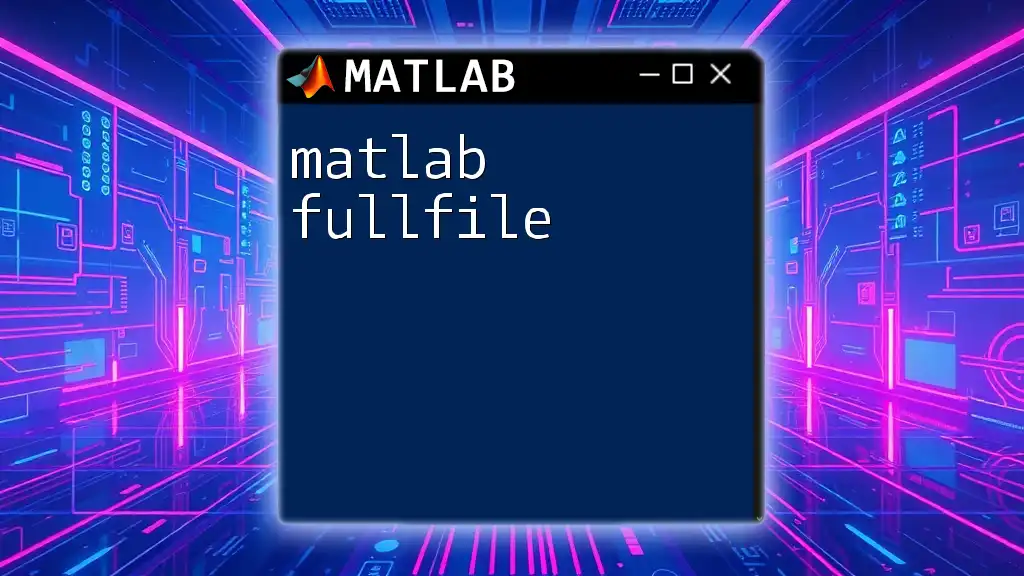
Conclusion
Through the effective use of MATLAB `uigetfile`, you enhance user interaction significantly and streamline the process of file selection. By incorporating this function into your MATLAB scripts, you enable your applications to be more intuitive and reduce the common pitfalls associated with file path management. Now, as you progress in your MATLAB programming journey, don’t hesitate to experiment with `uigetfile` and see how it transforms your user experience. Engage with the MATLAB community and explore new ways to elevate your programming skills!
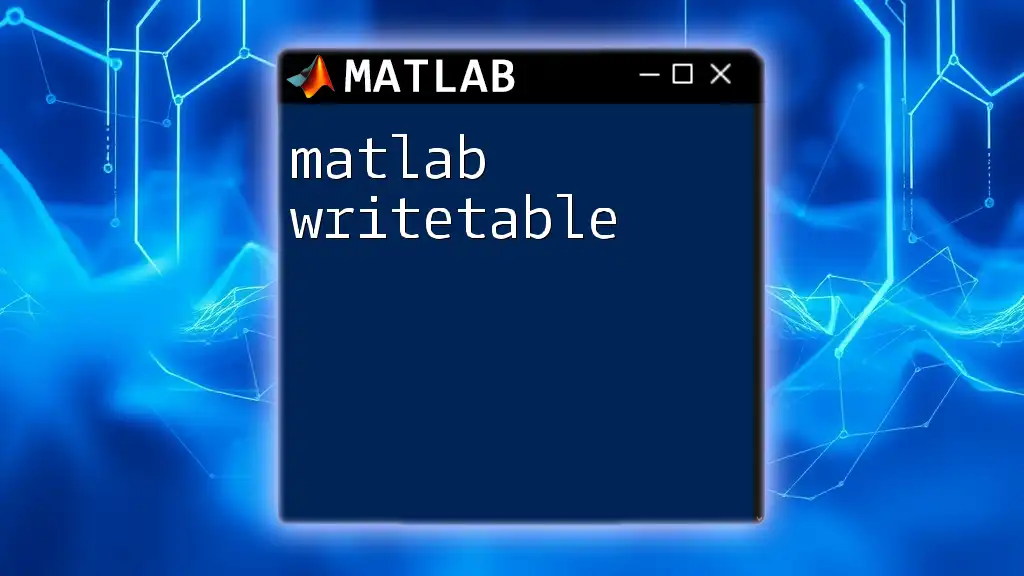
Frequently Asked Questions (FAQ)
What types of files can I select using `uigetfile`?
You can select any types of files that your system recognizes, depending on the filters you provide. By specifying filters like `'.txt'`, `'.csv'`, or custom filters, you can control what the user sees in the dialog.
Can I select multiple files using `uigetfile`?
Yes! By using the optional argument `'MultiSelect', 'on'`, users can select multiple files at once, making it efficient for batch processing tasks.
How can I get the path of the selected file?
The `uigetfile` function returns two outputs: `filename` and `pathname`. By combining these, you can create the full path to the selected file, which is crucial for subsequent operations that depend on the file's location.