The MATLAB Profiler is a tool that helps you analyze the performance of your MATLAB code by identifying bottlenecks and measuring execution time for different functions.
Here's a simple code snippet to get you started with the MATLAB Profiler:
profile on; % Start the profiler
% Your MATLAB code here
pause(1); % Example code to simulate a time-consuming operation
profile off; % Stop the profiler
profile viewer; % Open the profiler report
Understanding MATLAB Profiler
What is MATLAB Profiler?
The MATLAB Profiler is a powerful built-in performance analysis tool designed to help users identify inefficiencies in their MATLAB code. By understanding where the time is being spent during execution, developers can pinpoint bottlenecks and optimize their code performance. Profiling is essential not only for improving code execution speed but also for enhancing overall efficiency—crucial in applications that demand high performance.
How MATLAB Profiler Works
MATLAB Profiler operates by tracking various performance metrics while your code runs. During the profiling process, it monitors events such as function calls, execution timings, and memory usage. By gathering this data, MATLAB creates a detailed report that allows users to analyze the performance of each function within their scripts or applications.
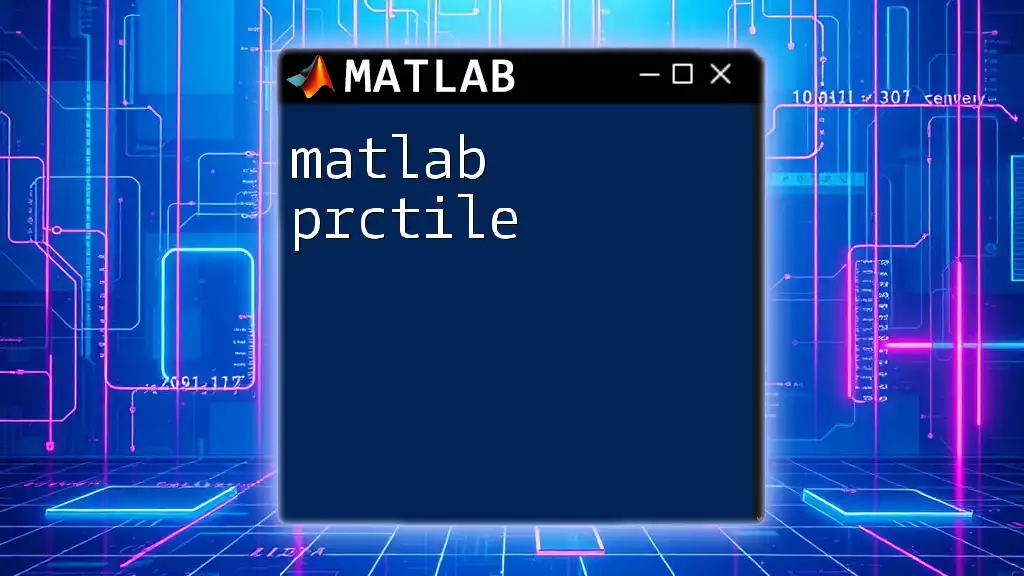
Getting Started with MATLAB Profiler
Enabling the Profiler
To start profiling your code, you must first enable the profiler using the command:
profile on
This command tells MATLAB to begin recording information about function calls and execution times. It's the initial step in your profiling journey. Once you have executed the necessary code sections, you will need to stop profiling:
profile off
Running Your Code with the Profiler
After enabling the profiler, you can run your function or script as usual. Here is a simple example to illustrate this:
profile on
myFunction(); % Replace with your specific function
profile off
In this example, replace `myFunction` with your actual function that you wish to analyze. Once profiling is turned off, MATLAB will collect all the relevant performance data.
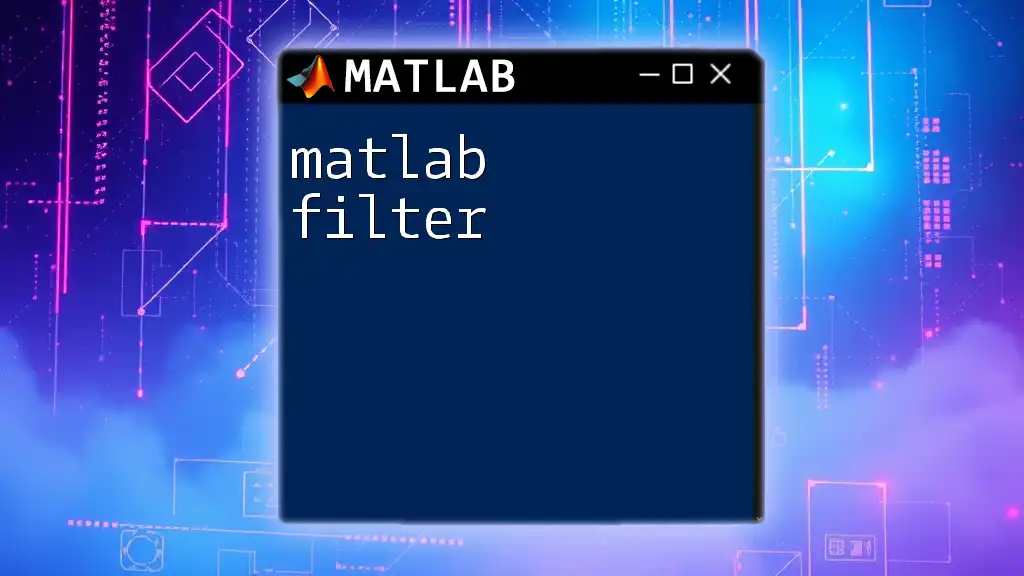
Analyzing Profiler Results
Accessing Profiler Report
To view the profiling results, use the following command:
profile viewer
Executing this command opens a graphical user interface (GUI) that presents the profiling information collected. This report includes a wealth of insights into your code's execution performance.
Understanding the Profiler Output
A typical profiler output contains several critical components that need interpretation. Here, you will find columns representing the execution time, the number of calls made to each function, and more. Understanding how to navigate this information is crucial as it allows you to see not just where time is spent, but also how many times functions are invoked.
Common Terms Explained
To make the most of profiler outputs, it's important to understand a few key terms:
-
Self Time vs. Total Time: Self time signifies the time spent within a function itself, while total time includes the time spent in all the functions called by it. Identifying high self time functions is a priority, as it often points to inefficiencies.
-
Function Calls Count: This indicates how many times a function is executed during the profiling session. A high number of calls might highlight potential areas for optimization.
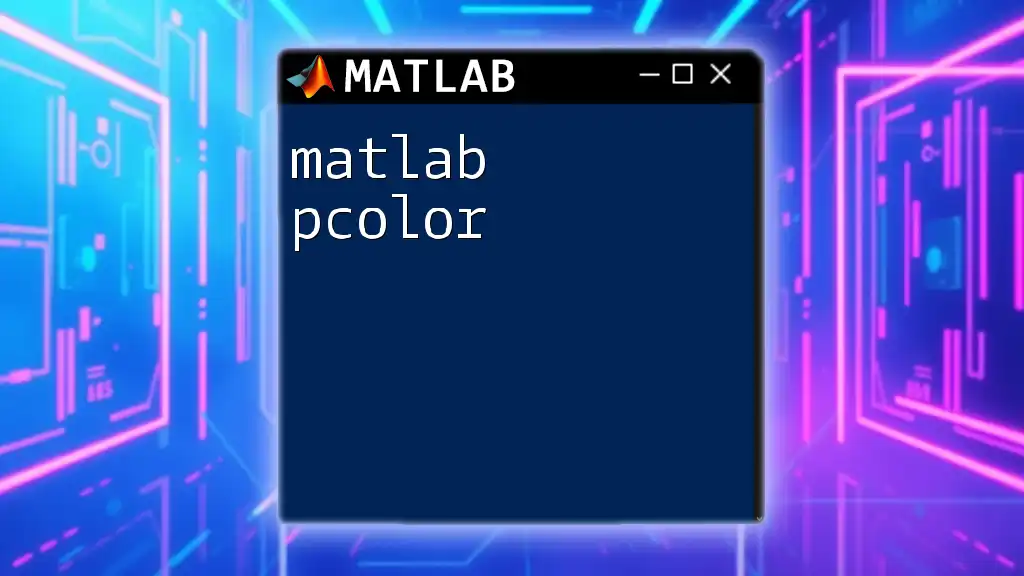
Interpreting Profiling Data
Identifying Performance Bottlenecks
One of the primary goals of using the MATLAB Profiler is to find performance bottlenecks. By examining the report, you can identify functions that take the longest execution time. Focus on these functions for potential refactoring and optimization.
Analyzing Recursive Functions
Recursive functions often require special attention during profiling, as they can create complex behavior that skews output data. Use profiling to examine how often the recursive calls occur and assess if the recursion depth can be reduced or if iterative solutions might be more efficient.
Visualizing Data
MATLAB's GUI provides a clear and comprehensive visualization of profiling data. Here, you can easily navigate through function call hierarchies, view heat maps of execution times, and quickly spot performance issues.
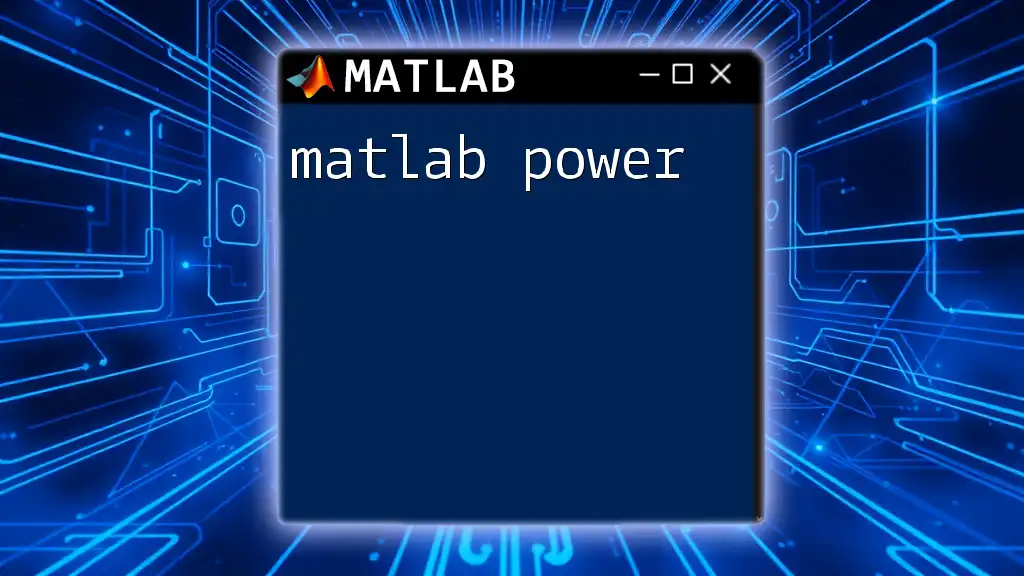
Optimizing MATLAB Code
Best Practices for Performance Optimization
To enhance your coding efficiency, consider adopting various best practices:
-
Vectorization: Vectorized code allows you to perform operations on whole arrays at once rather than iterating over them with loops. This greatly increases performance efficiency.
% Inefficient version for i = 1:length(A) B(i) = A(i) * 2; end % Efficient vectorized version B = A * 2;
-
Preallocation of Arrays: Always preallocate space for arrays when possible. This can significantly reduce execution time, especially in larger loops.
Using Built-in Functions
MATLAB provides a vast array of built-in functions that are heavily optimized for speed and efficiency. Whenever possible, try to utilize these functions instead of writing custom code. They are often faster and more reliable because they have been tested and optimized for performance.
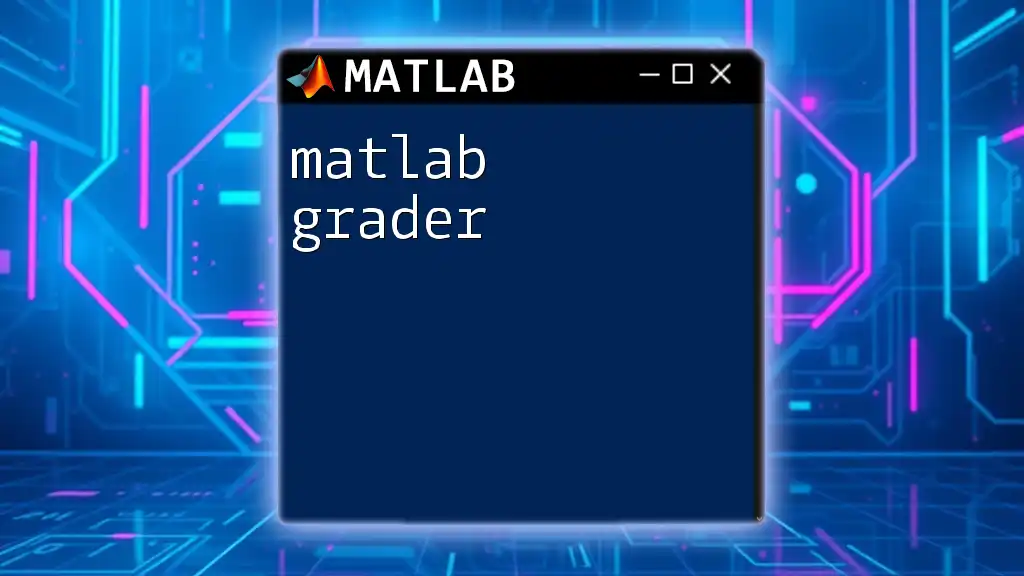
Advanced Profiling Techniques
Profiling in Parallel Computing
When working with parallel computing, consider that profiling might behave differently. The profiler can still provide insights, but it’s essential to understand that certain metrics may be less relevant. Implementing profiling techniques tailored for parallel processes can ensure you gain accurate insights.
Analyzing Memory Usage
In addition to execution time, it is vital to analyze memory usage. MATLAB offers the `memory` command, which you can use to view the memory consumption of your workspace. Understanding how your code utilizes memory can lead to optimizations that greatly improve performance, particularly in memory-intensive applications.
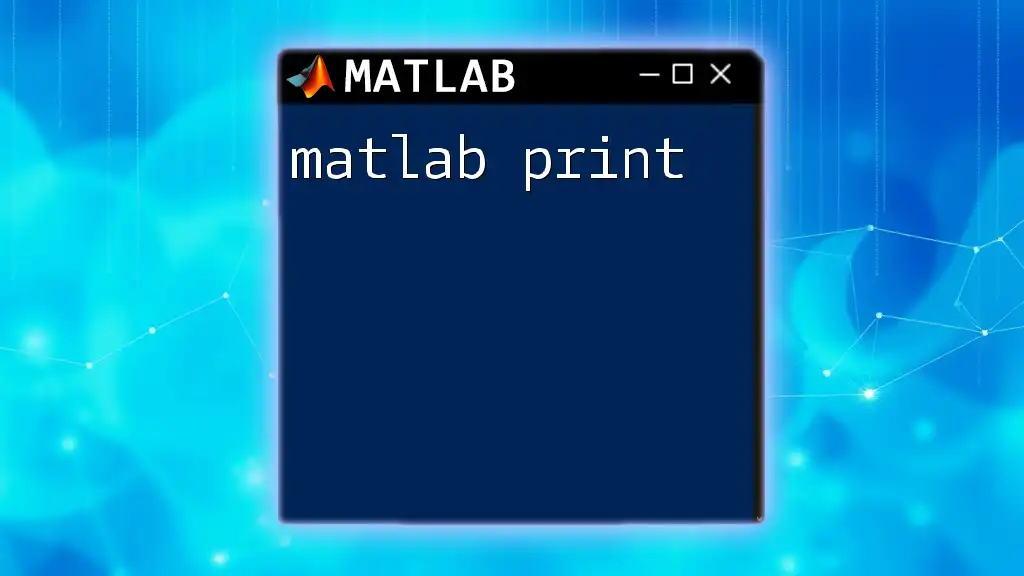
Real-World Examples
Case Study: Function Optimization
Considering a real-world example can illuminate the power of profiling. Imagine a complex function that initially takes a substantial amount of time to execute. By profiling this function, you may discover that a particular algorithm is inherently inefficient. After refactoring the code based on profiling data, performance improves significantly, providing clear evidence of the impact of using the MATLAB Profiler.
Comparing Different Approaches
It’s often beneficial to compare different approaches to achieve the same outcomes. For instance, implementing two different algorithms for solving a problem and profiling both can yield insights into their performance metrics. This methodical approach gives you a clearer picture of which implementation is preferable in terms of speed and efficiency.
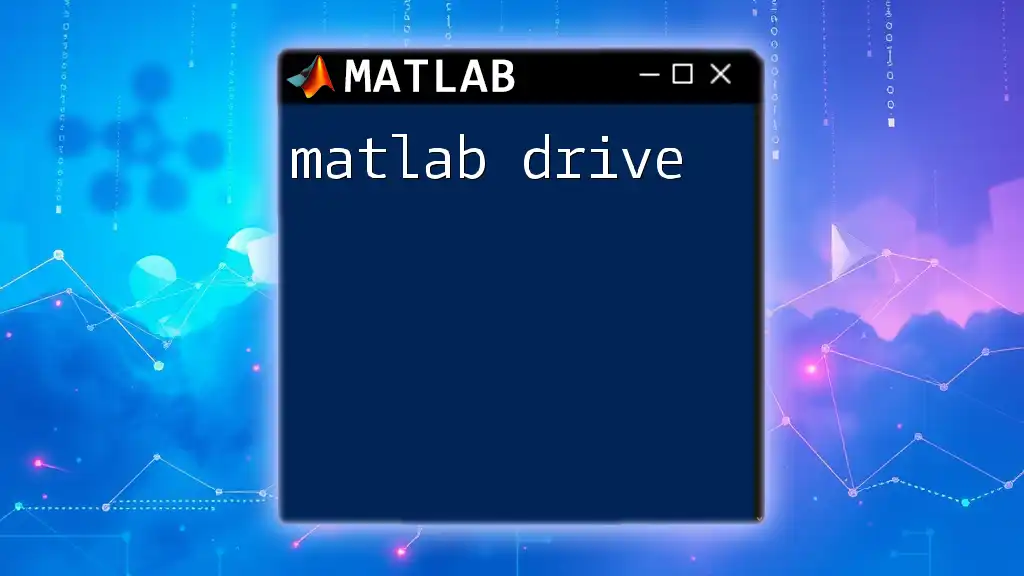
Common Pitfalls and Misconceptions
Misunderstanding Profiling Outputs
Many users misinterpret the results obtained from profiling. It's crucial to understand that not all time spent in a function reflects inefficiency. Sometimes, time-consuming functions are performing necessary tasks that cannot be easily optimized.
Over-Optimization
A common trap that programmers fall into is the lure of premature optimization. This involves optimizing parts of the code without concrete evidence from profiling data. It's essential to focus on measured performance issues rather than assumptions to avoid unnecessary complications and delays in development.
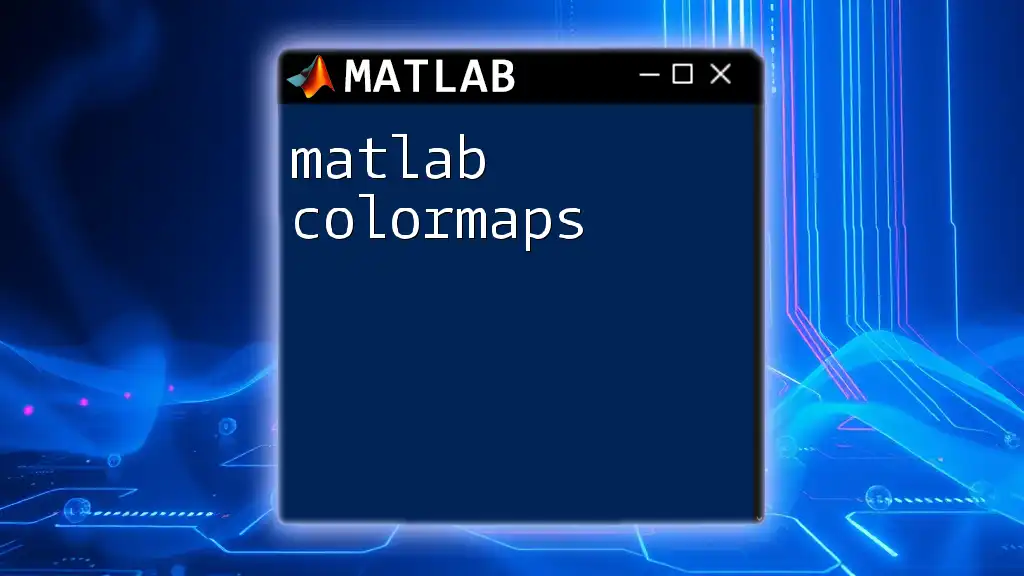
Conclusion
Summary of Key Takeaways
In summary, regular use of the MATLAB Profiler can uncover significant opportunities for improving code performance. It is essential to profile consistently throughout the development process rather than waiting until the end to assess your performance.
Encouragement to Explore Further
Consider diving deeper into MATLAB documentation, books, and online courses for further exploration of MATLAB Profiler. Leveraging these resources will enhance your understanding and capabilities, facilitating better coding practices.
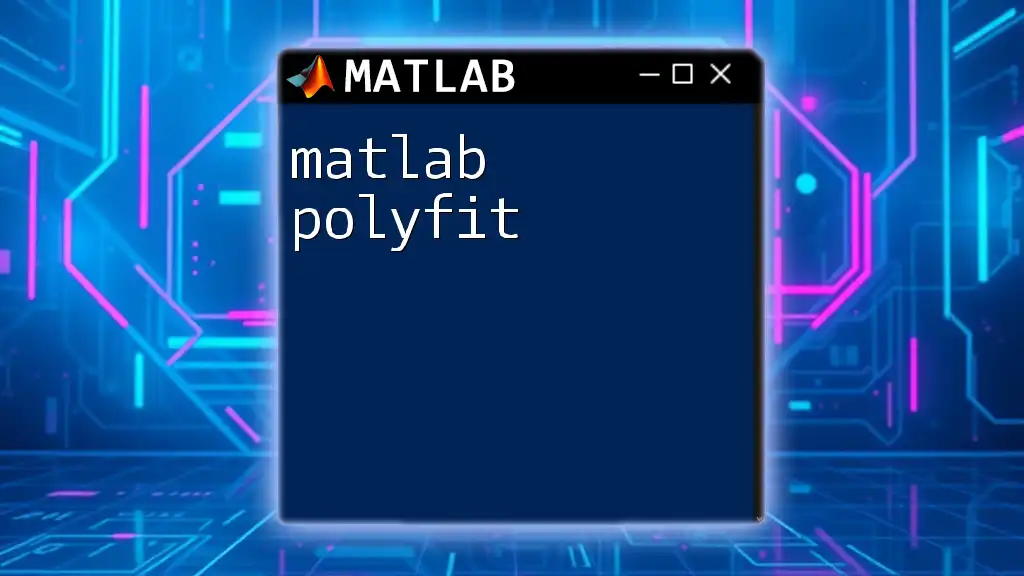
FAQs About MATLAB Profiler
What is the quickest way to analyze code performance?
The quickest way to analyze code performance is to enable the profiler, run your code, and then use `profile viewer` to access the detailed report. This immediate feedback allows for quick identification of problematic areas.
How can I check which functions slow down my script?
Use the MATLAB Profiler to analyze the execution time of all functions within your script. By examining the profiler report, look for functions with high execution times and call counts to determine where optimizations are needed.
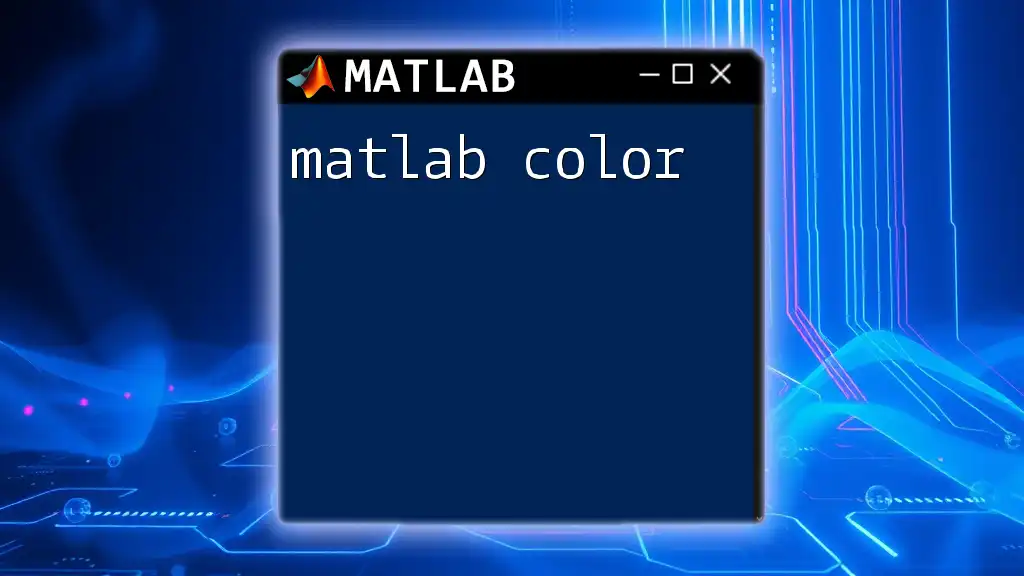
Additional Resources
For further reading, check out the official MATLAB documentation, which provides in-depth explanations and examples related to the profiler and other optimization techniques. Joining community forums can also be beneficial, as they offer platforms for discussion and support among MATLAB users.