The `prctile` function in MATLAB computes the percentiles of a data set, allowing users to determine the value below which a given percentage of observations falls.
Here’s an example of how to use the `prctile` function:
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; % Sample data
percentiles = prctile(data, [25, 50, 75]); % Calculate the 25th, 50th, and 75th percentiles
Understanding Percentiles
Definition of Percentiles
Percentiles are statistical measures that divide a dataset into 100 equal parts. Each percentile indicates the relative standing of a value within the dataset. For example, the 25th percentile marks the value below which 25% of the data falls. Percentiles are critical in determining the distribution and variation of data points, allowing analysts to summarize large datasets effectively.
How Percentiles are Used in Data Analysis
Percentiles are particularly useful in various fields such as education, finance, and healthcare. They help analysts interpret data patterns and shifts. For instance, in educational assessments, the 50th percentile can signify the median score, while the 90th percentile may indicate exceptional performance in a particular subject. In finance, understanding income distribution through percentiles allows businesses to identify target market segments.
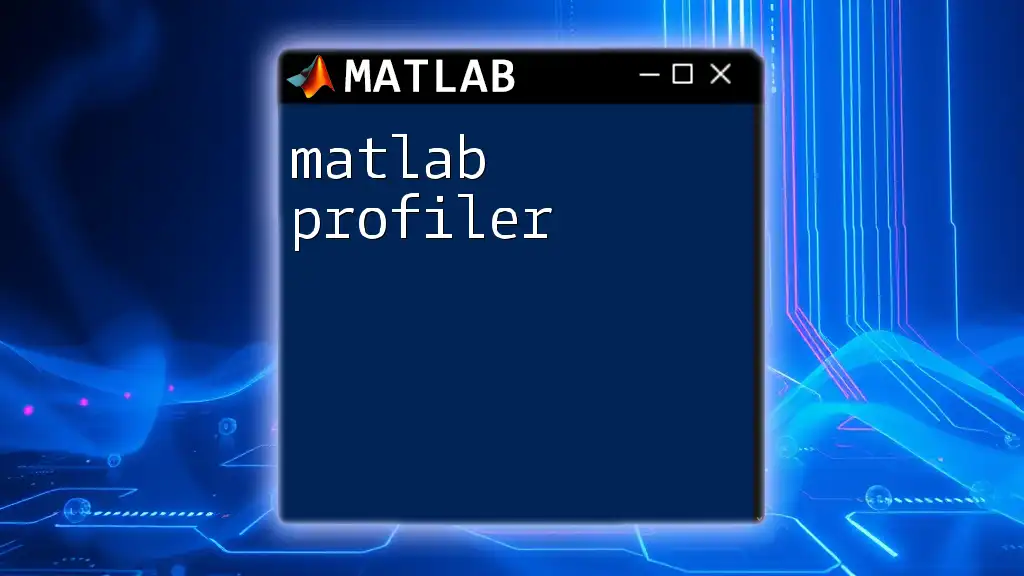
Overview of MATLAB `prctile`
What is MATLAB `prctile`?
In MATLAB, `prctile` is a function specifically designed to calculate percentiles of data arrays. It streamlines the process of assessing data distributions by providing direct percentage measures. This function is invaluable for data scientists and analysts who need to analyze and interpret data quickly.
Syntax of `prctile`
The syntax for the `prctile` function in MATLAB is straightforward, comprising the following components:
-
Basic syntax:
P = prctile(data, percentiles)
-
Input arguments:
- data: An array containing the data to analyze.
- percentiles: A scalar or vector indicating the percentiles to compute (between 0 and 100).
-
Output results: The output, `P`, corresponds to the calculated percentiles based on the provided data.
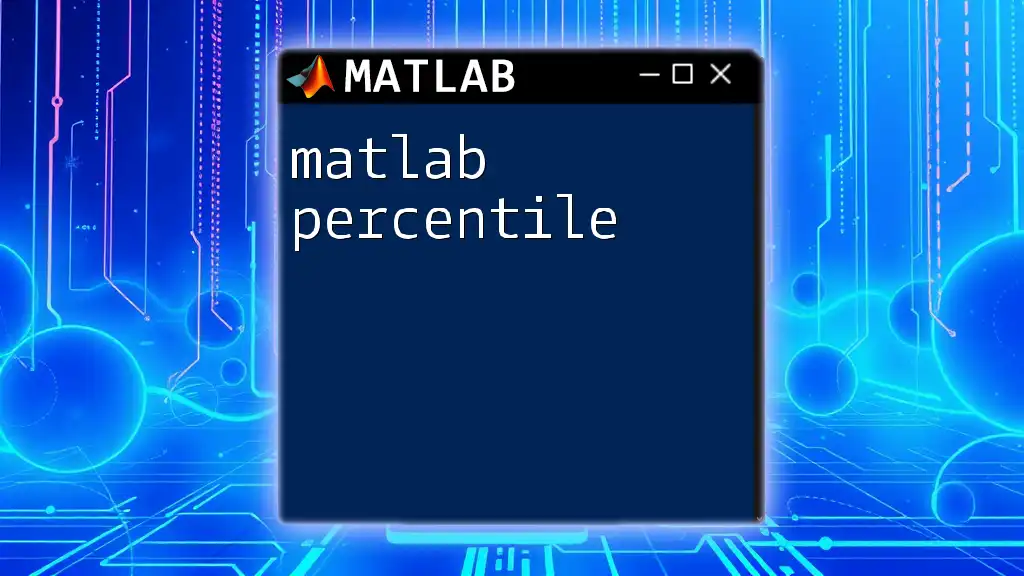
How to Use `prctile` in MATLAB
Basic Usage Examples
Example 1: Calculating Simple Percentiles
To calculate a basic percentile from a randomly generated dataset, you can use:
data = rand(100, 1); % Generate 100 random numbers
p = prctile(data, 25); % Calculate the 25th percentile
disp(p);
In this example, `data` consists of 100 random numbers, and `prctile` will return the 25th percentile, which gives insight into the lower quarter of the data distribution.
Example 2: Finding Multiple Percentiles
You can also retrieve multiple percentiles simultaneously:
data = rand(100, 1);
p = prctile(data, [25 50 75]); % Calculate 25th, 50th, and 75th percentiles
disp(p);
This code snippet calculates and displays the 25th, 50th, and 75th percentiles, providing a larger context for understanding the data distribution.
Advanced Usage
Using `prctile` with Multidimensional Arrays
MATLAB's `prctile` function can compute percentiles across different dimensions of an array. By using the dimension argument, users can specify whether to calculate percentiles across rows or columns:
data = rand(4, 5);
p = prctile(data, 75, 1); % 75th percentile for each column
In this case, the 75th percentile is calculated for each column in the matrix `data`, providing insight into the performance across different features.
Handling Missing Data
Dealing with missing data is another crucial aspect when using `prctile`. MATLAB's implementation of `prctile` returns NaN if any input contains NaN values. To handle this, one must often clean the dataset first:
data = [1; 2; NaN; 4; 5];
p = prctile(data(~isnan(data)), 50); % 50th percentile ignoring NaN
In this example, the `isnan` function filters out NaN entries before calculating the percentile, ensuring accurate results.
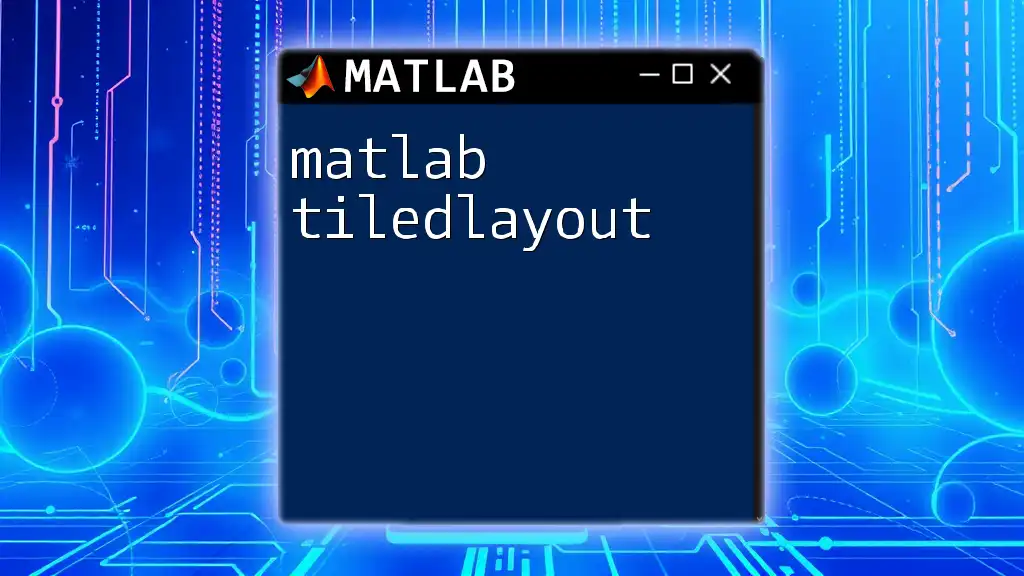
Practical Applications of `prctile`
Data Analysis in Research
In research, percentiles are used for comparative analysis. By utilizing the `prctile` function in MATLAB, researchers can evaluate how groups perform over time. For example, analyzing test scores of students can help educators determine effectiveness by comparing performance across different percentiles.
Financial Applications
Percentiles play a significant role in finance, particularly in risk assessment and investment analysis. By applying the `prctile` function, analysts can understand investment returns and determine where an investment stands relative to market trends. This helps in strategizing asset allocations.
Marketing and Customer Analytics
In the marketing domain, percentiles can aid in customer segmentation. Businesses often use the `prctile` function to identify high-value customers by calculating the top percentiles of customer spending or engagement. This enables targeted marketing efforts and resource allocation.
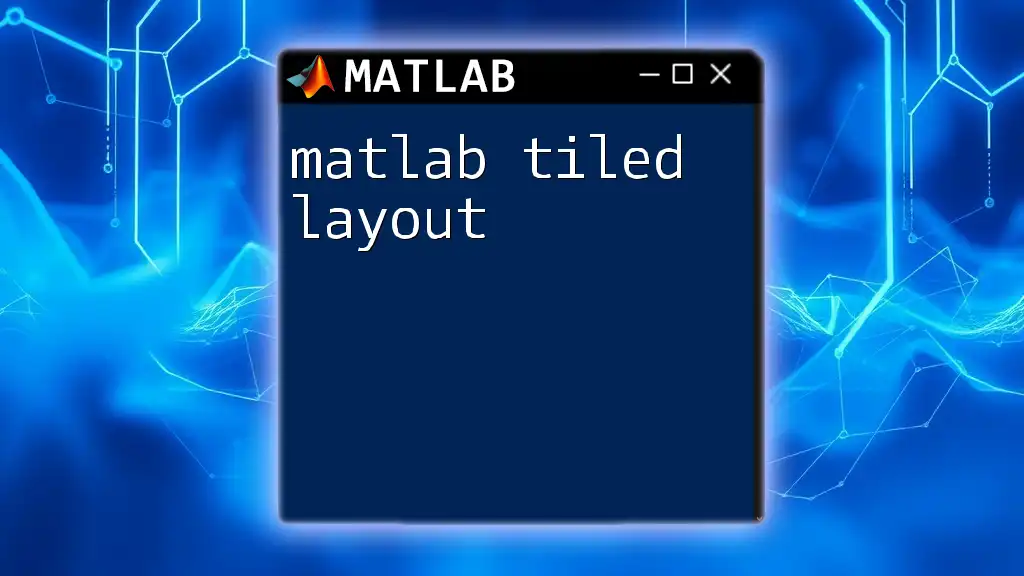
Visualization of Percentiles
Using MATLAB for Data Visualization
Visual representation of percentiles greatly enhances data analysis. MATLAB provides powerful plotting tools to represent distributions clearly. For instance, you can plot a histogram of your data and overlay horizontal lines for key percentiles:
data = randn(1000, 1); % Normally distributed data
histogram(data);
hold on;
yline(prctile(data, 25), 'r--', '25th Percentile');
yline(prctile(data, 50), 'g--', '50th Percentile');
yline(prctile(data, 75), 'b--', '75th Percentile');
hold off;
This code snippet generates a histogram illustrating the overall distribution of the data, while the horizontal lines represent the 25th, 50th, and 75th percentiles, visually indicating significant benchmarks.
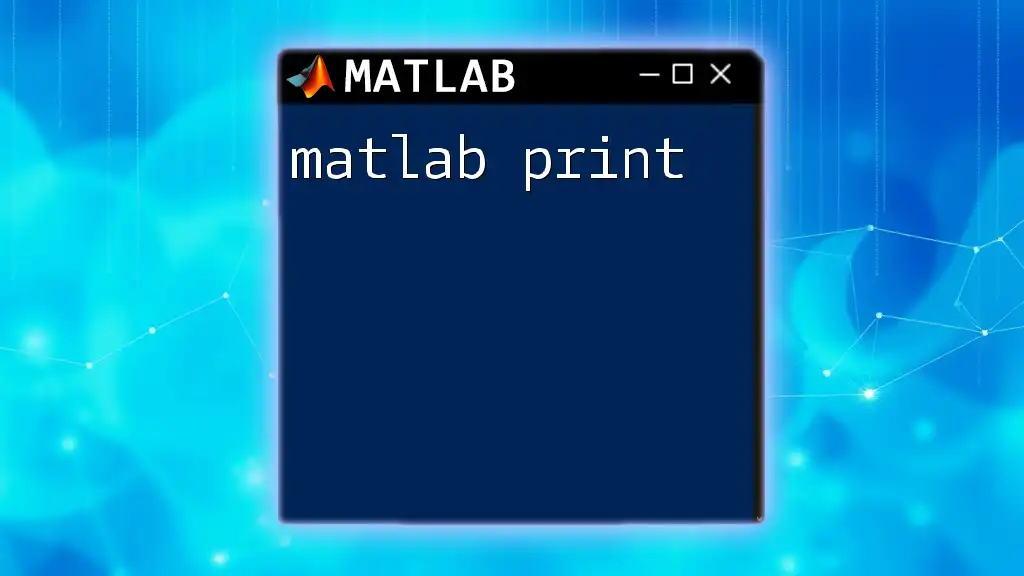
Tips and Best Practices
Efficiently Using `prctile`
When working with large datasets, efficiency is key. Consider organizing data and checking for NaN values before using `prctile`. Ensure that you utilize the dimension argument effectively when calculating percentiles across multidimensional data.
Combining with Other Functions
The `prctile` function can be used in conjunction with other statistical functions such as `mean`, `median`, and `std`. This can provide comprehensive insights into your data analysis. For instance, comparing the mean with the 75th percentile can help you understand skewness in your data distribution.
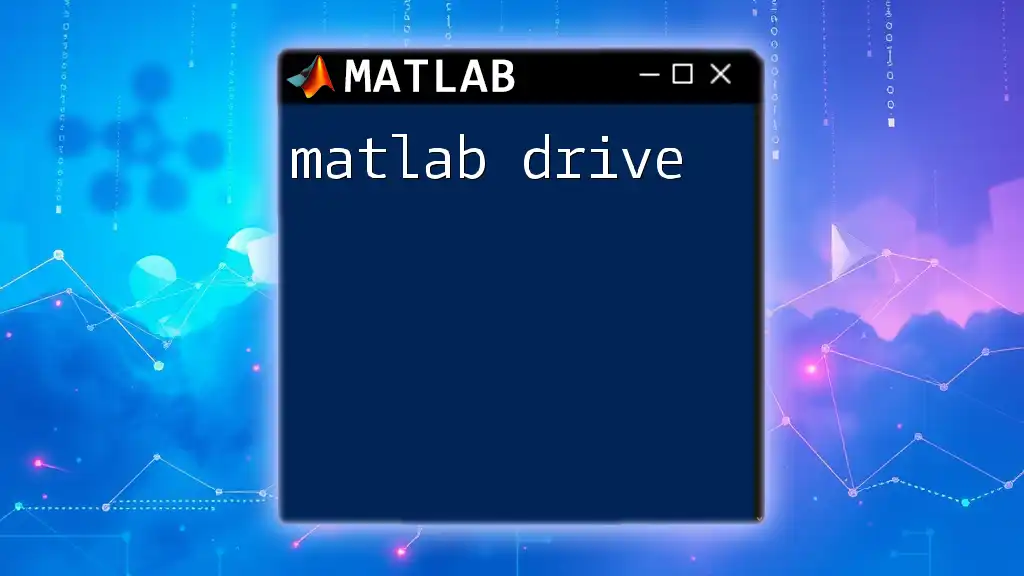
Conclusion
The MATLAB `prctile` function is an essential tool for anyone working with statistical data. Understanding its syntax, usage, and potential applications empowers data analysts and researchers to derive meaningful insights quickly. By mastering how to compute and visualize percentiles, you can enhance your data analysis skills significantly, allowing for more informed decision-making in various fields.
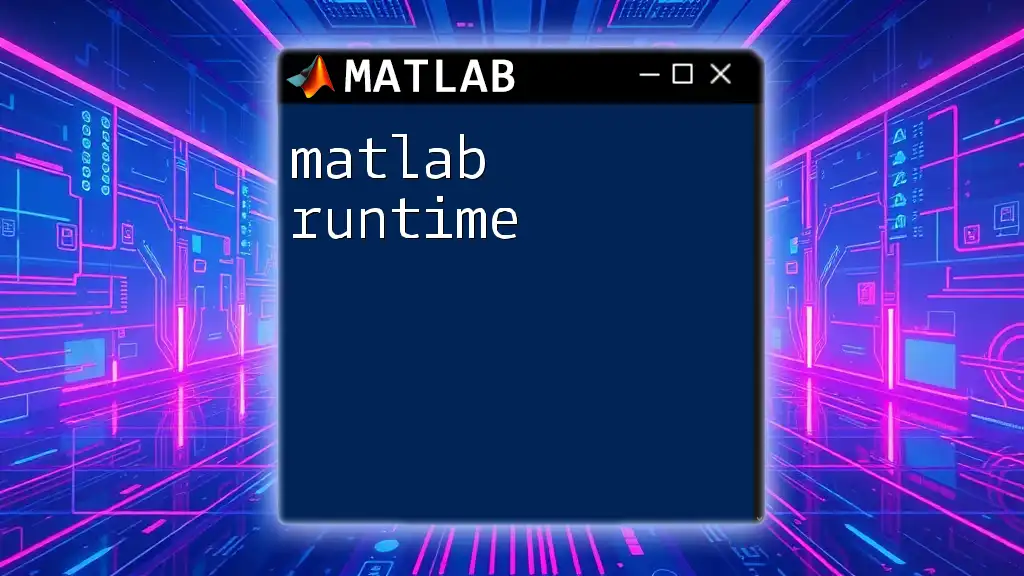
Additional Resources
For further reading and exploration, consider checking the official MATLAB documentation for `prctile`, as well as online tutorials and courses tailored for more in-depth learning about statistical analysis in MATLAB.