The `fullfile` function in MATLAB constructs a full file path from individual folder and file name components, ensuring the correct file separators are used for the operating system.
Here’s a code snippet demonstrating its usage:
filePath = fullfile('C:', 'Users', 'Username', 'Documents', 'myfile.txt');
Understanding Path Construction
When working with MATLAB, managing file paths correctly is essential. Using the matlab fullfile function, you can effectively build file paths while ensuring compatibility across different operating systems. Inconsistent path usage can lead to run-time errors and difficulty in locating files, thus hindering your workflow.
The Role of Base Paths in MATLAB
Base paths serve as the foundation for all your file interactions in MATLAB. Whether reading data from a file or saving outputs to a designated directory, outlining clear and consistent base paths helps you stay organized. Often, you might have separate folders for scripts, data, and results, which can be hierarchically structured.
Why Proper Path Construction Matters
Path errors can result in a frustrating debugging experience. In many cases, hardcoding paths (manually typing out complete directory structures) introduces a range of issues, especially when sharing code across different operating systems. This is where using the matlab fullfile function shines by abstracting these complexities.
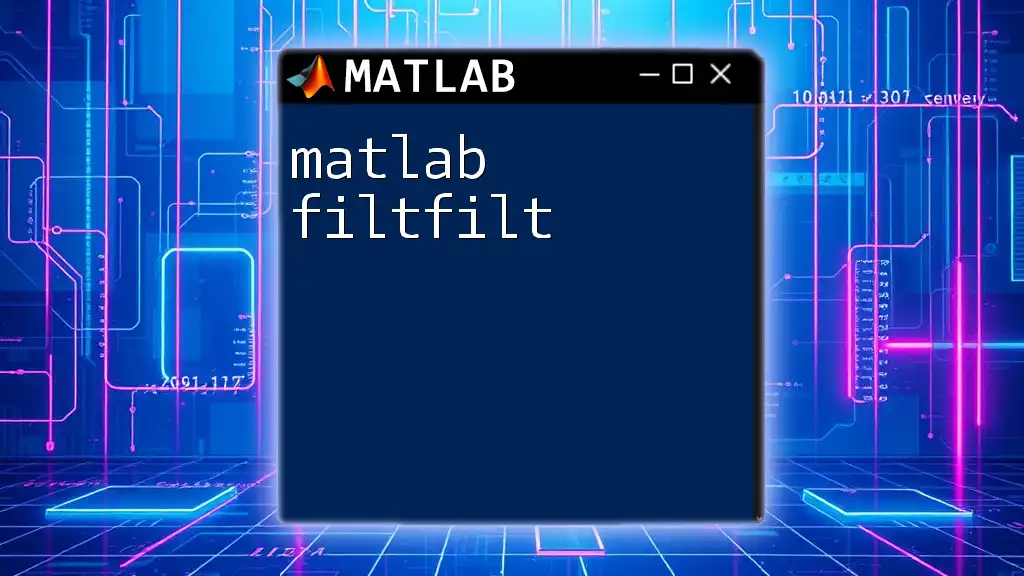
The Syntax of fullfile
The syntax of the matlab fullfile function is straightforward, allowing you to concatenate folder paths and file names seamlessly.
-
Basic Syntax:
fullfile(folderPath, filename)
-
Handling Multiple Arguments:
fullfile(path1, path2, …)
Using this syntax, you can create a path that is automatically adjusted to use the correct file separator for the operating system, whether it be a forward slash (`/`) or a backslash (`\`).
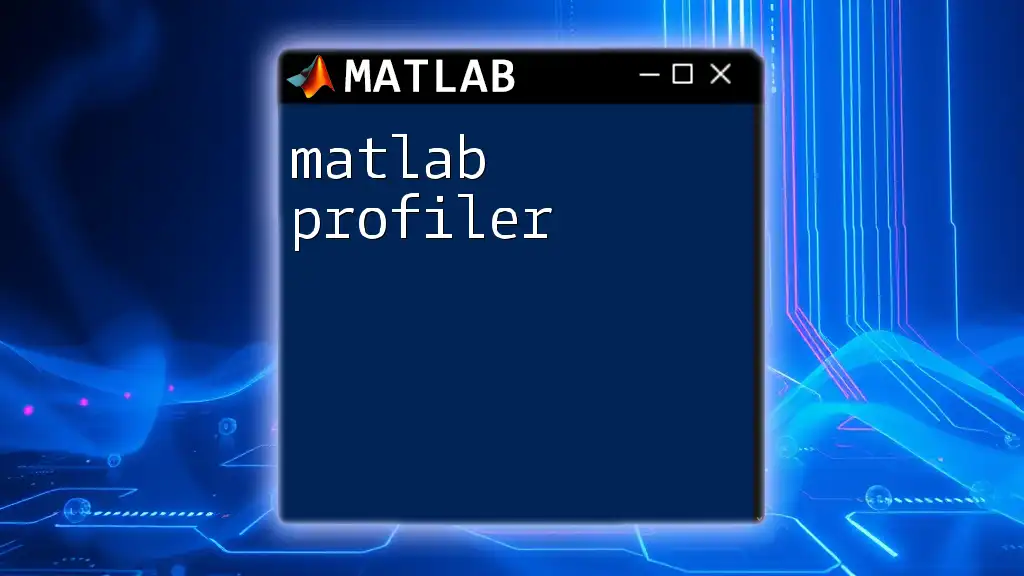
How fullfile Works
The matlab fullfile function effectively streamlines the process of creating file paths. One of its key features is automatic handling of file separators. This removes the guesswork, making your code more robust and easier to read.
Example: Basic Usage of fullfile
For a simple demonstration, consider the following code snippet:
folderPath = 'C:\Data';
filename = 'results.mat';
fullPath = fullfile(folderPath, filename);
disp(fullPath);
In this example, fullfile concatenates `folderPath` and `filename` into a single string that represents the complete file path. The expected output will be:
C:\Data\results.mat
This output showcases how matlab fullfile handles the file separator automatically, streamlining your path management.
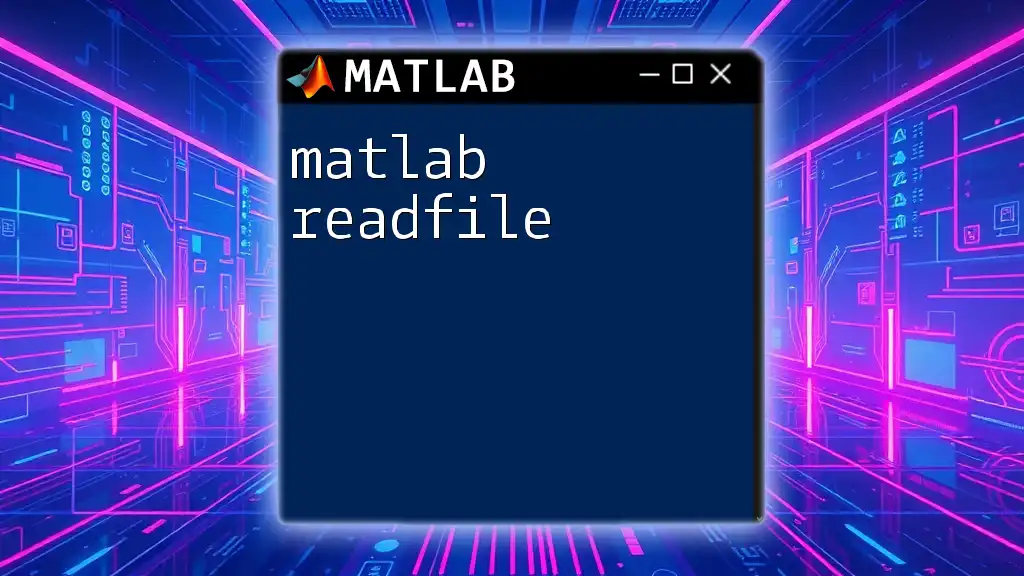
Practical Applications of fullfile
Managing Project Directories
When working on complex projects, organizing your files into well-defined directories can greatly improve your efficiency. Using matlab fullfile, you can easily manage diverse folder structures.
Consider the prevalent project structure:
- `project_name/scripts`
- `project_name/data`
- `project_name/results`
Example: Using fullfile in Real Projects
In a practical scenario, you might need to reference data and output files within your project. Here’s how fullfile simplifies that:
scriptFolder = 'project_name/scripts';
dataFolder = 'project_name/data';
resultFolder = 'project_name/results';
dataFile = fullfile(dataFolder, 'input_data.csv');
resultFile = fullfile(resultFolder, 'output_results.mat');
In this snippet, fullfile allows you to easily construct paths for your data file and results file. This not only enhances code readability but also minimizes the potential for errors arising from incorrect path formatting.
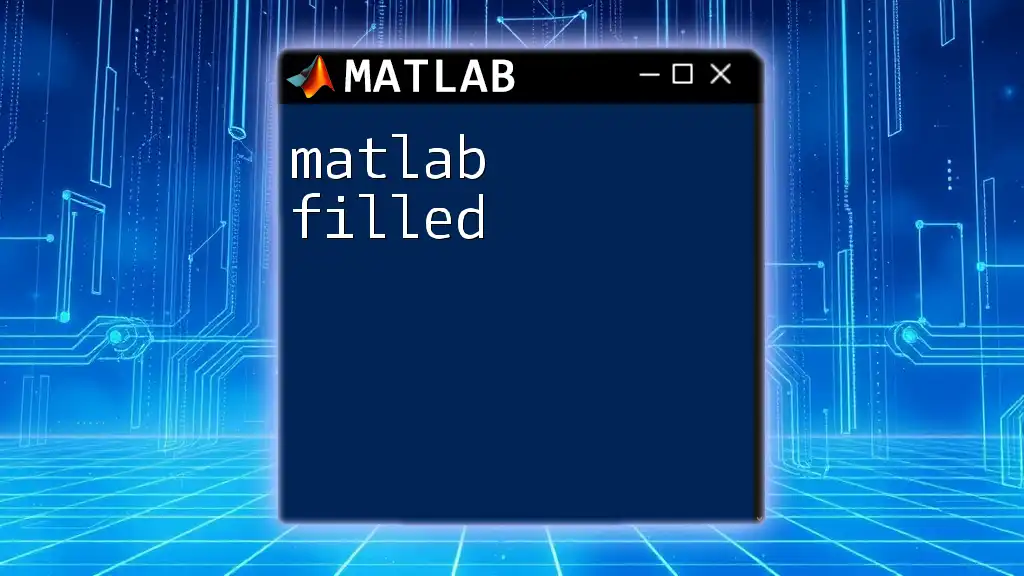
Advantages of Using fullfile
Utilizing matlab fullfile provides several advantages:
-
Cross-Platform Compatibility: By employing fullfile, your paths will automatically adjust to the user's operating system. This ensures that your scripts remain functional regardless of the environment.
-
Reducing Hardcoding of File Paths: As demonstrated, you can construct paths dynamically, which mitigates the risk of hardcoded paths leading to broken code.
-
Enhancing Code Readability: Your code will be cleaner and more maintainable, making it easier for you and others to understand the structure of file paths at a glance.
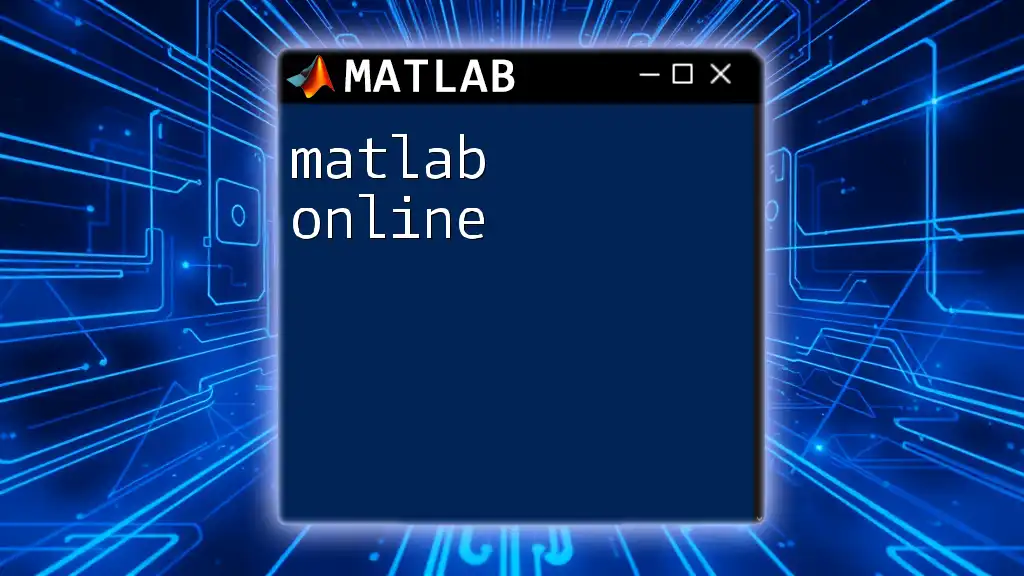
Common Mistakes with fullfile
Avoiding Common Pitfalls
While using matlab fullfile can be straightforward, several mistakes can still occur:
-
Leaving Out a Folder in the Path: Always double-check your directory structures to ensure you are including all necessary folders when constructing paths.
-
Hardcoding File Separators: Avoid manually inserting file separators in your strings; instead, rely on fullfile to handle these automatically.
Troubleshooting Tips
If you face path-related errors, debugging is essential. Utilize the `exist` function to verify file availability before attempting operations:
if exist(dataFile, 'file') ~= 2
disp('Data file not found!');
end
This snippet checks for the existence of `dataFile` before proceeding, providing a safeguard against runtime errors.
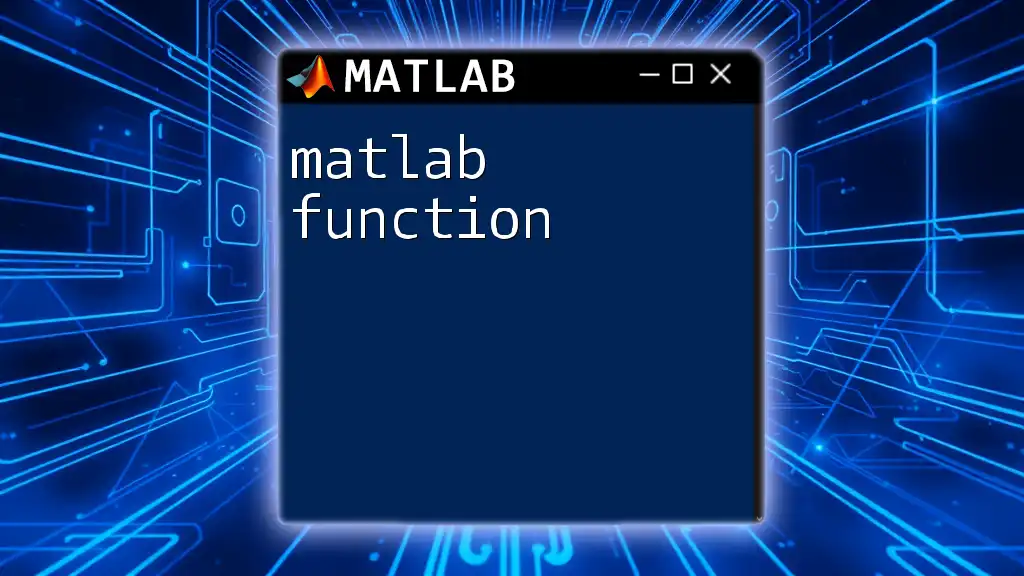
Best Practices for Using fullfile
To fully leverage the potential of matlab fullfile, consider the following best practices:
-
Combining with Other MATLAB Functions: Pair fullfile with functions like `dir` or `load` to streamline data retrieval processes.
-
Using fullfile in Scripts Versus Functions: Maintain consistency in how you use paths. In functions, consider passing paths as parameters to enhance versatility.
-
Keeping Directory Structures Organized: Ensure your project directory is clean and well-documented, making it easier to locate files using matlab fullfile.
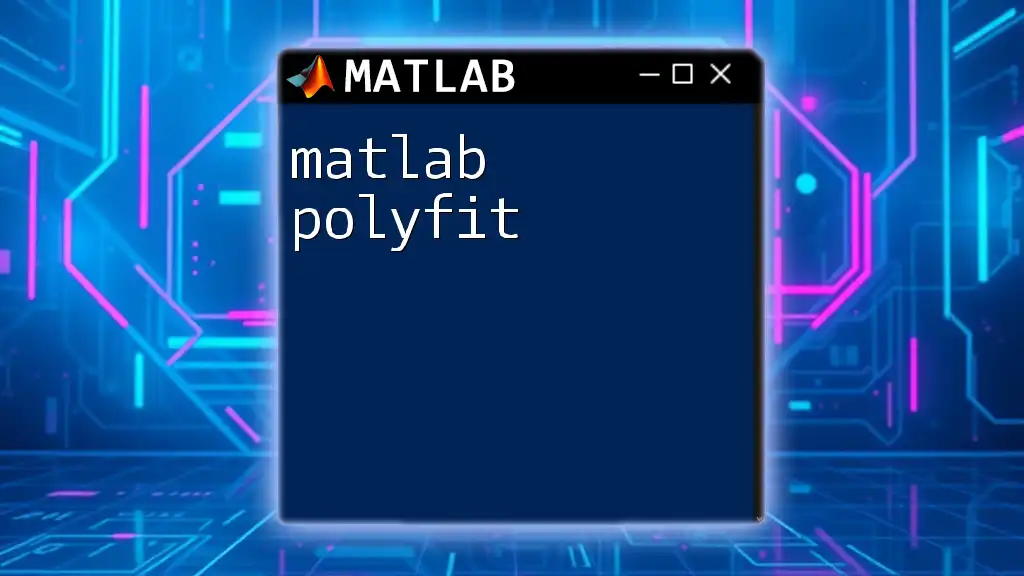
Alternative Methods for Path Management
While matlab fullfile is highly recommended, you may encounter alternative methods such as:
-
`strcat`: Useful for simple concatenation but lacks automatic file separator handling.
-
`filesep`: This function returns the appropriate file separator for the operating system, which can be used in conjunction with other methods.
Despite these alternatives, matlab fullfile is often preferred due to its simplicity and efficiency in managing paths without worries about manual formatting.
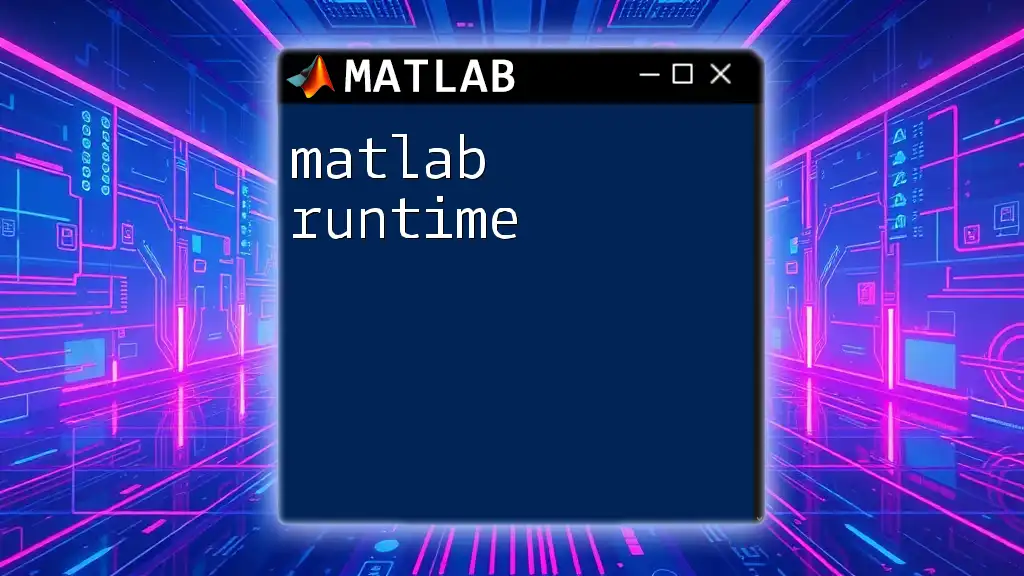
Conclusion
In conclusion, mastering the matlab fullfile function will significantly enhance your ability to manage file paths effectively. Whether you are working on small scripts or large projects, utilizing fullfile will save you time, reduce errors, and create a cleaner coding environment. Begin incorporating fullfile into your MATLAB practice today to experience its benefits firsthand.
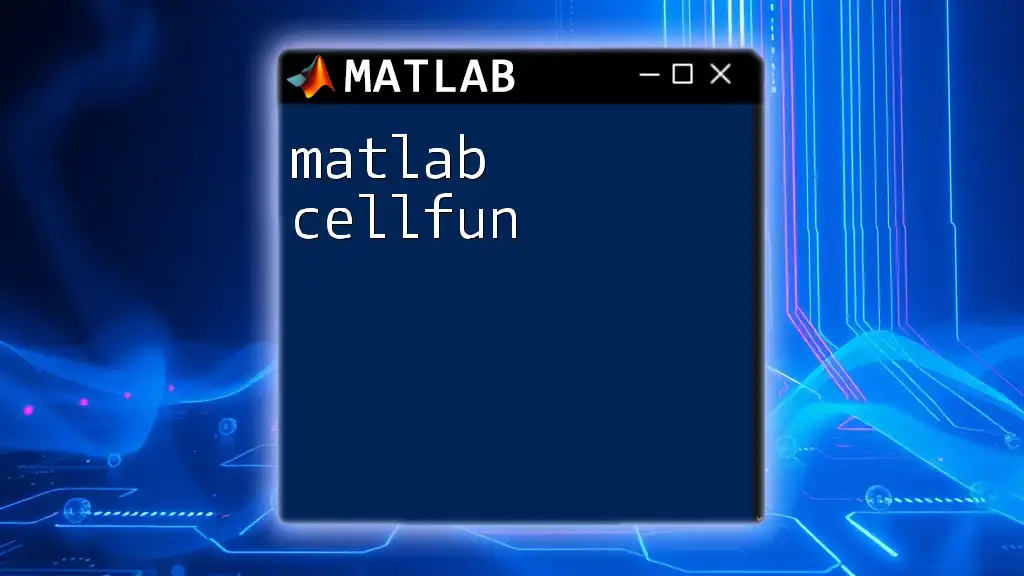
Additional Resources
For further exploration, consider consulting the official [MATLAB Documentation](https://www.mathworks.com/help/matlab/ref/fullfile.html). Joining online MATLAB communities can also provide valuable insights and problem-solving strategies to enrich your coding experience. Keep learning and refining your MATLAB skills!