The `movmean` function in MATLAB calculates the moving average of a specified input array over a defined number of consecutive elements, making it useful for smoothing data series.
Here’s a concise example:
% Calculate the moving average of the array 'data' using a window size of 3
data = [1, 2, 3, 4, 5, 6];
movingAvg = movmean(data, 3);
Understanding Moving Averages
What is a Moving Average?
A moving average is a statistical calculation that analyzes data points by creating averages of different subsets of the complete dataset. Employing moving averages helps smooth out fluctuations in your data, making it easier to identify trends over a specified period.
Types of Moving Averages
- Simple Moving Average (SMA): The simplest form, calculated by averaging a fixed number of past data points.
- Weighted Moving Average (WMA): This method assigns different weights to data points, giving more significance to recent values.
- Exponential Moving Average (EMA): Similar to WMA, but with weights declining exponentially from the latest data point.
In MATLAB, the `movmean` function primarily calculates the simple moving average. The advantage of using this function lies in its simplicity and efficiency, allowing users to focus on data without extensive coding.
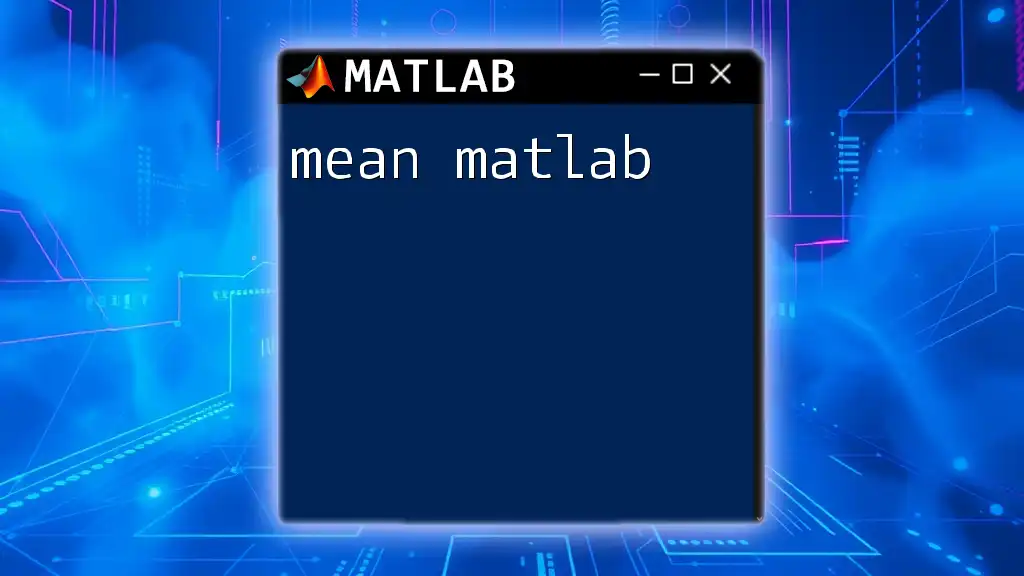
How to Use `movmean` in MATLAB
Basic Syntax of `movmean`
To implement the `movmean` function, you can use the following syntax:
Y = movmean(X, window)
- X: This parameter represents your input data, which can be a vector or matrix.
- window: Specifies the number of elements you want to include in the moving average. This is a critical choice that can significantly affect your results.
Examples of Basic Usage
Example 1: Basic Moving Average
Let’s take a look at how you can apply `movmean` with a simple dataset.
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
mov_avg = movmean(data, 3);
disp(mov_avg);
In this example, we calculate the moving average with a window of 3. The result shows that for each point in the output, it averages over the three preceding points.
Specifying Window Sizes
The ability to customize the window size is one of the key features of `movmean`.
Example 2: Larger Window Size
Let's see what happens when you increase the window size.
mov_avg_large = movmean(data, 5);
disp(mov_avg_large);
By changing the window to 5, you will find that the moving average effectively smooths the data even further, providing a broader view of the trend over time.
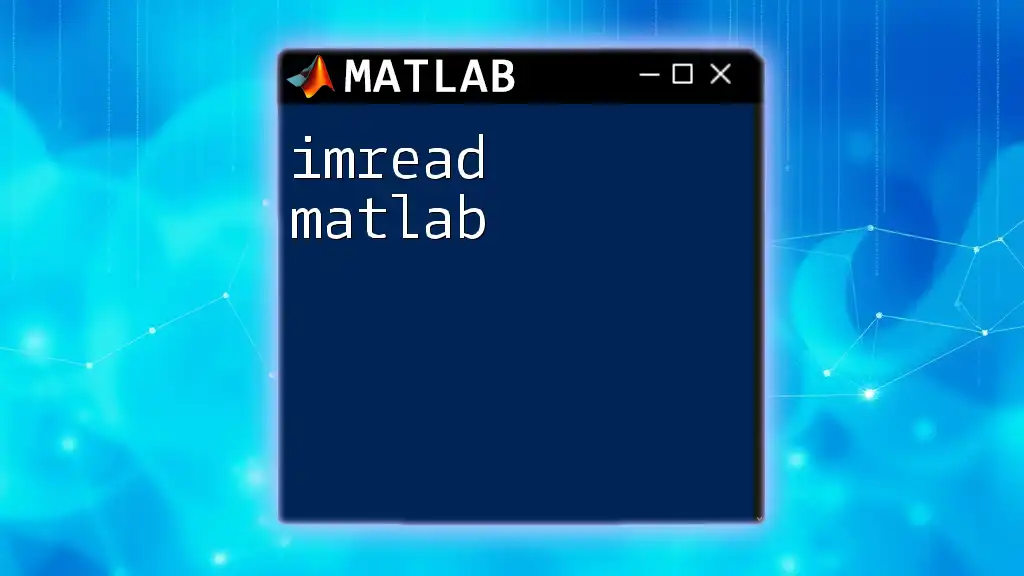
Advanced Features of `movmean`
Handling NaN Values
MATLAB’s `movmean` is equipped to handle NaN values seamlessly. If you're working with datasets that contain NaNs, you can still perform calculations without interruptions.
Example with NaN
Consider the following dataset that includes NaN values:
data_with_nan = [1, 2, NaN, 4, 5, NaN];
mov_avg_nan = movmean(data_with_nan, 3);
disp(mov_avg_nan);
Here, `movmean` will ignore the NaNs when computing the average, allowing for accurate results without manual data cleaning.
Moving Averages with Custom Weights
If you need to give different importance to values in your moving average, `movmean` can also accept custom weights.
Example: Custom Weighted Moving Average
Using custom weights allows you to apply varying significance to your data points.
weights = [0.1, 0.3, 0.6];
custom_mov_avg = movmean(data, [0 2], 'omitnan', 'Weights', weights);
disp(custom_mov_avg);
In this case, a user-defined weight vector effectively shapes how the average is computed, providing a flexible analytical tool.
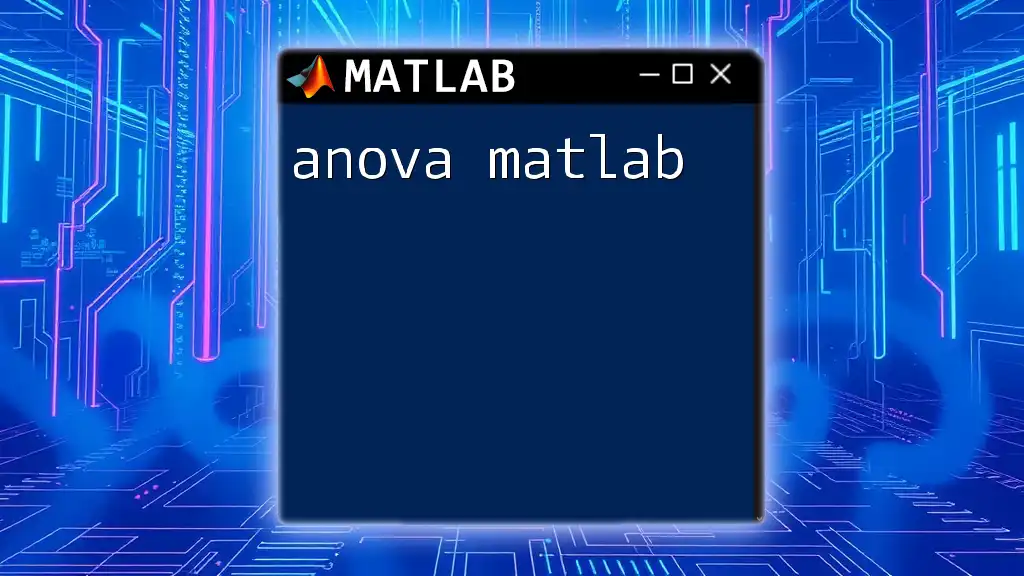
Practical Applications of `movmean`
Signal Processing
In fields like signal processing, applying moving averages can help eliminate noise and highlight significant trends. For instance, in audio signals, moving averages may smooth abrupt changes, making it easier to analyze characteristics.
Financial Market Analysis
Another prime application is within financial analysis, where moving averages are utilized for various trading strategies. Investors often apply a moving average to smooth price data, identify trends, or generate trading signals based on crossovers.
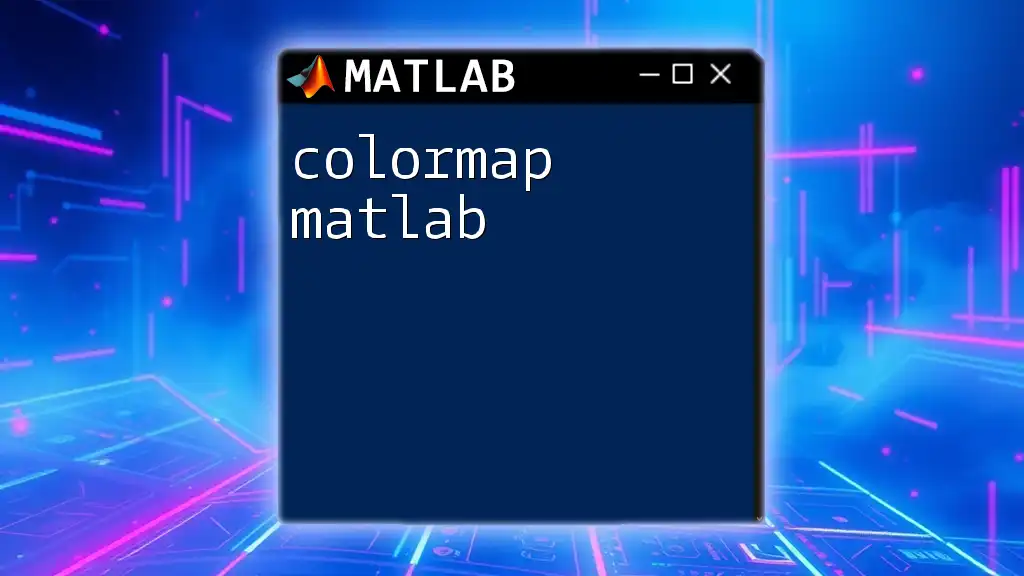
Best Practices for Using `movmean`
- Choosing the Right Window Size: The effectiveness of your moving average is intrinsically tied to the window size. Larger windows provide more smoothing but may mask important changes in the data.
- Avoiding Over-Smoothing: While it can be tempting to use a large window, be conscious of losing significant signals, which can lead to incorrect conclusions.
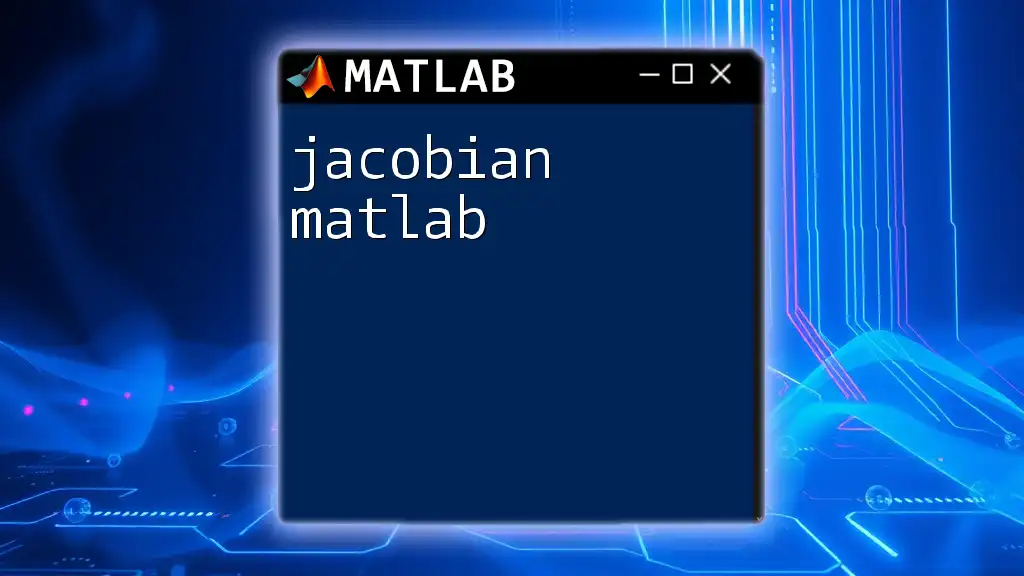
Common Pitfalls and Troubleshooting
Issues with Time Series Data
Ensure you manipulate time-based datasets correctly. For example, if you're applying `movmean` to daily sales data, be mindful of holidays or other outliers that may skew the average.
Performance Considerations
When working with substantial datasets, performance might become a concern. If you experience slow performance, consider optimizing your code or utilizing more efficient data structures to hold your datasets.
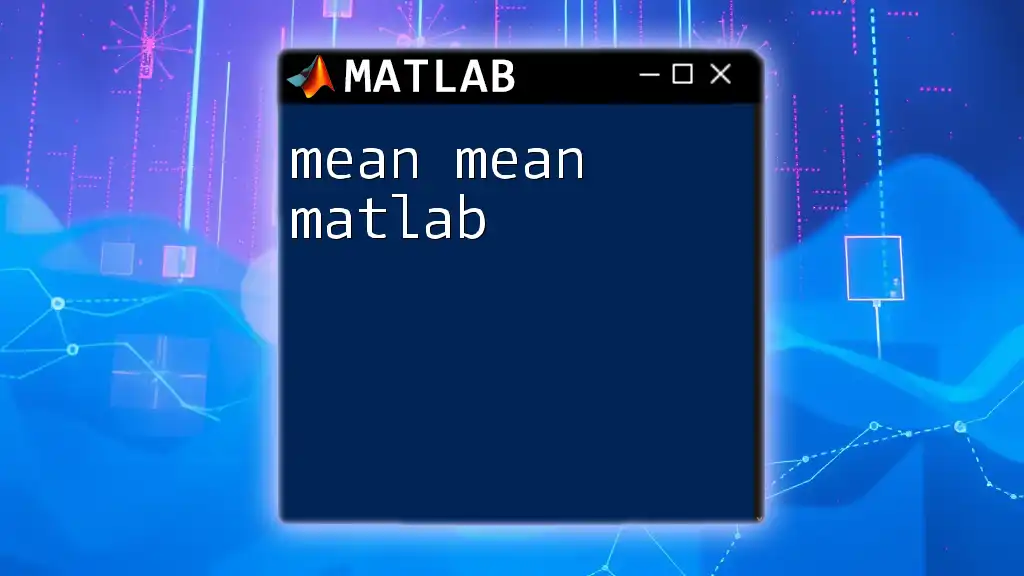
Conclusion
Using the `movmean` function in MATLAB offers a robust method for analyzing data. With its simple syntax and powerful features, incorporating moving averages into your analysis can lead to clearer insights and better decision-making. Whether you are smoothing signals or evaluating financial trends, mastering `movmean` equips you with essential tools for effective data analysis.