The `polyval` function in MATLAB evaluates a polynomial for a given set of values, using the coefficients of the polynomial defined in a vector format.
Here’s a code snippet demonstrating its usage:
% Define the coefficients of the polynomial (highest power first)
coefficients = [2, -3, 5]; % Represents 2x^2 - 3x + 5
% Define the values at which to evaluate the polynomial
x_values = [0, 1, 2, 3];
% Evaluate the polynomial at the given x_values
y_values = polyval(coefficients, x_values);
% Display the results
disp(y_values);
Understanding Polynomials
What is a Polynomial?
A polynomial is a mathematical expression that consists of variables (also known as indeterminates) raised to whole number powers, along with coefficients. The general form of a polynomial is represented as:
\[ P(x) = a_n x^n + a_{n-1} x^{n-1} + \ldots + a_1 x + a_0 \]
Here, \(a_n\) are the coefficients, and \(n\) is the degree of the polynomial.
Characteristics of Polynomials
Polynomials have several key characteristics that are important in both theoretical and applied mathematics.
- Degree of a Polynomial: This refers to the highest power of the variable in the polynomial. For instance, in the polynomial \(3x^4 + 2x^3 - x + 7\), the degree is 4.
- Roots of a Polynomial: The values of \(x\) for which \(P(x) = 0\) are called the roots of the polynomial. Finding these roots is fundamental in various applications, from solving equations to analyzing system behaviors.
Polynomials play a critical role in data fitting, interpolation, and modeling real-world phenomena, making their evaluation a common requirement.
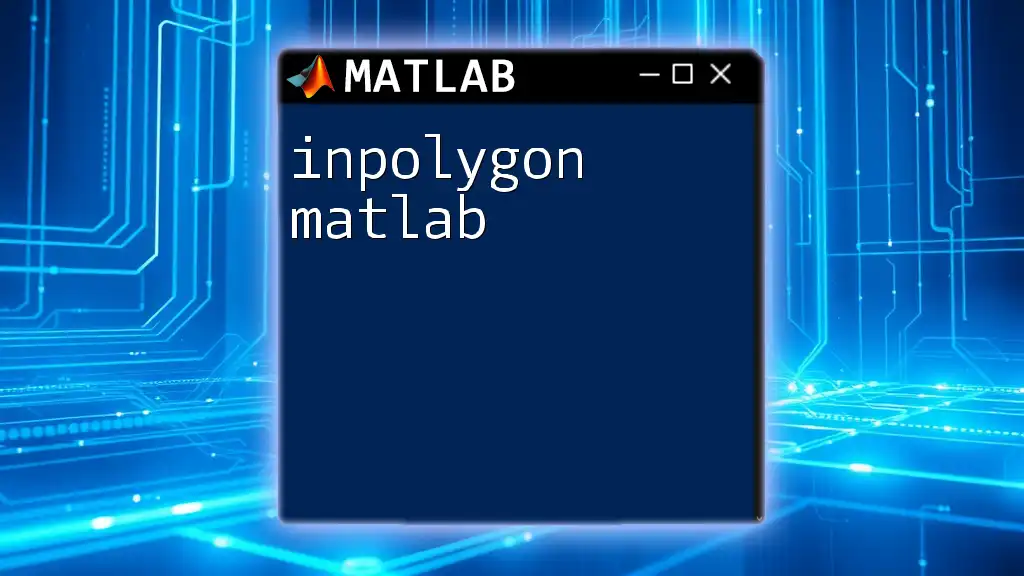
Overview of the `polyval` Function
What is `polyval`?
The `polyval` function in MATLAB is a powerful tool used to evaluate polynomials at specific points. This function takes in a vector of coefficients and an input value (or values) and computes the corresponding polynomial values.
The general syntax is:
y = polyval(p, x)
Here, `p` is the coefficients vector, and `x` is the point (or points) at which you want to evaluate the polynomial.
Parameters of `polyval`
Coefficients Vector
The coefficients vector, `p`, is an array where each entry corresponds to a coefficient of the polynomial, starting from the highest degree down to the constant term. For example, if you have the polynomial \( P(x) = 2x^2 + 3x + 4 \), the coefficients vector is represented as:
p = [2, 3, 4];
Input Values
The input variable `x` can be a scalar, a vector, or even a matrix. This flexibility allows you to evaluate the polynomial at multiple points simultaneously, which is particularly useful in analysis and visualization.
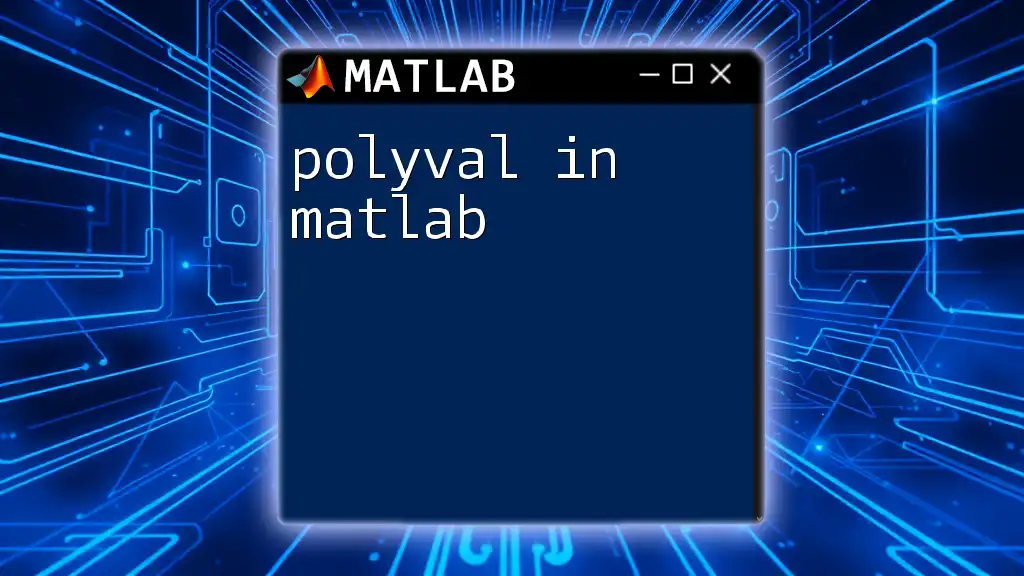
How to Use `polyval` in MATLAB
Basic Usage
To begin using `polyval`, you might start with a straightforward example. Let's evaluate the polynomial \( P(x) = 2x^2 + 3x + 4 \) at a single point.
p = [2, 3, 4]; % coefficients for 2x^2 + 3x + 4
x = 5; % point of evaluation
y = polyval(p, x); % evaluating the polynomial
disp(y) % Outputs: 69
This code evaluates the polynomial at \(x = 5\), resulting in \(y = 69\).
Evaluating Polynomials at Multiple Points
Using Vectors
`polyval` allows you to evaluate a polynomial at multiple input points by providing a vector. For example:
x = [0, 1, 2, 3]; % points of evaluation
y = polyval(p, x); % evaluating the polynomial at these points
disp(y) % Outputs: [4, 9, 16, 25]
Here, the polynomial \( P(x) \) is evaluated at four different points, returning the corresponding output values.
Using Matrices
You can also evaluate polynomials on matrices. Each entry in the matrix is treated individually. For instance, consider this example:
X = [0, 1; 2, 3]; % matrix of points
Y = polyval(p, X); % evaluating polynomial on a matrix
disp(Y)
This would yield results by evaluating the polynomial at each element in `X`.
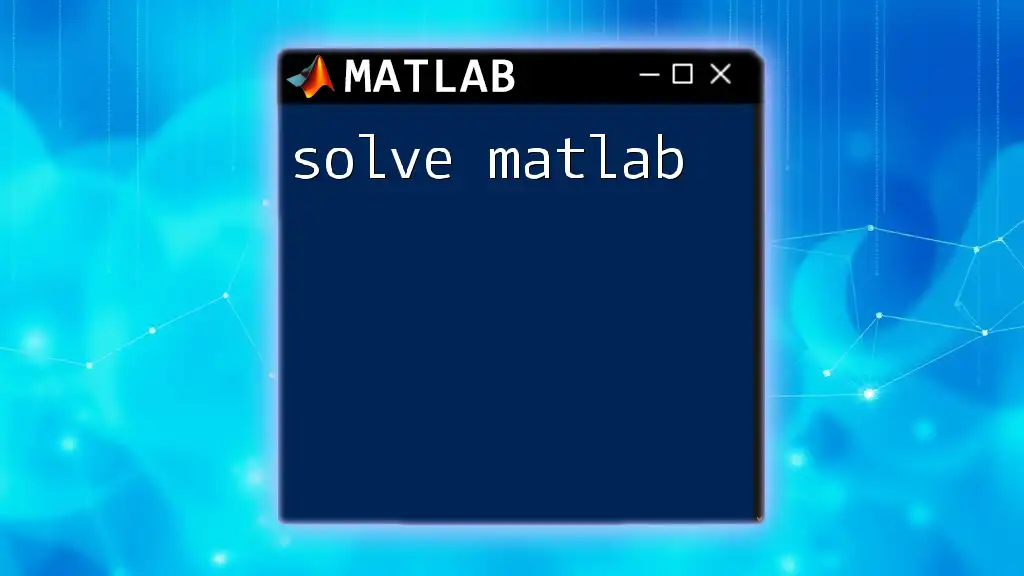
Advanced Features and Techniques
Working with Complex Coefficients
The `polyval` function can also handle polynomials with complex coefficients, significantly broadening its application range. For example:
p = [1 + 2i, 3 - i, 4]; % complex coefficients for (1 + 2i)x^2 + (3 - i)x + 4
x = 1 + 1i; % complex point of evaluation
y = polyval(p, x);
disp(y)
Evaluating Derivatives using `polyval`
Sometimes, you may need to evaluate the derivative of a polynomial at certain points. First, differentiate the polynomial:
The first derivative of \( P(x) = 2x^2 + 3x + 4 \) is \( P'(x) = 4x + 3 \). The coefficients vector for the derivative would be:
p_derivative = [4, 3];
You can now use `polyval` to find the value of the derivative at a specific point:
x = 2;
y_derivative = polyval(p_derivative, x); % evaluating the derivative at x=2
disp(y_derivative) % Outputs: 11
Application in Curve Fitting
`polyval` is often used in conjunction with `polyfit`, which fits a polynomial to data points. This is useful in various fields such as statistics and engineering. For example:
xData = [1, 2, 3, 4];
yData = [2.2, 2.8, 3.6, 4.5];
p = polyfit(xData, yData, 1); % Fit a linear polynomial
xFit = linspace(1, 4); % Generate points for the fit line
yFit = polyval(p, xFit);
plot(xData, yData, 'o', xFit, yFit, '-'); % Plot the original data and the fit
This code fits a linear polynomial to the data and evaluates it across a range of values for visualization.
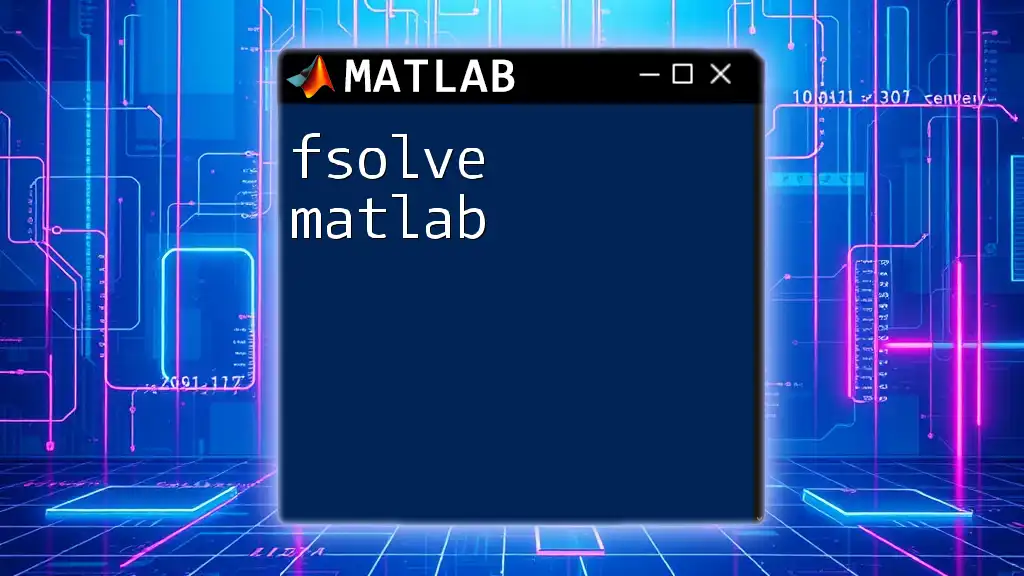
Practical Examples and Use Cases
Example 1: Engineering Applications
In engineering, the evaluation of polynomials can help analyze systems. For instance, in structural analysis, polynomial equations can describe load-deflection relationships or stress-strain curves. Using `polyval`, engineers can quickly calculate responses at various load conditions.
Example 2: Data Analysis
In data analysis, evaluating polynomials provides insights into trends. Analysts often use polynomial regression to model relationships between variables and `polyval` to explore predicted outcomes across various scenarios.
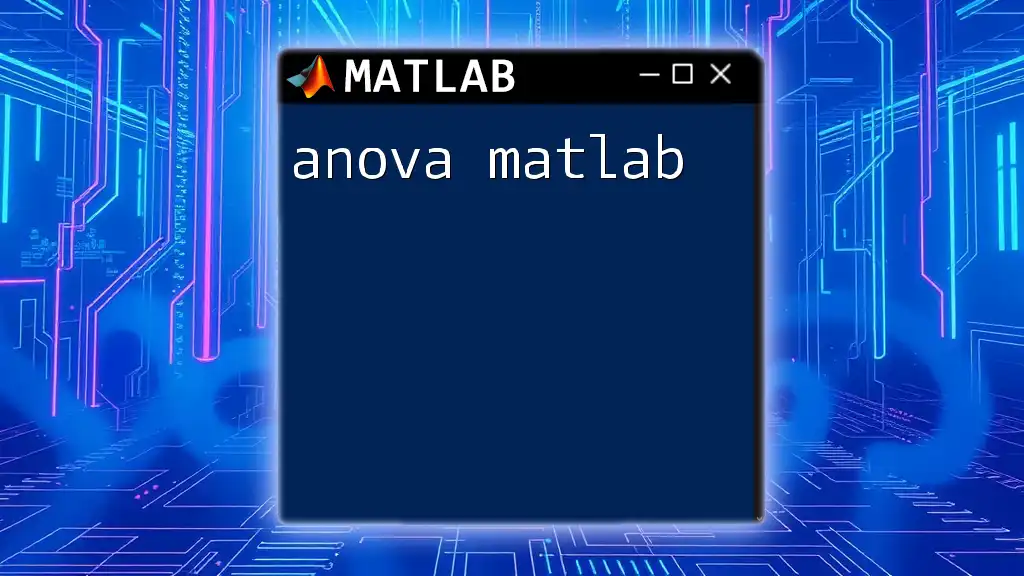
Common Errors and Troubleshooting
Frequent Mistakes
Users often encounter common pitfalls when using `polyval`. One frequent error is misconfiguring the coefficients vector. Ensure your coefficients are provided in descending order of degrees.
How to Debug
If you receive unexpected outputs, inspect your inputs first. Verify your coefficients and the shape of your input values. Using `disp` can help print intermediate results, allowing for easier debugging.
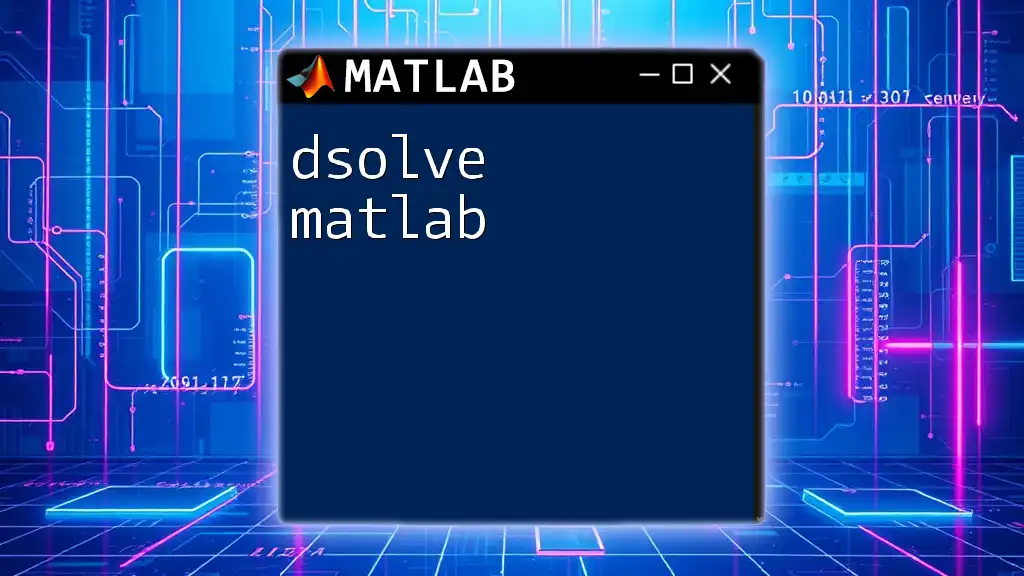
Conclusion
The `polyval` function in MATLAB is an indispensable tool for anyone working with polynomials. Whether in engineering, data analysis, or theoretical mathematics, understanding how to evaluate polynomials using `polyval` enhances your capability to tackle complex problems effectively.
Additional Resources
For further learning, refer to the official MATLAB documentation and consider exploring online tutorials dedicated to polynomial functions and their applications. Engaging with MATLAB's community through forums or workshops can also deepen your understanding.
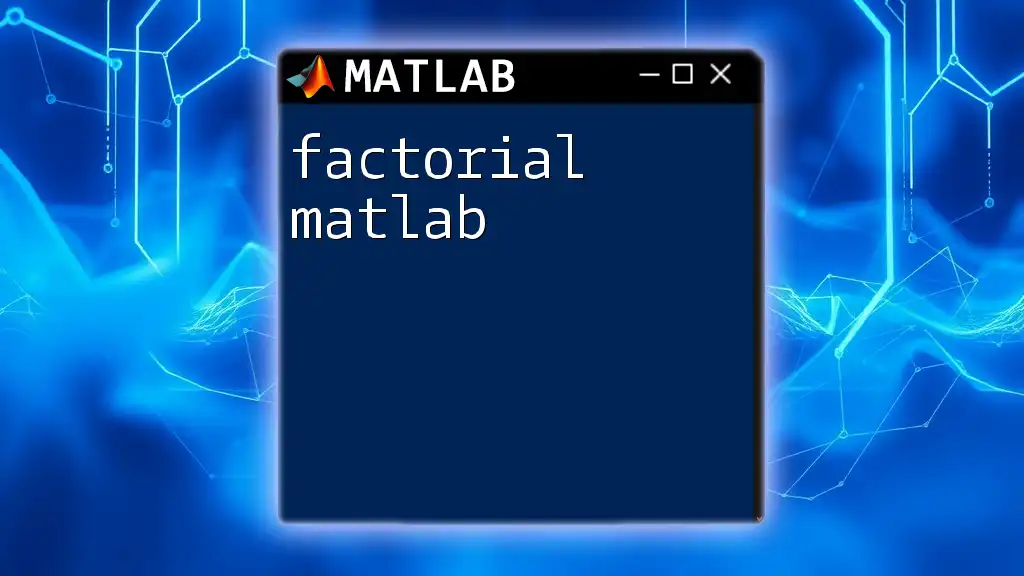
Call to Action
As you continue your exploration of MATLAB, consider sharing your experiences with the `polyval` function in the comments below. We encourage you to join our workshops or online discussions to connect with fellow learners and enhance your MATLAB skills!