The `mesh` function in MATLAB is used to create 3D surface plots, allowing for the visualization of matrix data on a three-dimensional grid.
Here's a simple example code snippet demonstrating how to use the `mesh` command:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
mesh(X, Y, Z);
Understanding Mesh in MATLAB
What is a Mesh?
A mesh in the context of MATLAB refers to a network of points or elements that define a geometric shape, data set, or a domain in numerical methods and graphical representations. In numerical computing, meshes serve crucial functions, especially in finite element analysis (FEA) and computational fluid dynamics (CFD). They provide the framework for discretizing continuous spaces into manageable sections, allowing for detailed calculations and simulations.
Benefits of Using Mesh in MATLAB
Utilizing meshes in MATLAB offers a range of advantages:
- Enhanced Data Visualization: Meshes allow for the graphical representation of multi-dimensional data, facilitating better understanding and interpretation of complex datasets.
- Improved Computational Accuracy: A well-defined mesh can significantly improve the precision of numerical simulations, yielding more reliable results.
- Versatility in Applications: Meshes find applications across diverse fields, including engineering, physics, biology, and more, proving their adaptability and usefulness.
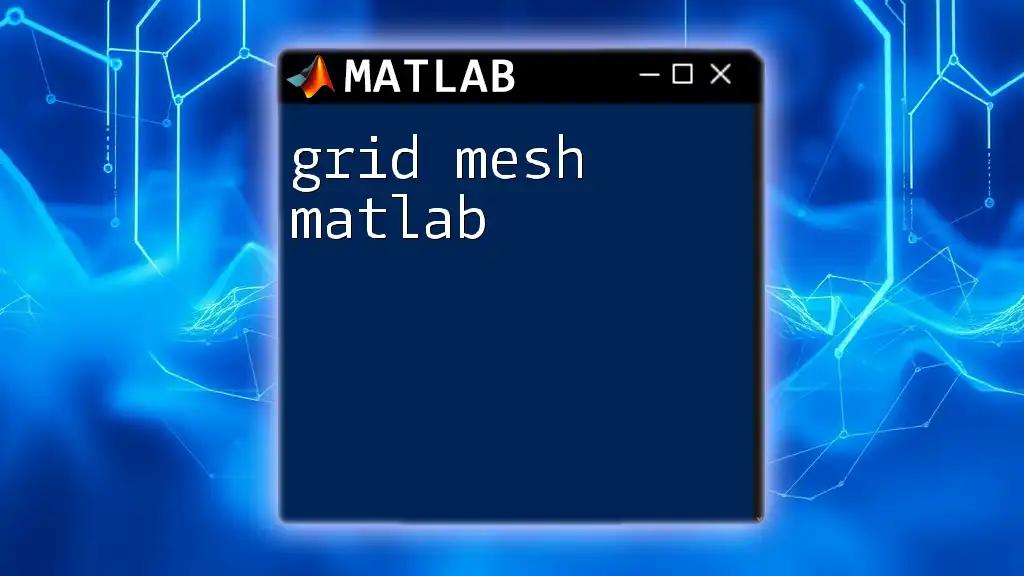
Key Concepts Related to Mesh
Grids and Meshes
It’s essential to distinguish between a grid and a mesh. A grid typically refers to a structured layout of points that are evenly spaced, while a mesh is a more general term that encompasses various structures, including irregular layouts. In MATLAB, grids help define simple domains where calculations and visualizations can be performed easily.
Types of Meshes
Meshes can be categorized into two primary types:
- Structured Meshes: These consist of regularly spaced nodes and are easier to work with, particularly for simple geometries.
- Unstructured Meshes: These allow for more flexibility and are beneficial for complex geometrical domains, often used in advanced simulations.
Furthermore, understanding the importance of choosing between triangular and rectangular meshes is vital, as this can greatly affect the accuracy and computational efficiency of simulations.
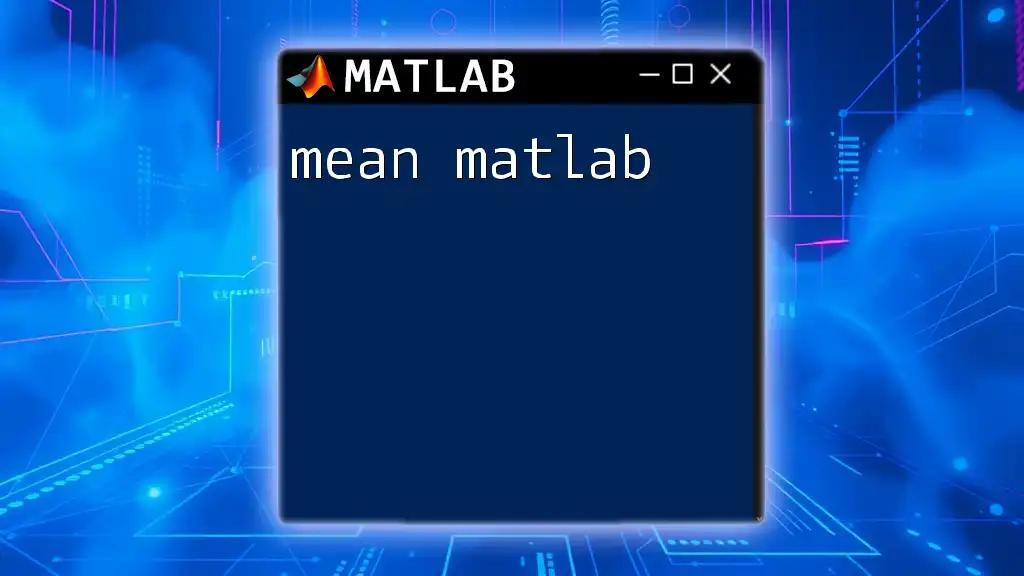
Getting Started with Mesh Commands in MATLAB
Essential Mesh Functions
meshgrid
The function `meshgrid` is essential for creating a 2D grid from two vectors, which is often the first step in visualizations and calculations involving mesh.
Example Code Snippet:
[X, Y] = meshgrid(-5:1:5, -5:1:5);
In this example, `meshgrid` generates matrices `X` and `Y` that span across the specified ranges. These matrices can be used in conjunction with other functions to define a surface.
mesh
The `mesh` function is used to create 3D surface plots. It takes three arguments — X, Y, and Z coordinates — representing the vertex positions in space.
Example Code Snippet:
Z = X.^2 + Y.^2;
mesh(X, Y, Z);
This code will plot a 3D surface that represents a paraboloid. The `mesh` command visualizes the values of Z over the grid defined by X and Y, resulting in a dynamic plot that aids in understanding surface relationships.
Visualizing Mesh Data
3D Mesh Plots
Creating and customizing 3D mesh plots is straightforward in MATLAB, allowing users to visualize mathematical functions or data structures effectively.
Example Code Snippet:
mesh(X, Y, Z, 'FaceColor', 'interp');
In this case, the face color of the mesh is interpolated between the vertex colors, enhancing the visual appeal and helping to convey information about slopes and gradients across the surface.
Surface vs. Mesh Plots
While both surface and mesh plots represent 3D data, they serve different purposes. Surface plots (`surf`) display solid surfaces and emphasize color gradients, whereas mesh plots provide a wireframe look that reveals the structure of the surface. When choosing between them, consider the information you want to convey.

Advanced Mesh Techniques in MATLAB
Creating Custom Meshes
Using triangulation
Creating custom triangular meshes can be accomplished using the `triangulation` function, which is particularly useful for finite element methods where complex shapes are involved.
Example Code Snippet:
% Define vertices and connectivity
vertices = [0 0; 1 0; 0 1];
connectivity = [1 2 3];
t = triangulation(connectivity, vertices);
In this snippet, `vertices` are points that define the corners of a triangle, while `connectivity` establishes how these points are connected. Custom meshes enable fine-tuning of simulations and control over mesh quality.
Refining and Coarsening Meshes
Adjusting mesh density is fundamental for achieving the desired computational accuracy. The `refine` function allows you to enhance a mesh, creating more elements in regions requiring greater detail.
Example Code Snippet for refinement:
refined_t = refine(t);
Understanding when to refine or coarsen meshes is paramount; refining is beneficial in regions with high gradients, while coarsening is more efficient in smoother areas to improve computational performance.
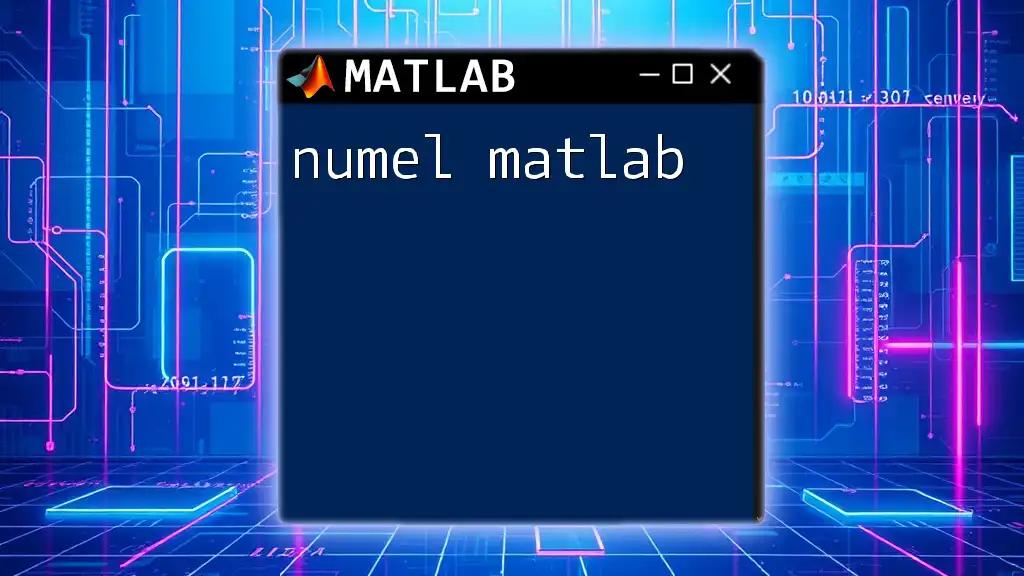
Practical Applications of Mesh in MATLAB
Engineering Simulations
In engineering, meshes serve as the backbone for simulations involving structural analysis (like bridge and building designs) and fluid dynamics (like airflow around objects). The accuracy gained through proper meshing can lead to significant improvements in design safety and functionality.
Scientific Visualization
Researchers often need to visualize complex data sets, and meshes provide the means to simplify these complexities into interpretable graphics. They can effectively display results from computational analyses and experimental data.
Educational Uses
Meshes play a critical role in teaching mathematical concepts such as partial differential equations and geometry. By visually demonstrating these concepts, educators can help students grasp complex theories more intuitively.

Troubleshooting Common Mesh Issues
Common Errors and Their Solutions
Errors in mesh generation often stem from incorrectly defined vertices or misaligned connectivity. It's crucial to validate input data and ensure the mesh's structure is suitable for the desired calculations.
Performance Considerations
When handling large meshes, performance can become an issue. Best practices include simplifying meshes where possible, using efficient algorithms, and leveraging MATLAB’s built-in capabilities for handling large datasets effectively. Utilizing functions like `pdeplot` for visualizing PDE solutions can also enhance performance.
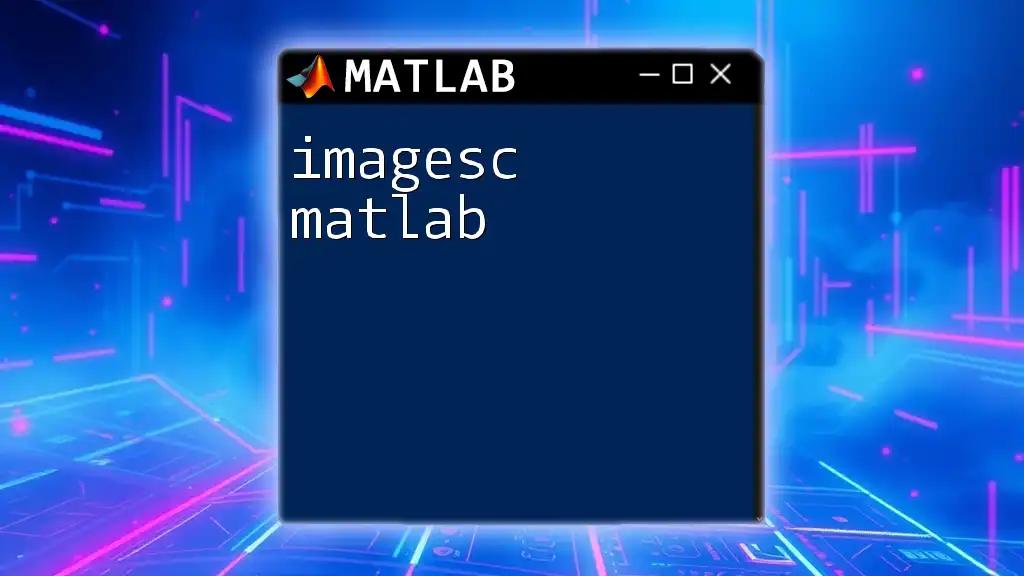
Conclusion
The concept of mesh in MATLAB transcends basic data visualization, extending into critical applications across engineering, education, and scientific research. By mastering the mesh commands and their applications, you unlock a powerful toolset for tackling complex computational problems. Armed with essential skills and knowledge, you can confidently experiment with mesh functionalities to create insightful simulations and visualizations. Engage with the resources available, practice the provided examples, and embark on your journey into the world of mesh MATLAB!
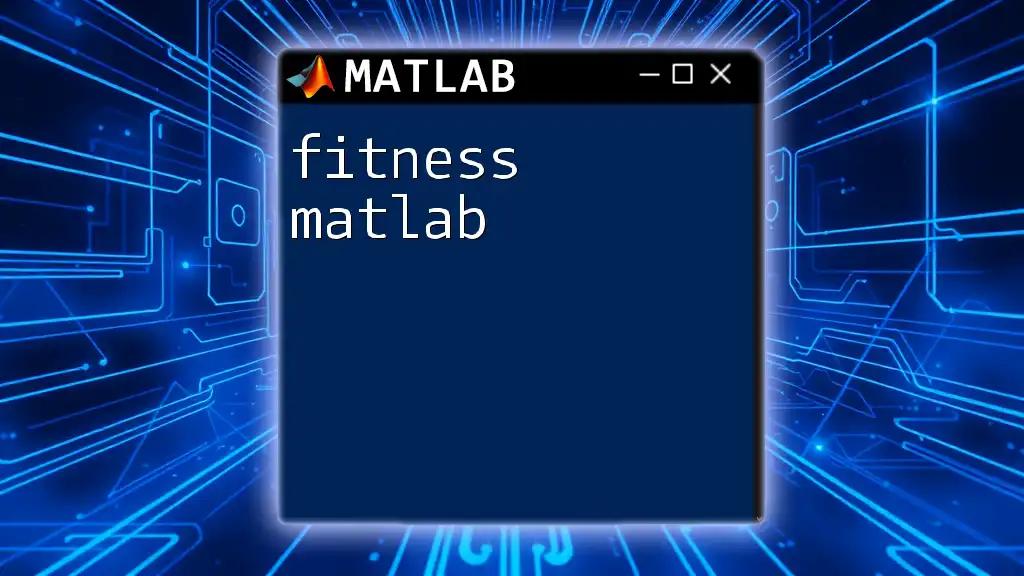
Additional Resources
For further exploration of mesh commands, consider the official MATLAB documentation where you can find extensive details and examples to enhance your learning and practical applications.