Optimizing PID controller performance using MATLAB or LabVIEW involves fine-tuning the proportional, integral, and derivative parameters to achieve desired response characteristics in control systems, often utilizing tools for simulation and analysis to visualize performance.
Here's a code snippet in MATLAB to tune a PID controller:
% Define the plant transfer function
num = [1];
den = [1, 10, 20];
plant = tf(num, den);
% PID controller tuning using PID tuning function
Kp = 1; % Proportional gain
Ki = 1; % Integral gain
Kd = 0.1; % Derivative gain
pidController = pid(Kp, Ki, Kd);
% Closed-loop system
closedLoopSystem = feedback(pidController * plant, 1);
% Step response for performance evaluation
step(closedLoopSystem);
title('Step Response of PID Controlled System');
grid on;
Understanding PID Control
What is a PID Controller?
A PID controller is an essential component used in control systems to maintain the desired output by adjusting the rate of control inputs. Its three fundamental components—Proportional (P), Integral (I), and Derivative (D)—work together to minimize the error between a setpoint and the process variable.
-
Proportional Control: This component produces an output that is proportional to the current error value. The larger the error, the greater the control output.
-
Integral Control: This aspect focuses on the accumulation of past errors. By integrating the error over time, it aims to eliminate residual steady-state error that can be present when using a proportional controller alone.
-
Derivative Control: This part predicts future errors based on the rate of change. It responds to how quickly the error is changing, thereby providing a smoothing effect and improving system stability.
The mathematical representation of a PID controller can be expressed as: \[ C(s) = K_p + \frac{K_i}{s} + K_d \cdot s \] where \( C(s) \) is the controller output, \( K_p \), \( K_i \), and \( K_d \) are the proportional, integral, and derivative gains, respectively.
Performance Metrics of PID Controllers
To evaluate PID controller performance, several key metrics should be considered:
-
Settling Time: The time required for the system output to settle within a certain percentage of the final value.
-
Rise Time: The time taken for the output to go from a defined low value to a high percentage of the final value.
-
Overshoot: The extent to which the output exceeds the desired setpoint.
-
Steady-State Error: The difference between the desired setpoint and the process variable after the system has settled.
These performance indicators are crucial for assessing the quality of your PID control implementation and for making necessary adjustments during the optimization process.
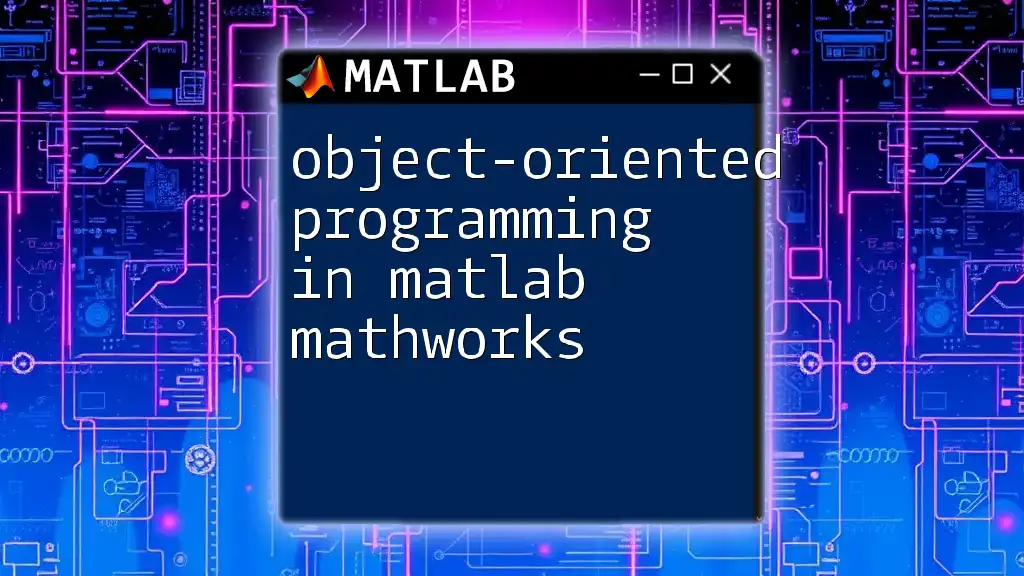
Overview of MATLAB and LabVIEW for PID Control
The Case for MATLAB
MATLAB is a powerful environment for numerical computing and control system design. It provides a rich set of built-in functions and specialized toolboxes that allow users to design and tune PID controllers effortlessly.
-
Built-in Functions: MATLAB has functions like `pid()`, `step()`, and control system design apps such as the Control System Toolbox that enable easy manipulation, simulation, and visualization of PID controllers.
-
Simulink Interface: Simulink offers a visual way to model dynamic systems and simulate PID control in a straightforward manner, providing an intuitive workspace for users.
The Case for LabVIEW
On the other hand, LabVIEW is a visually-oriented programming environment commonly used for data acquisition and control.
-
Visual Programming Advantages: LabVIEW employs a graphical programming language where users can build programs by connecting functional blocks. This approach can make complex control systems easier to understand.
-
Integration with Hardware: LabVIEW’s ability to interface seamlessly with various hardware devices is a significant advantage, particularly for real-time control applications.
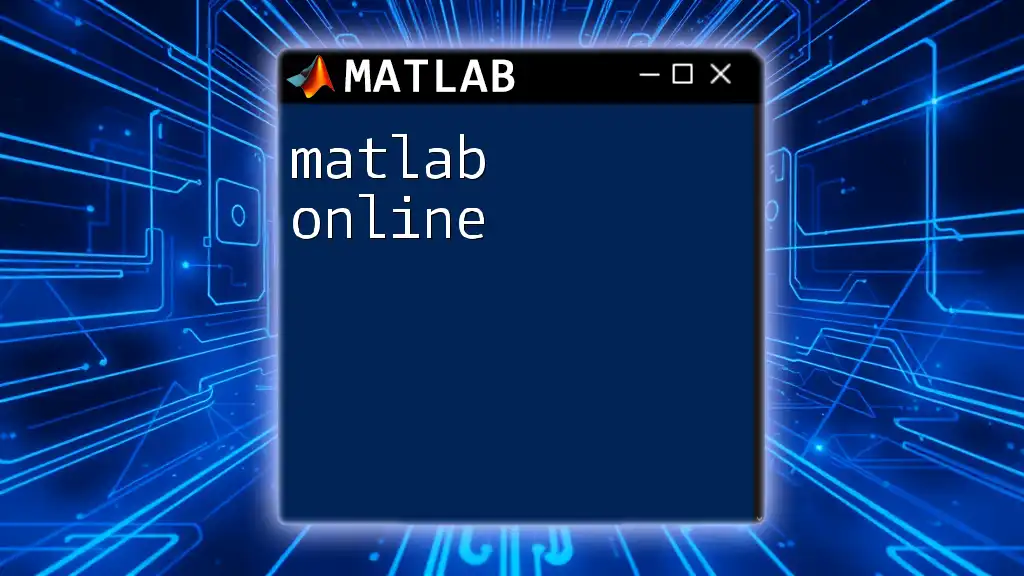
Setting Up Your PID Controller in MATLAB
Creating a Basic PID Controller
To define a PID controller in MATLAB, you can utilize the `pid()` function. The following snippet illustrates how to create a PID controller with specified gains:
Kp = 1; Ki = 1; Kd = 1; % PID parameters
C = pid(Kp, Ki, Kd); % Create PID controller
This command invites you to explore how each gain influences the control response, enabling you to experiment with values.
Tuning the PID Controller
Tuning is a critical aspect of PID control. There are several methods to achieve optimal tuning:
-
Manual Tuning: This method involves adjusting the gains based on empirical observation and results. While this approach can lead to effective results, it may require significant trial and error.
-
Ziegler-Nichols Method: This well-known tuning method provides a systematic approach to determine the PID parameters based on the system's response to a step input. It is often viewed as a benchmark for tuning methodologies.
Simulating Control Systems
Once you have set up your PID controller, simulation forms an integral part of performance assessment. You can simulate the system using MATLAB’s control functions:
G = tf(1, [1, 5, 6]); % Transfer function of the system
sys = feedback(C*G, 1); % Close-loop system
step(sys); % Step response
The `step()` function allows you to visualize the system's response to a step input, providing insightful feedback on performance metrics such as overshoot and settling time.
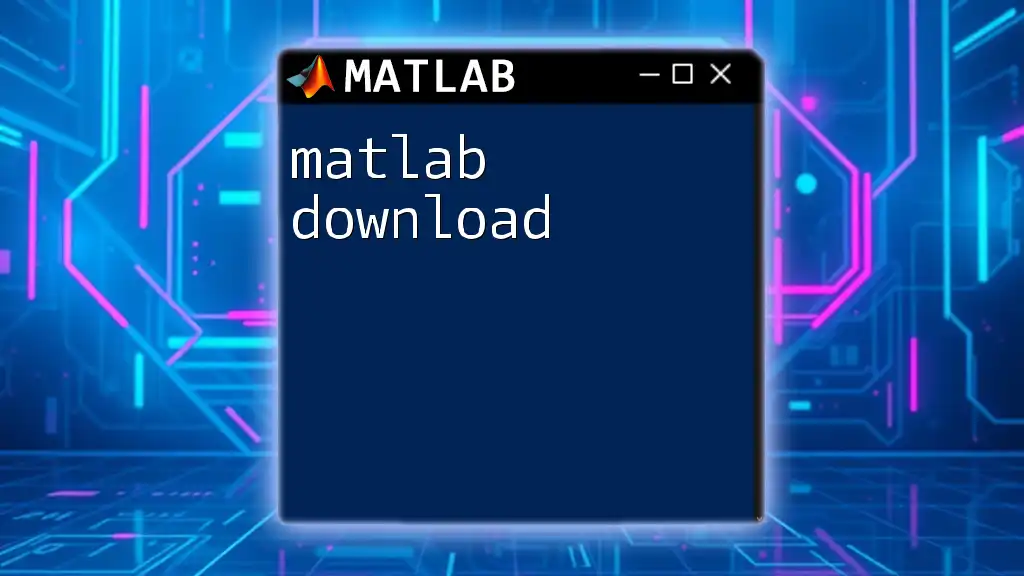
Implementing PID Control in LabVIEW
Setting Up LabVIEW for PID Control
Creating a basic PID controller in LabVIEW involves inserting PID control blocks into a block diagram. The block configuration is intuitive, allowing users to modify parameters right within the interface.
Tuning the PID in LabVIEW
LabVIEW has a specialized PID Tuner that simplifies the tuning process:
-
Using LabVIEW PID Tuner: This interactive tool allows you to adjust PID parameters in real-time while monitoring system performance. It uses algorithms that automatically tune the response, helping you achieve both stability and responsiveness with ease.
-
Visual Feedback Mechanism: LabVIEW provides real-time visual feedback through graphs and indicators, facilitating an interactive learning process that enhances understanding and control over PID dynamics.
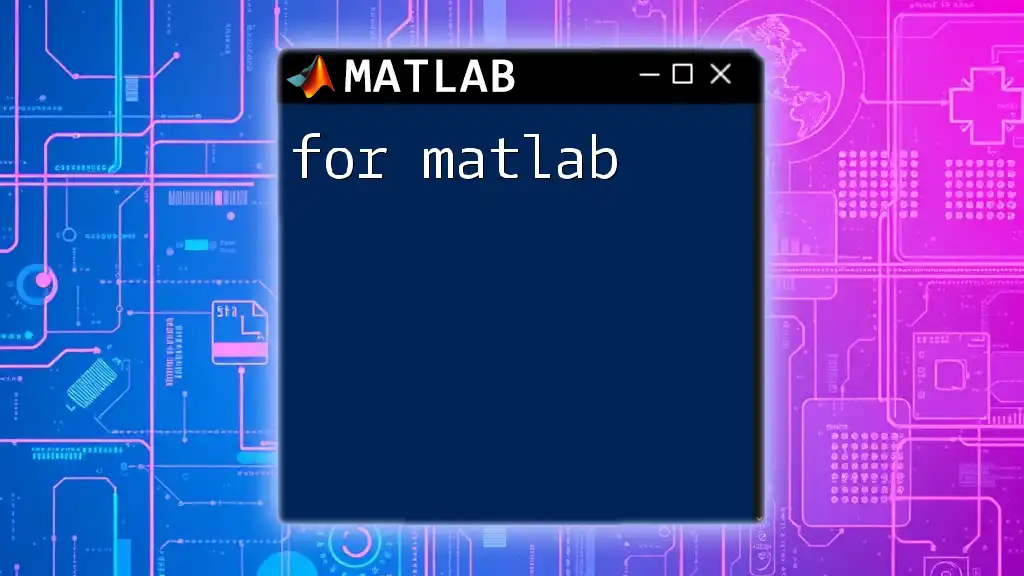
Advanced PID Controller Optimization Techniques
Auto-Tuning Solutions
Auto-tuning solutions greatly simplify the PID tuning process:
-
Benefits of Auto-Tuning: With automatic tuning, you minimize human error and can achieve optimal performance under varying conditions precisely and efficiently.
-
Examples of Auto-Tuning: Both MATLAB and LabVIEW offer tools for auto-tuning that use heuristic methods to adjust PID parameters based on system behaviors without complex manual calculations.
Adaptive PID Control
Adaptive control varies PID parameters in response to changing conditions:
-
Concept of Adaptive Control: This approach is beneficial in systems where dynamics change over time. Adaptive PID control adjusts gains based on real-time feedback.
-
Code Example: Adaptive Tuning in MATLAB
% Example code for adaptive PID tuning % Base PID structure with varying coefficients based on error feedback Kp = 1; Ki = 0.5; Kd = 0.1; % Real-time gain modification based on the system's error if error > threshold Kp = Kp * 1.2; % Increase proportional gain end
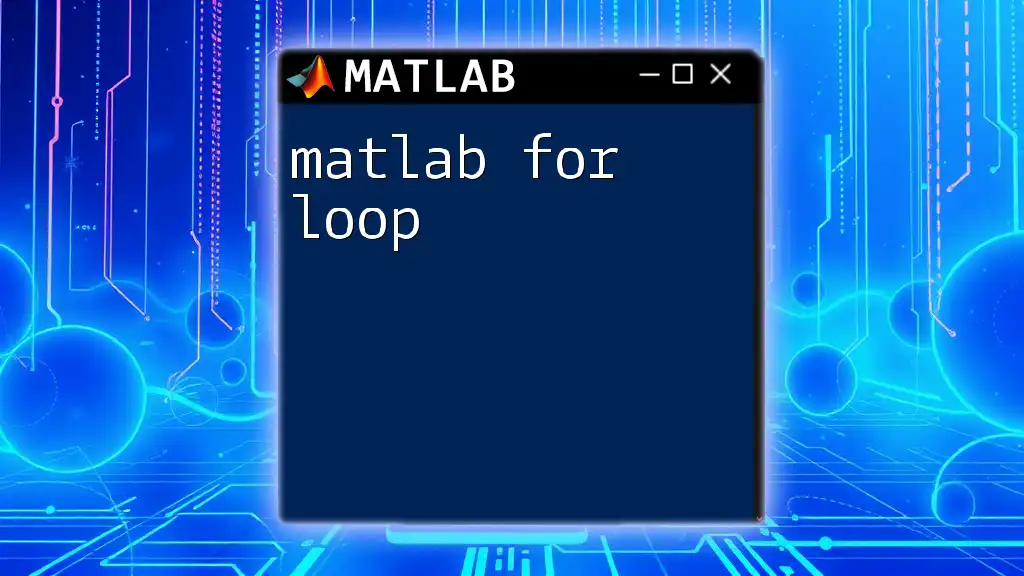
Practical Examples and Case Studies
Case Study: Industrial Application
PID controllers have extensive real-world applications. For instance, in a manufacturing setting, PID controllers regulate temperature, pressure, or speed.
- Performance Results and Lessons Learned: Effective implementation often leads to reduced variability and increased efficiency. The key takeaway is that precise tuning tailored to the specific dynamics of the system ultimately yields the best performance.
Quick Reference Guide for MATLAB and LabVIEW Commands
- Common MATLAB Commands for PID Control: `pid()`, `feedback()`, `step()`, `tune()`
- Key LabVIEW Functions for Control Systems: PID Control Toolkit, Control Design and Simulation Module
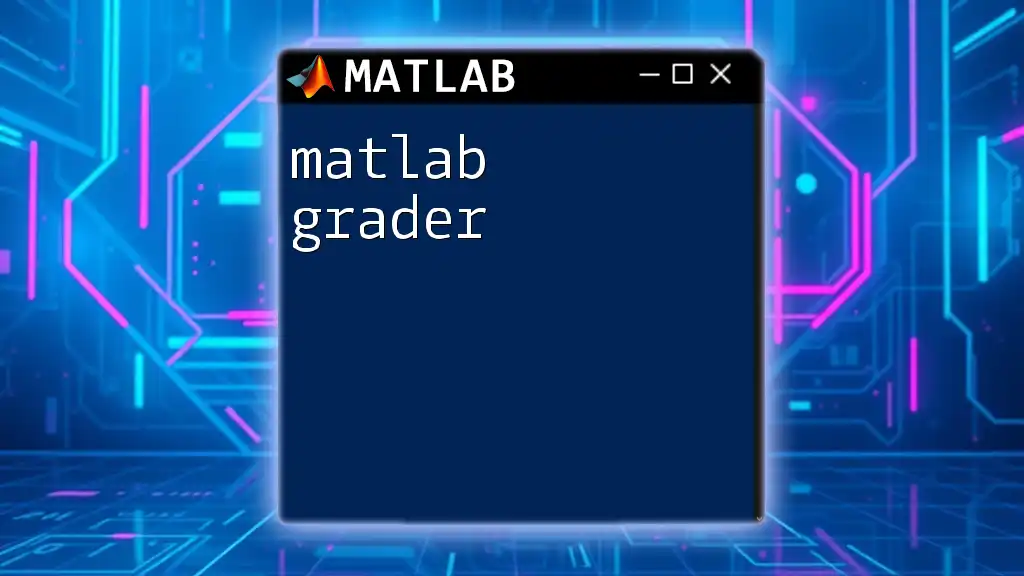
Conclusion
Optimizing PID controller performance using MATLAB or LabVIEW requires a solid understanding of the underlying principles, effective tuning techniques, and advanced optimization methods. By leveraging the capabilities of these powerful platforms and following a systematic approach, you can achieve superior control performance.
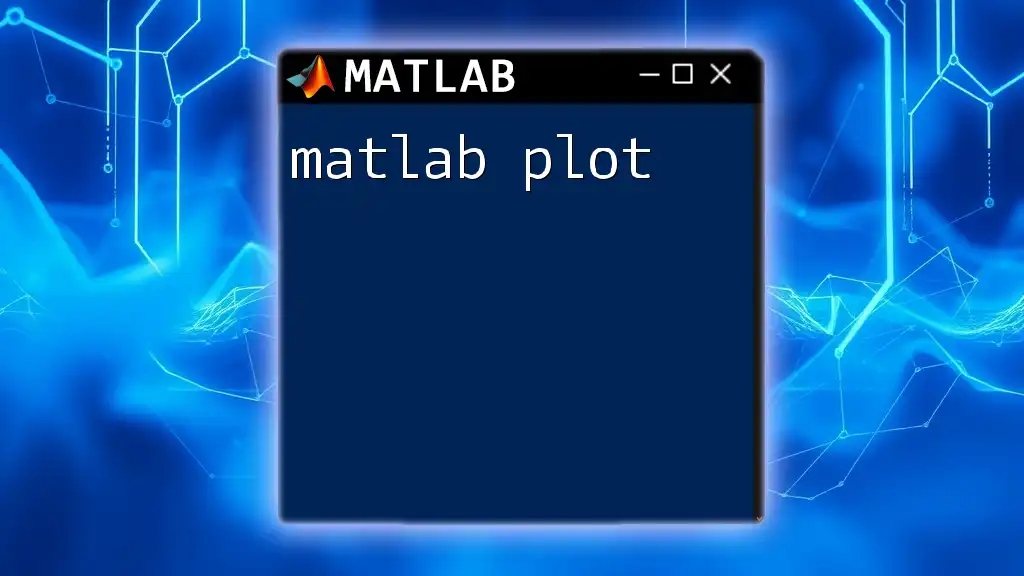
Additional Resources
To deepen your knowledge in PID control, consider exploring:
- Books on control systems and PID theory
- Articles focusing on practical applications
- Online courses specifically targeting MATLAB and LabVIEW for control engineers
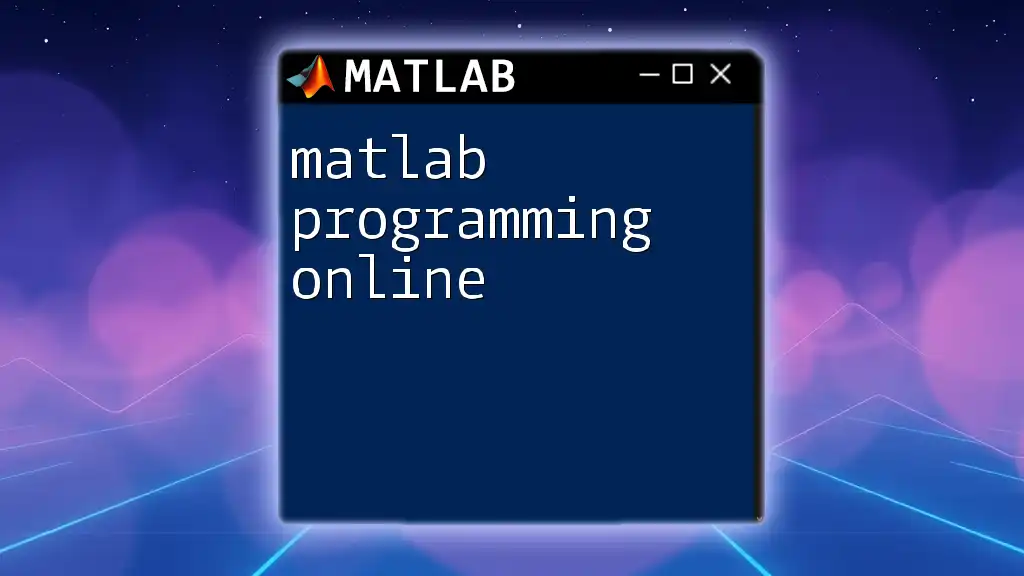
FAQs
Addressing common questions regarding PID controllers can enhance understanding and clarity. Troubleshooting tips and expert advice can facilitate a smoother learning curve for those new to the field.
By mastering these tools and techniques, you will be well on your way to becoming proficient in optimizing PID controller performance using MATLAB or LabVIEW.