A "for loop" in MATLAB allows you to execute a block of code repeatedly for a specified number of iterations, using a counter variable to control the loop.
Here’s a simple example:
for i = 1:5
disp(['Iteration: ' num2str(i)]);
end
Understanding the 'For Loop'
What is a For Loop?
A for loop is a fundamental programming construct that allows you to execute a block of code repeatedly, based on a defined range of values. Utilizing loops enables developers to automate repetitive tasks efficiently, reducing code duplication and improving maintainability. In the context of MATLAB, the for loop is essential for handling matrix and vector operations, making it a pivotal element of your programming toolkit.
When to Use a For Loop
You might choose to utilize a for loop in various situations, such as:
- When you know the exact number of iterations required.
- When iterating through arrays or matrices to apply operations to each element.
- In data analysis and visualizations where each data point needs to be processed or displayed iteratively.
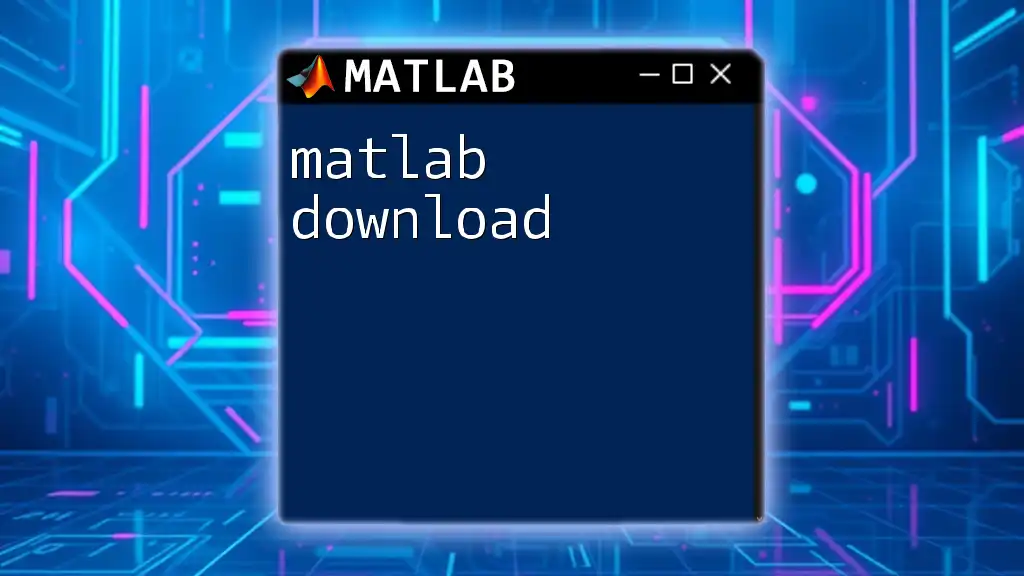
Syntax of a For Loop in MATLAB
Basic Structure
The general syntax of a for loop in MATLAB is straightforward:
for index = startValue:endValue
% Code to execute
end
In this structure:
- index is the loop control variable.
- startValue is the initial value of the index.
- endValue is the maximum value for the index; the loop will run until this value is reached.
Examples of Syntax
Here’s a basic example that sums numbers from 1 to 10:
sum = 0;
for i = 1:10
sum = sum + i;
end
disp(sum);
In this snippet, the loop iterates through values from 1 to 10, accumulating each value into the `sum` variable. This example highlights the for loop's ability to manage repetitive addition in a clean and compact manner.
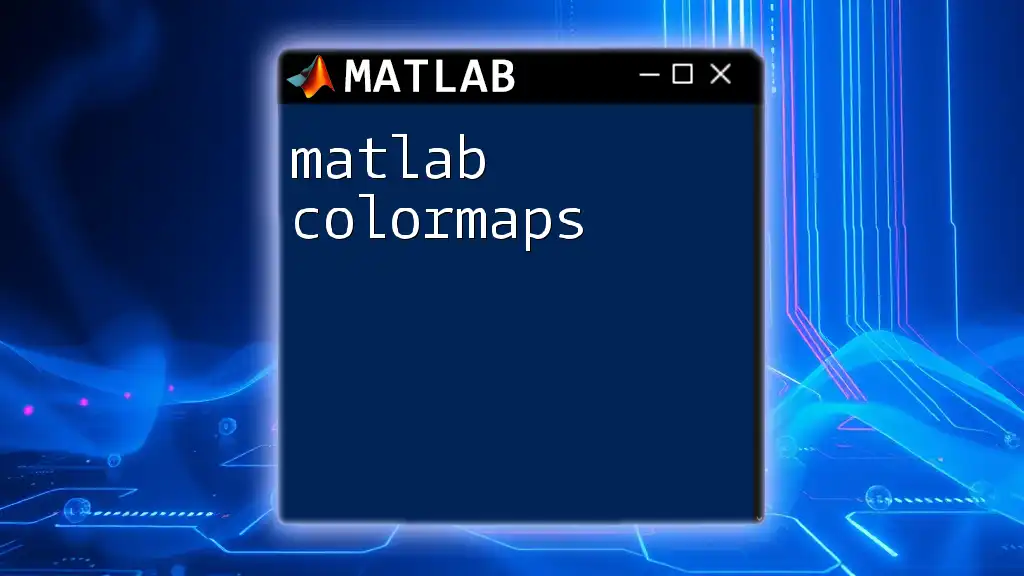
Key Concepts of For Loops
Loop Index
The loop index is a variable that changes with each iteration of the loop. It serves as a placeholder for the current value in the defined range, allowing you to perform operations with it. Understanding how the loop index evolves through iterations is critical for effective programming.
Direction and Steps in For Loops
The for loop syntax can be modified to change the step size, effectively allowing you to skip certain values. For instance:
for i = 1:2:10
disp(i);
end
In this example, the loop increments the index `i` by 2 every iteration, resulting in the output of odd numbers from 1 to 9 (1, 3, 5, 7, 9).
Loop Nesting
For loops can also be nested, meaning you can place one for loop inside another. This structure is particularly useful when working with multi-dimensional arrays or when you need to perform operations over multiple layers of data. Here’s an example:
for i = 1:3
for j = 1:2
fprintf('i=%d, j=%d\n', i, j);
end
end
In this nested loop, the outer loop iterates three times, while the inner loop runs twice for each iteration of the outer loop, producing a series of paired outputs.
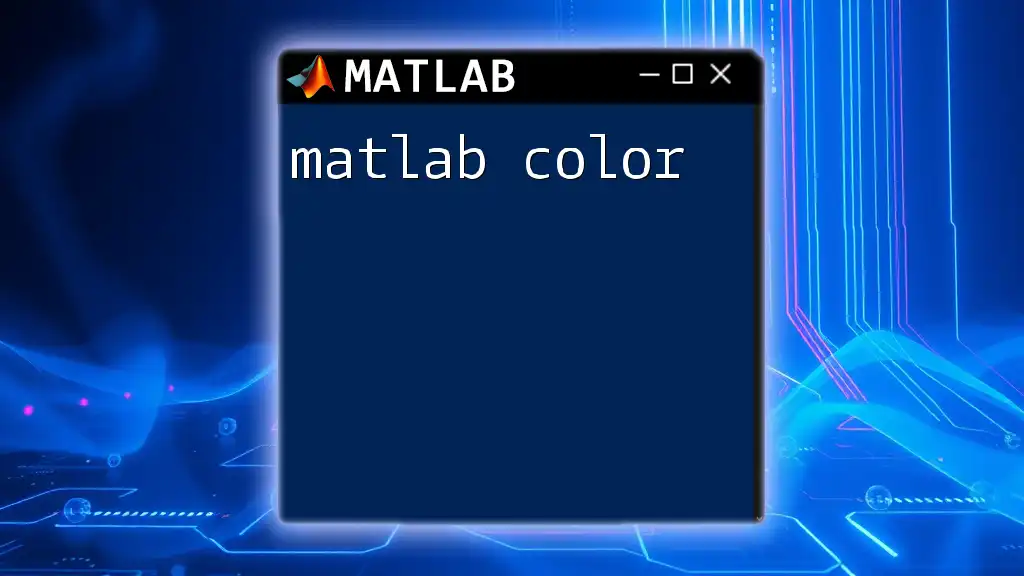
Practical Applications of For Loops
Array Manipulation
For loops shine when it comes to manipulating arrays. Here’s an example that modifies each element in a 2D array:
A = [1, 2, 3; 4, 5, 6];
for i = 1:size(A, 1)
for j = 1:size(A, 2)
A(i,j) = A(i,j) * 2; % Doubling each element
end
end
In this code snippet, we loop through each element of the 2D array `A`, doubling its value. The use of `size(A, 1)` and `size(A, 2)` ensures that we dynamically adapt to the dimensions of the array.
Data Visualization
For loops can also be instrumental in visualizing data points. For instance, consider the following code, where we plot individual points based on calculated values:
x = 0:0.1:2*pi;
y = sin(x);
figure;
for i = 1:length(x)
plot(x(i), y(i), 'ro'); % Plotting each point
hold on;
end
hold off;
In this example, we visualize the sine function by plotting red circles at each point (x, y) derived from the `sin` function. The loop processes each element of the `x` array and ensures a clear display of the sine wave.
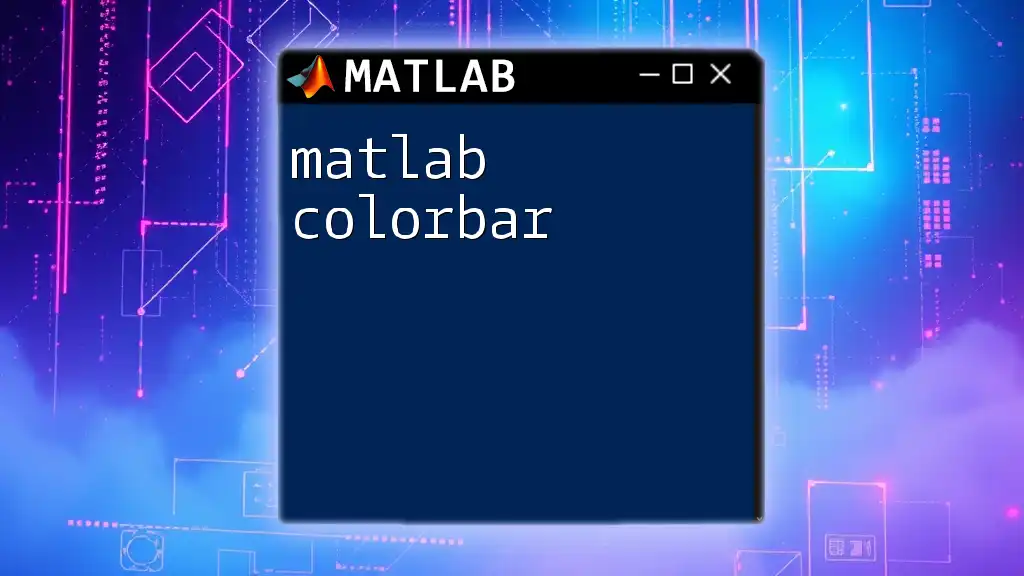
Best Practices in Using For Loops
Efficiency Considerations
Writing efficient loops is crucial. MATLAB is optimized for vectorized operations, which often perform better than looping through arrays. When possible, use vectorized expressions to achieve the same results without explicitly writing loops. This can significantly enhance the performance of your code.
Avoiding Common Mistakes
When using for loops, there are several pitfalls to watch out for:
- Variable Overwrite: Ensure that your loop index isn't overwritten unintentionally within your loop.
- Forgetting the End Statement: Always remember to include the `end` keyword to close your loops; missing it can lead to errors or unintended behavior.
- Infinite Loops: While less common with for loops, it’s crucial to ensure your loop parameters are set up correctly to avoid driving your scripts into infinite loops.
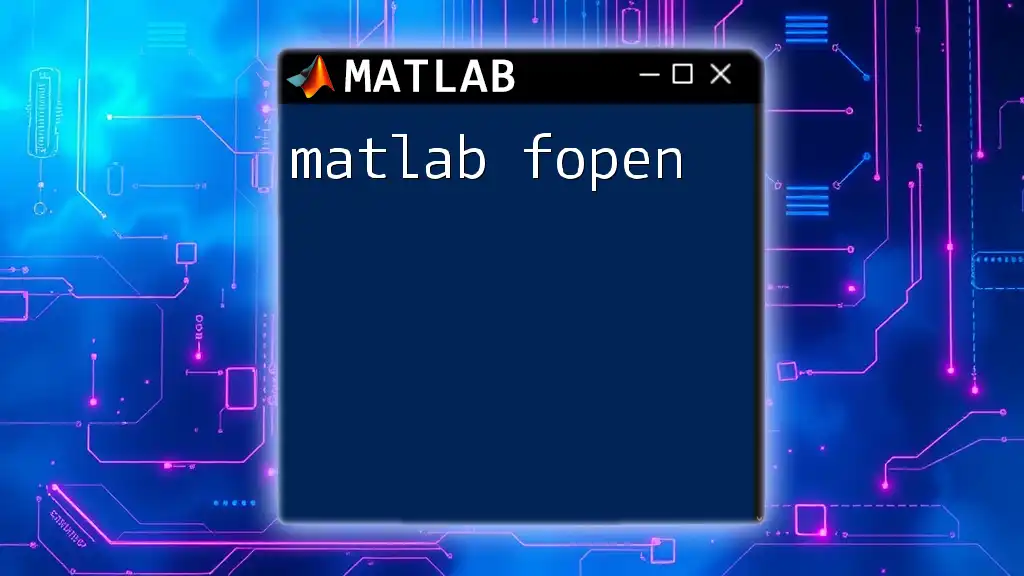
Conclusion
The for loop in MATLAB is a powerful and flexible tool for handling iterations efficiently. By mastering this construct, you can automate a wide range of tasks, manipulate data effortlessly, and enhance your programming capabilities. Engaging with practical examples will not only solidify your understanding but also make you more adept at tackling complex programming challenges.
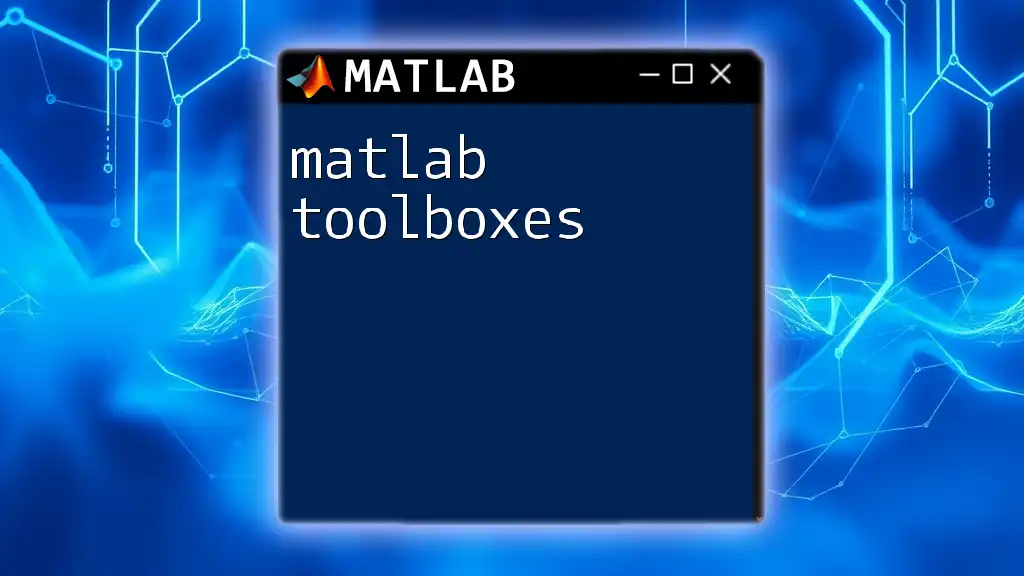
Additional Resources
To continue your journey with MATLAB, explore resources like official documentation, community forums, and specialized tutorials. Hands-on practice with exercises centered around the for loop will reinforce your learning and proficiency in MATLAB programming.