In MATLAB, you can create a simple 2D plot of a sine wave using the following commands:
x = 0:0.1:2*pi; % Create an array of x values from 0 to 2π
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Plot y versus x
title('Sine Wave'); % Add a title to the plot
xlabel('X Values'); % Label the x-axis
ylabel('Sine of X'); % Label the y-axis
Understanding MATLAB Plots
What is a MATLAB Plot?
A MATLAB plot is a graphical representation of data that allows users to visualize relationships and trends within their datasets. Through various plotting functions, MATLAB provides a robust platform for creating clear and customized visual depictions of numerical data, essential for analysis and interpretation.
Importance of Data Visualization
Effectively visualizing data can significantly enhance one's understanding of patterns, distributions, and anomalies. For instance, showing trends with line graphs or comparing distinct categories with bar graphs can tell a story far more intuitively than raw numbers alone. Data visualization is critical in scenarios such as academic research, business analytics, and scientific data interpretation, where making insights quickly can lead to impactful decisions.
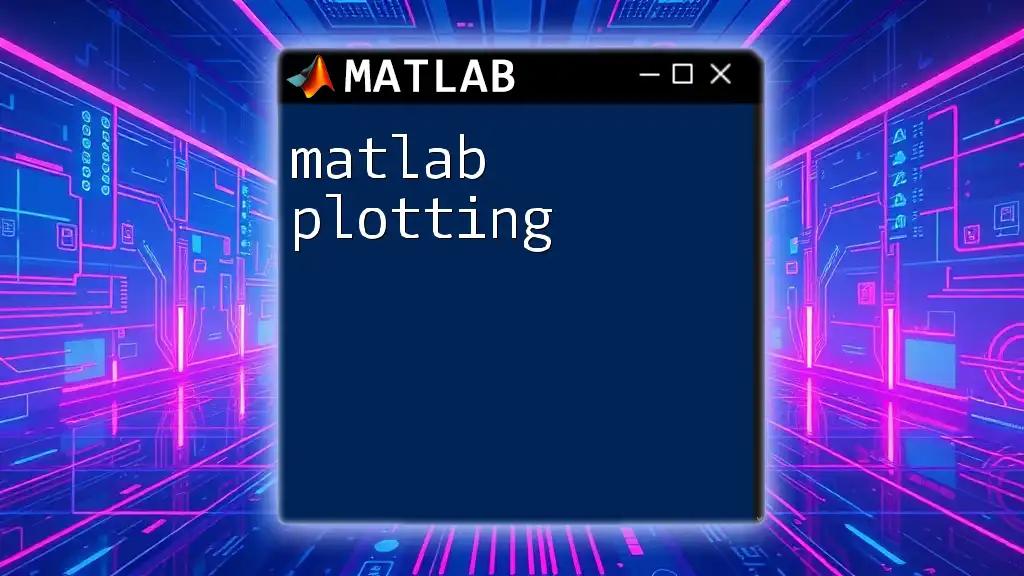
Types of MATLAB Plots
Basic Plotting Functions
2D Plots
The simplest and most common way to visualize data in MATLAB is through 2D plots. The `plot` function allows users to generate line graphs easily.
For example, consider creating a simple sine wave:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
In this example, `0:0.1:10` generates a vector of x-values from 0 to 10 with increments of 0.1, while `y = sin(x)` computes the sine of those values. The `grid on` command adds a grid for better visibility.
3D Plots
3D visualization takes data exploration a step further. The `plot3` function allows you to display three-dimensional data points.
Here's how to create a 3D spiral plot:
t = 0:0.1:10;
x = sin(t);
y = cos(t);
z = t;
plot3(x, y, z);
title('3D Spiral');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
grid on;
In this snippet, `t` generates a time vector from 0 to 10, with `x`, `y`, and `z` defined as sine, cosine, and time, respectively. This plotting function is invaluable for visualizing the relationship between three variables.
Specialized Plots
Bar Plots
Bar graphs are another potent way to present categorical data. The `bar` function creates a bar plot, which can highlight differences in magnitude between groups.
For instance, to create a simple bar graph of data:
data = [10, 20, 30];
bar(data);
title('Bar Graph of Data');
xlabel('Categories');
ylabel('Values');
This simple representation quickly communicates information, making it clear how each category compares against others.
Scatter Plots
Scatter plots are essential for showing correlations between two variables. The `scatter` function can display data points in a two-dimensional plane.
Here's an example:
x = rand(1, 100);
y = rand(1, 100);
scatter(x, y);
title('Scatter Plot Example');
xlabel('X-axis');
ylabel('Y-axis');
In this case, `rand(1, 100)` generates 100 random values for both x and y, creating a visual map of correlation or dispersion within the dataset.
Customizing Plots
Adding Titles, Labels, and Legends
Providing context to your plots is vital. Titles and axes labels can convey crucial information about what is being represented.
Here’s how to add these features:
plot(x, y);
title('My Customized Plot');
xlabel('My X-axis Label');
ylabel('My Y-axis Label');
legend('Data Legend');
A well-labeled plot can eliminate ambiguity, aiding in the interpretation of results.
Adjusting Axes
Using the `xlim` and `ylim` functions, you can manipulate the visible range of the axes, which can focus the viewer's attention on specific data points.
xlim([0 10]);
ylim([-1 1]);
By defining these limits, you can enhance the readability of your plots, presenting only the relevant portion of your data.
Changing Line Styles and Colors
MATLAB allows you to customize the visual aspects of your plots, such as line styles and colors.
For instance:
plot(x, y, 'r--'); % Red dashed line
This statement changes the line color to red and the style to dashed, allowing users to construct more visually appealing and informative plots.
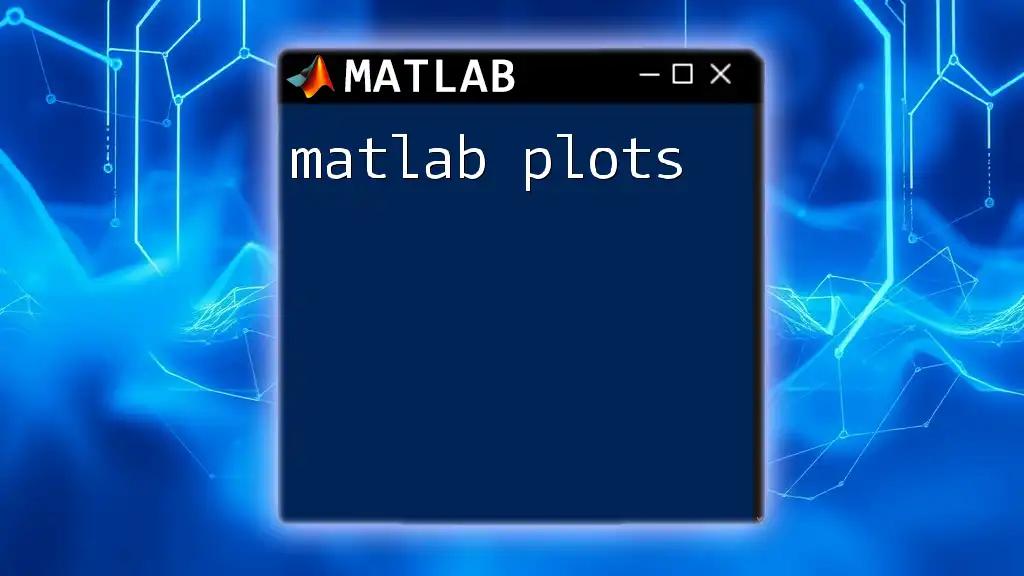
Advanced Plotting Techniques
Combining Multiple Plots
You can also overlay multiple plots within a single figure using the `hold on` command. This is useful for comparing datasets directly.
plot(x, y);
hold on;
plot(x, y + 0.5, 'r'); % overlaying another plot
hold off;
Using hold on ensures that the second plot appears on the same figure rather than creating a new one, allowing for direct comparison.
Exporting MATLAB Plots
Once you've created your visualizations, you might want to save them. MATLAB provides several functions for exporting plots to various file formats, including PNG and JPEG.
For example, to save a plot, you can use:
saveas(gcf, 'MyPlot.png');
This command saves the current figure as a PNG file named "MyPlot," enabling you to share or include it in reports seamlessly.
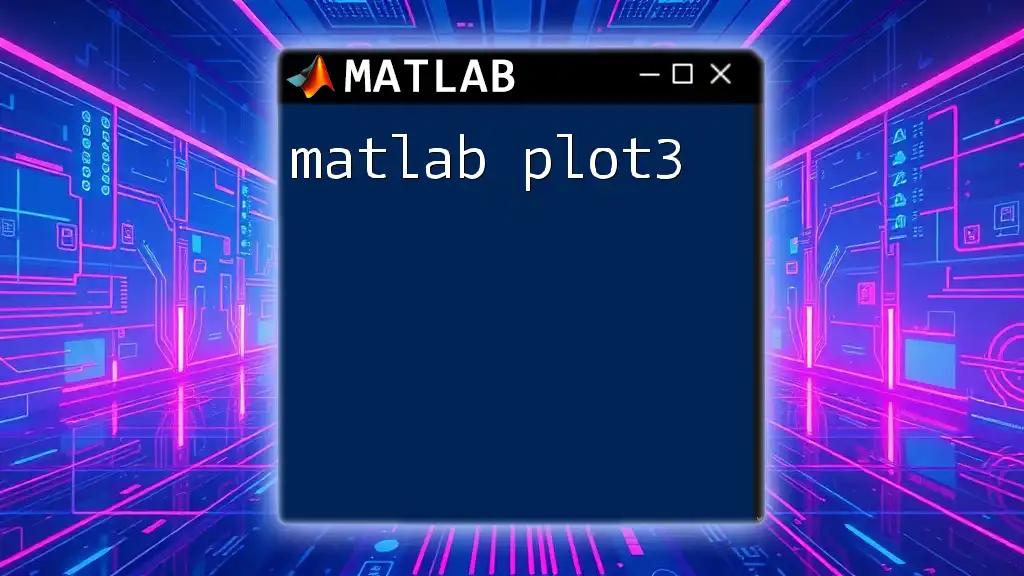
Common Plotting Errors and Troubleshooting
Error Handling in Plotting
Even experienced users can run into plotting issues, often due to dimension mismatches or incorrect function usage.
Common pitfalls include trying to plot vectors of differing sizes. Always ensure that your x and y-values match in dimensionality. MATLAB will throw an error if you attempt to plot vectors with different lengths. To troubleshoot, review your data and ensure alignment.

Conclusion
Mastering MATLAB plots is essential for anyone looking to analyze and present data effectively. With the various types of plots at your disposal, along with the ability to customize and combine them, you can create visually compelling representations of your data. Experimentation is key, so dive into MATLAB and start visualizing your datasets today!
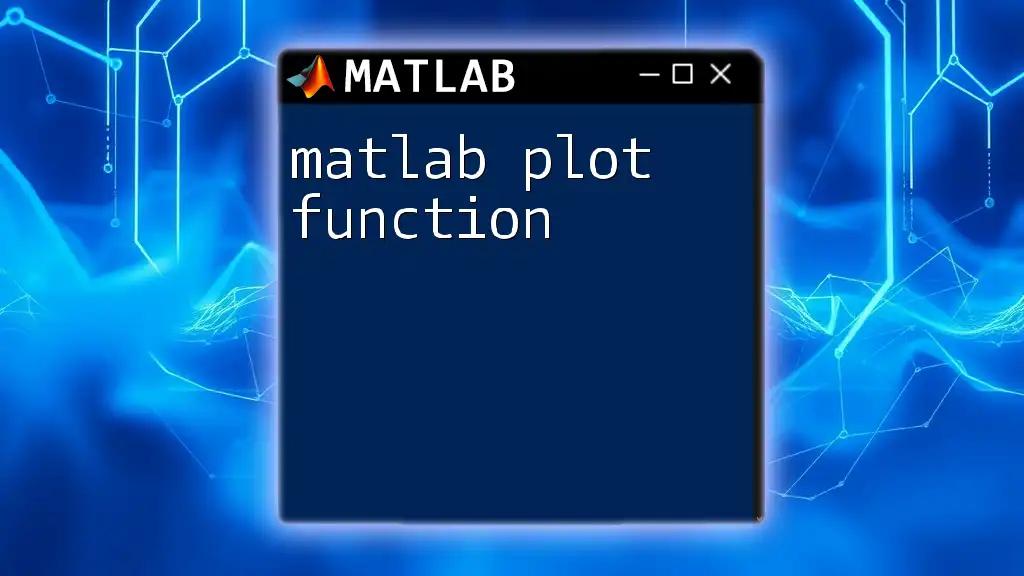
Additional Resources
To deepen your understanding of MATLAB plotting, look into official MATLAB resources and documentation. Joining MATLAB communities online can also provide valuable support and insights from fellow users.
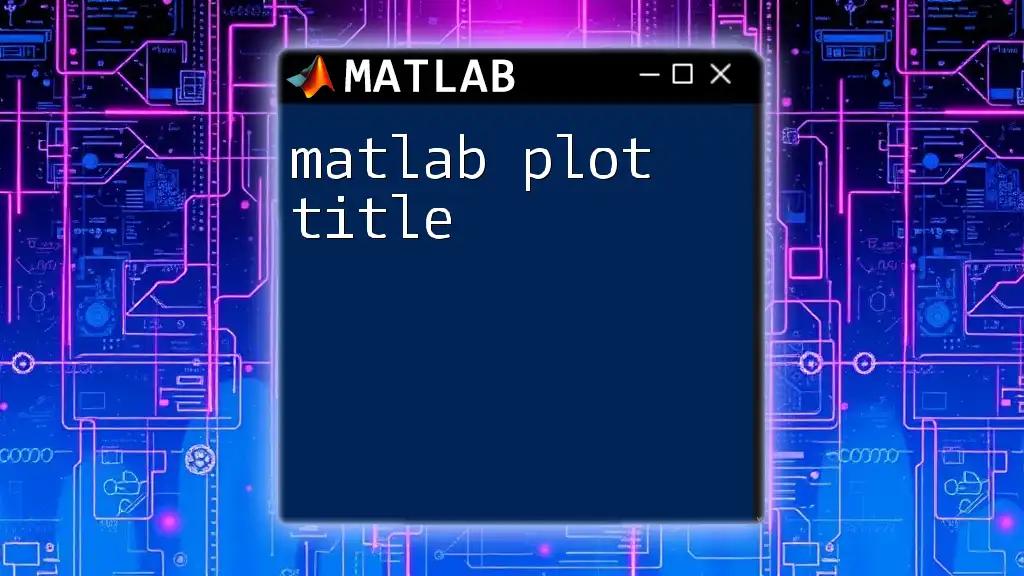
Call to Action
Ready to elevate your MATLAB skills? Sign up for our courses focused on mastering MATLAB plotting and unlock the power of data visualization!