Object-oriented programming in MATLAB allows you to define classes and objects, enabling encapsulation, inheritance, and polymorphism to create more modular and reusable code.
classdef Dog
properties
Name
Age
end
methods
function obj = Dog(name, age)
obj.Name = name;
obj.Age = age;
end
function bark(obj)
fprintf('%s says Woof!\n', obj.Name);
end
end
end
What is OOP?
Object-Oriented Programming (OOP) is a programming paradigm that uses "objects" to represent data and methods in a structured way. This approach is particularly vital in managing larger and more complex software projects, where modularity and reusability of code become paramount. The key principles of OOP—encapsulation, inheritance, and polymorphism—allow programmers to model real-world problems efficiently.
Benefits of Using OOP in MATLAB
Utilizing object-oriented programming in MATLAB MathWorks can significantly enhance your coding experience. Some benefits include:
- Simplifying Complex Problems: By organizing related data and functions together into objects, OOP makes it easier to manage and understand the code.
- Promoting Code Reusability: Classes can be reused in different projects with minimal modification, making programming faster and more efficient.
- Enhancing Software Maintenance: Changes can be localized within classes, preventing widespread alterations and facilitating easier updates to the codebase.
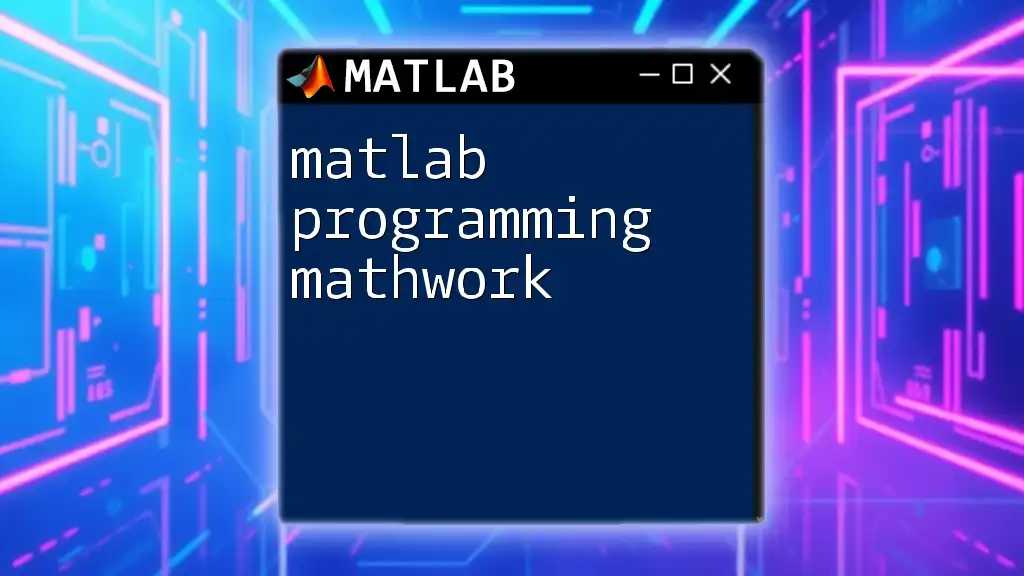
Getting Started with OOP in MATLAB
MATLAB as an OOP Language
MATLAB supports OOP principles, allowing you to define classes with properties and methods. This is a departure from the traditional procedural programming approach, where functions and data structures stand apart. Understanding how to leverage classes enables captivating programming possibilities.
Creating Your First Class
To create a class in MATLAB, you'll employ the `classdef` keyword. Here’s a simple example of a `Circle` class:
classdef Circle
properties
Radius
end
methods
function obj = Circle(radius)
obj.Radius = radius;
end
function area = getArea(obj)
area = pi * (obj.Radius)^2;
end
end
end
In this snippet, the `Circle` class has a property called `Radius` and two methods: a constructor and a method to calculate the area.
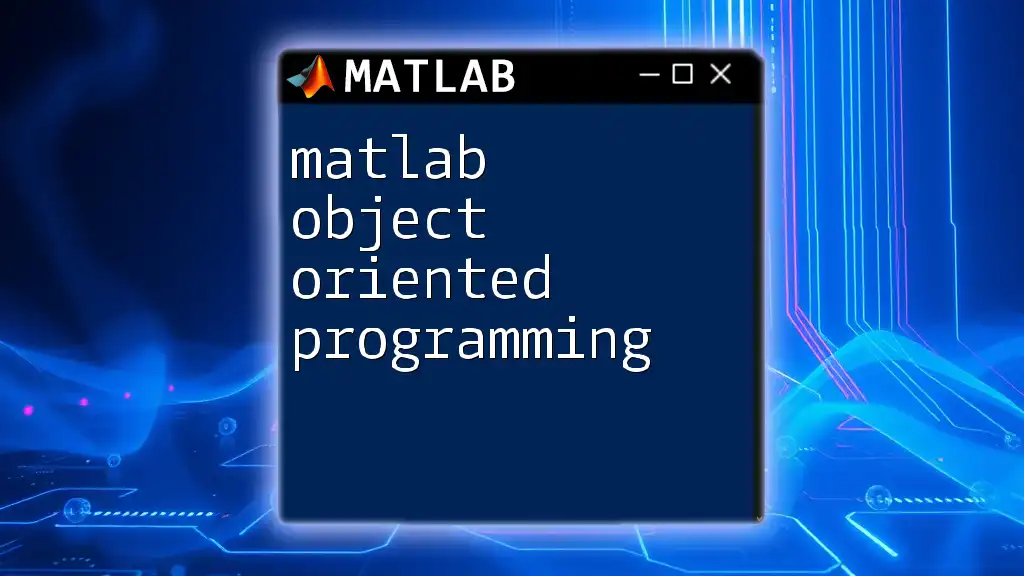
Key Concepts in MATLAB OOP
Classes and Objects
Classes are blueprints for creating objects. When you instantiate a class, you create an object that encapsulates both data and methods defined in that class.
Example: Creating and using a `Circle` object:
myCircle = Circle(5);
disp(['Area of the circle: ', num2str(myCircle.getArea())]);
This code instantiates a circle with a radius of 5 and displays its area.
Properties and Methods
Properties define the attributes of a class, while methods describe its behaviors. You should think of properties as variables that store information, and methods as functions that operate on that information.
Constructors and Destructors
Constructors are special methods called when you instantiate an object. They initialize the properties of the object. You can define a destructor to manage resource deallocation. Here’s how you might define a constructor in the `Circle` class:
function obj = Circle(radius)
obj.Radius = radius;
end
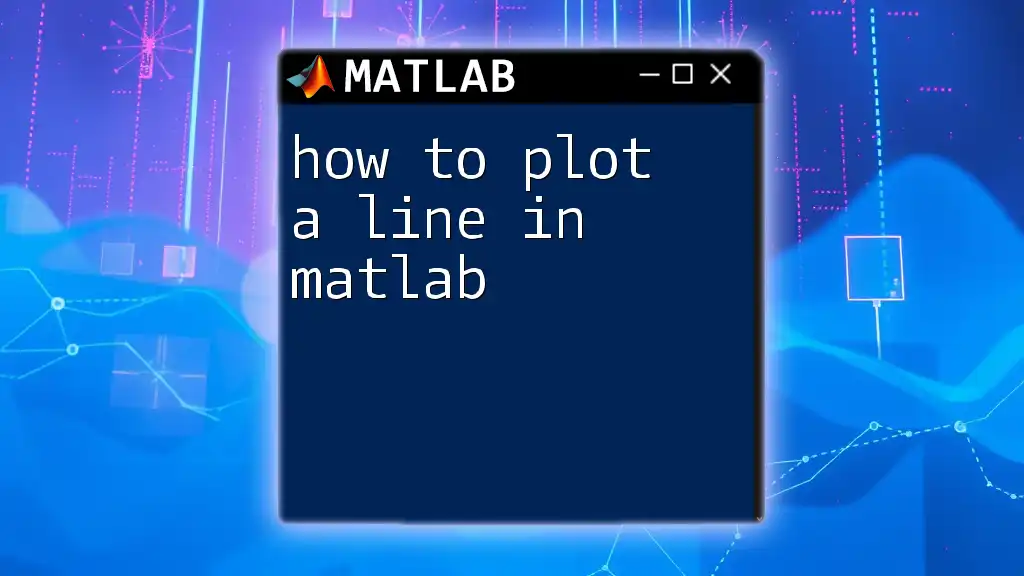
Advanced OOP Concepts in MATLAB
Inheritance
Inheritance allows you to create a new class (the subclass) that extends an existing class (the superclass). This is useful for creating a hierarchy of classes.
Example: A `Cylinder` class that inherits from `Circle`:
classdef Cylinder < Circle
properties
Height
end
methods
function obj = Cylinder(radius, height)
obj@Circle(radius); % call constructor of parent class
obj.Height = height;
end
function volume = getVolume(obj)
volume = obj.getArea() * obj.Height;
end
end
end
In this example, `Cylinder` has both the properties and methods of `Circle` while adding its own.
Polymorphism
Polymorphism allows different classes to be treated as instances of the same class through method overriding. This means you can define a method in a subclass that has the same name as a method in a superclass but is implemented differently.
Abstract Classes and Interfaces
Abstract classes are templates that cannot be instantiated directly. They define common behavior for their subclasses. Interfaces define a contract that must be adhered to without providing any implementation, giving flexibility to your code structure.
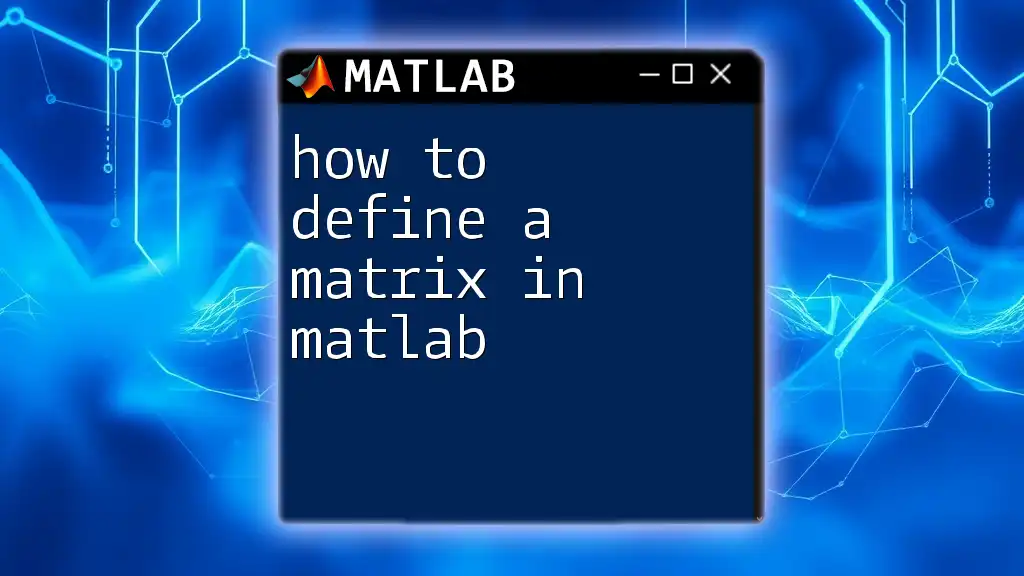
Best Practices for OOP in MATLAB
Designing Classes for Flexibility and Reusability
Design your classes to be as flexible as possible. Consider potential future use cases and ensure your class can be adapted without requiring extensive changes.
Using Access Modifiers
MATLAB allows you to define the accessibility of properties and methods using access modifiers such as `public`, `protected`, and `private`. This encapsulation is crucial in controlling how other parts of your code interact with your objects.
Example: Using Access Modifiers
properties (Access = private)
secretCode
end
Such an implementation protects core attributes and maintains the integrity of the object.
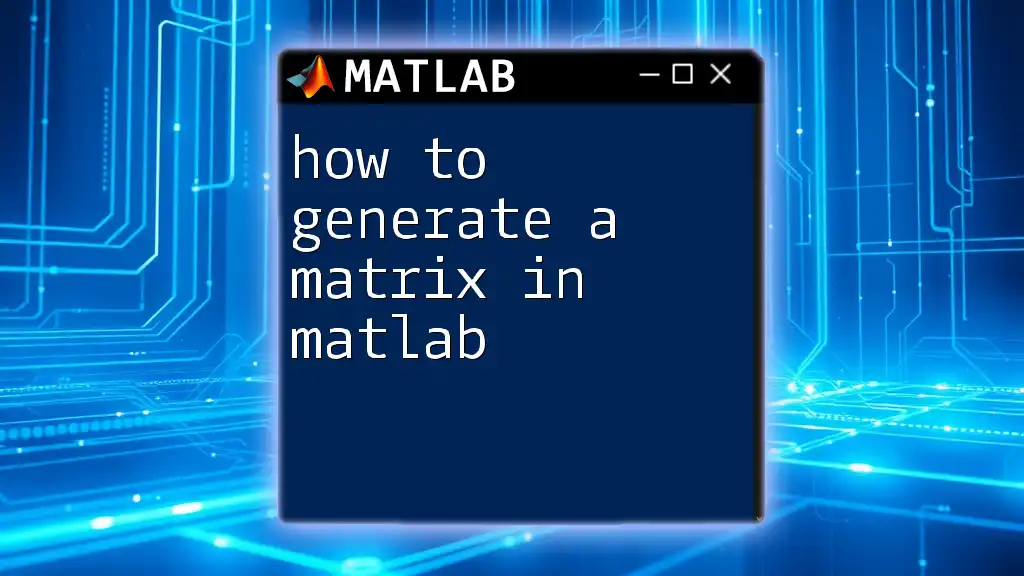
Common Use Cases for OOP in MATLAB
Simulation and Modeling
OOP shines in simulation and modeling tasks. You can create modular objects representing different components, making it simpler to manage interactions between various entities.
Data Analysis and Visualization
Classes can be designed to handle data pipelines and produce visualizations, thus abstracting away complex operations and making your code cleaner and more manageable.
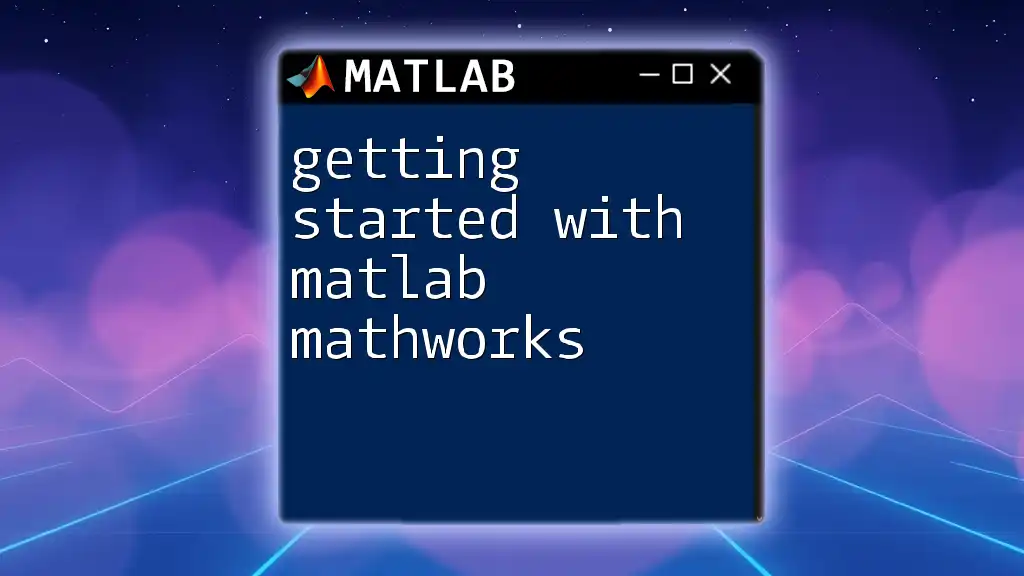
Conclusion
To sum up, object-oriented programming in MATLAB MathWorks provides powerful tools for organizing your code and enhancing functionality. By embracing OOP principles such as classes, methods, and inheritance, you can effectively tackle complex problems, promote code reuse, and maintain software more easily. Experiment with these concepts, and you'll find that OOP will transform the way you approach programming in MATLAB.