PCA (Principal Component Analysis) in MATLAB is a statistical technique used to reduce the dimensionality of data while preserving as much variance as possible, and can be implemented using the built-in `pca` function.
Here's a simple code snippet to perform PCA on a dataset:
% Sample data matrix X (rows: observations, columns: variables)
X = [1.0, 2.0; 2.0, 3.0; 3.0, 4.0; 4.0, 5.0];
% Perform PCA
[coeff, score, latent] = pca(X);
% coeff: principal component coefficients
% score: representation of X in the principal component space
% latent: eigenvalues of the covariance matrix (variance explained)
Understanding PCA
What is PCA?
Principal Component Analysis (PCA) is a statistical technique that transforms a dataset into a set of orthogonal (uncorrelated) variables, called principal components. These components capture the most variance present in the data. The main purpose of PCA is to reduce the dimensionality of large datasets while preserving as much variance as possible. This is particularly useful in data analysis, enabling us to simplify complex datasets without losing significant information.
Importance of PCA
PCA finds its significance across various fields such as finance, biology, image processing, and more. By reducing the dimensionality, PCA allows for more efficient data compression and noise reduction. Its broad applicability includes improving the effectiveness of machine learning algorithms and enhancing data visualization.
Among the various benefits PCA offers, its ability to reduce computational costs, improve model performance, and provide clearer visual interpretations cannot be overstated. PCA simplifies complex datasets, making them easier to work with and analyze.
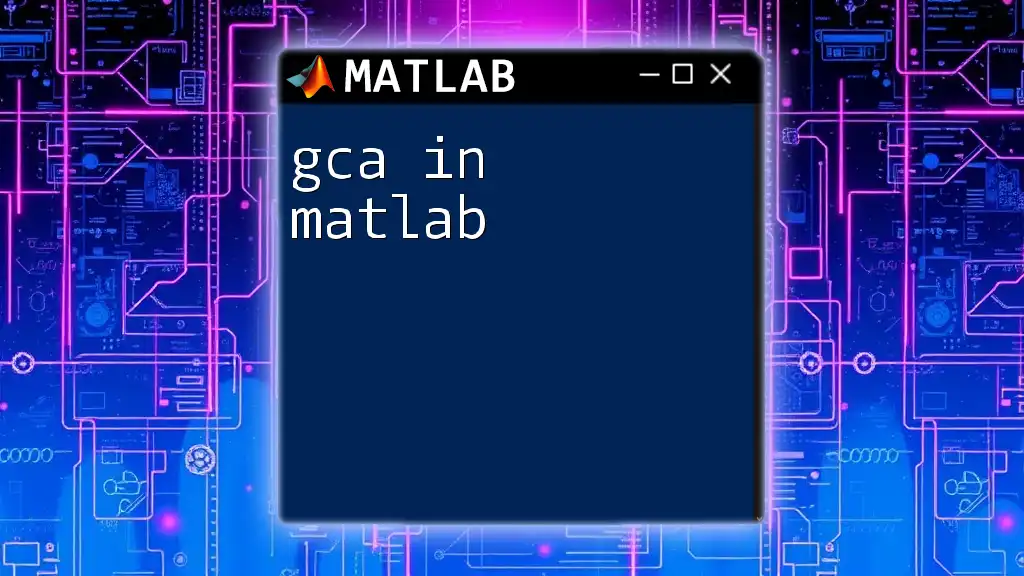
Getting Started with MATLAB for PCA
Setting Up Your MATLAB Environment
Before diving into PCA in MATLAB, ensure that you have MATLAB installed on your system. The installation process is straightforward; visit the official MathWorks website and follow the installation instructions that suit your operating system.
For PCA, it is essential to have the Statistics and Machine Learning Toolbox installed, as it provides useful functions for statistical analysis and multivariate techniques.
Basic MATLAB Commands Overview
MATLAB’s intuitive syntax allows users to perform computations easily. A few key commands are especially beneficial while conducting PCA:
- `mean`: Computes the mean of an array.
- `cov`: Calculates the covariance matrix.
- `eig`: Computes the eigenvalues and eigenvectors of a matrix.
Familiarizing yourself with these commands will streamline the PCA implementation process.
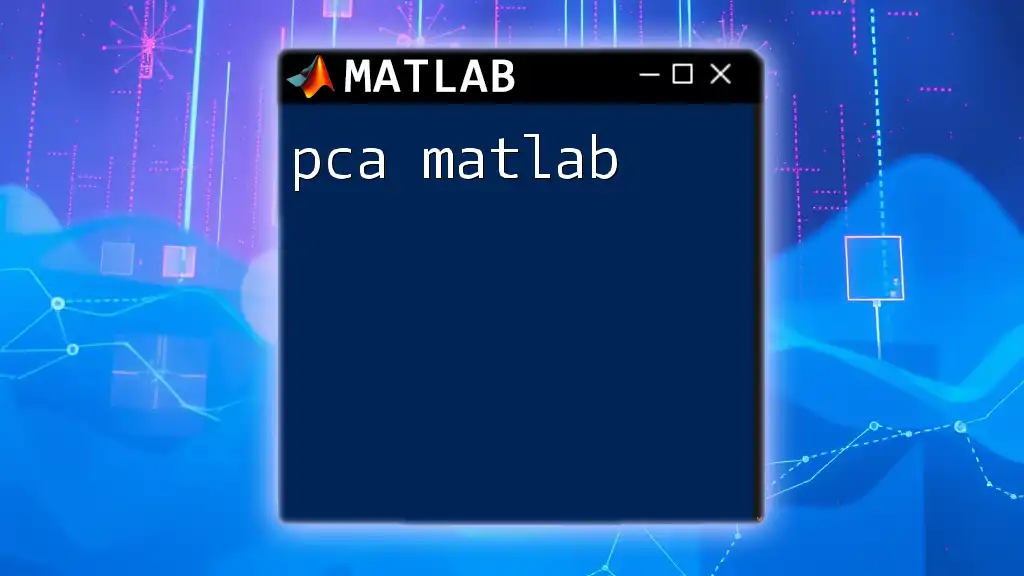
Implementing PCA in MATLAB
Preparing Your Data
The first step in applying PCA is preparing your data. Loading your dataset into MATLAB can be done via commands like `load` or `readtable`. This enables you to work directly with your data in MATLAB’s workspace. Remember, data preparation also includes normalizing your dataset to ensure that every feature contributes equally to the distance calculations involved in PCA.
Normalizing your data can be achieved using the `zscore` function, which standardizes the data:
data = [ ... ]; % Replace with your actual data matrix
normData = zscore(data); % Standardizing the data
Performing PCA
Step 1: Calculate the Covariance Matrix
The covariance matrix is crucial because it describes how much the dimensions of your dataset vary with each other. To calculate the covariance matrix of your normalized data, use the following command:
covarianceMatrix = cov(normData);
This matrix serves as the foundation for the subsequent steps.
Step 2: Compute Eigenvalues and Eigenvectors
Eigenvalues and eigenvectors are pivotal in PCA as they reveal the directions of maximum variance in your data. By computing them, you gain insights into which components account for the most variance.
Use the `eig` function to compute eigenvalues and eigenvectors from the covariance matrix:
[eigenVectors, eigenValues] = eig(covarianceMatrix);
Step 3: Sort Eigenvalues and Eigenvectors
To effectively select the most significant principal components, sort the eigenvalues in descending order. This sorting tells us which components to keep for reducing dimensionality. The corresponding eigenvectors should also be sorted based on the sorted eigenvalues.
Here’s how to sort them in MATLAB:
[sortedEigenValues, sortOrder] = sort(diag(eigenValues), 'descend');
sortedEigenVectors = eigenVectors(:, sortOrder);
Step 4: Forming the Feature Vector
A feature vector comprises the selected eigenvectors that define the new feature space. Choosing the right number of principal components to retain is a key decision in PCA.
For instance, if we decide to keep two principal components, use the following code:
numComponents = 2; % Choose the number of principal components
featureVector = sortedEigenVectors(:, 1:numComponents);
Step 5: Recasting the Data into the New Space
Finally, project the original normalized data onto the newly formed principal component space. This transformation yields a new dataset with reduced dimensions.
Implement the projection with this command:
pcaData = normData * featureVector;
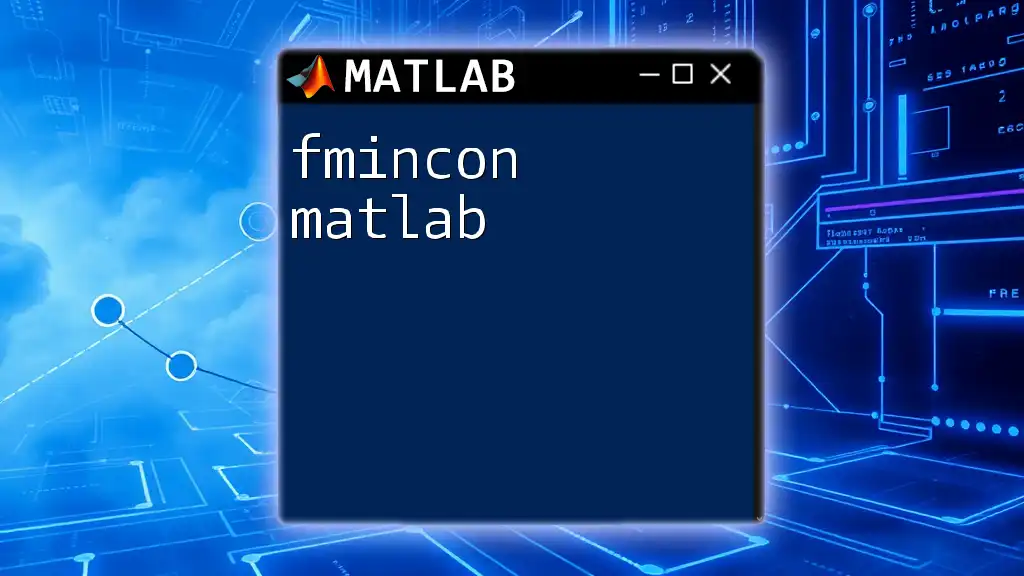
Visualizing PCA Results
Visualizing PCA results is vital for interpreting the findings. A scatter plot can provide insights into how the original data points cluster in the new feature space. Use the following code snippet to create a basic scatter plot of the PCA results:
scatter(pcaData(:, 1), pcaData(:, 2));
title('PCA Result');
xlabel('Principal Component 1');
ylabel('Principal Component 2');
This graphical representation allows you to discern patterns or groupings within the reduced data, which can be critical in analysis and decision-making.
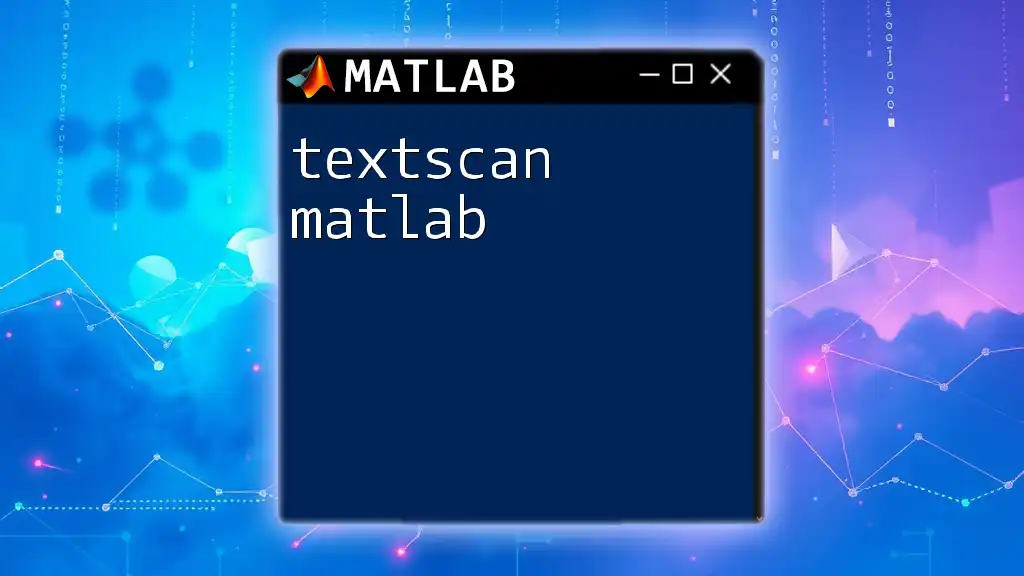
Applications and Use Cases of PCA in MATLAB
Case Study 1: Image Compression
PCA can significantly reduce the storage requirements for images. By transforming image data into a lower-dimensional space, we can store only the most relevant features while discarding less significant data. For instance, given a dataset of images, applying PCA allows for reconstruction of the original images using only the principal components. This technique effectively compresses the images for easier storage and transmission.
Case Study 2: Genomic Data Analysis
PCA is widely utilized in bioinformatics for visualizing high-dimensional genomic data. For example, gene expression data often contains thousands of genes for relatively few samples. By implementing PCA in MATLAB, researchers can visualize clusters of similar samples or identify outliers, making it easier to interpret genetic links and biological significance.
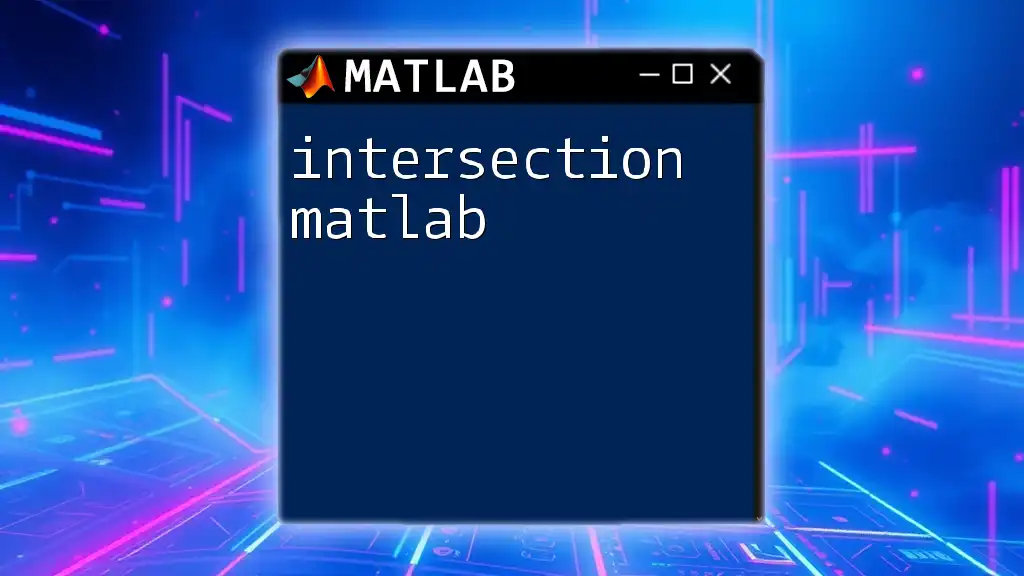
Best Practices for PCA
Choosing the Right Number of Components
Selecting the optimal number of components to retain is crucial. Two effective techniques include calculating the explained variance or creating a scree plot. The explained variance measures how much variance each principal component captures, guiding you in selecting an adequate number of components.
Here’s a code snippet to compute the cumulative explained variance:
explainedVariance = cumsum(sortedEigenValues) / sum(sortedEigenValues);
Avoiding Common Pitfalls
When implementing PCA, avoid common mistakes, such as failing to normalize your data or choosing too many or too few components. It is vital to understand that PCA is sensitive to scaling, and applying it to unnormalized data can lead to misleading results. Additionally, be wary of overfitting by retaining too many dimensions.
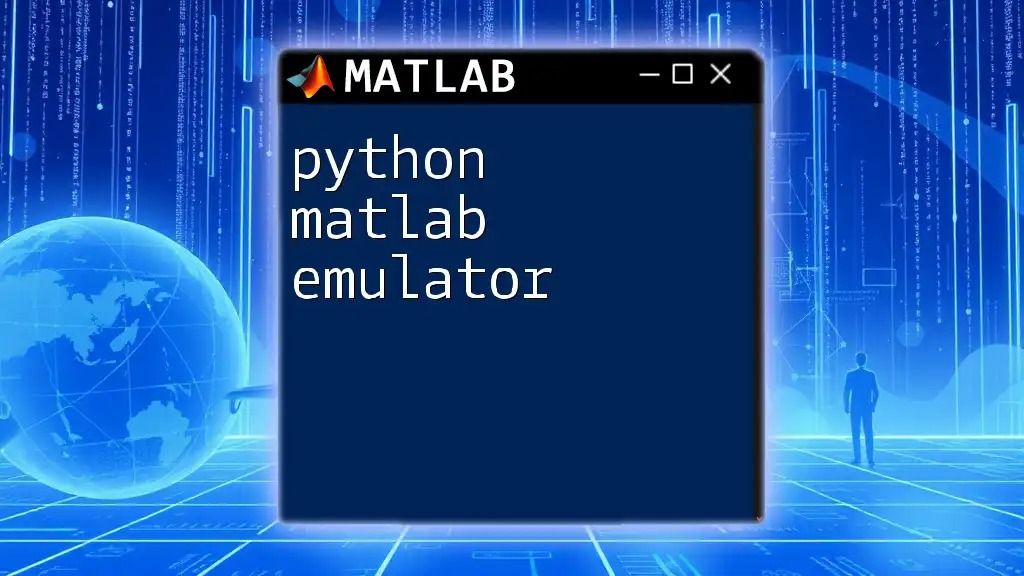
Conclusion
PCA on MATLAB is a powerful method for simplifying complex datasets while retaining essential information. By following the above steps and best practices, you can leverage PCA effectively in your projects. With its wide applications across various fields, mastering PCA will enrich your data analysis skills and enhance your ability to derive meaningful insights from data.
Additional Resources
To deepen your understanding, consider exploring books and tutorials on PCA and MATLAB. Many platforms offer comprehensive online courses that can help you master this powerful technique. By continuously learning, you can elevate your proficiency in data analysis and research methodologies.