A Python MATLAB emulator allows users to run MATLAB commands in a Python environment, making it easier to integrate MATLAB functionalities with Python tools.
Here’s an example of a simple MATLAB command in a Python environment using the `matlab.engine` package:
x = 0:0.1:10; % Create a vector from 0 to 10 with a step of 0.1
y = sin(x); % Compute the sine of each element in x
plot(x, y); % Plot the sine wave
What is a Python MATLAB Emulator?
A Python MATLAB emulator refers to a tool or framework that allows users to execute MATLAB commands and scripts within a Python environment. Emulators aim to recreate the functionality of MATLAB in Python, offering a seamless experience for those familiar with MATLAB syntax. The primary advantage of using such an emulator is that it extends the powerful features of MATLAB while leveraging the open-source and versatile nature of Python.
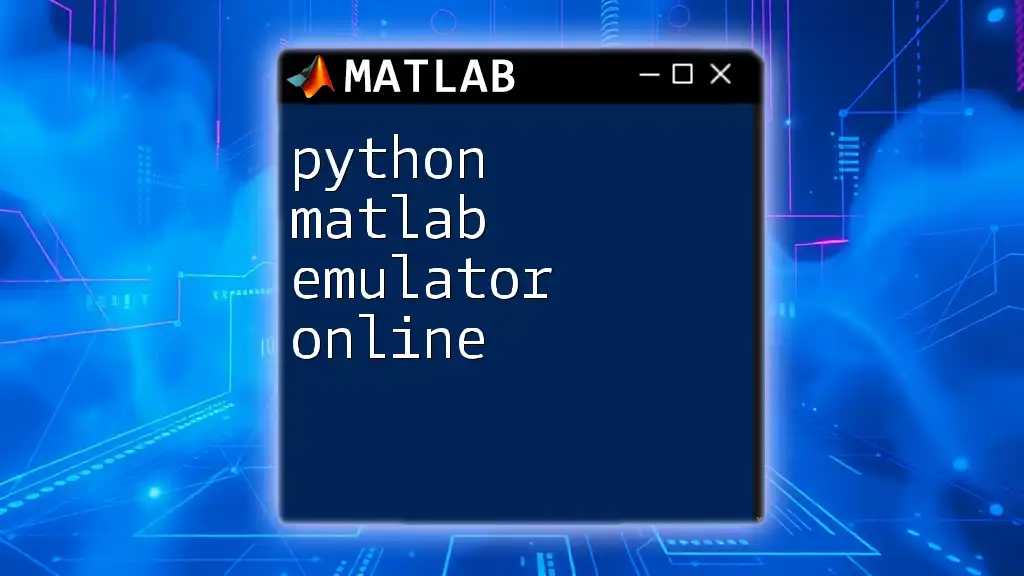
Why Use Python as a MATLAB Emulator?
Choosing Python as a MATLAB emulator provides several benefits:
- Open-source: Unlike MATLAB, which is proprietary software requiring a license, Python is free and open-source. This makes it an accessible option for both students and professionals.
- Cost-effective: With no licensing fees, learners can utilize Python and its packages without financial barriers.
- Community support: Python has a vibrant community with countless libraries and frameworks. Support can often be found through forums, documentation, and tutorials which enable users to enhance their skills rapidly.
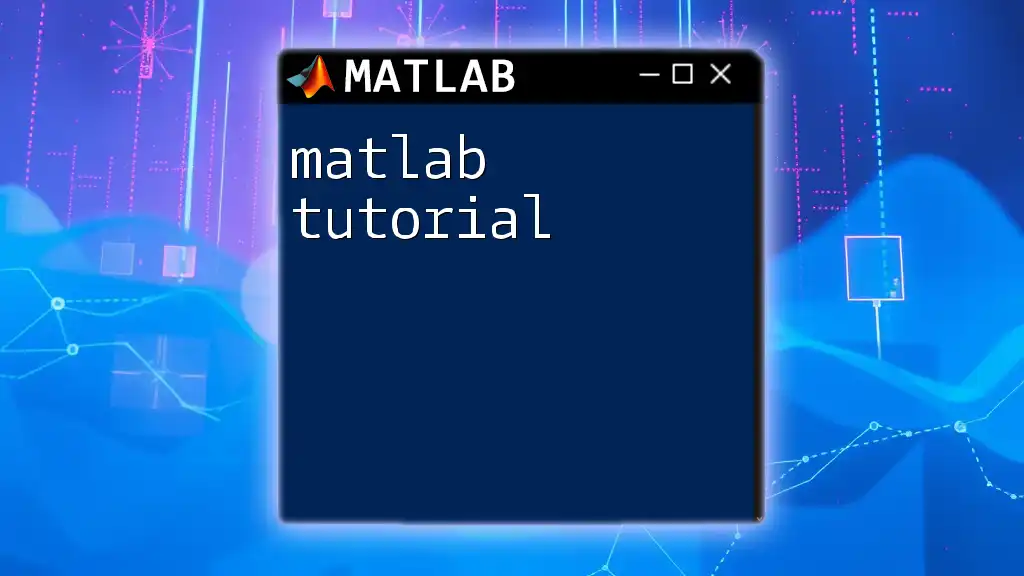
Popular Python MATLAB Emulators
Pymatbridge
Pymatbridge is one of the well-known tools that facilitate communication between Python and MATLAB. It provides a convenient way to run MATLAB commands directly from Python.
To get started with Pymatbridge, follow these installation instructions:
- Install the library via Python's package manager.
- Ensure MATLAB is installed on your machine and is accessible through the command line.
Basic usage example:
import pymatbridge
# Initializing MATLAB connection
matlab = pymatbridge.Matlab()
matlab.start()
# Example of running a MATLAB command
result = matlab.run_code('x = 1:10; y = x.^2;')
print(result)
In this example, the command initializes a connection to MATLAB and executes a basic command to create a vector and compute the square of its elements.
Octave
GNU Octave is often regarded as a MATLAB-compatible language, and it is an essential tool for users seeking a robust alternative to MATLAB.
While it differs from MATLAB in certain aspects, many common functionalities remain intact. Key features include:
- Compatibility with many MATLAB scripts
- A syntax that is nearly identical to MATLAB
Users who wish to embrace an open-source MATLAB-like environment frequently find Octave an excellent choice.
MATLAB Engine API for Python
Another robust emulator is the MATLAB Engine API for Python. This API allows users to call MATLAB functions from Python scripts, effectively bridging the two languages.
To utilize the MATLAB Engine API, follow these steps for installation:
pip install matlab.engine
Example of connecting Python to MATLAB:
import matlab.engine
# Start MATLAB engine
eng = matlab.engine.start_matlab()
# Run MATLAB commands from Python
result = eng.eval('sqrt(16)')
print(result) # Output: 4.0
In this code snippet, you can see how easy it is to start the MATLAB engine and run a simple command to compute the square root.
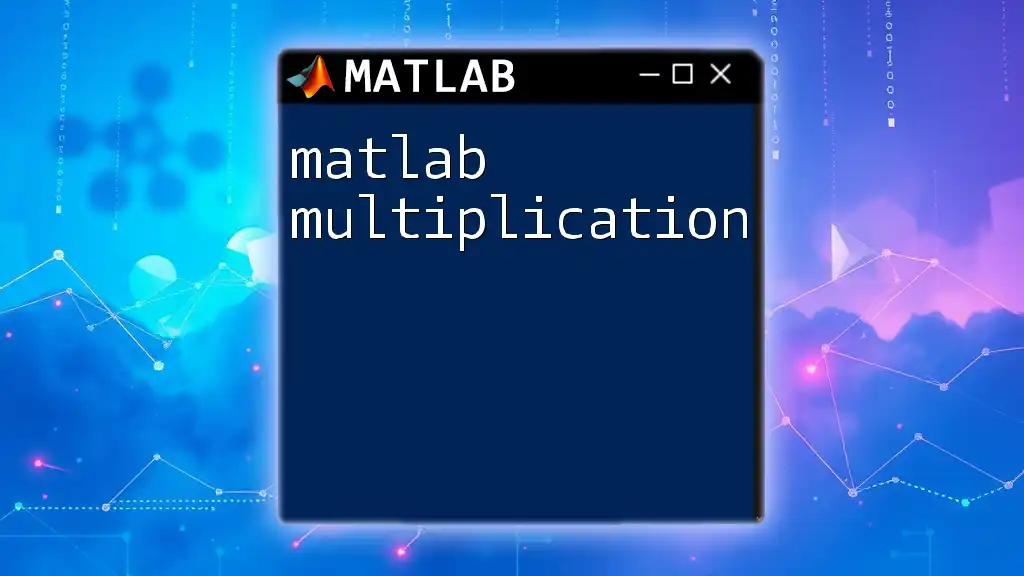
Key Features of Python MATLAB Emulators
Compatibility with MATLAB Functions
A primary goal of any Python MATLAB emulator is to ensure compatibility with MATLAB functions. This includes:
- Matrix operations: Emulators allow you to perform complex array manipulations fundamentally similar to MATLAB.
- Plotting capabilities: You can leverage plotting functions to visualize data, much like you would in MATLAB.
Libraries and Packages
Python's extensive libraries enhance the capability of emulators:
NumPy
NumPy is a foundational library in Python for numerical computing. It provides MATLAB-like functionality, especially around matrix operations.
Example of using NumPy for matrix operations:
import numpy as np
a = np.array([[1, 2], [3, 4]])
b = np.linalg.inv(a)
print(b)
This example demonstrates how a NumPy user can easily compute the inverse of a matrix, similar to MATLAB.
Matplotlib
Matplotlib is a powerful plotting library in Python that provides capabilities comparable to MATLAB's plotting functions.
Example of creating a simple plot:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [10, 20, 25, 30]
plt.plot(x, y)
plt.title('Example Plot')
plt.show()
This code creates a basic line plot, demonstrating how easy it is to visualize data with Matplotlib.
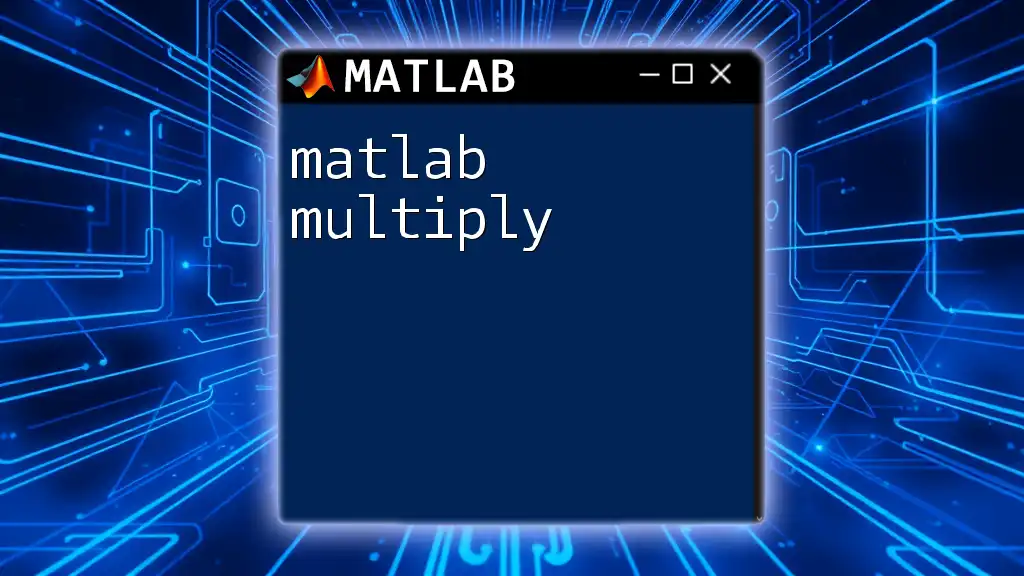
Installation and Setup
System Requirements
Before diving into using a Python MATLAB emulator, ensure you meet the following system requirements:
- Compatible operating systems (Windows, macOS, Linux)
- A supported version of Python and MATLAB (or the emulator-specific dependencies)
Step-by-Step Installation Guide
- Installing the emulator of your choice: Follow the specified instructions for installing Pymatbridge, Octave, or the MATLAB Engine API.
- Configuring the environment: Ensure that path variables are set so that Python can locate your MATLAB installation and relevant libraries.
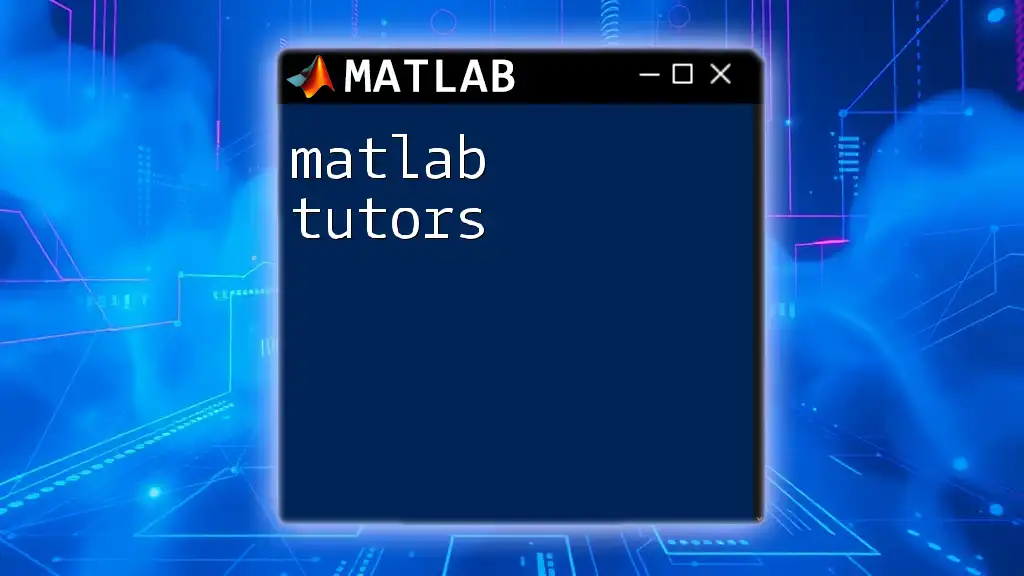
Performance Considerations
Speed and Efficiency
When using Python MATLAB emulators, speed may vary based on the complexity of the MATLAB functions called and the underlying performance of the Python interpreter. While basic operations execute rapidly, some advanced MATLAB features may perform slower due to additional overhead or data type conversions.
To enhance performance:
- Utilize vectorized operations instead of loops whenever possible.
- Always monitor computational complexity in your code.
Memory Usage
Efficient memory management is crucial. Python’s memory handling may differ from MATLAB’s. Here are strategies to manage memory:
- Use data types that fit your needs; choose `float32` over `float64` when precision allows.
- Regularly clear unused variables to free up memory.
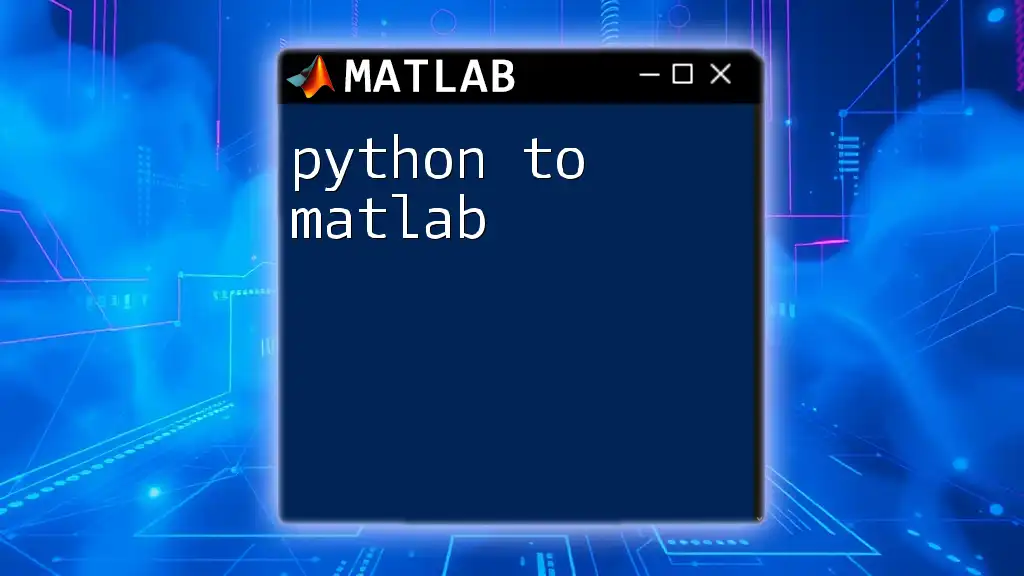
Practical Examples and Use Cases
Simple Data Analysis
Imagine you need to analyze a dataset. Using a Python MATLAB emulator, you can create a basic script that imports libraries, loads data, performs operations, and visualizes results.
For instance, consider the following steps:
- Import the necessary libraries.
- Load your data into Python from a CSV file.
- Perform data manipulations (e.g., computing statistics).
- Use Matplotlib to visualize the data.
Utilizing a Python MATLAB emulator can help streamline this process, making it enjoyable and efficient.
Advanced Simulation Techniques
For those interested in advanced techniques, emulators can facilitate complex simulations analogous to what you would achieve in MATLAB. For example, implementing numerical simulations for differential equations can be done effectively, allowing users to harness MATLAB's numerical prowess in a Python environment.
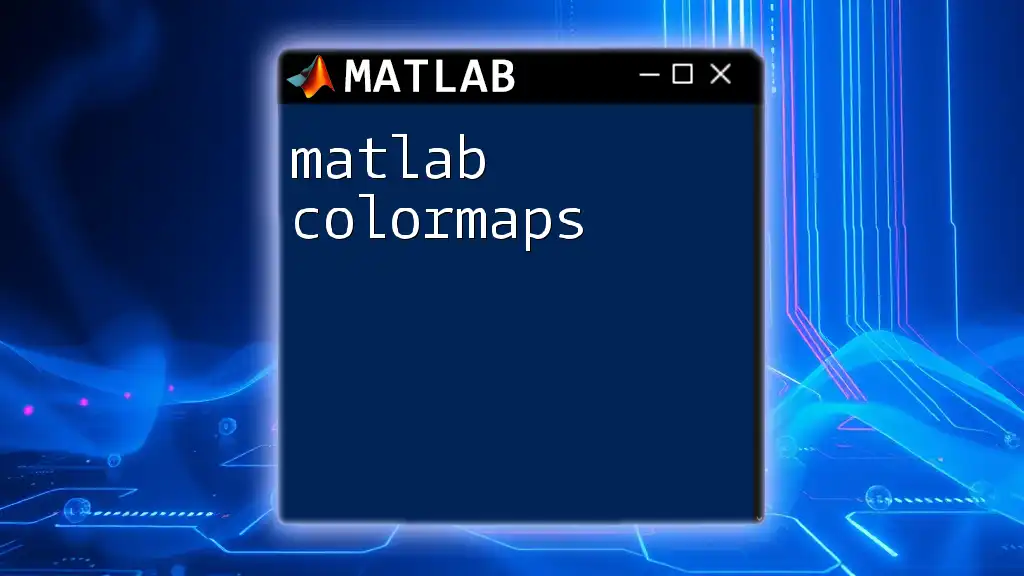
Troubleshooting Common Issues
Connection Problems
Common connectivity issues may arise between Python and MATLAB. Here are solutions to address them:
- Ensure the MATLAB executable is in your system path.
- Restart both Python and MATLAB, attempting to reconnect.
Execution Errors
Errors in execution might stem from:
- Non-existent MATLAB functions or methods.
- Data type mismatches when passing parameters between Python and MATLAB.
Regularly check the compatibility of functions when working with an emulator to avoid such issues.
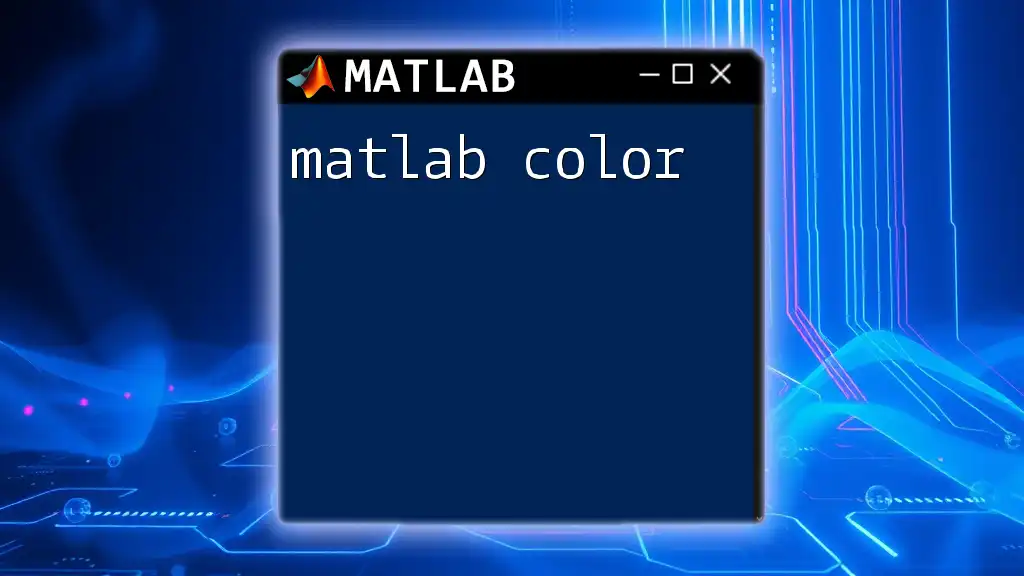
Conclusion
Utilizing a Python MATLAB emulator opens a world of possibilities for users transitioning between MATLAB and Python. The flexibility and power of Python, combined with its vast libraries, make it a strong candidate for anyone looking to recreate a MATLAB-like experience. As you explore these tools, you will not only bolster your programming skills but also enhance your efficiency in scientific computing.
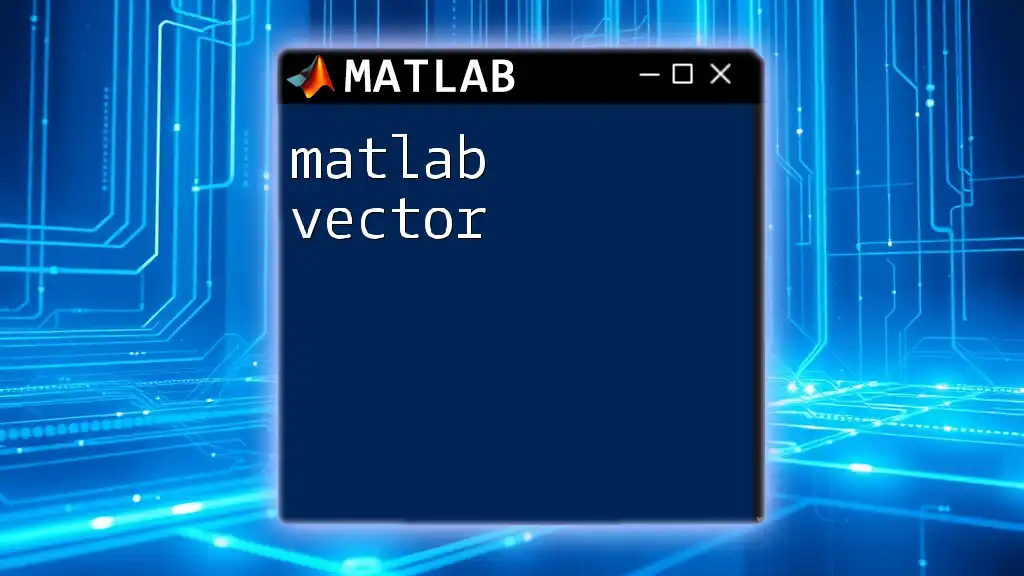
Resources for Further Learning
For those eager to dive deeper into Python and MATLAB integration, consider exploring various online courses, comprehensive textbooks, and numerous tutorials available across platforms. Engaging with these resources will enrich your learning experience.
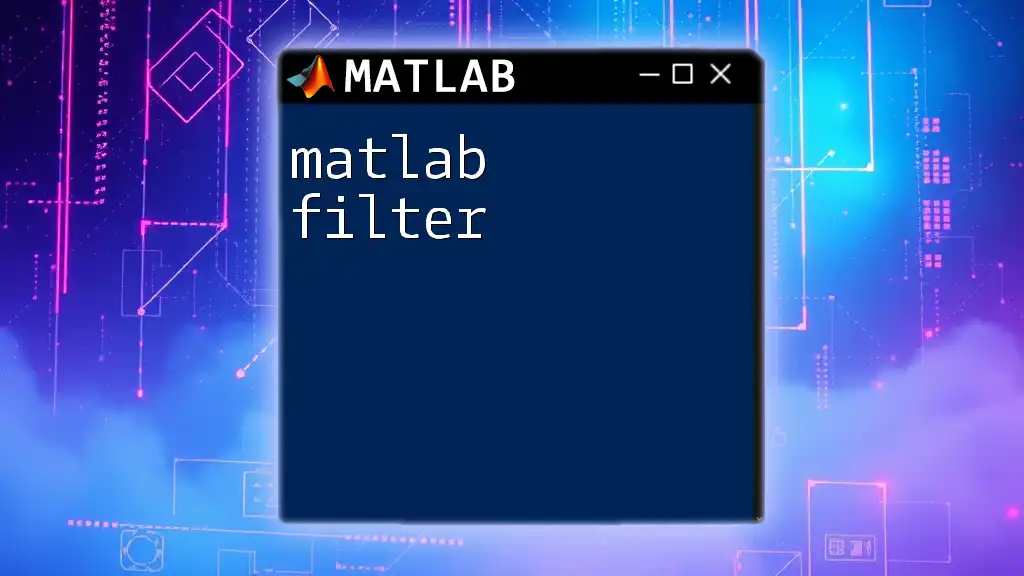
Call to Action
Are you excited to learn more about using a Python MATLAB emulator? Subscribe to our platform for insightful tutorials on MATLAB commands and discover how to quickly implement them in Python. Your programming journey awaits!