The `reshape` function in MATLAB is used to change the size of an existing array without changing its data, allowing you to rearrange elements into a different matrix structure.
A = 1:12; % Create a 1x12 array
B = reshape(A, [3, 4]); % Reshape it into a 3x4 matrix
Understanding the `reshape` Function
What is `reshape`?
The `reshape` function in MATLAB is a powerful tool used for changing the dimensions of an array without altering its data. It allows users to reorganize the way data is structured, giving flexibility in handling and visualizing numerical data.
Syntax of `reshape`
The basic syntax for the `reshape` function is as follows:
B = reshape(A, new_dim1, new_dim2, ...)
- A: This is the input array you want to reshape.
- new_dim1, new_dim2, ...: These parameters specify the desired dimensions of the output array. It is crucial to remember that the total number of elements must be the same before and after reshaping.
Key Points to Consider
When using `reshape` in MATLAB, ensure that the total number of elements in the original array matches the total number you are trying to create. For example, if you have an array with 12 elements, you can reshape it into any dimension combination that also totals 12, such as (3, 4), (2, 6), or (1, 12).
MATLAB uses row-major order for reshaping, meaning that it fills the array in row-wise fashion. Additionally, the `reshape` function can be used with various data types, including vectors, matrices, and multidimensional arrays, providing a versatile approach to data manipulation.
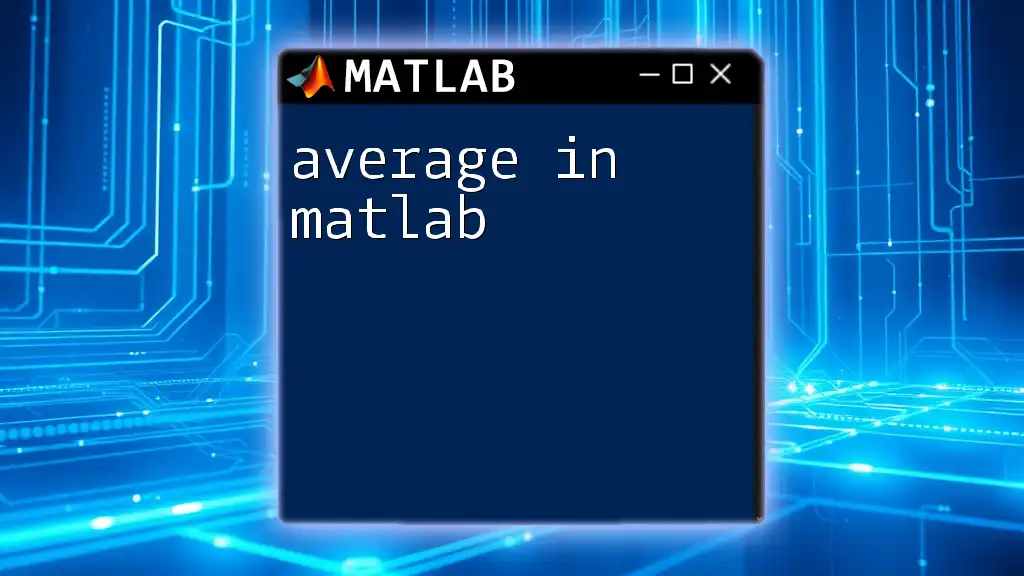
Using `reshape` in MATLAB
Reshaping a 1D Array to a 2D Array
One of the simplest applications of `reshape` is converting a one-dimensional array into a two-dimensional matrix. Consider the following code example:
A = 1:12; % Create a 1D array
B = reshape(A, [3, 4]); % Reshape it into a 3x4 matrix
disp(B);
Explanation: In this example, we start with a one-dimensional array `A` containing the integers from 1 to 12. We reshape `A` into a 3x4 matrix `B`. The resulting output will visually appear in three rows and four columns, effectively organizing the data for easier analysis or presentation.
Reshaping a 2D Array to a 3D Array
The `reshape` function can also be utilized to transform a two-dimensional array into a three-dimensional array. Here’s an example:
C = [1, 2; 3, 4; 5, 6]; % 2D array
D = reshape(C, [2, 3, 1]); % Reshape into 2x3x1
disp(D);
Walkthrough: Here, we have a 2D array `C` with 3 rows and 2 columns. After reshaping, `D` becomes a 3D array with dimensions 2x3x1, effectively stacking rows into new planes. This capability is crucial in applications where depth or multiple layers of data representation are needed.
Invalid Reshape Attempts
It’s important to understand that incorrect usage of the `reshape` function can lead to errors. For instance, consider the following code:
E = reshape(C, [2, 4]); % This will produce an error
Explanation: In this instance, trying to reshape the array `C` into dimensions that do not match the total number of elements will result in an error. MATLAB requires that the specified dimensions total the same number of elements as the original array, reinforcing the concept of dimensional integrity when reshaping data.
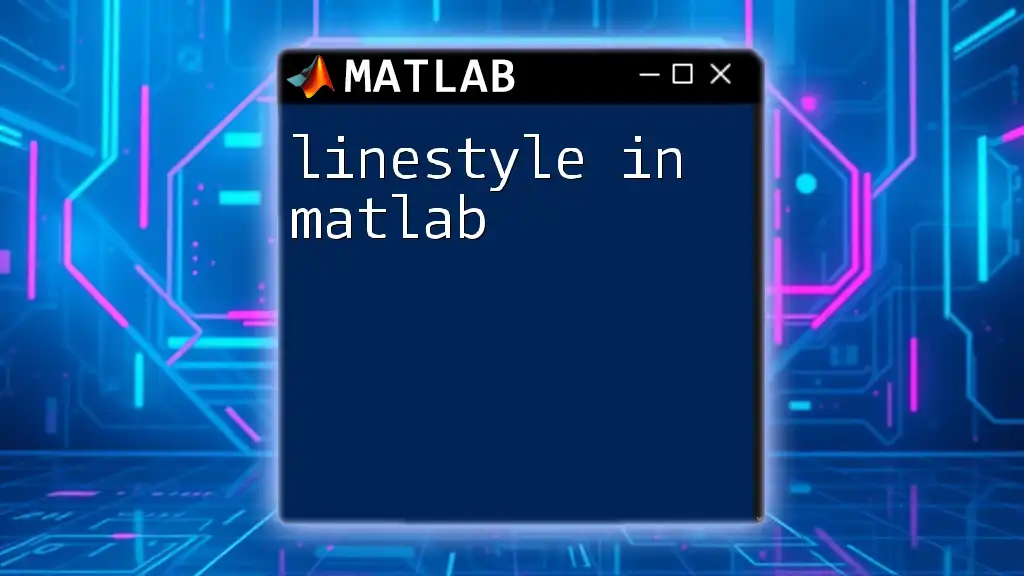
Advanced Reshape Scenarios
Reshaping with Different Dimensional Arrangements
Expanding on the capabilities of `reshape`, users can manipulate higher dimensions effectively. Here’s how to reshape a 3D array:
F = rand(2, 2, 2);
G = reshape(F, [4, 2]);
disp(G);
Discussion: In this example, we create a random 3D array `F`. Reshaping it into dimensions of 4 x 2 with output `G` allows the conversion of multi-level data into a more manageable format. This intermediate representation is ideal for data analysis or creating visualizations.
Using `reshape` with Multidimensional Arrays
Using `reshape` with multidimensional arrays is another scenario that underscores its versatility. Consider the following example:
H = rand(4, 4, 4);
I = reshape(H, [16, 4]);
disp(I);
Explanation: Here, we have a 4x4x4 array `H` filled with random numbers, which we reshape into a 16x4 array `I`. The result is a matrix where each set of 4 values originates from the original 3D array, demonstrating how data can be reorganized efficiently for various applications.
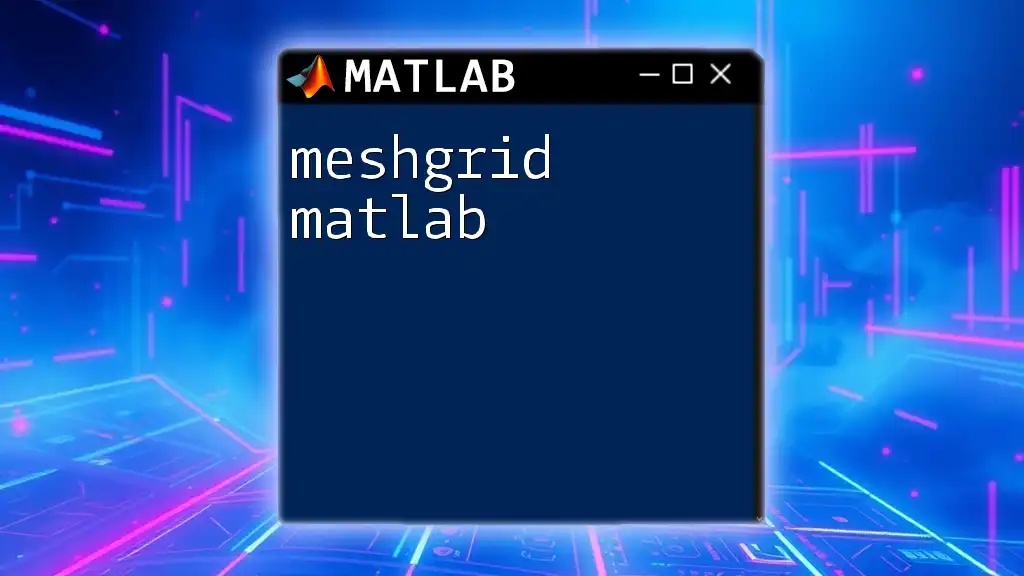
Tips for Using `reshape` Effectively
Best Practices
While working with `reshape`, it’s helpful to keep track of the dimensions of your arrays to avoid errors. Utilizing the `size` and `length` functions can aid significantly in verifying the dimensions before attempting to reshape:
-
Using `size`: This function returns the dimensions of an array, which is fundamental when determining how to reshape correctly.
-
Using `length`: Useful for obtaining the number of elements in the largest dimension of an array.
Common Mistakes to Avoid
When using `reshape`, some common pitfalls include:
- Ignoring the requirement for equal total components across dimensions.
- Misinterpreting the output shape and order of data, leading to incorrect assumptions about the data arrangement.
To minimize errors, always double-check the results of your reshaping operations to confirm they match your expectations.
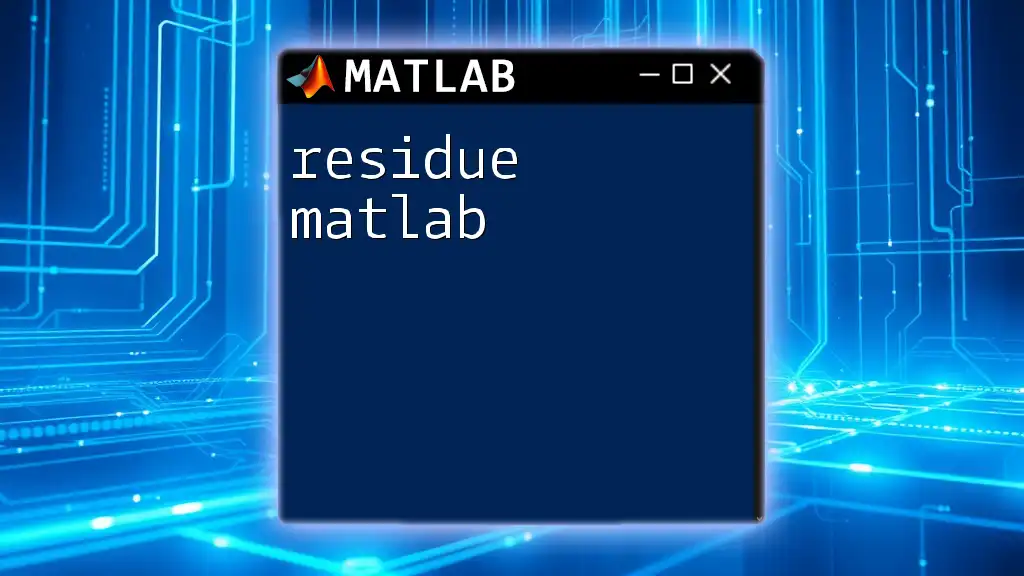
Conclusion
The `reshape` function in MATLAB is an essential tool for data manipulation that allows users to transform arrays into new configurations without losing their underlying values. Mastering this function enhances your ability to analyze, visualize, and manipulate data effectively.
We encourage you to experiment with `reshape` using various arrays to strengthen your understanding. By practicing reshaping techniques, you will discover a new level of proficiency in working with numerical data in MATLAB.
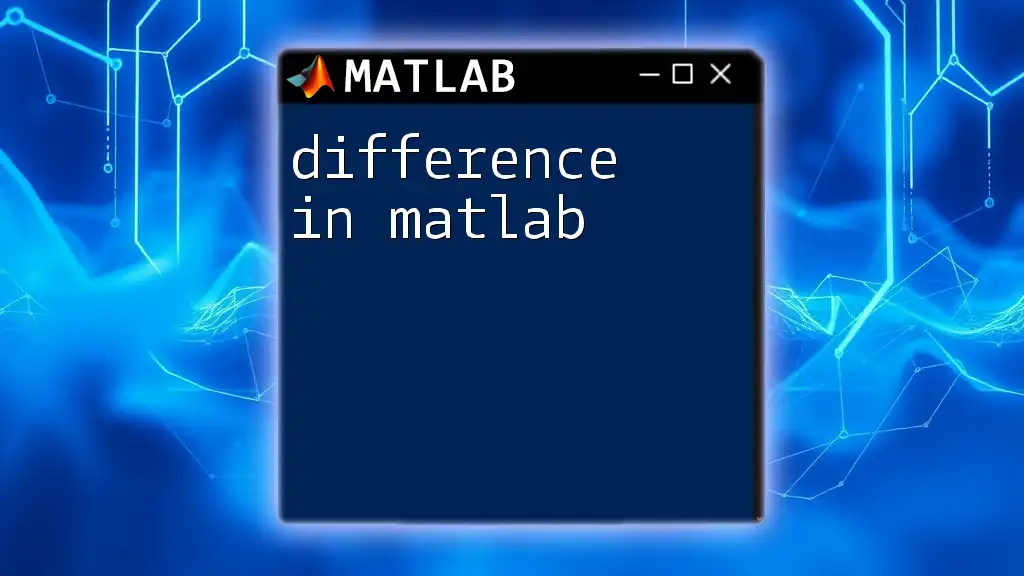
Additional Resources
MATLAB Documentation
For more in-depth learning and examples, refer to the [official MATLAB documentation on `reshape`](https://www.mathworks.com/help/matlab/ref/reshape.html). This resource offers comprehensive information on usage and best practices.
Community and Forums
Participating in MATLAB user communities and forums can provide additional insights and assistance. Engaging with fellow users will not only enhance your skills but also allow you to exchange ideas and methodologies regarding array manipulation techniques.
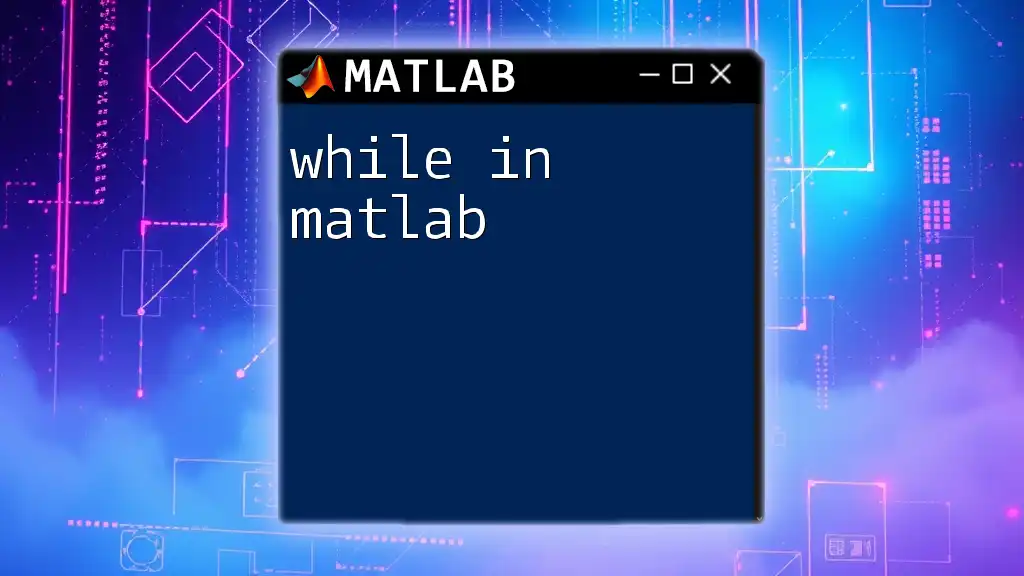
Call to Action
Now that you have a solid understanding of how to use `reshape in matlab`, we invite you to apply your knowledge. Try reshaping different data structures and share your experiences, questions, or discoveries about the function. Engage with the MATLAB community to further your learning journey!