In MATLAB, the `while` loop repeatedly executes a block of code as long as a specified condition remains true. Here's a simple example demonstrating a `while` loop that counts from 1 to 5:
count = 1;
while count <= 5
disp(count);
count = count + 1;
end
Understanding the While Loop in MATLAB
What is a While Loop?
A while loop is a fundamental control flow statement in MATLAB that allows you to execute a block of code repeatedly as long as a specified condition remains true. This dynamic capability makes while loops essential for tasks that require iterative processes until certain criteria are met. While loops are often used when the number of iterations is not known beforehand.
Structure of a While Loop
The basic syntax for a while loop in MATLAB is as follows:
while condition
% code to execute
end
Each while loop consists of a condition that must evaluate to true for the enclosed code block to execute. The loop continues to run until this condition becomes false, at which point control exits the loop and continues with the subsequent lines of code.
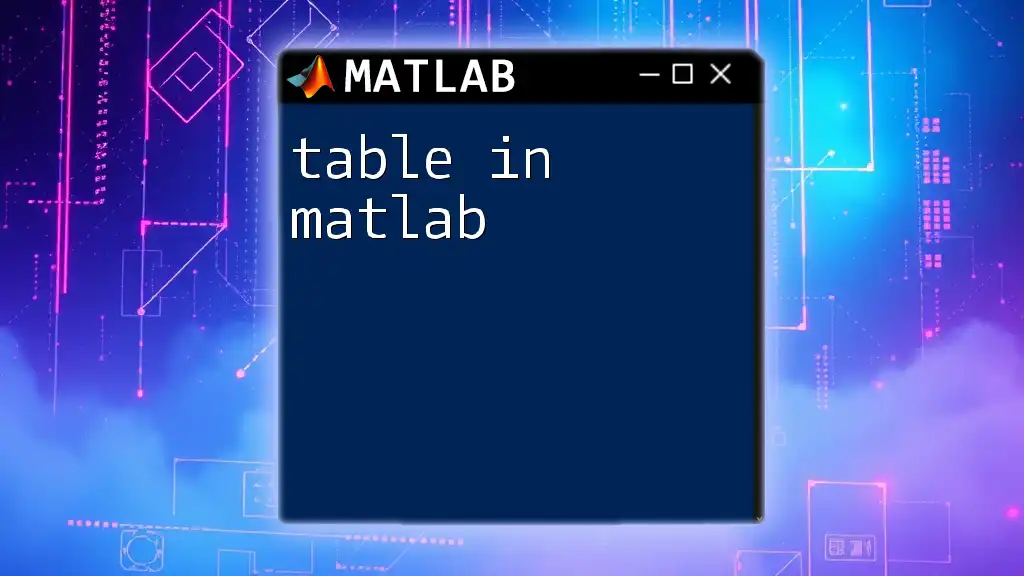
Implementing the While Loop in MATLAB
Setting Up Your Environment
Before diving into coding with while loops, it's important to familiarize yourself with the MATLAB environment. The MATLAB workspace features a command window where you can run scripts and commands interactively, and an editor where you can write and save your MATLAB scripts.
Basic Examples
Example 1: Simple Countdown
To illustrate how a while loop operates, consider a simple countdown from 5. The following code snippet demonstrates this functionality:
count = 5;
while count > 0
disp(count)
count = count - 1;
end
In this example, the variable `count` is initialized to 5. The loop's condition checks whether `count` is greater than 0. Each time the loop executes, the current value of `count` is displayed, and then it is decremented by 1. When `count` reaches 0, the condition evaluates to false, and the loop terminates.
Example 2: User Input Validation
Another practical use of a while loop is to validate user input. The following code ensures that a user provides a non-empty string as input:
userInput = '';
while isempty(userInput)
userInput = input('Please enter a non-empty string: ', 's');
end
disp(['You entered: ', userInput]);
Here, the loop continues to prompt the user for input until they enter a non-empty string. The `isempty` function checks if the variable `userInput` is empty, and if it is, the program repeats the request for input.
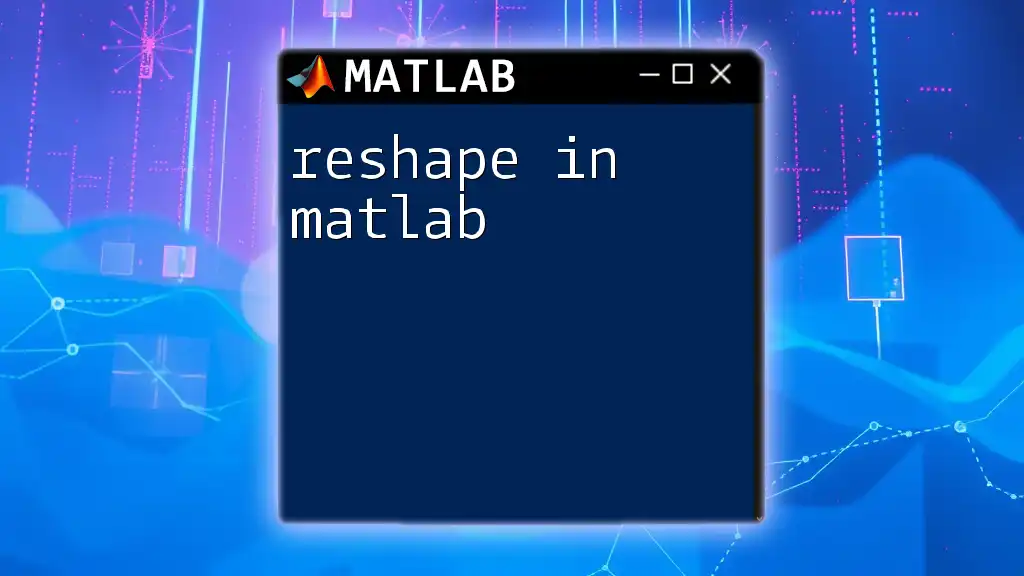
Advanced While Loop Concepts
Nested While Loops
Nested while loops allow you to place one while loop inside another. This is useful for situations like iterating over multidimensional data. Consider the following code snippet that demonstrates how nested loops can operate:
i = 1;
while i <= 3
j = 1;
while j <= 2
fprintf('i: %d, j: %d\n', i, j);
j = j + 1;
end
i = i + 1;
end
In this example, the outer loop iterates with the variable `i` ranging from 1 to 3, while the inner loop iterates with `j` ranging from 1 to 2 for each value of `i`. This generates a series of outputs that reflect the values of both `i` and `j`.
Breaking Out of a While Loop
In certain cases, you might want to exit a loop prematurely. You can achieve this with the break statement. For instance:
count = 10;
while count > 0
if count == 5
break;
end
disp(count);
count = count - 1;
end
In this example, the loop counts down from 10, but when `count` equals 5, the `break` statement causes the loop to exit, and the subsequent numbers are not displayed.
Using Continue in While Loops
The continue statement is another tool you can employ within while loops. It allows you to skip the current iteration and jump to the next one. Here’s an example:
count = 0;
while count < 10
count = count + 1;
if mod(count, 2) == 0
continue;
end
disp(count);
end
In this case, the while loop increments `count` and checks if it’s an even number. If it is, the loop skips displaying that number, resulting in only odd numbers being printed to the console.
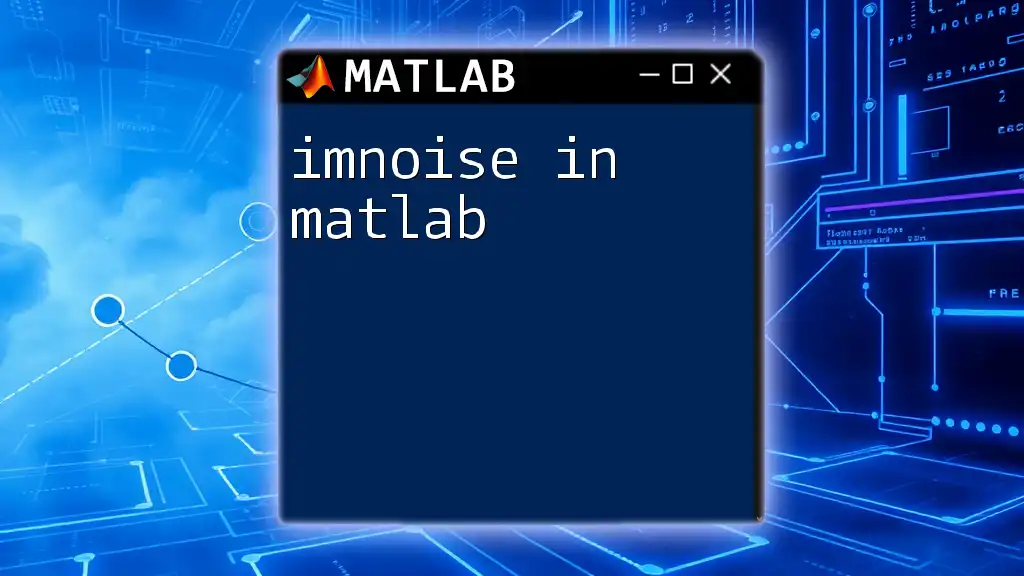
Common Mistakes and Debugging While Loops
Infinite Loops
One common error when using while loops is creating an infinite loop. This often occurs when the loop’s termination condition is never met. For instance:
i = 1;
while i <= 10
disp(i);
% Missing i increment leads to infinite loop!
end
In this example, since there is no code to modify `i`, the condition `i <= 10` will always evaluate to true, resulting in an infinite loop. To prevent such issues, ensure your loop condition and any logic that modifies it are correctly implemented.
Debugging Techniques
When dealing with complex while loops, debugging can become necessary. A simple yet effective approach is to use breakpoints within MATLAB's editor to pause execution at certain points. This allows you to inspect variable values and understand the flow of the loop. Additionally, using the `disp()` function to display variable states throughout the loop can help you track down logical errors.
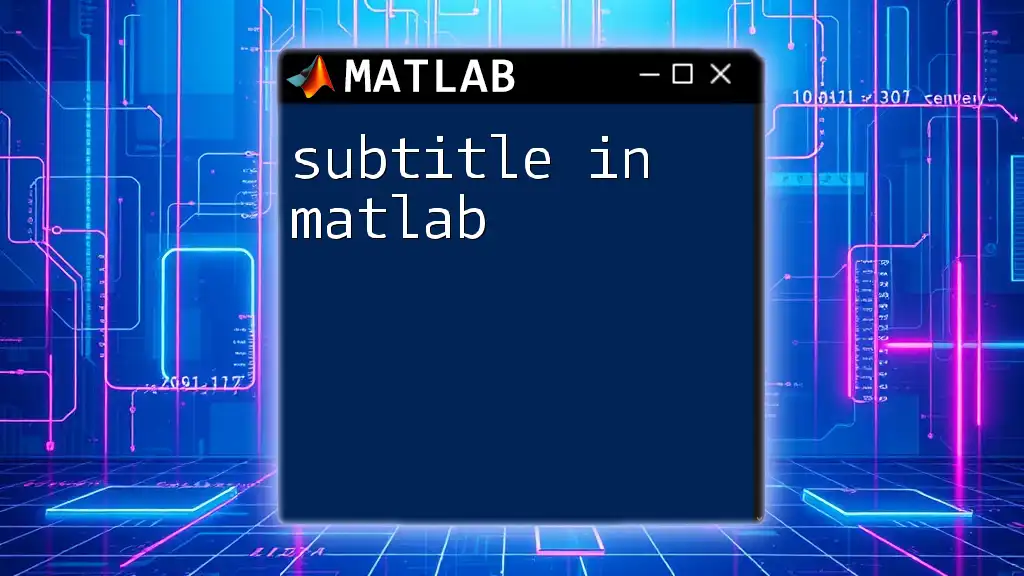
Best Practices for Using While Loops
Performance Considerations
When working with while loops, it's essential to consider performance. While loops are versatile, for loops are often better suited for iterating a known number of times. Always assess whether a while loop is the best choice for your specific situation.
Code Readability
Finally, remember to prioritize code readability. Commenting is crucial in complex while loops to clarify the intent behind your logic. Aim for simplicity in your coding structure so that you or others can easily understand the workflow.
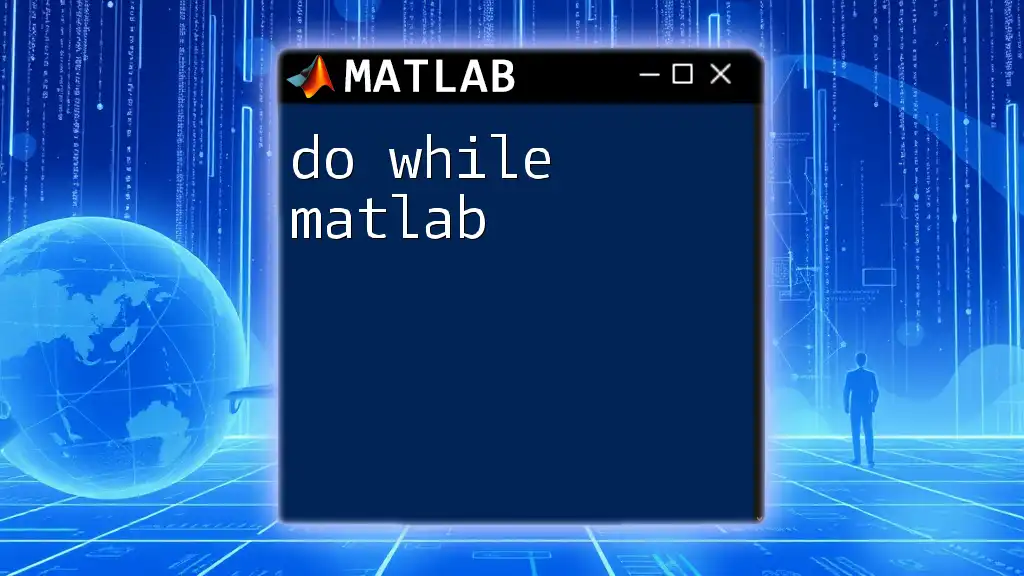
Conclusion
Understanding the intricacies of while loops in MATLAB opens up a range of possibilities for controlling program flow and performing iterative tasks. Through practice and attention to best practices, you'll master the use of while loops, enhancing your programming skills and ability to produce efficient MATLAB code. Continue experimenting with the examples provided, and don’t hesitate to seek assistance or ask questions within the community. Happy coding!