The `rand` function in MATLAB generates uniformly distributed random numbers in the range (0,1), with the ability to specify the size of the output array.
% Generate a 3x3 matrix of random numbers
randomMatrix = rand(3, 3);
Understanding `rand` Function in MATLAB
What is `rand`?
The `rand` function in MATLAB is a powerful tool designed to generate uniformly distributed random numbers. These numbers are crucial for simulations, statistical modeling, and a wide array of engineering applications. By default, `rand` produces values in the range of 0 to 1, making it ideal for functions like random sampling and probabilistic modeling.
Syntax of `rand`
Basic Syntax
The simplest invocation of the `rand` function is through the command `rand()`, which returns a single random number. For example:
r = rand();
In this case, `r` will contain a random number between 0 and 1.
Generating Arrays with `rand`
A more powerful use of the `rand` function allows users to specify the size of the output array. This is done by passing dimensions as arguments. For instance, to generate a 2-by-3 matrix of random numbers:
r_array = rand(2, 3);
This code results in a 2x3 array where each element is a random number uniformly distributed in the range of 0 to 1.
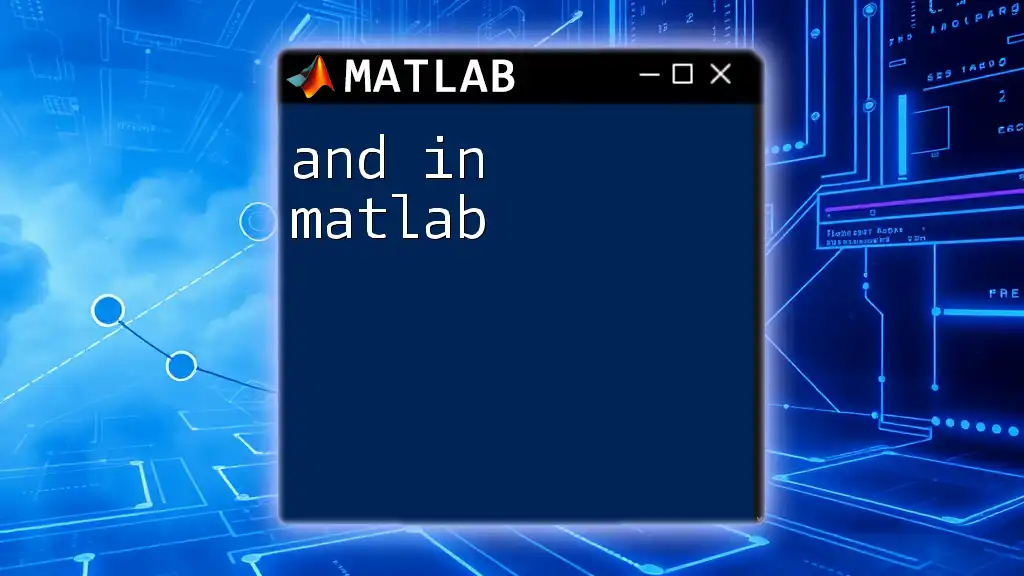
Key Features of `rand`
Uniform Distribution
Understanding Uniform Distribution
The random numbers generated by `rand` follow a uniform distribution, meaning that every number in the range from 0 to 1 has an equal chance of being selected. This property is essential for various applications, including simulations that require unbiased random selection.
Handling Different Dimensions
Creating Vectors and Matrices
MATLAB's `rand` makes it easy to generate different forms of random numbers, including row vectors, column vectors, and matrices. Here are some examples:
- Row Vector:
r_vector = rand(1, 5); % Generates a 1-by-5 array
- Column Vector:
r_column = rand(5, 1); % Generates a 5-by-1 array
These distinct formats allow for flexibility in data representation and manipulation.
Random Seed Control
Setting the Random Seed
For many applications, it is crucial to ensure reproducibility in random number generation. MATLAB offers the `rng` function to set the initial seed for the random number generator. This means you can produce the same sequence of random numbers across different runs. For instance:
rng(1); % Setting the seed for reproducibility
random_numbers = rand(1, 5);
By setting the seed, users can ensure that every time this code snippet is executed, the same random numbers will be generated.
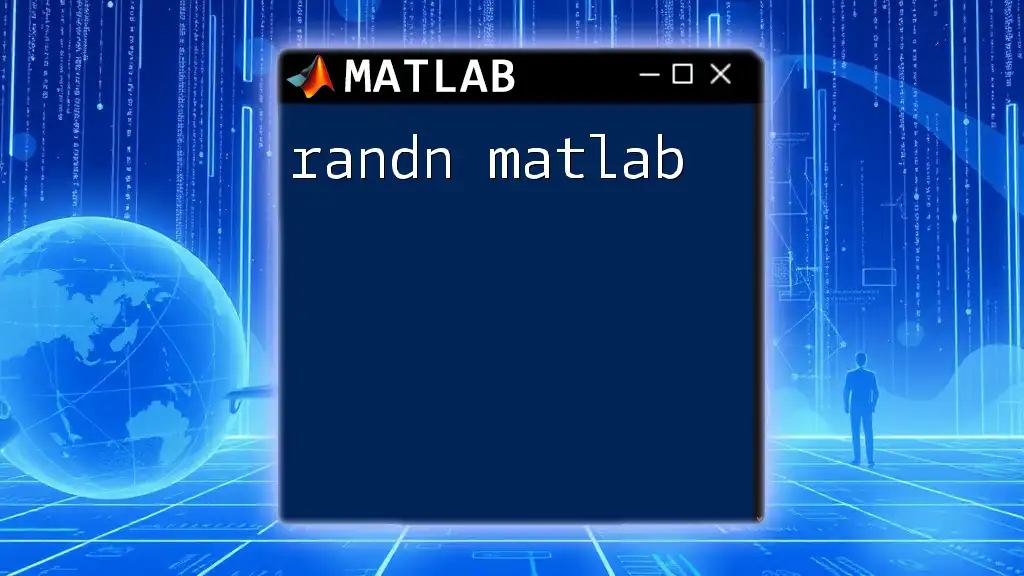
Practical Applications of `rand`
Simulations
Using `rand` in Simulations
The `rand` function is extensively used in simulations, particularly in scenarios involving Monte Carlo methods. For example, if you want to simulate a random walk (a mathematical model used in various fields such as physics and finance), you can do it as follows:
steps = 100; % Number of steps
walk = cumsum(rand(1, steps) * 2 - 1); % Random walk equation
plot(walk);
In this example, the random walk is generated by taking the cumulative sum of random movements (either left or right) derived from `rand`.
Random Sampling
Generating Random Samples
The `rand` function offers an efficient way to extract random samples from datasets. Say you have a dataset of numeric values, and you want to select a random sample. You can accomplish this using the following code snippet:
dataset = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
sample_indices = randperm(length(dataset), 4); % Generate 4 random indices
random_sample = dataset(sample_indices); % Select random samples
This code selects 4 unique random samples from the given dataset, showcasing the utility of the `rand` function in practical data analysis tasks.
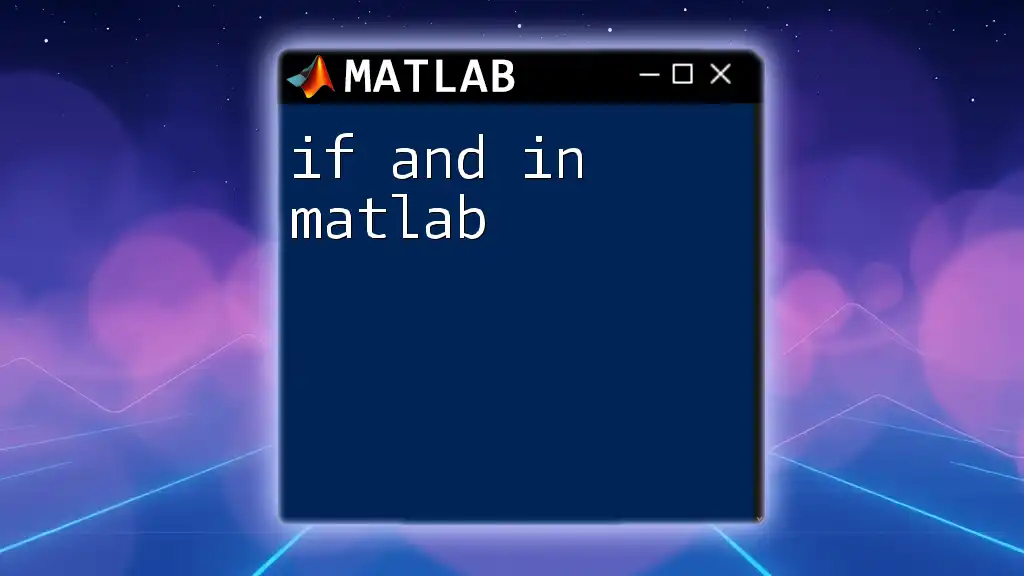
Advanced Usage of `rand`
Specialized Functions
Comparison with Other Random Functions
In addition to `rand`, MATLAB also provides other random number generation functions that cater to specific needs:
- `randi`: Generates uniformly distributed random integers. For example:
r_int = randi(10, 1, 5); % Generates 5 random integers from 1 to 10
- `randn`: Creates random numbers from a standard normal distribution (mean 0, standard deviation 1):
r_normal = randn(1, 5); % Generates a 1-by-5 array of normally distributed random numbers
- `randperm`: Generates a random permutation of integers. Useful for shuffling:
shuffled_indices = randperm(10); % Shuffles numbers 1 through 10
Performance Considerations
Performance Tips
When generating large datasets using `rand`, users should be mindful of computational efficiency. Generating random numbers can be resource-intensive; thus, it's important to leverage matrix assignments when possible. For example:
large_array = rand(1000, 1000); % Generates a large 1000x1000 array at once
This approach is more efficient than generating random numbers in a loop, as it utilizes MATLAB's optimized matrix operations.
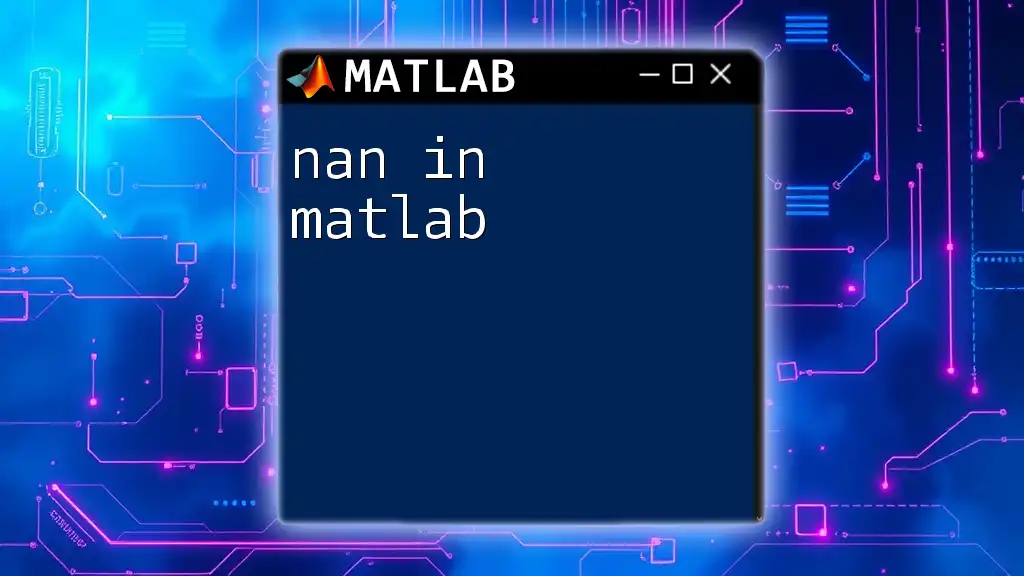
Common Mistakes and Troubleshooting
Common Errors
One of the frequent mistakes when using the `rand` function is misinterpreting how dimensions affect the output. For instance, requesting `rand(2, 'str')` instead of valid numeric dimensions may lead to errors. Always ensure that the arguments you pass to `rand` are valid.
To debug issues, consider checking the size of the output arrays or using functions like `size()` and `length()` to confirm data structures.
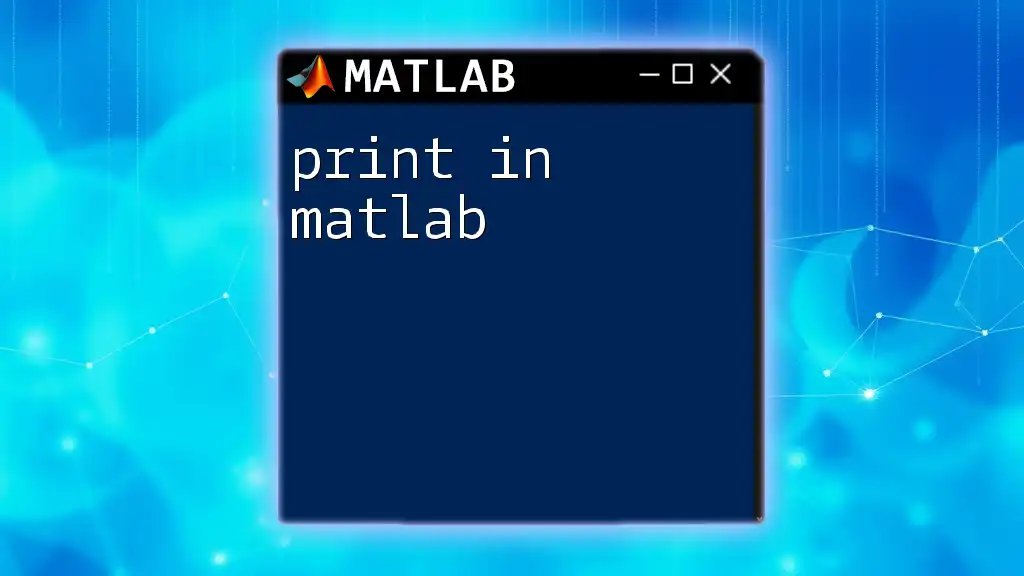
Conclusion
In summary, the `rand` function in MATLAB is a versatile tool essential for generating random numbers and performing simulations. By understanding its syntax, features, and applications, users can effectively incorporate it into their MATLAB workflows for a variety of tasks, ranging from simple random generation to complex simulations.
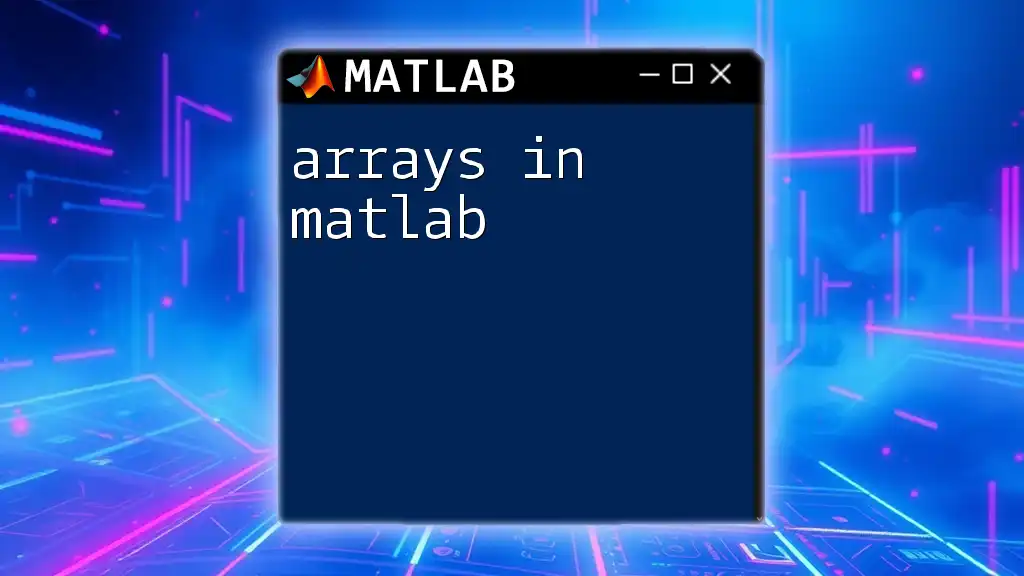
Additional Resources
For further exploration of the `rand` function and its related capabilities, consider referring to the official MATLAB documentation. Engage with online forums, MATLAB user communities, and tutorials to deepen your understanding and refine your skills.
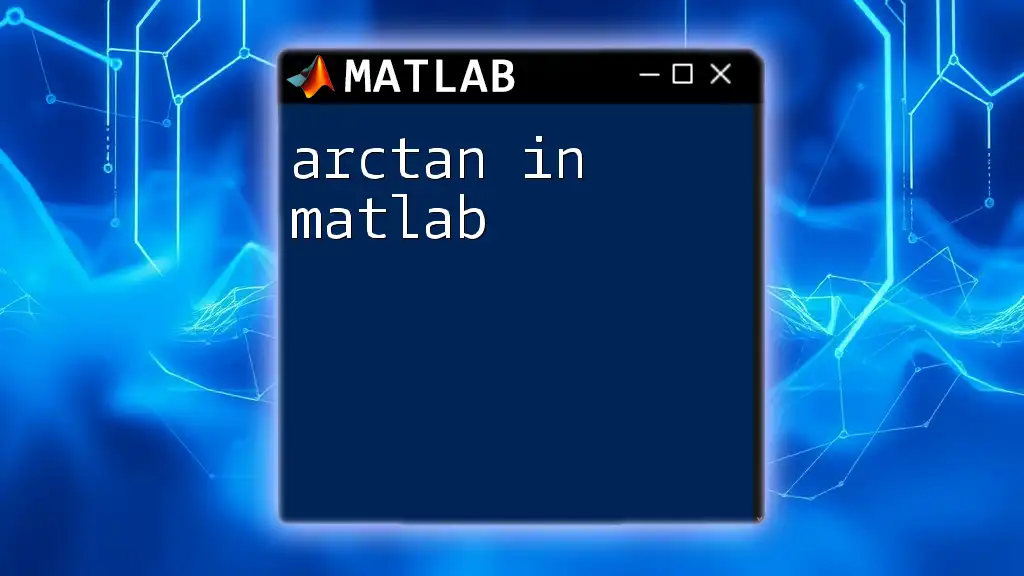
Call to Action
Now it's your turn to practice using the `rand` function in your MATLAB environment. Experiment with the examples above, create your random number scenarios, and share your experiences in the comments section below!