The `imshow` function in MATLAB is used to display an image in a figure window, facilitating easy visualization of image data.
Here's a simple example of how to use `imshow`:
% Read and display an image
img = imread('example_image.jpg'); % Load an image file
imshow(img); % Display the image
Understanding the Basics of `imshow`
What is `imshow`?
`imshow` is a powerful function in MATLAB that allows users to visualize images effectively. It serves as a vital tool for image processing and analysis, enabling easy exploration of image data. By displaying images in MATLAB, users can visually assess their content, manipulate, and analyze pixel data.
Syntax of `imshow`
The basic syntax for `imshow` is straightforward:
imshow(I)
In this syntax, `I` is the input image, which can be in various formats such as grayscale, RGB, or binary. The command is designed to take any valid image matrix and display it in a figure window. Understanding the required input type is crucial for effective use of `imshow`.
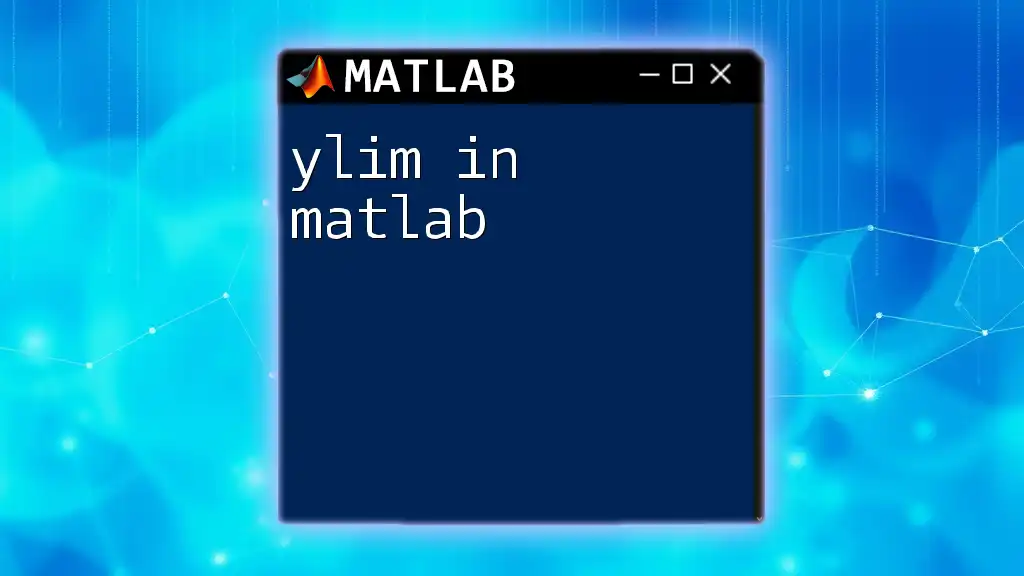
Types of Input for `imshow`
Displaying Images
Grayscale Images
Grayscale images consist of varying shades of gray, represented as single-channel matrices. Each pixel intensity ranges from 0 (black) to 255 (white). To display a grayscale image in MATLAB, you first need to read an image, convert it to grayscale (if it’s not already), and then use the `imshow` command. Here’s an example:
I = imread('image.jpg'); % Load the image
imshow(rgb2gray(I)); % Convert and display as grayscale
This command sequence reads an image file and converts it to grayscale before displaying it with `imshow`.
RGB Images
RGB images are stored as three separate channels (red, green, and blue) and represent true color images. Displaying an RGB image with `imshow` is very direct, as shown below:
I = imread('image.jpg');
imshow(I); % Display the RGB image
This command will show the image as it is, without any modifications.
Displaying Other Data Types
Binary Images
Binary images consist of only two values: 0 for black and 1 for white. They are often used in image processing for tasks like segmentation. To convert an image to binary and display it:
BW = imbinarize(I); % Convert to binary
imshow(BW); % Display the binary image
This snippet converts a grayscale image into a binary format and displays it, allowing for easy visual interpretation of segmented areas.
Image Matrices
Using Numeric Matrices
You can also display numerical matrices directly as images with `imshow`. This is useful for visualizing data matrices or any numerical representation. Here’s how you can do it:
A = rand(100,100); % Generate a random 100x100 matrix
imshow(A); % Display the matrix as an image
This command generates a random matrix and displays it in a grayscale format.
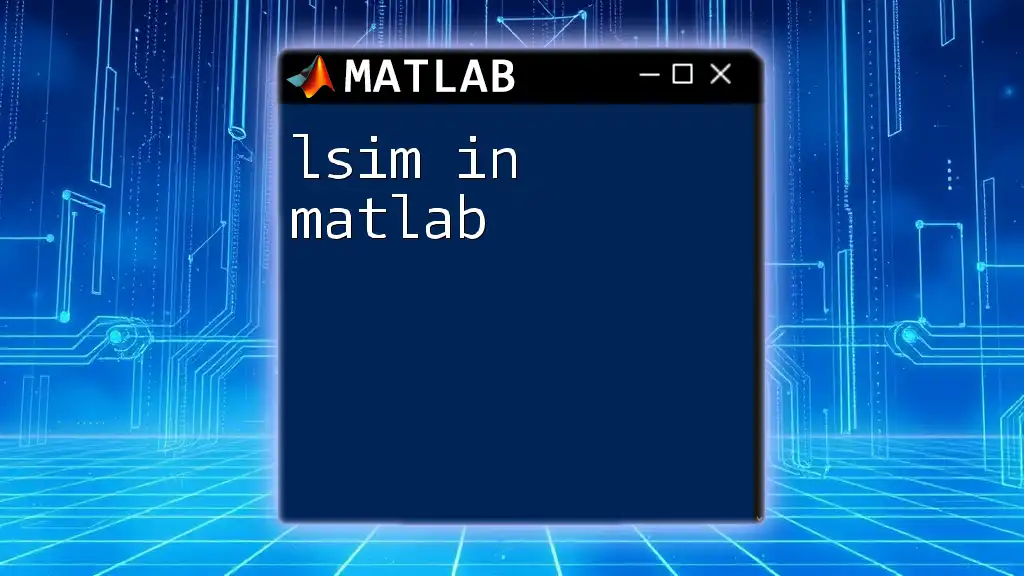
Customizing Image Display with `imshow`
Adjusting Display Range
Adjusting the display range is essential for accurately visualizing data. For instance, if you want MATLAB to automatically scale the display based on the pixel values, you can use the following syntax:
imshow(I, []);
Using an empty array `[]` tells `imshow` to use the full range of the data automatically, which can be critical in ensuring all details of the image are visible.
Utilizing Colormaps
Colormaps enhance visualization, especially for single-channel data. By applying a colormap, you can represent data meaningfully. Here’s how to use the `jet` colormap:
imshow(I);
colormap(jet); % Apply the 'jet' colormap
colorbar; % Show the colorbar
The `colormap` function alters the color representation of the displayed image, making it easier to interpret certain data characteristics.
Image Display Properties
Setting Display Properties
`imshow` offers several properties to customize how images are shown. For instance, you can set the initial magnification and border options:
imshow(I, 'InitialMagnification', 'fit');
This command ensures that the image is fitted to the figure window, enhancing visibility on lower resolution displays.
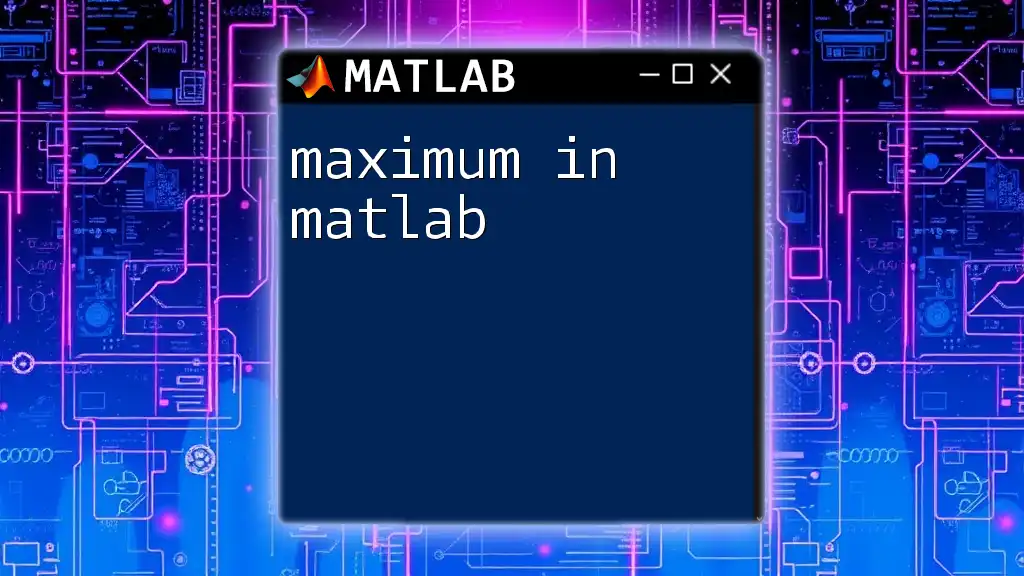
Working with Multiple Images
Displaying Multiple Images Using Subplots
When analyzing multiple images side by side, `subplot` is invaluable. By utilizing subplots, you can create a compact and informative visual comparison.
subplot(1, 2, 1); imshow(I);
subplot(1, 2, 2); imshow(rgb2gray(I));
In this example, the first subplot displays the original image, while the second shows the grayscale version, allowing for instant visual comparison.
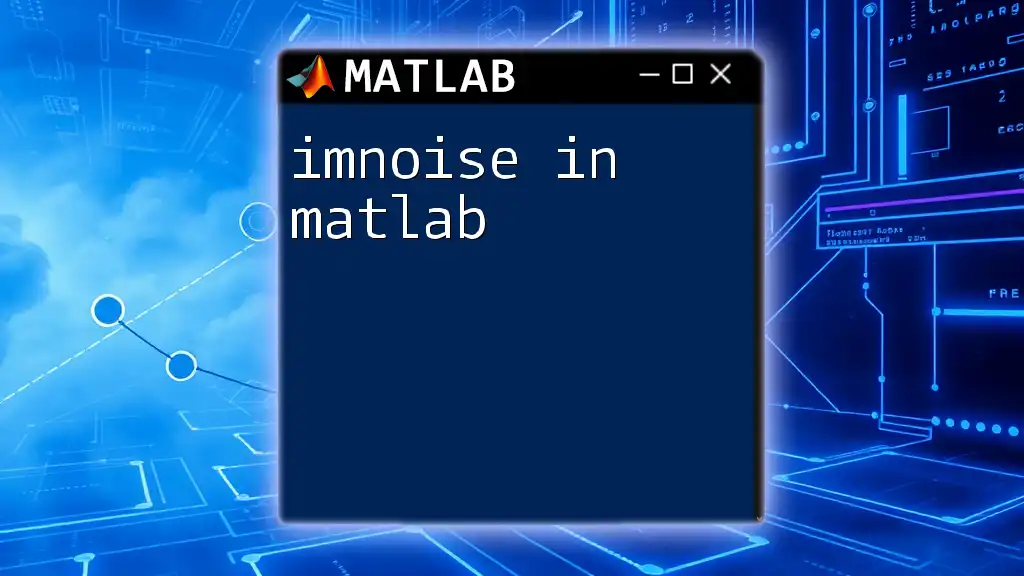
Error Handling with `imshow`
Common Errors and Solutions
Users of `imshow` may encounter several common errors, such as trying to display data types or sizes that do not meet the requirements. For instance, trying to visualize a non-image matrix or an unsupported format may yield an error. To troubleshoot, ensure your input `I` is a valid image type (2D or 3D array).
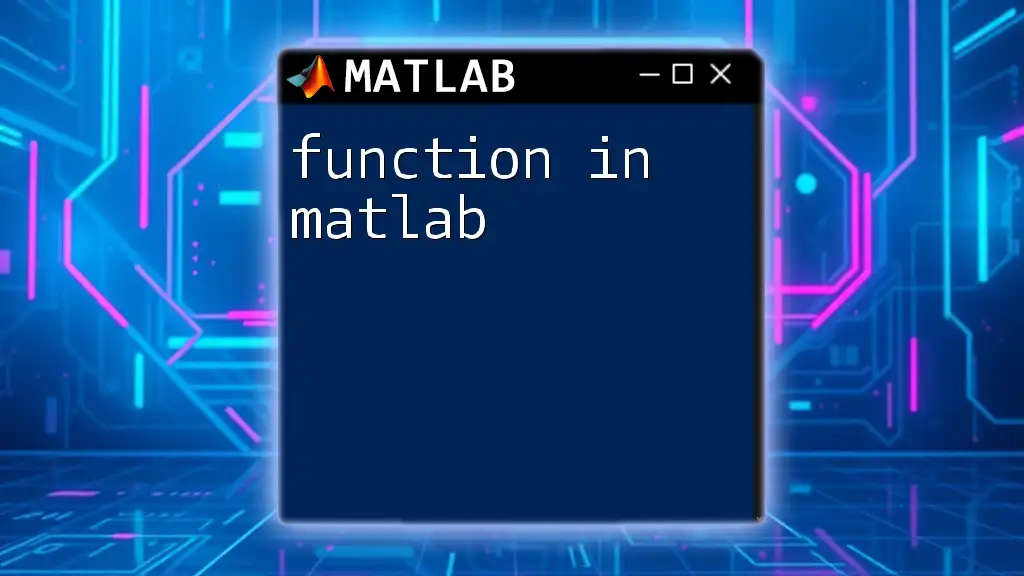
Practical Applications of `imshow`
Image Processing Techniques
`imshow` is often used in conjunction with various image processing techniques like filtering or edge detection. For instance, after applying a filter, you can use `imshow` to visualize the effect immediately:
filteredImage = imfilter(I, fspecial('gaussian'));
imshow(filteredImage); % Display the filtered image
This code applies a Gaussian filter and displays the result, allowing for analysis of smoothening effects.
Visualizing Image Analysis Results
It’s essential to visualize results effectively, especially after applying image analysis techniques such as segmentation or feature detection. For instance, if you have a mask of detected features, overlaying this mask on the original image enhances understanding. Use `imshow` to show both images together.
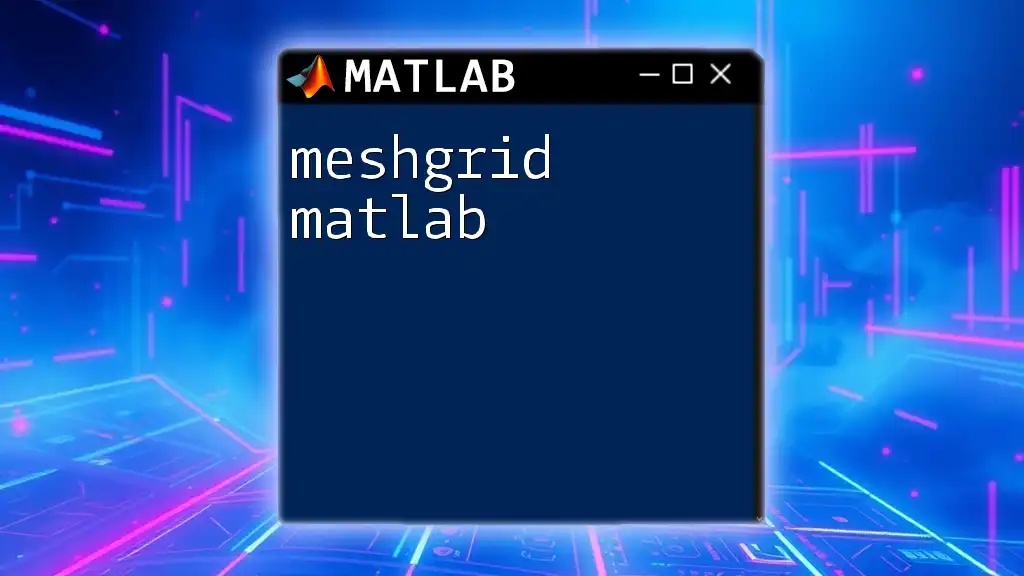
Conclusion
In summary, `imshow` is a versatile and essential function in MATLAB for image visualization. It serves as the foundation for further image processing and analysis, enabling users to gain insights from their data effectively. Mastering `imshow` will significantly enhance your image processing capabilities within MATLAB.
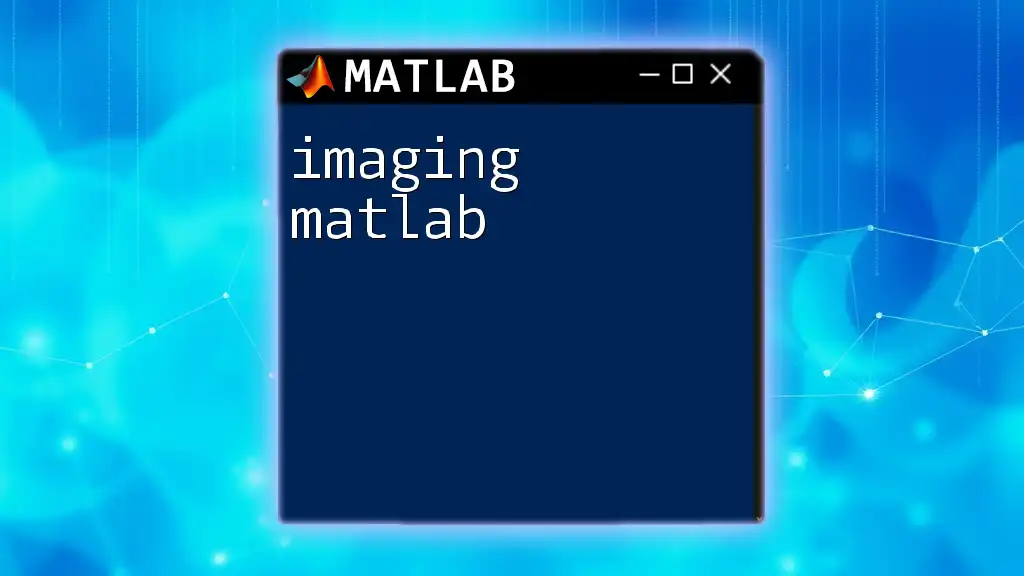
Additional Resources
For those looking to deepen their knowledge of image processing in MATLAB, consider exploring the official MATLAB documentation on `imshow`, as well as joining MATLAB online communities for tips, tutorials, and further assistance.
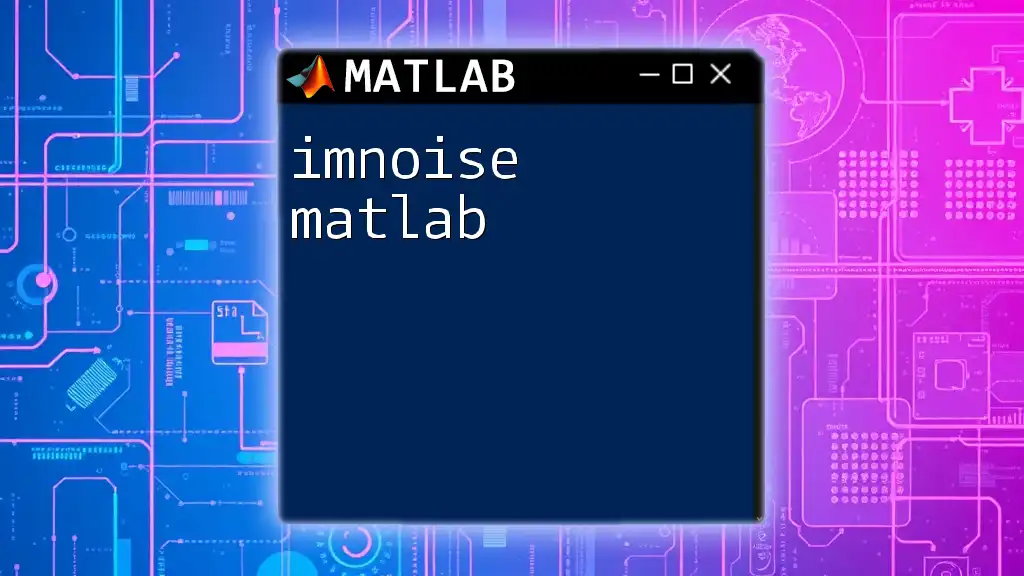
Call to Action
Feel free to follow this blog for more concise MATLAB tutorials, and don’t hesitate to share your feedback or questions in the comments section!