The `atan` function in MATLAB computes the arctangent of a given input value, returning the angle in radians corresponding to the tangent of that value.
% Calculate the arctan of a number
x = 1; % Input value
angle_radians = atan(x); % Computes arctangent
Understanding the Arctan Function
What is Arctan?
The arctangent function, often denoted as arctan or atan, is the inverse of the tangent function in trigonometry. This means that for a given value \( y \), if \( y = \tan(x) \), then \( x = \text{atan}(y) \). Arctan takes a real number input and gives an angle in radians, which is essential for converting between linear and angular measurements.
Properties of Arctan
The arctan function possesses several important properties:
- The domain of arctan is all real numbers, while its range is restricted to \( (-\frac{\pi}{2}, \frac{\pi}{2}) \).
- It is defined as an odd function, satisfying \( \text{atan}(-x) = -\text{atan}(x) \). This is significant for computational symmetry and properties in Cartesian coordinates.
- The derivative of the arctan function is \( \frac{d}{dx} \text{atan}(x) = \frac{1}{1+x^2} \), which is not only useful in calculus but also computationally relevant in optimization problems.
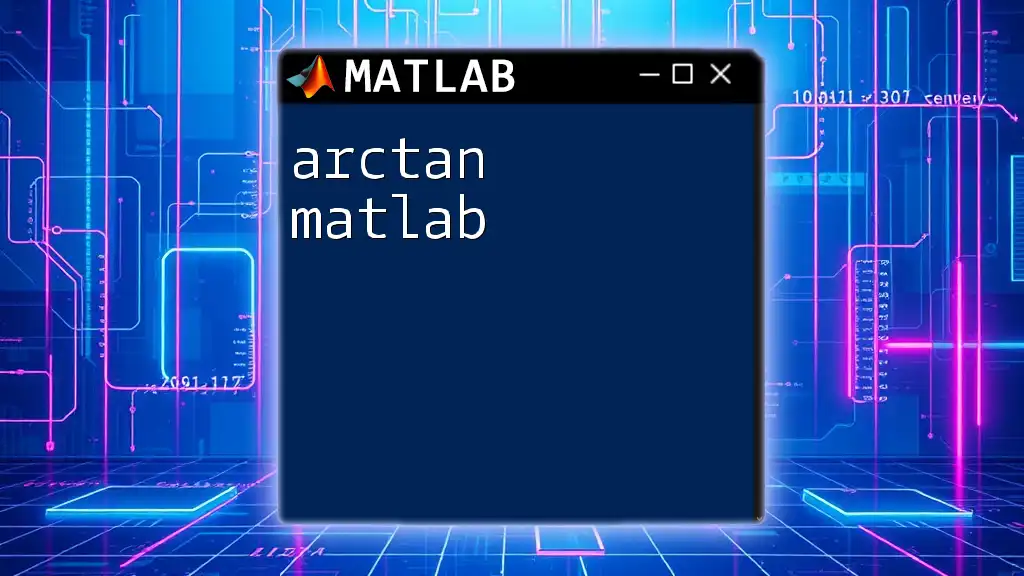
Using the Arctan Function in MATLAB
Syntax and Basics
In MATLAB, the syntax for the arctangent function is as follows:
result = atan(x);
Where `x` is the input value, and the output `result` is the angle in radians. MATLAB provides this function to streamline calculations that involve angles and distances efficiently.
Explanation of the `atan` Function
The `atan` function computes the angle whose tangent is `x`. It is important to remember that \( \text{atan}(1) \) returns \( \frac{\pi}{4} \) radians (or 45 degrees):
x = 1; % Example input
result = atan(x);
disp(result); % Displaying the output
When executed, this code will yield approximately 0.7854, which corresponds to \( \frac{\pi}{4} \).
Introduction to `atan2`
`atan2(y, x)` is a variant of the arctangent function that computes the angle of the point \( (x, y) \) relative to the x-axis, considering the signs of both inputs to determine the correct quadrant of the resulting angle. This is particularly useful in applications like navigation, robotics, and graphics where point quadrant matters.
An example usage would be as follows:
y = 1; % y-coordinate
x = 1; % x-coordinate
angle = atan2(y, x);
disp(angle); % Result in radians
Here, the output will be 0.7854 radians, again equivalent to \( \frac{\pi}{4} \).
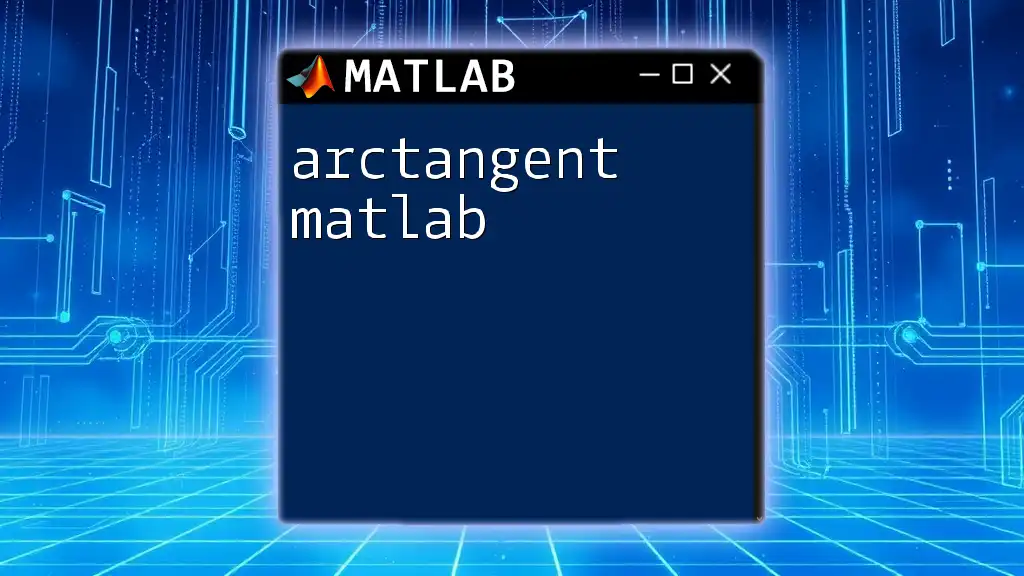
Practical Applications of Arctan in MATLAB
Use Cases in Engineering
The arctan function finds numerous applications in engineering disciplines:
- Signal Processing: To determine phase angles in wave analyses.
- Control Systems: Identifying the phase of signals in stability analysis.
- Robotics: Used in determining angles of limbs or joints.
Examples in Data Analysis
The `atan` function is also crucial in statistical data analysis. It helps in calculating angles for data points, standardizing distributions, or visualizing trends. For example, to plot the arctangent function:
% Create sample data
x = linspace(-10, 10, 100);
y = atan(x);
% Plotting
plot(x, y);
title('Arctangent Function');
xlabel('x');
ylabel('atan(x)');
grid on;
When executed, this code will generate a plot that illustrates the characteristic S-shape of the arctan function, highlighting its gradual approach towards \( -\frac{\pi}{2} \) and \( \frac{\pi}{2} \) but never reaching those extremes.
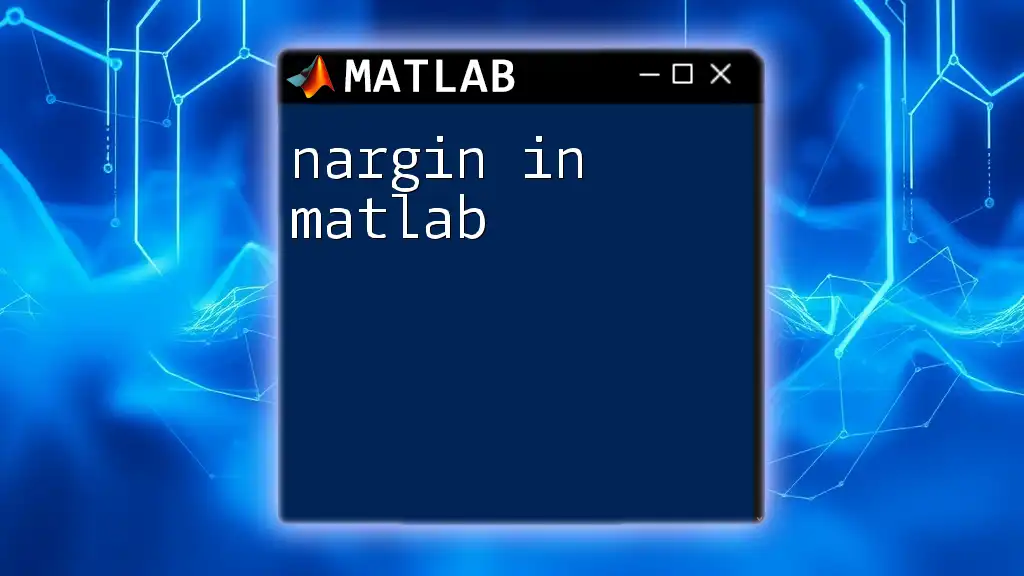
Advanced Features of Arctan in MATLAB
Handling Complex Numbers
MATLAB’s `atan` function can also handle complex numbers, offering versatility for various applications. For instance:
z = 1 + 1i; % Complex number
complex_result = atan(z);
disp(complex_result);
This code will compute the arctangent of the complex number, returning a complex angle associated with the input.
Custom Functions with Arctan
One can create custom functions that leverage `atan` to simplify complex calculations. For instance:
function angle = myAtan(y, x)
angle = atan2(y, x); % Utilizes atan2 for custom calculation
end
This function, `myAtan`, accepts two inputs (y and x) and returns the angle using the `atan2` method, thus allowing modular reusability in larger projects or scripts.
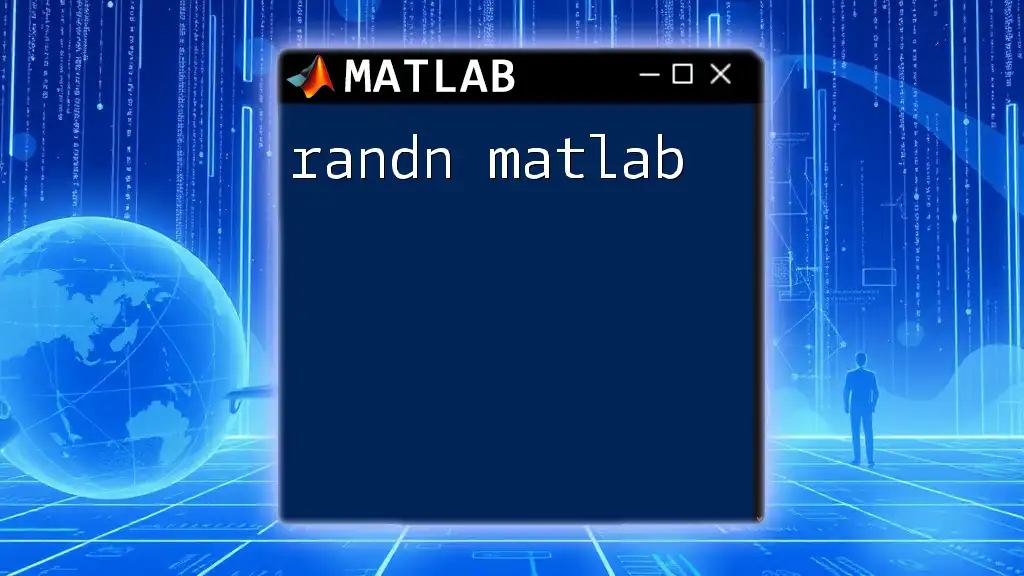
Best Practices for Using Arctan in MATLAB
Performance Considerations
When handling large datasets, utilize MATLAB’s vectorization capabilities for performance efficiency. Instead of processing elements one by one, you can leverage:
% Vectorized operation
x = linspace(-1000, 1000, 1e6);
result = atan(x); % Fast computation using vectorization
This will perform the arctan operation on an entire array of values at once, significantly speeding up calculations compared to iterative methods.
Common Pitfalls
A common mistake is misinterpreting the output of `atan` versus `atan2`. Remember:
- `atan` assumes \( -\infty < x < \infty \) and produces values from \( -\frac{\pi}{2} \) to \( \frac{\pi}{2} \).
- `atan2` considers both x and y coordinates, avoiding ambiguities in quadrant representation.
Debugging these issues often requires reevaluation of the input values to ensure accurate outputs, especially when dealing with trigonometric functions in complex algorithms.
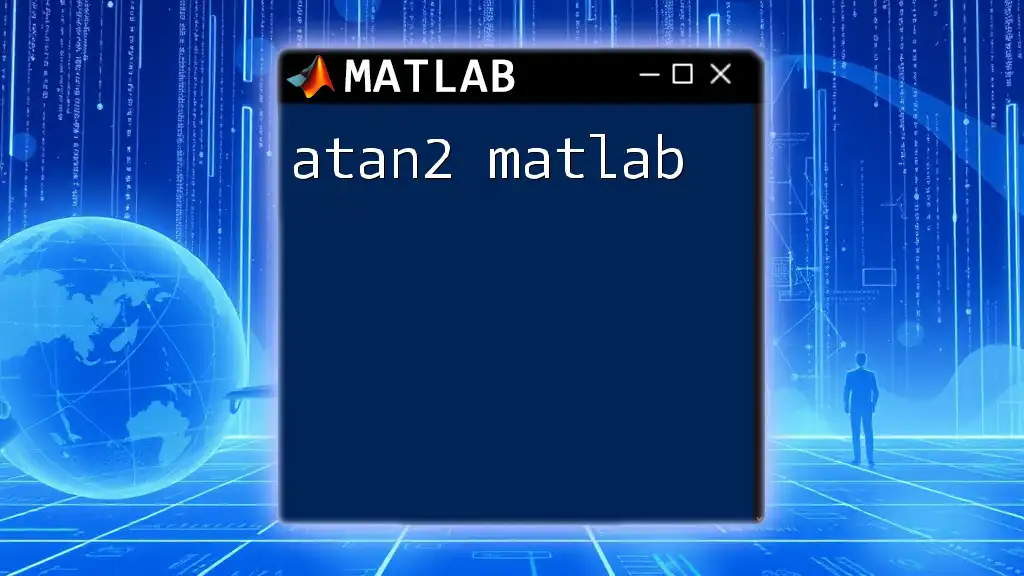
Conclusion
The arctan function in MATLAB presents a powerful tool for mathematical computations and engineering applications. It is integral for converting between linear and angular measurements and has a wide variety of practical uses. By grasping its properties, mastering its implementation, and understanding its applications, you can significantly enhance your efficiency in MATLAB. Consider further practice in these areas, and for deeper insight, join our MATLAB classes.
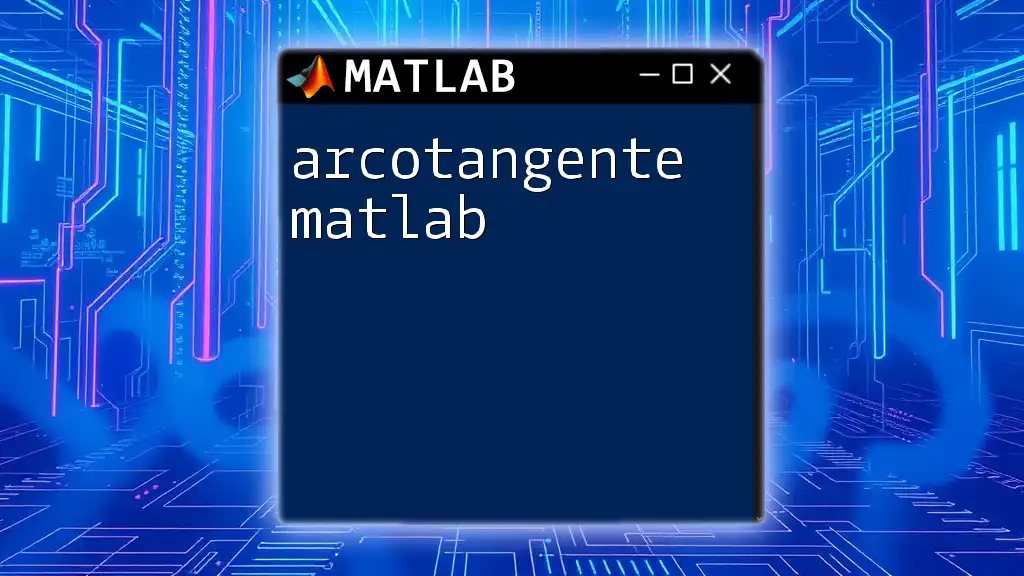
Additional Resources
For further clarification and details, you can check the official MATLAB documentation related to `atan` and `atan2`. Moreover, don’t hesitate to consult recommended mathematical textbooks or online forums for community assistance when needed.