In MATLAB, arrays are fundamental data structures that store collections of values, allowing for efficient computation and manipulation of numerical data.
Here’s a simple example of creating and accessing elements in a 1D array:
% Creating a 1D array
myArray = [1, 2, 3, 4, 5];
% Accessing the third element
thirdElement = myArray(3);
What are Arrays?
Arrays in MATLAB are fundamental data structures designed for storing collections of values. Whether you're working on mathematical computations, data analysis, or programming general applications, arrays serve as the backbone for organizing and manipulating multiple values efficiently. An array can represent a single value, a row of values, or even a collection of matrices, making it incredibly versatile.
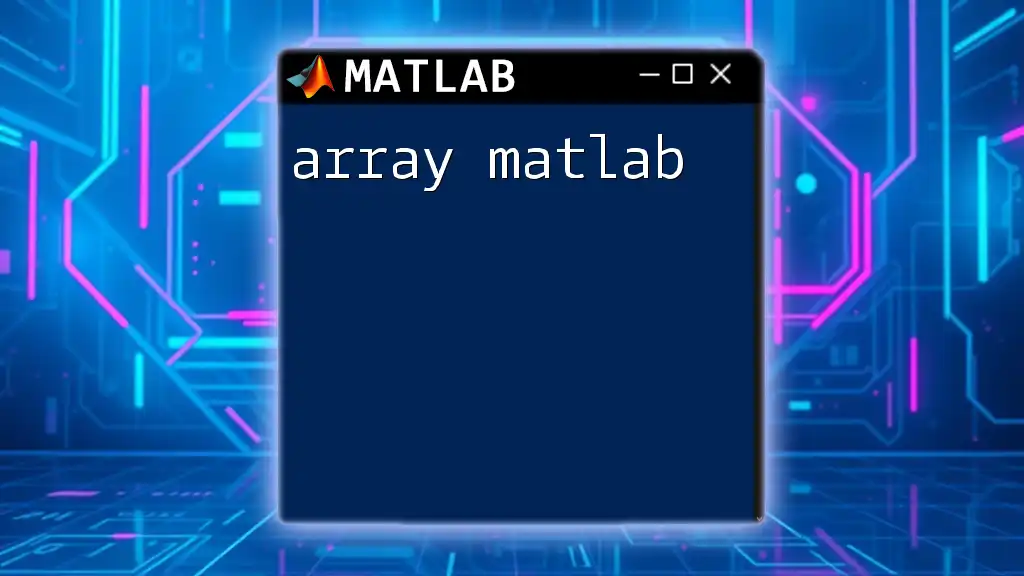
Types of Arrays
In MATLAB, arrays are categorized primarily into three types:
-
1-D arrays: These can be either row vectors or column vectors. Row vectors are created using square brackets `[]` with space or commas separating the elements, while column vectors use semicolons to separate the elements vertically.
-
2-D arrays: Represented as matrices, these are two-dimensional arrays containing rows and columns. Elements are separated by spaces or commas, with rows dictated by semicolons.
-
Multi-dimensional arrays: These extend beyond two dimensions, allowing you to work with more complex data structures using functions like `cat`.
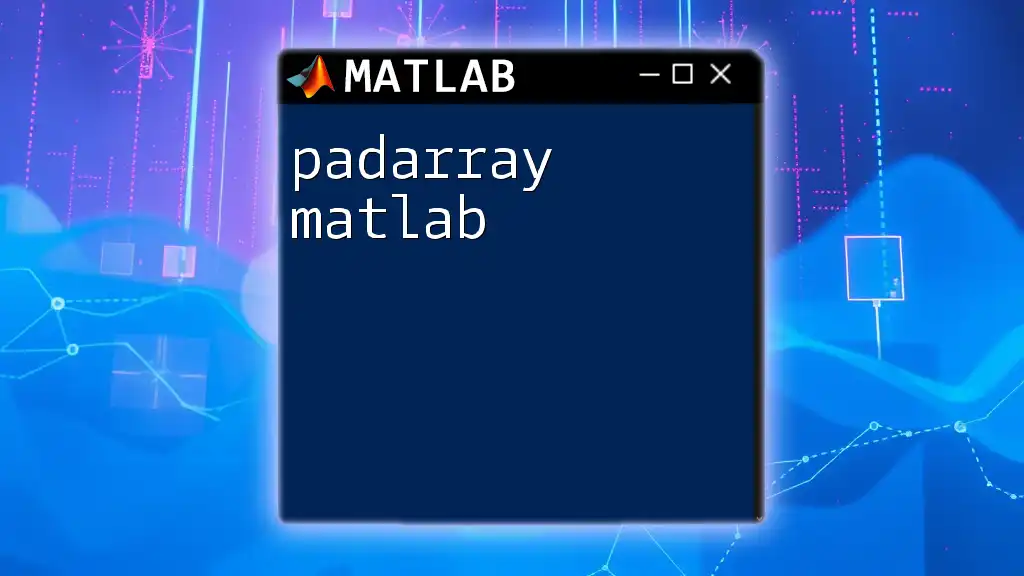
Creating Arrays
Creating 1-D Arrays
To create a 1-D array or vector in MATLAB, use square brackets:
a = [1, 2, 3, 4, 5];
In this example, `a` is a row vector. For a column vector, you'd structure it like this:
b = [1; 2; 3; 4; 5];
Creating 2-D Arrays
For 2-D arrays, which are basically matrices, you still use square brackets but include semicolons to separate rows:
B = [1, 2, 3; 4, 5, 6];
Here, `B` is a 2x3 matrix. Each row is separated by a semicolon, while elements within a row are separated by commas.
Creating Multi-Dimensional Arrays
Multi-dimensional arrays are constructed using the `cat` function for combining arrays along a specified dimension. For instance, to create a 3D array:
C = cat(3, [1, 2; 3, 4], [5, 6; 7, 8]);
In this example, `C` becomes a 3-dimensional array containing two matrices, making it suitable for more intricate data manipulations.
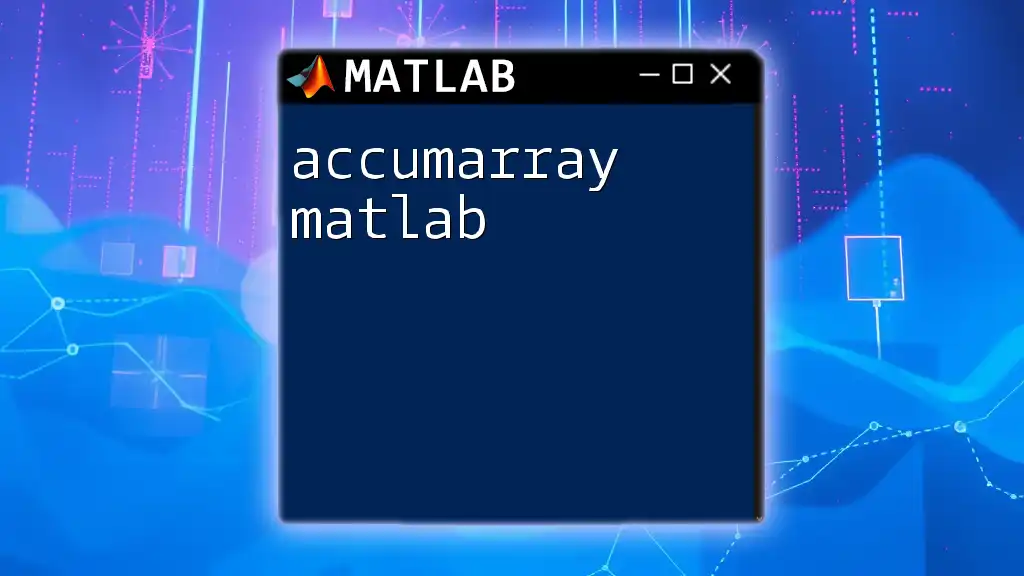
Accessing Array Elements
Indexing
In MATLAB, one-based indexing is used. This means the first element is accessed using an index of 1, for example:
elem = B(2, 3); % Accessing the element at the 2nd row, 3rd column
Here, `elem` will be `6`, which is located in the second row and third column of the matrix `B`.
Slicing Arrays
Slicing allows you to extract ranges or subsets of data from arrays. For example, to extract all elements of the first row of matrix B, use:
slice = B(1, :); % Extracting all columns from the first row
The colon operator (`:`) indicates selecting all elements in the specified dimension, which is particularly powerful when dealing with larger datasets.
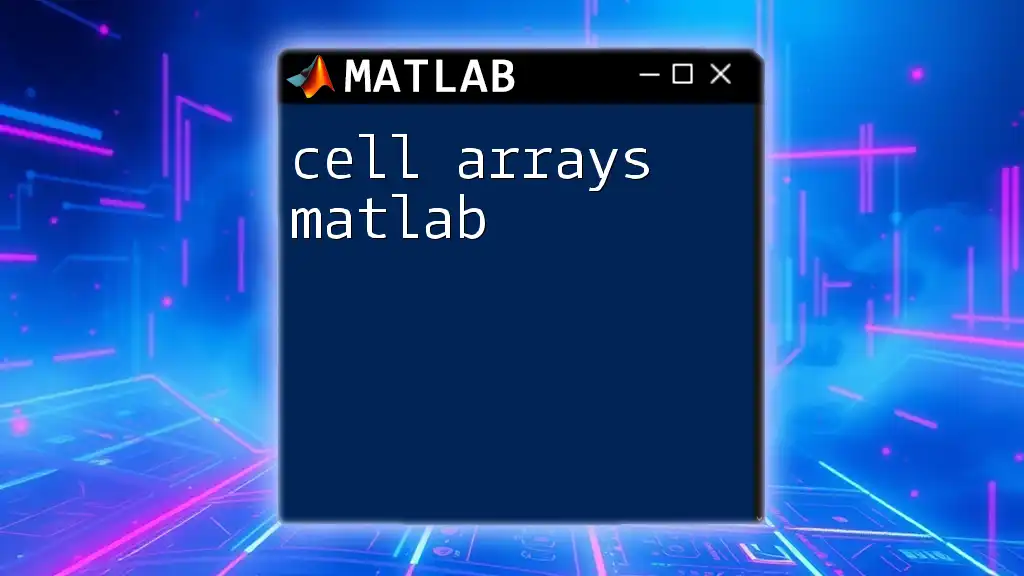
Modifying Arrays
Changing Array Elements
Modifications in MATLAB can be straightforward. You can modify any specific element simply by assigning a new value to it:
B(1, 2) = 10; % Changing the value in the 1st row, 2nd column to 10
This direct reassignment demonstrates how easily you can manage your data within arrays.
Adding and Removing Elements
MATLAB provides flexible ways to add or remove elements from arrays.
To add a new row to the bottom of a matrix, you can use `end`:
B(end + 1, :) = [7, 8, 9]; % Appending a new row
To remove an entire row, simply set it to an empty array:
B(1, :) = []; % Removing the first row
Such operations can significantly alter the structure of your data, but maintaining awareness of dimensions is key.
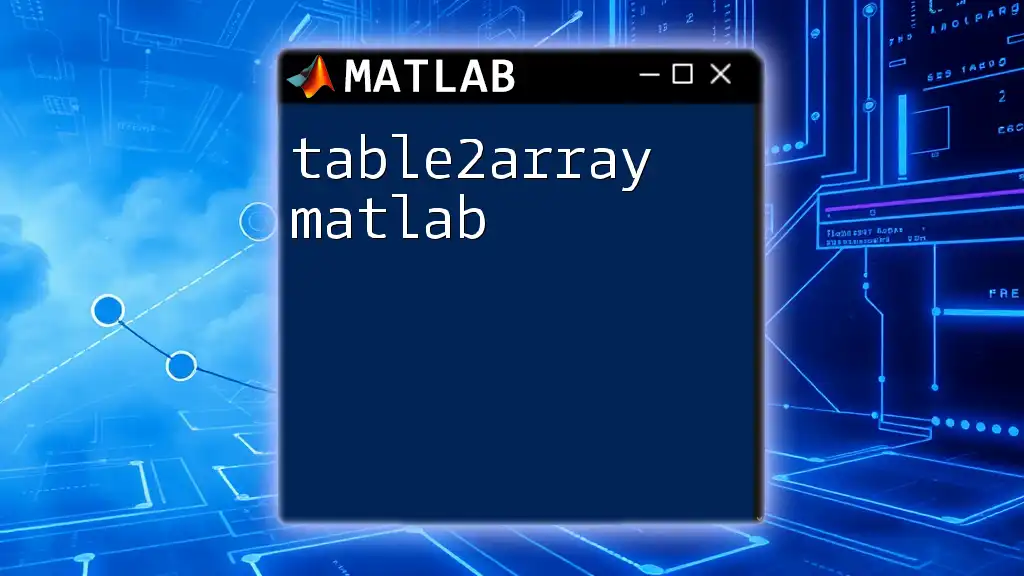
Operations on Arrays
Basic Array Operations
MATLAB allows for straightforward arithmetic operations on arrays. This includes addition, subtraction, multiplication, and division. Consider the following example:
A = [1, 2, 3; 4, 5, 6];
D = A + B; % Element-wise addition
The operation will take place element by element due to MATLAB's inherent capabilities for element-wise operations.
Array Functions
MATLAB includes built-in functions that enhance array usability. Functions like `sum`, `mean`, and `max` offer statistical insights directly:
total = sum(B, 1); % Sum across columns
In this case, `total` will return the sum of each column in matrix `B`. Additionally, understanding how to utilize `size` and `length` is essential. The `size` function reveals the dimensions of an array, while `length` informs you about the longest dimension.
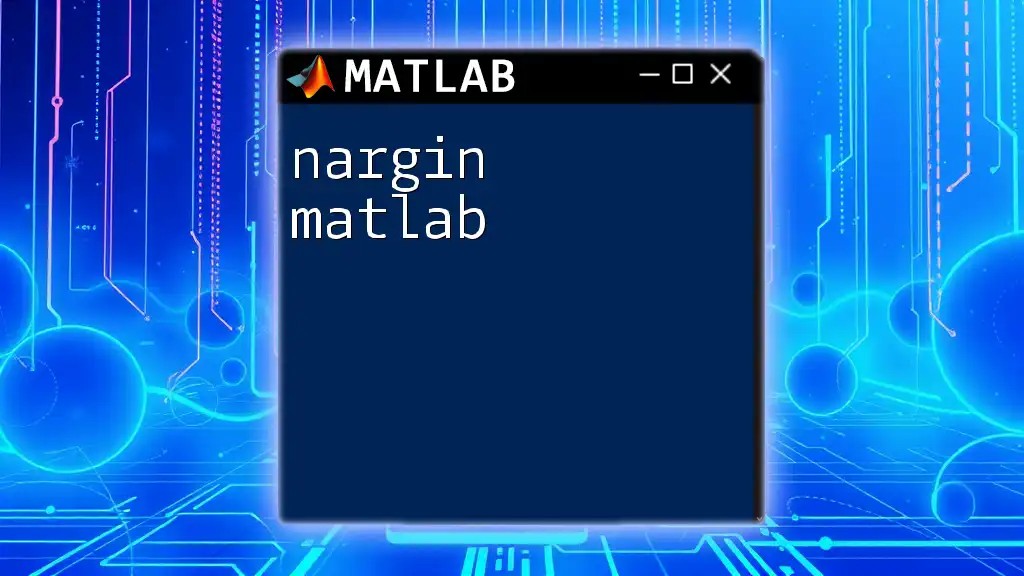
Array Manipulation Techniques
Reshaping Arrays
Reshaping array dimensions allows you to reorganize data effectively while maintaining the number of elements. The `reshape` function is particularly useful:
reshaped_array =reshape(B, [3, 2]); % Reshape to a 3x2 matrix
In this instance, `reshaped_array` becomes a new 3x2 matrix, assuming the total number of elements in `B` is compatible with this new shape.
Transposing Arrays
Transposing an array is as simple as using the transpose operator (`'`), which switches the rows and columns:
transposed = B'; % Transpose of matrix B
This manipulation is especially useful in many mathematical computations, particularly in linear algebra.
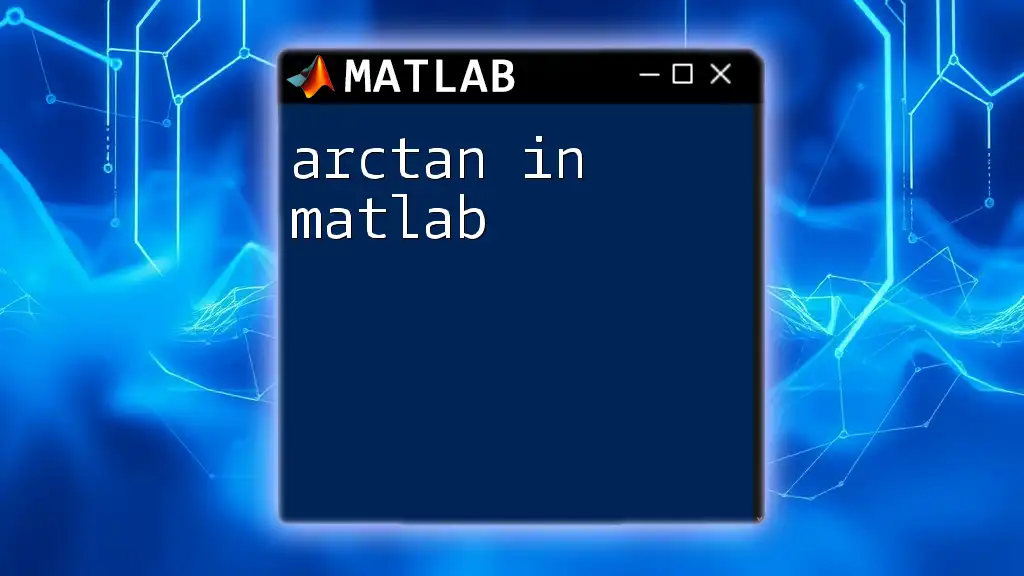
Conclusion
This comprehensive guide demystifies arrays in MATLAB, showcasing their significance as foundational data structures for various applications. From creation and modification to operations and advanced manipulation techniques, arrays form the bedrock of effective data management in MATLAB. By understanding and mastering these concepts, you are better equipped to handle diverse data-intensive tasks, whether in academia, research, or industry applications.
Next Steps
To deepen your understanding, practice using these commands in MATLAB and explore advanced topics related to array manipulations, such as cell arrays and structures, which offer even greater flexibility in handling complex data scenarios.
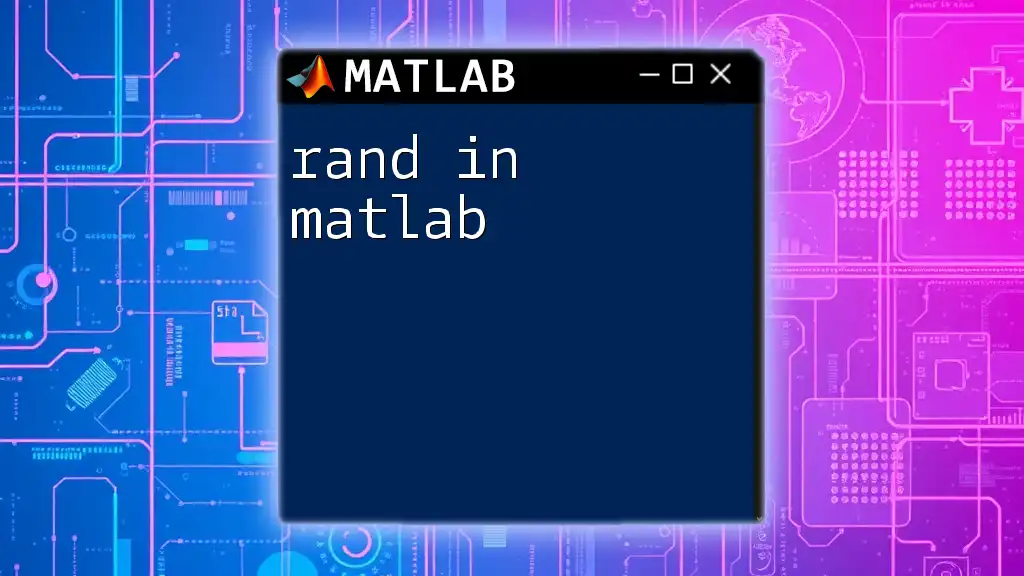
Additional Resources
For more insights on arrays in MATLAB, visit the official MATLAB documentation. Numerous tutorials and courses are also available to help you further your MATLAB skills.