The `return` command in MATLAB is used to exit a function and return control to the calling function or command prompt without producing any output.
function exampleFunction()
disp('This will be displayed.');
return; % Exits the function before reaching the next line
disp('This will NOT be displayed.'); % This line will be skipped
end
Introduction to MATLAB Functions
What is a MATLAB Function?
A MATLAB function is a standalone block of code that performs a specific task. Functions allow programmers to break their code into manageable pieces, enhancing reusability and readability. By grouping statements for a particular purpose, functions can be called multiple times without rewriting code. Functions in MATLAB begin with the keyword function, followed by the output variables, function name, and input parameters.
Understanding the Return Command
The return command in MATLAB is fundamentally linked to how functions operate. Its primary purpose is to indicate where the function should stop execution and output the computed results. While MATLAB implicitly returns values at the end of the function, using the return command can clarify the intended termination point, especially in complex functions.
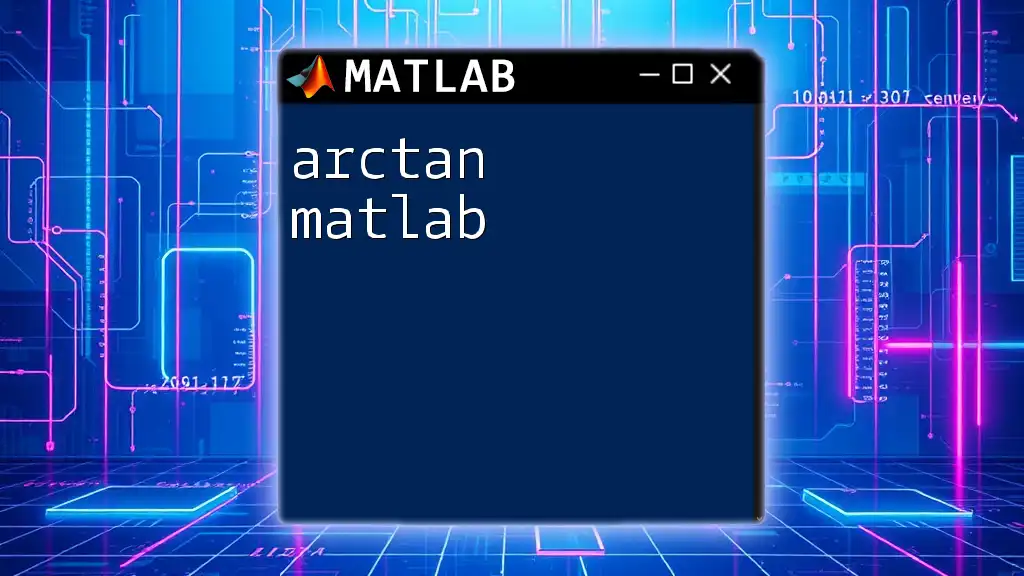
The Basics of the Return Command
Syntax of the Return Command
The syntax for the return command is straightforward. It does not require any arguments and simply looks like this:
return;
This command signals that the function has completed its operations. Importantly, it can return data of various types, including arrays, structures, and objects, thereby making MATLAB functions versatile.
Using Return in Simple Functions
When employing the return command in a simple function, it helps to better illustrate just how it works. Consider the following example:
function result = addNumbers(a, b)
result = a + b;
return; % Optional, as MATLAB will return upon function completion
end
In this function, `addNumbers`, two inputs, `a` and `b`, are added together, and the result is returned. The use of `return` here is optional since MATLAB will automatically return when the function execution is complete. However, explicitly using the return command can allow readability and clarity in your code flow.
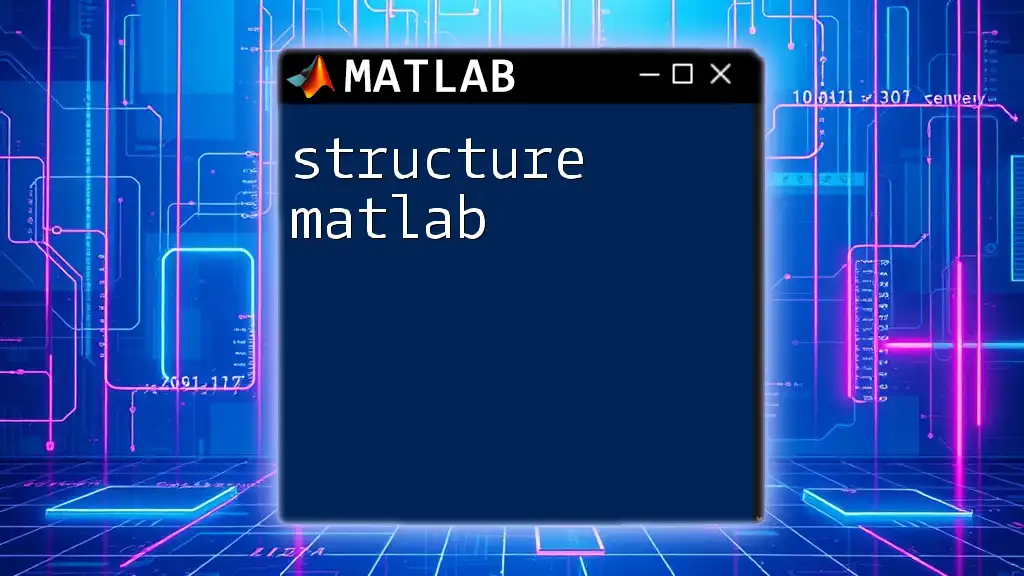
Returning Multiple Values
Understanding Multiple Returns
When coding in MATLAB, you often need to return multiple values from a function. This capability can streamline your workflow by allowing you to encapsulate several related outputs into a single function call, eliminating the need for multiple function calls.
Syntax for Returning Multiple Values
Returning multiple values involves listing the variables on the left side of the equal sign in the function definition. Here’s an example:
function [sum, difference] = arithmeticOperations(a, b)
sum = a + b;
difference = a - b;
return; % End of function
end
In this example, the `arithmeticOperations` function calculates both the sum and the difference of two numbers, `a` and `b`. It returns both values. This function can be called as follows:
[s, d] = arithmeticOperations(10, 5);
Thus, `s` will contain `15`, and `d` will contain `5`, making functions highly efficient for returning complex outputs.
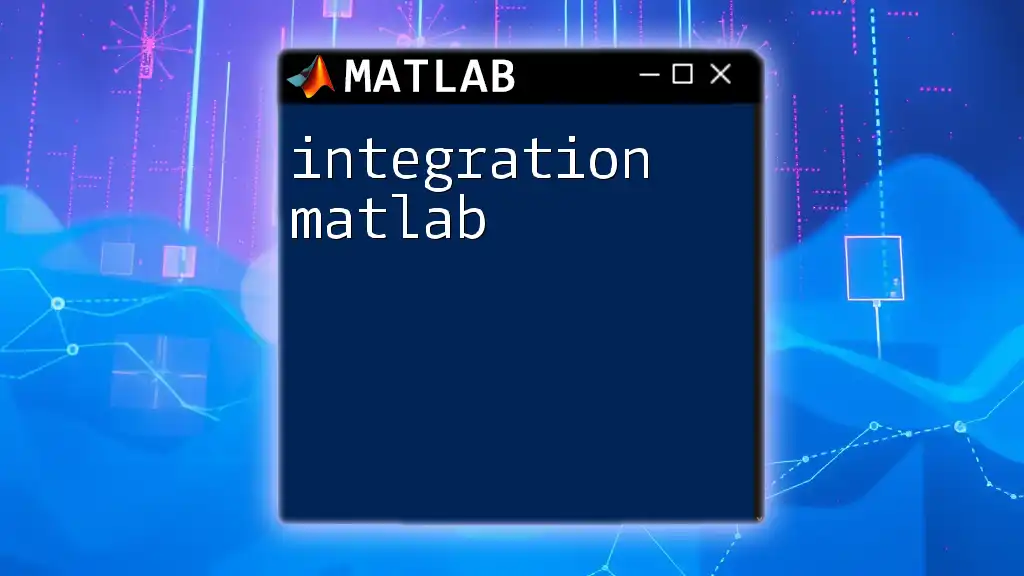
The Role of Return in Control Flow
Using Return to Exit a Function Early
The return command is especially useful for modularizing your code and managing its flow. When certain conditions are met, or errors occur, you may want to exit a function immediately—this is where the return command shines. For instance:
function result = safeDivide(a, b)
if b == 0
disp('Error: Division by zero');
return; % Exiting the function without performing the division
end
result = a / b;
end
In the `safeDivide` function, if the divisor `b` is zero, an error message is displayed, and the function halts execution before attempting to perform a division. This use of return not only prevents potential errors but also makes the function's intention clear.
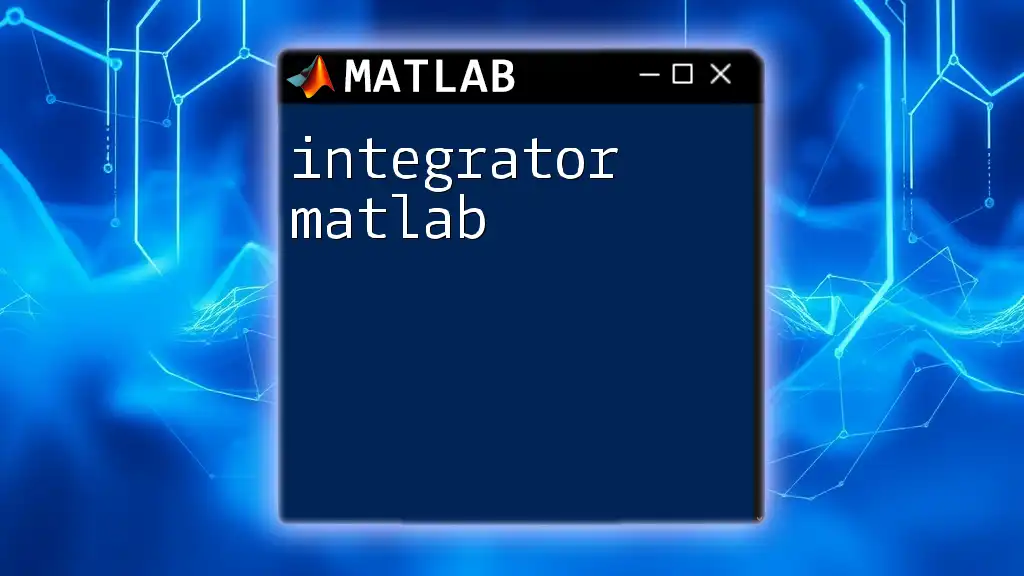
More Advanced Use Cases of Return
Complex Functions with Conditional Returns
In more advanced scenarios, the return command can be used effectively within conditional statements to manage the flow based on user-defined logic. For example:
function status = evaluateScore(score)
if score < 50
status = 'Fail';
return; % Early return on fail
elseif score < 75
status = 'Pass';
return; % Early return on pass
end
status = 'Excellent'; % Final return if score is 75 or above
end
This `evaluateScore` function evaluates a student's score and categorizes it. The presence of multiple return points aids in clarity. If the score is below 50, it immediately returns "Fail". If it is between 50 and 75, it returns "Pass". Only scores 75 and above will lead to "Excellent". This method enhances functionality and keeps the user informed throughout.
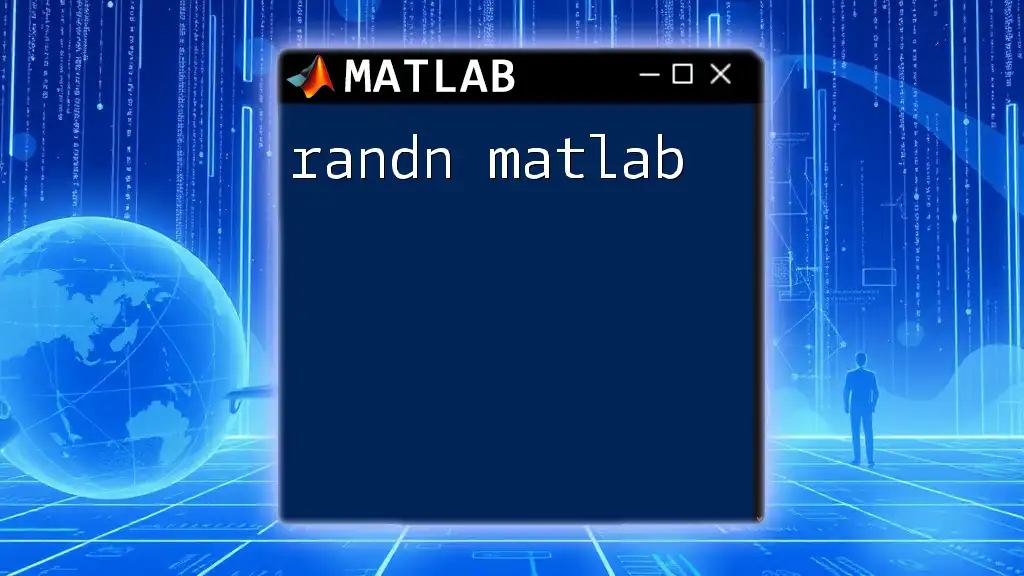
Best Practices for Using Return in MATLAB
Keep it Simple
When writing functions in MATLAB, simplicity should be a guiding principle. Clarity in function design improves maintainability. Long and complex functions should be broken down into smaller, more manageable sub-functions that can be called throughout your primary function.
Meaningful Return Values
Another best practice involves ensuring that your returned values contain meaningful, contextual information. Avoid ambiguous outputs; instead, provide descriptive results that make it easy for users to understand what the function does. A descriptive variable name is paramount in communicating the purpose of the return value.
Documentation and Readability
To enhance readability and usability, include comments within your code. Documenting your functions and their expected behavior with clear comments will make understanding your code easier, especially for future users or collaborators. For example, before defining the function outputs, include notes describing known inputs or edge cases.
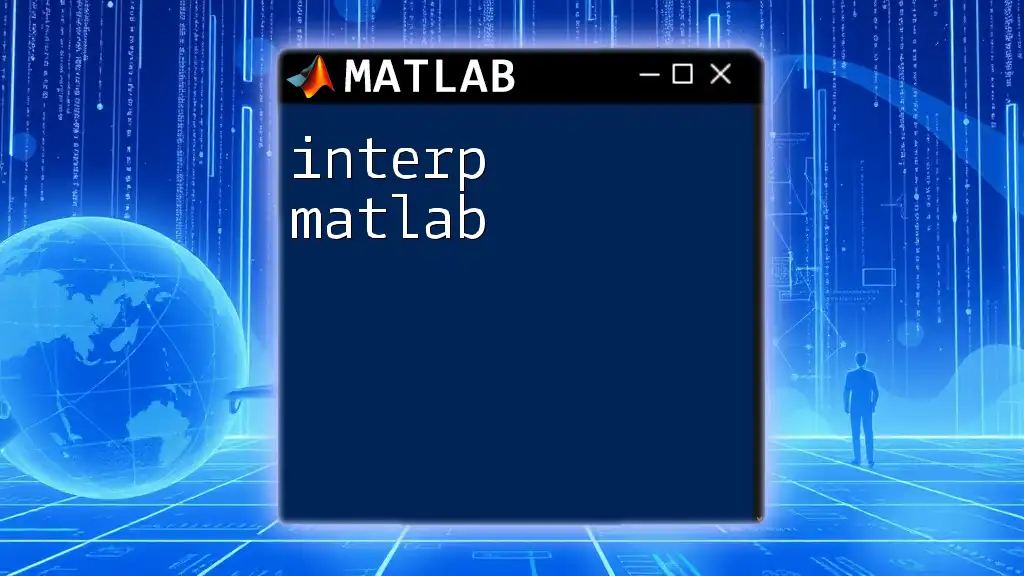
Conclusion
The return command in MATLAB is a fundamental aspect of writing effective functions. As we've explored, it plays a vital role in defining where your functions stop executing, returning either single or multiple values, and managing control flow. By adhering to best practices such as keeping functions simple and ensuring meaningful return values, you can enhance both the usability and clarity of your MATLAB code.
Practicing and mastering the return command will undoubtedly contribute to your proficiency in MATLAB. Enrich your coding journey by diving into various functions and understanding the depth of control you can achieve through effective use of returns.