The `regexp` function in MATLAB is used for matching and extracting substrings from text based on regular expression patterns.
Here's a simple code snippet that demonstrates how to use `regexp` to find email addresses in a string:
text = 'Please contact us at support@example.com or sales@example.org.';
emails = regexp(text, '[\w.-]+@[\w.-]+', 'match');
disp(emails);
Understanding the Basics of `regexp`
Syntax of `regexp`
The `regexp` function in MATLAB enables you to search for patterns in strings using regular expressions. The basic structure of the syntax is:
regexp(string, expression, options)
- `string`: This is the input text that you want to search through.
- `expression`: This refers to the pattern you are looking for, which is defined using a regular expression.
- `options`: This is an optional argument allowing further customization of the search, such as matching case sensitivity or returning the positions of matches.
Example of Basic Usage
Consider the following scenario where you want to find a specific word in a string.
str = 'Hello World';
pattern = 'Hello';
match = regexp(str, pattern);
In this example, the output of `match` will provide the starting index of the word "Hello" in the string. Understanding how to interpret this index is crucial—it indicates where the match is found.
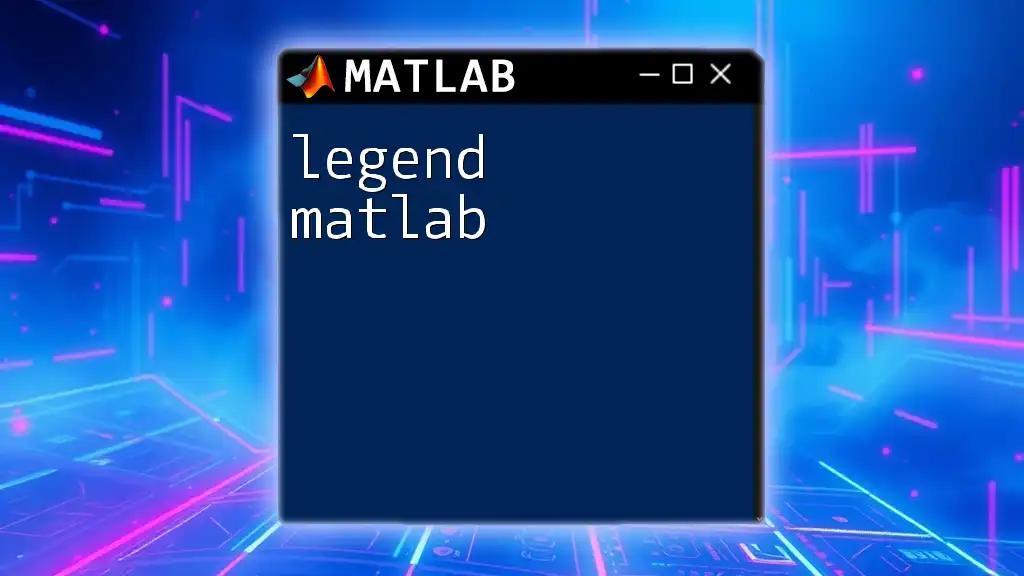
Advanced Pattern Matching Techniques
Types of Regular Expressions
Character Classes
Regular expressions allow you to define a set of characters to look for. Character classes are denoted by square brackets `[]` and can be combined for greater flexibility. For example:
str = 'abc123';
pattern = '[0-9]'; % Match any digit
matches = regexp(str, pattern, 'match');
In this case, every digit in the string 'abc123' will be captured, returning an array of matches containing `{'1', '2', '3'}`.
Quantifiers
Quantifiers help specify how many instances of a character or group should be matched.
- `*`: Match zero or more times.
- `+`: Match one or more times.
- `?`: Match zero or one time.
- `{n,m}`: Match between n and m times.
For example, using a quantifier:
str = 'aabbbcc';
pattern = 'a*b'; % Match 'a' zero or more times followed by 'b'
matches = regexp(str, pattern, 'match');
The output will show `{'ab', 'ab'}` indicating how the pattern was matched throughout the string.
Anchors and Boundaries
Anchors are essential for specifying positions in the string:
- `^`: Matches the start of the string.
- `$`: Matches the end of the string.
- `\b`: Matches word boundaries.
For instance:
str = 'Hello, Hello World';
pattern = '\bHello\b'; % Match 'Hello' as a whole word
matches = regexp(str, pattern, 'match');
This pattern will capture only the occurrences of "Hello" that stand alone, excluding partial matches.

Using `regexp` to Extract Data
Extracting Substrings
Capture groups in regular expressions allow you to extract specific parts of a match. These are created using parentheses `()` and can be accessed after the match.
For example, if we want to extract components from an email address:
str = 'Email: example@mail.com';
pattern = '(\w+)@(\w+)\.(\w+)';
[user, domain, tld] = regexp(str, pattern, 'tokens');
Here, the tokens will store the extracted parts of the email. `user`, `domain`, and `tld` provide access to 'example', 'mail', and 'com', respectively. This method is particularly powerful for data extraction tasks.
Replacing Text
The `regexprep` function in MATLAB allows you to replace matched patterns with new strings. This can be very useful for cleaning or modifying text.
For instance:
str = 'abc123xyz';
pattern = '123'; % Pattern to replace
new_str = regexprep(str, pattern, '456');
The output stored in `new_str` will now be `'abc456xyz'`, demonstrating how specific parts of a string can be efficiently updated without unnecessary complications.

Practical Applications of `regexp`
Text Cleaning
Regular expressions can be invaluable for cleaning up data. Removing unwanted characters can streamline text analysis processes. For example, if you want to remove non-alphabetical characters:
str = '123 abc. #$$';
clean_str = regexprep(str, '[^a-zA-Z ]', ''); % Remove everything except letters and spaces
`clean_str` will result in `' abc '`—the unwanted characters are neatly stripped away.
Data Validation
Validating user input can enhance data quality, and regular expressions are an ideal tool for such tasks. When validating emails, for example, you can use a regular expression to ensure proper formatting:
email = 'user@example.com';
pattern = '^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$';
isValid = ~isempty(regexp(email, pattern, 'once'));
In this case, `isValid` will be `true` if the email format is correct, ensuring cleanliness in data collection and management.
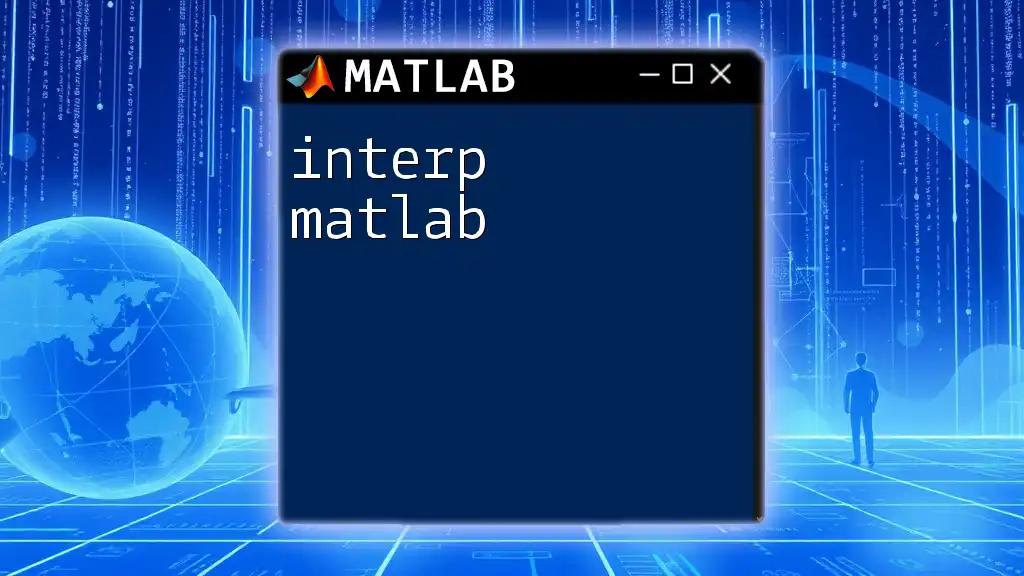
Best Practices for Using `regexp`
Tips for Efficient Regular Expression Creation
- Readability: Ensure your regular expressions are clear and concise. Use comments to explain complex patterns if necessary.
- Testing Patterns: Before implementing expressions in larger code, test them using tools like Regex101 or MATLAB’s built-in functions to ensure they work as intended.
Common Pitfalls to Avoid
- Overcomplicating Patterns: Keep patterns as simple as possible to avoid confusion and potential errors.
- Case Sensitivity Issues: Be aware of how MATLAB handles case sensitivity and use the appropriate flags in the options parameter if needed.
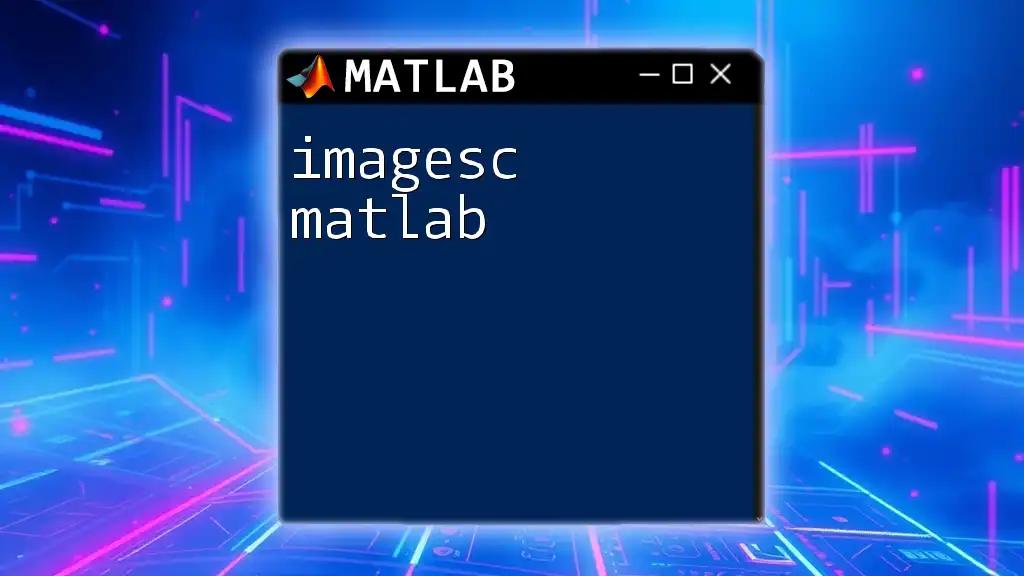
Conclusion
Using `regexp` in MATLAB unlocks a plethora of possibilities for string processing. From straightforward searches to intricate data extraction and validation tasks, becoming proficient with regular expressions can significantly enhance your coding efficiency and accuracy. Regular practice and experimentation with various pattern types will build your confidence and skills in using `regexp matlab` to its fullest potential.
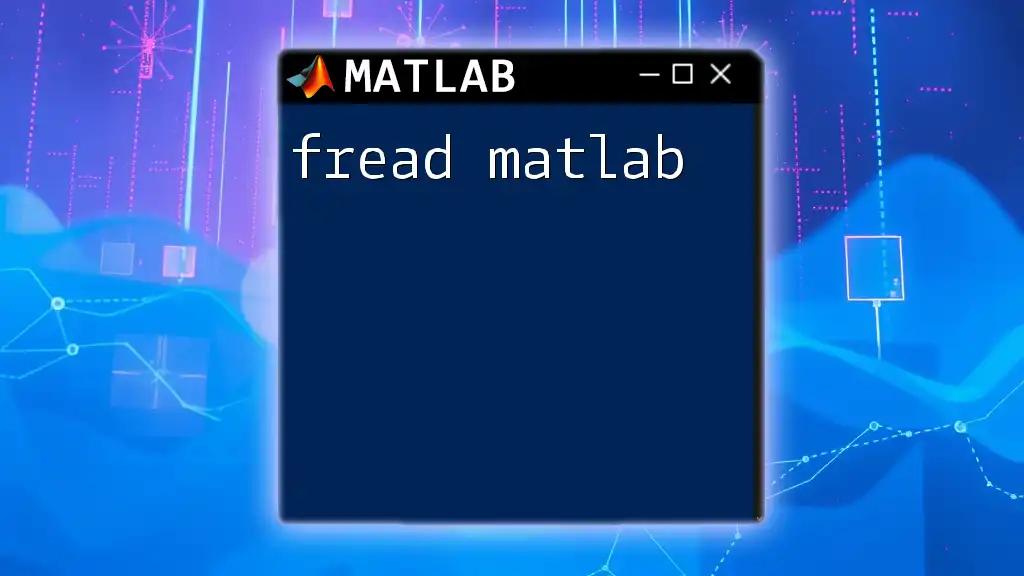
Call to Action
Join our MATLAB Learning Community to delve deeper into topics like `regexp` and other powerful MATLAB functions. Subscribe to receive additional tips and tutorials that can help you master MATLAB commands and improve your coding efficiency!