The `detrend` function in MATLAB removes the linear trend from a given dataset, helping to stabilize the mean of the data over time.
Here's a simple code snippet to demonstrate its usage:
% Sample data with a trend
t = 0:0.1:10;
data = sin(t) + 0.5*t;
% Detrending the data
detrended_data = detrend(data);
% Plotting the original and detrended data
figure;
subplot(2,1,1);
plot(t, data);
title('Original Data with Trend');
xlabel('Time');
ylabel('Value');
subplot(2,1,2);
plot(t, detrended_data);
title('Detrended Data');
xlabel('Time');
ylabel('Value');
Introduction to Detrending
What is Detrending?
Detrending is the process of removing trends from data. It is essential in data analysis, particularly for time series and other types of data that may have underlying trends. By eliminating these trends, analysts can better focus on the inherent characteristics or fluctuations in the data itself and make more accurate interpretations.
Common Applications of Detrending
Detrending is widely used in various fields, including signal processing, economics, and natural sciences. It helps improve data forecasting, noise reduction, and clarity in cyclical patterns, making it easier to analyze phenomena such as stock market trends or seasonal customer buying behavior.
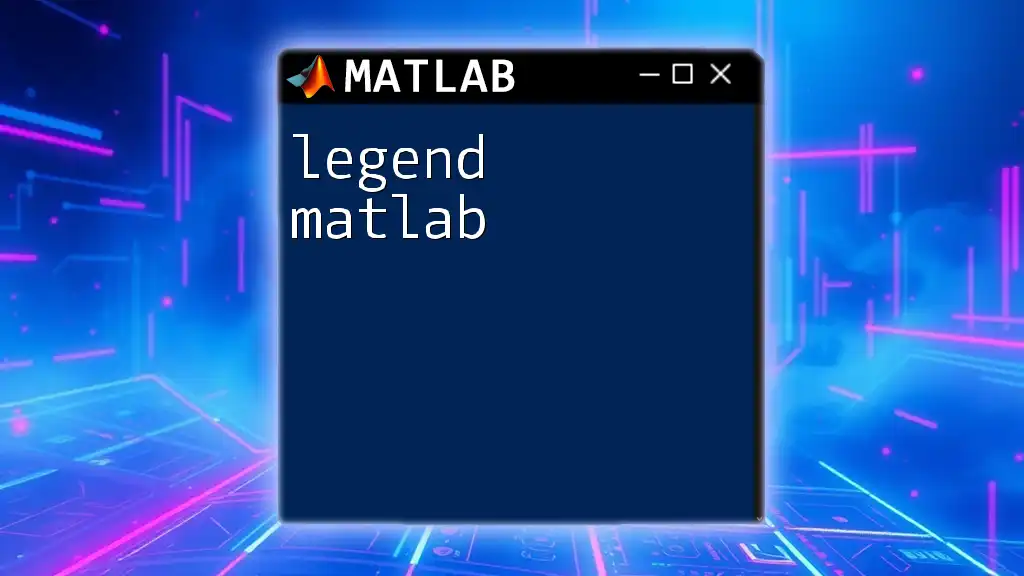
Understanding Detrend in MATLAB
MATLAB’s Detrend Function
MATLAB provides a built-in `detrend` function, which makes it simple to remove trends from your data. Its syntax is straightforward, allowing users to specify how they want to detrend their data. The primary syntax is:
detrended_data = detrend(data, method)
Where `data` is the input data to be detrended, and the `method` specifies the type of detrending (e.g., 'linear', 'constant', 'quadratic').
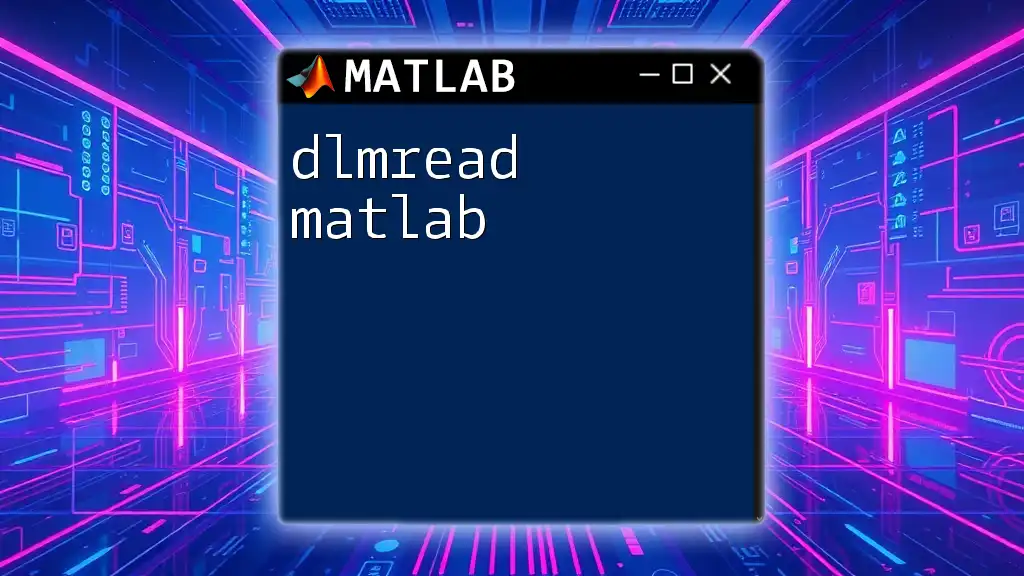
Types of Detrending
Linear Detrending
Linear detrending is one of the simplest methods, where a linear trend is fitted to the data, and the fitted line is subtracted from the original data. This method is effective when the data exhibits a consistent upward or downward trend. It is important to choose this method when trends are predominantly linear in nature.
Polynomial Detrending
Polynomial detrending involves fitting a polynomial function to the data before subtracting it. This technique allows for the removal of non-linear trends and is particularly useful when the data shows more complex patterns.
When implementing polynomial detrending, choosing the degree of the polynomial is crucial as it influences the robustness of the detrending.
% Polynomial detrending example for degree 2
x = (1:100)';
y = 5 * x + randn(size(x)) * 10; % Simulated data
p = polyfit(x, y, 2);
detrended_y = y - polyval(p, x);
Removing the Mean
A basic method of detrending is simply removing the mean from the data. By centering the data around zero, this technique effectively stabilizes the dataset without imposing a specific trend.
This straightforward method is ideal when the primary concern is to analyze deviations from the mean.
% Mean removal example
data = rand(100, 1); % Simulated data
detrended_data = data - mean(data);
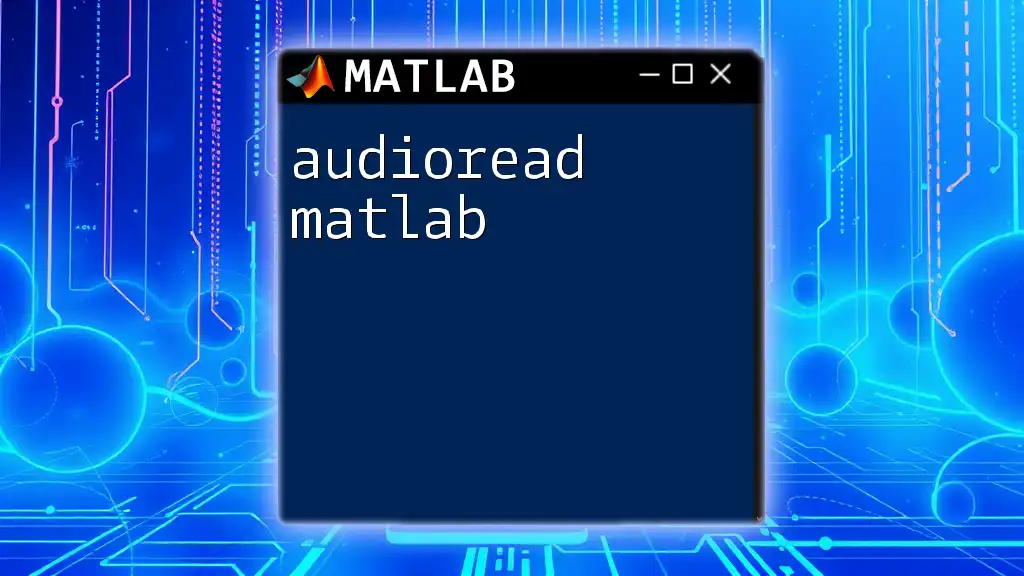
Practical Examples of Detrending
Example 1: Detrending a Time Series Dataset
Let’s consider a common scenario—detrending a time series dataset. Here’s a step-by-step procedure:
- Generate a time vector and a signal with a trend.
- Use the `detrend` function to remove the trend.
Here’s the code:
% Detrending a time series
t = 0:0.01:10; % Time vector
y = sin(t) + 0.5 * t; % Simulated sinusoidal signal with linear trend
detrended_signal = detrend(y);
plot(t, y, 'b', t, detrended_signal, 'r');
legend('Original Signal', 'Detrended Signal');
title('Detrending Example');
In this example, the original signal (blue) contains both a sinusoidal component and a linear trend, while the detrended signal (red) reveals the underlying oscillation clearly.
Example 2: Detrending a Sine Wave
When dealing with periodic functions, like sine waves, it is essential to remove any linear trends to observe the true periodic nature of the data.
% Detrending a periodic signal
time = 0:0.1:10*2*pi;
signal = sin(time) + 0.1 * time; % Original signal with trend
detrended_signal = detrend(signal);
figure;
subplot(2,1,1); plot(time, signal); title('Original Signal');
subplot(2,1,2); plot(time, detrended_signal); title('Detrended Signal');
In this case, the original signal with trend displayed in the first subplot is transformed into a clearer periodic function in the second subplot after detrending.
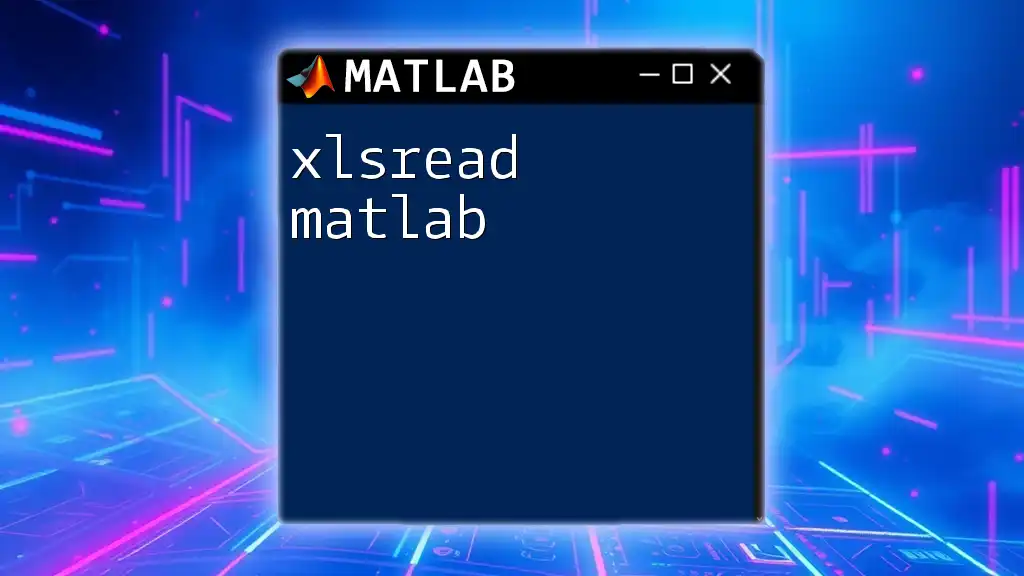
Working with Detrended Data
Analyzing Detrended Data
Once you apply detrending in MATLAB, evaluating the characteristics of the detrended data is crucial. Statistical methods, such as calculating means, standard deviations, or employing spectral analysis techniques, help understand the underlying patterns better.
Visual representation is also vital. Plotting both original and detrended data side by side allows for immediate assessment of the improvements gained through detrending.
Coherent Approach to Detrending in Projects
Before selecting a detrending method, always consider the nature of your data. Understanding the type of trends present, whether linear or polynomial, is fundamental. This alignment ensures that your detrending techniques match the objectives of your analysis, leading to better insights.
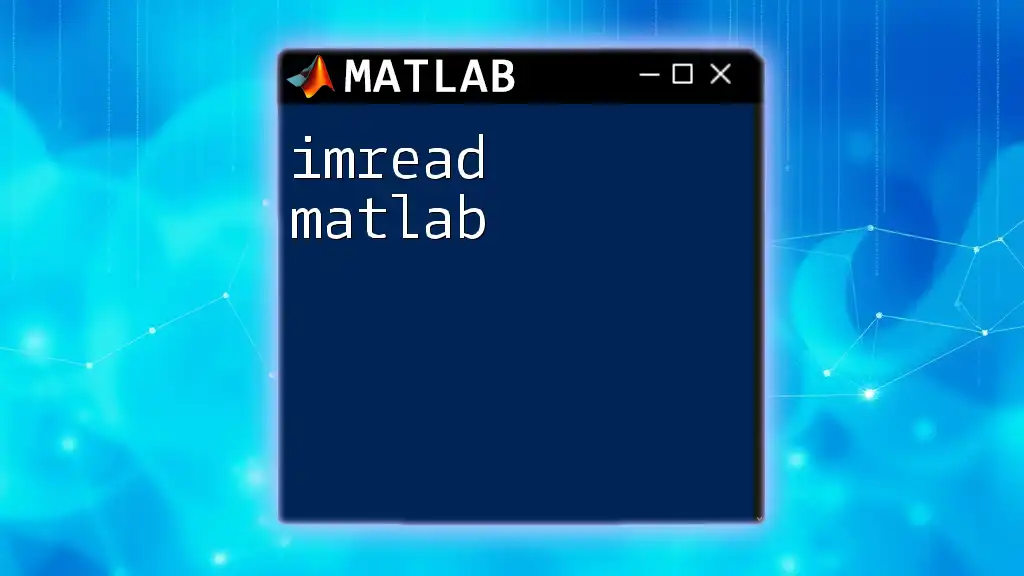
Troubleshooting Detrending Issues
Common Mistakes
Choosing the wrong method can drastically affect your analysis. Linear detrending on data with non-linear trends can obscure important features, while removing the mean may ignore significant structural shifts.
How to Debug Detrending Issues
Analyzing your detrended results is crucial. Always visualize the data before and after detrending to confirm loss of relevant trends or information in the process. This step ensures that you retain the important characteristics of your data while eliminating unwanted trends.
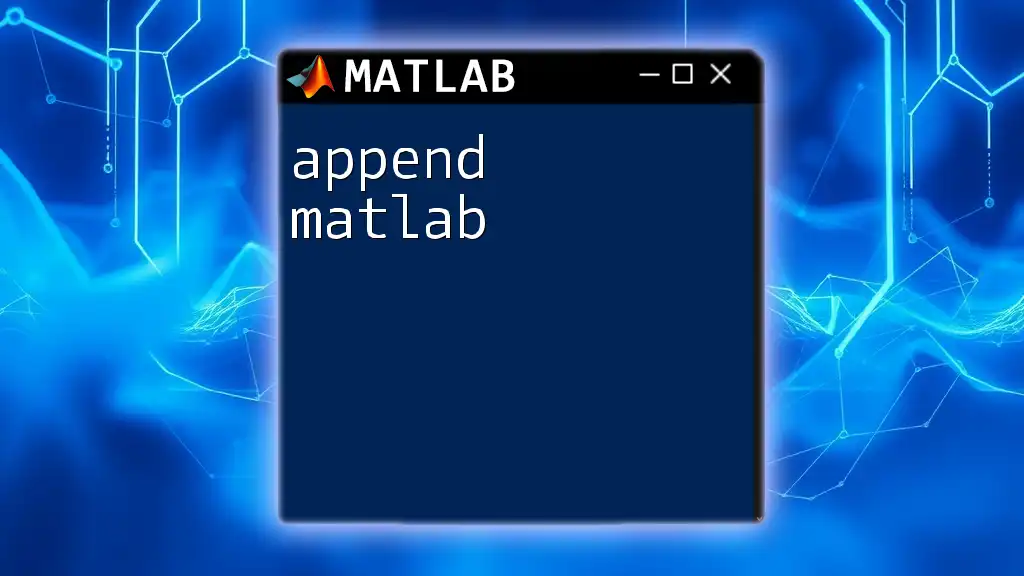
Summary and Conclusion
In conclusion, the ability to detrend data in MATLAB is a powerful tool for any analyst. By leveraging methods such as linear and polynomial detrending, or straightforward mean removal, one can significantly enhance the clarity and interpretability of data.
Detrending is not just about cleaning data; it’s also about preserving the essence of the information. By experimenting with various techniques and understanding their impact, analysts can unlock deeper insights and improve their analyses significantly.
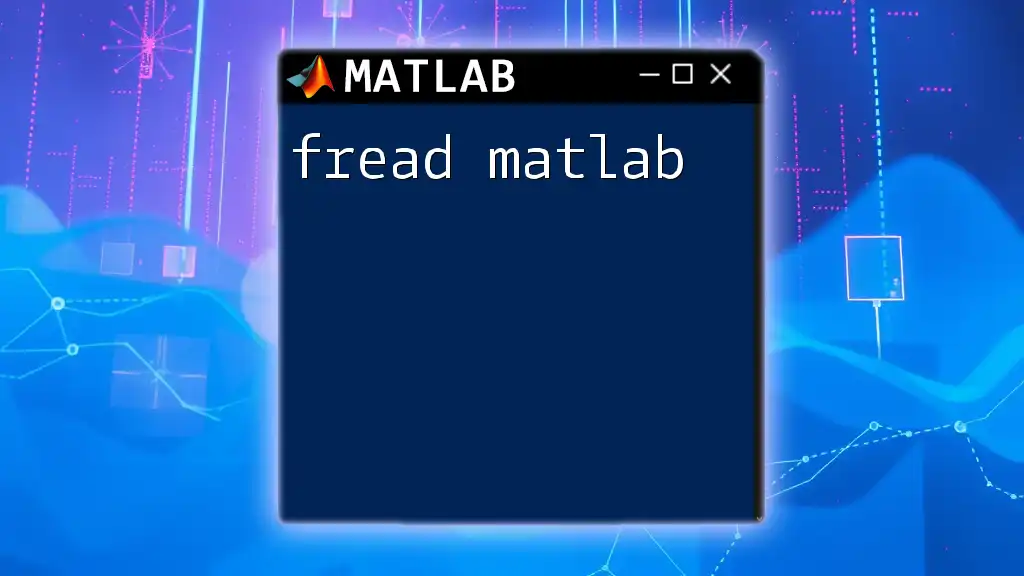
Additional Resources
For those looking to expand their knowledge about detrending, MATLAB offers a wealth of documentation and resources. Further reading on related functions, tutorials, and guides can greatly enhance your understanding and practical application of these techniques.