The `spline` function in MATLAB is used to perform cubic spline interpolation, allowing you to create a smooth curve through a set of data points.
% Define data points
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 1, 4];
% Create a spline interpolation
xx = linspace(1, 5, 100); % Generate 100 points between 1 and 5
yy = spline(x, y, xx); % Compute spline values
% Plot the results
plot(x, y, 'o', xx, yy, '-');
title('Cubic Spline Interpolation');
xlabel('X');
ylabel('Y');
legend('Data Points', 'Spline Curve');
Introduction to Spline Interpolation in MATLAB
What is Spline Interpolation?
Spline interpolation is a method used to create a smooth curve through a series of points. Unlike polynomial interpolation, which can lead to oscillations between data points (Runge's phenomenon), spline interpolation uses piecewise polynomial functions, which are more stable and provide smoother transitions.
Importance of Spline in Data Analysis
The necessity for spline interpolation arises in various fields, including engineering, computer graphics, and statistical modeling. Splines allow for flexible data fitting and are especially useful when dealing with noisy data or when creating smooth visualizations. They are adaptable to different shapes of data and can interpolate or approximate functions effectively.
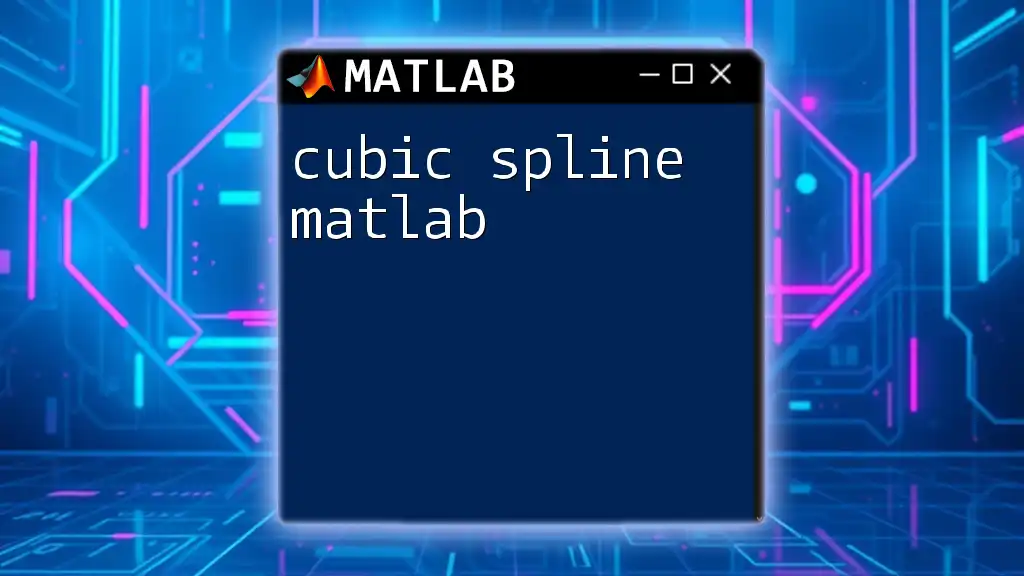
Types of Splines
Linear Splines
Linear splines connect data points with straight lines, resulting in a piecewise linear function. While they are simple to compute, they may not provide a smooth transition between segments due to abrupt changes in slope. Linear splines are suitable for scenarios requiring quick approximations without needing smoothness.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
pp = pchip(x,y); % Piecewise linear spline
Quadratic Splines
Quadratic splines use piecewise quadratic polynomials, allowing for curvature between data points. They are more flexible than linear splines but not as smooth as cubic splines. Quadratic splines can produce nice curves when the data is relatively simple or when fewer computations are desired.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
pp = csaps(x, y, 0.5); % Quadratic spline with smoothing
Cubic Splines
Cubic splines are the most commonly used type due to their desirable properties of smoothness and continuity. A cubic spline is a piecewise cubic polynomial that minimizes overall curvature, ensuring that the first and second derivatives are continuous across segment junctions. This makes them ideal for applications requiring smooth curves.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
splineFit = spline(x, y); % Cubic spline fitting
B-Splines
B-splines, or basis splines, offer additional control over the degree of smoothness and shape of the spline. They are particularly useful in computer graphics and applications where flexibility in modeling is essential. B-splines can define complex curves without oscillations typical of high-degree polynomial splines.
x = linspace(0, 1, 4);
y = [0 1 0 1];
sp = spapi(4, x, y); % B-spline representation
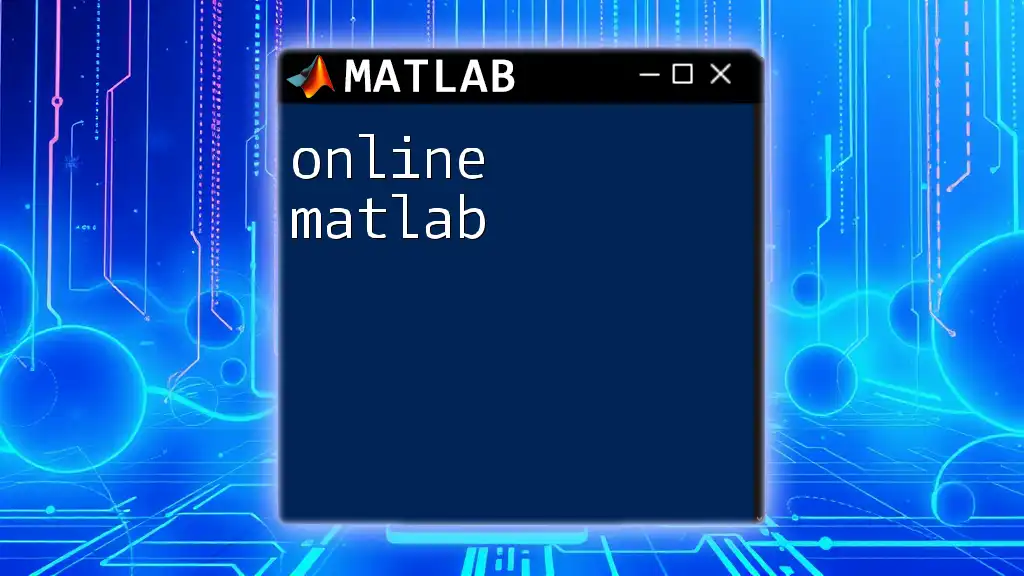
MATLAB Functions for Spline Interpolation
`spline` Function
The `spline` function in MATLAB offers a straightforward interface for performing cubic spline interpolation. The syntax is simple, using the data points along with the function’s parameters to obtain the curve.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
pp = spline(x, y); % Generate the cubic spline coefficients
`csape` Function
The `csape` function provides the capability to create cubic spline approximations with additional flexibility. It allows specifying boundary conditions and different types of splines, including natural and periodic splines.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
s = csape(x, y, 'periodic'); % Create a periodic cubic spline
`spapi` and `spap2` Functions
For those interested in B-splines, the `spapi` function computes a B-spline curve given a specified degree and knot vector. The `spap2` function allows for fitting B-splines to data using least squares, providing greater control over the fit.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
sp = spapi(4, x, y); % Define a B-spline curve
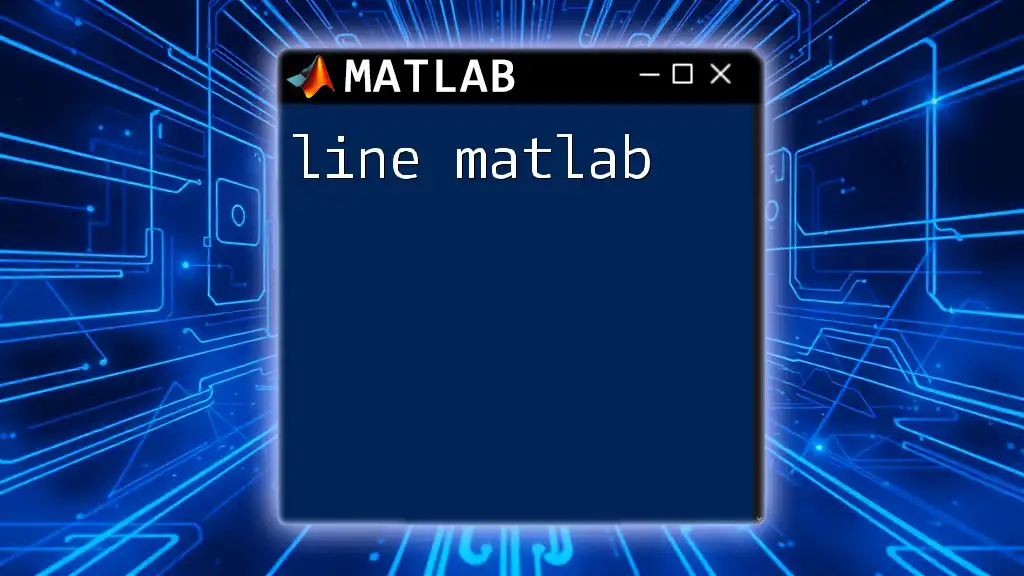
How to Fit a Spline to Data
Preparing Data for Spline Fitting
Before fitting a spline to data, ensure that the data is clean and well-prepared. Remove outliers and ensure that the data points are sorted in ascending order.
data = [4, 3, 7, 2]; % Sample unsorted data
sortedData = sort(data); % Sorted data for spline fitting
Fitting a Spline to Data Using `spline`
To fit a spline using the `spline` function, input your data points into the function and extract the spline coefficients. These coefficients can be used to evaluate or plot the fitted spline.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
pp = spline(x, y); % Fit a cubic spline
xi = linspace(1, 4, 100);
yi = ppval(pp, xi); % Evaluate spline at new points
Visualizing the Fitted Spline
Visualization is critical. You can plot the original data points alongside the fitted spline to assess the fit quality visually.
plot(x, y, 'ro', 'MarkerFaceColor', 'r'); % Original data points
hold on;
plot(xi, yi, 'b-'); % Fitted spline
title('Cubic Spline Fitting');
xlabel('X-axis');
ylabel('Y-axis');
legend('Data Points', 'Fitted Spline');
hold off;
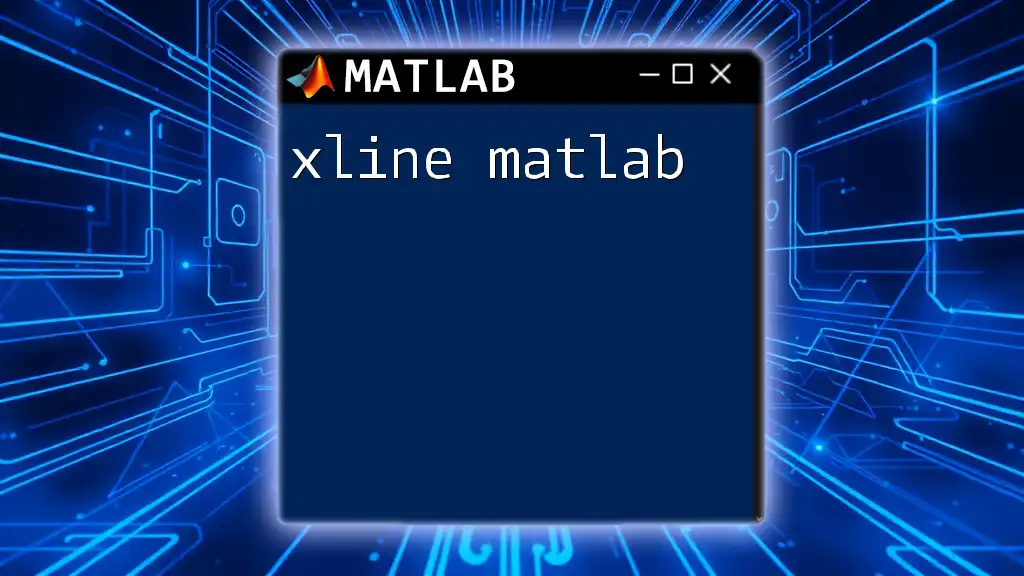
Advanced Spline Techniques
Spline Smoothing
Spline smoothing involves adjusting the spline fit to minimize overfitting. By using a smoother instead of a straight interpolation, you can create a curve that captures the general trend without noise. The `csaps` function can be utilized to generate a smooth spline.
x = [1, 2, 3, 4];
y = [1, 4, 3, 2];
s = csaps(x, y, 0.5); % Create a smoothed spline
Evaluating Spline Performance
It’s essential to evaluate the quality of your spline fit. Various metrics like Root Mean Square Error (RMSE) can help assess the goodness of fit. Cross-validation techniques can also provide insights into the robustness of your spline model.
errors = norm(y - ppval(pp, x)); % Calculate error between original and fitted
RMSE = sqrt(mean(errors.^2)); % Root Mean Square Error
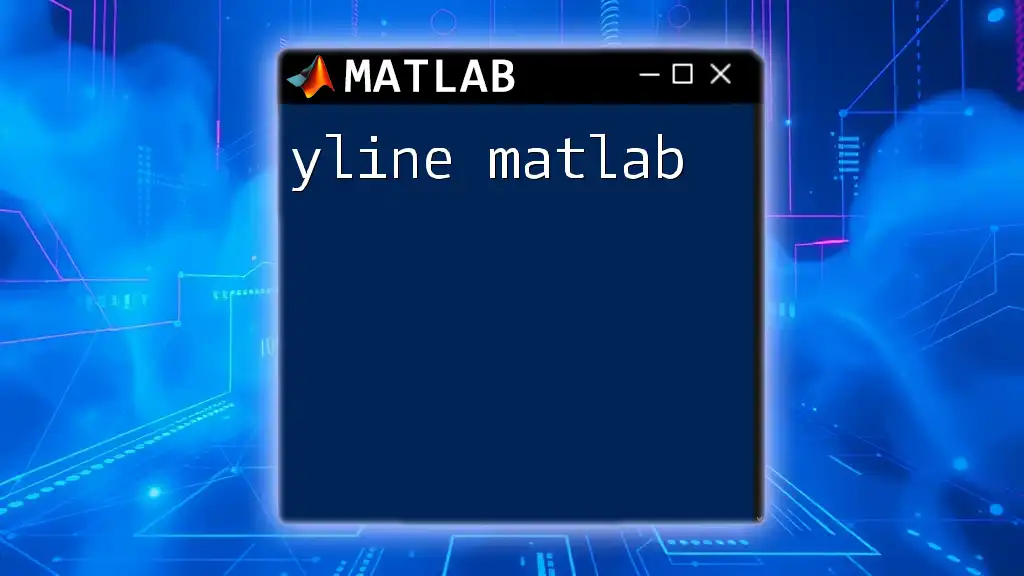
Common Issues and Troubleshooting
Overfitting vs. Underfitting
Finding the right spline complexity is key. Overfitting occurs when the spline matches the data too closely, capturing noise rather than the underlying trend. Conversely, underfitting occurs when the spline does not capture important features of the data. Adjusting the parameters of the spline functions can help in balancing these concerns.
Choosing the Appropriate Spline
The choice of spline type depends on the data characteristics and desired smoothness. Cubic splines are generally a good default, but consider quadratic splines for simpler datasets and B-splines for more control over shape and smoothness.
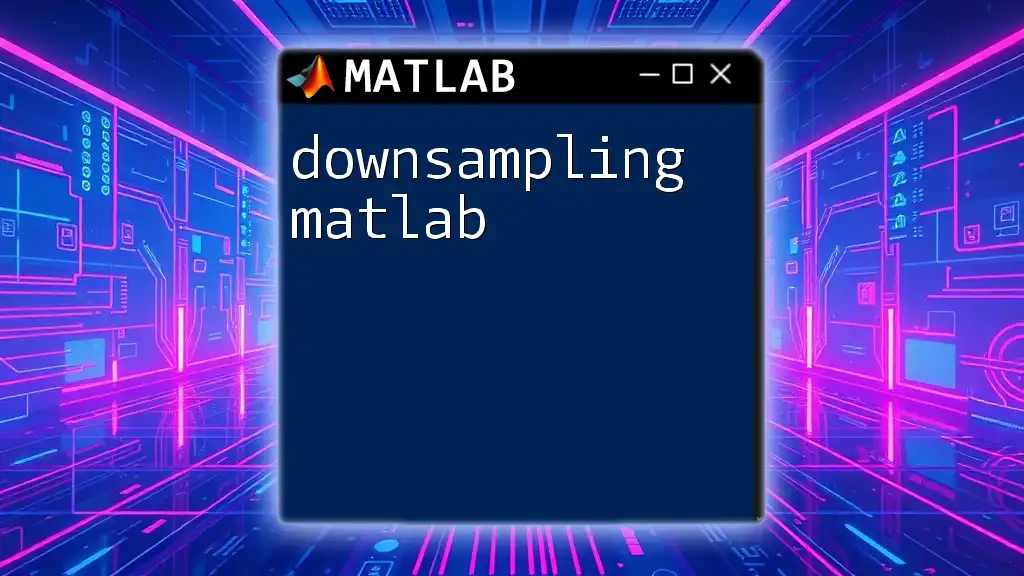
Conclusion
In summary, spline interpolation in MATLAB provides powerful techniques for creating and manipulating smooth curves through data points. Understanding the different types of splines, their MATLAB implementations, and how to evaluate their performance is crucial in many scientific and engineering applications. As technology evolves, the integration of splines into data analysis continues to prove beneficial for developing models and visualizations, enhancing both insight and accuracy.
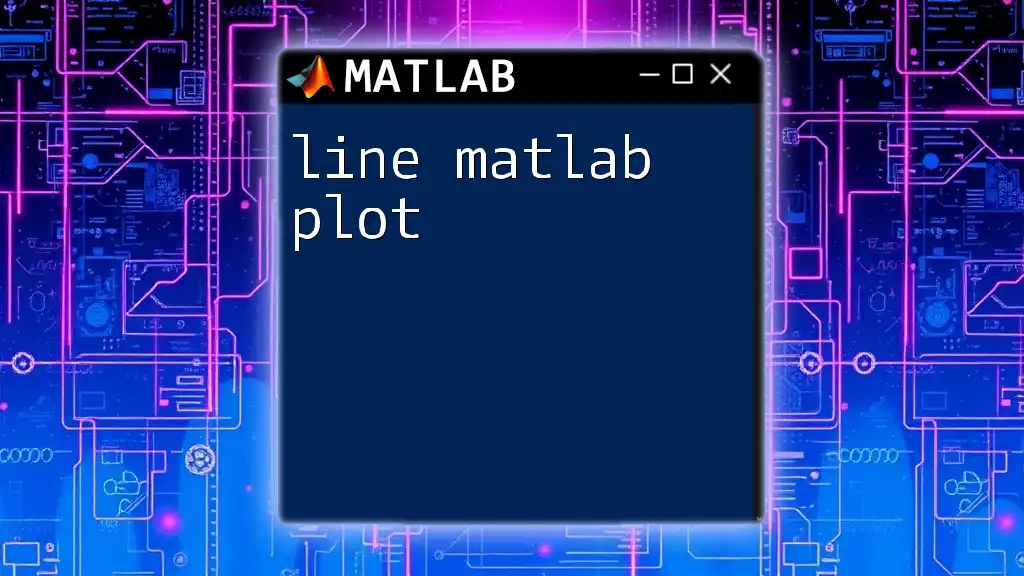
FAQs
What are the advantages of using splines over traditional interpolation methods?
Splines offer improved stability and smoothness compared to traditional polynomial interpolation methods, effectively reducing oscillations and artifacts.
Can splines be used for high-dimensional data?
Yes, splines can be generalized to higher dimensions, but the complexity of implementation increases significantly.
How do I implement splines for real-time data processing in MATLAB?
For real-time applications, consider implementing functions that update spline coefficients dynamically as new data points are obtained, using techniques like recursive spline adjustments.