The `isempty` function in MATLAB checks whether a given array or variable is empty, returning a logical value of `true` if it is empty and `false` otherwise.
Here’s a code snippet demonstrating its usage:
% Example of using isempty function
A = [];
result = isempty(A); % result will be true since A is an empty array
What is `isempty`?
`isempty` is a built-in MATLAB function designed to determine whether an array is empty. An empty array is one that has no elements, meaning its size is zero. This function is crucial in programming because it allows developers to validate inputs and ensure that operations are only performed on arrays with actual content.
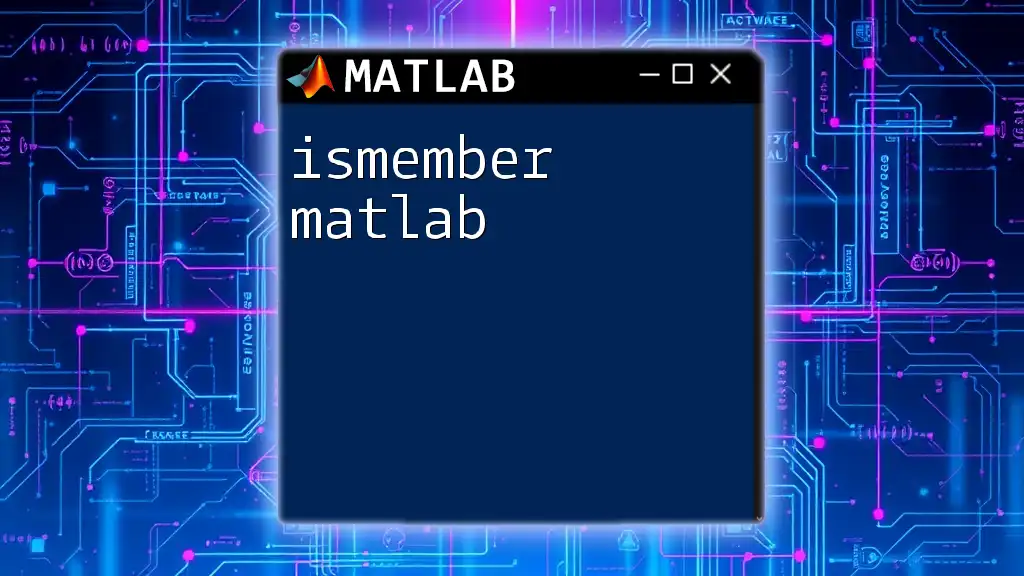
When to use `isempty`?
You should employ `isempty` in any scenario where the potential absence of data could lead to runtime errors or unexpected behavior. This includes validating user inputs, checking array states before processing, or managing iterations where the absence of data could disrupt the logic of your program.
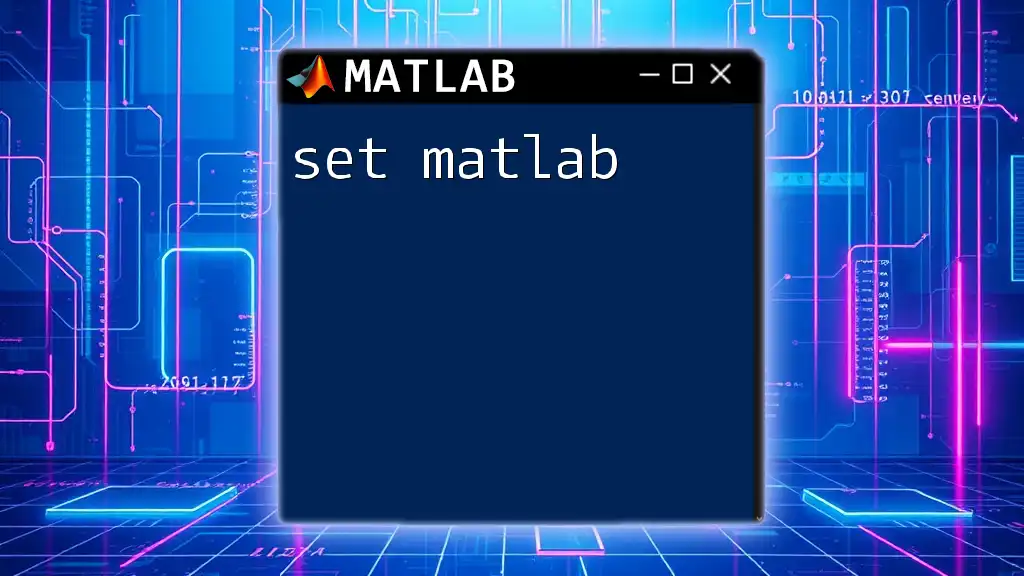
Understanding Empty Arrays in MATLAB
What Constitutes an Empty Array?
An empty array in MATLAB can take several forms, including:
- Numeric arrays defined as `[]`.
- Cell arrays initialized as `{}`.
- Character arrays expressed as an empty string `''`.
Understanding what qualifies as an empty array is essential for effective programming in MATLAB.
How MATLAB Represents Empty Arrays
MATLAB uses `[]` to denote an empty numeric matrix. This representation is concise and allows for easy recognition of an array's state.
For example, you can create different types of empty arrays as follows:
numericArray = []; % Numeric array
cellArray = {}; % Cell array
charArray = ''; % Character array
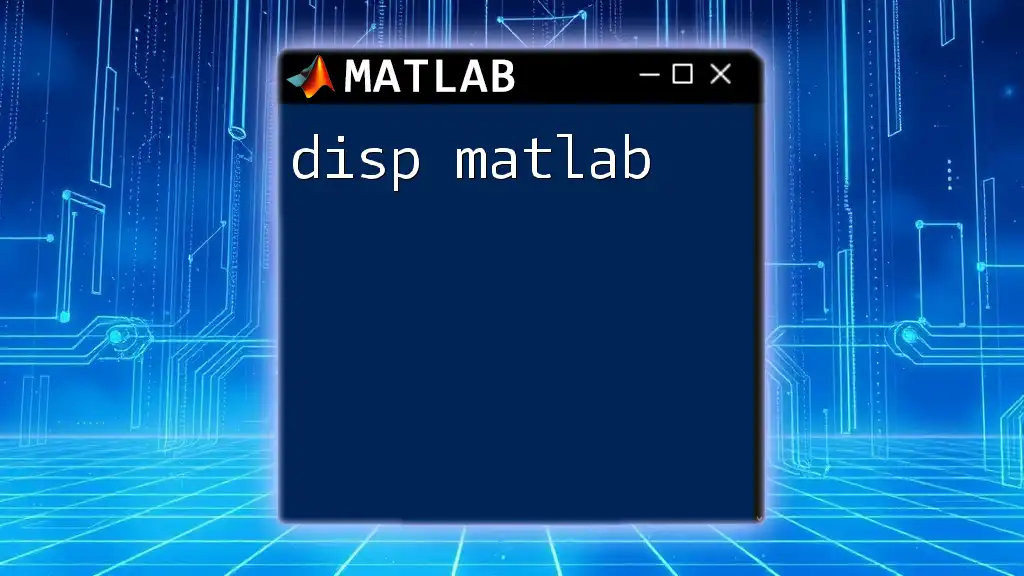
Syntax of `isempty`
Basic Syntax
The function accepts one input, `A`, and its signature is:
result = isempty(A)
This function returns a logical value: `true` if the array is empty and `false` otherwise. This direct feedback helps maintain clarity in your code logic.
Input Arguments
`isempty` can accept various input types, including numeric arrays, cell arrays, strings, and more. This versatility makes it an essential tool for developers working with different data structures.
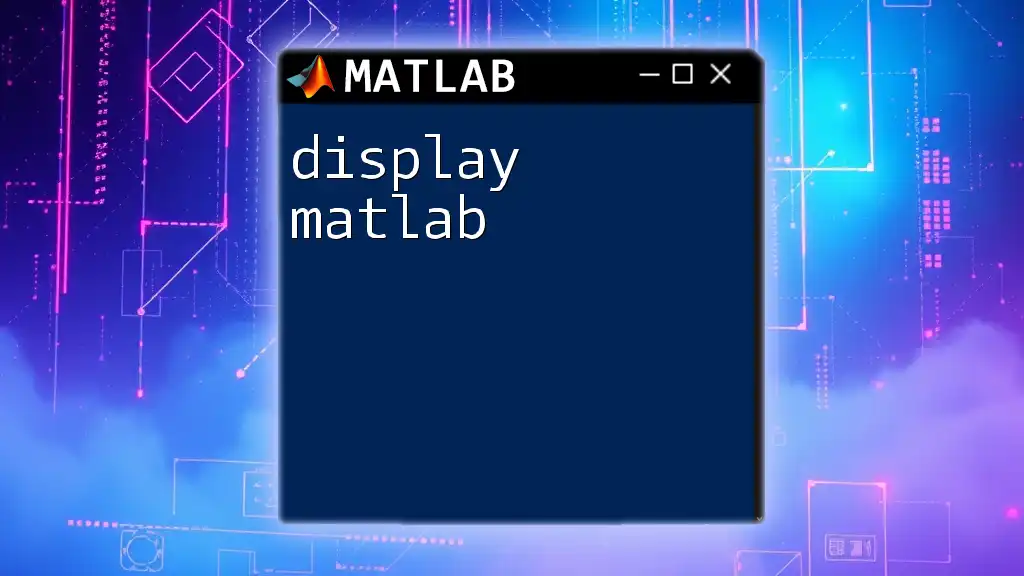
Usage of `isempty`
Simple Examples
To illustrate the function's application, consider the following examples:
Checking numeric arrays:
A = [];
result = isempty(A); % This will return true
Checking cell arrays:
B = {};
result = isempty(B); % This will return true
These straightforward checks ensure that programmers can efficiently validate their data.
Output Explanations
The output of `isempty` is crucial for controlling the flow of your program. If it returns `true`, you can implement appropriate logic to handle the empty case, ensuring your program behaves as expected.
Common Conditional Statements
Using `isempty` within conditional statements is a common practice. Here's an example:
if isempty(A)
disp('Array A is empty');
else
disp('Array A has elements');
end
This structure allows for error prevention by ensuring operations are only performed when necessary data is present.
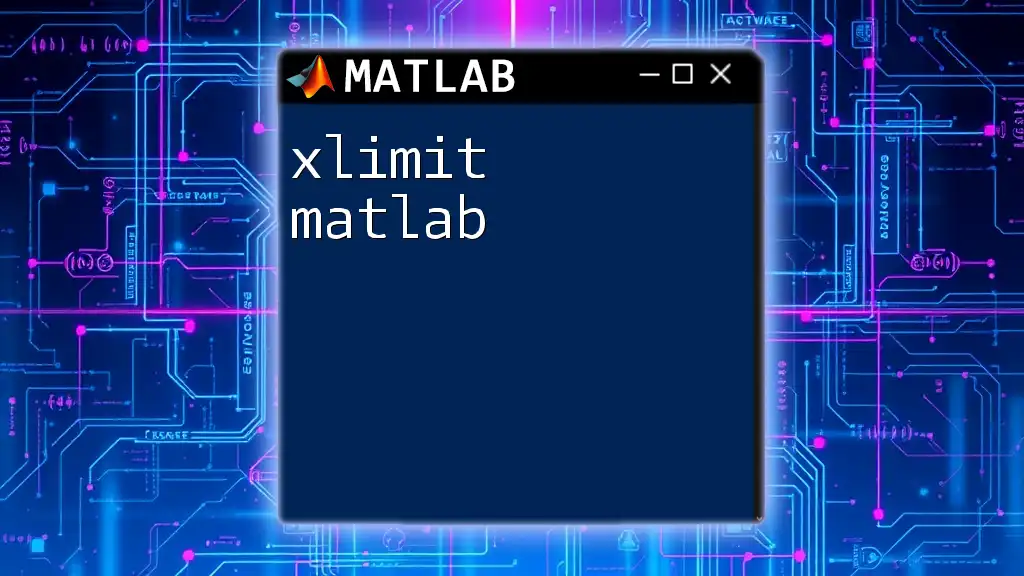
Advanced Use Cases
Nested Data Structures
When dealing with nested arrays, `isempty` becomes even more essential. Consider a scenario where a cell array contains both empty and filled arrays:
C = {[], [1, 2, 3], []};
for i = 1:length(C)
if isempty(C{i})
disp(['Element ' num2str(i) ' is empty.']);
else
disp(['Element ' num2str(i) ' is not empty.']);
end
end
In this case, `isempty` effectively checks each element within a nested structure, allowing for fine-grained data validation.
Performance Considerations
Using `isempty` is not only convenient but also efficient. It requires minimal resources to check if an array contains elements, making it a recommended practice in performance-sensitive applications.
Error Handling with `isempty`
Incorporating `isempty` in your error handling strategy is a robust programming practice. By checking for empty inputs, you can avoid exceptions or logic errors, ensuring that your functions gracefully handle any unexpected states.
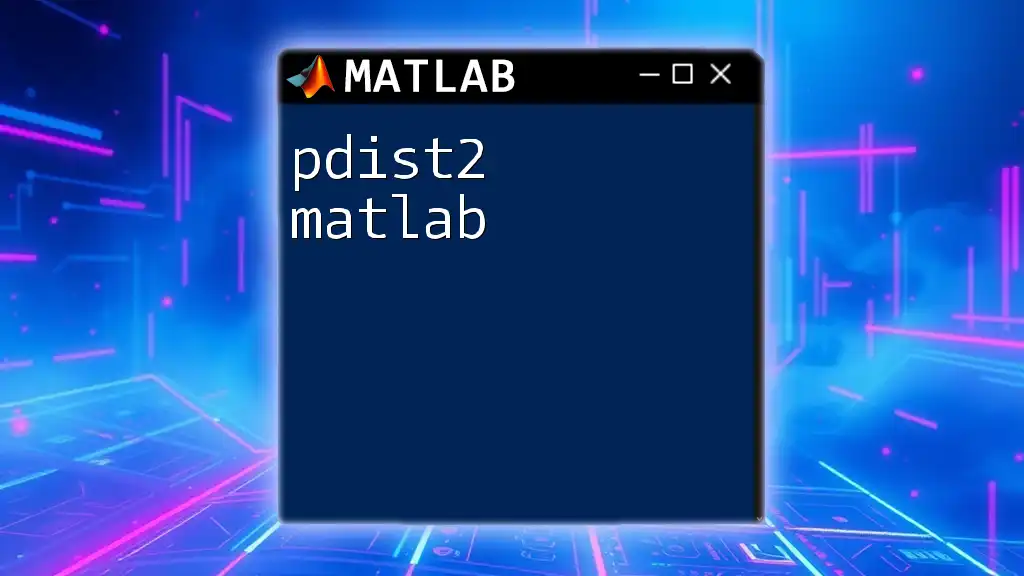
Comparing `isempty` with Similar Functions
Difference Between `isempty` and Other Functions
While `isempty` is a straightforward function for checking emptiness, other functions like `numel`, `length`, and `size` serve related but distinct purposes. Understanding these differences enhances your programming toolkit.
- `numel`: Returns the number of elements in an array. An array is empty if `numel(A) == 0`.
if numel(A) == 0
disp('A is empty');
end
Here, `numel` serves as an alternative to `isempty`, but it is less direct.
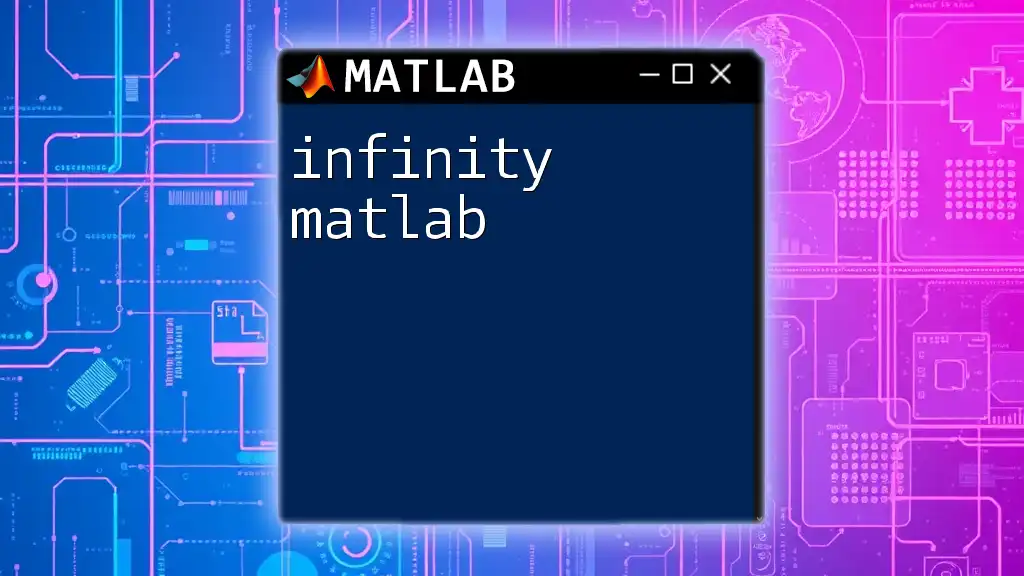
Practical Applications
Data Validation
In user interface programming or data processing pipelines, verifying that inputs are not empty is critical. Implementing `isempty` ensures that data validation occurs early in the process, allowing developers to catch issues before they propagate through the program.
Algorithm Implementation
`isempty` plays a vital role in iterative algorithms and functions where the logic depends on the presence or absence of data. For instance, algorithms that search through datasets must respect empty entries to avoid runtime errors or invalid outputs.
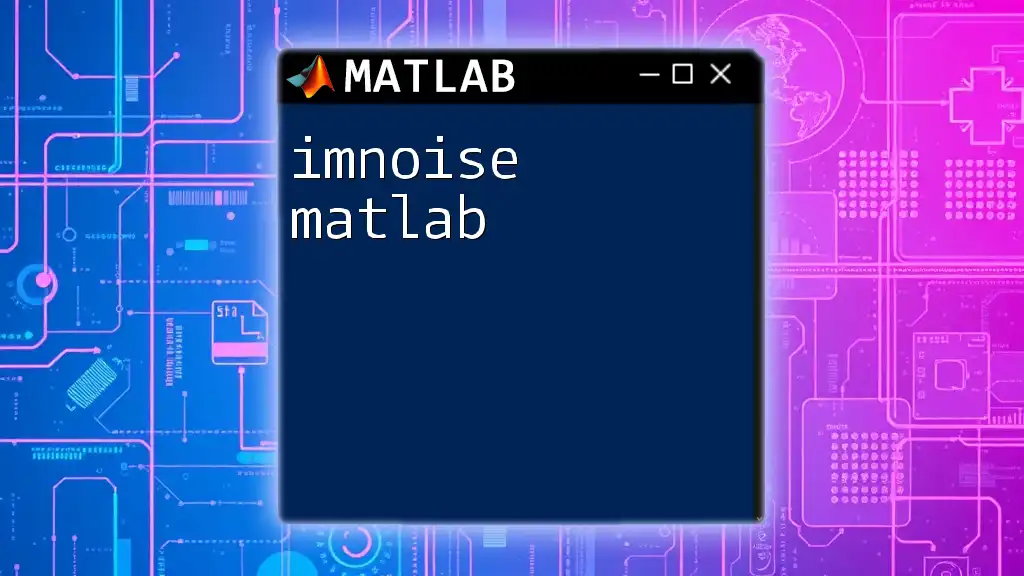
Conclusion
In summary, `isempty` is a vital function in MATLAB that checks whether an array is empty, returning a logical value that enables programmers to maintain robust control over their code. By integrating `isempty` into your programming practices, you not only enhance the reliability of your programs but also ensure cleaner, more maintainable code.
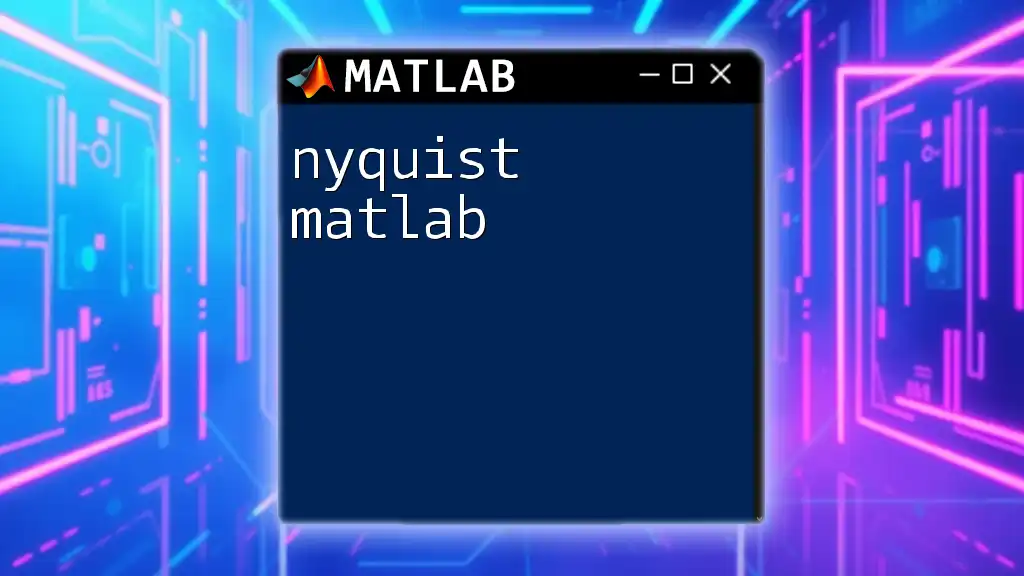
Additional Resources
For further exploration, consider consulting MATLAB's official documentation and tutorials, which provide valuable insights into using `isempty` and other built-in functions effectively.
By mastering `isempty` and its applications, you will be better equipped to handle various programming challenges in MATLAB gracefully.