The `setdiff` function in MATLAB returns the values in one array that are not present in another array, effectively performing a set difference operation.
Here’s a code snippet demonstrating its usage:
A = [1, 2, 3, 4, 5];
B = [4, 5, 6, 7];
C = setdiff(A, B);
disp(C); % Output will be: 1 2 3
What is `setdiff`?
The `setdiff` function in MATLAB is a powerful tool for performing set operations on arrays. It allows you to find the elements in one array that are not present in another, essentially helping you determine the difference between two sets. This functionality is essential not only in mathematical calculations but also in data processing tasks where unique values are crucial.
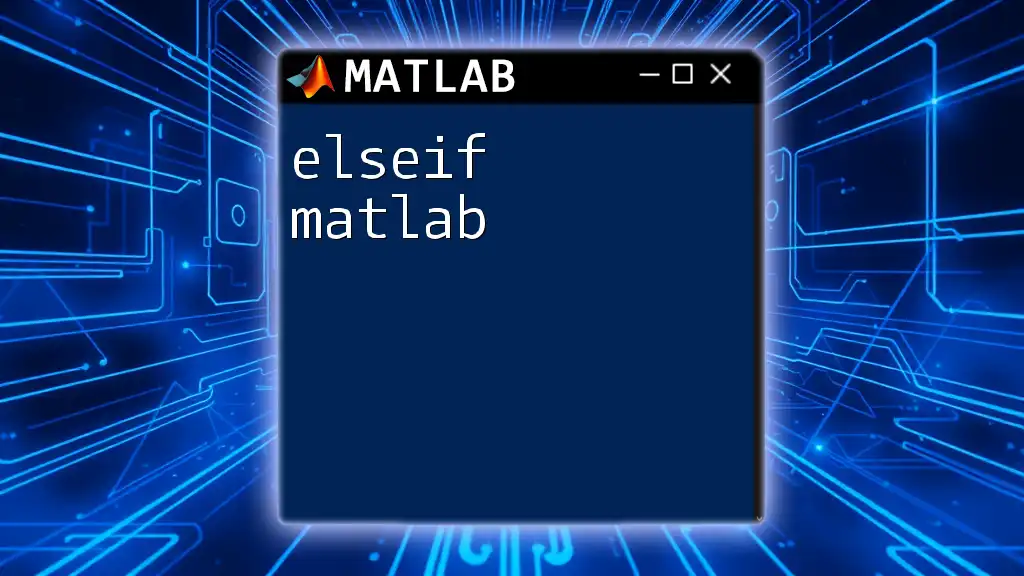
Syntax of `setdiff`
Basic Syntax
The basic syntax for using `setdiff` is simple:
C = setdiff(A, B)
Here, A is the input array from which you want to extract unique elements, and B is the array containing the elements you want to exclude. The result, stored in C, will be the elements of A that do not appear in B.
Additional Syntax Options
For cases involving matrices, you can use an optional argument to find the difference based on rows:
C = setdiff(A, B, 'rows')
This syntax allows you to treat rows of a matrix as single entities, making it useful for more complex data structures.
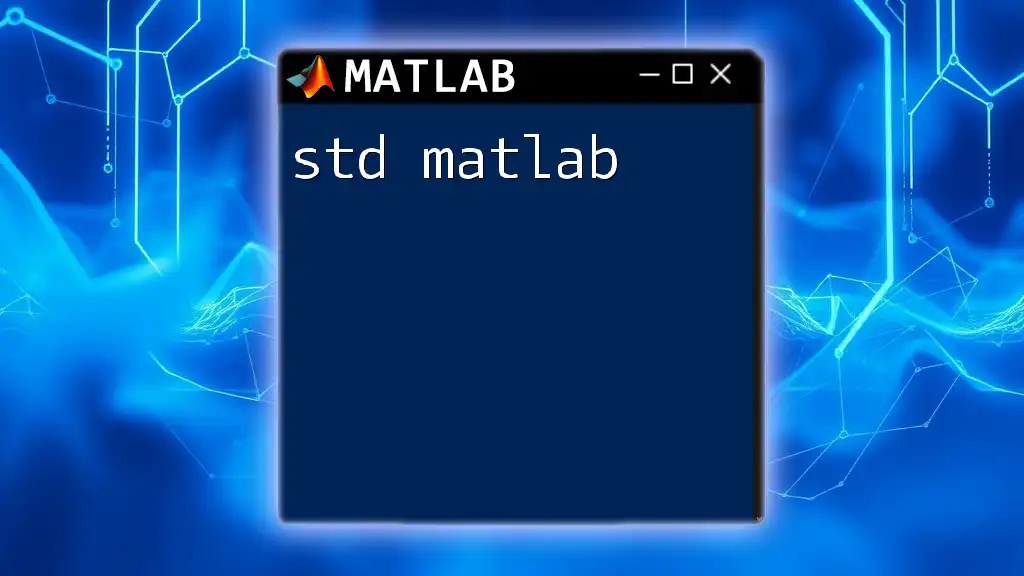
Functionality of `setdiff`
Basic Functionality
The primary purpose of `setdiff` is to compute the difference between two arrays effectively. For example:
A = [1, 2, 3, 4, 5];
B = [2, 3];
C = setdiff(A, B); % Output: [1, 4, 5]
In this case, the function identifies that 1, 4, and 5 from array A are not present in array B, returning them in the result C.
Working with Unique Values
In many scenarios, you may be working with lists that contain duplicate values. `setdiff` inherently deals with unique elements, which is particularly beneficial for data integrity. Consider the following example:
A = [1, 2, 3, 4, 5, 5];
B = [2, 3];
C = setdiff(A, B); % Output: [1, 4, 5]
Even though 5 appears twice in A, `setdiff` will return it only once in the output, maintaining the uniqueness of elements.
Multidimensional Arrays
When working with multidimensional arrays, `setdiff` can still be effectively utilized. By specifying the 'rows' option, you can perform operations on matrices. For instance:
A = [1, 2; 3, 4];
B = [3, 4];
C = setdiff(A, B, 'rows'); % Output: [1, 2]
This example demonstrates how the function can compare rows between the two matrices and return the rows that are not present in the second matrix.
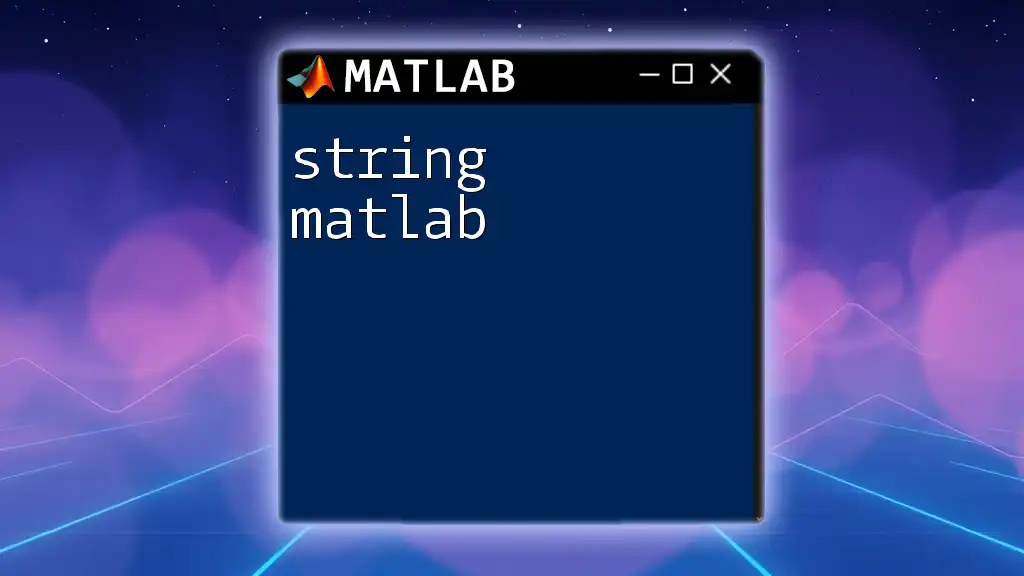
Practical Applications of `setdiff`
Data Cleaning
One of the most common applications of `setdiff` is in data cleaning procedures. When analyzing datasets, it may often be necessary to identify or remove duplicates or unwanted entries. By leveraging `setdiff`, you can streamline this process, ensuring that your dataset contains only the desired unique entries.
Analyzing Survey Data
In survey analysis, you may want to find which participants did not respond to specific questions. Using `setdiff`, you can easily identify these individuals and examine the effects of non-response on your results.
Set Operations in Data Science
The `setdiff` function is a critical part of set theory in data science that includes operations like union and intersection. Understanding these operations can significantly improve your ability to manipulate and analyze datasets efficiently.
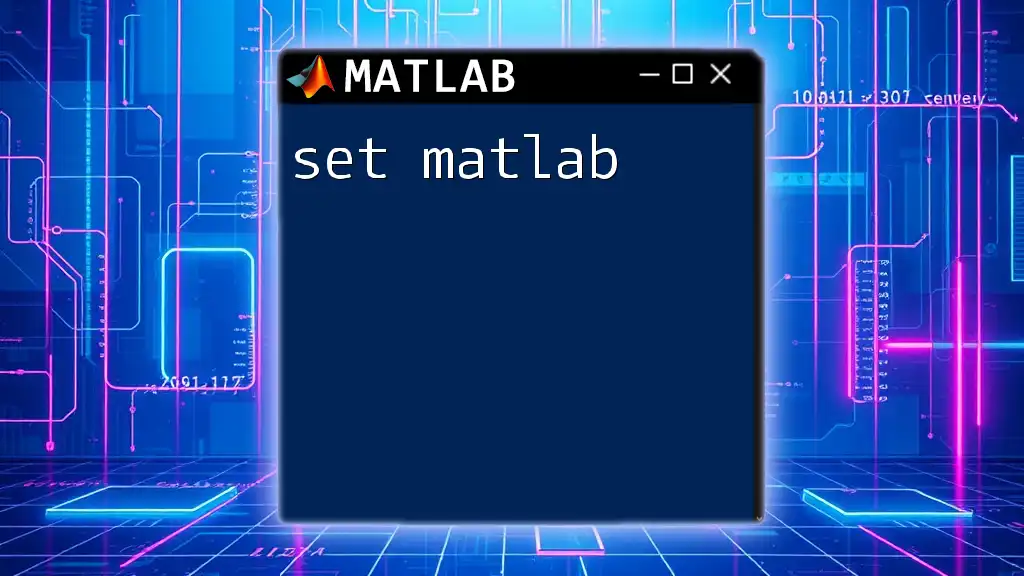
Performance Considerations
Efficiency of `setdiff`
Performance can be a concern when handling large datasets. `setdiff` is quite efficient for reasonably sized arrays, but for exceptionally large datasets, the performance may vary. It is essential to consider the size of your data and test the function’s performance in your specific context.
For better results:
- Consider using logical indexing for operations if you need to perform multiple set operations.
- Ensure your datasets are as efficient as possible with regard to data types and sizes.
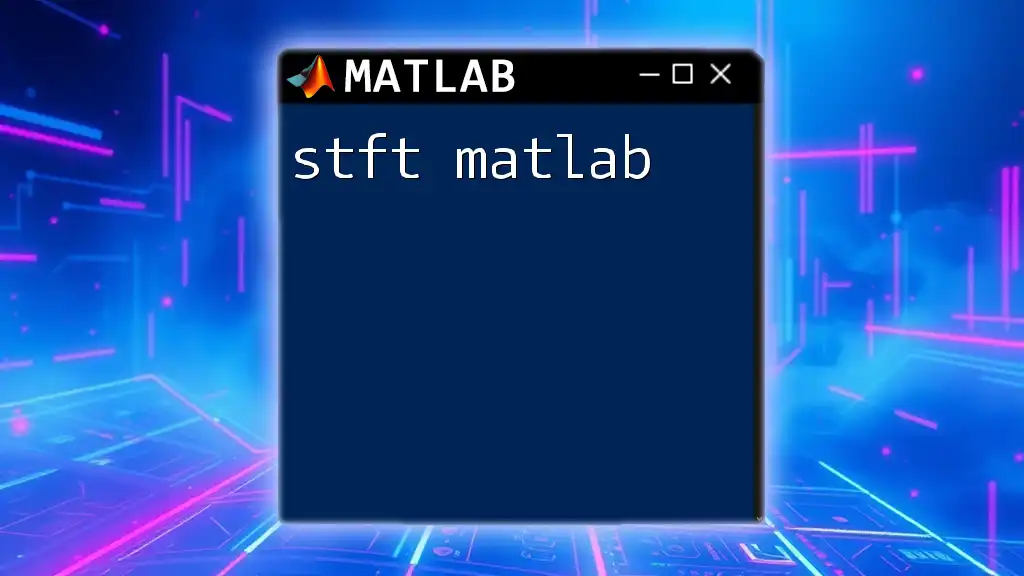
Common Mistakes and Misunderstandings
Misinterpretation of Output
A frequent issue users face is misunderstanding the output of the `setdiff` function. For instance:
A = [1, 2, 3];
B = [1, 2, 3, 4];
C = setdiff(A, B); % Output: []
In this case, one might expect some values to remain in C. It’s essential to realize that `setdiff` returns elements in A minus those in B. Since all elements of A exist in B, the result is an empty array.
Dealing with NaNs and Special Cases
When utilizing `setdiff`, keep in mind how it treats NaN values. It is important to note that NaN is considered an unequal quantity in MATLAB. Therefore, if you have:
A = [1, NaN, 3];
B = [NaN, 2];
C = setdiff(A, B); % Output: [1, NaN, 3]
The output includes NaN since it is treated as a unique entry, reinforcing the need for careful consideration when handling special cases in your data.
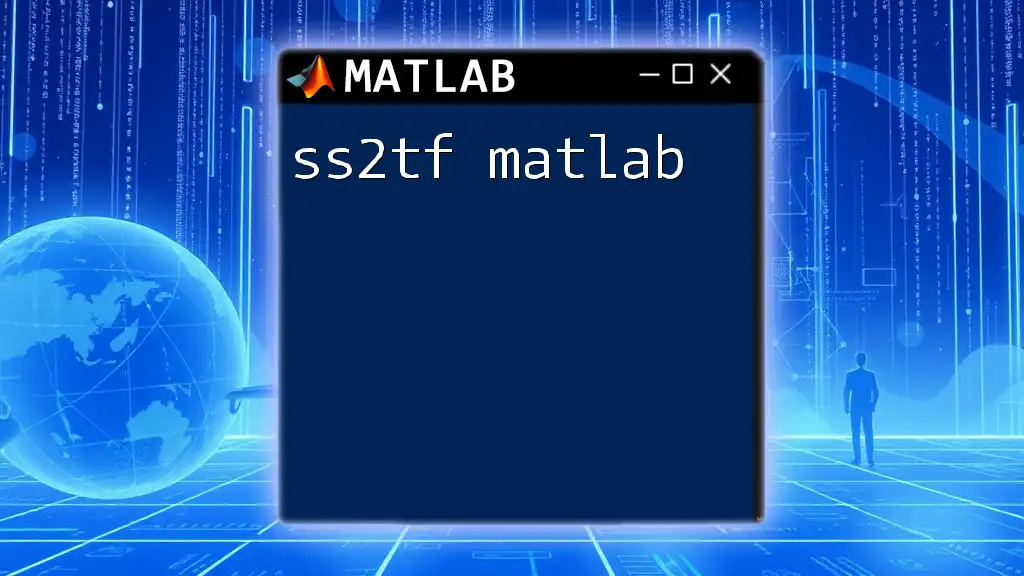
Troubleshooting `setdiff`
Error Handling in MATLAB
Users might encounter various errors when using `setdiff`, mainly due to improper input types. For example:
A = [1, 2, 3];
B = 'not a number'; % Incorrect input
C = setdiff(A, B); % Will generate an error
In scenarios like this, ensure that both inputs are arrays of the same data type to avoid type mismatch errors.
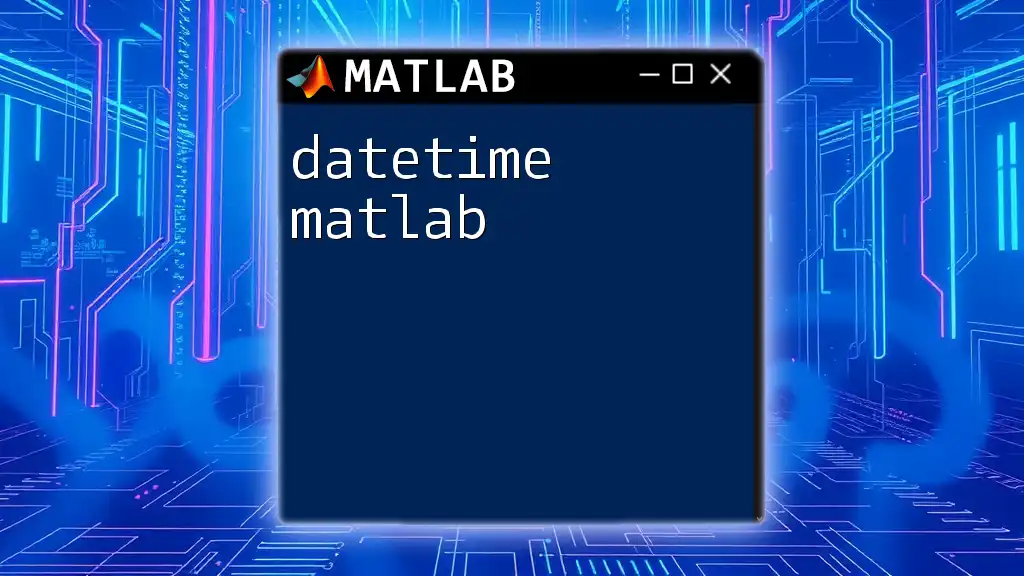
Conclusion
In summary, `setdiff` is an invaluable function in MATLAB for anyone looking to manipulate and analyze data effectively. Through understanding its syntax and functionality, you can unlock a myriad of applications in data cleaning, analysis, and operations related to sets. By practicing with the examples provided, you will enhance your proficiency in using this function and improve your overall MATLAB skills.
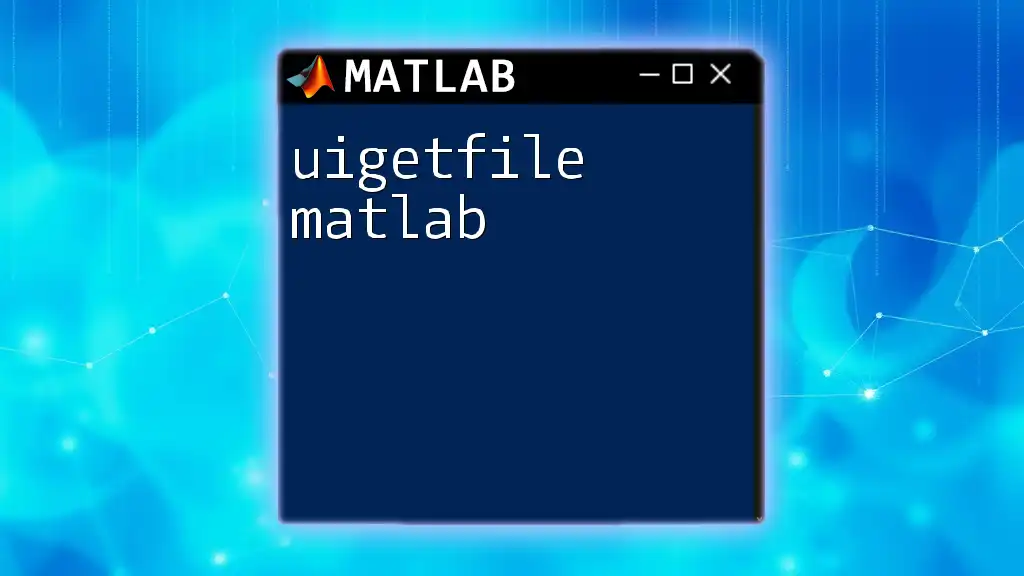
Additional Resources
For an in-depth grasp of `setdiff` and its applications, refer to the official MATLAB documentation and user forums where you can learn from other users' experiences. As you become more familiar with `setdiff`, explore related functions that may further enhance your data analysis capabilities.
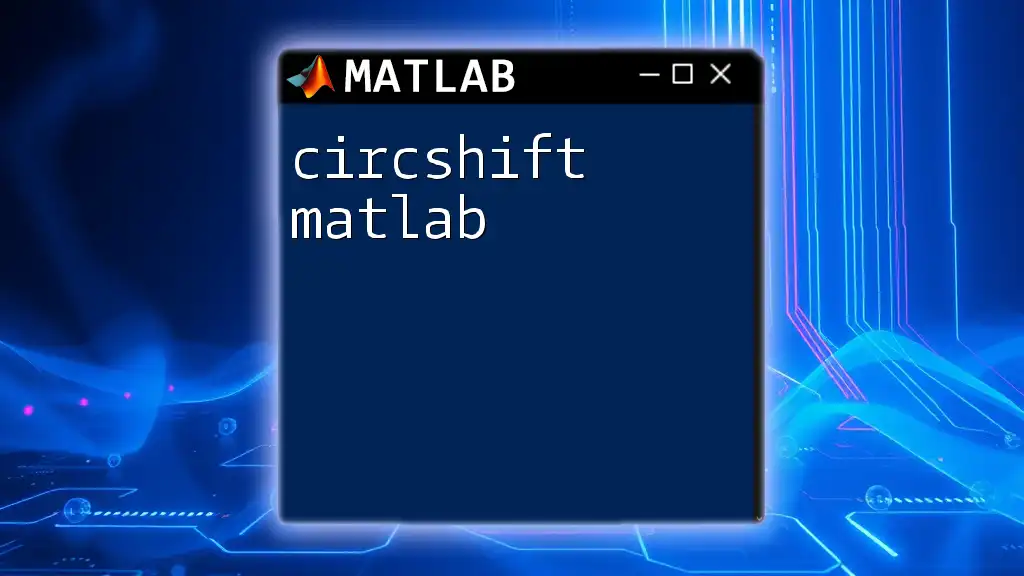
Call to Action
Now that you’ve gained insights into using `setdiff`, I encourage you to apply what you’ve learned through various exercises. Feel free to share your experiences and insights on your journey to mastering this essential MATLAB function!